A Comprehensive Guide to JavaScript for Developers
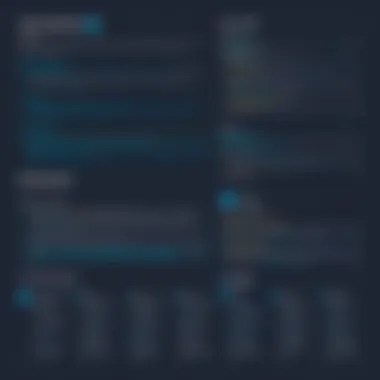
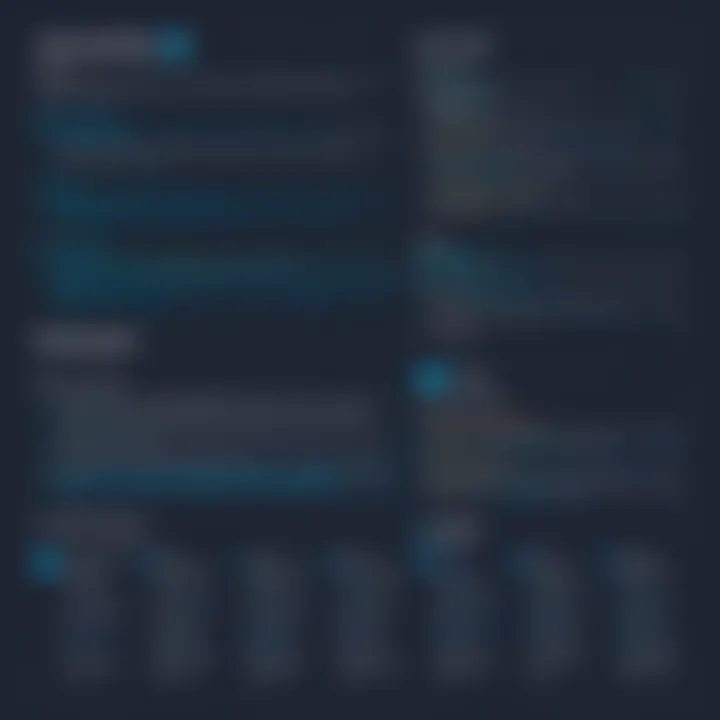
Prologue to Programming Language
JavaScript is a versatile programming language that has become a cornerstone of modern web development. Often referred to simply as JS, this language facilitates the creation of dynamic and interactive websites, enabling developers to bring their web content to life. Understanding JavaScript is essential for anyone looking to forge a path in the technology landscape today.
History and Background
JavaScript was born in the mid-1990s, conceived by Brendan Eich while working at Netscape. Originally meant to add lightweight interactivity to web pages, its rapid evolution captured the interest of the programming community. What started as a modest scripting language has transformed into a powerful tool that underpins a significant portion of the web. Over the years, it adapted through numerous updates and enhancements, most notably the introduction of ECMAScript, which standardized its various features and functionalities.
Features and Uses
The allure of JavaScript lies in its diverse features and capabilities. Some key characteristics include:
- Interactivity: It allows developers to create engaging user experiences.
- Versatility: It can be used on both client and server sides, especially with frameworks like Node.js.
- Rich Ecosystem: A multitude of libraries and tools, such as React.js and jQuery, are available to streamline development tasks.
JavaScript finds application across various domains: from game development to mobile apps, and even server-side programming. This breadth of usage highlights its significance in today’s tech environment.
Popularity and Scope
JavaScript’s popularity is undeniable; it ranks as one of the most frequently used languages among developers worldwide. According to recent surveys from platforms like Stack Overflow, a staggering number of professionals are utilizing it in their projects. Moreover, with the increasing demand for web applications, JavaScript's scope continues to expand.
"JavaScript is not just a language; it's a movement that drives the web forward."
In summary, as more organizations pivot towards digital solutions, JavaScript remains a vital skill for aspiring developers.
Basic Syntax and Concepts
Understanding JavaScript's syntax and fundamental concepts is crucial for beginners. These elements serve as the building blocks for more advanced programming techniques.
Variables and Data Types
In JavaScript, variables are essential for storing information. The primary data types include:
- String: Represents text, e.g.,
- Number: Represents numerical values, e.g.,
- Boolean: Represents true or false values.
Variables can be declared using , , or , each serving different purposes in scope and mutability.
Operators and Expressions
JavaScript offers various operators to manipulate data. These include:
- Arithmetic Operators: Such as , , ,
- Comparison Operators: Such as , , ``,
- Logical Operators: Such as , ,
An expression combines variables and operators to yield a value, making it a core concept in programming.
Control Structures
Control structures dictate the flow of the program. Common constructs are:
- If statements for decision-making.
- Loops (like and ) for repetitive tasks.
These structures allow for more complex decision-making processes and enhance the flexibility of the code.
Advanced Topics
As developers grow more proficient, delving into advanced topics becomes essential.
Functions and Methods
Functions are reusable blocks of code designed to perform specific tasks. JavaScript supports both function declarations and expressions. Here’s a simple function:
Methods, on the other hand, are functions associated with objects. Understanding these concepts allows developers to write cleaner, more efficient code.
Object-Oriented Programming
JavaScript is not purely object-oriented but supports object-oriented programming principles. It utilizes objects to model real-world entities, enhancing code organization and reusability.
Exception Handling
Error handling is a fundamental aspect of robust programming. JavaScript offers , , and blocks to manage exceptions gracefully, ensuring that the user experience remains smooth even when issues arise.
Hands-On Examples
Putting theory into practice is vital for mastering JavaScript.
Simple Programs
Starting with straightforward programs, like calculating the sum of two numbers or greeting users, allows beginners to get a feel for code syntax and logic.
Intermediate Projects
As skills improve, tackling larger projects such as a simple to-do list app can further enhance understanding. Such projects provide real-world application of JavaScript concepts and present challenges that require problem-solving skills.
Code Snippets
Sharing code snippets can also be a great way to learn. Here’s a snippet that displays the current date:
Resources and Further Learning
Learning JavaScript doesn’t stop here. Many resources are available for continuous growth.
Recommended Books and Tutorials
Books like "Eloquent JavaScript" and tutorials found on platforms like Mozilla Developer Network offer invaluable insights for learners at all levels.
Online Courses and Platforms
Websites like Udacity and Codecademy provide structured learning programs that guide users through the various facets of the language.
Community Forums and Groups
Joining forums such as Reddit (subreddits like r/learnjavascript) or Facebook groups can help learners connect with others, share knowledge, and seek advice on challenges faced during their journey.
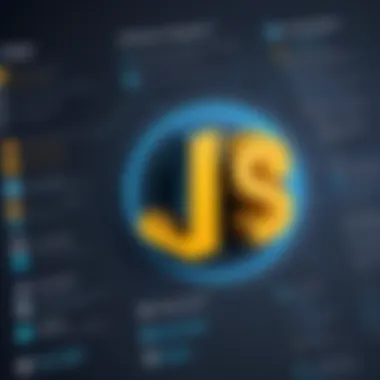
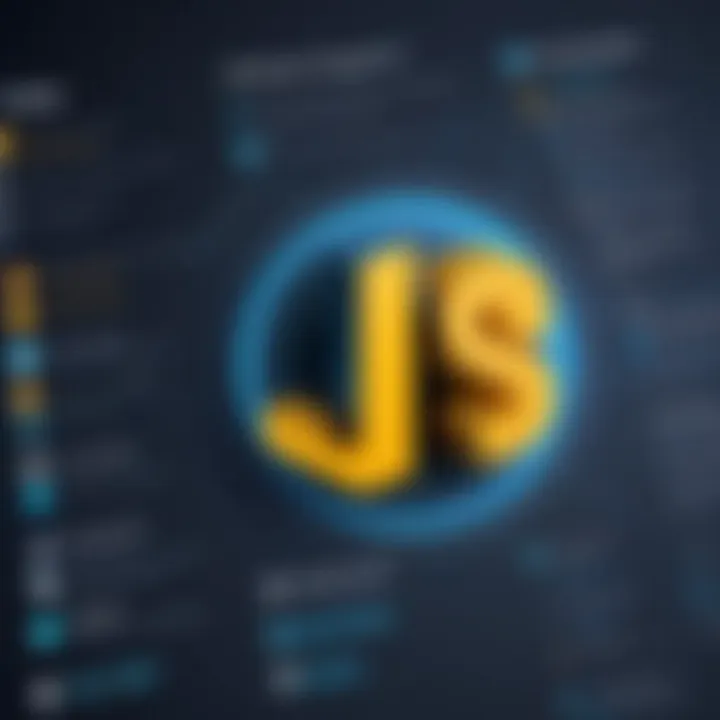
Understanding JavaScript
JavaScript stands as a cornerstone in the realm of web development. Its multifaceted nature makes it indispensable for building dynamic, interactive websites that capture user engagement. At its core, JavaScript enables developers to create rich, responsive user experiences. This influence stretches beyond mere functionality; it directly impacts the aesthetic and behavioral elements of web pages. By understanding JavaScript, practitioners can harness its potential to craft more compelling digital experiences.
In the vast landscape of programming languages, JavaScript manages to balance complexity and accessibility. It offers a robust syntax that allows students and novice programmers to grasp fundamental concepts fairly quickly. When learning JavaScript, the blending of theoretical knowledge with practical application becomes paramount. The benefits of mastering this language extend into various areas, such as career opportunities, innovation in project creation, and collaboration within teams.
Furthermore, JavaScript's versatility means it can be utilized both on the client-side and server-side, empowering developers to create end-to-end solutions. In a digital world that increasingly seeks seamless integration across platforms, JavaScript remains at the forefront, leading to its rampant adoption in diverse applications.
The Role of JavaScript in Web Development
JavaScript plays a vital role in transforming static web pages into interactive experiences. With its ability to manipulate HTML and CSS, JavaScript acts as the bridge connecting form and function. When a user interacts with a web page, such as clicking a button or submitting a form, JavaScript executes scripts that create immediate feedback, enriching user experience.
The influence of JavaScript extends to various realms of front-end development. Through libraries and frameworks like React.js or Vue.js, developers leverage the power of JavaScript to build efficient user interfaces and handle data with ease. This fluid interaction with client-side technologies allows for optimized web performance, making applications feel lightweight and responsive.
Moreover, JavaScript is not limited to front-end tasks. With the advent of Node.js, developers can execute JavaScript on the server-side, enabling full-stack development without necessarily switching languages. This capability simplifies the development process and strengthens JavaScript’s position in the programming community.
JavaScript's Evolution Over Time
From its inception in 1995 to its current state, JavaScript has undergone significant evolution. Initially designed for simple tasks, it has matured into a powerful language that supports complex applications. The introduction of ES5 and subsequent updates have brought new features that enrich the language, such as arrow functions, promises, and async/await functionality.
"JavaScript's journey is a testament to the adaptability of technologies in an ever-changing digital landscape."
The community-driven improvement of JavaScript has resulted in a robust set of tools, practices, and community support. Developers can now utilize modern transpilers and bundlers like Babel and Webpack, which have further streamlined the development process, allowing them to write better-structured, maintainable code.
As technology continues to advance, so too does JavaScript, which now not only powers web browsers but also plays a key role in mobile applications and Internet of Things (IoT) devices. Keeping up with these changes and innovations is crucial for anyone looking to excel in programming. Understanding JavaScript in this dynamic context opens up avenues for creative problem-solving and professional development.
Core Features of JavaScript
Understanding the core features of JavaScript is crucial for any budding programmer or web developer. These features form the bedrock upon which JavaScript functions effectively, enabling developers to create dynamic and interactive web experiences. Each aspect has its unique characteristics, advantages, and implications for coding practices. Examining these core features reveals why JavaScript remains at the forefront of web development, driving innovation and efficiency.
Dynamic Typing and Flexibility
Dynamic typing is one of the most attractive features of JavaScript. Unlike statically typed languages like Java, where variables must be declared with a specific type, JavaScript allows variables to hold any type of data at any time.
This means you can easily change a variable's type without much hassle. Imagine a simple variable that starts off holding a number:
And later, this same variable could be reassigned to a string:
This flexibility fosters speed in development but does come with its challenges, such as type coercion errors that can arise when mixing types. The advantage, however, is clear: developers can prototype and iterate faster, adapting their code to meet changing requirements without rigid frameworks restricting creativity.
First-Class Functions
JavaScript treats functions as first-class citizens, which is a key feature that distinguishes it from many other programming languages. This means that functions can be assigned to variables, passed as arguments to other functions, and even returned from other functions.
This leads to powerful programming techniques. For example, a simple function could look like this:
This function can be assigned to a variable, sent to another function, or returned from a function. The ability to treat functions as values opens the door to functional programming paradigms, enabling developers to write cleaner, more concise code that can be reused and maintained more efficiently.
"In coding, how you think about functions can change everything, from structure to concept. Embracing first-class functions is embracing versatility."
Prototype-Based Inheritance
Prototype-based inheritance is another fundamental aspect of JavaScript's design. While many languages use class-based inheritance, JavaScript opts for a more flexible approach through prototypes. This means that objects can inherit directly from other objects.
For instance, if you create a prototype for a object, any new instance can inherit properties and methods from the prototype:
What sets prototype-based inheritance apart is its dynamic nature, allowing for changes to be made at runtime. It grants flexibility that can be advantageous, especially in large software projects requiring adaptable structures. While it might seem confusing at first, grasping this concept enriches a developer's toolkit, promoting efficiency in code reuse and maintenance.
In summary, the core features of JavaScript — dynamic typing, first-class functions, and prototype-based inheritance — form a vital part of understanding how this language operates. These elements not only enhance developer productivity but also contribute to creating robust, adaptable applications that meet diverse user needs. By mastering these concepts, one lays down a solid foundation for further exploration into the powerful and evolving ecosystem of JavaScript.
JavaScript Syntax and Semantics
Understanding JavaScript's syntax and semantics is paramount for anyone venturing into the world of this versatile programming language. Syntax refers to the set of rules that dictates how the code must be written for the JavaScript engine to comprehend it. On the other hand, semantics pertains to the meaning behind those syntactical constructs. Both elements work hand in hand, and getting a grip on them is essential for writing efficient, readable, and maintainable code.
The clarity of syntax not only reduces errors but also makes collaboration with others much smoother. When programmers follow consistent syntax rules, it becomes easier for fellow coders, future maintainers, and even themselves to understand and modify the code as required.
Moreover, understanding the semantics helps prevent common pitfalls where the syntactically correct code could produce unintuitive or unexpected outcomes. Therefore, this section will break down key concepts within these two components, ensuring readers grasp the underlying principles necessary for mastering JavaScript.
Basic Constructs: Variables and Data Types
In JavaScript, variables are the primary storage units. They hold data values that you can manipulate throughout your code. The beauty of JavaScript is showcased right at the beginning with its flexible variable types. To define a variable, developers can employ keywords such as , , or depending on the requirements of their code.
For instance:
The types of data you can store range from primitive types like strings, numbers, and booleans to complex types such as objects and arrays. This dynamic typing allows programmers to create robust applications quickly. However, with great power comes great responsibility.
Variable hoisting can lead to unexpected results if you don't pay attention to scoping rules. Therefore, a solid understanding of how variables and data types operate is essential, especially when working on larger applications.
Control Structures and Flow Control
Control structures in JavaScript steer the execution path of the program. They enable programmers to dictate conditions under which certain parts of the code will run, creating a logical flow in applications. The primary control structures are , , , among others.
For example, let’s consider a simple conditional statement:
Understanding flow control is like reading a map; you need to know where you are heading and the routes available to get there. Loops such as , , and are also crucial, allowing you to execute blocks of code repeatedly under specific conditions. However, misuse or misunderstanding of loops can lead to infinite loops, wreaking havoc on application performance.
Functions: Declaration vs. Expression
Functions in JavaScript can be created in two primary ways: through declarations and expressions. A function declaration is defined using the keyword followed by its name and body. For instance:
This function can be invoked anywhere in the code after its declaration.
On the flip side, function expressions are defined as part of an expression. They can be anonymous, making them a bit different in terms of usage. Here's an example:
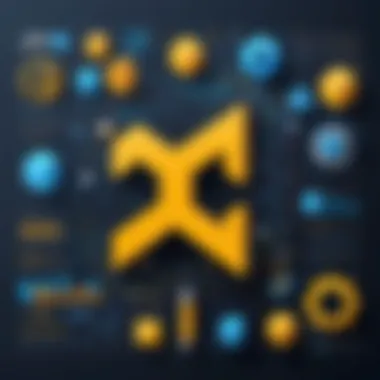
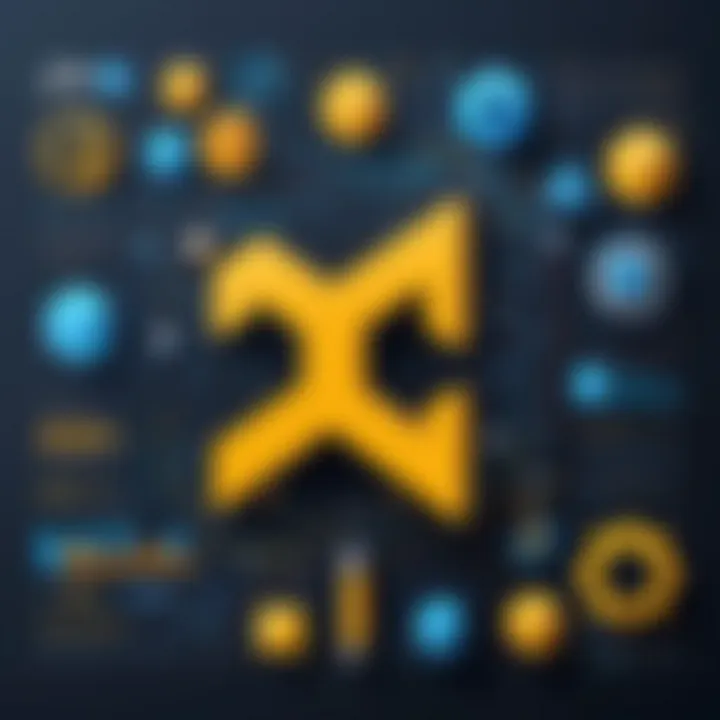
This variation can often lead to confusion around scope and when a function can be executed. Understanding both forms is essential, as they offer flexibility in defining behavior in your applications.
Using functions effectively can streamline your code and enhance reusability, allowing you to separate concerns and encapsulate functionality.
Ultimately, the mastery of JavaScript's syntax and semantics provides the foundation for adept programming. With it, developers can avoid common mistakes, craft clean code, and efficiently solve complex problems.
In summary, a firm grasp of these concepts makes all the difference in writing high-quality code. As you continue your journey, keep in mind that each construct serves a purpose, and understanding when and how to use them appropriately is key to becoming proficient in JavaScript.
Working with the DOM
Working with the Document Object Model (DOM) is a cornerstone of JavaScript's functionality, especially when it comes to creating dynamic and interactive web pages. Without understanding how to manipulate the DOM, developers might be like fish out of water, unable to effectively breathe life into their applications. The DOM is essentially the bridge between the HTML structure of a web page and the JavaScript code that operates on it, allowing developers to read and modify the webpage in real time.
Understanding the Document Object Model
To grasp the significance of the DOM, let’s start with its essence. The DOM represents the document structure as a tree of objects. Each node in this tree corresponds to a part of the document: elements, attributes, and text. This hierarchical representation allows JavaScript to traverse the structure and manipulate it, modifying content, style, and even the layout on the fly. Imagine a chef in a kitchen, having all the ingredients laid out clearly; the DOM gives you a clear view over every part of the page, making it easy to adjust things as you see fit.
"The Document Object Model is like a map of your website; it helps you navigate through the complexities of a document's structure."
One crucial thing to understand is that the DOM is not static. When you change a web page with JavaScript, you can add, remove, or modify elements. This is where the idea of interactivity comes into play. Users expect a lively experience — think of how annoying it would be if buttons didn’t respond or content didn’t change upon interaction.
Manipulating Elements with JavaScript
Manipulating elements in the DOM is where JavaScript shines. Through various methods and properties, developers can interact with all components of a web page. Consider the following methods:
- allows you to select an element based on its unique ID.
- and let you find elements based on CSS selectors.
- Once you select the desired element, you can modify its inner content with , adjust styling using the property, or even append new elements using methods like .
For example, if you have a button that should change the text of a header, you might write:
This kind of flexibility is what makes JavaScript integral to user experience, allowing the seamless flow of information and feedback.
Event Handling Mechanics
Event handling is where JavaScript goes from being merely a scripting language to becoming a powerhouse of interactivity. Events are actions that occur in the browser, like clicks, typing, or mouse movements. JavaScript can listen for these events and react accordingly, making it possible to create engaging web applications.
To put it simply, you can think of events as triggers that can fire off responses from your JavaScript code. For instance, when a user hovers over a button, you can change its color, or when a form is submitted, you can validate the input. Here’s a basic example of event handling:
In this snippet, when the user clicks the button, an alert pops up, effectively giving instant feedback. This level of interaction is what keeps users engaged and can significantly enhance the user experience.
By using the DOM effectively, developers can craft fluid, responsive, and immersive experiences, which are essential in the modern web environment.
Performance Considerations
Performance in JavaScript is not just a buzzword but a core principle that can make or break a web application. In an online environment where milliseconds count, understanding how to optimize JavaScript execution is key for ensuring smooth user experiences. This section aims to dig into the nitty-gritty of performance considerations, focusing on specific elements like execution efficiency, resource management, and strategies to elevate performance.
JavaScript Execution and Optimization
At the heart of performance lies JavaScript execution. The JavaScript engine, like Google Chrome’s V8 or Mozilla’s SpiderMonkey, executes code, turning the high-level script into something the machine can work with. This process involves parsing the code and compiling it just-in-time (JIT). Understanding this flow is vital for optimizing performance.
To get the best out of JavaScript, consider these points:
- Minimize DOM Manipulations: Accessing or modifying the DOM can slow things down. Instead of making several changes, batch updates when possible. It’s like painting a room—doing it in one go is faster than making multiple trips to the paint store.
- Use Efficient Algorithms: Sometimes it’s the little things that count. Using the right algorithms can drastically reduce computational time. Instead of a bubble sort, consider using quicksort, especially with larger datasets.
- Leverage Caching: Frequently used computation results can be stored, avoiding repeated processing. Think of caching as a memo; it’s an excellent way to remember answers without retracing your steps.
- Profile Code Regularly: Use browser developer tools to identify bottlenecks. It’s like checking the engine lights on your car; a little tuning can lead to better performance.
Minification and Bundling Techniques
Minification and bundling are two practical techniques that profoundly influence performance. Minifying code involves stripping out unnecessary characters, such as whitespaces and comments, reducing file size with minimal to no effect on functionality. This technique results in faster downloads and quicker execution.
Bundling is equally crucial. Instead of having multiple script files loading separately, bundling combines these into a single file. This not only minimizes the number of HTTP requests a browser makes but also enhances page load times.
- Benefits of Minification and Bundling:
- Reduced file sizes lead to faster downloads.
- Fewer HTTP requests mean lesser latency.
- Improved overall performance of web applications.
Incorporating these methods can be likened to organizing a kitchen: one pot instead of five saves time and reduces mess, making the cooking process smoother.
These performance considerations are crucial for web developers, especially when building complex applications. Fostering a solid understanding of execution and optimization will elevate any JavaScript project to greater heights.
"Performance optimization isn’t about being clever just because you can; it’s about making applications that interact with users in a manner that feels seamless."
By implementing smart strategies and techniques, developers can ensure they are not just coding efficiently but also elevating the functionality and user experience of their applications.
Advanced JavaScript Concepts
Understanding advanced JavaScript concepts is crucial for anyone looking to master the language. These concepts not only provide depth to your coding skills but also equip you with tools that can significantly enhance your workflow. Engaging with these topics opens up a range of possibilities in practical applications, from managing asynchronous operations to organizing your code effectively. Here, we delve into the importance of asynchronous programming and module patterns, addressing their benefits and considerations in the broader JavaScript ecosystem.
Asynchronous Programming with Promises and Async/Await
Asynchronous programming in JavaScript is an essential skill, particularly in an age where responsive web applications are a norm. Traditionally, JavaScript operated in a single-threaded environment, which posed challenges when handling multiple operations simultaneously. Enter Promises and – two powerful constructs that allow developers to write cleaner, more manageable code for handling asynchronous actions.
Promises represent the eventual completion (or failure) of an asynchronous operation and its resulting value. This can help simplify complex chaining of tasks, making it easier to read and maintain the code. Here’s a simple illustration:
On the other side, allows us to write asynchronous code in a synchronous-looking fashion. This not only reduces complexity but also improves error handling by utilizing traditional methods. Consider this example:
This syntactical sugar enables you to write asynchronous code that is almost indistinguishable from synchronous code, greatly improving readability.
Understanding these mechanisms is critical for building responsive user interfaces, as they help avoid blocking the main thread during potentially lengthy operations.
The Module Pattern and ES6 Modules
The way we organize our JavaScript code has evolved significantly, leading us to the concept of modules. The Module Pattern was one of the first approaches to achieve better organization in JavaScript programming. This pattern allows you to encapsulate functionality within a single scope, which keeps the global namespace clean and reduces potential conflicts.
For instance, using the Module Pattern, you can define private and public variables and methods like this:
With the introduction of ES6, modules were standardized, bringing in and statements that streamline the way modules are created and consumed. This modern approach enhances code maintainability and encourages reusability across different parts of an application.
Example of an ES6 module:
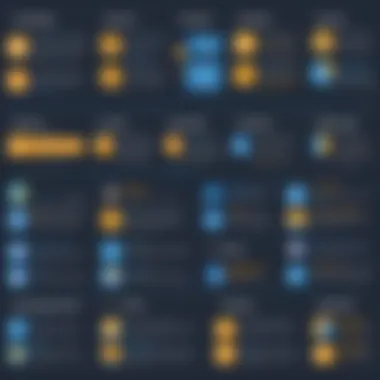
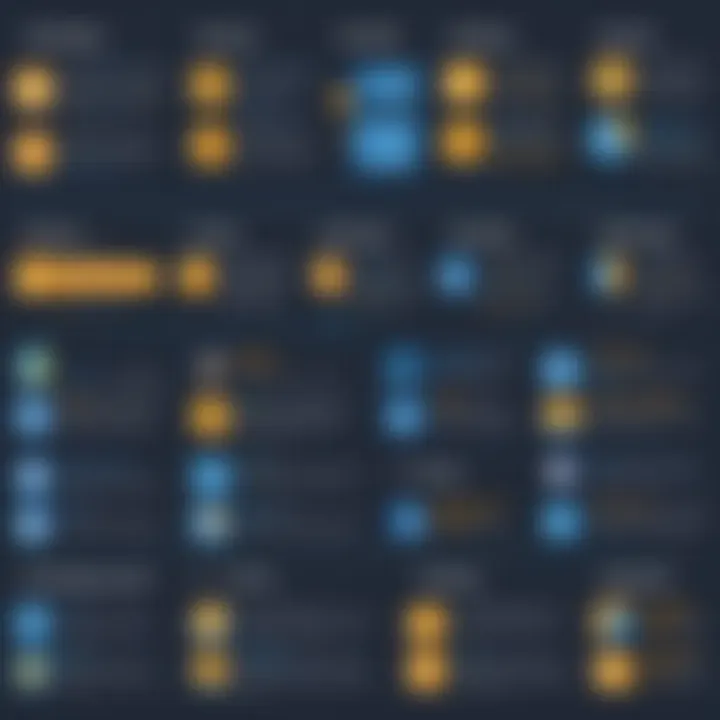
In essence, these modular systems reduce dependencies, thus saving developers from the headaches of tangled code while promoting better architectural patterns.
Understanding advanced JavaScript concepts such as asynchronous programming and module organization can drastically impact your development practices. As you dig deeper into these topics, you'll find that they not only streamline your code but also open doors to collaborative programming and efficient project management.
Popular JavaScript Frameworks and Libraries
In the rapidly evolving landscape of web development, popular JavaScript frameworks and libraries hold a crucial place. They simplify the complexities of coding, making it more manageable, efficient, and productive. One of the primary reasons these tools are immensely valued is their capacity to streamline the development process. With built-in functionalities and ways to handle common tasks, developers can build interactive applications faster. More importantly, they foster a community of practice, allowing developers to share code, techniques, and solutions.
Prolusion to React
React has emerged as a front-runner in the sphere of JavaScript libraries, primarily used for building user interfaces. It was introduced by Facebook and has garnered significant traction over the years. React’s component-based architecture allows developers to design encapsulated components that manage their own state. This makes it easier to create interactive UIs as changes in data automatically update the rendered components.
One remarkable aspect of React is its virtual DOM. Instead of updating the entire page whenever a change is made, React updates only the part of the UI that has changed. This leads to substantial performance enhancements because rendering processes are minimized. Developers favor React for its adaptability, the vast ecosystem around it, and the robust community support.
Exploring Vue.js
Vue.js is another popular JavaScript framework that has made waves among developers, especially for its gentle learning curve. It’s designed to be incrementally adaptable, letting newcomers build applications in stages while offering options to integrate it with other projects. Vue is known for its simplicity, making it a favorite for small to medium projects. Yet, it has the ability to scale for larger applications as well.
A key feature of Vue is its reactivity system that allows data binding to the UI. For example, when the underlying data changes, the UI reflects this change automatically, which simplifies the process of syncing data with the users' view.
"Vue might seem like a small player compared to giants like React and Angular, but its focus on the view layer and easy integration makes it a worthy contender."
Node.js and JavaScript on the Server Side
Node.js represents a paradigm shift in how JavaScript is employed, extending its reach from the client side to the server side. Built on Chrome’s V8 JavaScript engine, Node.js allows developers to execute JavaScript code outside of a browser environment. This means that you can write your server-side logic using the same language as your client-side code, creating a more seamless development process.
One of the key benefits of using Node.js is its non-blocking, event-driven model, which makes it exceptionally suitable for handling multiple connections simultaneously. This architecture is perfect for applications with high I/O operations requiring real-time data, such as chat applications or live updates.
Using Node.js also opens the door to many powerful modules available through npm (Node Package Manager), enabling developers to easily incorporate a vast array of functionalities into their applications. The flexibility and efficiency that Node.js offers make it a vital tool in the modern developer's toolkit.
Testing and Debugging JavaScript
In the ever-evolving landscape of web development, the significance of robust testing and debugging practices in JavaScript cannot be overstated. The primary goal of these methodologies is to ensure that applications run smoothly, deliver the expected results, and maintain a high degree of reliability. Every developer, regardless of proficiency, understands that errors are part of the programming journey. However, how these errors are identified, managed, and rectified makes a significant difference in the development process and user experience.
Testing serves as a preventive measure. By thoroughly examining code prior to deployment, developers can catch technical issues that could escalate into larger problems post-launch. With JavaScript being a flexible language, it’s essential to employ testing techniques that accommodate its dynamic nature, which can unexpectedly lead to bugs if not carefully handled. This is especially useful when utilizing libraries and frameworks that may introduce additional complexities into applications.
Debugging, on the other hand, is the art of unraveling those pesky bugs that slip past testing phases. It often requires a keen eye for detail, logical reasoning, and experience with various tools. As the popular saying goes, "measure twice, cut once." In software development, taking the time to test and debug can save a tremendous amount of time and frustration later.
Debugging Techniques and Tools
When confronting bugs in JavaScript, developers can rely on a myriad of debugging techniques and tools designed to streamline the process. Below are some common approaches that might come handy:
- Console.log: This fundamental technique enables developers to sift through data by logging output directly to the console. It’s simple, but effective in displaying what’s happening at specific points in the code.
- Browser Developer Tools: Modern web browsers come equipped with powerful developer tools (like Chrome DevTools or Firefox Developer Edition). These provide a suite of options for inspecting HTML, monitoring network requests, and debugging JavaScript.
- Breakpoints: Setting breakpoints allows developers to pause code execution at specific lines, giving them the chance to inspect the state of variables and the call stack.
A brief code snippet below highlights how to utilize console.log effectively:
"Debugging is like being the detective in a crime movie where you are also the murderer." – Filipe Fortes
These tools and techniques work together to help the coder pinpoint the root of issues and fine-tune the application’s behavior.
Unit Testing Frameworks Overview
Unit testing plays a crucial role in the software development lifecycle by verifying each part of the code functions as intended. This is done through various frameworks designed to facilitate the testing process in JavaScript. Here’s a look at some prominent frameworks that developers commonly utilize:
- Jest: Developed by Facebook, Jest provides a comprehensive testing framework that's particularly robust for testing React applications. It features built-in assertion libraries and is known for its ease of use and parallel test execution.
- Mocha: A flexible testing framework that works well with a variety of assertion libraries, like Chai. Mocha is highly customizable and is known for its asynchronous support, making it suitable for testing applications involving asynchronous operations.
- Jasmine: This behavior-driven development framework is great for testing JavaScript code. Its syntax is clear, which can help in writing tests that are easy to read and maintain.
The adoption of these frameworks allows developers to ensure that functions and components behave predictably. Increased code reliability translates to better end-user experiences, making unit testing an integral aspect of JavaScript development.
From debugging tools to rigorous unit testing frameworks, understanding and implementing testing methodologies is essential for any developer who wishes to produce high-quality, maintainable code in the JavaScript ecosystem.
Best Practices in JavaScript Development
In today’s fast-paced world of web development, adhering to best practices in JavaScript development is essential. Such practices help not only in writing clean and maintainable code but also in fostering collaboration among team members. When a project scales up, having a shared understanding of code structures and styles can make all the difference. Here, we delve into some key principles that can significantly enhance your JavaScript coding journey.
Code Readability and Maintenance
Code readability is about making sure that your code speaks clearly to anyone who reads it, including your future self. When writing JavaScript, the aim should be to ensure that the code is easily understandable. Clear, readable code can save considerable time in the long run—like finding a needle in the haystack if the code is messy.
Here are a few strategies to enhance code readability:
- Consistent Naming Conventions: Use descriptive variable and function names. Avoid vague names like or ; instead, go for something meaningful, such as or .
- Modularization: Break down large functions into smaller, reusable ones. This keeps the code organized and allows you to isolate issues more effectively.
- Use of Whitespace: Don’t skimp on whitespace; proper spacing makes code visually appealing and easier to read. It helps in separating logical blocks of code.
- Avoid Deep Nesting: Too many nested functions can lead to complex and convoluted code. Aim for a flat structure where the logic flows cleanly from one point to the next.
Maintaining this readability is crucial. As projects evolve, updates will be inevitable. If the code is organized, making changes or adding new features will feel like a walk in the park rather than climbing a mountain.
Effective Use of Comments and Documentation
Comments serve as a guide for anyone reading your code. They should clarify complex logic, explain the purpose of functions, and provide context where necessary. However, it’s important to strike a balance—comments should support the code, not overshadow it.
Some best practices for commenting include:
- Commenting Intent, Not Implementation: Rather than stating what the code does line-by-line, focus on why it exists. This can provide insight into the thought process behind the code.
- Keep Comments Updated: Changing code without updating comments can lead to confusion. Always revise comments to reflect any modifications in the code.
- Documentation Generation: Consider using documentation tools like JSDoc to automate the creation of documentation from comments. This ensures that the documentation stays in sync with the code.
In addition, maintaining a good level of documentation can be tremendously beneficial. It serves as a valuable resource both during the development process and for onboarding new team members.
"Writing good code is one thing, but explaining it well is a skill that can’t be overlooked."
The Future of JavaScript
As we glance into the future of JavaScript, it’s clear that this programming language will continue to hold an integral spot in the ecosystem of web development. Since its inception in the mid-1990s, JavaScript has constantly evolved in response to emerging needs—not just among developers, but also to meet the expectations of users. This section will delve into what lies ahead for JavaScript, highlighting some key elements and benefits that could shape its trajectory.
Emerging Trends and Technologies
JavaScript is not synonymous with web browsers anymore; rather, it has expanded into diverse realms such as mobile application development, server-side programming, and even desktop applications. Here are a few emerging trends that merit attention:
- WebAssembly: This is making waves by allowing developers to run code written in multiple languages alongside JavaScript in the browser. The potential for speed and efficiency can’t be ignored.
- Serverless Architectures: With platforms like AWS Lambda and Azure Functions gaining traction, JavaScript’s role in building modern serverless applications is undeniable. This shift allows developers to focus on writing code without worrying about the underlying infrastructure.
- Machine Learning: Libraries like TensorFlow.js have burst onto the scene. This allows JavaScript developers to tap into machine learning models directly in the browser, opening new avenues for innovation. As more libraries emerge, the synergy between JavaScript and artificial intelligence seems to deepen.
- Progressive Web Apps (PWAs): These apps blur the lines between web and mobile, allowing users to install web applications on their devices while providing an experience akin to native applications. JavaScript's adaptability makes it the backbone of PWAs.
This range of emerging trends indicates that JavaScript is set to maintain its relevance. Anyone keeping an eye on the future would do well to consider the cardinal shifts and how they might adapt their skills accordingly.
The Role of JavaScript in Full-Stack Development
Full-stack development encompasses both client and server-side software, where JavaScript shines remarkably in modern tech stacks. It chimes in with frameworks like Node.js on the server side, which allows developers to build scalable network applications.
- Uniform Language: Using JavaScript across both client and server simplifies the development process. Developers can tackle both ends without constantly switching mindsets or learning different syntaxes, which enhances productivity.
- Community Support: The JavaScript community is vast. New developers can find countless resources, libraries, and tools to assist them as they navigate full-stack development more efficiently.
- Job Market Opportunities: Companies increasingly seek developers who are well-versed in full-stack technologies. JavaScript remains a key player in this landscape, underlining a career path rife with opportunities.
In essence, the future of JavaScript holds ample potential for its growth and relevance in the tech ecosystem. As new technologies consistently emerge, embracing these changes while honing JavaScript skills is key for aspiring developers looking to keep pace with current trends.
"JavaScript is the most versatile language that can bridge the gap between development paradigms and future advancements."
By preparing for these developments today, developers can secure a robust future in an ever-evolving digital landscape.