Creating APIs in Java: A Complete Development Guide
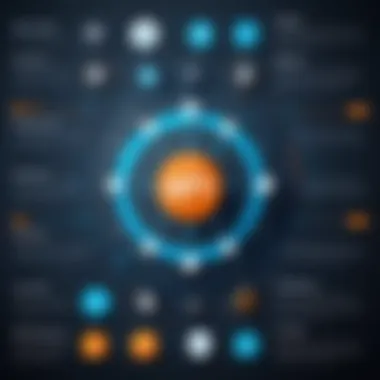
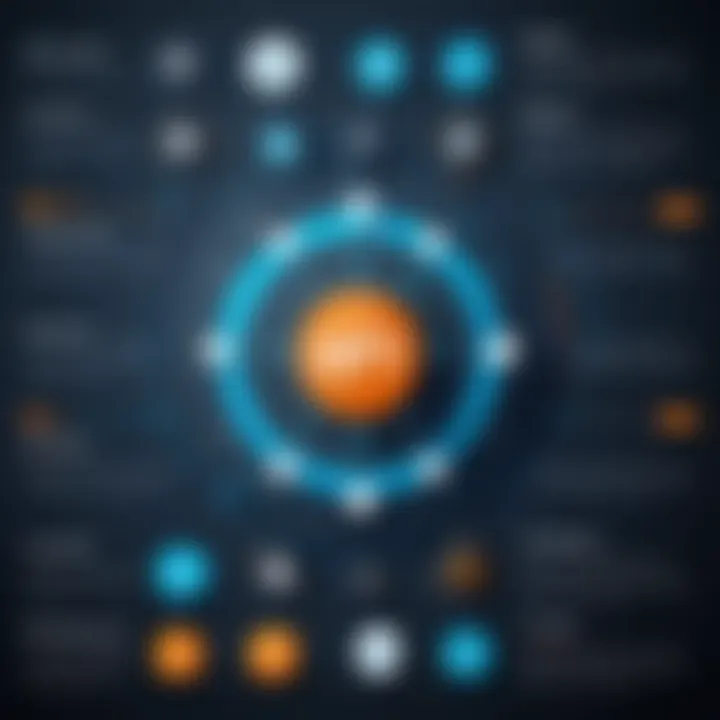
Intro
Creating an API in Java is a journey through the alleys of software development. Itâs not just about writing code; itâs embarking on a quest to facilitate communication between different software systems. An Application Programming Interface, or API as itâs commonly known, serves as the backbone for many modern applications. With the widespread use of mobile apps, web services, and cloud-based solutions, understanding how to build a robust API has become an essential skill for developers.
The relevance of APIs in our current technological landscape cannot be overstated. These interfaces enable different software modules to interact seamlessly, thus opening doors for integration and data sharing. In Java, a language famed for its robustness and versatility, creating an API can be both a challenging and rewarding endeavor. From beginners eager to dive into the world of programming to seasoned developers looking to enhance their skill set, this guide provides comprehensive insights.
In the sections that follow, we will peel back the layers of API development, exploring fundamental concepts, best practices, and advanced techniques. Youâll get to grips with essential topics like API architecture, designing RESTful services, and handling exceptions. The aim is to equip you with both theoretical knowledge and practical skills, ensuring that you come away with a well-rounded understanding of Java API development.
So strap in, and letâs get started!
Preamble to APIs
In todayâs interconnected digital landscape, APIs are becoming the bread and butter for software development. APIs, or Application Programming Interfaces, serve as the bridge that enables different software programs to communicate with one another. They fulfill a crucial role, one that goes far beyond mere data exchange. Understanding APIs is not just a technical necessity; itâs fundamental for anyone engaged in programming, particularly with Java.
Definition of APIs
APIs are essentially a set of rules and protocols for building and interacting with software applications. Think of an API as a waiter in a restaurant; you give the waiter your order, the waiter communicates your request to the kitchen, and then delivers your food back to you. In this analogy, your meal represents the data or function you want from the software service. The waiter (API) manages the communication between you and the kitchen (the server).
To put it simply, an API specifies how software components should interact. They allow applications to use functionalities of other applications or services, without needing to know how they are implemented. An API can take various shapes depending on its specific use case: web-based APIs, library-based APIs, or operating system APIs, to name a few. Each serves a unique purpose, yet they all facilitate the same underlying concept: connection.
Importance of APIs in Software Development
The significance of APIs in software development cannot be overstated. They are like the glue that holds disparate software systems together. Using APIs not only enhances interoperability but also propels innovation. Here are a few key points on why APIs hold such value:
- Facilitating Integration: APIs allow different software programs to work together. For instance, if you're building a web application that requires payment processing, you might integrate with the Stripe API rather than developing a payment solution from scratch.
- Enhancing Efficiency: With APIs, developers can reuse functionality instead of reinventing the wheel. If one programmer built a robust library for data encryption, others can tap into that library via an API rather than coding their own.
- Streamlining Updates: Changes in one application do not necessarily mean a full system overhaul. APIs can allow developers to update their software components independently.
- Improving Scalability: As projects grow, APIs enable developers to expand the system's capabilities without major disruptions.
"APIs are the connective tissue of modern software development, allowing for greater efficiency, better integration, and limitless innovation."
In other words, APIs have transformed the way software is created and maintained. Understanding how APIs operate and how to create them is essential for any programmer looking to thrive in the tech industry. Java, being a versatile and popular programming language, offers numerous frameworks and libraries that can simplify API development. In the sections that follow, we will explore the core Java fundamentals necessary for creating APIs, the various types of APIs, and the popular frameworks to consider when diving into Java API development.
Java Fundamentals for API Development
When creating APIs in Java, a solid grounding in fundamental concepts is paramount. This knowledge not only sets the groundwork for efficient API design but also facilitates effective communication with other developers. Understanding Java fundamentals enriches one's grasp of how APIs function, especially in terms of object manipulation and communication across platforms.
Core Java Concepts
Core Java concepts are the building blocks every Java developer must master. These principles include the elements of the Java programming language that are used repeatedly across applications and frameworks. Knowing these will allow developers to craft more efficient and reliable APIs.
- Syntax and Data Types: Javaâs syntax is critical; itâs how one communicates with the compiler. The basic data typesâlike integers, floating points, and charactersâare foundational. Knowledge of these types helps in correctly handling API data, which may come in various formats.
- Control Statements: Understanding loops and conditional statements is vital. Control statements dictate the flow of the application, allowing developers to build logic around API requests.
- Collections Framework: Javaâs built-in collections framework is a lifesaver when handling groups of objects. Knowledge of lists, sets, and maps provides the tools needed to store, retrieve, and modify data efficiently.
"Mastering core concepts in Java is akin to laying bricks to build a solid foundation; without it, the structure will surely crumble."
- Exception Handling: Writing robust APIs means anticipating errors and handling them gracefully. Exception handling in Java allows developers to catch and manage different forms of errors, ensuring the API remains functional even when unexpected situations arise.
- Multi-threading: In today's fast-paced environment, multi-threading empowers developers to execute multiple operations simultaneously. Understanding this aspect can tremendously enhance API performance, particularly in handling numerous requests concurrently.
Understanding Object-Oriented Programming
Object-oriented programming (OOP) is not just a trend; itâs a paradigm shift thatâs reshaped software development, especially in Java. It revolves around the use of objects to encapsulate data and behavior. Fostering a firm grasp on OOP principles can significantly amplify the capability and scalability of APIs.
- Classes and Objects: At the heart of OOP are classes and objects. Classes serve as blueprints for creating objects, which represent real-world entities in code. Knowing how to effectively structure classes allows developers to encapsulate and abstract data, crucial for creating clean and maintainable APIs.
- Inheritance: This concept enables one class to inherit properties and behavior from another. Itâs particularly useful in APIs as it allows for code reusability and the creation of more specialized methods without the need for boilerplate code.
- Polymorphism: Polymorphism allows for method overloading and overriding, offering flexibility in API functionality. By defining multiple methods with the same name but different parameters, developers can create more dynamic APIs.
- Encapsulation: This principle is all about restricting access to certain components of an object and hiding the internal state. By controlling how data is accessed or modified, APIs become more secure and reliable.
- Abstraction: Abstraction focuses on simplifying complex systems by providing a clear interface while hiding the intricate workings behind it. This principle is vital in API design, ensuring that consumers interact with a straightforward interface while the underlying complexities remain concealed.
By solidifying the understanding of these core Java concepts and OOP principles, developers will be better equipped to navigate the complex landscape of API development, crafting solutions that are not only functional but also elegant in their design.
Types of APIs
APIs are the backbone of modern software development, facilitating communication between different systems. Understanding the various types of APIs is crucial for any developer looking to create effective and efficient software solutions. This section will break down the three main types of APIs commonly used in Java development: RESTful APIs, SOAP APIs, and GraphQL APIs. Each type has its unique strengths, weaknesses, and use cases that significantly influence design choices and implementation.
RESTful APIs
RESTful APIs have surged in popularity over the years due to their simplicity and flexibility. REST stands for Representational State Transfer, and these APIs leverage the HTTP protocol for communication. One of the main advantages of RESTful APIs is their statelessness; this means that each request from a client must contain all the information the server requires to fulfill that request.
This independencia from any stored context simplifies load balancing and scalability. Moreover, the use of standard HTTP verbs like GET, POST, PUT, and DELETE makes it easier to understand the actions that can be performed on resources.
Consider the following key features of RESTful APIs:
- Resource-Based: Each resource is represented by a unique URL, allowing developers to easily retrieve, modify, or delete resources.
- JSON Format: REST APIs commonly use JSON for data exchange, which is lightweight and easy to work with.
- Caching: Server responses can be cached for better performance, reducing latency during high-traffic periods.
However, itâs important to consider that RESTful APIs may struggle with complex queries that require multiple resource manipulations, as they can involve multiple network calls.
SOAP APIs
SOAP, or Simple Object Access Protocol, is another type of API that is often associated with enterprise-level applications. Unlike REST, which is generally easier to use and more flexible, SOAP APIs are heavily protocol-driven and rely on XML for message formatting.
One of the primary strengths of SOAP APIs is their built-in support for security and transaction compliance. They offer features like ACID compliance, which makes them suitable for applications that demand strict integrity and reliability. Here are some key points to note about SOAP APIs:
- Strict Specifications: SOAP protocols are well-defined, with rules about messaging structure, making them predictable.
- Built-in Security: They come with various standards like WS-Security for secure messaging.
- Extensibility: SOAP allows for extensibility through its enveloping mechanism, which can facilitate more complex message structures.
Nonetheless, this complexity comes with increased overhead both in terms of coding and performance. SOAP may not be the go-to choice for every project, especially when rapid development is crucial.
GraphQL APIs
GraphQL represents a newer approach in the API landscape, offering a different way of sending and receiving data. Developed by Facebook, this query language allows clients to request only the data they need, which can reduce the amount of data transferred over the wire. This is particularly advantageous in mobile applications, where bandwidth can be a limiting factor.
GraphQL APIs operate on a single endpoint that handles all queries and mutations, simplifying the client-side logic. Here are a few points that highlight the benefits:
- Client-Specified Queries: Clients can specify the exact structure of the response, minimizing over-fetching or under-fetching of data.
- Strongly Typed Schema: The API is defined by a schema that details the available types and operations, enhancing predictability.
- Versionless APIs: Since clients determine their data needs, updating existing APIs doesnât require versioning, easing the burden on developers.
Yet, this modern approach may come with its challenges, such as more extensive upfront learning and configuration requirements. Additionally, for extremely simple APIs, GraphQL might be overkill.
"Choosing the right API type depends on your project needs, the complexity of operations, and development speed. Each has trade-offs, so weigh your options carefully."
Frameworks for Developing APIs in Java
When we discuss building APIs in Java, one cannot overlook the significance of frameworks. They streamline development processes, enhance code maintainability, and provide powerful tools that help developers craft robust APIs efficiently. In a nutshell, a well-chosen framework acts as a solid foundation, allowing you to focus on your application's logic rather than getting bogged down in boilerplate code.
Frameworks typically offer a suite of pre-built functionalities that can help handle the heavy lifting of request processing, response serialization, and even security concerns. Additionally, they provide integrations with various libraries and protocols - making life easier for developers.
Spring Boot
Spring Boot has emerged as a leading framework for developing APIs due to its simplicity and versatility. With a gentle learning curve, it enables developers to start building applications almost immediately, giving them the liberty to configure just what they need. Spring Bootâs auto-configuration feature means you can set up your projects quickly, without extensive manual setup.
One of the crown jewels of Spring Boot is its powerful integration with the Spring ecosystem. This allows you to utilize components like Spring Security for securing your APIs or Spring Data to ease database interactions. By adhering to the conventions of the Spring framework, Spring Boot lets you focus on developing business functionalities rather than getting lost in unnecessary configurations.
In addition, Spring Boot's support for RESTful APIs aligns perfectly with modern application needs. You can expose resources via HTTP endpoints, providing a clear and standardized interface for clients to interact with.
Jakarta EE
Jakarta EE, formerly known as Java EE, is another heavyweight in the realm of enterprise applications. It offers a comprehensive set of specifications aimed at building large-scale, multi-tiered applications. One of its notable features is the enterprise JavaBeans (EJB) technology which fosters seamless transactional management.
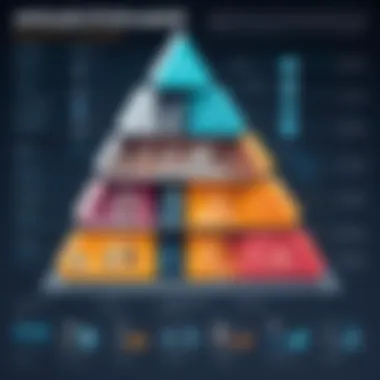
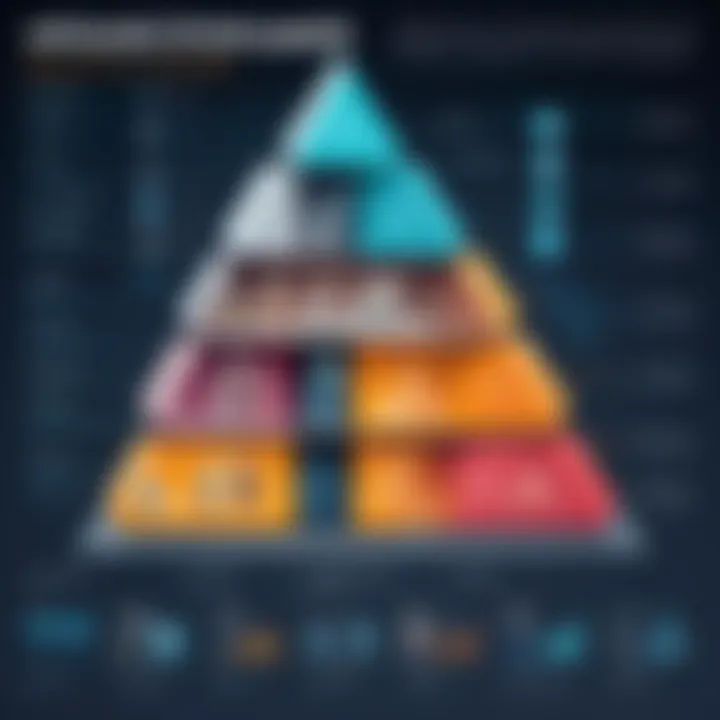
For API development, Jakarta EE emphasizes the use of JAX-RS (Java API for RESTful Web Services), which simplifies the task of creating REST interfaces. It provides annotations that help you define resources and operations in a clean and intuitive manner.
Moreover, Jakarta EE operates on a robust platform suited for heavy-duty applications. The integrated features, from dependency injection to security models, enable developers to focus on building secure, scalable APIs without reinventing the wheel.
MicroProfile
MicroProfile emerged to cater specifically to the modern needs of microservice architecture. It enhances the capabilities of Jakarta EE by introducing a set of APIs and specifications that are designed to work seamlessly within microservices frameworks. MicroProfile provides support for configuration, fault tolerance, and JWT authentication, crucial for building APIs that are both resilient and secure.
One of the key benefits of using MicroProfile is its emphasis on cloud-native development. This framework works well with containerization technologies like Docker, making it easier to deploy APIs in various environments, be it local, staging, or production.
Additionally, MicroProfile promotes fast-paced development and simplicity, allowing developers to concentrate on writing functional code. Its annotations and a lean footprint make for an efficient coding experience, minimizing performance overhead while maximizing productivity.
"Choosing the right framework is like selecting the right tool for the job; it can make or break your development experience."
All in all, when it comes to creating APIs in Java, the right framework can significantly influence your workflow and production outcomes. Frameworks like Spring Boot, Jakarta EE, and MicroProfile each bring unique strengths to the table, catering to different project requirements and developer preferences. Understanding these frameworks can aid you in determining which one suits your needs the best.
Designing the API
Designing an API is like crafting a blueprint for a building; it establishes the structure and flow of interactions between different software components. A well-designed API not only makes the implementation more straightforward but also enhances the user experience for those who consume it. This section will explore the critical elements of API design, including how to choose the right API style, model resources effectively, and define endpoints that facilitate efficient communication.
Choosing the Right API Style
When embarking on the journey of API design, the first decision revolves around selecting the right API style. This choice sets the tone for how users will interact with your service. Various styles exist, each catering to different needs:
- RESTful APIs: Widely popular, they use standard HTTP methods and are stateless, focusing on resources and their representations.
- SOAP APIs: Known for their strict standards and protocols, these APIs come with built-in security features and are ideal for enterprise-level services.
- GraphQL APIs: A newer approach that allows clients to request only the data they need, solving over-fetching and under-fetching problems.
Choosing the right style depends on factors like the project's complexity, user requirements, and how easily it integrates with existing systems. For instance, a small application might thrive with a simple RESTful approach, while a large-scale enterprise solution could benefit from SOAPâs robustness.
Modeling API Resources
Modeling API resources effectively is crucial. It ensures that your API is intuitive and mirrors the real-world entities it represents. Here, object-oriented principles come into play. Start by identifying the core resourcesâthese could be users, products, or any relevant entity in your domain.
Next, consider the relationships between these resources. For example:
- One-to-Many: A user can have multiple orders.
- Many-to-Many: A product can belong to multiple categories.
This thoughtful structuring bolsters clarity and usability, enriching the consumer's interaction with the API.
Example Resource Modeling
This code snippet illustrates how to design a user resource. Notice how it leverages a list to signify a one-to-many relationship, allowing a user to have several orders.
Defining Endpoints
Endpoints act as the access points that API consumers interact with. They should be designed with simplicity and predictability in mind. A clean endpoint structure significantly improves user experience. Consider the following best practices:
- Use Nouns instead of Verbs: Endpoints should represent data or resources.
- Stay Consistent: Maintain a uniform naming convention throughout the API.
- Utilize Versioning: This helps manage changes over time without breaking existing consumer integrations.
Example of Endpoint Design
- GET /api/users â retrieves a list of users
- POST /api/orders â creates a new order for a user
Good practices establish a solid foundation for an API, making it easier to scale or adapt to new requirements in the future.
Designing your API with careful thought pays dividends in maintainability and user satisfaction.
In summary, focusing on the right API style, effective resource modeling, and sensible endpoint definition can lead to an API that not only functions well but is also user-friendly. Ensuring these components are well thought out will enhance both the developer experience and user satisfaction.
Implementing the API
Implementing an API is the crux of putting your plans into action. This is where the rubber meets the road, and your designs start to take shape in a tangible, usable form. A well-structured implementation not only meets user requirements but also sets the foundation for a maintainable and scalable codebase. Thereâs a lot to consider during this phase: from setting up your development environment to handling incoming requests, each element contributes to a seamless user experience.
Setting Up the Development Environment
Getting your development environment right is the first step on the road to successful API implementation. Think of it as laying the groundwork before building a house. You wouldnât want to skip this important step, as a solid foundation is crucial for stability.
To set things up, you typically need a few tools: an IDE like IntelliJ or Eclipse, a version control system like Git, and a build tool such as Maven or Gradle. Here are a few pointers to get you started:
- Install Java Development Kit (JDK): Ensure you have the latest JDK installed. Itâs the backbone of Java development, and keeping it updated helps avoid common pitfalls.
- Choose Your IDE: Each Integrated Development Environment (IDE) has its quirks. IntelliJ IDEA is known for its robust features, while Eclipse is favored for its versatility. Choose one based on your comfort.
- Set Up Version Control: Use Git for version control. It not only keeps track of changes but also enables collaboration if you're working in a team.
A well-configured environment enables smoother coding and can save you a lot of headaches down the line, so don't rush through this step.
Writing the API Code
With your environment set up, itâs time to write some code. This is where clarity and structure become vital. A well-structured code can significantly ease the debugging process and future development iterations. Here are some key elements to keep in mind:
- Follow Conventions: Standardizing your code with proper naming conventions and organization will make your API more understandable. For instance, use clear, descriptive names for methods and classes.
- Utilize Frameworks: If youâre using Spring Boot, leverage its annotations. They can simplify your code and help with common tasks, such as defining routes and handling responses.
- Chunk Your Code: Break the code down into smaller, manageable functions. This approach not only boosts readability but also aids in testing individual components.
Hereâs an example of a simple REST endpoint in Java:
This snippet shows how easy it can be to set up a standardized API endpoint using Spring Boot, making your life that much easier.
Handling Requests and Responses
Once your API code is written, you must handle requests and responses adeptly. This is the part where your API communicates with the outside world. If done poorly, it can lead to frustrating user experiences.
Here are several points to consider:
- HTTP Methods: Use appropriate HTTP methodsâGET for data retrieval, POST for creating resources, PUT for updates, and DELETE for removals. Each method serves a purpose and enhances API clarity.
- Status Codes: Properly respond with HTTP status codes. For example, a 404 for not found or a 200 for successful requests can provide clear feedback to API consumers.
- Error Handling: Consider how your API will manage errors. Create a unified error response structure. This not only helps in debugging but gives consumers a consistent message format.
Remember, a well-implemented API does more than just functionality; it provides a smooth interaction experience for developers and users alike.
"In programming, as in life, the best designs often begin with a deep understanding of the essentials."
Nailing the implementation phase is all about attention to detail and keeping the user experience in mind at every step. From setting up the environment to writing coherent code and effectively handling requests and responses, each aspect is crucial in delivering an API thatâs both functional and user-friendly.
Testing the API
Testing is a cornerstone of API development, serving not just to validate functionality but also to establish confidence in stability and performance. When you throw an API into production, you want to know itâs as solid as a rock. Poorly tested APIs can lead to bugs, vulnerabilities, and ultimately, a bad user experience. This section outlines the types of testing you should consider, including unit testing, integration testing, and load testing. Each plays a crucial role in building resilient APIs that can withstand the rigors of actual use.
Unit Testing
Unit testing is all about ensuring that individual components of your API work as intended. Itâs the first line of defense against errors that can cascade into larger issues, compromising functionality. By testing smaller pieces of code in isolation, developers can catch bugs early, often before they become a thorn in the side of users.
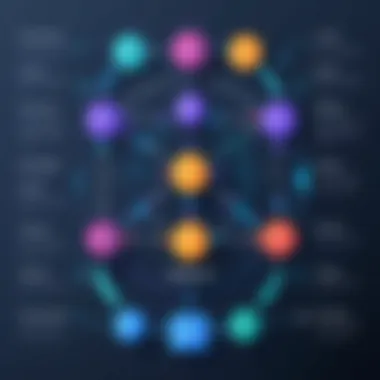
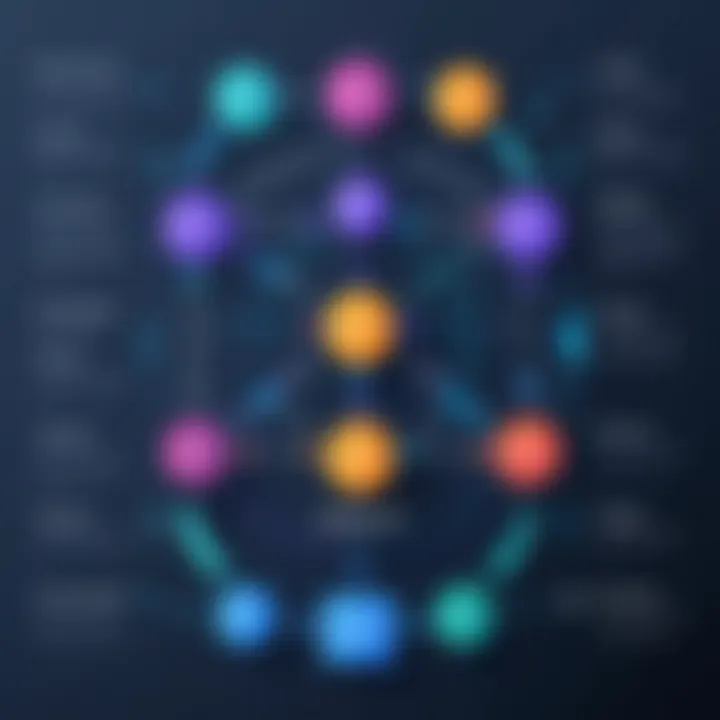
- Why Unit Testing Matters: Unit tests are quick and efficient. You can run them often, catching logical errors and incorrect assumptions made during coding.
- Tools: Java provides frameworks like JUnit and TestNG, which make writing and managing tests straightforward.
- Example: If you have a function that calculates the total price of items in a shopping cart, a unit test would check whether this calculation is correct across various scenarios: different numbers of items, zero items, and so on.
Integration Testing
While unit testing focuses on single units of code, integration testing takes it up a notch by examining how various parts of the system work together. Think of it as a dress rehearsal for your API. This type of testing ensures that the interactions between different modules are smooth and function as expected, especially in terms of data flow.
- Why Integration Testing Matters: Bugs can sometimes slip through the cracks when different components interact. Focusing on integration helps cross-check assumptions about how sub-systems interact with each other.
- Tools: Mockito can be used for mocking dependencies in integration tests, allowing for more controlled test environments.
- Example: If you're integrating a payment service with your API, you would test that the API correctly sends customer data and receives the right confirmation.
Load Testing
Load testing is where the rubber meets the road, so to speak. It simulates real-world traffic to determine how well your API handles it. The goal is to identify bottlenecks and understand how the API performs under stress. Itâs crucial for ensuring that an API can scale and respond promptly, even when usage spikes.
- Why Load Testing Matters: The last thing you want is for your API to crumble when several thousand users hit it all at onceâthink Black Friday sales.
- Tools: JMeter and Gatling are popular choices for conducting load tests. They can generate requests at varying levels to simulate different conditions.
- Example: Run a load test that sends thousands of requests to your API within a short time to see how it holds up. Check if the response time grows unmanageable or if it times out under load.
Proper testing is not just a phase in development; it's an integral process that can save time, resources, and user trust.
In summary, testing an API is an ongoing commitmentânot a one-time task. Itâs vital for fostering trust and ensuring that your application performs in real-life scenarios. By implementing unit, integration, and load tests effectively, you can make strides toward creating a reliable API.
Securing the API
In todayâs digital landscape, where sensitive data flows through APIs like water in a river, securing your API transcends mere best practice; itâs a necessity. APIs act as the gateways between different software components, and without proper security measures, these points of access can become vulnerabilities. Security breaches can cause anything from minor data leaks to catastrophic business failures. Therefore, understanding the key components and strategies for securing APIs is essential for anyone involved in software development.
Authentication Methods
Authentication refers to the process of verifying the identity of a user or system trying to access an API. There are various methods of authentication, each with distinct advantages and scenarios where they shine.
- Basic Authentication: This method relies on a simple idea: a username and password are sent with each request. While straightforward, it is vulnerable to sniffing if not paired with SSL/TLS for encryption, making it unsuitable for sensitive applications.
- Token-Based Authentication: This approach uses tokens instead of traditional credentials. Once a user logs in, they receive a token that they must send with subsequent requests. JSON Web Tokens (JWT) are a popular choice due to their compactness and nature of being self-contained.
- OAuth: OAuth is a more complex system typically used for granting third-party applications limited access to user accounts without exposing their credentials. For linking accounts across platforms (like logging into a site using a Google account), OAuth shines, though it requires proper implementation to prevent common pitfalls.
Choosing the right authentication method is essential. It's about finding the balance between security, complexity, and user experience. Sometimes, it's even beneficial to combine methods for an extra layer of protection, like using OAuth with token-based authentication.
Authorization Techniques
Once a user is authenticated, the next step is authorizationâdetermining what actions that user is allowed to perform. A robust authorization strategy is key to maintaining a secure system. Here are some common techniques:
- Role-Based Access Control (RBAC): This is a widely used method that restricts access based on the roles assigned to individual users. For instance, an admin might have access to all endpoints, while a regular user only has access to a specific subset. This model simplifies management and is straightforward to implement.
- Attribute-Based Access Control (ABAC): Unlike RBAC, which is role-centric, ABAC evaluates user attributes and environmental factors before granting access. This allows for more fine-grained control, as decisions can be based on various criteriaâlike time of the day, location, and user permissions. Complex but powerful, ABAC is gaining traction as organizations seek to tighten their security posture.
- Policy-Based Access Control: This method uses policies to decide access rights and is similar to ABAC but adds another layer by relying on rules that govern the conditions for access. It can be tailored to fit specific organizational needs, providing flexibility in addressing potential threats.
Establishing strong authorization is not just a matter of protecting data but also about ensuring compliance with regulations like GDPR or HIPAA, which mandate strict access controls.
Data Protection Strategies
Protecting the data flowing through APIs is paramount. Here are some integral strategies for safeguarding this valuable asset:
- Encryption: Transmitting sensitive information in plain text is like sending a postcardâanyone can read it. Using encryption protocols like TLS ensures that data remains confidential during transit. End-to-end encryption is another layer that keeps data secure even when it passes through multiple servers.
- Input Validation: This is about ensuring that the data sent to the API endpoints is valid and secure. Failure to validate inputs can lead to vulnerabilities such as SQL Injection or Cross-Site Scripting (XSS). Always sanitize and validate incoming data before processing it.
- Rate Limiting: To fend off Denial-of-Service (DoS) attacks, revenue loss, or resource depletion, implementing rate limiting helps control the number of requests a user can make in a given timeframe. This keeps the API accessible to legitimate users while warding off potential threats.
Utilizing these data protection strategies contributes significantly to an API's overall security framework. APIs should not only focus on controlling access but also on watching over the data they manage.
"Security is not a product, but a process." By prioritizing security at every stage of API developmentâfrom authentication methods to data protection strategiesâdevelopers can create safer interfaces that protect users and businesses alike. Each measure contributes to building trust and safeguarding relationships between consumers and service providers.
Documenting the API
Proper documentation is the backbone of any successful API. It serves multiple purposes, from facilitating efficient communication with consumers to promoting better understanding and usability. When developers are creating APIs in Java, they often focus on the code, losing sight of how critical documentation is. However, without clear and concise documentation, even the most well-crafted API can become as confusing as a maze without a map. The emphasis here is on the importance of meticulous documentation practices, which stem from several core elements.
Importance of API Documentation
The role of documentation cannot be overstated. A well-documented API offers several key benefits:
- Ease of Use: When developers interact with an API, they shouldn't have to sift through lines of code to understand how to use it. Comprehensive documentation provides them with the necessary guidance, understanding how to effectively utilize the API.
- Reduced Support Efforts: When documentation is clear, it lessens the number of inquiries from users. They can solve problems and troubleshoot using the information without always needing to reach out for help.
- Faster Onboarding: New developers can come up to speed more quickly when they have access to detailed documentation. A good reference can shorten the learning curve considerably, making teams more efficient.
- Maintenance and Updates: Documentation helps maintain the API code. If future developers understand how the existing API works, they're less prone to introducing errors during updates or changes.
Whether youâre developing an API for internal use or for external consumers, neglecting documentation is like building a bridge without ensuring itâs mapped out â it might get you halfway across, but youâre likely to encounter pitfalls along the way.
"Documentation is a love letter that you write to your future self."
â Damian Conway
Tools for API Documentation
Various tools can streamline the documentation process, making it easier to generate and maintain clear and effective references. Some popular options include:
- Swagger: An open-source tool that simplifies API documentation generation, Swagger allows developers to create interactive API documentation that can be easily accessed by users. It provides capabilities for both API design and documentation in a single package.
- Postman: While widely known for testing APIs, Postman also offers features for creating and publishing comprehensive documentation. Its user-friendly interface is particularly beneficial for developers who prefer a visual aspect to documentation.
- JavaDoc: This is the go-to option for documenting Java code. By adding comments in a specific format, developers can create HTML documentation directly from the codebase.
Integrating these tools into your workflow can enhance the quality and accessibility of your API documentation. The aim here is to make sure all relevant aspects are covered, allowing consumers of the API to not just use it but to master it.
Best Practices for API Development
In the world of software development, APIs serve as the backbone connecting various services and systems. For students and emerging developers, grasping the best practices for API development is crucial. It not only leads to a better user experience but also enhances maintainability and overall performance. To put it simply, a well-designed API stands the test of time in a rapidly evolving tech landscape.
Versioning the API
Versioning is a fundamental aspect in the API life-cycle. Imagine launching an API and needing to make a significant change. If you modify the existing API abruptly, it might break functionality for users depending on it. To prevent this, implement a versioning strategy.
- Semantic Versioning: This is a widely accepted approach which uses a format like Major.Minor.Patch. If there are breaking changes, increase the Major version. For new features that do not break existing functionality, bump up the Minor version. Bugs should be resolved by updating the Patch number.
- URI Versioning: A straightforward approach is to include the version in the URL path. For example, . This ensures clarity about which version clients are interacting with and is easily understandable.
By utilizing these strategies, you can manage changes and maintain smooth operations effectively.
Error Handling and Reporting
Handling errors gracefully is another vital practice. Poor error management can lead to bad experiences for users trying to interact with your API. A few key points to keep in mind:
- Consistent Error Responses: Each error should include a status code, an error message, and ideally a description of what went wrong. For instance, returning HTTP status 404 along with a message like "Resource not found" provides a clear indication of the issue.
- Logging Errors: Always log errors for further analysis. This helps in identifying patterns or recurring issues in your API, which is crucial for ongoing improvements.
- Client Guidance: Include hints or next steps in your error messages whenever possible. For example, when a required field is missing, specify what that field is.
Implementing robust error handling fosters trust. Users appreciate knowing that when something goes awry, there's clear communication.
Monitoring and Analytics
Monitoring your API is not just a luxury but a necessity. By keeping an eye on performance and usage metrics, you can identify potential bottlenecks and areas for enhancement.
- Performance Metrics: Regularly track key performance indicators such as response time, error rates, and throughput. Aim for low latency and high reliability.
- Dashboard Integration: Utilize API management tools to create dashboards that reflect real-time data. This visual representation can be invaluable for grasping how the API performs under load or during peak times.
- User Behavior Tracking: Understanding how consumers interact with your API can inform future feature developments. Tools like Google Analytics or custom-built monitoring solutions can help gather this data.
"Monitoring an API isnât just about keeping the lights on; it's about shining a spotlight on performance and ensuring a seamless user experience."
By embracing these best practices in versioning, error handling, and monitoring, you'll be paving the way for an API that stands robust against the test of time and adapts to future demands.
Deployment Strategies
Deployment strategies play a critical role in the lifecycle of an API. The way you choose to deploy your API can impact its performance, scalability, and even overall user satisfaction. When you consider that a well-deployed API often makes the difference between seamless user experiences and frustrating downtime, itâs clear that strategic deployment is not merely an afterthought but a foundational element of successful API development.
Moreover, the deployment of an API affects its accessibility and ease of use. The right strategy ensures that the API can handle everything from light traffic to heavy usage without breaking a sweat. This reliability is crucial, especially as users begin to rely on the API for their applications.
In this section, we shall dive into two fundamental aspects of deployment strategies: choosing the right hosting environment and implementing a CI/CD pipeline for your API deployment.
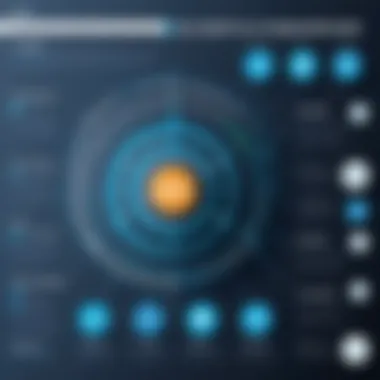
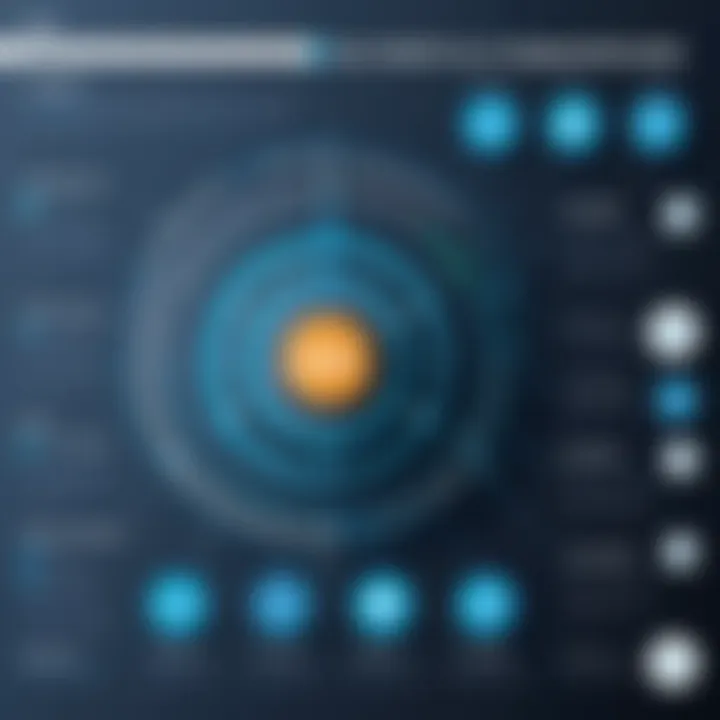
Choosing the Right Hosting Environment
Finding the right hosting environment is like picking the best stage for your band; it can make all the difference in how the audience receives the performance. The hosting choice affects both the API's initial deployment and its long-term maintainability.
Key factors to consider include:
- Performance: Your API should respond swiftly to requests. Look for hosting that minimizes latency, as slow responses can turn users away.
- Scalability: As your user base grows, so too should your APIâs capacity. Choosing a cloud provider that supports autoscaling can be a game changer.
- Reliability: Downtime is the enemy of any public-facing API. Evaluate the server's uptime guarantees and past performance metrics of potential providers.
- Security: Hosting environments vary in terms of security features. Ensure that your chosen environment can help safeguard your API against malicious attacks.
For example, cloud providers like AWS, Google Cloud, and Microsoft Azure offer diverse hosting options tailored for different needs. If you are looking at building something that might go big, these platform provide flexibility and power.
/ for API Deployment
Setting up a Continuous Integration/Continuous Deployment (CI/CD) pipeline for your API deployment can streamline the process from development to production. It automates the building, testing, and deployment phases, ensuring that changes to your codebase can transition smoothly into your live environment.
Implementing CI/CD embraces several benefits:
- Faster Turnaround Times: By automating testing and deployment, youâre not left waiting. Code is pushed to production quickly, allowing for rapid iteration.
- Reduced Errors: Automation helps catch issues early in the testing phase, reducing the likelihood of bugs moving into production.
- Increased Efficiency: With a well-oiled CI/CD machine, your development team can focus on writing code rather than spending excessive time on deployment chores.
A typical CI/CD pipeline for an API might look like this:
- Code Commit: Developers push code changes to a central repository.
- Build & Test: Automated tests are run to ensure code integrity.
- Deploy: After passing tests, the new version of the API is deployed to a staging environment for additional testing.
- Production Release: Finally, once verified, the API shifts to the live environment.
Handling API Consumers
When it comes to building APIs, the journey doesnât end once youâve successfully implemented the backend functionality and deployed your code. In fact, how you handle API consumers is a crucial part of the process that can determine the longevity and usability of your API. Understanding this segment ensures that developers not only create functional APIs but also ones that effectively serve and meet the needs of those who might depend on them.
Understanding Consumer Needs
To create APIs that genuinely resonate, one must take a deep dive into the desires and challenges faced by the end-users. API consumers can range from mobile app developers to front-end engineers, and even third-party services. Each can have specific requirements and expectations to fulfill their projects.
- Identify Audience: Knowing who your consumers are drives all subsequent decisions. For instance, if your API will support mobile apps, you might focus on minimizing latency or providing lightweight responses.
- Gather Feedback: Engaging with the consumer base through surveys or direct dialogue provides insight into what features they value or find cumbersome. It's beneficial to create a feedback loop where consumers can voice their opinions and suggestions for improvements.
- Analyze Usage Patterns: Tracking how your API is used can illuminate potential pain points. For example, if most users are consistently hitting a particular endpoint, it might indicate that feature is popular or, conversely, problematic.
By taking these steps, developers can construct APIs that are not only functional but also user-friendly, leading to better adoption rates and satisfaction.
Providing Support and Resources
Once your API is in the hands of consumers, the next critical aspect is ensuring they have all the support and tools necessary to use it effectively. Providing quality documentation, sample code, and platforms for discussion is essential to fostering a thriving ecosystem around your API.
- Documentation: This is the bread and butter of API usability. Comprehensive documentation should be clear and informative, explaining how to authenticate, request data, and handle errors effectively. It shouldnât just scratch the surface; it should include real-life examples and perhaps even a few troubleshooting tips. An API with poor documentation is likely to face issues, leading to frustrated users.
- Developer Community: Encouraging a community where developers can discuss potential issues, share solutions, or even showcase their projects built with your API can generate a supportive atmosphere. Platforms like Reddit can be useful to create discussion boards where consumers can find others with similar challenges.
- Sample Projects and Tutorials: Providing code samples that demonstrate common use cases can help consumers get started quickly. Consider crafting a few tutorials that guide them through making their first requests or integrating your API into their applications. This not only bolsters confidence in using your API but also highlights its versatility.
"Good documentation might be the difference between a thoroughly used API and one that languishes unnoticed in the developers' toolbox."
Navigating through the world of API consumers is a continual process of engagement and refinement. By understanding their needs and supporting them with resources, your API stands to become a reliable player in the broader software ecosystem.
Real-world Applications of Java APIs
Java APIs play a pivotal role in the software ecosystem today. They serve as the bridge between different software components, enabling them to communicate effectively. The significance of Java APIs can not be overstated, especially as businesses increasingly rely on software solutions for their processes.
The Importance of Java APIs
Real-world applications of Java APIs demonstrate their versatility and capacity to enhance productivity in various domains.
- Integration: APIs facilitate the integration of diverse systems, allowing organizations to merge their existing tools with new technologies. For instance, a retail business can integrate its inventory management system with its e-commerce platform using RESTful APIs. This seamless interaction can lead to better stock management, ultimately enhancing the customer experience.
- Scalability: The introduction of APIs allows firms to scale their services without significant overhauls. For instance, a startup may start with a simple Java API to serve a specific customer base. As it grows, it can expand its API to handle a larger influx of requests while maintaining efficient performance.
- Modularity: APIs promote modular design, enabling organizations to build applications where different components can evolve independently. When a new feature is necessary, developers can create a new API endpoint without disrupting the existing services.
Benefits of Java APIs
Adopting Java APIs in application development comes with distinct benefits:
- Cost-effectiveness: It cuts down development time and costs as teams leverage existing APIs rather than building new functionalities from scratch.
- Enhanced Collaboration: Multiple teams can work on different parts of an application simultaneously through APIs, promoting collaborative efforts.
- Security: Well-designed APIs come with built-in security protocols which can safeguard data during transmission and shield applications from unauthorized access.
- Quick Adaptation to Change: The tech landscape evolves swiftly. APIs allow businesses to quickly adopt new technologies and adjust to changing market demands without a complete redesign.
"APIs are the backbone of modern software development, driving innovation and efficiency."
Considerations for Implementing Java APIs
While there are numerous advantages, itâs essential to keep in mind some considerations:
- Documentation: Comprehensive documentation is crucial for both developers and API consumers. This guides users on how to utilize the API effectively.
- Versioning: As systems evolve, breaking changes may occur. Implementing a versioning strategy helps manage these transitions smoothly without disrupting existing users.
- Testing: Regular testing of APIs ensures reliability. Performance issues can be mitigated through proper testing practices.
In summary, real-world applications of Java APIs are indispensable across various industries. Whether itâs for integration, scalability, or security, they provide essential features that modern software solutions cannot do without. Understanding their implications in practical settings lays the groundwork for a deeper mastery of API development, setting the stage for the next crucial components in real-world scenarios.
Future Trends in API Development
The landscape of technology is a fast-moving river, and APIs are at the core of this ever-evolving environment. As the interplay of data, systems, and networks continues to grow, understanding the future trends in API development becomes indispensable for modern developers. APIs serve as the bridges between applications, allowing businesses to enhance connectivity and streamline operations. This section will illuminate various emerging trends and considerations that will shape the future of API development.
Emerging Technologies Impacting APIs
Several emerging technologies are recalibrating how APIs are designed, developed, and utilized. Here are a few key ones:
- Artificial Intelligence (AI): With AI's integration into systems, APIs are becoming smarter. Developers can harness AI-driven services to bring more intelligence into their applications, automating complex tasks and improving user experiences.
- Internet of Things (IoT): The billions of connected devices create a demand for APIs that can efficiently manage communication between these devices. APIs for IoT need to focus on low-latency communication and robust data handling capabilities.
- GraphQL: This query language for APIs is gaining significant traction. It addresses some of the limitations of RESTful APIs by allowing consumers to request only the data they need, resulting in more efficient data transactions.
"As APIs evolve, the focus will shift to enabling secure, real-time data exchanges across diverse applications, supporting the increasing complexity of software ecosystems."
By leveraging these technologies, developers can create APIs that are not only functional but also innovative, resulting in enhanced performance and user satisfaction.
The Role of APIs in Microservices Architecture
Microservices architecture, which advocates for building applications as a collection of loosely coupled services, relies heavily on APIs as the communication conduit. In this architectural style, each microservice is responsible for a specific task, and APIs facilitate interaction between them. Key considerations include:
- Decoupling Services: APIs provide a means to decouple services, meaning changes in one service won't affect others, as long as the API contract remains intact. This fosters easier updates and maintenance.
- Scalability: Microservices can be independently scaled based on demand, and APIs help manage these interactions efficiently. Developers can create robust APIs that can handle fluctuating traffic loads without compromising system performance.
- Faster Deployment: With APIs managing the interactions between services, organizations can push updates to individual microservices without redeploying the entire application. This agility aligns with rapid development cycles that many firms now embrace.
In summary, APIs stand as a cornerstone for microservices architecture, enhancing flexibility and scalability. As businesses continue to adopt microservices, the relevance of well-designed APIs becomes even more pronounced, shaping the future of software development.
Incorporating these future trends into API strategies will provide developers with a significant edge. As technology evolves, staying ahead of the curve means embracing these changes and considering how they can be implemented for better connectivity, efficiency, and user experience.
Culmination
Ending a journey into the world of API development in Java, itâs vital to recognize the factors that contribute to its success and efficiency. The creation of APIs isnât simply about writing code; it's about establishing connections and facilitating communication between disparate software solutions. In todayâs fast-paced technology landscape, APIs serve as the backbone, enabling applications to interact seamlessly, which fosters innovation and enhances user experience.
Recap of Key Concepts
Throughout this article, we have navigated through key elements essential for understanding and building robust APIs in Java. To encapsulate:
- API Definition and Importance: We unraveled what APIs are and why they are indispensable in modern software development. They allow different services to work together, making it necessary for businesses aiming for efficiency and scalability.
- Java Fundamentals: A dive into core Java concepts equipped readers with the necessary tools for API creation, stressing Object-Oriented Programming principles that serve as a foundation.
- Different API Types: We explored RESTful, SOAP, and GraphQL APIs, each with its unique structure and use-cases, providing a comprehensive overview that allows developers to choose based on project needs.
- Frameworks and Implementation: Tools like Spring Boot and Jakarta EE present frameworks that simplify API development. We walked through the stages of setting them up and writing the code.
- Testing and Securing APIs: Robust testing mechanisms ensure functionality, while security methods protect data integrity and privacyâtwo cornerstones of API validity in todayâs digital age.
- Documentation and Best Practices: Good documentation fosters collaboration and maintenance. Implementing best practices in error handling, versioning, and monitoring leads to better service delivery.
"APIs are the connective tissue that binds applications, platforms, and even entire ecosystems together."
Next Steps in API Development Journey
Having grasped the concepts, the next steps involve diving deeper into practical application and continuous learning:
- Hands-On Practice: Utilize platforms like GitHub to explore existing projects or contribute to open source APIs. The experience gained from real-world projects is invaluable.
- Stay Updated: The tech landscape evolves rapidly. Following resources like Reddit and engaging in forums will provide insights into emerging trends and technologies.
- Expand Knowledge: Consider exploring advanced topics such as microservices, serverless architecture, and machine learning integration with APIs. Further studies or certifications could be beneficial.
- Networking: Attend workshops, webinars, or conferences. Connecting with peers can provide new perspectives and enhance your learning experience.
- Build a Portfolio: Document your projects and findings to build a portfolio. This not only showcases your skills but also serves as a personal resource for referral in future projects.
In summary, the endeavor to master Java API creation is just the beginning. The journey continues as technology advances and new practices emerge. Stay curious and keep coding.
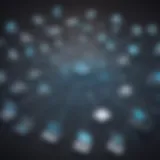
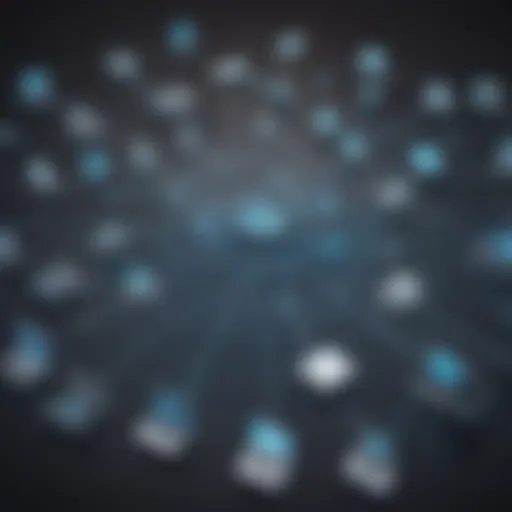