Mastering Color Changes in Programming Elements
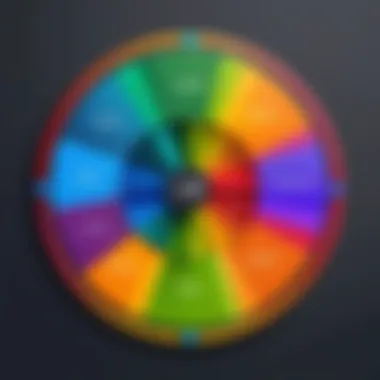
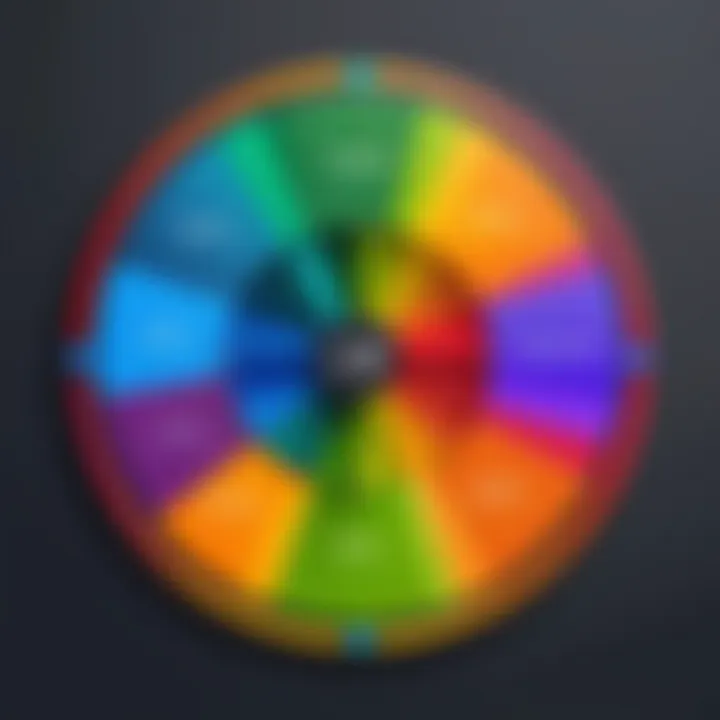
Preamble to Programming Language
When diving into the world of programming, understanding how to manipulate elements, including their colors, is fundamental. Colors can evoke emotions, signal actions, and improve user experience. Thus, knowing how to change an element's color appropriately in various programming languages becomes essential knowledge for any budding programmer.
History and Background
Programming languages, much like the colors they help create, have a colorful history of development. From the early assembly languages that communicated directly with hardware to high-level languages like Python and JavaScript that abstract complexities, the evolution has been rapid and dynamic. This development paved the way for user interfaces that rely on visual elements. The significance of colors in programming became evident as designers and developers sought to enhance user engagement.
Features and Uses
Different programming languages have distinct features that influence how colors can be managed. For instance, in web development, Cascading Style Sheets (CSS) provide a vast array of options for color manipulation, while languages like Java or C++ may require more intricate programming logic to achieve similar effects. This diversity reflects the versatility required by developers to adjust code to suit aesthetic and functional purposes.
Popularity and Scope
Today, programming languages such as JavaScript have garnered immense popularity, primarily due to the rise of web applications and interactive designs. Understanding how to change colors in JavaScript, for instance, can directly contribute to making web applications user-friendly and visually attractive. As technology continues to evolve, the skills for working with user interface aspects such as color will remain in demand, making it a critical focus for learners.
Basic Syntax and Concepts
To change colors programmatically, one must grasp the underlying concepts and syntax of the programming language in use. Here's a brief overview of the basics.
Variables and Data Types
In most programming languages, one starts with defining variables that represent colors. For example, in JavaScript, a color might be stored in a variable as a string:
Common color representations include hexadecimal codes, RGB values, or simple color names, which will become pivotal as you start coding.
Operators and Expressions
Understanding how to manipulate these variables is crucial. Operators allow programmers to perform calculations and combine strings, giving rise to dynamic color changes. For example:
Control Structures
Control structures let you decide when certain color changes happen. If you want to change the background color on a button click, for instance, you'll likely use conditional statements or event listeners:
Advanced Topics
Knowing how to change colors opens up conversations about more advanced programming concepts.
Functions and Methods
Functions encapsulate color-changing actions. A simple function can make your code more organized:
Object-Oriented Programming
In object-oriented programming, color attributes can be encapsulated within objects, creating a more structured approach:
Exception Handling
Handling errors is paramount. What happens if an invalid color is provided? Exception handling can safeguard the program against crashes due to unexpected inputs.
Hands-On Examples
Learning by doing often solidifies concepts.
Simple Programs
A straightforward color changer program can be written in HTML and JavaScript:
Intermediate Projects
Consider building a color palette app where users can select and save their favorite colors. This involves arrays and user inputs alongside event listeners.
Code Snippets
Here's a code snippet to create a color-changing animation using CSS and JavaScript:
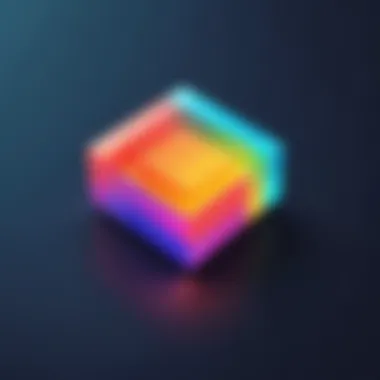
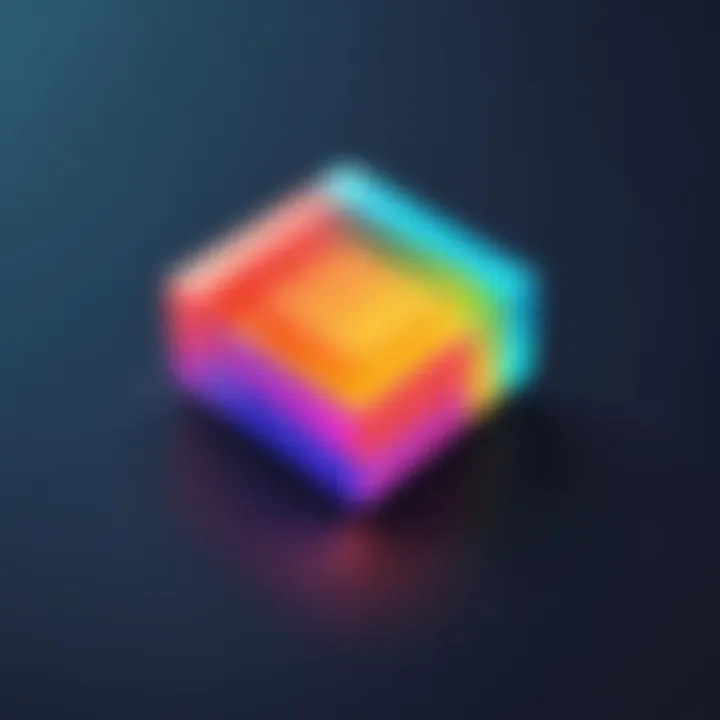
Resources and Further Learning
For those eager to expand their knowledge:
Recommended Books and Tutorials
- Eloquent JavaScript by Marijn Haverbeke offers insights into JavaScript, including color manipulation techniques.
- You Don’t Know JS series for a deeper understanding of JavaScript foundation.
Online Courses and Platforms
- FreeCodeCamp offers free courses on web development.
- Codecademy provides interactive coding lessons, making color changes hands-on.
Community Forums and Groups
- Reddit's Learn Programming is a friendly community for learners.
- Facebook groups related to coding and web design can also serve as valuable support networks.
"The mastery of colors in programming is not merely functional; it is the flench of artistry combined with technical skill."
Understanding Color Theory
Understanding color theory is pivotal in the realm of programming since it serves as the backbone for any manipulation involving color. Grasping how colors interact, evoke feelings, and visually represent information allows developers to create more intuitive and engaging applications. It isn’t just about choosing a color; it’s about understanding the impact that color can have on user experience and interface design. The finesse of color applications can range from simple aesthetic enhancements to crucial accessibility measures that aid usability for diverse audiences.
The layering of colors, the effects of different hues on perception, and their ability to communicate meaning are all steeped in color theory. For instance, blue can evoke trust, while red often signifies alertness or urgency. Knowing this can help programmers not only in designing eye-catching UI elements but also in ensuring their applications align with user expectations and needs. This makes familiarity with color theory not just beneficial but essential in crafting meaningful and effective programming solutions.
Basics of Color
At its core, color is a phenomenon created by light. It's how our eyes perceive the spectrum of light waves that results in what we identify as different colors. “Every color tells a story.” Understanding this a bit deeper involves looking at primary colors, which are the building blocks for creating a myriad of other colors. There are three primary colors: Red, Blue, and Yellow. Mixing these colors results in secondary and tertiary colors.
Colors can convey information and provide cues that can enhance or detract from user experience. Programmatically, colors are the basic canvas controlling the look and feel of interfaces. Therefore, knowing the basics can prime developers to make informed choices as they transition into more complex applications.
Color Models
Color models are systematic ways to describe and communicate color. These models help in defining how colors are represented in various digital formats.
RGB Model
The RGB model, which stands for Red, Green, and Blue, is perhaps the most widely adopted by digital devices like computer screens and cameras. Its contribution to programming and design lies in its additive color mixing principles: when you combine different intensities of red, green, and blue light, you create various colors. A characteristic of the RGB model is its direct correlation with how we perceive light in the digital realm.
One notable advantage of the RGB model is its simplicity and versatility in web design. By adjusting the intensity of each color component, developers can achieve a vast palette of colors. However, the downside is that translating RGB colors to print media can present challenges, as printers typically use color models like CMYK.
CMYK Model
CMYK, standing for Cyan, Magenta, Yellow, and Key (Black), is primarily used in the realm of color printing. The significance of CMYK lies in its subtractive color mixing: instead of adding light, this model absorbs varying wavelengths of light to produce colors. This model is specifically designed for the printing industry.
The key characteristic is that it accurately represents colors on paper, making it a go-to for designers who transition between digital designs and print materials. However, understanding CMYK can be complex, as it needs careful calibration with printers to avoid unexpected hues once printed.
HSL Model
The HSL model simplifies representation even further by breaking down colors into three components: Hue, Saturation, and Lightness. This makes it a popular choice among designers and programmers. Hue represents the color itself, while saturation refers to the intensity of that color, and lightness denotes its brightness.
An important aspect of HSL is its intuitive approach since altering saturation and lightness tends to yield instantly recognizable results. For instance, decreasing lightness can produce darker shades of the same color. However, a drawback is that HSL may be less accurate in terms of representing colors in all devices, similar to RGB.
Understanding the various color models not only enhances the creativity of a programmer but also significantly improves the usability and accessibility of applications.
Importance of Color in Programming
Understanding the significance of color in programming extends beyond mere aesthetics; it's a fundamental principle that affects user interaction and overall experience. From the vibrant hues of a well-designed website to the subtle tones of a desktop application, color transforms how users perceive and engage with the content. Here, we'll delve into two crucial aspects of this importance: user interface design and accessibility considerations.
User Interface and Experience
In the realm of user interface (UI) design, color plays a pivotal role in shaping user experiences. It's not just about choosing colors that look good together; it’s about creating an intuitive flow that guides users through an application or a site. For instance, consider the warm colors often used for call-to-action buttons on a website.
- Psychology of Color: Colors evoke emotions and convey messages. A red button might indicate urgency, while blue conveys trust. UI designers harness these meanings to enhance user interaction.
- Hierarchy and Clarity: Colors can establish visual hierarchy. By using contrasting colors for different elements, programmers can make navigation more intuitive. Think of the pastel tones that are gentle on the eyes for background elements compared to bold colors for pivotal action items.
The importance of color management is further highlighted during the development phase. Using tools like CSS color variables can promote consistency across applications. Maintaining a uniform color palette helps in building a recognizable brand identity. This unity fosters a seamless experience, reducing cognitive load on users.
Accessibility Considerations
Color choice is deeply entwined with accessibility, a crucial aspect of modern programming. Developers must ensure that all users—including those with color vision deficiencies—can navigate and understand their applications. Thus, accessibility guidelines, such as the Web Content Accessibility Guidelines (WCAG), become paramount.
- Contrast and Readability: One of the most critical elements is ensuring sufficient contrast between text and background colors. For example, light grey text on a white background makes it nearly impossible for many to read effectively. As a rule of thumb, aim for a contrast ratio of at least 4.5:1 for normal text.
- Color as Only Information: Programmers often fall into the trap of using color as the sole means of conveying information. For example, a form may indicate errors using red highlights. It's essential to pair such signals with icons or text descriptions to benefit all users.
"Accessibility shouldn’t be an afterthought; it should be a fundamental principle of design."
Incorporating accessible color choices not only broadens the audience but also aligns with ethical programming practices. The goal is to create applications that everyone can use without barriers.
In summary, the importance of color in programming cannot be overstated. By thoughtfully considering color choices, developers enhance user experience while remaining inclusive. This foundation sets the stage for the practical approaches discussed in subsequent sections of this article.
Changing Colors in Web Development
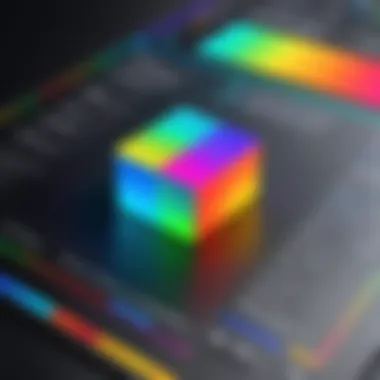
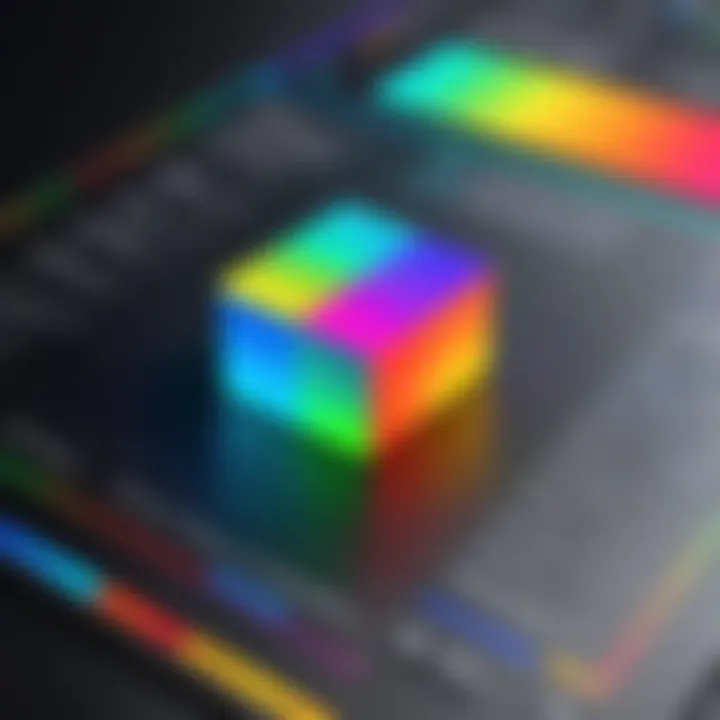
Changing colors in web development is a vital skill for any programmer. It plays a key role in how users interact with a webpage. Getting the color scheme right can mean the difference between a user feeling comfortable navigating your site or quickly clicking away. So many aspects of design are influenced by color: it affects readability, accessibility, and overall aesthetic appeal. Each of these elements is fundamental in creating an engaging user experience. In this section, we will explore various techniques developers employ to successfully change colors across different web development frameworks.
CSS Techniques
Inline Styles
Setting colors with inline styles is like putting a fresh coat of paint directly onto a wall. The feature allows developers to apply unique styles to individual elements without touching the rest of the document. This method, while sometimes overlooked, can be quite beneficial. One major advantage is the simplicity of implementation; it’s often just a matter of adding a attribute to an HTML tag. However, it comes with downsides. If there are multiple instances of a similar element, adjusting a color means changing it in many places, leading to messy code.
For instance:
Key characteristic: Immediate effect on single elements. Advantage: Quick adjustments for minor changes. Disadvantage: Could lead to repeated code, making maintenance cumbersome in the long run.
Internal Stylesheets
Internal stylesheets can be thought of like having a dedicated room for all the painting supplies. This method allows developers to define styles in the section of the HTML document using tags. It happens to be quite popular due to its ease of use when working on small projects or when individual pages require unique themes. By grouping styles in one location, changes become easier to manage.
For instance:
Key characteristic: Centralized styles for single documents. Advantage: Simplifies management of styles when working on limited pages. Disadvantage: Does not promote reusability across multiple pages, so larger projects can quickly become unwieldy.
External Stylesheets
External stylesheets offer the cleanest and most organized approach to color styling. By linking a separate file, developers can keep their style rules separate from HTML, which encourages a clear structure and reusability. It’s particularly effective for larger projects with many pages; a singular CSS file can standardize colors across the entire site, preserving consistent branding.
Consider this example:
And the link in HTML:
Key characteristic: Separation of concerns. Advantage: Easier to maintain and promote consistent site-wide color application. Disadvantage: Requires additional HTTP requests to fetch the external stylesheet, which could slightly affect load times.
Using JavaScript for Dynamic Changes
Event Listeners
Event listeners give developers a powerful way to make color changes interactive. By linking functions to user actions—like mouse clicks or hover events—developers can create responsive designs that engage users in real-time. Event listeners are beneficial as they make webpages feel more alive and can enhance user experience.
For example:
Key characteristic: Real-time reaction to user actions. Advantage: Dynamic and interactive user experience. Disadvantage: Larger JavaScript files can complicate debugging and increase load times if not carefully managed.
Dynamically Updating Styles
Dynamically updating styles using JavaScript can transform the way colors are presented on a webpage. It allows for changes without reloading the page, which can lead to a more seamless experience for users. Utilizing functions to alter styles in response to events or certain conditions can keep users engaged.
Key characteristic: Provides flexibility in design. Advantage: Ability to create responsive designs. Disadvantage: May become complex, impacting loading times or browser performance if not optimized well.
Ultimately, whether it’s through CSS techniques or JavaScript, knowing how to change colors effectively can tremendously enhance a programmer’s toolkit. Understanding the strengths and weaknesses of each method ensures that users will have a polished, accessible, and visually appealing experience on their websites.
Changing Colors in Desktop Applications
Changing colors in desktop applications is a crucial aspect that goes beyond mere aesthetics; it significantly enhances the user experience and interaction. In desktop development, where components often have fixed placements and behaviors, the ability to manipulate colors dynamically becomes a powerful tool for developers. Colors can convey messages, highlight important features, and set the mood of the application. Leveraging color effectively ensures that apps not only look appealing but also facilitate usability, making comprehension easier for users.
To achieve harmonious color changes, developers must understand the frameworks and languages specific to desktop application development. For instance, using Java or C++ can provide distinct benefits, depending on the nature of the application—be it a sophisticated business tool or an engaging game. Each framework has its own methods for handling color, which can vary in complexity and efficiency.
Color Handling in Java Applications
Using Swing
Swing is a widely used framework for building graphical user interfaces (GUIs) in Java. Its strength lies in its lightweight components, which can be easily customized and manipulated. One of the key features of Swing is its flexibility in modifying color properties across various components, from buttons to panels. Developers can easily change the foreground and background colors, making it fantastic for creating responsive interfaces. Furthermore, it adheres to a robust MVC (Model-View-Controller) design, which promotes clean separation between the business logic and user interface.
However, one should keep in mind that Swing’s performance can lag if not properly managed. Heavy color modifications in complex GUIs can sometimes lead to sluggish responses, especially in older systems. Yet, for many applications, the benefits of enhanced visual consistency often outweigh the drawbacks.
Using JavaFX
JavaFX, on the other hand, represents a newer approach to Java GUI development. It introduces a richer set of graphics and media capabilities that facilitate crisp and modern-looking applications. A notable aspect is its built-in support for CSS (Cascading Style Sheets), which allows developers to style UI elements using familiar syntax. This makes it easier to create dynamic themes and responsive color schemes without extensive coding.
One unique feature of JavaFX is its support for animations and effects that can be color-centric—making the application not just functional but also visually engaging. However, a downside could be its learning curve for those accustomed to Swing. Transitioning from Swing to JavaFX may require an adjustment period, but the payoff is often worth it for more visually appealing applications.
++ and GUI Frameworks
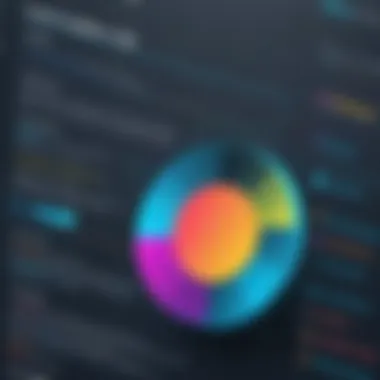
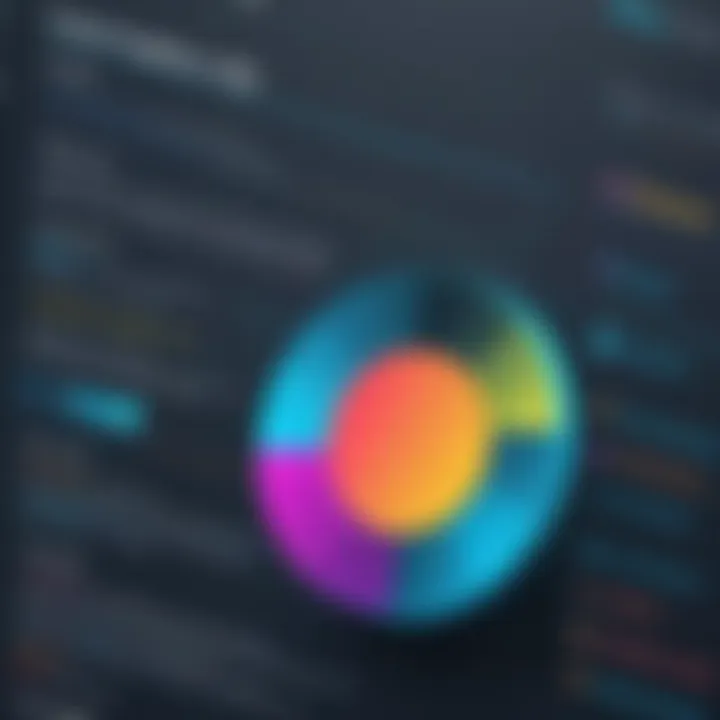
Qt Framework
The Qt Framework is highly acclaimed for its powerful capabilities in cross-platform application development. One of its standout characteristics is the ability to handle complex color manipulations effortlessly. Whether you’re changing colors in button states or dynamically altering them based on user interactions, Qt provides a simple API to achieve these effects. This makes it a favorite among C++ developers for desktop applications.
The integrated signal and slot mechanism in Qt is another advantageous feature, allowing for seamless event-driven programming. However, it's worth noting that while Qt offers powerful features, the framework's licensing can sometimes be a consideration for open-source projects, which may limit its use in certain contexts.
GTK Framework
GTK (GIMP Toolkit) serves as another popular framework, especially among developers creating applications for Linux. Its key defining characteristic is its immediate feedback with GTK’s widget styling, enabling straightforward color adjustments. With GTK, you can easily apply colors to widget backgrounds, borders, and even text, leading to a cohesive design.
Despite its advantages in Linux environments, GTK can lack some of the robustness found in cross-platform capabilities like Qt. Depending on the target environment, developers might find that GTK's performance and styling flexibility can differ across operating systems, but it remains an excellent choice for Linux-based applications.
The right framework can make all the difference in how colors are handled in desktop applications, impacting both performance and user interaction.
Changing Colors in Game Development
The vibrant world of gaming relies heavily on visual aesthetics, and color plays a pivotal role in shaping the player’s experience. Whether you’re developing a sprawling fantasy realm or a simple puzzle game, the right colors can enhance mood, convey information, and even guide player behavior. In this section, we’ll explore the significance of color manipulation in game development, as well as the tools and methodologies you can utilize.
Game Engines Overview
Game engines are the foundational tools that allow developers to bring their visions to life. These platforms, such as Unity, Unreal Engine, and Godot, come equipped with their own color management systems. Understanding how these engines handle color can significantly impact how you design your game.
- Unity: This widely used engine offers a flexible approach to color manipulation. Unity allows for real-time color changes that can be applied to materials, UI elements, and even lighting. Its shader system permits developers to craft custom visual effects that respond dynamically to player input.
- Unreal Engine: Known for its stunning graphics, Unreal Engine comes with advanced features like color grading tools. These tools let you adjust colors across scenes to create a cohesive visual narrative, ensuring that your game conveys the desired emotions.
- Godot: As an open-source option, Godot provides straightforward methods for color change. Its node system allows developers to apply color effects easily, making it an excellent choice for beginners.
Adapting colors to various elements within these engines can enhance gameplay, sharpen player focus, and seamlessly deliver thrilling narratives.
Using Color Palettes in Games
Color palettes are essential in game development; they provide a consistent visual language that enhances both gameplay and storytelling. A well-crafted color palette not only set the mood but also aids in player orientation and comprehension of game mechanics.
- Establishing Mood: Colors evoke emotions and can signal a player’s journey—bright colors might indicate joy or adventure, while darker hues can suggest mystery or danger. Think about using blue tones for serene environments and red for danger zones.
- Clarity of Gameplay: Selecting contrasting colors can help differentiate between interactive and non-interactive elements. For example, if you’re designing a platformer, ensuring the character contrasts well against the background allows for easier navigation.
- Color Accessibility: It's crucial to consider players with color vision deficiencies. Tools like Color Oracle can help simulate how colors appear to users with common visual impairments, ensuring your game remains accessible.
Colors can also be arranged in specific ways to create harmonious or dynamic effects.
"The use of a limited color palette can create a striking aesthetic while ensuring clarity in gameplay."
In applied examples, you might design a classic platformer game where blue signifies safe zones and red indicates hazards, thus enhancing player understanding through color comprehension. Additionally, traditional palettes like the 8-bit colors of retro games can lend a sense of nostalgia while maintaining functionality.
By iterating on these principles within your game design, you can create immersive and visually stunning experiences that resonate with players and keep them engaged.
Best Practices for Color Changing
Changing colors in programming isn't just about picking a hue that looks good. It's an art and a science combined, deeply rooted in functionality and aesthetics. Adhering to best practices ensures that color changes enhance user experience rather than detract from it. When coding, consider factors like readability, accessibility, and brand consistency. Here’s a closer look at why these practices are not just guidelines but necessities.
Consistency Across Applications
Consistency plays a crucial role in the visual integrity of an application. When users navigate through different pages or components, they expect certain visual familiarity. Here are some key points to maintain consistency:
- Color Palette: Stick to a defined color palette throughout your project. This will help in creating a cohesive look.
- Usage of Colors: Use colors in the same context. For instance, if red indicates alerts in one section, it should do the same across the entire application.
- Brand Alignment: Colors should reflect the brand's identity. If a company’s logo features specific colors, those should be incorporated throughout its software applications.
When colors are used consistently, users are more likely to trust the interface, which can lead to increased engagement.
Testing Color Choices
Picking colors is one thing; validating them is another. To ensure your color choices resonate with users as they should, testing is key. Here are some strategies to employ:
- A/B Testing: Show two versions of the same element with different colors to users and gather feedback. This approach can provide insights into what colors attract attention or result in more clicks.
- Accessibility Testing: Aim for color contrasts that meet WCAG standards. This is especially important for users with visual impairments. There are tools available that can simulate color blindness, helping you refine your choices.
- Usability Tests: Observe how users interact with your interface. Sometimes colors might look good on paper but behave differently in real-world navigation.
By testing color choices rigorously, developers can fine-tune their elements to ensure they’re not just appealing but functional. Remember the quote, "In the world of pixels, perception becomes reality."
Common Pitfalls and Solutions
When venturing into the world of programming, understanding how to change colors effectively might seem straightforward. However, a few common pitfalls can trip up even seasoned programmers. This section aims to shed light on these challenges and offers practical solutions. Grasping these nuances can enhance both the visual appeal of your applications and user experiences.
Overusing Bright Colors
Bright colors can be quite enticing. They draw attention, energize layouts, and can make an interface pop. But here’s where the conundrum lies—overusing them is a slippery slope. Excessive bright colors can overwhelm users, making interfaces off-putting and difficult to navigate. The brain often finds it hard to focus amidst a riot of hues. This can lead to user frustration, which is the last thing you want for your application or website.
To keep clear of this pitfall, consider the following strategies:
- Use Bright Colors Sparingly: Select strategic elements like buttons or icons to apply vibrant shades. It creates a sense of focus without overwhelming the viewer.
- Balance with Neutrals: Pair bright colors with neutral tones for a well-rounded aesthetic. A bright button set against a soft gray background can shine without burning the eyes.
- Color Harmony: Understanding complementary color schemes can help find a middle ground between a lively look and usability. Explore tools such as color wheel to sketch out a balanced palette.
Ultimately, moderation is key. Users appreciate visual stimulation, but not at the cost of comfort. Keep it balanced—your audience will thank you.
Debugging Color Issues
Debugging color issues is another aspect that many programmers grapple with. A change in a color might lead to something unexpected—maybe a shade's text becomes unreadable, or elements overlap due to miscalculations in the styling code. Such pitfalls can be particularly vexing and derail your productivity.
To tackle these issues efficiently, here are some practical steps:
- Inspect Elements: Use browser developer tools. Right-click on any component and select "Inspect". This reveals the CSS properties being applied.
- Check Specificity: CSS rules vary in weight. If a color isn't displaying as expected, another rule may be overriding it. Look into the specificity of your rules to identify conflicts.
- Use Color Contrast Checkers: Tools like the WebAIM contrast checker help ensure that foreground and background colors meet accessibility standards. This ensures readability for all users.
- Console Logging: When working with JavaScript, console logs can reveal dynamic styling issues. Log the color values to see where things might be going haywire.
Above all, patience is a virtue. Debugging may feel tedious at times, but it’s often in the details that you find solutions. Embrace the problems as learnings rather than setbacks.
"Great programming is about finding solutions to problems—every obstacle can lead to a sharper skillset if you approach it the right way."
By keeping your eyes peeled for these pitfalls and remaining diligent in your approach, you can navigate the complex landscape of color changes in programming with confidence. Both the aesthetic and functional aspects of your project will benefit significantly.