Creating Entities in Programming: A Comprehensive Guide
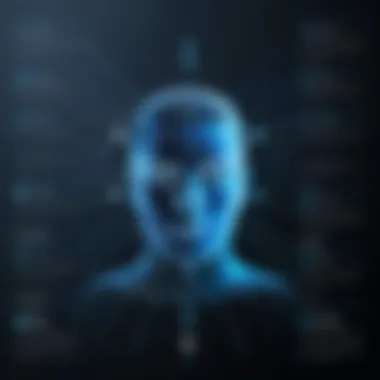
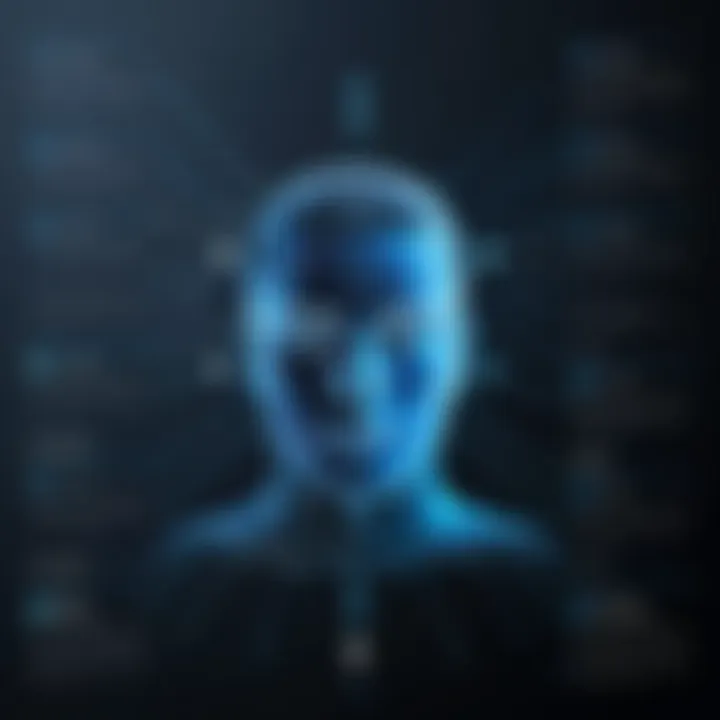
Intro
Creating entities within programming represents a fundamental aspect of software development. By definition, an entity can be understood as an object that holds a unique identity and represents a distinct data structure in code. This concept transcends individual programming languages, showcasing its significance across various paradigms such as object-oriented programming, functional programming, and more.
Despite variations in implementation, the core idea remains similar: entities serve as building blocks that encapsulate both data and behavior. Identifying and correctly managing these entities is crucial to developing robust applications. As such, this guide will dissect the essentials of creating and managing entities, offering practical insights that cater to both students and those looking to deepen their programming knowledge.
By taking a closer look at the theory, practical examples, and common pitfalls of entity creation, learners can achieve a deeper understanding of how to effectively construct entities in their own programming endeavors.
Prelude to Entities
Entities play a crucial role in the realm of programming. They represent objects or concepts that developers model in their code. Understanding entities is foundational for structuring software effectively. Each entity can encapsulate properties and behaviors, making it easier to manage complexity within applications. Without a clear grasp of what entities are, programmers may struggle to create robust software solutions.
Understanding Entities
At its core, an entity can be defined as a thing with a distinct existence. In programming, entities can represent real-world objects like a user, a product, or even abstract concepts such as a transaction. Entities usually comprise attributes, which describe their state, and methods, which define their behavior. For example, a entity might have attributes like , , and . It could also have methods like or .
This definition extends beyond just naming a variable or class. It encompasses a broader understanding of how these abstractions interact with each other in an application. Essentially, entities are the building blocks of an application’s architecture.
Importance of Entities in Programming
Understanding entities offers several benefits:
- Modularity: Entities allow for better organization of code. Each entity can function independently yet collaborate with others, leading to cleaner and more manageable codebases.
- Reusability: Once an entity is defined, it can often be reused in multiple parts of an application. This reduces redundancy and fosters efficient development practices.
- Easier Maintenance: Clear separation of concerns makes the maintenance of code easier. When each entity has a defined role, modifications can be made with minimal impact on other parts of the system.
Defining Entities
In any programming paradigm, defining entities forms the cornerstone of effective coding. It lays the groundwork for how data is organized and managed within software. Understanding entities helps programmers create more structured, maintainable, and scalable applications. This part of the article will explore what counts as an entity and how to articulate their attributes and behaviors. These insights will assist both budding programmers and seasoned developers in crafting better code.
What Constitutes an Entity?
An entity is a distinct thing or object in the context of programming. It can be real-world objects, concepts, or even abstract data points. For instance, in a banking application, entities could be a customer, an account, or a transaction. Recognizing what constitutes an entity is vital for accurate data modeling.
There are several attributes that can define an entity:
- Identity: Each entity must have a unique identifier, such as an ID number or name. This ensures that entities can be distinguished from one another.
- Properties: These are characteristics or attributes that describe the entity, like a customer's name or account balance.
- Relationships: Entities might have relationships with other entities. For example, a customer might own multiple accounts.
Being clear about what an entity is and its role within the system contributes to easier management and manipulation of data.
Attributes and Behaviors of Entities
Attributes and behaviors are crucial components when defining entities. Attributes refer to the properties that describe an entity. Behaviors, on the other hand, refer to the functions or methods associated with an entity.
Attributes
Attributes can vary based on the type of entity. For a entity, typical attributes might include:
- Name: The name of the customer.
- Email: An online contact point.
- Account Number: A unique identifier for the customer's account.
Choosing relevant attributes not only makes the entity more meaningful but also aids in data validation and manipulation later on.
Behaviors
Behaviors are actions that an entity can perform or that can be performed on the entity. For instance, a entity could have behaviors such as:
- Open Account: A method for creating a new account for the customer.
- Withdraw Funds: A method to deduct funds from the customer's account.
Defining both the attributes and behaviors helps encapsulate data effectively, promoting reusability and clarity in your code.
In summary, defining entities with well-thought-out attributes and behaviors is crucial. It facilitates better software design, contributing to more organized and efficient applications.
Creating Entities in Object-Oriented Programming
Creating entities within Object-Oriented Programming (OOP) is central to modern software development. OOP creates a framework in which entities can be modeled using classes and objects. This section explores how entities are structured in OOP and why they are vital in programming. By understanding entities, programmers can design software that is modular, reusable, and easier to maintain.
In OOP, entities are conceptualized as classes that serve as blueprints for creating individual object instances. Each object can have its own state and behavior, making it possible to represent real-world entities in code. This encapsulation enhances code clarity and emphasizes the relationships between different entities.
Classes as Blueprints for Entities
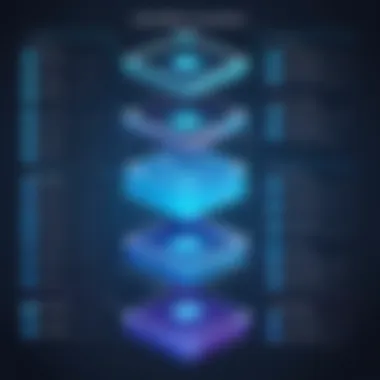
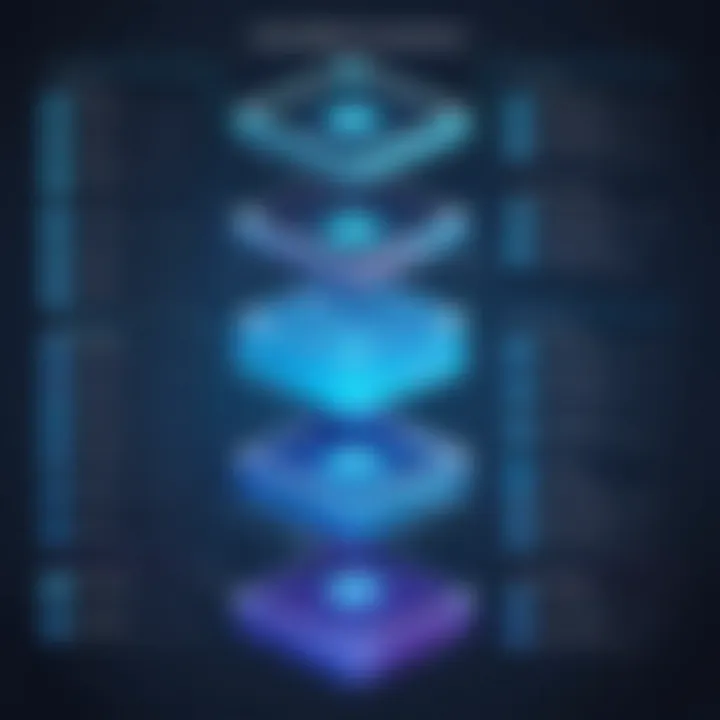
Classes are the foundations of OOP, acting as templates from which objects are instantiated. A class defines attributes and methods that an entity possesses. For example, consider a class called . The attributes might include , , and , while methods could include and .
The use of classes allows for encapsulation, where internal object data is hidden from the outside world. This means that users of the object interact with it through defined interfaces rather than manipulating the data directly. This approach fosters a level of protection for object integrity and allows for well-defined interactions.
Instantiating Entities in Java
In Java, instantiating an entity involves creating an object based on its class. You utilize the keyword to create a fresh instance of the class. For instance:
This line creates a new object named . Once created, you can assign values to the attributes and invoke methods associated with the object:
In Java, constructors can also be defined within the class to initialize objects with specific values. This method enhances flexibility and ensures that entities maintain valid states when instantiated.
Instantiating Entities in ++
C++ follows a similar approach for instantiating entities. In C++, you can declare an object of a class as follows:
Here, is an instance of the class. To initialize attributes, you can use a constructor or directly access them:
C++ gives the programmer more control over memory management, so it’s important to handle object lifetimes correctly. Knowing when to allocate and deallocate memory can prevent memory leaks and undefined behaviors.
In summary, creating entities in OOP facilitates the development of organized and efficient software systems. By leveraging classes, attributes, and methods, programmers can construct applications that mirror real-world systems, ultimately leading to better modularity and code maintainability. The choice of programming language may influence the specifics of instantiation, but the underlying principles remain consistent across languages.
Data Structures to Represent Entities
In programming, data structures serve as a critical foundation for representing entities. An entity can be thought of as a distinct object or concept within a system, encompassing its properties and behaviors. Understanding how to choose an appropriate data structure to represent these entities can greatly affect the efficiency, scalability, and maintainability of your code.
Selecting the right data structure is not just about functionality; it's also about how well the chosen structure integrates with the larger architecture of the software. The representation of entities must allow for easy access, modification, and management of their attributes.
Choosing the Right Data Structure
When it comes to choosing a data structure, several factors need to be considered:
- Type of Entity: Different entities like users, products, or transactions may require different representations. For instance, a user might be represented using a class or an object with attributes like name and email, while a product could have properties like title and price.
- Operations Required: Consider what operations will be performed on these entities. Will there be frequent updates to their states? If yes, a structure that allows for quick modification is ideal, such as a linked list or hash map.
- Memory Efficiency: Data structures vary in terms of space efficiency. For instance, an array could be less memory-intensive than a linked list when the number of entities is predictable, but linked lists may offer better performance if entities are added or removed frequently.
- Ease of Use: Sometimes, a simple structure might be more effective than a complex one. If your programming environment supports built-in data types which fulfills your purpose, it might be wiser to stick with those.
In summary, the right data structure aligns with the specific context of the entities involved, balancing performance, ease of use, and memory management effectively.
Examples of Entity Representation
Real-life entity representation can take many forms. Here are some common examples using different data structures:
- Classes and Objects (in Object-Oriented Programming): A basic way to represent an entity in languages like Java and C++ is through a class. For example:This snippet defines a entity with attributes.
- Dictionaries or Hash Maps (in Python): A user can also be represented in Python using a dictionary, which provides key-value pairs for easy data access.
- Structs (in C): When working in C, a struct can be an efficient way to combine related variables under a single name. For example:This defines a User struct that holds name and email attributes.
These representations highlight how various languages provide different mechanisms and structures for defining entities. Adapting your choice based on context, operation, and efficiency will set you on the right path for robust programming.
Working with Entities in Functional Programming
Understanding how to work with entities in functional programming provides a different perspective from object-oriented paradigms. In functional programming, entities are treated as immutable data structures. This immutability is crucial as it facilitates predictability and reduces side effects. Programs become easier to reason about because the same input will always yield the same output.
Entities as First-Class Citizens
In functional programming, entities are considered first-class citizens. This term means that entities can be passed as arguments to functions, returned from other functions, and assigned to variables. By treating entities this way, you empower functions to manipulate and operate on data with increased flexibility.
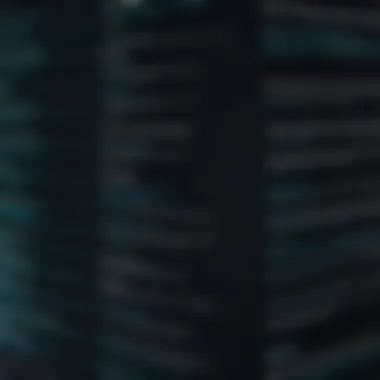
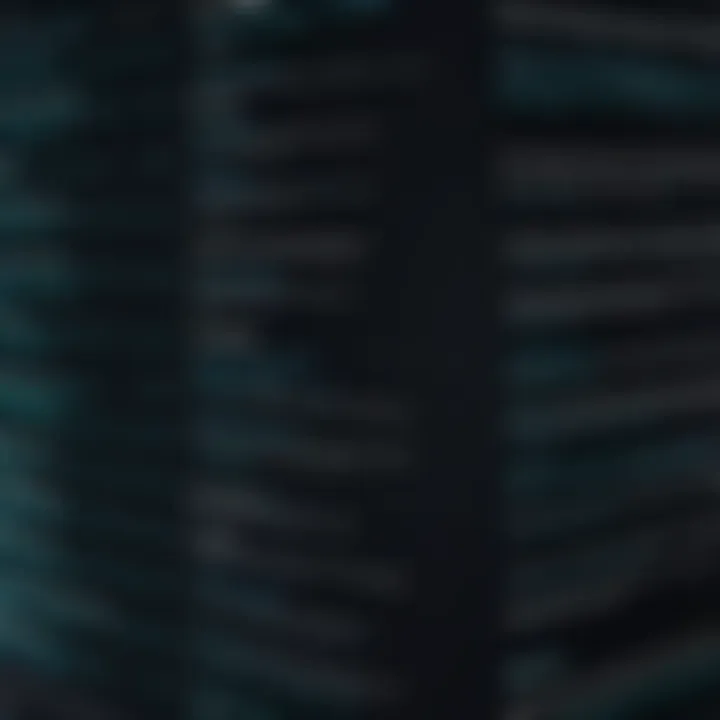
For instance, in a functional language such as Haskell, you can create data structures to represent complex entities:
In this example, the entity is defined as a type with specific attributes, which can then be used in various functions. This pattern showcases how entities seamlessly integrate with functions.
Additionally, functions that operate on entities can have higher-order capabilities. They can accept other functions as arguments, allowing for advanced behaviors like mapping, filtering, or reducing sets of entities.
Creating Entities in JavaScript
JavaScript, while primarily object-oriented, also supports functional programming principles. You can create entities using JavaScript objects and assign them to variables. However, when working functionally, keeping entities immutable is a good practice. This can be achieved using libraries like Immutable.js or by utilizing the spread operator to construct new objects based on existing ones.
Example of creating an entity in JavaScript:
In this snippet, an object representing a person is created. The function takes the entity, and rather than modifying it, it returns a new object with an updated age. This pattern ensures that the original entity remains unchanged, reflecting the principles of both functional programming and immutability.
The approach of managing entities as first-class citizens and creating them in an immutable fashion in JavaScript not only enhances readability and maintainability but also aligns with mathematical functions' predictability.
"The beauty of functional programming lies in treating functions as the primary building blocks of your applications, with data flowing through them rather than being hidden in objects."
By understanding and implementing entities as first-class citizens and practicing immutability, programmers can leverage functional programming’s strengths effectively.
Managing Entity States
Managing the states of entities is crucial in software development. The state represents the current status or condition of an entity at any given moment. Its proper management ensures that an application's entities behave predictably and efficiently. This section emphasizes the importance of understanding entity state management and its implications for overall software reliability and maintainability.
A well-structured state management system provides numerous benefits:
- Predictability: Clearly defined states lead to predictable behaviors. Developers and users can anticipate how entities will react under various conditions.
- Debugging: When issues arise, understanding the state flow helps in isolating problems quickly. By tracking state changes, it is easier to pinpoint errors.
- Performance: Efficient state transitions minimize resource usage. This can lead to better performance, specifically in applications with multiple entities.
Understanding State Management
State management involves tracking and controlling the state of various entities throughout their lifecycle. This can include heavy interactions in systems like games or simplified flow in data-driven applications. To manage state effectively, developers must consider the following elements:
- Initialization: Each entity begins with a set initial state. Understanding how to decide this initial state is key.
- Transitions: States change based on actions or external inputs. Defining clear transition rules is vital.
- Persistence: Depending on the application, you might need to save entity states. This is common in save-game features or user sessions.
- Event Handling: Events trigger state changes. Just like in real life, a button press or a timer can change an entity's state. Developers need to implement event listeners and handlers accordingly.
"Effective state management is not just about controlling entity status; it’s about ensuring that the flow of logic is coherent and intuitive."
Common State Management Patterns
Practicing structured patterns can significantly ease the complexity of entity state management. Some common patterns include:
- Finite State Machines (FSM): An FSM outlines a limited number of states and strict rules for transitions. This pattern is useful in applications that need predictable outcomes, like AI behavior in games. Implementation can be seen in JavaScript with the following code snippet:
- Observer Pattern: Here, entities subscribe to changes. This is particularly powerful in reactive programming, allowing an entity to react to changes in another entity’s state, promoting a decoupled architecture.
- Command Pattern: This involves encapsulating actions as objects. Each command can trigger state changes in an entity while maintaining clear separation of logic.
By adopting these programs, developers can create more responsive, manageable, and predictable software systems.
Entity Relationships and Interaction
Understanding how entities connect and interact is crucial in programming. These relationships shape how data flows through a system and how it can be manipulated. Effectively managing entity relationships allows for a more organized codebase and enables clearer communication between components.
Defining these relationships is not only about recognizing which entities exist, but also how they relate. Dependencies, ownership, and interaction types greatly influence functionality. When relationships are well-defined, programmers can predict outcomes and behavior more accurately. This reduces bugs and aids in future modifications.
Defining Relationships Between Entities
In programming, entities may have various types of relationships. Here are the common categories:
- One-to-One: One entity directly correlates with another entity. For instance, a user might have one profile.
- One-to-Many: A single entity can be related to multiple entities. For example, a blog may have many comments related to one post.
- Many-to-Many: Two entities can relate to many entities at once. A typical example is students enrolling in multiple courses.
By clearly defining these relationships, developers can better structure their data models. This leads to improved efficiency and maintainability. Likewise, considering the lifespan of interactions is also critical. Entities should be defined in a manner that reflects their interactions in real-world scenarios.
Interaction Patterns Among Entities
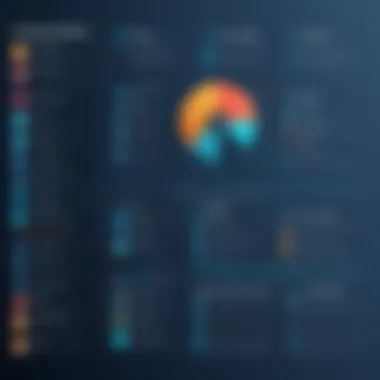
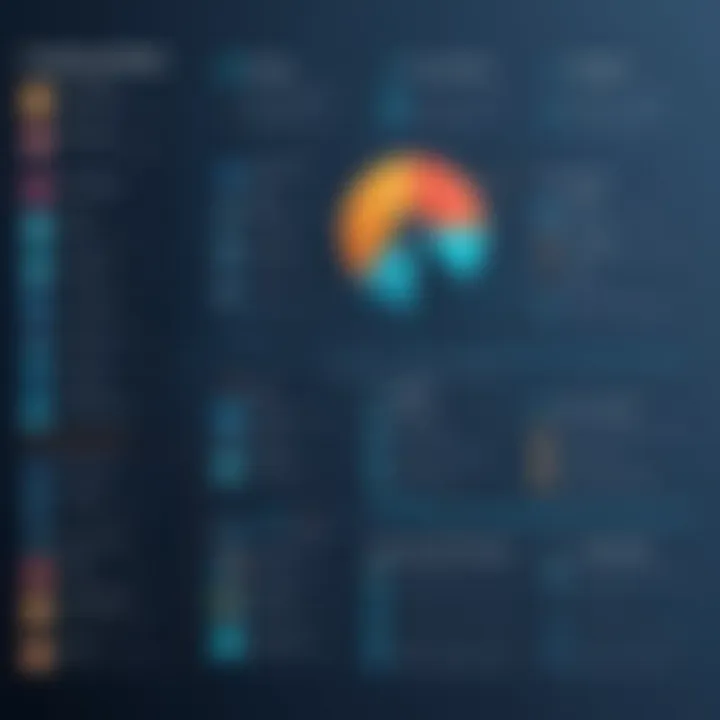
Interaction patterns among entities dictate how they communicate and operate together. Various patterns exist to facilitate entity interactions:
- Direct Interaction: This occurs when one entity directly manipulates or requests information from another. For instance, if an order requests information from a customer record.
- Event-Driven Interaction: Here, one entity generates an event that another entity listens for. This model promotes decoupling, as entities don't need to know about each other directly.
- Message Passing: This pattern involves the sender and receiver communicating through messages. For example, a notification service handling alerts for numerous users.
Error Handling in Entity Creation
In programming, error handling is critical, especially when it comes to creating entities. Entities are foundational components in software development, serving as the building blocks for systems. As such, any mistake in their creation can lead to significant issues in functionality and performance. Proper error handling not only improves the reliability of code but also enhances maintainability and debugging efforts. Moreover, identifying and addressing errors during the entity creation phase can reduce the risk of cascading failures in other parts of the application. By prioritizing error handling, programmers ensure that issues can be traced and rectified easily, fostering a more robust programming environment.
Common Errors in Entity Creation
Several common errors can occur during the creation of entities:
- d Attributes: These errors arise when defining attributes. A small typo can lead to problems in objects where those attributes are used.
- Missing Required Fields: If an entity is meant to have certain required fields and they are omitted during creation, this can lead to unexpected behavior later on.
- Type Mismatch: Incorrect data types assigned to attributes can cause runtime errors. For instance, trying to assign a string instead of an integer.
- Circular References: In creating entity relationships, it is possible to inadvertently create a circular reference, leading to memory leaks and system crashes.
These errors may seem minor but can have amplifying effects on system reliability. Therefore, understanding these pitfalls is a prerequisite for successful entity creation.
Strategies for Error Prevention
Preventing errors in entity creation requires proactive measures. Here are some strategies to consider:
- Input Validation: Always check inputs before processing them further. This can prevent invalid data from being assigned to entity attributes.
- Define Clear Schema: Create a clear schema for the entities that outline required fields, data types, and relationships. Documentation helps avoid confusion.
- Error Handling Mechanisms: Implement error handling mechanisms, such as try-catch blocks in Java or exception handling in C++. These mechanisms can catch errors when they occur and allow graceful degradation.
- Unit Testing: Incorporate unit tests that specifically focus on entity creation. This ensures that all expected attributes and relationships behave as intended under various scenarios.
- Use of Linters: Linters are tools that analyze code for potential errors or stylistic issues. Using a linter can help catch common mistakes before the code is run.
By applying these strategies, developers can minimize the likelihood of errors, leading to smoother entity creation and an overall better coding experience.
Best Practices for Entity Creation
Creating entities in programming requires attention to detail and thoughtful practices to ensure clarity and maintainability. The significance of adopting best practices cannot be overstated. These practices facilitate easier collaboration, reduce bugs, and enhance overall code quality. By employing established conventions and methodologies, developers increase the readability of their code, making it more accessible for both themselves and others who may work on it in the future.
Consistent Naming Conventions
One of the fundamental aspects of entity creation is establishing consistent naming conventions. Naming is not merely a formality; it plays a crucial role in understanding and navigating codebases. Consistent and descriptive names for entities, attributes, and methods improve clarity. For instance, using for a class and for a corresponding attribute provides immediate context rather than cryptic or vague identifiers.
There are various strategies to enforce naming consistency:
- Camel Case: Common in languages like Java, where the first letter of each word except the first letter is capitalized. For example, .
- Snake Case: Often used in Python, where words are separated by underscores, such as .
- Pascal Case: Similar to Camel Case but applies to types and namespaces, e.g., .
All developers in a team should agree on a naming format to maintain consistency throughout the project. This agreement reduces misunderstandings and makes it easier to locate and modify components when necessary.
Use of Comments and Documentation
Commenting code and maintaining documentation are vital practices in entity creation. Well-placed comments clarify the purpose of complex logic, outline the usage of a particular function, or explain why certain decisions were made during development. Comments bridge the gap between developers and their code, especially for those who are new to the project or returning after a time away.
Moreover, thorough documentation accompanies the code with explanations about the structure and functionality of entities. This may include:
- Overview of Entities: Describe the purpose and functions of entities within the software system.
- Usage Instructions: Provide explicit guidance on how to create, manipulate, and destroy entities.
- Change Logs: Keep a record of modifications made to entities or their relationships over time.
The ideal approach is to ensure that comments evolve with the code. They should be updated or removed if they cease to provide value. This attention to detail in documentation keeps the codebase engaged and maintains relevance.
"Good comments are better than bad code."
— This underscores the crucial role comments play in enhancing the clarity and maintainability of code.
By adopting these best practices, programmers can create entities that are not only functional but also maintainable and understandable. This approach fosters a culture of collaboration, ultimately leading to more robust and efficient software development.
Epilogue
The conclusion of this article plays a crucial role in synthesizing the information presented throughout the sections. It serves as the final opportunity to reinforce the importance of creating and managing entities in programming. By bringing together the key concepts discussed, readers are reminded of the fundamental principles that underpin the theory and practical application of entities.
In the realm of software development, a solid understanding of entities enhances the programmer’s ability to design robust and efficient applications. This knowledge aids in the creation of scalable software architectures where entities can interact seamlessly. Furthermore, acknowledging the best practices in naming conventions and documentation helps maintain clarity and consistency in code.
Recap of Key Concepts
This section succinctly revisits the main points explored in previous sections. Entities, as discussed, are the building blocks of software systems. They consist of attributes and behaviors that define their characteristics and functions. Key topics covered include:
- The definition and importance of entities in programming
- How to create and instantiate entities using object-oriented programming languages like Java and C++
- The representation of entities through various data structures
- Managing state and interactions between entities
- Strategies for handling errors during entity creation
- Best practices in writing and documenting entity-related code
By revisiting these concepts, the reader can consolidate their understanding and identify any areas requiring deeper exploration.
Future Directions in Entity Management
The landscape of programming is continually evolving, and so are the methodologies and technologies associated with entity management. As we look towards the future, several key trends emerge:
- Integration of Artificial Intelligence: The implementation of AI can automate entity creation processes, allowing for more efficient resource allocation in application development.
- Increased Focus on Microservices: With the rise of cloud computing and microservices architecture, the concept of entities may evolve, creating smaller, more focused entities that communicate over well-defined interfaces.
- Enhanced Data Models: As data structures grow more complex, adapting entity management to accommodate changing data requirements will become a necessity.
- Working with NoSQL Databases: As traditional relational databases face challenges with scalability, future practices will likely embrace NoSQL databases, reshaping how entities are created and interacted with.
In summary, as the programming landscape changes, entity management will also adapt. Keeping abreast of these developments ensures that programmers maintain relevant skills and knowledge for effective software development.