JavaFX Tutorial: A Comprehensive Guide for Developers
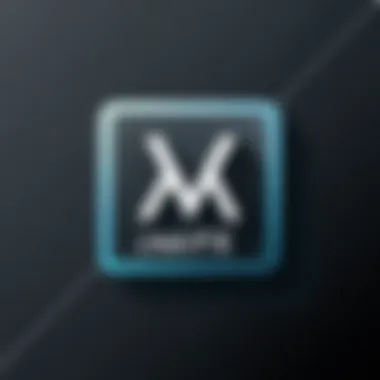
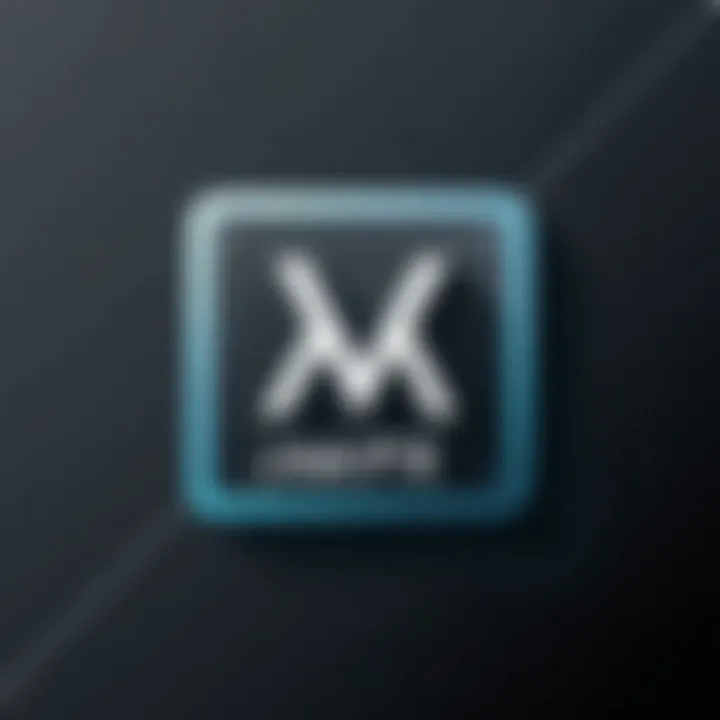
Prologue to Programming Language
JavaFX is a powerful framework designed for creating rich desktop applications in Java. Understanding its foundation requires a look into the programming language itself. Java, which is the backbone of JavaFX, has a robust history and a wide variety of features that make it essential in the world of software development.
History and Background
Java was launched by Sun Microsystems in 1995. It was intended to be a versatile language that could run on any device, leading to its slogan, "Write Once, Run Anywhere." The introduction of JavaFX in 2008 came as a response to the growing need for rich internet applications and graphical user interfaces in Java. JavaFX has evolved through various updates, aligning with the technological advancements in application development.
Features and Uses
JavaFX provides multiple features, including:
- Rich Graphics: JavaFX supports modern graphics programming features, including 2D and 3D graphics.
- CSS Styling: Similar to web applications, developers can use CSS to style JavaFX applications.
- FXML Support: This XML-based language allows developers to separate design from code, enhancing productivity.
- Responsive UI: JavaFX supports various screen sizes, making development for multiple devices easier.
It finds applications in various areas such as business applications, educational software, and complex data visualizations.
Popularity and Scope
Java continues to hold a strong position as one of the most popular programming languages. The demand for desktop applications has seen a resurgence, making JavaFX increasingly relevant. Many organizations rely on Java for their critical applications due to its stability and community support. JavaFXโs capability to deliver exceptional user experiences further solidifies its place in the realm of application development.
"JavaFX is more than just a framework; it provides an ecosystem for building modern applications with rich user interfaces."
As we delve deeper into JavaFX, understanding its syntax, concepts, and advanced features will pave the way for developing high-quality applications.
Intro to JavaFX
JavaFX is more than just a technology; it is an essential component in modern desktop application development using Java. As developers seek to create rich and interactive user interfaces, JavaFX emerges as a powerful tool that bridges the gap between performance and aesthetic appeal. This section serves to introduce JavaFX, highlighting its significance in the realm of application development.
Understanding JavaFX is crucial for those who wish to harness the full potential of Java in creating desktop applications. It offers flexibility, allowing developers to manage complex graphics and multimedia content with ease. Moreover, with a declarative approach using FXML and the backing of CSS for design, JavaFX encourages clean separation of logic and presentation. This aspect is particularly beneficial for collaborative projects where design and coding teams can work alongside each other.
The relevance of JavaFX today cannot be underestimated. In a landscape where user experience is paramount, JavaFX provides a robust platform that supports 2D and 3D graphics, media playback, and touch interactions. As applications evolve in their need for interactivity and responsiveness, JavaFX stands out as an ideal framework to meet these demands.
"JavaFX is a game changer for developers looking for modern, feature-rich UIs in Java applications."
As we delve deeper into the specifics of JavaFX, we will uncover its foundational elements, historical context, and core features that make it a compelling choice for developers at all levels. Understanding these facets will equip learners with the necessary tools to excel in their Java programming journey.
Setting Up JavaFX Environment
Setting up the JavaFX environment is a crucial first step in developing JavaFX applications. It provides the necessary tools and libraries that allow developers to create, test, and deploy desktop applications effectively. Ensuring that the environment is configured correctly can save significant time and effort. This section focuses on the requirements, installation procedures, and configuration options necessary to get started with JavaFX.
Requirements for JavaFX Development
To begin developing with JavaFX, specific requirements need to be met. First, a compatible version of Java is essential; JavaFX typically works best with Java Development Kit (JDK) 8 and above. It is advisable to download the latest version to make use of performance improvements and additional features.
You should also consider the operating system used as it may affect certain installation and runtime behaviors. JavaFX has support for Windows, macOS, and Linux.
Other essential elements include:
- An Integrated Development Environment (IDE) to facilitate coding. IDEs like IntelliJ IDEA or Eclipse are commonly used.
- JavaFX SDK, which contains libraries, documentation, and samples necessary for JavaFX development.
Meeting these requirements ensures a smooth development experience and reduces the likelihood of encountering issues during development.
Installing JavaFX SDK
Installing the JavaFX SDK is straightforward. The SDK must be downloaded from the official Oracle or OpenJFX websites. During installation, it is vital to pay attention to where the SDK is installed, as this path will be needed later for configuration.
Here are the steps for installation:
- Download the JavaFX SDK from the official site.
- Extract the downloaded archive to a suitable location on your filesystem.
- Verify the installation by checking that the libraries are available in the directory.
This process enables developers to leverage the full potential of JavaFX in their applications. Having the JavaFX SDK installed opens the door to building custom user interfaces efficiently.
Configuring IDE for JavaFX
Configuring the IDE is essential for integrating JavaFX properly. It ensures that your IDE recognizes JavaFX libraries and can handle your applications appropriately. Below are specific instructions for two popular IDEs: IntelliJ IDEA and Eclipse.
Using IntelliJ IDEA
IntelliJ IDEA provides a robust environment for Java development, and its integration with JavaFX is user-friendly. The IDE automatically recognizes JavaFX libraries once configured in the project settings. This feature minimizes setup time and allows for a more streamlined development process.
To configure IntelliJ IDEA for JavaFX development:
- Open IntelliJ IDEA and create a new Java project.
- Go to the Project Structure settings.
- Under Libraries, add the JavaFX SDK library path where you installed it.
- Set VM options to include the JavaFX modules with and parameters if needed during run configurations.
This seamless integration makes IntelliJ IDEA a popular choice among JavaFX developers due to its rich features and support.
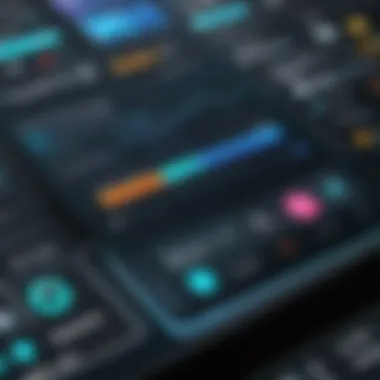
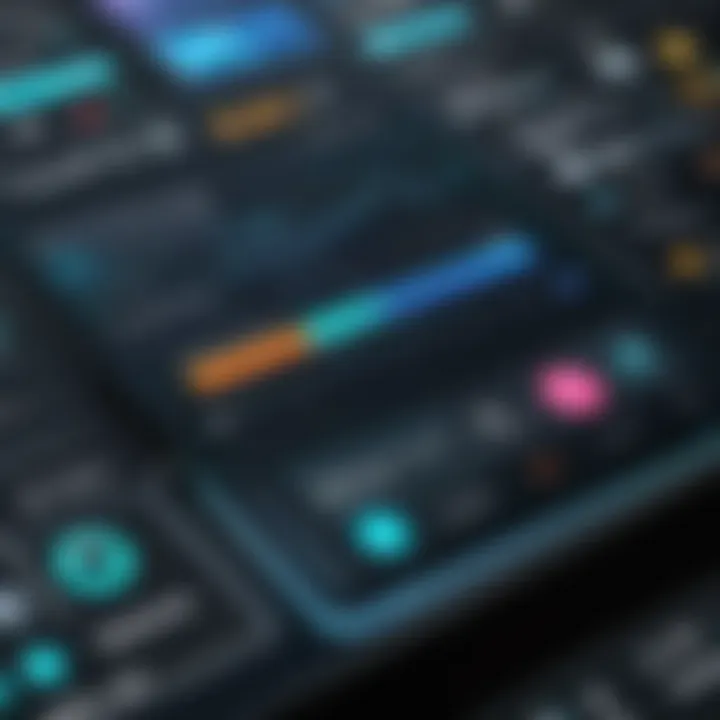
Using Eclipse
Eclipse is another well-known IDE in the Java community. Configuring Eclipse for JavaFX requires a few extra steps, but it is quite manageable. Eclipse supports JavaFX through the addition of necessary libraries manually.
To configure Eclipse:
- Open Eclipse and create a new Java project.
- Right-click on the project and select "Build Path" > "Configure Build Path".
- In the Libraries tab, add the JavaFX SDK as an external library.
- Next, you may need to add VM arguments in your run configuration to ensure JavaFX components work correctly.
Eclipse is favored for its customizable interface and project management capabilities, making it a solid option for developers working with JavaFX. Both IntelliJ IDEA and Eclipse have their unique strengths, offering developers choices based on their preferences.
"Choosing the right IDE for JavaFX development can significantly enhance your productivity and satisfaction throughout the development process."
In summary, having a correct and fully configured JavaFX environment is critical for successful application development. Properly meeting the requirements and configuring your IDE lays a foundation for building impressive JavaFX applications.
Getting Started with JavaFX
Getting started with JavaFX is crucial for understanding how to create rich desktop applications in Java. This section lays the foundational knowledge that will guide new developers through their initial steps in JavaFX programming. The importance of this topic lies not only in the hands-on application of coding but also in fostering a comprehensive understanding of user interface design and event handling.
By exploring how to create a simple application, you will develop the confidence needed to progress in building more complex projects. JavaFX provides a robust platform with various tools and features that make application development more accessible. Benefiting from a strong community, the support available often proves invaluable, making resources like forums and tutorials highly beneficial.
Creating Your First JavaFX Application
Taking the initial leap into any programming language can be intimidating. However, JavaFX offers a relatively smooth entry point. Start by setting up the JavaFX environment following previous sections. Once established, creating a basic application is straightforward. Here is a simple example of a JavaFX application:
This basic program creates a window displaying a button. When the button is clicked, a message is printed to the console. Running this code confirms a successful setup of your JavaFX environment and serves as a great starting point for further exploration.
Understanding the Application Lifecycle
A solid grasp of the application lifecycle in JavaFX enhances a developer's ability to manage resources effectively. JavaFX applications follow a specific lifecycle encompassing several stages: initialization, loading the user interface, handling user input, and closing the application.
- Initialization: The application begins its journey with the method. Developers often use this for pre-loading resources or setting up necessary configurations.
- Start Method: This is where developers define the user interface components. The method is called to set up the primary stage, making it visually accessible.
- Events: Here, event handling takes place. This phase is crucial, as it determines how the application responds to user interactions.
- Stop Method: Finally, the method allows for cleaning up resources when the application is closed. Attention to this phase ensures data safety and application stability.
Understanding these stages is key for developing efficient JavaFX applications. It allows for better planning and structuring of code and also aids in debugging processes, making it an essential aspect for anyone learning JavaFX.
JavaFX Main Class Structure
The main class structure in JavaFX holds significant importance. Every JavaFX application is essentially a subclass of the class. Here are the primary components you should understand:
- Main Method: This is the entry point of every JavaFX program. The command initializes the application.
- Application Class: By extending the class, developers inherit essential methods such as , , and , making lifecycle management straightforward.
- Stage and Scene: The represents the main window, while the defines the contents and layout within that stage. Mastery of these components is crucial as they form the backbone of the user interface.
The structure is straightforward and logical, allowing developers to build upon it easily. Studying and practicing this structure will equip learners with the tools they need to approach more advanced programming tasks in JavaFX.
JavaFX User Interface Components
The user interface components in JavaFX play a critical role in creating functional and engaging applications. They serve as the building blocks of the GUI, allowing developers to design interactive experiences for users. JavaFX offers a comprehensive set of UI controls and layouts, providing versatility and simplicity in designing applications. Proper understanding of these components ensures that one can create intuitive interfaces that facilitate user engagement and streamline interactions.
Layouts in JavaFX
Layouts in JavaFX manage the arrangement of UI elements. They dictate the structure and flow of components within a window, making it easier for users to navigate through an application's interface. Each layout type serves a specific purpose and helps in organizing content efficiently. Below are some key layouts used in JavaFX:
VBox
The VBox layout aligns children vertically in a single column. This characteristic makes it highly useful for forms and simple menus where elements need to be stacked. Its key advantage is straightforward vertical alignment, which simplifies the design and encourages an organized visual flow. Notably, VBox allows dynamic resizing based on the content, maintaining proportions and minimizing overflow issues. However, a potential disadvantage is that it can create a longer-than-usual page if too many components are added above each other.
HBox
In contrast to VBox, the HBox layout arranges components horizontally in a single row. This key feature makes it ideal for toolbar designs or when elements need to be presented side by side. The benefit of HBox is its ability to maximize space utilization along a single line, which can enhance the user experience. A unique attribute of HBox is its adjustable spacing between elements, which can tighten or loosen the layout as needed. However, this layout can become cluttered if numerous components are included, leading to a poor visual hierarchy.
BorderPane
The BorderPane layout fills the space in a structured manner by providing five distinct areas: top, bottom, left, right, and center. This makes it a very useful choice for applications requiring a flexible, organized layout. It allows developers to place components in specific zones, enhancing usability. One strength of BorderPane is its ability to adapt to window resizing without misplacing components. However, the requirement to manage these zones can complicate layout design if not done properly.
GridPane
The GridPane organizes UI elements in a grid structure composed of rows and columns. This characteristic offers incredible precision in placement, allowing for a compelling arrangement of controls. GridPane is especially beneficial for complex forms that require multiple components in a systematic order. The flexibility it provides in arranging controls can significantly enhance the user experience. However, layout management can become cumbersome with many components, possibly creating a need for extensive debugging and testing to ensure layout performance.
Common UI Controls
JavaFX provides a range of UI controls that facilitate interaction. Understanding these controls is paramount for any JavaFX developer. Below are some essential UI elements:
Buttons
Buttons are fundamental UI controls that trigger specific actions when clicked. They are vital for user interactions in any application. The distinctive aspect of buttons is their versatility; they can be labeled and customized easily, enhancing the overall appeal of the user interface. However, excessive use of buttons, especially without clear labeling, may lead to confusion in the application.
Labels
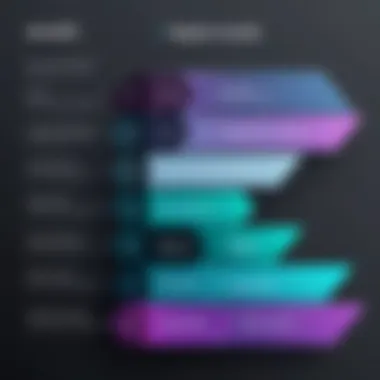
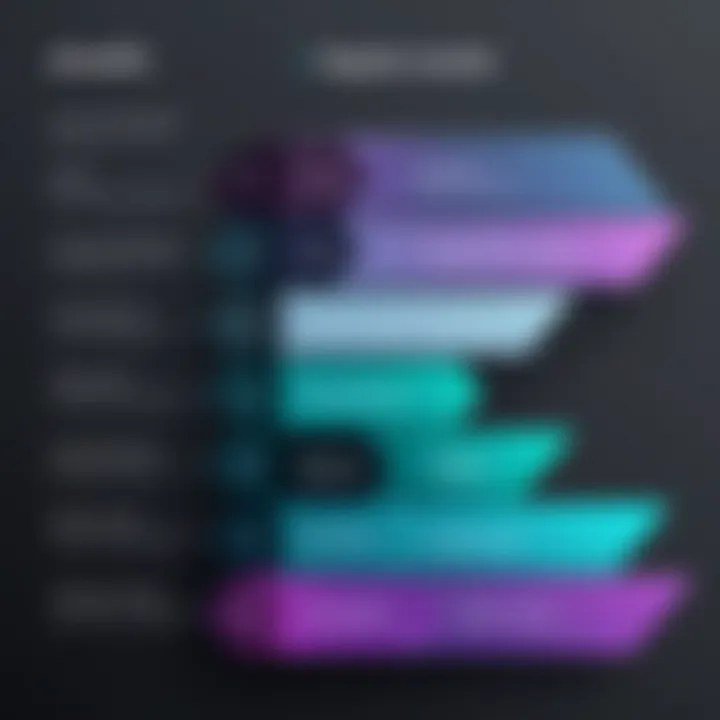
Labels serve the primary purpose of displaying text or graphics. They are significant in providing context and information to the user, helping to enhance usability. The key characteristic of labels is their simplicity; they are easy to implement and can effectively convey messages. However, if too much information is pushed into labels, it can overwhelm users rather than inform them adequately.
TextFields
TextFields are interactive controls that allow users to input text. They are essential for obtaining user data and form submissions. Their unique feature is real-time editing, which provides immediate feedback for user entries. While TextFields enhance interactivity, poor validation can lead to errors if user inputs are not handled correctly.
Lists and Tables
Lists and Tables are used for displaying collections of items in an organized manner. Lists provide a simple way to display a single column of items, while tables offer a more complex structure with rows and columns. The benefit of these controls lies in their capacity to handle large datasets efficiently. However, a potential downside is the need for extensive styling and configuration to present data meaningfully.
Styling JavaFX Applications
Styling is an important aspect of UI design. It helps create visually appealing applications that keep user attention. JavaFX supports various styling techniques, making it flexible for developers.
Using CSS
JavaFX allows developers to style applications using CSS. This feature is significant as it separates the styling from the application logic, enhancing maintainability. The flexibility of CSS enables developers to create attractive designs without complicating the codebase. However, learning and implementing CSS effectively requires additional effort, especially for those new to styling.
Applying Themes
The use of themes enables quick changes to the application's appearance. This characteristic is vital for maintaining a consistent look throughout the app. Applying themes can enhance user engagement and provide a coherent experience. Nevertheless, relying too much on themes may limit customizability for specific components, potentially leading to a generic design.
A well-structured user interface greatly enhances the overall user experience and defined functionality of any JavaFX application.
Event Handling in JavaFX
Event handling is a crucial aspect when one engages with JavaFX. It essentially allows applications to react to user input, thus providing a dynamic and interactive user experience. Understanding how event handling works will empower developers to create applications that respond seamlessly to user actions, be it clicking buttons, entering text, or navigating through menus. In this section, we will dive into how event handling operates in JavaFX, its significance, and best practices for implementation.
Understanding Event Handling
Event handling in JavaFX is a mechanism that captures user interactions and allows the developer to define responses accordingly. Each interaction, whether it's a key press or mouse click, generates an event that the application can listen for. This is pivotal in creating user-centric applications where feedback and results depend on user actions.
By organizing events in a systematic manner, JavaFX facilitates a responsive design that enhances user engagement and satisfaction. The event handling model in JavaFX is built around the observer pattern, allowing components to subscribe and respond to specific events. Some of the core concepts include:
- Event Sources: These are the components that generate events. For instance, buttons and text fields are typical sources of events.
- Event Types: Each type of interaction generates a specific event, such as ActionEvent for button clicks.
- Event Listeners: Listeners are classes that handle the events generated by the sources. They contain the logic to respond to events appropriately.
Creating Event Listeners
To facilitate event handling in JavaFX, developers create event listeners. Listeners are designed to respond to specific events. Implementing an event listener involves defining a class that implements the appropriate event handler interface. This is straightforward in JavaFX due to its well-structured API.
In this example, a button click generates an ActionEvent. The listener associated with this event prints a message to the console.
Common Event Types
JavaFX supports a diverse range of event types to cater to various user actions. Understanding these event types allows developers to tailor their applications to enhance user experience. Below is a list of some common event types:
- ActionEvent: Triggered by actions such as button clicks or menu selections.
- MouseEvent: Related to mouse actions like clicking, entering or exiting a component.
- KeyEvent: Generated from keyboard actions like pressing or releasing a key.
- ScrollEvent: Occurs during scrolling actions within a node.
JavaFX also provides the capability to handle events in a prioritized manner, which can be especially beneficial in complex applications with multiple components.
Understanding and effectively implementing event handling is fundamental for developing responsive JavaFX applications. Proper event management not only enhances the user experience but also contributes to the overall efficiency and performance of the application.
Advanced JavaFX Concepts
JavaFX is multifaceted and powerful. Understanding its advanced concepts can significantly enhance the development experience and end-user experience. This section will explore advanced functionalities, focusing on how they contribute to creating sophisticated applications. Knowledge of these concepts allows developers to leverage JavaFX to its fullest potential, making applications more responsive, maintainable, and feature-rich.
Working with FXML
Preamble to FXML
FXML is an XML-based markup language that allows developers to define the user interface separately from application logic. This separation of concerns is invaluable for developing complex applications. FXML promotes a clear structure. Using FXML makes it easier for designers to collaborate with developers, keeping UI design separate from application control code. This helps in organizing development workflow efficiently.
One key characteristic of FXML is its ability to reflect a visual layout. This offers a more intuitive way to manage the user interface, as it closely resembles how components are positioned in an application. Despite its benefits, some might find adapting to XML syntax slightly challenging, especially when transitioning from traditional Java coding styles.
FXML is not just a tool; it is a philosophy of design that enhances collaboration and maintainability in JavaFX projects.
Loading FXML Files
Loading FXML files is the process that enables JavaFX to render a UI defined in FXML. This method is crucial for integrating the XML-defined layout into a Java application seamlessly. The load method from the FXMLLoader class plays a major role here, fetching UI definitions and constructing the actual scene graph.
A critical feature of loading FXML is that it allows for dynamic changes in the UI. For instance, different FXML files can be loaded based on user interaction or application state, making applications highly adaptable. However, developers must be cautious with resource management, as improper loading can lead to memory leaks or performance degradation. Thus, a thorough understanding of resource handling is required when working with loading FXML.
Creating Custom Controls
Creating custom controls in JavaFX adds flexibility and uniqueness to applications. Custom controls allow developers to encapsulate functionality, enhancing modularity and reusability across multiple applications. Through extending base classes, developers can introduce new UI components tailored to specific needs.
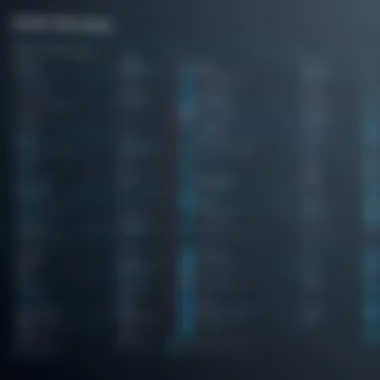
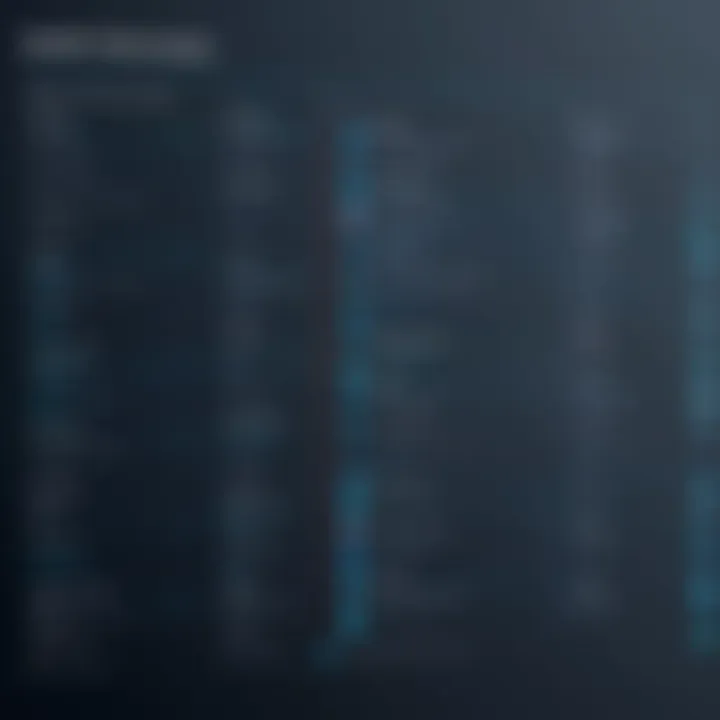
When designing custom controls, it is essential to adhere to JavaFX design principles to ensure seamless integration with existing components. This practice not only enhances the look and feel of applications but also promotes consistency across different areas of the UI. However, creating custom controls may require more effort and thorough testing, especially in ensuring compatibility with existing JavaFX components.
Multimedia Support in JavaFX
JavaFX supports a wide range of multimedia formats, providing developers with tools to integrate audio and video functionalities into applications easily. This support enriches user interactions and creates immersive experiences. Media, MediaPlayer, and MediaView are core classes that simplify handling multimedia content.
A significant advantage of multimedia support is that it opens doors for innovative application types in areas such as gaming, education, and entertainment. However, developers need to be aware of the licensing implications of the media formats they choose. They should also consider potential performance impacts, especially when dealing with high-resolution video or audio content. Ensuring a balance between quality and performance is vital for maintaining user engagement.
Understanding these advanced JavaFX concepts strengthens a developerโs ability to create complex, user-friendly applications. Utilizing FXML for separation of UI and logic, loading FXML for dynamic interfaces, crafting custom controls for personalized experiences, and incorporating multimedia support enables comprehensive development that meets modern user expectations.
JavaFX and Databases
Understanding how to integrate databases with JavaFX is vital for creating comprehensive desktop applications. JavaFX provides a rich set of user interface components, while databases serve as backend systems to manage data efficiently. This connection allows developers to build applications that not only present data attractively but also handle data operations seamlessly. In this section, we will explore how to connect JavaFX applications to databases and display the database data in the UI. The insights provided here will help learners grasp the fundamental techniques required for database integration.
Connecting to a Database
To begin, you need a database system. Commonly used databases include MySQL, PostgreSQL, and SQLite. Each of these offers its own benefits depending on the application context. For instance, SQLite is lightweight and great for simple applications, while MySQL is better for larger scale solutions. Once you have selected a database system, follow these steps to establish a connection:
- Add JDBC Driver: Ensure that you have the JDBC driver for your chosen database. This driver facilitates the connection between your JavaFX application and the database.
- Load Driver Class: Load the driver in your application using:
- Establish Connection: Use the to create a connection object. For example:
- Handle Exceptions: Always include exception handling to manage any issues during connection, such as .
This connection allows you to execute SQL queries and manipulate the database directly from your JavaFX application. Understanding this process is crucial as it establishes the foundation for all subsequent operations involving data manipulation.
Displaying Database Data in JavaFX
Once you have a connection to the database, the next step is to retrieve and display this data within your JavaFX application. Presenting data from the database in a user-friendly manner enhances the application's usability. Here are the steps to effectively display data:
- Execute SQL Query: Prepare a SQL statement to query the database. For example, fetching data from a table:
- Parse Results: Use the object to traverse the data. You can iterate through the results with:
- Update UI Components: Create UI elements such as or in JavaFX to display the data. For instance, using to show users:
This method ensures that the user interface reflects the data currently stored in your database. It is crucial for applications that rely on dynamic data changes, such as inventory systems or user management interfaces.
"Integrating JavaFX with databases elevates the capability of desktop applications significantly, making them data-driven and interactive."
Deploying JavaFX Applications
Deploying JavaFX applications is a critical phase in the software development lifecycle. This section will explore the fundamentals of deployment, outlining key aspects that developers must take into account. Understanding how to effectively package and distribute JavaFX applications is essential for delivering a reliable and user-friendly product. Whether for professional use, educational purposes, or personal projects, the deployment process ensures that users have a seamless experience when utilizing JavaFX applications. Therefore, having a robust deployment strategy is important for maintaining the integrity and performance of applications in varying environments.
Packaging JavaFX Applications
Packaging is the first step in the deployment process. It involves preparing your JavaFX application into a distributable format that users can easily install and run. This can include creating executable JAR files or native installers. The choice of packaging method often depends on the target platform. Here are some important considerations when packaging your JavaFX applications:
- Executable JAR File: This is one of the simplest ways to package a JavaFX application. It allows users to run the application directly with Java Runtime Environment installed. To create an executable JAR, developers must ensure the is correctly specified in the manifest file.
- Native Image: For enhanced performance and reduced memory overhead, consider using GraalVM to compile your JavaFX application into a native image. This method can lead to faster startup times and less resource consumption, making it a viable option for larger applications.
- Installers: Utilizing tools like Install4j or Launcj, developers can create installers for their applications. These installers can include setup wizards that guide users through the installation process, which adds a layer of professionalism to the final product.
Once packaging is complete, it is also beneficial to test it on different platforms to identify and rectify any potential issues users might face.
Distributing JavaFX Applications
Distribution is the next step after packaging. It concerns how the application reaches users, and various methods can be employed depending on the audience and requirements. Some common distribution methods are:
- Direct Downloads: Hosting your application on a personal website or a cloud storage solution allows for straightforward distribution. It's important to ensure that users have access to clear instructions on how to download and run the application.
- App Marketplaces: Publishing your application on platforms like Microsoft Store or Mac App Store can enhance visibility and credibility. Each store has specific requirements that must be fulfilled, including signing your application and adhering to platform guidelines.
- Version Control Systems: For educational or developmental distribution, using version control repositories like GitHub can be effective. You can share your project while also allowing for community collaboration and feedback.
"The key to a successful deployment is thorough testing and clear communication with users regarding the installation process."
Epilogue
JavaFX is much more than a framework. It is a rich platform that empowers developers to create compelling desktop applications in Java. Understanding its functionalities can enhance developers' ability to design user interfaces that are both aesthetically pleasing and responsive. Throughout this article, we explored various critical aspects that form the cornerstone of JavaFX development.
When considering its significance, we discussed how JavaFX stands out through its ease of use, versatility, and encapsulating features, which enable applications to leverage multimedia, seamless animations, and rich graphical elements. The capabilities reflected in its components expand beyond standard UI elements, allowing integration with other technologies, such as databases, ensuring that applications can perform efficiently.
Additionally, deploying JavaFX applications is simple yet powerful, allowing developers to engage a wide audience through various distribution methods. The knowledge gained here not only equips readers with the skills to work with JavaFX but also prepares them for real-world applications of Java, ultimately broadening their scope in software development.
Here are the summarized takeaways from this article:
Summary of Key Points
- JavaFX as a Framework: It provides an advanced means of creating graphical user interfaces in Java.
- Core Features: Understanding elements such as CSS styling, event handling, and FXML enhances application interactivity.
- Integration with Databases: Back-end functionality can be achieved through database connectivity, enriching applications further.
- Deployment Flexibility: Applications can be packaged and distributed across different platforms easily, reaching various users effectively.
Future Learning Paths
To continue building on what we have already covered, several key areas can be explored:
- Advanced UI Techniques: Learning more about advanced layout management and custom controls can improve user experience.
- Integration with Web Technologies: Exploring how JavaFX can work with web services or RESTful APIs can widen the scope of application features.
- Performance Optimization: Delving into best practices for optimizing JavaFX applications can enhance performance and loading times.
- Further Java Learning: As JavaFX relies heavily on Java, advancing oneโs knowledge in Java is crucial.
Engaging with the JavaFX community on platforms such as reddit.com can provide insights, updates, and shared experiences which can greatly benefit any learner's journey.