Mastering C++: A Comprehensive Guide to Coding
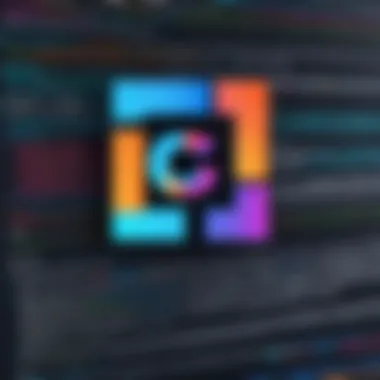
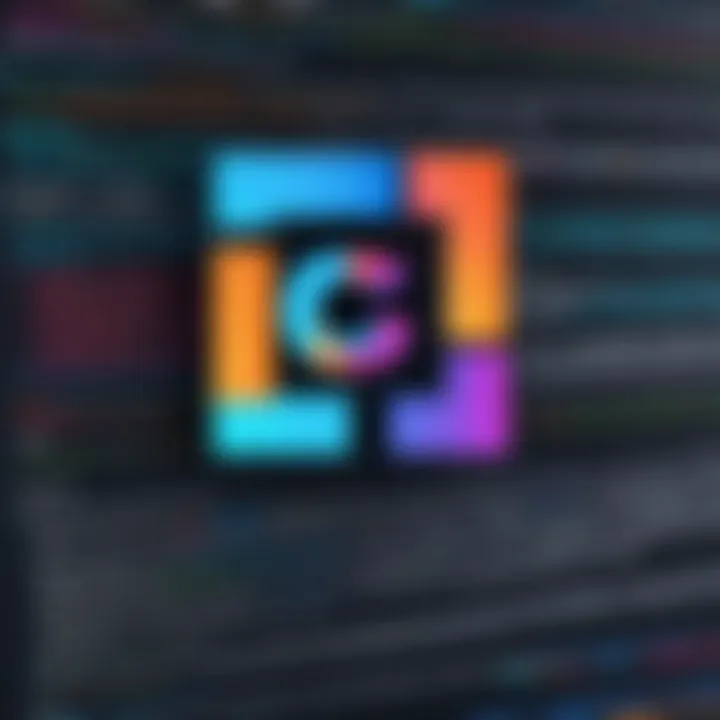
Preface to Programming Language
Programming languages are the core of software development. Understanding them is important for those wishing to delve into technology. C++ is a significant language influencing modern programming paradigms. It provides a foundation that has propelled careers and inspired innovations.
History and Background
C++ was designed by Bjarne Stroustrup at Bell Labs in the late 1970s. The language emerged to add object-oriented features to the C programming language. Since its inception, C++ has adapted to the changing needs of developers. The varied implementations reflect its flexibility and power over decades. Many application areas owe their development to innovations born from C++.
Features and Uses
C++ stands out due to several key features:
- Low-level manipulation: It allows precise control over resources.
- Object-oriented programming: This makes design easier through the use of classes and objects.
- Standard Template Library (STL): Offers a collection of algorithms and data structures, increasing code efficiency.
C++ finds applications in different domains, such as:
- Game development (e.g., Unreal Engine)
- Systems software (in compliance with UNIX/Linux)
- Real-time systems for various industries
Popularity and Scope
Popular among computer scientists and developers, C++ ranks high in language usage statistics. Its flexibility makes it a go-to choice for projects needing both high performance and complex data handling. Many coding competitions, educational institutions, and corporations prefer C++ due to its features.
Understanding C++ not only enhances programming skills, but it deeply influences computer science principles. Mastery of C++ sets a strong foundation for learning other languages, giving newcomers the tools to grow in their journey of programming.
C++ is an enduring language that continues to thrive in an ever-evolving technological landscape.
Basic Syntax and Concepts
C++ syntax might appear daunting at first, but familiarity will quickly reveal its logical structure. Gaining a firm grip on basic concepts lays valuable groundwork for further exploration.
Variables and Data Types
Every programming language has a way to store data, and C++ is no different. Here are common data types:
- int: Represents integers.
- float: Used for floating-point values.
- char: Designed for single characters.
Variables must be declared before use. For example:
Operators and Expressions
C++ offers a variety of operators to perform operations, such as:
- Arithmetic Operators: These help perform mathematical computations. E.g., , , , and .
- Relational Operators: They check the relationship between variables. For instance, , , and ``.
Expressions combine various components to form compound statements. Every developer must understand how to build precise expressions.
Control Structures
Control structures dictate how statements are executed. Fundamental control structures to know include:
- if statements: To execute code based on conditions.
- for loops: Used for repeating blocks of code a defined number of times.
- while loops: Continues until a specific condition becomes false.
Mastering these will significantly influence your coding efficiency and flow.
Advanced Topics
Stepping into advanced topics signals significant mastery.
Functions and Methods
Functions help organize code into reusable blocks. In C++, functions can return values, provide arguments, and contain any logic defined by the programmer. A simple example is:
Object-Oriented Programming
OOP is a paradigm fundamental to C++. It includes concepts such as:
- Classes and Objects: Blueprint and instance of classes. These bring structure to programs.
- Inheritance: Allows a new class to adopt properties and methods of an existing class.
- Polymorphism: Enables methods to do different things based on the object.
Exception Handling
Handling exceptions is crucial for robustness. C++ provides a mechanism to catch errors during runtime without crashing applications. Using , , and statements ensures smoother program execution.
Hands-On Examples
Real code plays a significant role in comprehension.
Simple Programs
Even the simplest C++ programs encapsulate idiomatic structures. A typical
Prelude to ++
C++ is a programming language with significant importance in the software development landscape. It is a multi-paradigm language that supports both procedural and object-oriented programming, making it versatile for various applications. Understanding C++ can open numerous pathways for beginners and experienced programmers alike. This section focuses on the foundational elements of C++ that every learner should grasp to appreciate the language's potential and significance in modern programming.
Historical Context
C++ was created by Bjarne Stroustrup at Bell Labs in the early 1980s. It evolved from the C programming language, introducing an object-oriented structure to enhance software development. The initial version, titled
Setting Up the Environment
Setting up the development environment is one of the first essential steps when learning C++. This phase involves preparing the tools that will help you to write, compile, and debug your code. Selecting the right IDE, installing the appropriate compiler, and establishing your first project lays the groundwork for your journey into C++ programming. Mistakes made during this phase can hinder your progress later, making this section crucial for all learners aiming for depth in this language.
Choosing an IDE
Choosing an Integrated Development Environment (IDE) is a significant part of setting up your C++ environment. An IDE consolidates various tools into one interface, allowing for easier management of coding projects. A few popular choices include Microsoft Visual Studio, Code::Blocks, and Clion. Each has its pros and cons that need consideration.
Consider factors like ease of use, community support, and features such as code completion and debugging tools. An effective IDE can increase your productivity and minimize friction while coding. This helps you to learn more efficiently. For instance, depending on the type of project you're embarking on, using Visual Studio might be more beneficial if you're developing applications for Windows since it has optimization features specific for the OS.
When selecting an IDE, keep in mind personal preference: try a few out and see which fits your workflow the best.
Installing the Compiler
The compiler is a crucial piece of software in C++. It translates your C++ code into machine language, making it executable for your machine. Common C++ compilers include GCC (GNU Compiler Collection) and Clang. Installation procedures vary by operating system.
For Linux Users:
Run the following command in the terminal:
For Windows Users:
Sinstall the MinGW toolkit which offers GCC by downloading from their official site. After install, ensure you add the directory to your system's PATH variable for easy access to the compiler from the terminal.
Keep in mind that version compatibility is key. Always download a version that fits your learning project requirements, as different features might be present in various releases.
Creating Your First Project
After picking an IDE and installing your compiler, creating your first C++ project can be an exciting moment. This will familiarize you with your chosen tools, setting in motion what you learn thereafter.
To begin:
- Open your IDE and select 'Create New Project'.
- Name your project—something simple like 'HelloWorld' does fine for starters.
- Set the project type, usually a Console Application for beginners.
- Write a simple program. Here is an example:
Once you input this, hit the 'Run' button. Seeing the output on the screen is validating and an empowering moment for any beginner programmer.
Remember, this is a learning process. Collecting small victories, such as running your first program, contributes to your experience as a programmer and sets the stage for tackling advanced topics later.
Setting up your environment appropriately is your foundation in learning C++. By dedicating time to understand your IDE decisions, compiler selection, and initial coding practices, you're setting yourself up for greater understanding later in your studies.
Basic Syntax and Structure
Understanding the basic syntax and structure of C++ is crux for anyone handling coding in this language. It lays the foundational blueprint on which all C++ programs are built. This foundation includes aspects like data types, variables, constants and operators, which act as building blocks for writing effective C++ code. Mastery of syntax leads to fewer errors and aids in debugging, ultimately improving the efficiency and understandability of code. Therefore, grasping these principles is essential for those aspiring to become proficient in C++.
Understanding Data Types
Data types in C++ are categorized as fundamental types and derived types. Each data type serves a specific purpose when it comes to storing values in a program. The fundamental types include int, float, char, and double, while derived types consist of arrays and pointers, among others.
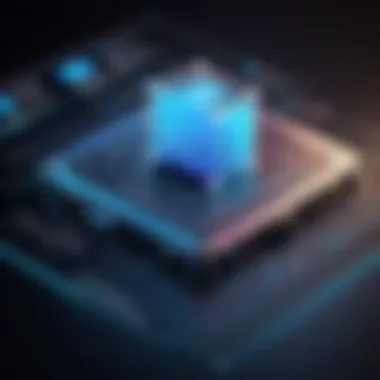
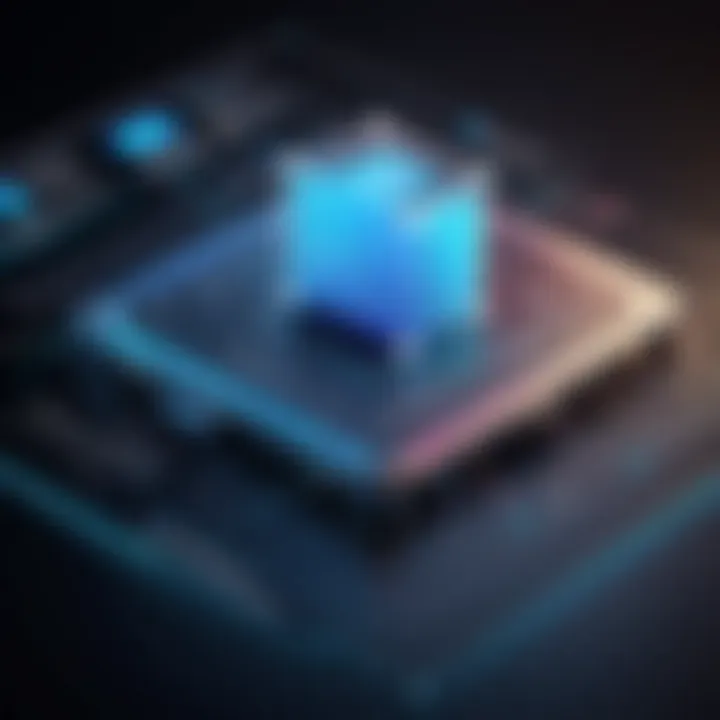
- Int: Stores integers.
- Float: Holds single-precision floating-point numbers.
- Char: Used for storing single characters.
- Double: Used for double-precision floating-point numbers.
- Array: A collection of variables of the same type.
- Pointer: A type that holds memory addresses.
Understanding these types assists programmers in selecting the right data type for their variables to maximize performance and minimize wasted memory resources.
Variables and Constants
In C++, variables and constants are crucial for storing and managing data values during program execution. A variable is an area in memory to hold a value that can change during the program's run-time, while a constant holds a value that remains unchanged throughout its scope.
- Declaring Variables: Variables need to be defined with a data type before they can be used. For example:
- Creating Constants: To declare a constant, the keyword is used. For instance:
Proper usage of variables and constants can prevent unexpected behaviors and enhances code stability, ensuring that inappropriate changes to important values are minimized.
Operators and Expressions
Operators are symbols that perform actions on operands, and they are essential for constructing expressions in C++. C++ provides various operators, including arithmetic, relational, logical, and bitwise operators. Understanding how to use these operators is critical for carrying out computations and making decisions within a program.
- Arithmetic Operators: Used to perform basic mathematical calculations.
- Relational Operators: Compare values and returns boolean results.
- Logical Operators: Combine conditional statements.
- Bitwise Operators: Work with binary values.
In C++, an expression is formed by combining operators and operands. For example, evaluates to the sum of and and assigns this value to . Mastering operators and expressions is vital as it allows you to execute complex calculations and create dynamic branched logic in your programs.
Remember: Knowledge of syntax can lead to better coding practices. Consistent documentation and clean coding not only enhances readability but significantly boosts collaboration within software teams.
Control Structures
Control structures are essential in C++ programming. They dictate the flow of execution of code based on certain conditions, allowing the developer to make decisions during runtime. Understanding control structures are key for writing logical and efficient code. They not only optimize the decision-making process within the program but also enhance its readability. Control structures make code functional and serve as the backbone for developing complex algorithms. The main categories within control structures include conditional statements, loops, and switch statements.
Conditional Statements
Conditional statements are vital for guiding the execution of code based on whether certain conditions are met. The most common form of a conditional statement is the if statement. Using this structure allows the programmer to specify a condition to evaluate; if the condition is true, it executes the block of code within it.
Example of an If Statement:
This code prints a message indicating the number is greater than five when the condition is satisfied. Alongside the if statement, there are additional constructs such as if-else and else if, which enable branching logic for more complex conditions. These constructs can enhance decision-making processes and allow programmers to respond dynamically to changing data.
Loops and Iterations
Loops facilitate the repetition of a set of instructions, which is crucial for handling tasks such as iterating through arrays or collections. The three primary types of loops in C++ are for loops, while loops, and do-while loops. Each serves a specific functionality depending on the requirement of the application.
Example of a For Loop:
In the above example, the for loop iterates five times, printing the current value of at each step. This iterative process is particularly useful for scenarios where the number of repetitions is known beforehand. Utilizing loops efficiently can simplify large tasks and avoid redundancy in code.
Switch Statements
Switch statements provide a streamlined mechanism for executing one out of multiple possible blocks of code based on the value of an expression. They can be more readable than a series of nested if-else statements, especially when evaluating one variable against several values. Each case within a switch statement represents a potential matching condition.
Example of a Switch Statement:
This switch statement prints “Wednesday” when is equal to three. Using switch statements can result in cleaner code in situations with many possible outcomes.
Control structures empower developers to create dynamic, adaptable, and efficient applications. Mastery of these elements is pivotal for achieving programming proficiency.
Understanding and applying control structures properly will enable learners to strengthen their programming capabilities and find solutions to complex problems in C++. Leveraging these tools effectively leads to better practice and robust programming strategies.
Functions in ++
Functions are fundamental to the development of any program in C++. They encapsulate blocks of code that perform specific tasks, making it easier for programmers to organize and maintain their code. Functions promote reusability, reducing redundancy and improving clarity in programming. In this section, we will explore how functions work in C++, including defining functions, function overloading, and recursion, each of which provides unique advantages.
Defining Functions
In C++, functions are defined with a specific syntax. A function consists of a return type, a function name, parentheses containing parameters, and a block of code enclosed in braces. The return type specifies what type of value a function was designed to produce, which can be a fundamental type or a complex object.
Syntax of a Function
Here is a simple example for defining a function:
This function called takes two integer parameters and returns their sum. Understanding how to define a function is a pivotal skill, as it helps structure a program and fosters efficient coding practices. Additionally, each function operates independently, allowing for better debugging and testing. Functions can return values but they are not limited to this; functions can also modify the state, especially when passed parameters in reference.
Function Overloading
Function overloading is a powerful feature of C++. It allows multiple functions to have the same name as long as their parameter lists differ in either type, number, or both. This enables programmers to use the same operation in different contexts without needing to change function names.
For example:
Here, is overloaded to cater for both integers and doubles. The advantage of using function overloading lies in its convenience. It leads to cleaner and more readable code. In scenarios where a certain operation needs to deal with various data types, overloading can be a real boon.
Remember that the return type alone cannot be used for overloading. The compiler depends solely on the function's parameters to distinguish them.
Recursion
Recursion is the practice of a function calling itself to solve a problem. This technique can be very useful for breaking down complex problems into smaller, manageable tasks. The functions continue to call themselves with modified arguments until a certain condition is met, commonly known as the base case. This helps prevent infinite loops which could cause stack overflow.
Here’s a simple illustration through a factorial function:
In this example, calculates the factorial of a number by calling itself with decreasing values. While recursion may introduce complexity in debugging due to multiple function calls, it offers elegant solutions to problems that involve trees or graphs.
The in-depth understanding of these concepts can immensely elevate one's skill set in C++. Engaging with such complex ideas, as they manifest in practical applications, is essential for all programmers aiming toward proficiency. Incorporating diverse function types will fuel efficient coding habits and structured program design.
Object-Oriented Programming Concepts
Object-oriented programming (OOP) is a fundamental aspect of C++, influencing how we design and structure our code. Embracing OOP concepts simplifies complex programming tasks, making them more manageable. The principles of OOP—encapsulation, inheritance, and polymorphism—are essential for writing modular and reusable code.
The encapsulation principle allows the bundling of data and the methods that operate on that data. This offers better control over access and modification patterns, which enhances data security and integrity. Furthermore, using OOP enables programmers to model real-world entities more naturally, contributing to clearer and more organized code.
Classes and Objects
Classes and objects are the core components of OOP. A class serves as a blueprint for creating objects. It defines the properties (or attributes) and methods (or functions) that the object instantiated from the class will possess.
Here’s a simple representation:
In this example, is a class with three attributes: , , and , and one method called . When you create an object from the class, you can access these attributes and methods easily. This organization leads to code that is cleaner, less error-prone, and easier to maintain. You can create multiple objects with different attributes from this single class outline.
Inheritance
Inheritance is a mechanism that allows a new class, known as a derived class, to inherit the properties and behaviors of an existing class, known as a base class. This encourages code reusability and establishes a relationship between classes, which enhances flexibility in code design.
For instance, let’s say there is a base class . You can create a derived class that inherits common attributes like and from while adding specific features like .
With inheritance, maintaining code becomes easier. Changes to the base class will automatically propagate to derived classes, reducing redundancy and the risk of inconsistencies across the codebase.
Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon. Specifically, there are two types of polymorphism in C++: compile-time (method overloading) and runtime (method overriding).
Method overloading occurs when multiple functions have the same name with different parameters within the same scope. For example:
In this example, the method is defined for both and , enabling the same method name to function with different data types.
Polymorphism is key to implementing the concept of interface, not forcing the user to know workinwith details of each object's functionalities, thus promoting lesser coupling.
Method overriding, on the other hand, involves a derived class redefining a method present in the base class. This capability provides a powerful means to tailor class behavior without altering existing code edges.
Memory Management
Memory management is a critical concept in C++ programming. It involves allocating and freeing memory space dynamically to optimize resource use. This topic is of paramount importance, particularly when dealing with large applications or systems where effective memory utilization significantly impacts performance. Programmers need to understand the implications of memory allocation, how to access memory, and ways to avoid leaks that can occur when memory is not properly managed.
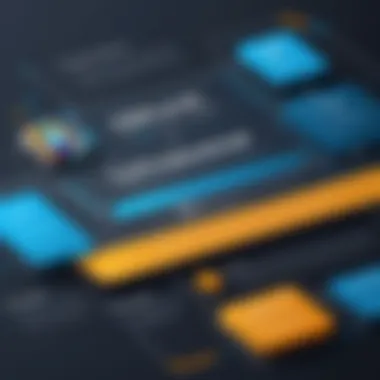
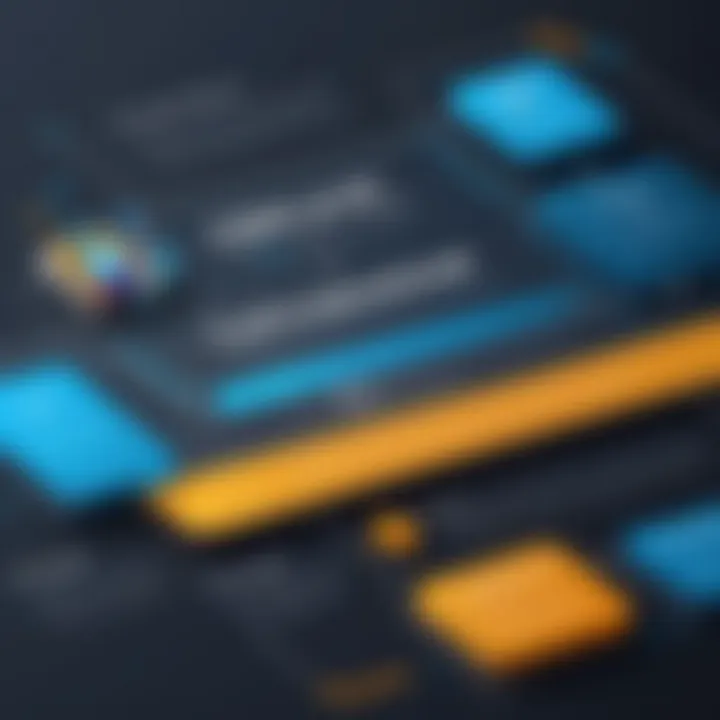
Dynamic Memory Allocation
Dynamic memory allocation allows programmers to allocate memory during runtime using several functions provided in C++. This is essential when the memory space needed can't be determined at compile time. Rather than using fixed-size arrays, dynamic allocation makes it easier to handle data sets of varying sizes.
C++ offers functions like and for this purpose. Using , a programmer can request a specific amount of memory:
When finished, it's necessary to release the memory manually with :
Without correct management, memory leaks can happen. This occurs in situations where allocated memory is not released, consequently causing waste of memory resources.
Effective dynamic memory management is paramount in crafting efficient and reliable C++ applications.
Pointers and References
Pointers and references are fundamental to understand in C++ for managing memory. A pointer is a variable that stores the memory address of another variable. This feature allows for efficient memory usage when dealing with functions and dynamic arrays. For example:
A reference, on the other hand, creates an alias for a variable. It behaves like a pointer but is easier to use and doesn’t require dereferencing. Consider this example:
Both pointers and references allow fine control over memory access and manipulation, which is vital in C++. When working correctly, they help avoid performance issues and promote efficient coding practices.
Destructor and Constructor Usage
In C++, constructors are special member functions which are executed when objects are created. Using constructors allows initializing object properties easily. On the contrary, destructors are similar member functions that are invoked when an object goes out of scope or is deleted. Proper use of constructors and destructors ensures that valuable resources are correctly allocated and freed.
For instance:
Having constructors prepare resource allocation and destructors handle deallocation vastly improves memory management efficiency. Without these principles in place, programmers run the risk of leaving memory allocated, leading to leak problems, hence degrading application performance.
Error Handling
Error handling is a critical aspect of programming in C++. It is essential to manage unexpected situations that can arise during the execution of a program. Without appropriate error handling, programs may crash, leading to data loss and frustration.
Effective error handling allows developers to create robust applications that can gracefully handle exceptions and maintain stability. This teaches learners the importance of anticipating and correcting error scenarios in real-world applications. Therefore, mastering error handling is indispensable for those seeking proficiency in C++.
Exceptions in ++
In C++, exceptions are a mechanism to signal problems or unexpected behavior during program execution. When an abnormal situation arises, such as dividing by zero or attempting to access an out-of-bounds array element, exceptions are thrown. Using exceptions helps keep error-handling code separate from regular business logic.
Types of Exceptions
- Standard Exceptions: These are defined in the standard library. Popular ones are , , and .
- User-defined Exceptions: Developers can create their own exception classes to cater to specific needs, providing flexibility in error handling.
When coding, catch specific exceptions rather than broad classes to have more precise control over error resolution. Proper use of exceptions enhances code clarity and maintainability.
Try-Catch Blocks
Try-catch blocks are the backbone of exception handling in C++. The block contains code that may throw an exception, while the block defines how to handle the exception if one occurs. The syntax is straightforward. Here is a simple example:
In this example, we attempt to access an invalid index within an array, which would result in an exception. The associated error message is captured and displayed instead of crashing the program.
Benefits of Try-Catch
- Separation of Concerns: It keeps error handling separate from actual logic.
- Redux in Crashes: Applications can handle errors without crashing completely.
- Clear communication: It opens a way to notify the user about nature of the error.
Debugging Techniques
Debugging is an essential skill for any programmer. Debugging is the process of locating an error or bug in your code. C++ provides several methods to debug successfully.
Common Debugging Techniques
- Print Statements: Print intermediate states or variable values to analyze flow. Although basic, they effectively simplify complex logic.
- Debuggers: Tools such as GDB can let you step through the program, inspect variables, and analyze the state at various breakpoints.
- Static Analysis Tools: Programs like CLang Static Analyzer identify potential problems in code without needing execution. This proactive approach can catch various issues before execution.
Understanding how to debug effectively can significantly reduce development time and improve code quality.
With the integration of these error-handling tools and techniques, C++ programming becomes more accessible and efficient. Well-structured error handling leads to resilient code that developers can confidently rely upon.
Template Programming
Template programming is a key aspect of C++ that allows for maximizing code reusability and flexibility. It plays an important role in creating functions and classes that operate with various data types, significantly reducing code duplication and enhancing maintainability. Diving deeper into the concept helps to understand the groundwork of generic programming, which is crucial for building more adaptable and scalable systems.
Prelude to Templates
Templates are tools that allow developers to create generic classes and functions. When you define a template, you specify the structure and functionality without tying it to a specific data type. This adaptability empowers a programmer to write code once and utilize it for different data types without rewriting it. There are two primary kinds of templates in C++: function templates and class templates.
Benefits of Using Templates
- Code Reusability: Define once, use multiple times for different parameter types.
- Type Safety: The compiler checks the types used in the templates, which helps in reducing runtime errors.
- Performance Efficiency: Templates can generate optimized code at compile time.
Function Templates
Function templates allow you to create functions that can work with any data type. They offer the ability to generalize functionality while allowing the compiler to create the appropriate types at compile time.
In the example above, the function add is defined as a template that takes two parameters of the same type T and returns their sum. This means that add can perform addition on integers, floats, or any user-defined type provided that the operator is defined.
When to Use Function Templates
- When you have functions that perform the same operation on different types.
- When writing generic algorithms that can work with any container or sequence.
Class Templates
Class templates, just like function templates, provide a way to define classes that will work with any data type. This is especially useful for data structures like linked lists, stacks, and queues.
In the above example, the Stack class is defined as a template, making it able to handle any data type during usage. It uses standard vector to store the elements packed in a safe manner.
Advantages of Class Templates
- Promotes code reuse in creating complex data structures.
- Ensures type safety for both class members and methods.
- Separation of implementation and interface, making systems more manageable.
Template programming in C++ fosters a structured and efficient development practice. Utilizing function and class templates leads to cleaner code and aligns with better coding standards.
Templates serve all developers by allowing flexibility without the steep cost of code redundancy.
Understanding these concepts transforms how programmers approach problem-solving in C++. Templates elevate programming paradigms and ensure developers can deliver robust software solutions.
The Standard Template Library (STL)
The Standard Template Library (STL) is a powerful component of C++ that enhances its capabilities significantly. By providing a collection of template classes and functions, STL simplifies data structure management and algorithm implementation. It allows developers to write efficient and reusable code, which translates to better development speed and improved performance of the applications. Introducing STL into your C++ coding practice becomes essential not just for efficiency, but also for standardization in code structure.
Containers
Containers are the heart of the STL. They are objects that manage the storage of other objects. C++ includes various types of containers to handle different data situations. Common container types such as , , and come with prime features that distinguish them:
- Vector: Suitable for dynamic array functionality. It allows resizing and fast random access, making it ideal for scenarios where array size should change dynamically.
- List: A doubly linked list implementation suitable for situations needing frequent insertions and deletions.
- Map: A sorted associative container that provides a key-value mapping. It allows efficient retrieval based on keys, enhancing searches.
- Set: This container manages a collection of unique elements.
When deciding on which container to use, consider the specific needs of your program, such as the amount of data, required performance, and the frequency of insertions or deletions.
Iterators
Iterators in STL provide a mechanism to traverse containers without exposing their underlying representation. They act much like pointers, enabling access to container elements, with type safety and bound checking. Each container has associated iterators that can be categorized as:
- Input Iterators: Allow reading data from a container.
- Output Iterators: Permit writing data to a container.
- Forward Iterators: Support single-pass traversals in one direction.
- Bidirectional Iterators: Support traversal in both directions.
- Random Access Iterators: Allow direct access to any element in constant time.
Using iterators often results in cleaner and more manageable code. For example, the following demonstrates using an iterator with a container:
Algorithms
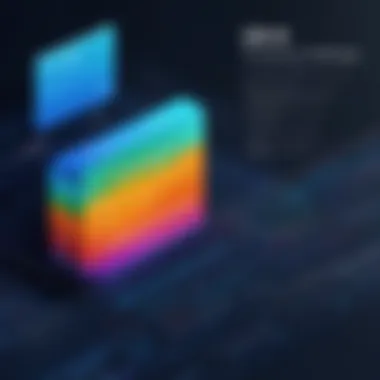
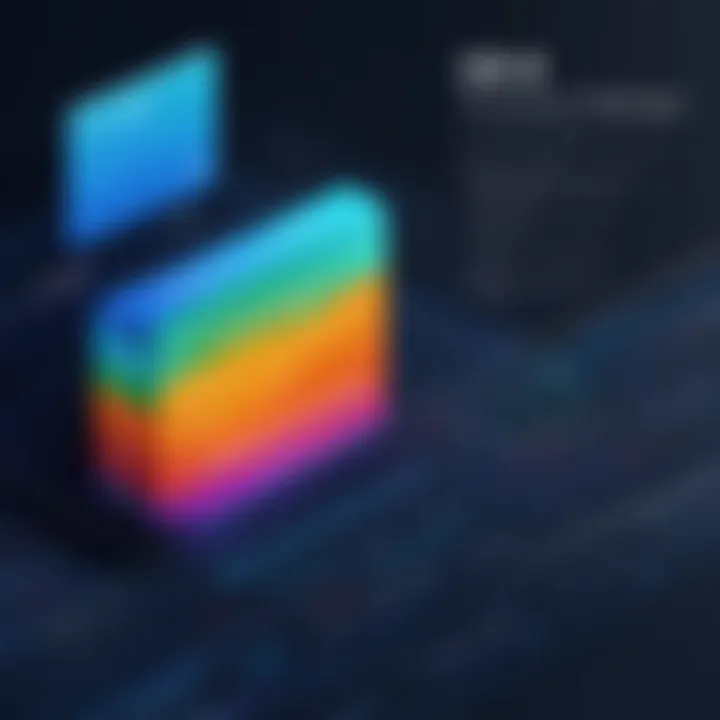
Algorithms are a set of functions that operate on data stored in containers. The power of the STL is amplified through these algorithms, making it possible to perform complex operations with minimal code. Algorithms can be divided into several categories depending on their purpose:
- Sorting: Such as , which arranges elements in ascending or descending order.
- Searching: Algorithms like enable the searching of elements by value efficiently.
- Transformations: These, like , allow you to modify elements based on a specific operation.
- Numeric Algorithms: Functions like facilitate complex mathematical operations.
By simply aligning these algorithms with appropriate containers, developers can create highly efficient and optimized code.
Overall, the intertwining of respected containers, iterators, and algorithms results in a seamless and powerful library that is key to proficient C++ programming. As such, mastering the STL is not just advisable but necessary for serious programming endeavors in C++.
Practical Applications of ++
C++ has played a crucial role in many fields due to its performance and flexibility. Understanding its applications is essential for grasping its importance in the programming world. Let’s explore some key areas where C++ is frequently used. These practical applications provide context and relevance to learning the language.
Game Development
C++ dominates the gaming industry. It's favored for its performance and memory management, permitting the development of resource-intensive and complex games. The functionality and control provided by C++ make it easy to optimize code, which is essential for rendering graphics efficiently.
Common engines like Unreal Engine utilize C++ as their primary programming language. Game developers often leverage the language for creating game logic, performing real-time physics, and rendering, thanks to its powerful libraries and tools.
Key Benefits of ++ in Game Development:
- Performance: C++ enables finer control over system resources, enhancing performance dramatically.
- Object-oriented Design: It supports OOP concepts, useful for managing game states and behaviors.
- Cross-Platform Capability: Developers can create games that run on multiple operating systems.
"Performance optimization is crucial in game development; C++ provides the tools to achieve this goal efficiently."
System Programming
C++ is also a popular choice for system programming. Many operating systems, drivers, and networking software are built using it. Its close-to-hardware programming ability maximizes efficiency while ensuring low-level access to system functions.
When developers need to create software that operates closely with hardware or requires efficient memory management, C++ shines. Operating systems such as Windows and Linux have components developed in C++. This closeness to the hardware allows the end product to run faster while consuming less memory resources.
Considerations for Using ++ in System Programming:
- Memory Management: Developers control memory with pointers and references. Mistakes here can lead to leaks or buffer overflows.
- Embedded Standards: Using C++ requires adherence to stringent standards.
Embedded Systems
Embedded systems benefit substantially from the use of C++. The language's efficiency and speed make it a perfect fit for applications with resource constraints. Devices like microcontrollers, medical devices, and industrial machines incorporate C++ due to these attributes.
In embedded systems, responsiveness and predictability are vital. Thus, C++ provides a good balance between high-level abstractions and low-level interactions, suitable for resource-constrained environments but needing high-level features.
Reasons to Use ++ in Embedded Systems:
- Deterministic Performance: This ensures that systems respond reliably and consistently under various conditions.
- Rich Libraries: C++ provides various libraries that help in building efficient and scalable solutions.
Understanding these practical applications of C++ helps programmers appreciate how their skills can translate into real-world solutions across multiple industries.
Challenges in ++ Programming
Understanding the challenges in C++ programming is essential in both mastering the language and building robust applications. C++ can be complex due to its rich features and intricate details. While the language offers flexibility and efficiency, many programmers face difficulties that can hinder their proficiency. Recognizing these challenges early serves as a key step in overcoming them, thus promoting better programming practices.
Common Mistakes
C++ programmers often fall into certain traps, which can lead to frustration and, ultimately, software bugs. Here are a few common mistakes to be aware of:
- Uninitialized Variables: Failing to initialize variables can lead to unpredictable behavior. Always ensure that your variables hold an expected value before use.
- Memory Leaks: Improper memory management can cause applications to use more resources over time. This can be remedied by using smart pointers and following RAII (Resource Acquisition Is Initialization) principles.
- Neglecting Exception Safety: Throwing exceptions without a strong error handling strategy can lead to resource leaks. It's crucial to implement try-catch blocks adequately.
Being mindful about these common mistakes helps in avoiding sets of more severe inherit problem later in the programming life cycle.
Performance Issues
Performance is a critical criterion in C++, especially for system-level programming and high-demand applications. Some typical performance issues include:
- Inefficient Use of Memory: C++ provides a variety of data structures, but not using them efficiently could lead to increased overhead. Using appropriate containers such as vectors or lists can optimize space and speed.
- Excessive Use of Virtual Functions: While polymorphism offers significant flexibility, overusing virtual functions can degrade performance due to additional overhead of dynamic binding.
- Inefficient Algorithms: Not applying the right algorithm can make programs much slower. Being aware of algorithm complexity and optimizing them enhances performance significantly.
Addressing these performance issues prevent potential bottlenecks in heavily loaded programs.
Resource Management
Effective resource management in C++ is paramount because improper handling can lead to runtime crashes or slower execution. Key points to consider include:
- Manual Memory Management: Unlike languages with garbage collection, C++ requires explicit resource handling. Using and correctly is a must, but implementing smart pointers (, ) can simplify this process.
- Ensuring Thread Safety: When programming in multi-threaded contexts, race conditions must be avoided. Proper use of mutexes and condition variables will help control access to shared resources.
- Scoped Resources: By utilizing RAII, resources are governed automatically, waiting for their “scope” to expire and then released.
Good resource management prolongs the application lifespan and offers better stability.
Continual focus on managing complexities in C++ can elevate a developer’s capabilities and increase confidence with the language.
Future Trends in ++
The landscape of programming languages is constantly changing. C++ is no exception. Discussing Future Trends in C++ illuminates the path the language is taking, ensuring that developers remain relevant. New technologies emerge at a rapid pace. They often shift how we utilize languages. For C++, understanding these trends can reveal new opportunities and challenges.
Emerging Technologies
Recent years have seen an explosion in new technologies that directly impact how C++ is used. Here are a few key areas:
- Artificial Intelligence and Machine Learning: C++ has found a place in the implementation of various AI algorithms. Performance-critical applications benefit greatly from C++ efficiency.
- Internet of Things (IoT): With devices becoming more interconnected, C++ supports lightweight applications crucial for resource-limited devices. The combination of C++ with real-time systems continues to grow.
- Quantum Computing: Although still in its infancy, C++ may have applications in quantum programming and simulations. As the need for more robust software grows with these technologies, C++ may adapt to meet those needs.
The ability for C++ to integrate with these emerging technologies makes its knowledge incredibly valuable for aspiring developers.
++ Standards Evolution
C++ has been evolving for decades. Each new standard introduces features that adapt to modern programming paradigms. The move from C++11 to C++20 has shown a commitment to continuous improvement. Some highlights include:
- Modules: Make code easier to manage and reuse. This can reduce compilation times.
- Coroutines: Help in managing asynchronous programming, simplifying the work developers need to do.
- Enhanced Standard Library: New algorithms and data structures create more functionality right out of the box.
Staying updated with C++ standards ensures that developers can leverage new features and write effective code. In the current environment, keeping pace with the standard's evolution is crucial for long-term success.
Industry Demand Insights
Understanding industry demand is vital for anyone learning C++. Here is what the current job market indicates:
- Increased Demand for C++ Coders: Driven by industries such as game development, finance, and embedded systems, the demand is robust. Companies look for programmers who can optimize performance.
- Cross-Platform Development: Those skilled in C++ can work across various platforms. The ability to write portable code remains an attractive feature.
- Legacy Code Maintenance: Many existing systems rely on C++. Consequently, firms are keen on hiring developers familiar with older standards. This skill allows them to effectively manage and improve legacy systems.
The key takeaway is that understanding future trends positions developers strategically in the job market, particularly in C++.
In summary, Future Trends in C++ encompass emerging technologies, ongoing standards evolution, and insights into industry demands. Staying informed allows developers to harness C++ effectively, ensuring relevance in a dynamic field.
Resources for Further Learning
In the constantly evolving landscape of programming, resources for further learning play a crucial role for students and individuals diving into C++. Access to knowledge is invaluable. Proper resources ensure that learners can deepen their understanding. In this section, we will explore different types of resources to assist in continued education regarding C++. Educational materials have a significant impact on one's coding journey.
Understanding the depth of what C++ can offer is vital. The right resources provide different avenues for acquiring knowledge. Readers seeking to advance their skills in C++ should utilize a mix of materials. It enhances the learning experience. Below are subcategories that highlight key resources available for anyone wanting to learn C++.
Books and E-books
Books and e-books are foundational elements in the education of C++. Many authors break down complex theories into manageable principles. These texts often act as benchmarks in the coding community. There are classics such as “The C++ Programming Language” by Bjarne Stroustrup and “Effective C++” by Scott Meyers. Utilizing traditional or electronic formats can significantly improve comprehension.
Benefits of books include:
- Comprehensive knowledge
- Effective strategy of study
- ISBN reference numbers provide updates
Reading varies from person to person, yet much accumulated insight comes from solid textbooks.
Online Courses and Tutorials
Online courses and tutorials have gained a foothold in modern education. Digital platforms offer various methods, such as video lessons, assignments, and interactive assessments. Websites like Udemy or Coursera provide diverse options tailored for different levels. Such mediums allow for a more flexible learning pace—for example, students can start courses like
Culmination
The conclusion of this guide emphasizes the significance of grasping C++ programming fundamentals nested within practical and theoretical frameworks. Understanding C++ involves not only learning its syntax and functions but also exploring its practical applications and nuances. This article has meticulously outlined the path you need to follow for building a solid understanding in C++. Essential components, such as control structures, dynamic memory management, and error handling, have wide-ranging implications in developing efficient applications.
From object-oriented programming principles to utilizing the Standard Template Library, the guide has aligned each topic to support your growth from beginner to more advanced programmer competencies. By highlighting particular challenges and upcoming trends in C++, you are encouraged to navigate your learning journey with an informed perspective. Encouraging this holistic understanding may enhance your ability to tackle real-world problems efficiently.
Mastering C++ equips you not just with knowledge of a programming language but broadens your problem-solving capabilities, making you a valuable asset in the tech industry.
Recap of Key Points
Recapping the key points discussed within the article:
- Historical Context: Acknowledging the evolution of C++ shows how its features have matured over time.
- Core Features: Key aspects, such as syntax and functions, stand as foundations for writing effective code.
- Important Concepts: Understanding constructs like loops, conditionals, and templates improves code efficiency.
- Memory Management: Proper resource usage that prevents leaks is critical in C++开发.
- Error Handling: Implementing try-catch blocks to manage exceptions contrasts with unassisted error processes.
- Practical Applications: Visualizing C++ in-game development, system programming, and other domains highlights real-world relevance.
- Future Trends: Awareness of evolving standards keeps your skills contemporary in a fast-paced field.
Thus, covering these aspects adds dimensions to your grasp of coding in C++.
Final Thoughts on Learning ++
In learning a language like C++, persistence is as significant as theoretical understanding. Keeping focused on building a structured knowledge base transforms abstract concepts into tangible skills. Insights from this guide advocate for continuous practice through exercises, projects, and real-world applications.
- Incorporate feedback: Engage with coding communities like reddit.com or dedicated forums to receive constructive criticism of your code.
- Utilizing resources: Supplement your journey with noteworthy books and online tutorials to enhance learning efficacy.
- Stay updated: Just as technology evolves, so should your skills with regular learning and exploration.
Every coder's journey is unique. Embrace the braces C++ as a friendly enabler, opening pathways to greater understanding in computer science. By consciously practicing and applying concepts, you not only acquire competence in C++ but also enrich your overall programming prowess.