Mastering Class Declarations in Java Programming
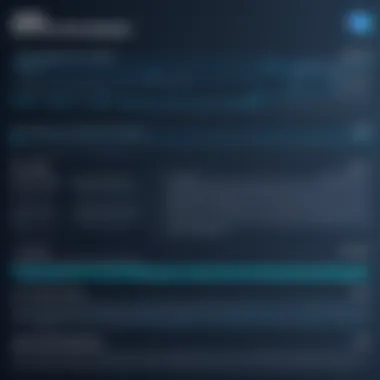
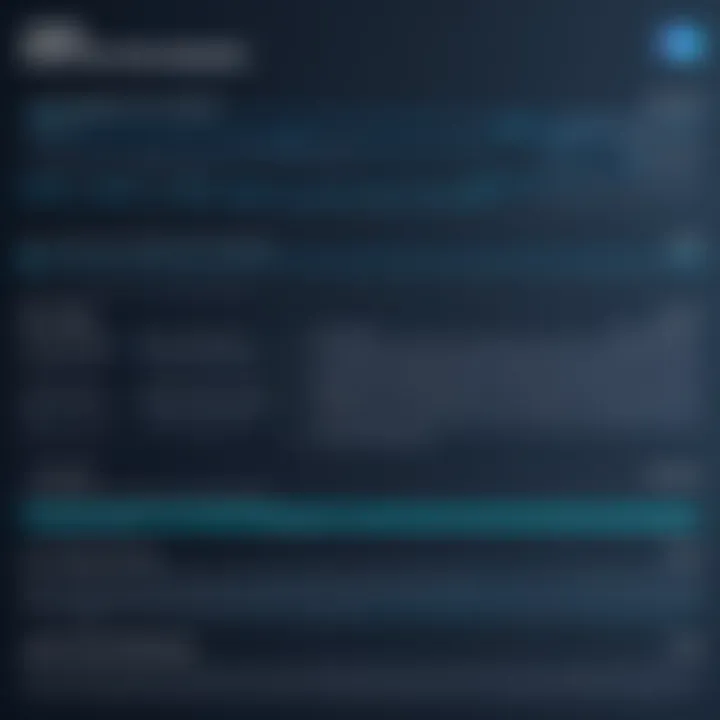
Intro
To grasp why class declarations are vital, it helps to start with a broad perspective of the programming language itself, rooted in its history and evolution.
Intro to Programming Language
History and Background
Java was introduced to the world in 1995 by Sun Microsystems, designed by James Gosling and his team. Originally meant for interactive television, it quickly pivoted towards becoming a platform-independent language, thanks to the philosophy of "write once, run anywhere". This means Java code, once written, can run on any device that has a Java Virtual Machine (JVM), reinforcing its widespread adoption in building everything from mobile applications to large-scale enterprise solutions.
Features and Uses
Java's feature set is extensive, supporting multiple programming paradigms while emphasizing simplicity and clarity. Some key features include:
- Object-Oriented: Enables encapsulation, inheritance, and polymorphism, making code more modular.
- Platform Independence: Javaās bytecode can run on any operating system with a JVM.
- Memory Management: It includes automatic garbage collection, minimizing memory leaks.
Additionally, Java finds uses in a variety of sectors, from web applications using Spring framework to Android app development, opening doors for developers in multiple arenas.
Popularity and Scope
Java remains one of the most popular programming languages, often ranking among the top 3 languages used by developers globally. According to multiple sources, its robust community support, extensive libraries, and frameworks contribute to its ongoing relevance in both academic and professional settings. Notably, platforms like GitHub reveal that a vast percentage of repositories leverage Java, underscoring its enduring demand.
Basic Syntax and Concepts
As we navigate class declarations, itās essential to be familiar with Java's basic syntax and fundamental concepts.
Variable and Data Types
Java is statically typed, requiring variable types to be explicitly defined. Key data types include:
- Primitive Types: , , .
- Non-Primitive Types: Objects and arrays.
To declare a variable, youāll typically write something like:
This statement clearly communicates the intention of holding an integer value for age.
Operators and Expressions
Operators in Java can manipulate variables and constants. Some common operators include:
- Arithmetic Operators: , , , .
- Relational Operators: , , , ``.
These operators help craft more complex expressions crucial for application logic. For instance:
Control Structures
Control structures dictate the flow of execution within Java applications. Key structures include:
- If Statements: For conditional execution.
- Loops: , , and loops enable repeated execution of a block of code.
Advanced Topics
As one dives deeper into Java, advanced topics emerge, painting a fuller picture of the languageās power.
Functions and Methods
Functions and methods are integral in Java, encapsulating behavior. The concept of method overloading allows defining multiple methods with the same name but different parameters, enhancing code usability.
Object-Oriented Programming
Understanding the principles of OOP is essential. Classes serve as blueprints, while objects are instances of these classes, paving the way for encapsulated data and behavior.
Exception Handling
Java provides robust exception handling mechanisms, allowing developers to manage errors gracefully. Using , , and blocks ensures that the program can handle unexpected situations without crashing.
Hands-On Examples
Now, letās illustrate the concepts through hands-on examples that cater to beginners and intermediate learners.
Simple Programs
A basic Java program illustrating class declaration might look like:
Here, we define a class with properties and a method, laying groundwork for further functionalities.
Intermediate Projects
Building on the simple program, you could create a more complex application with multiple objects:
This showcases instantiation and method invocation in action.
Code Snippets
Including useful snippets throughout enhances understanding. For example, the following code snippet demonstrates a method to calculate the area of a rectangle which can be integrated into a larger project.
Resources and Further Learning
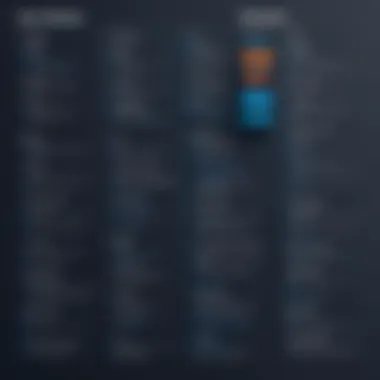
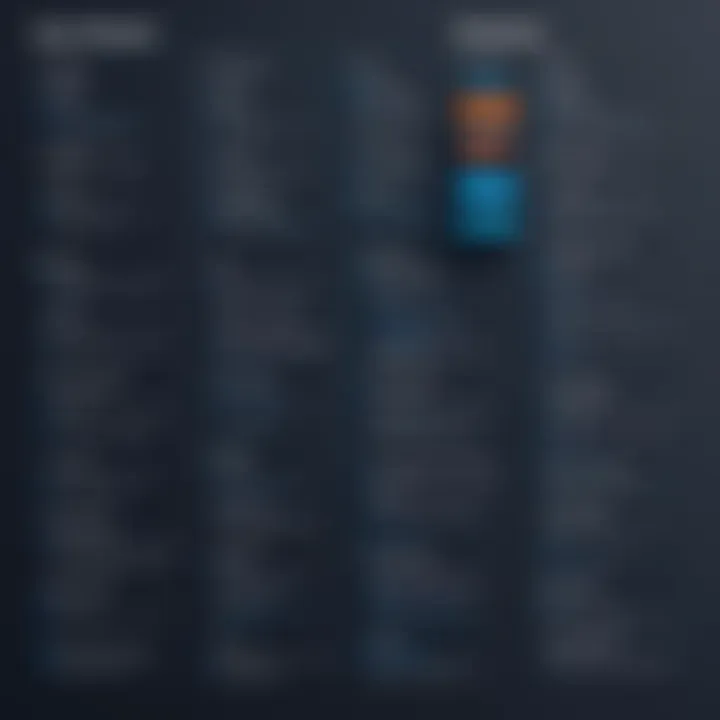
To deepen your understanding and enhance your skills, explore these valuable resources:
- Recommended Books and Tutorials: "Effective Java" by Joshua Bloch provides insights into best practices.
- Online Courses and Platforms: Websites like Coursera and Udemy offer structured courses tailored for all skill levels.
- Community Forums and Groups: Engage with communities on platforms like Reddit and Facebook to share knowledge and learn from others.
"The beauty of Java lies not just in its syntax or structure, but in the vast ecosystem around it that supports continuous learning and innovation."
As you embark on this educational journey, remember that mastery comes with practice and engagement with the programming community.
Foreword to Class Declarations
In the realm of Java programming, class declarations stand out as a vital component. They serve as building blocks for creating robust software applications. Understanding how to declare classes not only helps in structuring code effectively but also paves the way for using object-oriented principles, which are central to Java.
Classes encapsulate data and behavior, granting developers the ability to model real-world entities in their programs. When you think about a vending machine, a class would represent it, bundling methods and variables that define its operations and features. A study of class declarations can lead to better-organized code, making it easier to read and maintain.
The Role of Classes in Java
Classes act as templates for creating objects. Each class defines a specific set of properties, called fields, and methods that describe what the object can do. For example, if you have a class, it might contain fields like and , and methods like and .
This structure allows for code reusability. Once a class is defined, you can create multiple objects from it without rewriting the same logic over and over. Moreover, the ability to create subclasses through inheritance enhances code efficiency further. A class can easily inherit attributes and behaviors of the class, only needing to add its specific features.
Importance of Class Declarations
Class declarations are not just technical necessities; they play a crucial role in defining how we interact with software. When done correctly, they provide clarity and coherence to the code. This is particularly important for teams collaborating on larger projects. For instance, chance it can lead to confusion and ultimately diminish the software quality.
Here's why understanding these declarations matters:
- Encapsulation: Classes encapsulate both data and behavior in a single unit, promoting cleaner code organization.
- Modularity: Class decomposition allows developers to break down complex problems into smaller, manageable pieces.
- Maintainability: Well-defined classes make it easier to update or fix parts of the software, reducing the risk of unwelcome side effects elsewhere in the code.
In summary, class declarations form the backbone of Java programming. They not only dictate how data structures and functionalities are organized but also enrich the programming landscape by employing object-oriented principles.
Syntax of Class Declarations
The syntax of class declarations in Java serves as the foundational blueprint for creating all objects in the language. It's pivotal because a sound understanding of this syntax allows developers to write clear, efficient, and organized code. When a programmer grasps the nuances of how classes are declared, they can leverage the full power of object-oriented programming, making their applications not only functional but also maintainable.
Proper syntax ensures that class structures remain logical and intuitive, which is crucial when collaborating on larger projects or returning to code after a time lapse. In the world of software, where changes are the only constant, having a firm grasp of syntax is like having a trusty set of tools.
Basic Structure
At its core, the structure of a class declaration can seem straightforward, but a closer look reveals its layers. Hereās a simple outline:
In the example above, we see the keywords that initiate the class, define its name, and open a block where all its members will reside. This basic framework holds everything together, much like the framing of a house, not just giving it shape, but providing stability.
Access Modifiers
Access modifiers play a crucial role in Java class declarations, controlling who can see and use class members. They determine the visibility, encapsulation, and security of classes and their members, which makes them invaluable in designing robust applications.
Public Access
Public access allows members of a class to be accessible from any other class or method. This is especially beneficial in situations where collaboration is key, such as in large projects or open-source software. By marking a class or its members as public, you essentially throw open the doors for interaction, which can foster innovation.
A key characteristic of public access is its transparency. Being overly permissive can lead to potential misuse, which is a downside. Yet, the ability for other parts of an application to freely interact with public elements is often what makes solutions so versatile.
Private Access
On the flip side, private access locks down members of a class, making them accessible only within the defining class. This is like having a family secret, tightly guarded and shared only with those inside the circle. The beauty of private access is in encapsulation; it shields the internal state of the class from unintended interference.
Adopting private access is popular among developers because it leads to cleaner, more controlled code. However, the downside is that when you need to share these members with other classes, it can complicate things. Adding public getter and setter methods can alleviate some of these challenges, serving as a bridge between the internal and external worlds.
Protected Access
Protected access stands between public and private. It allows members of a class to be accessible to its subclasses and other classes within the same package. This is crucial for inheritance, as it enables subclasses to leverage the functionality of their parent classes more effectively.
A noteworthy feature of protected access is its flexibility. Managed exposure ensures that while derivatives gain access to necessary members, other unrelated classes remain at bay. Yet, developers need to weigh the risks; inappropriate use might expose sensitive data.
Class Keywords
Class keywords enrich class declarations, introducing modifiers that dictate how classes behave and interact within the Java ecosystem. They help define the role classes play, add structure, and bolster maintainability long-term.
Final Keyword
The final keyword is used to signify that a class cannot be subclassed, making it concrete and unyielding. This is significant when you want to prevent alteration or extension of a classās behavior, ensuring it remains stable.
It's beneficial in scenarios where core functionality should not vary, such as utility classes. However, the drawback is that it limits flexibility; once marked final, the class is set in stone.
Abstract Keyword
The abstract keyword, in contrast, indicates that a class is incomplete and cannot be instantiated directly. This keyword is useful for providing base functionality that can be shared among subclasses, essentially serving as a template.
While this approach promotes reusability, creating abstract classes demands a careful design to ensure that any subclass effectively implements the necessary features. Misusing abstract classes can lead to convoluted hierarchies if one isn't careful.
Static Keyword
The static keyword defines class members that belong to the class itself rather than instances of the class. This means that there exists a single shared resource across all instances, which can lead to memory efficiency. It offers speed, as common operations can avoid the overhead of instance creation.
Nonetheless, using static can come with implications regarding state management. If not properly handled, it could lead to unexpected results, especially in multi-threaded environments.
With all this said, mastering the syntax of class declarations pairs well with understanding access modifiers and keywords, leading to well-crafted, efficient Java applications. The clarity gained from mastering these elements can make the difference between code that runs smoothly and code that leads to confusion.
Types of Classes
Understanding the types of classes in Java is crucial for grasping the broader concepts of object-oriented programming. Classes are the blueprint of any program, fundamental to designing efficient and organized software. Recognizing the differences among class types allows programmers to choose the right approach for problem-solving, enhancing both code readability and maintainability.
Concrete Classes
Concrete classes are the most straightforward type of class youāll encounter in Java. They are fully implemented classes meant to instantiate objects. Think of a concrete class as a complete recipe that not only lists ingredients but also gives you instructions on how to cook.
For example, consider a class named . This class can have properties like , , and . You can define methods such as or , which dictate the behavior of the car. Here's a simple illustration:
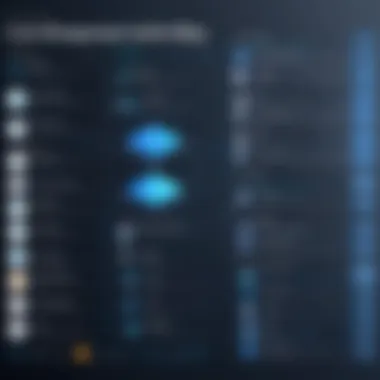
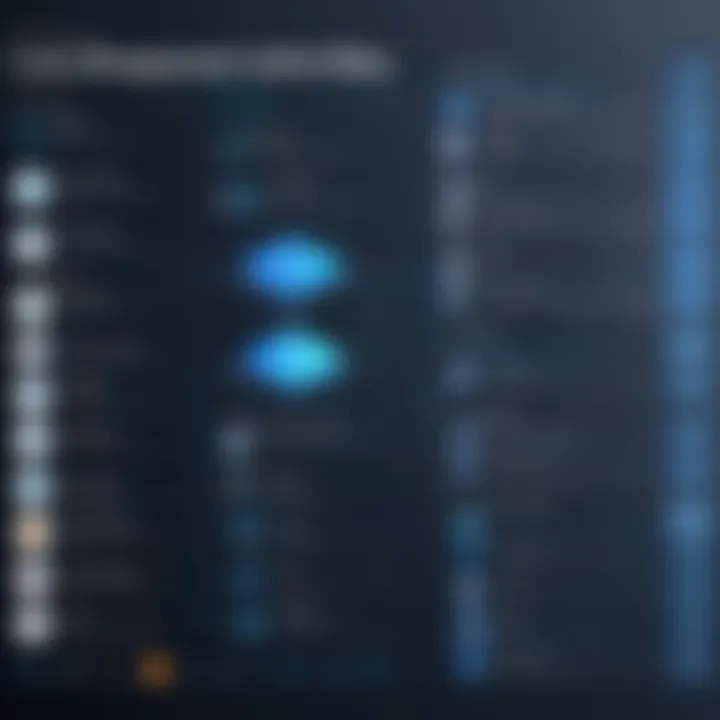
In this snippet, acts as a concrete class where each instance of a car can have different values for its properties but follows the same structure outlined in class definition. This type of class is essential for creating actual objects you can work with.
Abstract Classes
Abstract classes serve as a middle ground between concrete classes and interfaces. They allow you to define some methods that must be implemented by subclasses while providing a partial implementation that can only be used in child classes. Abstract classes are like an unfinished masterpiece. They have some traits but require more work to bring them to life.
Take, for instance, an abstract class . It could have a method that is intended to be implemented differently by derived classes like or :
In this case, can't be instantiated directly, meaning you can't create an object but can create or objects that provide specific implementations. This structure encourages code reuse and establishes a clear hierarchy in your program.
Interface as a Class Type
Interfaces are another critical component of Javaās class system, functioning as a form of contract. When a class implements an interface, it agrees to perform the specified behaviors defined by that interface. They act like a set of rules that can be applied across different classes, ensuring uniformity in certain functionality.
Hereās how an interface might look in a hypothetical scenario involving :
In this code, defines two methods, and , which the class must implement. This makes it easier to add new vehicle types while keeping code consistent.
Having a solid grip on these types of classes ā concrete classes for straightforward implementations, abstract classes for creating a partial blueprint, and interfaces for contractual agreements ā will usher you into a more organized programming approach in Java.
Class Members
In the realm of Java programming, class members represent the backbone of any class declaration. These members essentially define the attributes and behaviors of the objects created from the class. Understanding class members is crucial, as it shapes how we interact with data and methods within our Java applications. Proper utilization of class members leads to cleaner, more maintainable code, which every programmer aspires to achieve.
Field Declarations
Field declarations refer to the various variables defined within a class. They can be classified into two main types: instance variables and static variables. Grasping the differences between these two can dramatically influence program design and performance.
Instance Variables
Instance variables are those unique attributes tied to individual objects created from a class. For instance, if we imagine a class named , instance variables might include properties like , , and . Each object will hold its specific values for these variables, making instance variables essential to capturing object state.
The key characteristic of instance variables is their scope. They are accessible only through their respective objects, which helps ensure that no two instances mess with each other's data. This encapsulation aspect is particularly beneficial; it promotes reliability and simplicity in the way objects interact in larger systems. However, one should also be aware that having many instance variables can increase memory usage, which might pose a disadvantage in resource-constrained environments.
Static Variables
Static variables, in contrast, are tied to the class itself rather than its objects. When you declare a static variable within a class, there is only one copy of that variable, no matter how many instances of the class you create. For example, if we use a static variable in our class, it will keep count of all instances created, providing a convenient way to track common information.
The key characteristic of static variables is their accessibility. Static members can be accessed without creating an instance of the class, which simplifies certain programming tasks. This makes them a popular choice when storing constants or shared values. On the downside, abuse of static variables can lead to tightly coupled code, making it harder to maintain, especially if they are altered frequently.
Method Declarations
When it comes to defining behavior in Java classes, method declarations play a vital role. Methods are essentially blocks of code that perform specific tasks, and understanding how to properly declare them is fundamental to programming in Java.
Defining Methods
Method declarations consist of a method signature (name and parameters) and the body containing actionable code. Defining methods not only brings encapsulation to the design but also aids in code reusability. By putting common functionalities in separate methods, we can avoid redundancy and keep the code clean and understandable.
A standout feature of method declarations is their ability to return values, which adds flexibility and usability within applications. They can also have different access levels, making them either publicly accessible or restricted to the internal workings of the class. However, complex method signatures with too many parameters can be a disadvantage, leading to potential confusion and bugs.
Method Overloading
Method overloading allows multiple methods within the same class to have the same name but different parameters. This means we can use a single method name to handle different data types or numbers of arguments, a feature that provides convenience and clarity in usage.
The key characteristic of method overloading is its ability to enhance code readability. When properly utilized, it allows developers to express similar actions through a unified interface. Yet, overloading can come at a costācareless overloading can create ambiguity and make debugging more challenging.
Constructors
Constructors are special methods that are called when an object is instantiated. They play an indispensable role in initializing objects and ensuring that they have a valid state right from the start.
Default Constructor
A default constructor is one that does not take any parameters. Java provides a default constructor automatically if no other constructors are defined. This constructor initializes object attributes to default values.
The key characteristic here is simplicity. Using a default constructor streamlines the code and makes instantiation straightforward, especially for classes with basic initialization needs. However, relying solely on default constructors can limit flexibility when complex initialization is required.
Parameterized Constructor
In contrast, a parameterized constructor allows for more tailored object creation, enabling developers to set initial values at the point of instantiation. For example, if we want to create a object with specific values for and , we can utilize a parameterized constructor.
The unique feature of parameterized constructors is their flexibility. They allow for more robust object creation, ensuring each instance can be initialized according to specific needs. While they add complexity, they provide valuable control over object state right from the start, making them a preferred choice in many situations.
"Understanding class members not only helps in structuring your code but also enhances its efficiency and maintainability."
In summary, a thorough grasp of class members, including field declarations, method declarations, and constructors is fundamental to effective Java programming. By learning how to use these components appropriately, programmers can foster better practices that lead to cleaner, more efficient code.
Best Practices in Class Declarations
Creating class declarations that are both efficient and understandable is a cornerstone of programming in Java. Best practices ensure that your code is not just functional but also maintainable and adaptable. When you follow these guidelines, it makes the process of collaboration smoother and debugging easier. As the adage goes, "A stitch in time saves nine," applying best practices early helps prevent a myriad of problems later on.
Naming Conventions
When it comes to naming your classes, consistency is key. Following established conventions can save you time and headache down the road. In Java, it's common to use CamelCase for class names. For example, instead of naming a class , a more appropriate name would be . This practice enhances readability, allowing others (and future you) to quickly grasp the intent behind your class.
Additionally, names should be descriptive yet concise. Think about what the class represents and aim to reflect that in the name. For instance, if creating a class that handles bank accounts, naming it would be far clearer than a vague name like .
Remember, clear naming can sometimes prevent miscommunication and errors in larger projects.
Encapsulation Principles
Encapsulation is about bundling the data (variables) and methods (functions) that operate on the data into a single unit or class. This principle is critical because it strengthens data hiding. By keeping certain parts of your code private, you only expose what is necessary. This minimizes the interaction that outside classes can have with your classās internal workings.
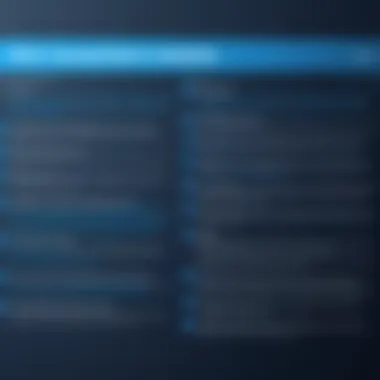
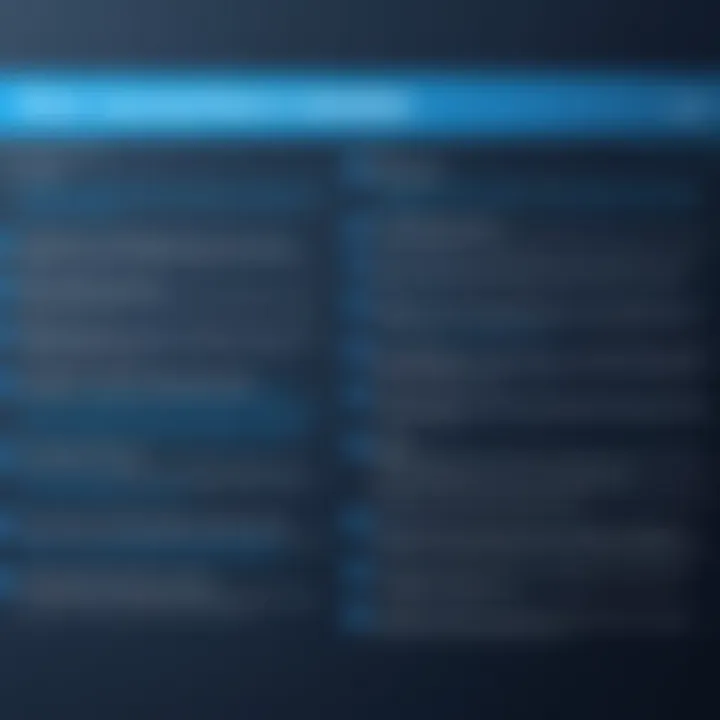
Consider having a class called where you might want to hide the implementation details of how the speed is calculated. You could define a method to get the speed but keep the internal method calculating the speed as private. This way the internal logic is not exposed to the world at large,
Taking advantage of encapsulation improves the robustness of your code, and makes it easier to adjust the internal implementation when needed without affecting other parts of your program.
Commenting and Documentation
Proper documentation is often the difference between code that just works and code that is a joy to read and maintain. When you write your classes, it's crucial to add comments that explain complex logic or the purpose of certain methods and variables.
A good practice is to comment not only on what the code does but why it does it. This can help future developers (or even your future self) understand the motivation behind your design choices. Hereās how you might approach it:
- Method Comments: At the start of each public method, you should explain what it does, its parameters, and what it returns.
- Class Comments: At the class level, outline its purpose and any important details about its usage.
Also, consider the use of tools like Javadoc, which can automatically generate documentation from your comments. This ensures the documentation stays up-to-date and helps in creating an organized view of your class functionalities.
"Code without comments is like a book without pages; you can guess the story, but know you are missing key points."
Real-World Applications
In this section, we delve into why understanding class declarations is crucial for anyone looking to navigate the Java programming landscape. Class declarations serve as the backbone of Java's object-oriented paradigm. They allow programmers to create blueprints for objects, an essential aspect of modular programming. The practical applications of these declarations extend far beyond theoretical knowledge; they significantly influence how we build applications.
When you learn to effectively declare and utilize classes, you understand not just how to write code, but also how to create software that is efficient and easy to maintain. In real-world scenarios, classes organize code into manageable sections. Without a structured approach, even a simple application could balloon into an unwieldy behemoth, making debugging and updates a nightmare.
Benefits of Understanding Class Declarations
- Code Reusability: Classes promote code reusability. Once you have a class, you can create multiple objects from that class without duplicating code, leading to cleaner and more efficient applications.
- Encapsulation: This principle enables developers to hide internal states and require all interaction to be performed through an object's methods. Itās like keeping your house's inner workings hidden while allowing guests to interact through the front door.
- Inheritance: By using class declarations, you can create new classes that inherit properties from existing ones, which is highly beneficial in creating scalable applications.
- Polymorphism: This allows methods to do different things based on the object it is acting upon. For example, a method could behave differently depending on whether it belongs to a or a class.
Understanding these benefits leads to better design decisions in software architecture, enabling developers to produce robust, well-structured programs.
Considerations About Real-World Applications
Real-world applications often determine the effectiveness of class declarations. A well-structured class can positively impact performance, while poorly designed classes can lead to inefficiency. As you begin building applications, consider the following:
- Design Patterns: Familiarizing yourself with design patterns like Singleton or Factory can aid in understanding how classes work together in complex systems.
- Framework Integration: Many frameworks rely heavily on classes. Being adept at class declarations allows you to leverage these frameworks to their fullest potential.
- Collaborative Development: When working in teams, understanding how class declarations work within the project bridge the gap between different developersā code, promoting consistency and coherence.
"In software development, the complexity often comes not from what we are trying to achieve, but from how we organize our code."
The relevance of class declarations becomes particularly palpable in industry scenarios where scalable, maintainable code is not just an idealāit's a necessity. Thus, mastering class declarations is not just about writing code; itās about shaping the future of your software engineering trajectory.
Case Study: Building a Simple Java Application
This section presents a practical example of class declarations in action, illustrating how to assemble a basic Java application. Weāll build a simple library management system that showcases various class declarations in a way that resonates with a beginnerās perspective.
Designing the Library Class
Imagine weāre tasked with managing books in a library. We start by declaring a class called . This class can include attributes like , , and . Hereās how this might look:
This code illustrates a basic structure, emphasizing the encapsulation aspect. Each book is an instance of our class, with its own unique properties.
Adding Methods to the Class
Next, we can add methods to allow interaction with the class. For example:
This method returns the details of a book in a formatted string. Itās an example of how we can interact with our class.
Building the Library Class
Now, imagine creating a class that holds these books. Hereās a skeleton for our class:
This library class allows you to add books to the collection. From here, methods to remove or search for books could be fleshed out, demonstrating how classes can be combined to create larger applications.
Class Usage in Frameworks
Java frameworks like Spring or Hibernate greatly benefit from class declarations. These frameworks embrace the object-oriented nature of Java to simplify complex tasks often encountered in software development.
Spring Framework
Consider Springās use of Dependency Injection (DI). The way Spring relies on class declarations makes managing application components much easier. You declare a class, annotate it with , and Spring takes care of instantiating the class and any dependencies.
Hibernate
Hibernate uses class declarations to map Java classes to database tables seamlessly. A simple declaration like this:
This demonstrates how class declarations serve not just as blueprints for objects but as bridges connecting backend data structures and frontend applications.
In summary, understanding how class declarations work fosters deeper insights into Java frameworks, enabling developers to leverage existing tools and libraries to their fullest potential. Whether itās building a simple application or integrating a robust framework, mastering class declarations is a vital step in oneās programming journey.
Ending
Concluding an exploration of class declarations in Java is like tying up the loose ends of a story. The significance of this section cannot be overstated. It serves as a final reflection on what readers have learned throughout the article, reinforcing the key points discussed while lighting the pathway for future endeavors in programming.
Recap of Key Concepts
Itās crucial to think back on the core themes presented. If we consider the foundations laid in the previous sections, we realize a few central ideas:
- Role of Classes: Classes enable encapsulation and abstraction, providing a sturdy structure around the code, much like a house around a family.
- Syntax and Structure: Implementing clear syntax helps in decluttering coding and avoiding pitfalls that often plague beginners.
- Types and Members: Understanding the difference between concrete classes, abstract classes, and interfaces is essential in leveraging the full power of Java.
- Best Practices: By adhering to naming conventions and commenting diligently, programmers can maintain code that is not only functional but also understandable.
It feels almost like revisiting familiar friends; these concepts are bound together, forming a cohesive understanding of Javaās class declarations. Grasping these principles allows a developer to build robust applications, reinforcing the importance of a solid foundation in programming.
Future Learning Paths
Looking ahead, the journey doesnāt stop at understanding class declarations. Thereās always more to learn, and the vast landscape of Java programming offers countless areas to explore. Here are some paths worth considering:
- Advanced Topics in OOP: Delving deeper into concepts like polymorphism and inheritance can enhance one's understanding and use of classes in more complex scenarios.
- Design Patterns: Learning design patterns can help streamline code and solve recurring design challenges in software development.
- Frameworks and Libraries: Familiarity with Java frameworks such as Spring or Hibernate could greatly enhance oneās programming efficiency and capability.
- Community Engagement: Engaging with communities on platforms like Reddit or contributing to forums can provide insights from other developers, offering real-world experiences and solutions.
As you embark on these new avenues, remember that efficiency and creativity often go hand-in-hand in programming. The skills acquired now will serve as the stepping stones to becoming a well-rounded developer in the future.