Comprehensive Insights into Java and Its Applications
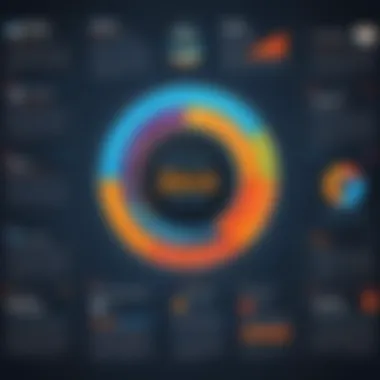
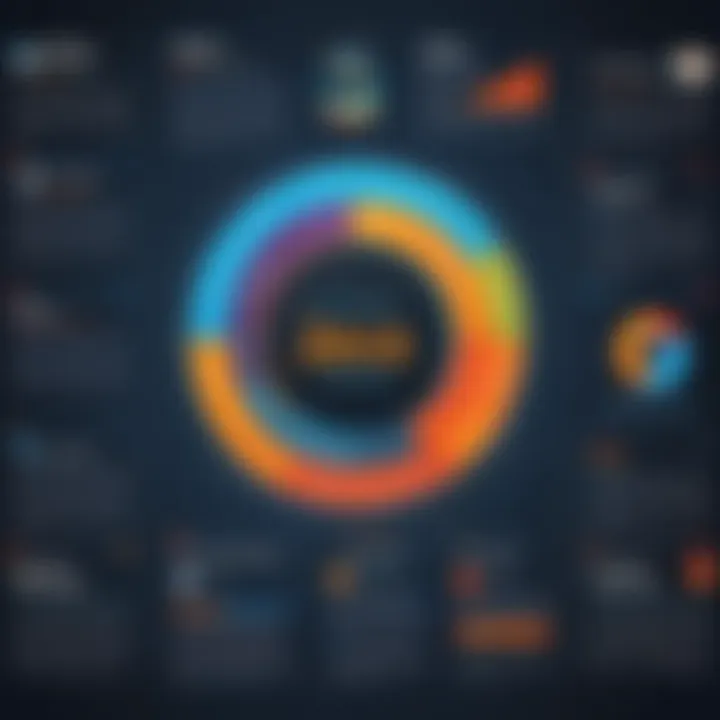
Intro to Programming Language
Java sits prominently among programming languages, widely recognized for its versatility and reliability. To fully appreciate Java, it is essential to explore the language’s origin, defining characteristics, and widespread use across various domains.
History and Background
Java was created in the mid-1990s by James Gosling and his team at Sun Microsystems. The goal was to develop a portable and platform-independent language that could operate seamlessly across different environments. Initially named Oak, it was eventually renamed Java to reflect a broader cultural appeal. After its release in 1995, Java quickly gained traction, largely due to its write-once, run-anywhere capability. This feature allowed programs to operate on any device that had the Java Virtual Machine installed, eliminating the barriers faced by developers working on distinct platforms.
Features and Uses
Java is characterized by several critical features:
- Object-Oriented: Java's design integrates the principles of object-oriented programming, fostering reusability and modular structure.
- Platform Independence: Thanks to the Java Virtual Machine, Java programs can run on various hardware without modification.
- Robust and Secure: Java includes automatic garbage collection and a strong security model, making it a reliable choice for enterprise applications.
Its applications are broad, found in web development, mobile applications, and server-side processing. For example, popular platforms like Android depend heavily on Java for mobile application development.
Popularity and Scope
Java consistently ranks among the top programming languages, a testament to its longevity and relevance. According to studies, many developers prefer Java for backend programming. Its rich ecosystem, supported by frameworks like Spring and Hibernate, further enhances its functionality, keeping it at the forefront of software development.
"The popularity of Java transcends industries and applications, cementing its place as a foundational language in programming education and practice."
Preface to Java
Java holds a significant place in the landscape of modern programming. It is not merely a programming language; it is a powerful ecosystem that allows developers to create robust applications across various platforms. This section serves as a gateway into understanding Java’s foundations, historical context, and its widespread appeal in the programming community. Exploring these elements provides new learners with a sense of direction and understanding of why Java remains relevant in today’s tech-driven world.
Historical Background
Java was created in the early 1990s by James Gosling and his team at Sun Microsystems. It was initially designed for interactive television, but it soon became clear that the language had broader applications. The first public release of Java was in 1995. Java introduced several revolutionary concepts, including portability, scalability, and built-in security features.
The motto “write once, run anywhere” encapsulates Java’s ability to run on any device that has the Java Virtual Machine (JVM) installed. This level of versatility propelled its adoption in various domains, including enterprise software, mobile applications, and web development. Consequently, Java grew from being a niche language to a foundational technology used by countless organizations around the globe.
Java's Popularity
Java’s popularity can be attributed to several key factors:
- Platform Independence: Java’s ability to run on multiple operating systems without requiring recompilation of the code is crucial for developers aiming for wide reach.
- Community Support: Since its inception, Java has cultivated a robust community. This community provides developers with resources, frameworks, and tools that enhance productivity.
- Strong Ecosystem: There are numerous libraries and frameworks available for Java, such as Spring and Hibernate, that simplify common development tasks and promote best practices.
The effectiveness of Java in large-scale enterprise environments has solidified its position. Many corporations rely on Java for backend development, utilizing its concurrency, security features, and extensive libraries. Moreover, platforms like Apache Hadoop and Apache Spark leverage Java to process large datasets, which further enhances its standing in the big data sector.
Java is not just a language; it is a platform that transforms how we think about and build software.
Understanding Java's Syntax
Java's syntax serves as the backbone for understanding how to write and interpret Java code effectively. This section is crucial because mastering syntax is not just about knowing how code looks; it directly impacts a programmer’s ability to create functional applications. With Java being a statically typed language, the importance of a well-structured syntax cannot be overstated. Proper syntax ensures that the code is not only readable but also error-free, facilitating the debugging and enhancement processes.
Basic Structure
The basic structure of a Java program is elegantly simplistic, yet it possesses a depth that allows for extensive functionality. Every Java application begins with a class definition. Here is a simple illustration:
In this example, the class named is defined, encompassing the main method, , which is the entry point of the program. This structure highlights the necessity of classes and methods in Java, reinforcing the object-oriented principles it upholds. The use of , , and other keywords dictate the accessibility and behavior of the methods and variables defined within the class.
Data Types and Variables
Data types in Java define what kind of data can be stored and manipulated within a program. Java is known for its extensive range of built-in data types, which include:
- Primitive Data Types: Such as , , , and .
- Object Data Types: Any class defined by the user.
Variables are containers for storing data values. Each variable must be declared with a specific data type, which ensures type safety. For instance:
Here, is an integer variable, and is a string variable. The explicit declaration of types helps in reducing errors during code execution, making this aspect fundamental to programming in Java.
Control Flow Statements
Control flow statements dictate the order in which statements are executed in a Java program. These statements allow a programmer to control the flow of execution based on certain conditions. The primary control flow structures in Java include:
- Conditional Statements: , , and statements. These are used to perform different actions based on varying conditions.
- Switch Statements: This offers a cleaner way to handle multiple conditions compared to chained statements.
- Loops: , , and loops facilitate repeated execution of a block of code.
For instance, a simple statement looks like this:
Implementing control flow statements is vital for creating dynamic applications that react to user inputs or other variables in real time. They enable the creation of more complex and functional applications.
Mastering Java's syntax will inevitably lead to greater fluency in writing effective and efficient code, which is essential for any programmer.
Object-Oriented Programming in Java
Object-oriented programming (OOP) is a crucial paradigm in Java programming. It offers several benefits, such as better modularity, code reusability, and easier debugging. Understanding OOP is fundamental for anyone looking to develop applications using Java since it aligns closely with how the language is structured. In this section, we will explore key concepts of OOP, specifically focusing on classes and objects, encapsulation, inheritance, and polymorphism.
Key Concepts
Classes and Objects
Classes and objects form the backbone of OOP in Java. A class is essentially a blueprint or a template from which objects are created. The primary aspect of classes and objects is their ability to encapsulate data and behavior.
A key characteristic of classes and objects is their organization of data. They allow the representation of real-world entities in a program. This organization leads to a more intuitive and manageable code structure, which is beneficial for developers. Objects represent instances of classes, bringing functionality and state together.
The unique feature of this relationship is that it promotes code reuse. Instead of rewriting code, developers can create new classes based on existing ones. However, while classes and objects make code easier to manage, they may lead to complexity if not structured properly.
Encapsulation
Encapsulation is another fundamental concept in OOP. It refers to the bundling of data and methods that operate on that data within a single unit. This ensures that internal implementation is hidden from the outside. A key characteristic of encapsulation is access control. By using access modifiers (like private and public), developers can control how the data is accessed.
Encapsulation is a popular choice because it enhances security. It prevents unauthorized access and modification of the internal state of an object. However, it could lead to verbosity in code since getters and setters need to be defined, which some may find cumbersome.
Inheritance
Inheritance allows new classes to inherit the properties and methods of existing classes. This promotes a hierarchical relationship between classes. The primary aspect of inheritance is code reusability. Developers can create a new class based on a parent class, reducing redundancy.
A significant characteristic of inheritance is the ease of extending functionalities. By adding new features to the child classes, developers can modify behavior without altering the parent class. This flexibility makes inheritance a beneficial aspect of Java programming. Nonetheless, excessive use of inheritance can create complex dependencies, making the code harder to follow.
Polymorphism
Polymorphism enables one interface to be used for a general class of actions. The exact action is determined at runtime based on the object that it is acting upon. One of the key aspects of polymorphism is method overriding and overloading.
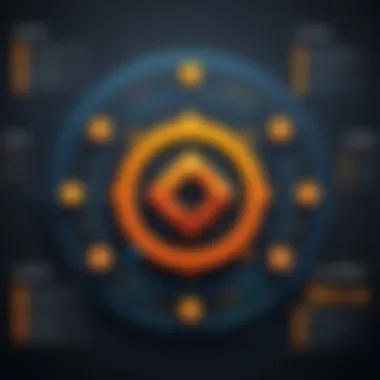
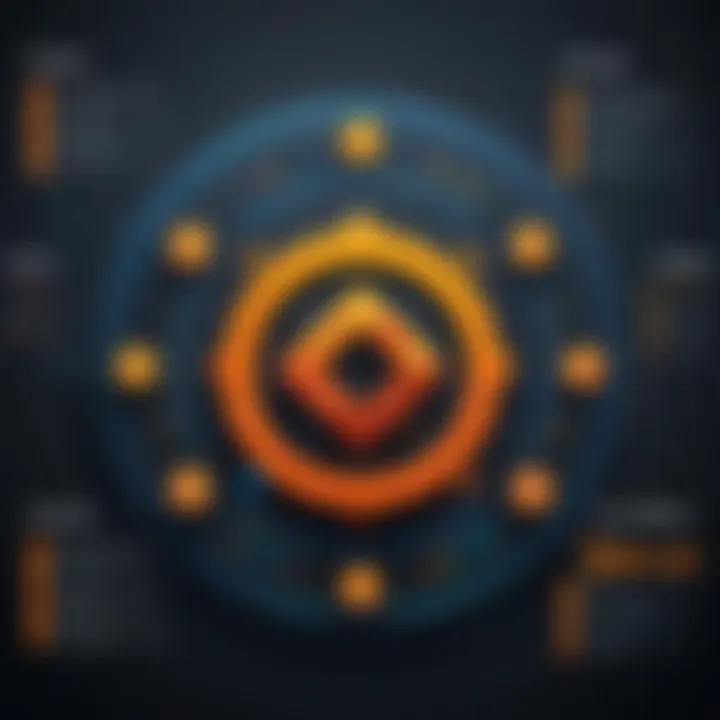
This characteristic contributes to more flexible code design. Through polymorphism, developers can perform the same operation on different classes. This not only makes the code cleaner but also promotes the implementation of design patterns effectively. However, the dynamic nature of polymorphism might add some overhead in terms of performance, which developers must keep in mind.
Java Interfaces
Java Interfaces define a contract for classes. They contain abstract methods that must be implemented by any class that agrees to the contract. The importance of java interfaces lies in their ability to support multiple inheritance, an aspect Java does not allow with classes. This promotes a clear separation between the definition of functions and their implementations, enhancing code flexibility and maintainability.
Java Development Environment
The Java Development Environment is crucial for anyone looking to enhance their programming skills in Java. It encompasses various tools and settings that facilitate code writing, debugging, and execution. Developers must set up a conducive environment that allows them to focus on coding rather than technical issues related to setting up the platform itself. A well-configured Java Development Environment can significantly improve productivity and streamline the coding process.
Setting Up Java Development Kit (JDK)
To start programming in Java, the very first step is to install the Java Development Kit (JDK). The JDK includes essential tools and libraries necessary for Java programming. It provides the Java Runtime Environment (JRE), which allows you to run Java applications, and includes development tools like the Java compiler and the Java debugger.
The installation of the JDK varies by operating system. After downloading the suitable version for your OS, you can proceed with the installation. It is important to configure the environment variables properly to allow for seamless execution of Java commands in the command-line interface.
Setting up the JDK is not just about running programs; it sets the foundation for your development activities and ensures that you have access to the latest features and updates.
Integrated Development Environments (IDEs)
IDEs offer powerful platforms for Java development, providing an integrated environment for coding, debugging, and testing. Each IDE may offer unique features that cater to various user needs. Here are three popular Java IDEs that stand out in the community.
Eclipse
Eclipse is a widely used IDE for Java development. Its modular architecture and extensive plugin system allow for customization and flexibility. One key characteristic of Eclipse is its Source Control Integration, which facilitates version control to manage code revisions effectively.
Eclipse is known for its user-friendly interface, which can help newcomers navigate the Java programming landscape more easily. A unique feature of Eclipse is its refactoring capabilities, allowing developers to restructure existing code without changing its external behavior.
However, Eclipse can sometimes be resource-intensive, which might slow down computers with limited RAM.
IntelliJ IDEA
IntelliJ IDEA is praised for its intelligent code completion and advanced navigation features. One of its key characteristics is the built-in support for code analysis, which identifies potential issues even as the code is being written. This feature contributes significantly to coding efficiency and robustness.
IntelliJ IDEA is considered a popular choice due to its ergonomic design and ease of use. The unique feature of the IDE is its integration with various build systems and frameworks, making it versatile for different projects. Nevertheless, the community edition has some limitations compared to the paid version.
NetBeans
NetBeans stands out for its simplicity and ease of use, making it a good choice for beginners. One of its key characteristics is that it is open-source, which encourages community contributions and support.
A unique feature of NetBeans is its built-in support for multiple programming languages beyond Java, allowing for versatility in projects. It includes features such as code templates and easy database integration, which can be beneficial for web development. However, some users report that it may lack the advanced features of Eclipse and IntelliJ IDEA, particularly for large-scale projects.
In summary, the Java Development Environment is essential for effective programming. Tools such as JDK and various IDEs, each with their unique advantages, allow developers to focus on writing quality code efficiently. Choosing the right tools will depend on individual needs and project requirements.
Error Handling in Java
Error handling is a critical aspect of Java programming. It enables developers to write more robust and reliable applications. When software fails, it is necessary to manage errors gracefully. Proper error handling ensures that the program can respond effectively without crashing. Faulty code can lead to unexpected behaviors. Therefore, implementing a solid error handling strategy is essential. This section focuses on various methods of error handling in Java, emphasizing their unique characteristics and benefits.
Exception Handling
Exception handling is the process by which Java reacts to runtime errors. This mechanism allows a program to catch and handle errors that occur during execution without terminating unexpectedly. It plays a vital role in making Java applications reliable and user-friendly. The key components of exception handling include try-catch blocks, throwing exceptions, and creating custom exceptions. Understanding how these elements work together is essential for effective programming.
Try-Catch Blocks
Try-catch blocks serve as the primary structure for handling exceptions in Java. They allow developers to define specific code sections where exceptions might occur. When an error is caught, the corresponding catch block executes. This isolation of error handling from the main code flow keeps applications stable.
Key characteristics of try-catch blocks include:
- They prevent abrupt termination of a program during runtime.
- They promote cleaner code by separating regular logic from error management.
A significant benefit of using try-catch blocks is their simplicity. Developers can focus on the main logic while defining how to respond to exceptions. However, excessive use of try-catch can lead to confusion, so it's vital to apply them judiciously.
Throwing Exceptions
Throwing exceptions is another core mechanism in Java error handling. This allows a method to signal that an unexpected situation has occurred. When an exception is thrown, the normal flow of the program is interrupted, and control is transferred to the nearest catch block.
A notable characteristic of throwing exceptions is its contribution to clearer code. Developers can specify error conditions directly, enabling better debugging. Its benefits include:
- Promotes clarity by explicitly signaling problems.
- Encourages developers to check for and handle potential issues.
Nonetheless, excessive throwing of exceptions can lead to performance issues. Java handles exceptions differently than standard control flow, which may incur overhead. Thus, it should be used correctly to avoid inefficiency.
Creating Custom Exceptions
Creating custom exceptions allows developers to define their unique error conditions tailored to specific application needs. This enhances the granularity of error handling. By using custom exceptions, developers can provide meaningful context about the error.
Key features of custom exceptions are their flexibility and specificity. They can be designed to capture any particular error and provide detailed messages relevant to the application. The advantages of using custom exceptions include:
- Increased clarity in error handling specific to the application.
- Better control over exception management strategies.
However, custom exceptions can introduce complexity. If misused, they can lead to code that is harder to maintain. Therefore, custom exceptions should be reserved for errors that cannot be managed adequately with standard exceptions.
"Error handling is not just about preventing mistakes; it is about creating resilience in a program."
Java Libraries and Frameworks
Java libraries and frameworks play a crucial role in software development. They provide pre-written code that developers can use to perform common tasks, thus saving time and effort. Understanding these libraries and frameworks can significantly enhance productivity and code quality. They help in maintaining consistency within projects and allow developers to focus on functionality rather than writing repetitive code.
Standard Libraries
Java's standard libraries are a collection of classes and interfaces that provide essential functionalities for application development. They offer utilities for data structures, networking, and input/output operations. The Java Standard Library streamlines many basic programming tasks, allowing developers to utilize existing solutions rather than creating new ones from scratch. As a result, this reduces potential errors and increases the reliability of the software.
Some commonly used standard libraries include:
- Java Collections Framework: for handling groups of objects.
- Java I/O: for input and output operations.
- Java Networking: for network communications.
These libraries not only make coding easier but also promote best practices in Java development.
Popular Frameworks
Frameworks in Java help create applications more efficiently. They provide a structured way to build software, allowing developers to follow specific patterns and principles. Here are three of the most prominent frameworks in the Java ecosystem:
Spring
Spring framework is widely recognized for its versatility and simplicity. It allows for the development of enterprise-level applications seamlessly. One of its key characteristics is dependency injection, which promotes loose coupling between application components.
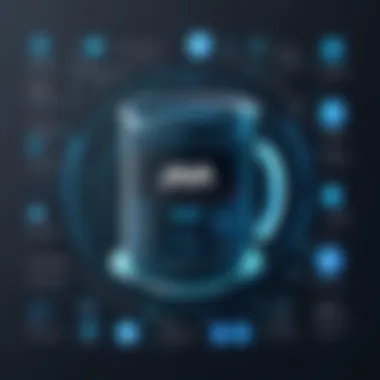
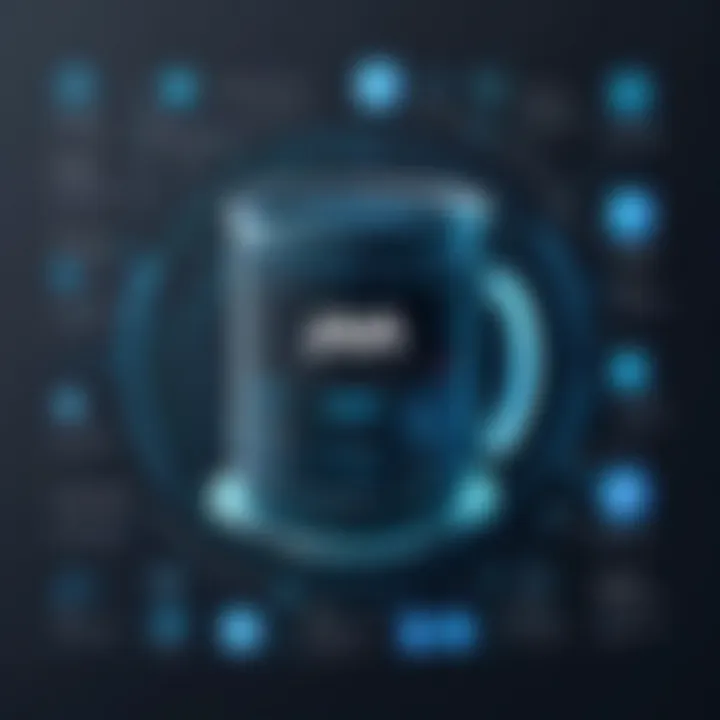
This feature enhances testability and maintainability. It is a beneficial choice because it integrates well with various Java technologies. However, Spring can be complex for beginners due to its extensive features and configuration options.
Hibernate
Hibernate is an ORM (Object-Relational Mapping) framework that simplifies database interactions. It maps Java classes to database tables, allowing developers to work with objects instead of SQL queries. This key characteristic makes Hibernate a popular choice among developers who aim for clean and maintainable code.
Its unique feature includes automatic schema generation that saves time during database creation. Nevertheless, new users might struggle with its learning curve, especially when it comes to understanding its configuration and caching mechanisms.
JavaFX
JavaFX is a framework for building rich internet applications. It allows developers to create visually appealing interfaces with ease. The key characteristic of JavaFX is its ability to support hardware-accelerated graphics, which ensures better performance for graphical applications.
Additionally, JavaFX provides a unified API for different platforms, making it cross-platform compatible, which is essential for modern application development. However, some developers find that it lacks community support compared to older frameworks like Swing.
In summary, understanding Java libraries and frameworks is vital for efficient software development. They not only provide essential tools and functionalities but also ensure best practices are adhered to within the Java programming environment.
Java for Web Development
Web development has become a critical area in software engineering, with increasing demands for robust server-side applications. Java plays a prominent role in this field. This language's capability to create dynamic, interactive web applications makes it a preferred choice among developers. Java's mature ecosystem, paired with its reliability and scalability, positions it effectively for both enterprise-level and small-scale web solutions.
One of the significant benefits of using Java for web applications is its platform independence. Programs written in Java can run on any device that has the Java Virtual Machine (JVM) installed. This characteristic offers flexibility when deploying applications across various platforms.
Before diving into specific technologies, it is essential to recognize several core considerations when using Java for web development:
- Security: Java provides built-in security features that help to protect applications from threats.
- Performance: The efficiency of Java’s Just-In-Time compiler enhances performance, making it suitable for web applications with heavy user loads.
- Community and Support: Java's extensive community means developers can access a wealth of resources, libraries, and support.
Servlets and JSP
Servlets and JavaServer Pages (JSP) are foundational technologies in Java web development. A servlet is a Java class that handles requests and returns responses. It acts as a middle layer between the web server and the client, processing incoming requests, performing logic, and generating dynamic content.
JSP, on the other hand, simplifies the creation of dynamic web pages using HTML and Java code. This combination allows for more straightforward integration of user interface elements with server-side processing.
The collaboration between servlets and JSP typically follows this flow: the client sends a request to a servlet, which may interact with a database or perform business logic. It then forwards the request to a JSP, which renders the final result as an HTML page. This architectural pattern is known as Model-View-Controller (MVC).
Benefits of using Servlets and JSP include:
- Reusability: Code can be written in reusable components, minimizing duplication.
- Separation of Concerns: JSP separates presentation logic from business logic, making maintenance easier.
JavaServer Faces
JavaServer Faces (JSF) is a framework that simplifies the development of user interfaces for Java web applications. It provides a set of reusable UI components, along with a standard way to build and manage them.
JSF follows the MVC architectural pattern. It allows developers to create user interfaces by assembling components from a library rather than writing HTML code directly. This abstraction layer makes it easier to build complex web applications because it manages UI state, navigation, and event handling.
Key features of JSF include:
- Component-Based Approach: Reusable UI components reduce the effort needed for building interfaces.
- Event-Driven Programming: Developers can define actions associated with events, promoting a more dynamic user experience.
- Integration with Other Technologies: JSF seamlessly integrates with various Java frameworks, making it adaptable to other technology stacks.
In essence, JavaServer Faces streamlines the creation of robust user interfaces, enhancing productivity for developers working on modern web applications.
By leveraging servlets, JSP, and JSF, Java provides a comprehensive toolkit for web development. The technologies outlined here exemplify Java's versatility, showcasing its capability to create scalable, secure, and maintainable web applications.
Concurrency in Java
Concurrency is a crucial concept in Java programming, allowing multiple tasks to execute simultaneously within a single program. This ability enhances efficiency and responsiveness, making it invaluable in today’s fast-paced computing environments. With the rise of multi-core processors, the effective use of concurrency can significantly boost application performance. Understanding the elements and benefits of concurrency aids developers in building robust and scalable applications.
Threads in Java
In Java, a thread is the smallest unit of processing that can be scheduled by the operating system. Java provides built-in support for multithreading, allowing developers to create threads easily. Each thread runs independently but shares the same memory space, which can lead to both performance boosts and synchronization challenges.
You can create a thread in Java by extending the class or implementing the interface. Here is a simple example of creating a thread:
This code defines a basic thread that prints a message when executed. The method is crucial, as it initiates the thread separately from the main program execution. Utilizing threads allows for better utilization of CPU time, particularly in I/O-bound applications.
Synchronization
Synchronization is essential in multi-threaded programming to prevent thread interference and memory consistency errors. When multiple threads access shared resources, it is vital to ensure that these resources are accessed in a controlled manner. Lack of synchronization can result in unexpected behaviors, such as data corruption.
Java provides several mechanisms for synchronization, including the keyword and locks:
- Synchronized Methods: This ensures that only one thread can execute a method at a given time for a particular object.
- Synchronized Blocks: More granular control can be achieved by defining specific sections of code that need synchronization.
Here is an example of a synchronized method:
In this code, the method ensures that only one thread at a time can increment the count variable, thus preserving data integrity.
Concurrency Utilities
Java also offers a set of concurrency utilities in the package, which simplifies managing multiple threads. These tools provide higher-level abstractions for common concurrency tasks, such as thread pooling and communication between threads. Some important utilities include:
- Executor Framework: This simplifies the management of thread pools, allowing for efficient task execution without manually handling thread creation.
- Locks: Java provides explicit lock mechanisms, enabling more control over synchronization when needed.
- CountDownLatch: Useful for coordinating multiple threads, allowing one or more threads to wait until a set of operations is completed.
Utilizing these concurrency utilities can greatly enhance the responsiveness and performance of Java applications.
"Concurrency is not a question of whether you should use it, but how you can effectively manage it."
Employing concurrency in Java strategically enhances not only application performance but also improves the user experience by ensuring swift data processing and responsiveness.
Java Testing Techniques
Java testing techniques are essential for ensuring the quality and reliability of Java applications. These techniques help developers identify bugs and errors before the software is deployed, which ultimately saves time and resources. A solid testing approach is crucial as it boosts confidence in the code, making it maintainable and scalable over time. There are various testing methods available in Java, each having its own specific use cases and methodologies.
Unit Testing with JUnit
Unit testing is one of the most critical practices in software development. JUnit is a widely used framework for unit testing in Java. It allows developers to write and run repeatable tests. The main purpose of unit testing is to verify that each unit of the software performs as expected. This practice aids in catching errors early in the development cycle, reducing the cost of fixing bugs later.
To get started with JUnit, you first need to include the JUnit library in your project. This can be done using build tools such as Maven or Gradle. Here’s a simple example of a basic unit test using JUnit:
This code demonstrates how a unit test verifies that the addition method of a class returns the expected outcome. In this case, it checks if the sum of 2 and 3 equals 5. The use of assertions here is crucial, as it defines the expected behavior that must be confirmed.
Test Automation Frameworks
Test automation frameworks provide a structured way to automate the testing process. They enhance the efficiency of running tests, especially for large applications that require extensive testing. Automation enables continuous integration and continuous delivery (CI/CD), which are vital practices in modern software development.
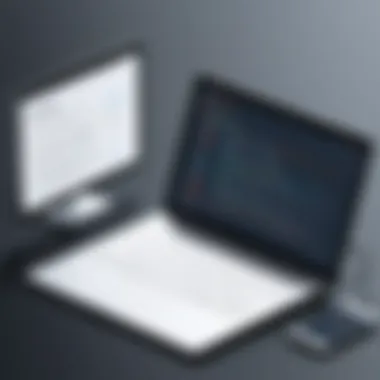
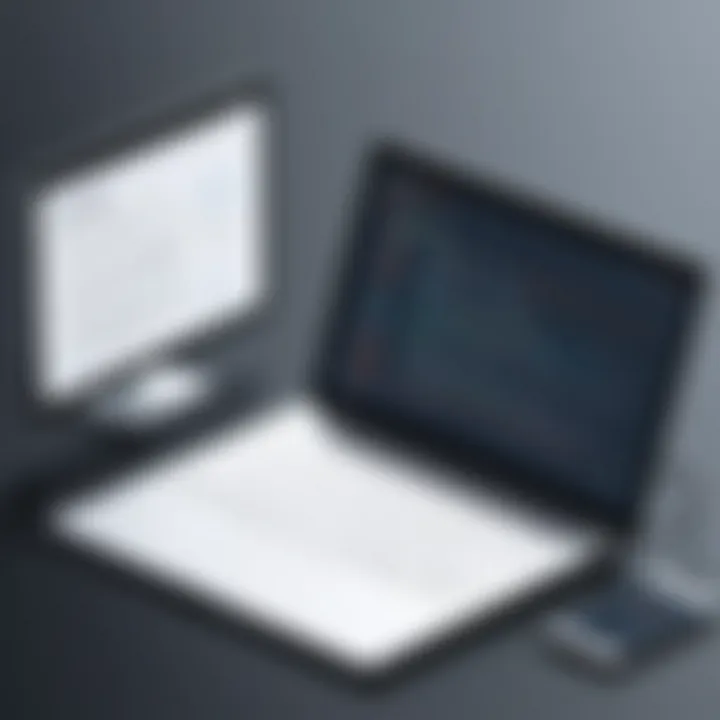
Several popular test automation frameworks exist in the Java ecosystem. Here are a few notable ones:
- Selenium: Primarily used for testing web applications, Selenium supports various browsers and operating systems.
- TestNG: This testing framework is inspired by JUnit and offers more advanced features such as parallel execution and data-driven testing.
- Cucumber: Known for behavior-driven development (BDD), Cucumber allows testers to write scenarios in plain language, making it easier for non-technical stakeholders to understand.
Using these frameworks can significantly reduce manual testing efforts. By automating repetitive test cases, teams can focus their efforts on more complex testing scenarios. This not only saves time but also enhances the overall quality of the software produced.
Java Performance Optimization
Java performance optimization is a crucial topic for developers aiming to create efficient and responsive applications. As Java applications scale in complexity and usage, optimizing performance becomes vital. Focusing on specific elements such as memory management and profiling tools can significantly improve an application's efficiency. The benefits of optimizing Java performance include reduced latency, enhanced user experience, and cost effectiveness in resource utilization. Understanding the considerations surrounding performance optimization allows developers to make informed choices during the development and deployment processes.
Memory Management
Memory management is essential in Java as it influences the application's performance directly. Java uses an automatic garbage collection mechanism to manage memory allocation and release. This process helps in automatically reclaiming memory used by objects that are no longer needed. However, it is important to understand how memory works in Java to avoid performance issues.
- Garbage Collection: Java's garbage collector runs periodically to free up memory. However, developers can optimize this process by minimizing the creation of unnecessary objects.
- Heap Management: The Java heap is where Java objects reside. Tuning heap sizes according to application needs can improve performance. Setting the right (maximum heap size) and (initial heap size) options can help in managing memory more effectively.
- Memory Leaks: Ensuring that objects are dereferenced when no longer in use can prevent memory leaks that lead to increased memory consumption and eventually affect application stability.
Profiling and Monitoring Tools
Profiling and monitoring tools assist developers in identifying performance bottlenecks within their Java applications. Employing these tools is a proactive measure to ensure that the application runs smoothly. These tools provide insights into memory usage, CPU cycles, and threading activities.
Some notable tools include:
- VisualVM: This tool provides a visual interface for monitoring Java applications. It includes features for memory and CPU profiling, thread analysis, and garbage collection monitoring.
- JProfiler: JProfiler integrates with various IDEs and helps pinpoint performance issues effectively. It offers insights into memory consumption, CPU profiling, and detailed views of thread executions.
- JConsole: This tool comes with the Java Development Kit (JDK). It offers monitoring capabilities to review memory usage and monitor application performance.
"Performance optimization is not just a feature; it is a necessity for modern applications."
Understanding memory management along with employing profiling tools is a step towards ensuring optimal performance in Java applications. Through consistent efforts in these areas, developers can create robust software ready to handle the demands of today's users.
Best Practices in Java Development
Best practices in Java development are critical for creating maintainable, efficient, and scalable applications. As the language continues to evolve, adhering to these principles can facilitate smoother collaboration among developers and help avoid common pitfalls. Efficient code management ensures long-term project success and reduces the overhead of debugging and refactoring later on.
Code Readability
Code readability is vital in Java development. It refers to how easily a programmer can understand another developer’s code. Clear and concise code enhances maintainability. When others read your code, they should grasp the intention behind it without confusion.
To improve code readability, consider the following elements:
- Consistent Naming Conventions: Use meaningful names for classes, methods, and variables. For instance, instead of using vague names like "x" or "y", opt for descriptive names such as "userCount" or "calculateTotal".
- Proper Indentation and Spacing: A well-indented code block helps visually segregate different sections of code. It makes the structures and logic clear.
- Avoid Complex Constructs: While Java allows for sophisticated coding patterns, avoid overly complex structures that obscure the code's purpose. Simplicity often leads to better understanding.
By prioritizing code readability, developers can ensure that the codebase can be easily navigated, understood, and modified as necessary. This consideration becomes even more crucial in team settings where multiple developers contribute to a project.
Effective Use of Comments
Comments in Java serve as an essential tool for explaining the purpose and functionality of code sections. They can significantly enhance the understandability of the code. However, comments should not be redundant or obvious. Instead, they should provide insights that are not immediately clear from the code itself.
Here are some key practices for using comments effectively:
- Explain Why, Not What: Comments should clarify the rationale behind a design decision or a specific approach. For example, instead of stating what a method does, explain why that method exists or what problem it addresses.
- Use TODOs Wisely: Insert TODO comments when further work is necessary, but ensure these are kept up to date. Neglected TODOs can lead to confusion.
- Keep Comments Up-to-Date: Outdated comments can lead to more confusion than clarity. Ensure that comments are reviewed and updated as the code changes.
Closure
Implementing best practices, such as focusing on code readability and effectively using comments, contributes significantly to the quality of Java applications. These practices ensure code remains accessible and intelligible to other developers, ultimately enhancing collaboration and reducing development costs.
Remember, well-commented and readable code acts as documentation that speaks for itself, making both current and future development easier.
Emerging Trends in Java
The realm of technology evolves rapidly. Java, a language that has been prevalent for decades, is no exception. Emerging trends in Java are crucial for staying relevant in software development. They highlight shifts in development practices, tool use, and application scopes. Understanding these trends allows developers to adapt their skills and strategies effectively in an ever-changing landscape. Additionally, these trends reveal the direction where Java is heading, helping businesses make informed decisions about technology investments.
Java in Cloud Computing
Cloud computing has changed how companies manage resources and deploy applications. Java is widely used in the cloud for several reasons. First, its platform independence allows applications developed in Java to run seamlessly on various cloud services. This agility is essential for organizations that leverage different cloud environments. Furthermore, popular service providers like Amazon Web Services and Google Cloud Platform offer Java SDKs that simplify integration with cloud services.
Java's robustness enhances its value in a cloud environment. With a strong focus on enterprise applications, it allows organizations to create scalable microservices architectures. Developers can use tools like Spring Boot to create lightweight applications that can be deployed in cloud environments easily. This approach also simplifies updates and management, reducing downtime during transition phases.
"The rise of cloud computing has made Java more versatile and accessible, helping developers meet dynamic business needs effectively."
Another significant consideration is security. As cloud deployments increase, so does the necessity for reliable security measures. Java provides built-in security features, ensuring that data is protected at multiple levels. These features are essential for businesses handling sensitive information in the cloud, such as financial or personal data.
Java for Big Data
Big data presents both challenges and opportunities for businesses. Java's contributions to big data processing are substantial. Frameworks like Apache Hadoop and Apache Spark are largely written in Java. This makes Java an excellent choice for organizations looking to implement big data solutions effectively. Developers with Java skills can take advantage of these frameworks to analyze and process vast amounts of data efficiently.
The versatility of Java is vital in the big data ecosystem. Its ability to support various data processing models, from batch processing to streaming, offers developers flexibility in choosing how to handle data. Additionally, Java's strong community support means that developers can access numerous libraries and tools designed for big data processing.
Moreover, as streaming data becomes more prevalent, Java’s concurrency utilities become critical for real-time data processing. This feature equips developers to build applications that respond to data as it arrives, making systems more dynamic and efficient.
In summary, the emerging trends of Java in cloud computing and big data illustrate the language's continued relevance. Understanding these trends can guide developers and organizations in leveraging Java to meet modern programming challenges. By embracing cloud technologies and the big data paradigm, Java users can improve application capabilities and data management strategies.
Community and Resources
Understanding the community and resources surrounding Java is crucial for learners and developers alike. The collective knowledge from various channels can significantly enhance one's programming capabilities and provide essential support throughout the development journey. In the fast-evolving tech landscape, being part of a vibrant community can open doors to opportunities and insights that are otherwise difficult to obtain. Here, we will explore two main aspects: online communities and further learning resources.
Online Communities
Online communities serve as hubs for Java enthusiasts to share knowledge, troubleshoot issues, and discuss the latest trends and developments in the language. These platforms are invaluable for both novice and experienced developers. Platforms like Reddit and Facebook host numerous groups and forums dedicated to Java programming. Here are some key benefits of participating in these communities:
- Peer Support: When encountering challenges, it is beneficial to seek help from experienced peers or mentors who can offer guidance and solutions.
- Knowledge Sharing: Communities foster an environment where members can exchange insights, tips, and resources that enhance learning.
- Networking Opportunities: Engaging with others in these communities can lead to valuable connections that may result in job offers, collaborations, or partnerships in the future.
- Up-to-Date Information: Staying informed about recent developments and best practices in Java programming is easier when engaging with a community that shares the latest news.
Moreover, platforms such as Reddit's r/java and Facebook groups dedicated to Java programming provide a space for asking questions, sharing projects, and gaining insights from discussions. However, remember to participate conscientiously and contribute positively, as the community thrives on mutual respect and collaboration.
Further Learning Resources
For anyone looking to deepen their knowledge of Java, numerous resources are available beyond traditional textbooks and documentation. Various online courses, tutorials, and official resources can facilitate continued learning in a structured manner. Below are some valuable resources to explore:
- Oracle's Official Java Documentation: This is the definitive resource for understanding Java from the creators of the language. It provides comprehensive guides and API documentation.
- Codecademy: Offers interactive courses that guide learners through the basics and advanced topics in Java, emphasizing hands-on practice.
- Coursera and edX: These platforms host courses offered by top universities. Topics range from beginner to advanced levels, often including certificates for successful completion.
- YouTube Tutorials: Many programmers share their knowledge through videos, providing visual explanations of Java concepts and coding practices.
- Java Books: Classics like "Effective Java" by Joshua Bloch offer deep insights into Java programming practices.
In sum, a well-rounded approach to learning Java should incorporate engaging with communities and utilizing diverse learning resources. By doing so, learners can cement their understanding of core concepts and practices in the Java ecosystem, leading to more effective programming skills.
Ultimately, the combination of community interaction and rich educational resources equips learners with not just knowledge and skills but also confidence to tackle real-world problems.
Epilogue
In reviewing the overall themes of this article, it becomes clear that understanding the importance of Java is crucial for anyone interested in programming. Java is not only a versatile language but also a foundational pillar in the realm of modern software development. Its widespread use across various domains, such as web applications, mobile platforms, and enterprise solutions, makes it relevant for today's technological landscape.
The Future of Java
Java has maintained its position as a leading programming language for decades. The future of Java seems promising, as it is constantly evolving to accommodate modern development practices. Here are a few critical aspects that highlight its ongoing relevance:
- Continual Updates: Java's regular updates with new features and improvements enhance its capabilities.
- Community Support: A strong community of developers ensures that Java stays relevant. Forums and online platforms like Reddit become great resources for knowledge sharing and support.
- Integration with New Technologies: Java adapts well with emerging technologies, such as cloud computing and big data. This adaptability helps Java developers to tackle the demands of new projects effectively.
- Legacy Systems: Many enterprises rely on Java-based systems. These established systems mean that Java will remain crucial in maintaining and enhancing existing software solutions.
Overall, as long as developers continue to harness its strengths and potential, Java will remain a vital tool and a popular choice.