Comprehensive Guide to C++ Literature for All Levels
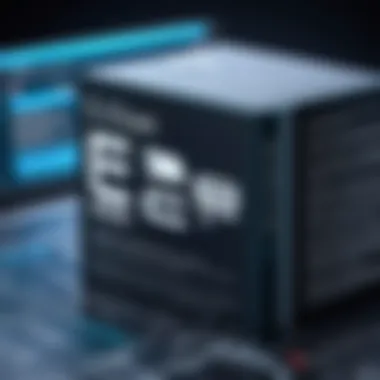
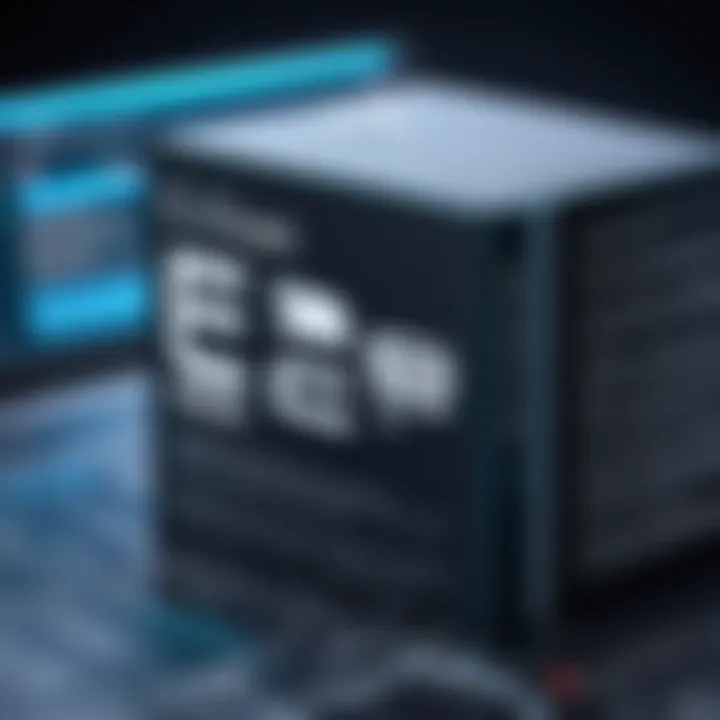
Foreword to Programming Language
Understanding C++ begins with grasping its historical roots and context. C++ was created in the early 1980s by Bjarne Stroustrup at Bell Labs. It evolved from the C programming language, which was developed in the 1970s. Stroustrup aimed to create a language that combined the efficiency of C with the capability for abstraction found in other programming languages. C++ thus became a multi-paradigm language supporting both procedural and object-oriented programming.
History and Background
Over the years, C++ has undergone numerous revisions, leading to the release of standardized versions like C++98, C++03, C++11, and more recently, C++20. Each iteration brought enhancements, including new language features and efficiencies. This evolution has kept C++ relevant in a landscape that includes dynamically typed and interpreted languages. Its robustness makes it suitable for system/software development, game programming, and high-performance applications.
Features and Uses
One of the standout features of C++ is its support for object-oriented programming, which enables modular and reusable code. Other significant features include strong type checking, low-level memory manipulation, and a rich Standard Template Library (STL). The range of applications extends from operating systems to desktop applications and even mobile applications. Programming with C++ allows developers to optimize performance critically, making it a preferred choice in areas like gaming, finance, and embedded systems.
Popularity and Scope
C++ continues to be widely used across various industries. According to Tiobe Index and RedMonk's rankings, C++ consistently ranks among the top programming languages. This stability in popularity suggests a reliable future for C++, as businesses acknowledge its importance for performance-driven software.
C++ strikes a balance between efficiency and control, making it indispensable for many applications.
In summary, C++ stands as a pivotal player in the world of programming languages. Its history highlights its resilience and adaptability, while its features and widespread use underscore its significance in contemporary software development. Understanding C++ literature is crucial for anyone looking to delve deeper into programming.
Basic Syntax and Concepts
C++ introduces various foundational concepts crucial for mastering the language. Learning these basics enables students and new programmers to build more complex applications confidently.
Variables and Data Types
Variables in C++ are essential for storing values. Each variable type—such as , , , and —serves distinct purposes. For example, an stores integer values, whereas a is suitable for decimal numbers. Here is a basic declaration:
Operators and Expressions
Operators in C++ include arithmetic, relational, and logical operators. They help manipulate data and are vital for constructing expressions. Common arithmetic operations include addition (+), subtraction (-), multiplication (*), and division (/).
Control Structures
Control structures dictate the flow of a program. C++ provides several mechanisms, such as statements, , and various types of loops. For example, a simple construct would look like this:
Advanced Topics
Once the fundamentals are mastered, learners can explore advanced concepts in C++, which are essential for building sophisticated applications.
Functions and Methods
Functions in C++ facilitate code reuse and organization. They break down a task into smaller, manageable parts. A simple function could look like:
Object-Oriented Programming
At the heart of C++ lies object-oriented programming (OOP). This paradigm revolves around the concepts of classes and objects. It promotes data encapsulation and inheritance, both crucial for creating scalable applications.
Exception Handling
C++ includes mechanisms for handling errors and exceptions. The use of , , and keywords allows developers to manage errors gracefully, crucial for creating robust applications. For example:
Hands-On Examples
Practical exposure solidifies theoretical knowledge. Engaging with hands-on examples is essential for adapting to real-world programming challenges.
Simple Programs
Beginning with straightforward programs can build confidence. An example includes a basic calculator that performs addition and subtraction.
Intermediate Projects
Progressing to intermediate projects like creating a text-based game can improve skills significantly. Here, users can apply concepts from both syntax and OOP.
Code Snippets
Sharing useful code snippets among peers fosters a collaborative learning environment. This practice can lead to efficiency and innovation in coding.
Resources and Further Learning
Learning C++ extends beyond reading. Numerous resources are available for those wishing to deepen their understanding further.
Recommended Books and Tutorials
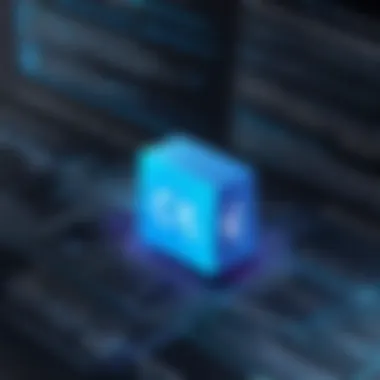
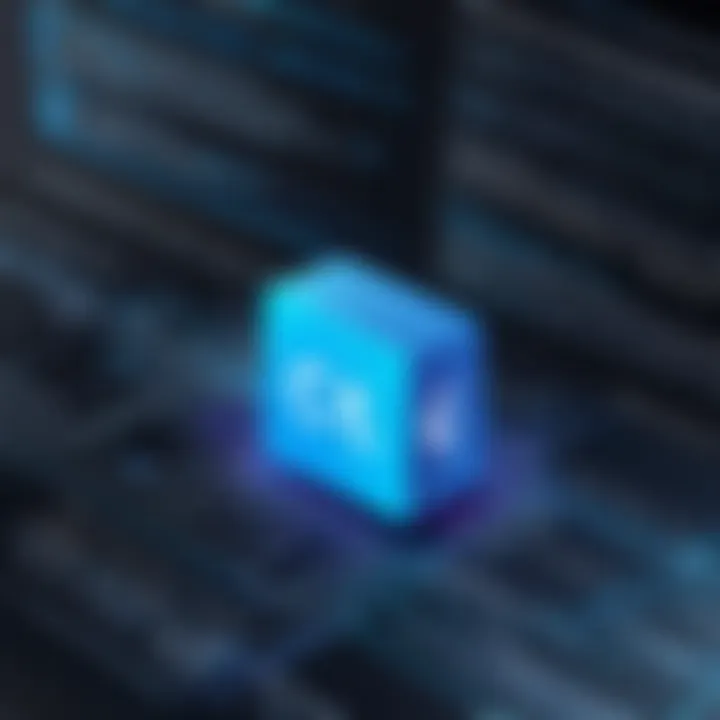
Several authoritative texts exist for learning C++. Noteworthy books include "The C++ Programming Language" by Bjarne Stroustrup and "Effective C++ by Scott Meyers. These texts provide both foundational knowledge and advanced techniques.
Online Courses and Platforms
Platforms like Coursera, Udacity, and edX offer courses tailored to C++. These platforms often include hands-on projects, which are invaluable for retaining knowledge.
Community Forums and Groups
Engaging with the community through forums like Reddit, Stack Overflow, and various Facebook groups creates a network of support. It is an excellent way to share experiences, troubleshoot challenges, and learn from others.
Preamble to ++ Literature
Understanding C++ literature is crucial for anyone serious about mastering the language. The wealth of resources available caters to various learning levels, from novices to experts. This section sets the foundation for the guide by emphasizing how the right books and materials can influence one's programming journey.
Importance of Choosing the Right Books
Selecting appropriate literature for learning C++ is not just beneficial; it is essential. A single book may emphasize one perspective or style of coding while another might excel in practical applications. The right literature can build a strong base, promote best practices, and ignite creativity. Furthermore, the accessibility of complex concepts in diverse formats is pivotal for effective learning. Readers should consider factors such as clarity of explanation, author credentials, and relevance to current C++ standards.
Here are some specific benefits of choosing well:
- Clarity: Well-written texts demystify challenging ideas.
- Updated Content: C++ evolves over time; recent books reflect the latest standards and practices.
- Diverse Approaches: Different authors offer unique insights and examples that enhance understanding.
Investing time in evaluating options can lead to a more engaging and fruitful learning experience. An informed choice pays dividends in reinforcing skills and confidence.
Overview of ++ as a Language
C++ is a versatile and powerful programming language. It was developed in the early 1980s by Bjarne Stroustrup. C++ combines the efficiency and flexibility of low-level programming with the features of high-level languages.
Several characteristics make C++ distinct:
- Object-Oriented: C++ supports encapsulation, inheritance, and polymorphism, making it ideal for complex software design.
- Performance: It is designed to work close to the hardware, offering fine control over system resources.
- Rich Standard Library: C++ provides a comprehensive library, enabling code reuse and enhanced productivity.
- Cross-Platform: It runs on various platforms, increasing its applicability.
For practitioners, C++ provides tools to manage and solve real-world problems. Understanding its features is paramount to effectively utilizing the resources available in C++ literature.
"C++ is not just a language but a toolkit that opens the door to creativity in programming."
In summary, familiarity with C++ as a language will pave the way for making informed decisions about which resources to engage with and how to navigate the extensive C++ literature.
Foundational ++ Textbooks for Beginners
The journey into programming often begins with the right literature. Foundational C++ textbooks lay the groundwork for beginners. These books are crucial because they introduce the basic concepts and syntax of C++, an important programming language used in software development, system programming, and game development. A structured approach to reading these texts allows learners to grasp core principles effectively, which helps in solving real-world problems.
Moreover, foundational texts provide benefits like clear explanations, practical examples, and exercises that reinforce learning. Readers can build confidence as they practice and implement concepts into their work. When considering which textbooks to explore, it’s wise to look for resources that not only explain theory but also encourage experimentation with code.
In these foundational resources, beginners should seek clarity, pedagogical effectiveness, and a balance between theory and practice. Understanding these elements leads to an informed choice of texts, thereby enhancing one's coding proficiency.
Essential ++ Programming by William F. Pugh
"Essential C++ Programming" by William F. Pugh serves as a solid entry point for programmers new to C++. Pugh's concise explanations and structured approach cater to beginners as they navigate through the complexities of the C++ language. The book, while simple, is comprehensive enough to cover essential principles.
Notably, it contains examples that connect theory to practice, making it easier to grasp concepts. Readers appreciate the author's focus on real-world applications, allowing new programmers to see the relevance of what they learn. Additionally, its use of clear language is beneficial for those who might struggle with technical jargon, thereby promoting accessibility.
++ Primer by Stanley B. Lippman
Stanley B. Lippman's "C++ Primer" is a distinct standout among C++ literature. It's often recommended for its thorough approach to teaching C++. The book effectively balances detail and clarity, guiding readers from beginner to more advanced topics.
Lippman emphasizes both the C++ language and programming principles. This holistic viewpoint helps readers understand not just how to code, but why certain practices are essential. Each chapter concludes with exercises that reinforce learned concepts, ensuring a hands-on approach. Its detailed explanations support various learning styles, making it a widely respected text in C++ literature.
Accelerated ++ by Andrew Koenig
"Accelerated C++" by Andrew Koenig takes a unique approach by immersing readers in practical programming from the beginning. This book is designed not only to teach the syntax of C++, but to instill a mindset of effective software design. Koenig begins with essential programming elements, advancing quickly to more sophisticated topics.
Readers often remark on the book's ability to challenge their understanding while remaining manageable for beginners. The exercises encourage experimentation and exploration, which are vital for developing coding skills. By focusing on practical applications, this book prepares readers for real-world programming challenges, making it an invaluable resource for newcomers to C++.
"Foundational texts in C++ not only inform but also inspire confidence in coding. They serve as stepping stones to more complex programming projects."
Intermediate Resources for Further Learning
Intermediate resources serve as an essential bridge between beginner texts and advanced material. In the context of C++, they build on the fundamental concepts learned earlier and introduce more complex topics. These materials offer a deeper understanding of C++ programming and its intricacies. For students aiming to enhance their skills, this section emphasizes the value of integrating intermediate literature into their learning journey.
Studying intermediate resources is important for several reasons. First, they cover more nuanced aspects of the language that are crucial for effective programming. Topics such as memory management, object-oriented design, and efficient code practices are often explored in greater detail. Second, these resources generally incorporate practical examples and exercises, allowing learners to apply theoretical knowledge in real-world scenarios. This hands-on approach helps solidify understanding and fosters confidence when tackling larger projects.
When selecting intermediate materials, consider factors such as the author's reputation, clarity of explanation, and alignment with personal learning preferences. Each student's journey will differ due to various learning styles, and some individuals may benefit more from certain types of content over others. Ultimately, integrating these intermediate resources can significantly enhance one’s capability to write robust C++ applications.
Effective ++ by Scott Meyers
"Effective C++" is a notable work by Scott Meyers that provides 55 specific ways to improve the C++ programming style. Each item in the book encapsulates its own principles and best practices, making it a comprehensive guide for programmers who seek to refine their code. The structured yet straightforward approach makes it easy to digest complex topics.
Reading this book helps programmers understand the intricacies of C++. The focus is on getting the most out of C++ features while avoiding common pitfalls. This unique angle allows learners to adopt best practices that enhance the overall quality of their software projects. Not only does it delve into recommended uses of the language, but it also highlights potential missteps.
More Effective ++ by Scott Meyers
Following the success of his first book, Scott Meyers published "More Effective C++" which builds further on the concepts introduced in the original. This work includes 35 additional items that deal with advanced usage patterns and techniques. It explores the bounds of C++ capabilities and encourages programmers to adapt their strategies for tackling complex programming challenges.
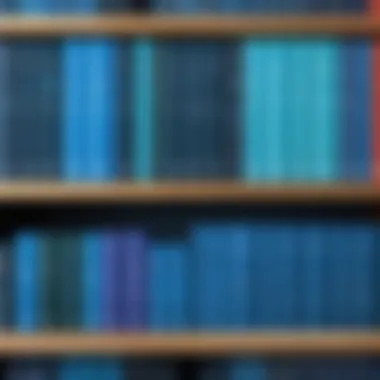
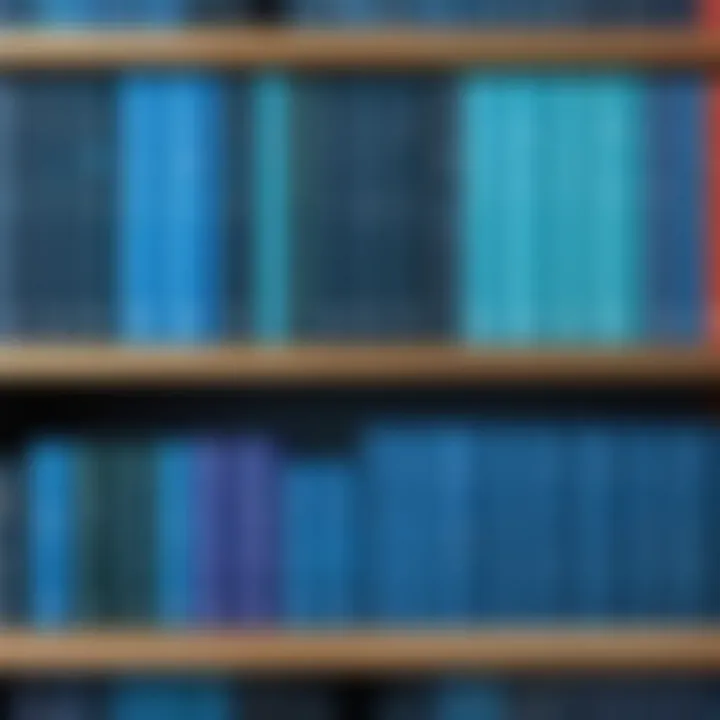
The accessibility of the content is a significant advantage. Each item is self-contained, allowing readers to jump in and out based on relevance. Whether it’s optimizing performance or understanding the subtleties of type safety, this book is a valuable resource for anyone looking to deepen their comprehension of C++. Reading it can substantially enhance one’s programming acumen.
++ Standard Library by Nicolai M. Josuttis
The "C++ Standard Library" by Nicolai M. Josuttis is an authoritative text that examines the vast collection of classes and functions provided within the Standard Template Library (STL). This resource is invaluable for those pursuing mastery in C++. It introduces users to the algorithms and data structures that are standard in modern C++ development, aiding in better application design and performance.
Josuttis covers essential components such as containers, iterators, and algorithms, providing an in-depth perspective on how these elements interact. The explanations are clear and supplemented with practical code examples that illustrate how to effectively utilize these resources in real applications. By studying this material, readers can gain confidence in employing the Standard Library functions to enhance their programming efficiency.
Learning the Standard Library is a major step towards writing modern C++ code that is efficient and readable. Incorporating its features allows developers to streamline code and leverage established best practices.
Advanced ++ Literature
Advanced C++ literature plays a crucial role for those seeking to elevate their programming skills to a higher level. These texts go beyond the basics, addressing complex topics such as concurrency, design patterns, and the nuances of the language itself. The benefit of engaging with advanced resources is not only in acquiring deeper knowledge but also in developing problem-solving capabilities. Advanced literature addresses real-world programming challenges, thus pushing learners to apply concepts in practical scenarios.
Focusing on advanced literature strengthens understanding of C++, which is not merely about syntax but also about applying abstract concepts to develop efficient, high-quality code. As the programming landscape evolves, a strong grasp of advanced topics is necessary to remain competitive. Furthermore, these resources often provide insights into best practices and advanced techniques that can greatly enhance one's programming portfolio.
++ Concurrency in Action by Anthony Williams
C++ Concurrency in Action is a seminal work that tackles the intricate subject of multi-threading and concurrent programming in C++. Written by Anthony Williams, this book is essential for programmers who want to master the complexities of concurrent programming.
The significance of this book lies in its practical examples and clear explanations. Williams adeptly balances theoretical discussions with applicable coding instructions. Key elements covered include mechanisms for creating threads, managing data, and ensuring thread safety. The concepts within this book equip developers with the ability to build responsive and efficient software systems that adequately utilize multi-core architectures, which is increasingly common in modern computing environments.
The ++ Programming Language by Bjarne Stroustrup
Authored by Bjarne Stroustrup, the creator of C++, The C++ Programming Language is monumental in the realm of C++ resources. This comprehensive guide provides a thorough exploration of the language, including its features, principles, and potential pitfalls.
This book's importance is underscored by its authoritative approach. Stroustrup explains not only how to use C++, but also the rationale behind the design decisions that shaped the language. Readers gain insights into object-oriented programming, templates, and the Standard Template Library. This understanding is paramount for creating robust and scalable software. The book serves as both a reference and a learning tool, making it a staple for anyone serious about C++.
Modern ++ Design by Andrei Alexandrescu
Modern C++ Design by Andrei Alexandrescu introduces advanced design techniques such as generic programming and design patterns in C++. Alexandrescu encourages programmers to think about flexibility and reusability when designing code.
One of the key contributions of this work is the introduction of policy-based design which facilitates the combination of object-oriented programming with template metaprogramming. Alexandrescu provides practical applications alongside theoretical frameworks, enabling readers to grasp abstract concepts more concretely. This text is invaluable for developers aiming to enhance code quality and maintainability, pushing the boundaries of what can be achieved with C++.
“Understanding the depth of C++ empowers you to write not just faster code but also clearer and maintainable software.”
In summary, engaging with advanced C++ literature is essential for developers who wish to excel in their field. These texts provide invaluable insights and examples that help refine skills and broaden perspectives on programming concepts.
Specialized ++ References
Specialized C++ references play a critical role in advancing knowledge and skills in C++. These books provide in-depth insights into specific areas of C++ programming, allowing learners to deepen their understanding of complex topics. They often bridge the gap between theory and practical application, making them invaluable for both aspiring programmers and experienced developers.
Selecting the right specialized book can enhance a programmer’s ability to solve particular problems. Topics like templates, numerical computations, and design patterns are essential in professional development. They enrich the programmer's toolkit, enabling them to handle diverse programming challenges efficiently.
++ Templates: The Complete Guide by David Vandevoorde
"C++ Templates: The Complete Guide" by David Vandevoorde is an essential text for anyone wanting to master the use of templates in C++. Templates allow for generic programming, a powerful feature that promotes code reusability and flexibility. This book goes beyond the basics, detailing advanced template techniques and their implications.
The book covers essential concepts such as template specialization, SFINAE (Substitution Failure Is Not An Error), and how templates interact with the C++ type system. Readers will gain insights into writing template code that is both effective and efficient. Thus, this text is significant for developers who are focused on writing high-performance applications.
Numerical Recipes in ++ by William H. Press
William H. Press’s "Numerical Recipes in C++" is a profound resource for any programmer interested in scientific computing. This book provides a comprehensive set of algorithms for numerical analysis, along with practical implementations in C++.
The contents cover a wide range of topics, including linear algebra, optimization, and statistical analysis. Each method is explained clearly, with code examples that facilitate understanding. This text serves not only as a reference but also as a guide to applying numerical methods effectively in real-world problems. Professionals in fields like engineering and data science find this book particularly valuable.
Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma
"Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma and co-authors delves into proven solutions for common software design problems. This book is essential for any programmer who wishes to build robust and maintainable systems.
The authors present the concept of design patterns, which are standard solutions to recurring design issues. It elaborates on patterns like Singleton, Observer, and Factory, providing clear descriptions and practical code examples. Understanding these patterns can significantly improve a programmer's ability to design scalable applications, making this text an indispensable tool in software development.
In the realm of software engineering, mastering design patterns is akin to wielding an advanced toolkit, enabling one to craft solutions with elegance and efficiency.
These specialized references provide specific knowledge valuable for deepening expertise in various aspects of C++. Incorporating them into your reading list allows for a more rounded understanding of the language, fostering skills that are essential in today's complex programming landscape.
++ in Practice: Project-Based Learning
Project-based learning holds immense significance for mastering C++, particularly because it reinforces the theoretical knowledge obtained through textbooks. Engaging with real projects allows learners to apply concepts practically. This hands-on approach fosters a deeper understanding of the language by bridging the gap between theory and real-world application. Developing projects helps reinforce problem-solving skills, critical thinking, and creativity. The benefits derived from such an approach cannot be overstated. Here are some key aspects and considerations in project-based learning for C++:
- Practical Application: Engaging in projects necessitates the use of C++ in realistic contexts, clarifying how the language is employed in software development.
- Skill Development: Projects encourage the honing of specific skills like debugging, code optimization, and understanding libraries.
- Portfolio Building: Completing projects adds valuable pieces to a programmer's portfolio. This is critical for job applications or showcasing capabilities to potential employers.
- Collaboration Opportunities: Many projects require teamwork, enhancing collaborative coding skills.
Overall, project-based learning in C++ is not just about writing code. It's about fostering an in-depth comprehension of programming principles, exposing learners to challenges they will face in actual professional environments.
++ Programming: Program Design Including Data Structures by D.S. Malik
D.S. Malik's book is a significant resource for learning C++ in a structured format. The text focuses on program design and includes an emphasis on data structures. The clear organization and pedagogical approach make complex subjects approachable. Malik integrates data structures seamlessly with C++ concepts, allowing learners to not just memorize but also to understand their application within the language.
Students will appreciate the detailed coding examples and exercises, which reinforce learning and provide immediate feedback on understanding. This resource is particularly beneficial for beginners, as it lays a solid foundation in both programming and problem-solving using C++. The real-world examples depicted throughout the book drive home the relevance of the material.
Game Programming Patterns by Robert Nystrom
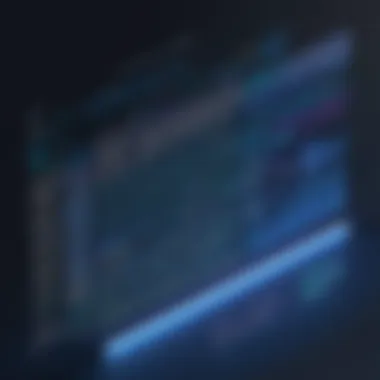
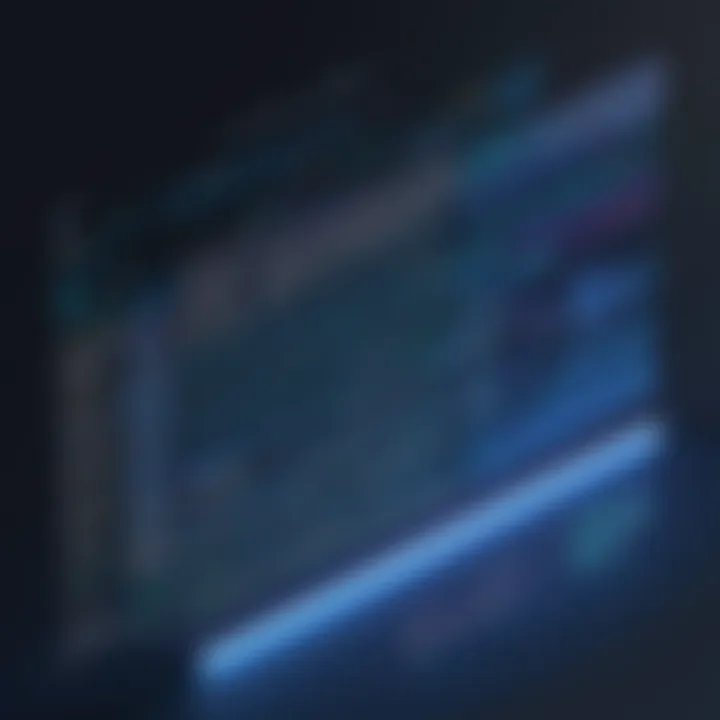
Robert Nystrom's Game Programming Patterns diverges from standard C++ textbooks by offering insights specific to game development. It provides a series of solutions to common problems in game programming, examined through C++ implementations. Understanding design patterns can fundamentally change how a programmer approaches problems.
The text delves into topics such as the observer pattern, state pattern, and command pattern, among others. Each pattern is presented through a pragmatic lens, with examples that clarify how one might implement them in a game engine. This resource is excellent not only for learners seeking to understand C++ but also for those wishing to specialize in game development.
Beginning ++ Through Game Programming by Mike Dawson
Mike Dawson's Beginning C++ Through Game Programming serves as an entry point for individuals new to both C++ and game development. The text is intentionally designed to engage learners by coupling programming skills with game creation. Dawson adopts a step-by-step approach, which makes it easier for readers to follow along.
This book covers important C++ fundamentals while gradually introducing game programming concepts. By guiding readers through small projects, it assists in retaining concepts in a lively and enjoyable manner. Those interested in both C++ and games will find this book particularly engaging. It serves to inspire confidence in coding while imparting essential gaming software development skills.
"The best way to learn programming is by doing. Engaging in actual projects cements knowledge and fosters creativity."
In summary, project-based learning through C++ literature provides invaluable experiences and opportunities for growth. Engaging with resources such as C++ Programming: Program Design Including Data Structures by D.S. Malik, Game Programming Patterns by Robert Nystrom, and Beginning C++ Through Game Programming by Mike Dawson empowers learners to explore their capabilities and deepen their understanding of C++.
Online Resources Complementing ++ Literature
Online resources have become an integral part of studying C++. As programming continues to evolve, supplementary materials available on the internet provide timely updates and diverse perspectives. The inclusion of online resources enhances the learning experience, making it more interactive and adaptable to different learning styles. They offer various modes of engagement, from tutorials to community discussions.
Online Tutorials and Documentation
Online tutorials are essential for grasping C++. They often break down complex concepts into manageable parts, which is beneficial for learners at all levels. The programming language's official documentation, available on websites like cppreference.com, is valuable for understanding syntax and library functions in depth. Furthermore, w3schools and learncpp.com provide structured paths for beginners.
When choosing tutorials, consider the learning method most effective for you. Some may prefer video formats, while others may find written guides clearer. An interactive environment, such as coding platforms like Codecademy or freeCodeCamp, allows for hands-on practice, reinforcing learning through application. These resources often include coding exercises, which enhance retention and understanding.
Forums and Community Resources
Forums such as Reddit’s r/cpp or Stack Overflow offer platforms for discussing challenges and sharing knowledge among peers. These forums serve as a vast repository of real-world problems and solutions. Users can ask questions and get responses from experienced developers, which helps in problem-solving.
Community resources also include tutorials shared by individuals who publish their solutions to commonly faced issues. Participating in discussions not only builds understanding but connects learners who share similar interests. Collaboration fosters a sense of belonging within the programming community, encouraging more continuous learning.
Webinars and Video Courses
Webinars and video courses provide a visual and auditory learning experience. Platforms like Coursera and Udemy offer high-quality courses on specific C++ topics taught by industry experts. These resources often feature interactive elements, such as quizzes or assignments, which can enhance the learning experience.
Watching coding demonstrations in video format can make complex topics more accessible. Detailed explanations alongside live coding can clarify concepts that a static text may not convey effectively. It’s also beneficial to seek out free resources, such as YouTube channels focused on programming, where learners can find diverse tutorials and techniques.
"The landscape of learning C++ is continually evolving, with online resources taking center stage in education."
Evaluating the Best ++ Books
Choosing the right C++ books is essential to effectively learn the language. With the vast number of resources available, knowing how to evaluate them can save time and enhance learning. A structured approach to this process ensures that individuals can find books that best meet their specific needs and learning styles. In this section, emphasis will be placed on two main components: the criteria for selecting books and the importance of user reviews and feedback. Each of these elements plays a significant role in the selection process, influencing a learner's trajectory in mastering C++.
Criteria for Selecting Books
When selecting C++ books, certain criteria can streamline the decision-making process. Here are some important factors to consider:
- Content Relevance: The book should align with your learning objectives. Whether you are a beginner or an advanced learner, ensure that the material is suitable for your skill level.
- Clarity and Style: The author's writing style should be clear and engaging. This helps maintain interest and facilitates understanding of complex concepts.
- Updated Information: C++ is an evolving language. Consider books that are current and reflect the latest standards and practices.
- Practical Examples: Look for texts that include practical examples and exercises. This fosters hands-on learning, reinforcing theoretical knowledge through practical application.
- Author Expertise: Research the authors’ backgrounds. Established authors in the field often provide insights based on extensive experience in programming.
- Target Audience: Understand the intended audience of the book. Some resources cater specifically to beginners, while others are designed for experienced programmers.
"Selecting a good book is as vital as the content it delivers; it shapes the learning experience."
User Reviews and Feedback
User reviews and feedback are invaluable when evaluating C++ literature. Reviews can provide insight that goes beyond the publisher's description. Here are ways user feedback contributes to book selection:
- Real-World Insight: Reviews often include personal experiences with the book, offering a perspective on its effectiveness in teaching concepts.
- Strengths and Weaknesses: Feedback typically highlights both strengths and weaknesses, assisting potential readers in making informed decisions.
- Learning Effectiveness: Many reviews discuss how well the book fulfills its purpose in advancing skills or knowledge.
- Community Recommendations: Platforms like Reddit or specialized forums often feature discussions around specific titles, providing a collective opinion from various users.
Finale and Recommendations
The section of Conclusion and Recommendations is vital for the readers to synthesize the information presented in the article about C++ literature. Analyzing and evaluating the cumulative knowledge from various resources allows one to formulate a clear understanding, which is paramount in mastering C++. Each book and resource varies not only in content but also in its pedagogical approach.
When engaging with C++, readers must select the right materials. This is not merely about reading; it is about enhancing practical skills and applying knowledge in real-world scenarios. The recommendations provided here should help any learner navigate their way effectively through the complex landscape of C++ literature.
This article presents a structured framework to encourage informed decisions. As readers conclude their review of the resources, they should weigh factors such as relevance, complexity, and applicability of the texts they choose. A thoughtful selection lays a strong foundation for deeper exploration in the subject.
"The right resources can transform the learning journey, taking it from someone trying to understand a language to someone who speaks it fluently."
Final Thoughts on ++ Studies
C++ research and study go beyond merely understanding syntax and semantics. It demands critical thinking about how to structure code for optimal performance and maintainability. Many learners find themselves overwhelmed by the extensive material available.
However, adopting a structured approach can simplify the process. By focusing on core concepts first, such as object-oriented programming or templates, students build a strong foundation. It is crucial to experiment frequently with code. Try writing and debugging snippets based on the concepts learned in the books. This practice solidifies understanding and leads to mastery over time.
Moreover, as the field evolves, staying updated with new editions and publications can greatly benefit learners. The evolution of C++ often aligns with new standards, which every programmer should be aware of. Continuous education is an integral part of becoming a proficient C++ developer.
Suggested Reading Path for Different Levels
Designing a reading path tailored to different skill levels ensures a well-rounded understanding of C++. Here is a suggested approach:
- Beginners
Start with foundational texts that introduce the concepts and syntax. Recommended books are: - Intermediate Learners
Once comfortable with basics, advance to more complex materials. Consider: - Advanced Practitioners
At this stage, focus on specialized topics:
- C++ Primer by Stanley B. Lippman
- Accelerated C++ by Andrew Koenig
- Effective C++ by Scott Meyers
- C++ Standard Library by Nicolai M. Josuttis
- Modern C++ Design by Andrei Alexandrescu
- C++ Concurrency in Action by Anthony Williams
By following this reading path, learners can gradually build their knowledge and expertise while minimizing confusion. Engaging with community resources or forums can offer additional support and insight as they progress.
In summary, thoughtful consideration of resources and structured progression through literature can significantly enhance one's capabilities in C++. This is essential not just for academic growth but for practical, industry-relevant application.