Creating Applications with Java: A Comprehensive Guide
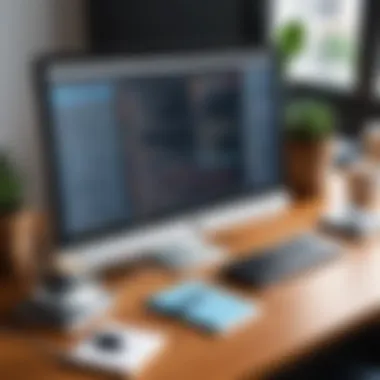
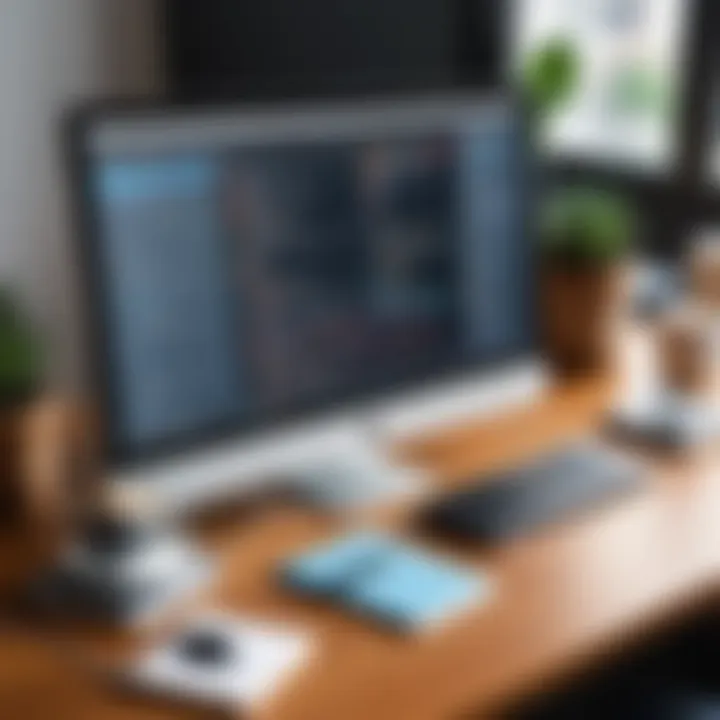
Prelude to Programming Language
Java is a widely used programming language, noted for its portability, efficiency, and security features. Understanding the foundation of Java can enable new developers to create a broad range of applications, from mobile apps to web servers.
History and Background
Developed by Sun Microsystems in the mid-1990s, Java emerged to solve the issue of platform dependency in software development. The language took inspiration from C and C++, but it added additional features that encouraged strong object-oriented programming principles. In 1995, Java became accessible to developers, and since then, it has undergone several updates, enhancing its capabilities and maintaining relevance in the constantly evolving tech landscape.
Features and Uses
Java comes with several notable features making it a preferred choice in various domains:
- Platform independence: Write once, run anywhere (WORA) philosophy enables Java programs to run on any device with a Java Virtual Machine (JVM).
- Automatic memory management: The garbage collector manages memory and helps prevent memory leaks.
- Robustness: Strongly typed and focuses on error checking, ensuring high reliability in applications.
Java is extensively used for building enterprise applications, mobile apps via Android, web applications with JavaServer Pages (JSP), and more. Its versatility has earned it a place in many development environments.
Popularity and Scope
Java consistently ranks as one of the most popular programming languages across various surveys and indexes, such as TIOBE and Stack Overflow. Its extensive libraries and frameworks, like Spring and Hibernate, enable developers to optimize the efficiency of their applications. Furthermore, a significant community supports Java, offering countless resources for beginners and professionals alike.
Basic Syntax and Concepts
Understanding the syntax and core concepts of Java is crucial before diving into coding. Here are the basic elements that every developer should grasp.
Variables and Data Types
In Java, a variable is a container used to store data values. Java has specific data types that dictate how much space a variable occupies in memory. Common data types include:
- int: For integer values
- double: For decimal numbers
- boolean: For true/false values
- String: For text information
Example of declaring a variable:
Operators and Expressions
Java operators allow performing different operations on variables and values. They are categorized into different types such as:
- Arithmetic operators: +, -, *, /
- Relational operators: ==, !=, >,
- Logical operators: &&, ||, !
Expressions are formed using variables and operators, carrying out calculations or conditional checks.
Control Structures
Control structures dictate the flow of a program. They include:
- Conditional statements: if, else if, switch.
- Loops: for, while, do-while.
They enable decision-making processes within Java applications.
Advanced Topics
Having established the basics, we can explore more advanced concepts necessary for creating robust applications.
Functions and Methods
Functions, also known as methods, are blocks of code that perform specific tasks. They promote code reusability and enhance clarity. Here’s a simple method example:
Object-Oriented Programming
Java is fundamentally an object-oriented programming (OOP) language. It utilizes objects to combine data and methods, making it easier to design complex applications. Key concepts of OOP include:
- Encapsulation: Bundling data and methods that work on that data.
- Inheritance: A mechanism wherein a new class derives properties from an existing class.
- Polymorphism: The ability to present the same interface for different underlying forms.
Exception Handling
Robust applications require effective error management. Java provides a structured way to handle errors using try-catch blocks:
Hands-On Examples
Practical coding examples help solidify theoretical principles. Here, we present programming assignments at varying levels.
Simple Programs
Creating a basic Hello World program is often the first step:
Intermediate Projects
An intermediate project might involve developing a simple calculator application that employs functions and control structures to perform calculations based on user input.
Code Snippets
Using Java's rich libraries can speed up development processes. A code snippet to read a file could look like this:
Resources and Further Learning
Expanding one's knowledge base is crucial for growth as a developer. Here are some valuable resources:
Recommended Books and Tutorials
Consider reading Effective Java by Joshua Bloch and Java: The Complete Reference by Herbert Schildt, which are excellent for beginners and seasoned programmers alike.
Online Courses and Platforms
Websites like Coursera, Udemy, and Codecademy offer extensive courses tailored to various skill levels.
Community Forums and Groups
Engaging with communities such as Stack Overflow and Reddit’s r/java helps foster learning and problem-solving.
Always seek out ongoing education and resources to stay updated in this dynamic programming language.
By understanding each phase from basics to advanced topics, you will better navigate the Java programming landscape, ultimately empowering your journey as a developer.
Prelims to Java Programming
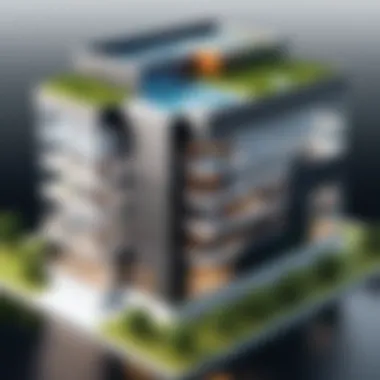
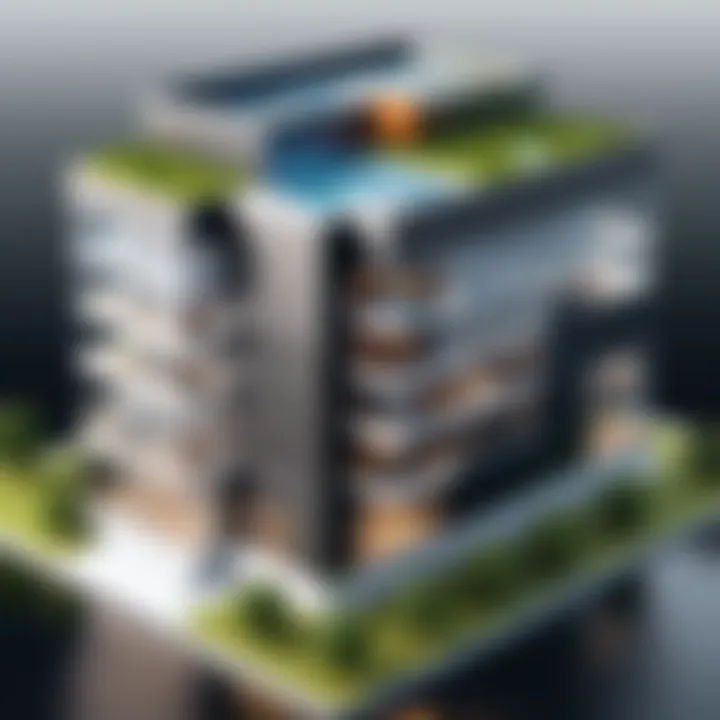
In the realm of software development, Java stands as a pivotal programming language. This section sets the groundwork for understanding why Java is crucial for aspiring developers and how it influences their approach to building applications. The importance of learning Java cannot be overstated. Its wide usage across different platforms and applications, from web development to mobile apps, makes it a versatile tool in any programmer's arsenal.
Understanding Java and Its Applications
Java was designed to be platform-independent, which means that applications developed in Java can run on any device that has the Java Runtime Environment (JRE) installed. This feature is often encapsulated in the phrase "write once, run anywhere." The language's syntax is clean and its structure promotes good programming practices, making it easier for beginners to grasp fundamental concepts.
The applications of Java are diverse. It is used in web applications, enterprise software, and even Android development. The ability to create scalable and robust applications often leads organizations to choose Java for their backend services. The strong community support and extensive libraries also contribute to Java's robustness.
Why Choose Java for App Development
Choosing Java for application development comes with several advantages:
- Object-Oriented Language: Java supports object-oriented programming principles, allowing for code reuse and better organization.
- Large Community and Resources: Many resources, including forums and documentation, can support learners. This community aspect means that assistance is readily available for new programmers.
- Security Features: Java provides a range of security features that help in building safe, secure applications, particularly important for enterprise-level software.
- Mature Ecosystem: With frameworks like Spring for enterprise-grade applications and libraries for handling everything from data access to user interface design, Java has a mature ecosystem that streamlines the development process.
As a newcomer, understanding these facets of Java can be beneficial. Engaging with Java means tapping into a broader community and utilizing a language that emphasizes maintainability and efficiency.
"Java remains a stalwart in programming because it evolves while holding tight to its core principles, making it a reliable choice."
Setting Up Your Development Environment
Setting up your development environment is a crucial step in creating applications using Java. This process not only lays the groundwork for your coding efforts but also ensures that all necessary tools and frameworks align for efficient development. A well-configured environment can significantly enhance productivity, allowing developers to focus on writing code rather than troubleshooting setup issues. Key elements to consider during this stage include the installation of the Java Development Kit (JDK) and the selection of an Integrated Development Environment (IDE).
Installing the Java Development Kit (JDK)
The Java Development Kit is essential for Java programming. It contains the tools needed to develop Java applications, including the Java Runtime Environment, the Java compiler, and various libraries. To begin using Java effectively, installation of the JDK is the first priority.
To install the JDK, follow these steps:
- Visit the official Oracle website or an alternative distribution site such as AdoptOpenJDK.
- Download the appropriate JDK version for your operating system. Both 32-bit and 64-bit options may be available, and you should choose based on your system architecture.
- Run the installer and follow the prompts to complete the installation.
- Set environment variables, such as PATH, to ensure the operating system recognizes Java commands.
- Verify the installation using the command line by typing . This should return the installed Java version.
Proper installation of the JDK is a fundamental step in Java app development. Without it, coding and running applications is not possible.
Choosing an Integrated Development Environment (IDE)
An Integrated Development Environment provides a comprehensive facility to programmers. This is where you will write, edit, test, and debug your code all in one place. A good IDE enhances efficiency through features like syntax highlighting, code suggestions, and integrated version control.
Overview of Popular IDEs
Several IDEs are popular among Java developers. Eclipse, IntelliJ IDEA, and NetBeans are noteworthy choices due to their various features and community support.
- Eclipse is an open-source IDE known for its extensibility via plugins, making it flexible for many types of development.
- IntelliJ IDEA offers a collection of advanced coding assistance features. It is praised for its user-friendly interface and powerful refactoring tools.
- NetBeans is also open-source and is suitable for beginners. It has a straightforward setup process and integrates well with various Java libraries.
The key characteristic of these IDEs is their ability to streamline workflow. For instance, IntelliJ IDEA’s intelligent code assistance can significantly reduce the number of errors in your code, enhancing overall productivity.
Configuring Your IDE
Once you choose an IDE, configuring it to fit your needs is the next step. Proper configuration allows the IDE to integrate smoothly with the JDK and any libraries you plan to use.
In general, configuring an IDE involves:
- Setting the correct JDK location in the IDE’s settings.
- Installing any necessary plugins for additional functionality, such as Git integration.
- Customizing the appearance and behavior to suit personal preferences.
The main advantage of configuring your IDE is that it creates a tailored development environment. A personalized setup can improve your coding speed and allow easier navigation in larger projects.
Ultimately, both JDK installation and IDE configuration play crucial roles in the success of Java application development. When developers invest the necessary effort in these foundational steps, it often results in smoother coding experiences and higher-quality applications.
Learning the Basics of Java Syntax
Understanding Java syntax is critical for anyone wanting to develop applications in Java. It lays the foundation for writing code that is not only functional but also efficient and maintainable. Learning the basics helps in reducing errors and streamlining the coding process. Java syntax gives structure to your code, making it easy to follow. New developers must grasp these core principles to build their programming expertise and create robust applications. This section discusses fundamental concepts, data types, and control flow statements essential for writing Java applications.
Fundamental Concepts and Data Types
Primitive vs. Non-Primitive Types
Primitive types in Java are simple data types that serve as the building blocks for more complex data structures. The key characteristic of primitive data types is their efficiency. They hold their values directly in memory and include types such as , , , and . On the other hand, non-primitive types are objects that are defined by the programmer. They include classes, arrays, and strings. Their usage in this article is beneficial for understanding how data is represented and manipulated in Java.
A significant aspect of primitive types is their ability to operate with minimal overhead. They are faster and use less memory compared to non-primitive types. However, non-primitive types provide added flexibility and functionality. For instance, strings are widely used in applications for user interaction, while arrays are useful for managing lists of data.
Variable Declaration and Initialization
Variable declaration and initialization is a fundamental concept in Java. This aspect refers to the process of defining a variable's name and its data type before using it in the code. The key characteristic of this process is clarity. When variables are declared with a specific type, the Java compiler can enforce type safety. This ensures that incorrect data types cannot be assigned to variables, leading to fewer runtime errors.
Declaring and initializing variables is straightforward but crucial for effective programming. For example, a variable defined as specifies that 'count' is an integer and starts with a value of zero. The unique feature of declaring a variable is its scoping rules, which define where the variable can be accessed. Proper initialization reduces the risk of errors and helps maintain cleaner code.
Control Flow Statements
If Statements
If statements are essential for introducing logic in a Java application. They allow the program to make decisions based on certain conditions. The key characteristic of if statements is their capability to evaluate conditions and execute specific blocks of code accordingly. This feature makes them popular in programming since they facilitate branching logic.
For example, consider the following code:
This illustrates how if statements control the flow of execution based on the evaluation of 'age'. Understanding if statements is vital for implementing decision-making processes within applications, thus improving their functionality.
Loops
Loops are another integral part of the control flow in Java. They enable repetitive execution of a block of code as long as a specified condition holds true. The key characteristic of loops is their ability to handle numerous iterations without duplicating code. They are essential for tasks that require repetitive actions, such as processing items in a list.
The loop is a common choice among developers. It allows control over the number of iterations, making it ideal for situations where the number of repetitions is known. For example:
This code outputs numbers from 0 to 9. Loops improve code efficiency and readability by reducing repetition, a crucial factor in modern programming practices.
Learning these basics of Java syntax enhances a developer's ability to write organized and functional code. Each concept plays a pivotal role in the broader context of application development, ensuring that beginners build strong foundational skills.
Object-Oriented Programming in Java
Object-oriented programming (OOP) is a foundational paradigm in Java that provides a clear structure for organizing code. This section emphasizes the significance of OOP within Java development, outlining its benefits and considerations. By utilizing OOP, developers can create modular, reusable code. This not only simplifies debugging and maintenance but also fosters collaboration among teams, as different modules can be worked on separately.
Understanding Classes and Objects
Defining a Class
A class serves as a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. This feature allows for better organization of code. Defining a class provides a clear structure that makes it easier to maintain and read. In the context of this guide, it’s a popular choice because it promotes reusability and prevents code duplication. A unique aspect of class definitions in Java is their use of access modifiers, which control accessibility. This means sensitive data can be protected from outside interference, an advantageous characteristic that enhances security in programming applications.
Creating Objects
Creating objects is the actual instantiation of a class. When an object is created, memory is allocated for its data. This step is crucial as it allows developers to create multiple instances of a class without rewriting code. Creating objects is straightforward in Java, which makes the language user-friendly for beginners. It provides a practical way to implement functionality through method calls and allows for easy interaction with class attributes. A unique feature here is the ability to use constructors, which are special methods invoked during the creation process. While creating object instances can consume resources, proper management leads to effective application performance.
Inheritance and Polymorphism
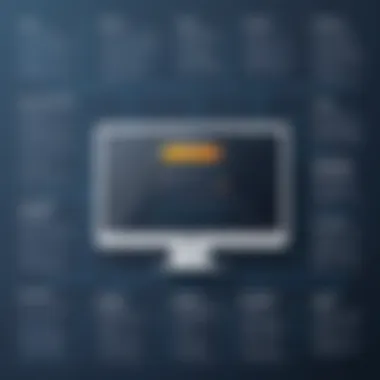
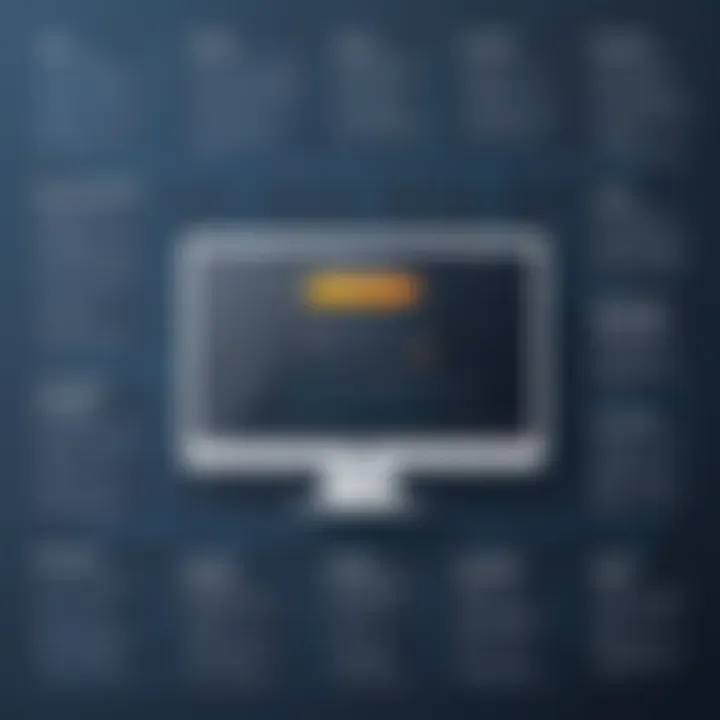
Inheritance Hierarchies
Inheritance is a mechanism that allows a new class to inherit attributes and methods from an existing class. This Inheritance hierarchies system enables code reuse and establishes a clear relationship between classes. For developers, using inheritance is beneficial as it simplifies code management and reduces redundancy. When establishing an inheritance hierarchy, it is essential to understand the hierarchy’s structure, as it determines how classes interact. This clarity can sometimes lead to over-engineering if not thoughtfully structured, highlighting the need to balance complexity and simplicity in design.
Method Overriding
Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. This feature promotes flexibility and enables developers to adjust behavior without altering the parent class. Utilizing method overriding is advantageous, particularly in frameworks where behavior needs to be adapted. However, it can introduce confusion if not documented properly, as future developers may unintentionally introduce bugs. Therefore, it’s vital to maintain clear communication and documentation regarding overridden methods, ensuring that the functionality remains understandable and manageable.
Developing Your First Java Application
The journey of software development culminates when one progresses to develop their first application with Java. This section is crucial as it not only marks the transition from theory to practical application but also introduces key concepts that will be essential for any aspiring developer. It involves both planning and implementation, which are fundamental elements in shaping a successful project.
Planning Your Application
Before writing any code, a thorough plan is essential. This planning phase involves defining the project scope, understanding user needs, and outlining the application's core functionalities. Clarity in this phase significantly reduces complications in later stages.
- Defining Project Goals: Identify what your application will achieve. Will it solve a specific problem or provide a service? Clear goals guide development.
- User Research: Understand your target audience. Getting feedback from potential users can inform your choices.
- Feature List: Create a list of desired features. Prioritize them to focus on must-haves over nice-to-haves, ensuring a functional MVP (Minimum Viable Product).
- Design Mockups: Visualizing the application through drawings or wireframes helps clarify the user interface and user experience.
By investing time in planning, you lay a strong foundation for your application that can adapt as needs evolve.
Implementing the Application Code
With a comprehensive plan in place, the next step is implementation. This is where the ideas transition into actual functionality through code. The two main tasks in this phase are writing functions and debugging.
Writing Functions
Functions are the building blocks of any application. They encapsulate reusable code, making it easier to manage and maintain. One significant aspect of writing functions is their ability to increase code readability and organization.
- Key Characteristics: Functions allow for breaking down complex problems into smaller, manageable pieces.
- Benefits: This modular approach means that changes can be implemented without affecting the entire code base. It also allows for easier testing.
- Unique Features: Functions can take parameters and return values, enabling interaction with other parts of the application.
However, it is vital to keep functions focused. A function should accomplish one specific task. If it tries to do too much, it becomes challenging to debug and maintain. This single-responsibility principle is a popular guideline among developers.
Debugging Techniques
After writing code, the next step is debugging. Debugging techniques are pivotal for ensuring that your application runs smoothly and performs the intended functions.
- Key Characteristics: Debugging involves identifying errors in code and correcting them. This process is iterative and often requires multiple passes.
- Benefits: Effective debugging helps maintain the integrity of the application. It can catch issues before they reach end users, enhancing overall quality.
- Unique Features: Tools like integrated debuggers in IDEs can visually aid in tracking down problematic code sections.
That said, debugging can be time-consuming. It requires patience and a thorough understanding of the application flow, which can sometimes lead to frustration.
By meticulously planning and implementing your first Java application, you set the stage for future projects. Each concept learned here builds towards greater programming mastery.
This phase combines creativity with technical skill, allowing developers to bring their ideas to life in concrete form. It is a fundamental step in the journey of becoming a proficient Java developer.
User Interface Design in Java
User interface (UI) design is a critical component in the development of applications. A well-designed UI can enhance usability and foster user engagement. In the context of Java development, the UI is not just about aesthetic appeal; it also serves as the bridge between the user and the underlying functionalities of the application. The effectiveness of an application can often hinge on how intuitively users can interact with it.
A fundamental principle in user interface design is to make the application intuitive. Users should be able to navigation seamlessly through the application without extensive instruction. This involves adhering to design standards and employing recognizable visual cues. Java provides several libraries, such as JavaFX, that allow developers to create responsive and attractive interfaces.
Understanding the potential audience and their needs is imperative. For example, if an application is aimed at elderly users, larger buttons and simpler layouts might be more suitable than a visually complex design.
Using JavaFX for GUI Development
JavaFX is a powerful library that enables the creation of rich user interfaces for Java applications. Unlike its predecessor Swing, JavaFX allows for a more modern approach to design, including features such as CSS styling, FXML for structure, and Integration with 2D and 3D graphics. It enables developers to create visually appealing applications that can run across various platforms.
Key Benefits of JavaFX:
- Rich Functionality: JavaFX supports complex controls and animations, which can enhance user engagement.
- CSS Support: Developers can utilize CSS to manage the appearance of UI elements, separating design from business logic.
- FXML: This XML-based language simplifies the layout description of a JavaFX application. Developers can define the UI in FXML and link it with Java code seamlessly.
Here is a simple example of a JavaFX application structure:
Event Handling in Java Applications
Event handling is fundamental in responsive UI design. It allows the application to react to user actions, enhancing interactivity and user experience. In Java, event handling is done through a listener pattern where listeners respond to specific actions like clicks, key presses, or mouse movements.
In JavaFX, various events can be handled easily. For example, when a button is clicked, an action event can be triggered, causing a specific method to execute. This makes the application not only responsive but also tailored to user interactions, which is key to maintaining user interest and satisfaction.
Important Considerations for Event Handling:
- Performance: Overly complex event handling can cause lag in the UI. Ensuring that listeners are appropriately registered and accounted for is critical.
- User Experience: Feedback is essential. If the application takes time to process an action, providing visual indicators of loading or progress helps manage user expectations.
- Error Handling: Robustness in event handling protects the application against unforeseen situations, ensuring errors are caught and appropriately managed.
Data Management and Persistence
Data management and persistence are vital components of application development. The ability to handle data effectively can determine the usability and functionality of the application. In Java, managing data usually involves databases and the interaction between the application and the data storage. Understanding how to effectively connect to databases and manage data operations is crucial for building reliable applications.
Data persistence refers to saving data in a stable storage medium for future use. When an application is restarted, it should be able to retrieve data from its previous state. This is not just about saving user inputs but also about ensuring data integrity and consistency across sessions. A well-thought-out data management approach simplifies this task, enhancing performance and reliability.
Connecting to Databases with JDBC
JDBC, or Java Database Connectivity, is a Java API that enables Java applications to interact with a variety of databases. It acts as a bridge between Java applications and databases, allowing developers to execute SQL statements and transfer data. The advantages of using JDBC include its portability and the ability to access different databases with minimal changes in code.
When configuring a JDBC connection, several elements must be addressed:
- Driver: This component allows the application to communicate with a particular database. Each database requires a specific JDBC driver.
- Connection URL: This string specifies the database location and credentials required.
- User Credentials: These details help authenticate the user accessing the database.
JDBC is suitable for many applications. However, it does require a good understanding of SQL and database management principles.
Handling Data with ORM Frameworks
Object Relational Mapping (ORM) frameworks simplify database interactions by mapping object-oriented programming models to database tables. One of the most popular ORM frameworks in the Java ecosystem is Hibernate.
Foreword to Hibernate
Hibernate streamlines database interactions in Java applications by bridging the gap between object-oriented programming and relational databases. Its key characteristic is the ability to reduce boilerplate code related to data operations.
Hibernate provides a configuration framework that allows developers to specify and manage mappings between classes and database tables easily. This leads to improved productivity, as it supports a rapid development cycle. A unique feature of Hibernate is its caching mechanism, which optimizes performance by minimizing database access.
While Hibernate is widely used, it may introduce complexity in smaller applications where the overhead may not be justified. Thus, developers must evaluate their specific needs before deciding to incorporate Hibernate into their project.
Defining Entities and Repositories
Defining entities and repositories is fundamental in managing data with ORM frameworks. An entity in Hibernate typically represents a table in a database. The attributes of the entity class correspond to the columns of the table.
Repositories act as intermediaries between the application and the data source, encapsulating data access logic. This separation of concerns is beneficial, allowing for easier maintenance and a clearer code structure. The ability to define query methods directly in the repository interface further enhances developer efficiency.
However, one potential disadvantage is that improper design of entities and repositories can lead to performance issues. Developers need to structure their business logic properly to avoid unnecessary complexity and overhead.
In summary, understanding data management and persistence is a critical skill for Java developers. JDBC provides foundational capabilities for connecting to databases, while ORM frameworks like Hibernate enhance data interaction processes, making the developer's job easier.
Testing and Quality Assurance
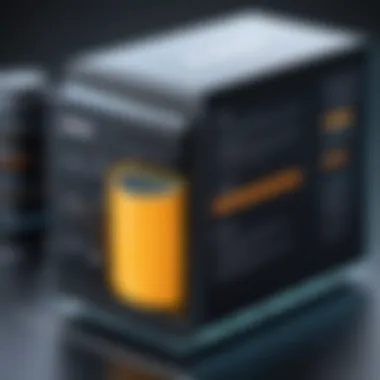
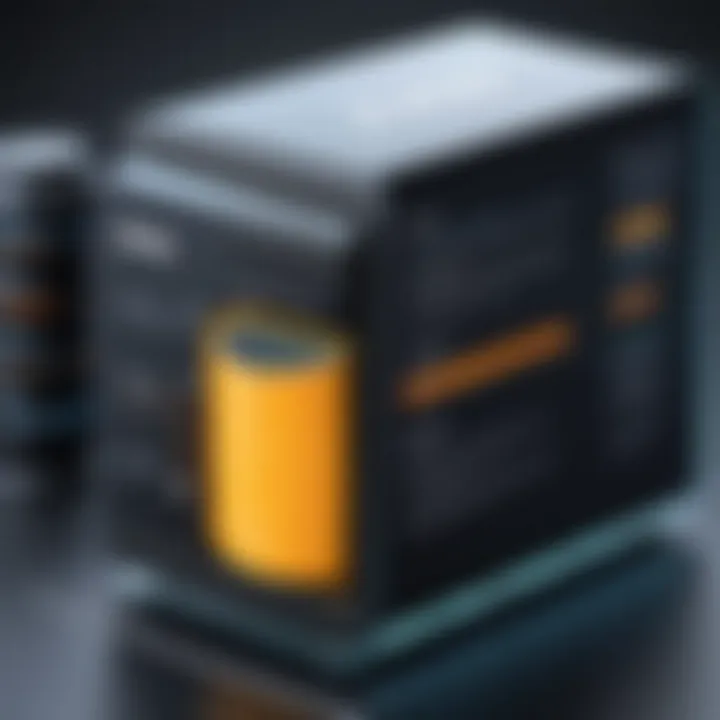
Testing and quality assurance are critical components in the software development lifecycle, especially when working with Java applications. Their significance cannot be understated; they ensure that your application is not only functional but also resilient and user-friendly. Testing helps identify defects early, reducing the cost and time associated with fixing issues later. It provides clarity and confidence in the functionality of the application, offering a solid foundation upon which further development can occur.
Quality assurance encompasses various practices aimed at ensuring that the software meets certain standards and requirements. In this context, the focus is not just on finding bugs but ensuring that the overall user experience is polished. Implementing effective testing and quality assurance procedures leads to reliable applications, enhances user satisfaction, and promotes long-term maintenance. Therefore, developers must prioritize these aspects throughout the development process.
Unit Testing with JUnit
Unit testing is the practice of testing individual components of an application in isolation to verify that each functions as intended. JUnit is a widely-adopted framework for unit testing in Java, allowing developers to write and execute tests effectively.
Some key benefits of using JUnit include:
- Simplicity: JUnit provides a straightforward syntax for defining tests, making it easy for developers to get started quickly.
- Automation: Tests can be run automatically, which helps integrate them into the overall development process. Automated tests can be executed frequently to catch regressions early.
- Ease of Integration: JUnit works smoothly with build tools like Maven and Gradle as well as various IDEs, enhancing its usability.
When creating unit tests with JUnit, it is important to focus on isolated tests. Each test should cover a specific piece of functionality. This can be done using annotations to specify test methods and assertion methods to verify expected behavior. A simple unit test might look like this:
This example demonstrates how to verify that the method correctly sums two integers.
Integration and Automated Testing
Integration testing takes a broader approach by evaluating how different modules or services interact with one another. While unit tests focus on individual components, integration tests ensure that a collection of components works correctly together. This is essential, as individual modules may work perfectly in isolation but can fail when combined.
The term automated testing refers to the practice of using scripts or software tools to execute tests automatically. Automation enhances efficiency by reducing the time spent running tests manually and allowing for more extensive test coverage. Automated tests can include both unit and integration tests, providing a comprehensive view of the system.
Benefits of integration and automated testing include:
- Increased Coverage: Automated tests can cover more scenarios compared to manual testing, uncovering issues that might not be identified otherwise.
- Speed: Automation can run tests much faster than a human can execute manual checks, making it feasible to run all tests frequently.
- Consistency: Automated tests can ensure that the same tests are run in the same way each time, minimizing human error.
A good practice is to implement continuous integration (CI) tools that run both unit and integration tests automatically upon code changes. This ensures immediate feedback for developers and aids in maintaining code quality in the long run.
In summary, thorough testing and quality assurance practices are vital in the context of Java application development. Developers are strongly encouraged to adopt JUnit for unit tests and automated testing strategies to facilitate integration testing. These steps will not only enhance the quality of their applications but also improve their overall development workflow.
Deploying Your Java Application
Deploying a Java application marks a critical phase in the development cycle. This step transforms your code from a development environment into a usable product for your target audience. Deployment is not just about running your application; it involves several specific elements that are pivotal for operational success. By understanding these components, developers can ensure their applications run smoothly and are accessible to users.
Packaging Your Application
Creating an executable, jar (Java Archive) file is an essential practice in Java deployment. This method simplifies the process of packaging your application with all its resources and dependencies into a single file. The primary benefit of using JAR files is convenience. They allow users to store and execute Java programs seamlessly, which enhances user experience.
Creating Executable JAR Files
To create an executable JAR file, developers generally gather all necessary classes and resources into one archive. The key characteristic of an executable JAR is its ability to be launched by the Java Runtime Environment directly, eliminating the need for users to understand the application’s structure. This contributes significantly to easier distribution of software.
A unique feature of executable JAR files is their self-contained nature. They encapsulate not just the files, but also any libraries and graphics that the application may depend upon. This simplifies deployment to end-users who might lack technical knowledge.
However, there are some considerations. JAR files can become large if numerous resources are included. This can lead to longer download times for users. Nonetheless, the advantages generally outweigh such drawbacks, especially as users tend to appreciate the simplicity of the installation process.
Distribution Considerations
Moving to the distribution aspect, it is important to carefully consider how your application will reach its end-users. Effective distribution can directly impact the accessibility and popularity of your software. A notable characteristic of this stage is clarity. Clear instructions on where to download and how to run the application are essential for user satisfaction.
One unique feature of distribution considerations is the decision on the platforms to target. This varies widely based on your audience. For instance, if you focus on desktop applications, you might want to distribute via direct download from your website or GitHub. On the other hand, if targeting mobile users, you may consider utilizing platforms like Google Play or the Apple App Store.
The main advantage of careful distribution planning includes maximizing user engagement. Conversely, a lack of thoughtful strategy can lead to confusion and dissatisfaction among users, which could inhibit the application’s success. Thus, it's intelligent to invest time in distribution tactics right from the beginning, ensuring that your application gets the visibility and user base it deserves.
Publishing to App Stores
Publishing your application to app stores serves as a crucial final step in the deployment process. It allows developers to reach a wider audience and enhances credibility. App stores provide a platform where users look for applications they can trust.
This can be beneficial for developers as well. Being listed in established stores offers visibility that individual websites cannot match. Additionally, app stores often come with built-in promotional aspects, such as user ratings and support systems, which can further drive downloads.
However, developers must consider the guidelines and requirements of each app store, which can often be stringent. This includes quality checks for security and performance. Preparing for these can be tedious, but it ultimately ensures only high-standard apps are available to users.
In summary, deploying a Java application efficiently requires thorough planning and execution across packaging, distribution, and publishing aspects. Focusing on these elements ensures your application reaches its audience effectively and retains user satisfaction.
Maintaining and Updating Your Application
Maintaining and updating your application is a crucial phase in the application lifecycle. As software evolves, user expectations change and technology advances. Proper maintenance ensures that the application remains functional, relevant, and efficient. Regular updates can fix bugs, enhance functionality, and even improve security. Users want reliable applications, and neglecting maintenance can lead to performance issues, security vulnerabilities, and dissatisfied users.
In this section, we will explore two critical components of application maintenance: version control and user feedback. Both are essential for achieving continuous improvement and keeping your application engaging and effective.
Version Control and Best Practices
Version control is a method to track and manage changes in code or documents. It is vital for collaborative environments where multiple developers might work on the same codebase. Without version control, keeping track of contributions becomes cumbersome, leading to potential conflicts and confusion.
Git is one of the most popular version control systems used today. It provides several advantages:
- Collaboration: Enables multiple developers to work on the same project without overwriting each other's changes.
- Backup: Maintains a history of changes that can be reverted if issues arise.
- Branching: Allows developers to create separate branches to experiment with new features safely.
Best practices when using version control include:
- Frequent Commits: Submit changes often to capture progress and reduce merge conflicts.
- Clear Commit Messages: Use descriptive messages that summarize the changes made. This enhances clarity and assists the team in understanding the timeline of development.
- Code Reviews: Encourage peer reviews before merging code. This practice ensures quality and minimizes the introduction of bugs.
Following these practices will help maintain a clean and manageable codebase. It will also enhance team collaboration and facilitate smoother updates.
User Feedback and Continuous Improvement
User feedback is crucial for identifying areas for enhancement and gauging satisfaction. Applications evolve based on real-world use cases, and direct input from users can guide development decisions effectively.
Collecting user feedback can take various forms:
- Surveys: Implement surveys within the application to gather insights about the user experience.
- User Interviews: Conduct interviews to dive deeper into specific pain points faced by users.
- Analytics: Utilize tools to track usage patterns, which can highlight popular features as well as those needing attention.
Incorporating feedback systematically in the development cycle is essential for continuous improvement. This does not mean reacting to every piece of feedback, but rather analyzing trends and making informed decisions. Consider keeping a feedback log to organize and prioritize suggestions, which can later inform roadmaps for feature development.
Continuous improvement is not a set-and-forget strategy; it is an ongoing commitment to evolve and adapt based on user needs and technological advancements.
In summary, maintaining and updating applications is vital to ensure their success over time. By leveraging version control effectively and integrating user feedback, developers can create applications that not only function well but also resonate with their intended audience.
End
In the realm of Java application development, concluding an article carries immense significance. The conclusion serves as a reflective summary that encapsulates the key elements discussed throughout the guide. It reinforces the foundational principles, design methodologies, and technical skills pertinent to crafting efficient Java applications.
The importance of this section extends beyond mere summary. It offers readers an opportunity to synthesize the information learned and solidifies their grasp of Java programming concepts. Delving into critical aspects, one can gain insights about best practices, the iterative nature of development, and the importance of adaptiveness in the evolving tech landscape. Each component discussed contributes to a holistic understanding of Java application creation.
Recap of Key Concepts
Throughout this article, several core concepts have emerged that are vital for anyone seeking to master Java application development. Here are some key takeaways:
- Java Basics: Understanding Java's data types, syntax, and control flow is foundational. Grasping primitive and non-primitive types helps to lay the groundwork for more complex programming.
- Object-Oriented Principles: Emphasizing classes and objects, inheritance, and polymorphism illustrates Java’s strengths in fostering code reuse and organization. This paradigm encourages developers to build scalable applications.
- User Interface and Experience: Utilizing JavaFX demonstrates how to enhance user engagement through effective GUI design. This section not only covered aesthetics but also user interactions.
- Data Management: Connecting with databases and using ORM frameworks like Hibernate signifies the importance of data handling and persistence in application development.
- Testing and Deployment: Exploring unit testing methodologies and deployment strategies highlights the necessity of ensuring quality assurance before applications reach end-users.
Future Directions in Java Development
Looking ahead, several trends and technological advancements are shaping the future of Java development. These include:
- Cloud-Native Applications: With the rise of cloud computing, developers are shifting towards cloud-native technologies that enhance scalability and resilience. Java frameworks that support microservices architecture are gaining traction.
- Integration with AI and ML: The integration of Java with artificial intelligence and machine learning signifies a shift towards creating smart applications. Leveraging Java's capabilities can lead to innovative solutions in various sectors.
- Kotlin and Multiplatform Development: As Kotlin emerges as a favorable alternative for Android application development, its interoperability with Java paves the way for more flexible and robust mobile applications.
- Continued Evolution of Java: The ongoing updates in Java, such as Project Loom and Project Panama, aim at simplifying concurrent programming and improving performance, furthering its relevance in modern software development.
Overall, Java remains a powerful tool for developers. Adapting to new technologies ensures its vitality in the application development landscape. This guide provides a comprehensive foundation, and aiming to stay informed on these developments will empower aspiring developers in their journey.