Comprehensive Guide to C Programming: Step-by-Step Insights for Proficiency
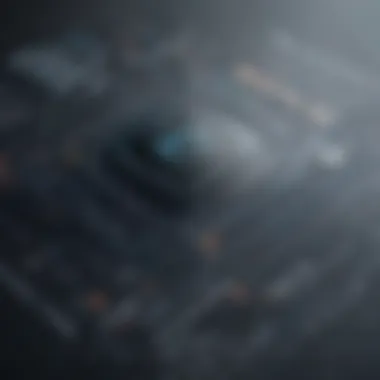
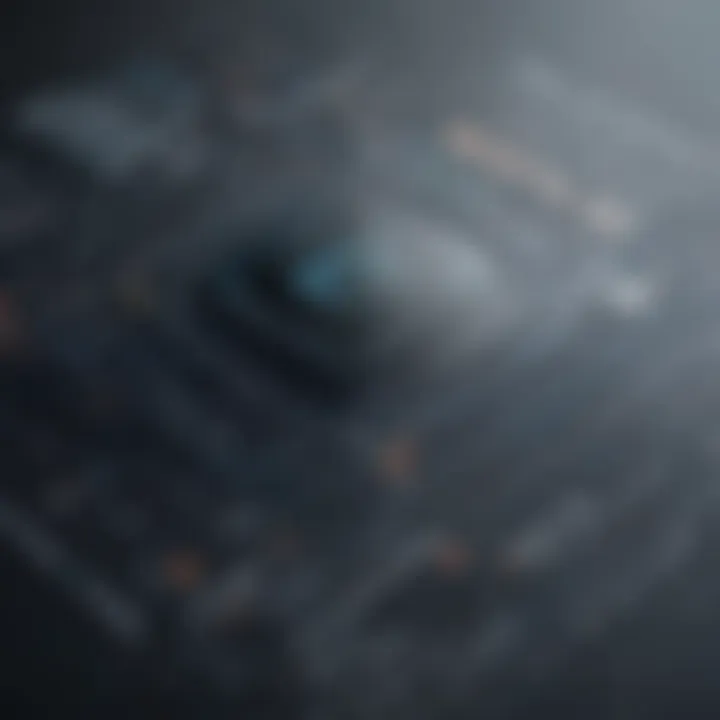
Introduction to Programming Language
Programming in C is a fascinating journey into the realm of computer programming. Understanding its history and background provides insights into the evolution of this powerful language. Delving into the features and uses of C offers a glimpse into its versatility and applicability across different domains. Exploring the popularity and scope of C reveals its enduring relevance and significance in the world of software development.
Basic Syntax and Concepts
Mastering C programming requires a solid grasp of fundamental elements such as variables and data types. These foundational concepts form the building blocks of any C program, enabling developers to store and manipulate data efficiently. Understanding operators and expressions is essential for performing computations and making logical decisions within a program. Control structures, including loops and conditionals, dictate the flow of execution and allow for complex algorithm design.
Advanced Topics
Venturing into advanced topics in C programming opens doors to enhanced functionalities and code organization. Functions and methods play a crucial role in breaking down complex tasks into manageable chunks, promoting code reusability and modularity. Object-Oriented Programming introduces a paradigm shift towards encapsulation, inheritance, and polymorphism, empowering developers to create sophisticated software solutions. Exception handling enables robust error management, ensuring smooth program execution even in challenging scenarios.
Hands-On Examples
Practical application is key to solidifying knowledge and honing one's programming skills. Simple programs serve as stepping stones for beginners to grasp basic concepts and practice coding methodologies. Intermediate projects offer a more immersive experience, challenging learners to apply their understanding in real-world scenarios. Code snippets provide succinct demonstrations of specific techniques or functions, aiding in comprehension and fostering experimentation.
Resources and Further Learning
Embarking on a journey to master C programming is an ongoing process that requires continuous learning and exploration. Recommended books and tutorials offer in-depth insights into advanced topics, supplementing hands-on practice with theoretical foundations. Online courses and platforms provide interactive learning experiences, allowing individuals to engage with programming concepts in dynamic ways. Community forums and groups foster a sense of collaboration and camaraderie, enabling learners to seek advice, share knowledge, and grow together in their programming endeavors.
Introduction to Programming
In this section, we delve into the fundamental concepts of C programming, setting the stage for a comprehensive understanding of the language. Exploring the basics is crucial as it forms the building blocks upon which advanced concepts are constructed. Understanding the intricacies of C programming is vital for individuals seeking proficiency in coding and software development. By grasping the essence of C programming at this foundational level, readers can progress with confidence into more intricate techniques and applications.
Understanding the Basics
History and Overview of
A critical aspect of C programming is its rich historical background and solid foundation. The history of C traces back to the development of Unix, making it one of the oldest and most influential programming languages in the industry. C's simplicity and power have made it a popular choice for system programming, embedded systems, and application development. Its efficiency and versatility have stood the test of time, proving its relevance in modern programming landscapes.
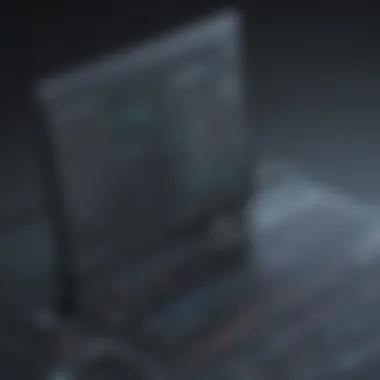
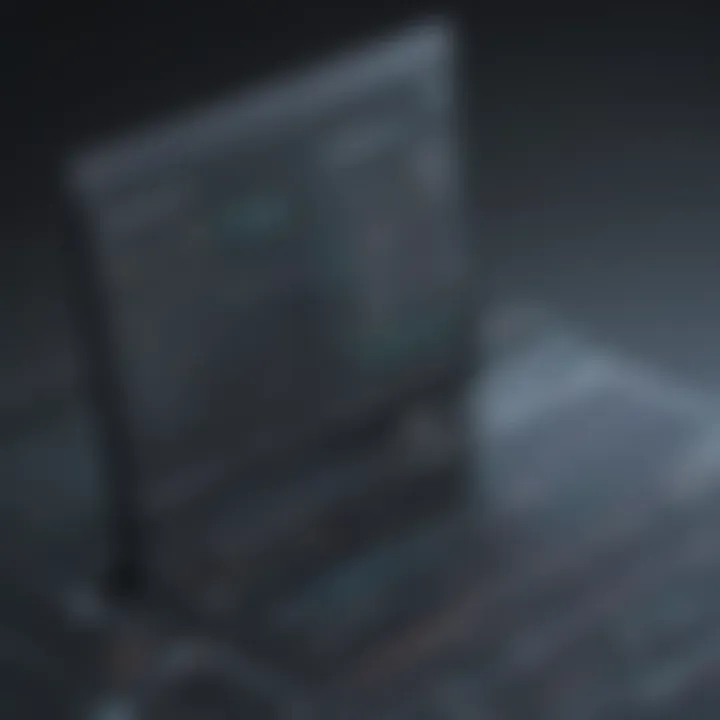
Setting Up the Development Environment
Setting up the development environment is an essential precursor to effective C programming. By configuring the environment to align with the requirements of C, programmers can optimize their workflow and ensure smooth execution of code. A well-established development environment provides access to necessary tools, libraries, and resources, streamlining the coding process. However, varying environments may pose challenges, requiring adaptability and problem-solving skills to overcome obstacles and maintain productivity.
Basic Syntax and Structure
Variables and Data Types
Variables and data types form the core components of C programming, allowing for the storage and manipulation of information within a program. Understanding the intricacies of variables and data types is fundamental to writing efficient and error-free code. Proper declaration and utilization of variables enhance program robustness and readability, contributing to overall code quality and maintenance.
Operators and Expressions
Operators and expressions in C facilitate operations and computations within a program, dictating logic and flow control. Mastery of operators and expressions empowers programmers to perform complex calculations with precision and efficiency. By leveraging a diverse set of operators, programmers can implement a wide range of functionalities, optimizing program performance and functionality.
Control Flow Statements
If-Else Statements
If-else statements in C enable conditional branching, altering program flow based on specified conditions. This control structure is integral to decision-making within a program, allowing for flexibility and adaptability in various scenarios. Effective utilization of if-else statements enhances program logic and functionality, enabling programmers to create dynamic and responsive applications.
Loops - For, While, Do-While
Loops, including for, while, and do-while variations, provide iterative capabilities within a program, executing a set of instructions repeatedly. These structures are essential for implementing repetitive tasks efficiently and dynamically. With carefully crafted loops, programmers can iterate through data, perform calculations, and manage program flow seamlessly, enhancing both productivity and effectiveness.
Creating Your First Program
In this section of the comprehensive guide on programming in C, we delve into the crucial aspect of creating your first program. Understanding the significance of this topic is paramount as it sets the foundation for all future programming endeavors. By focusing on writing the initial lines of code, programmers can grasp the essential syntax and structure of C, ultimately building a strong skill set. Attempting your first program initiates hands-on learning, promoting a practical approach to comprehend theoretical concepts and bridge the gap between knowledge and application. As beginners embark on this journey, they not only acquire technical expertise but also nurture problem-solving skills and logical thinking.
Writing the Code
Initializing Variables
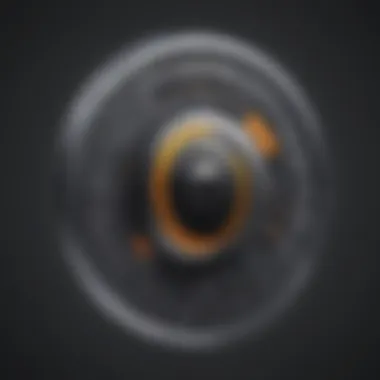
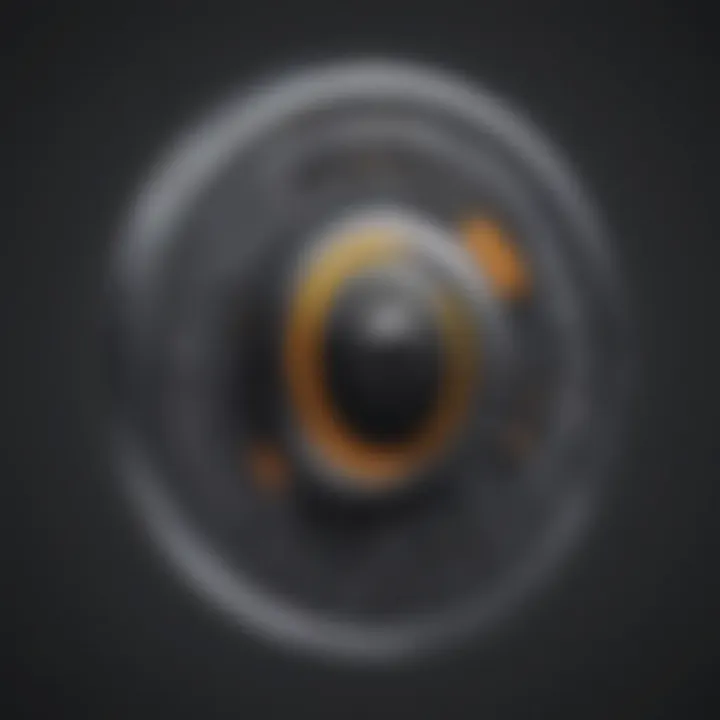
When it comes to programming in C, the process of initializing variables holds a pivotal role in ensuring the accurate functioning of a program. Initializing variables involves assigning an initial value to a variable, providing a starting point for computations and data manipulation. This practice not only prevents unpredictable results due to undefined variable values but also enhances code readability and maintenance. By explicitly defining the initial state of a variable, programmers can avoid errors and streamline the debugging process. The act of initializing variables is considered a best practice in programming as it promotes clarity, efficiency, and a structured approach to problem-solving.
Implementing Basic Functions
In the realm of C programming, implementing basic functions is a fundamental aspect that drives the core functionality of a program. Basic functions encapsulate specific tasks or operations, allowing programmers to modularize their code and promote reusability. By breaking down complex operations into smaller, manageable functions, developers can enhance code organization and maintainability. Implementing basic functions facilitates code comprehension, as each function focuses on a specific task, reducing overall complexity. Additionally, the utilization of functions enables developers to easily update and modify specific functionalities without impacting the entire codebase. Embracing basic functions in C programming empowers programmers to efficiently structure their programs, foster code reusability, and promote scalability.
Advanced Concepts and Techniques
In this section of the article, we delve into the pivotal topic of Advanced Concepts and Techniques in C programming. Understanding these advanced elements is crucial for elevating your programming skills to a higher level of proficiency. By focusing on intricate details such as Arrays and Pointers, Functions and Recursion, and File Handling, you will not only expand your knowledge but also enhance your problem-solving capabilities. Advanced Concepts and Techniques play a crucial role in empowering programmers to optimize their code, improve efficiency, and tackle complex challenges with precision.
Arrays and Pointers
Multi-dimensional Arrays:
Multi-dimensional Arrays serve as a fundamental component within the realm of C programming. These arrays enable the storage of data in a structured format, providing a systematic approach to manipulate and access elements. The key characteristic of Multi-dimensional Arrays lies in their ability to store data in multiple dimensions, offering a more organized and efficient way to represent complex data sets. The utilization of Multi-dimensional Arrays in this article proves beneficial as it enhances the handling of large datasets and facilitates the implementation of various algorithms with ease. Despite their advantages, Multi-dimensional Arrays may pose limitations in terms of memory allocation and access speeds, which programmers need to consider when working on projects.
Pointer Arithmetic:
Pointer Arithmetic holds a significant role in C programming, particularly in memory management and data manipulation. This advanced concept allows programmers to perform arithmetic operations directly on memory addresses, providing a more flexible and efficient approach to dealing with data. The key characteristic of Pointer Arithmetic lies in its ability to enhance the efficiency of memory handling and data traversal, making it a popular choice for optimizing code performance. The unique feature of Pointer Arithmetic lies in its capability to directly access memory locations, enabling faster data manipulation and seamless interaction with complex data structures. While Pointer Arithmetic offers substantial benefits in terms of speed and efficiency, improper usage can result in memory leaks and program crashes, mandating careful consideration during implementation.
Functions and Recursion
Passing Arguments:
Passing Arguments serves as a crucial aspect in C programming, allowing functions to receive input values for processing. The key characteristic of Passing Arguments is its role in enabling dynamic data manipulation within functions, enhancing the versatility and reusability of code. This aspect proves beneficial in this article as it facilitates the creation of modular and scalable programs, promoting code clarity and organization. The unique feature of Passing Arguments lies in its ability to pass data between functions, enabling seamless communication and coordination within a program. While Passing Arguments streamlines code structure and promotes code reusability, improper handling of arguments can lead to logical errors and program malfunction, necessitating meticulous attention during implementation.
Recursive Functions
Recursive Functions introduce a powerful programming technique, enabling a function to call itself during execution. This recursive approach offers an elegant solution to solving complex problems by breaking them down into simpler sub-problems. The key characteristic of Recursive Functions is their iterative nature, allowing for succinct and efficient code implementation for repetitive tasks. Utilizing Recursive Functions in this article enhances algorithmic design and problem-solving capabilities by introducing a structured and iterative logic flow. The unique feature of Recursive Functions lies in their ability to streamline code complexity and offer a clearer path to resolving intricate problems. While Recursive Functions offer advantages in terms of code readability and problem-solving efficiency, an improper recursive design can lead to stack overflow and performance issues, necessitating careful consideration in recursion depth and termination conditions.
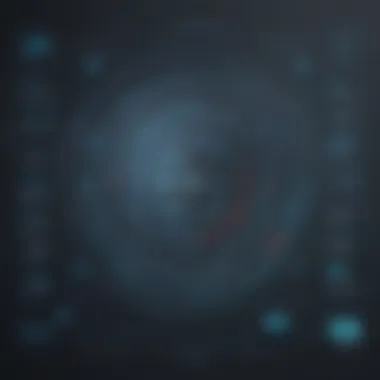
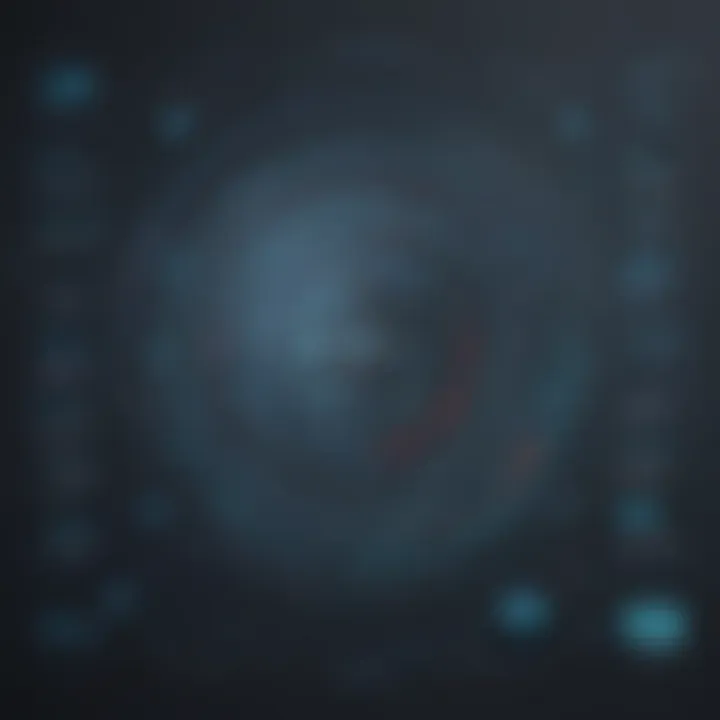
File Handling
O Operations
IO Operations stand as a fundamental aspect of C programming, enabling the interaction between programs and external files. The key characteristic of IO Operations lies in their capacity to read and write data to and from files, facilitating data storage and retrieval mechanisms. In this article, the integration of IO Operations enriches the practical application of C programming by enabling programs to save and retrieve information from files seamlessly. Despite their benefits, IO Operations may introduce potential vulnerabilities such as file corruption or unauthorized access, mandating rigorous error handling and security measures during implementation.
Working with Files
Working with Files extends the functionality of File Handling by providing capabilities to create, modify, and manage files within a program. The key characteristic of Working with Files lies in its versatility and flexibility in file management tasks, enhancing the program's ability to store and process data efficiently. Within this article, the incorporation of Working with Files enhances the data manipulation and storage aspects, offering a comprehensive approach to handling file-based operations. Despite offering diverse functionalities, Working with Files requires caution regarding file permissions, error handling, and data integrity to prevent unintended data loss or security breaches.
Debugging and Optimization
Debugging and optimization are crucial elements in the field of C programming, contributing significantly to program quality and efficiency. Debugging entails the identification and resolution of errors within the code, ensuring that the program functions as intended. Optimization, on the other hand, focuses on enhancing the performance of the program by refining its logic and structure. In this article, we will delve into the specifics of debugging and optimization, shedding light on key techniques and strategies to elevate your programming prowess.
Identifying and Fixing Errors
When it comes to debugging, the utilization of common debugging techniques plays a pivotal role in ensuring code reliability and functionality. These techniques encompass practices such as step-through debugging, logging, and unit testing, allowing programmers to pinpoint and rectify errors effectively. Common debugging techniques facilitate a systematic approach to error detection and resolution, streamlining the debugging process for greater efficiency and precision.
Common Debugging Techniques
Common debugging techniques, such as breakpoint insertion and variable inspection, provide programmers with tools to analyze program execution and inspect variable values at critical junctures. By leveraging these techniques, programmers can identify erroneous code segments, track program behavior, and isolate bugs for resolution. The unique feature of common debugging techniques lies in their ability to offer real-time insights into program execution, aiding in the swift identification of errors and expedited debugging.
Using Debugging Tools
Debugging tools serve as indispensable aids in the process of error identification and resolution, offering advanced features for code analysis and debugging. These tools, ranging from integrated development environment (IDE) debuggers to standalone debugging software, enhance the debugging workflow by providing comprehensive insights into program execution and error locations. The unique feature of debugging tools lies in their robust functionality, allowing programmers to analyze program behavior at a granular level and facilitate efficient debugging with minimal manual intervention.
Performance Optimization
Performance optimization in C programming focuses on refining code efficiency and resource utilization for enhanced program performance. Two critical aspects of performance optimization include code refactoring and efficient memory management, which collectively contribute to program speed and reliability.
Code Refactoring
Code refactoring involves restructuring existing code to improve clarity, efficiency, and maintainability without altering its external behavior. By eliminating redundant code, optimizing algorithms, and enhancing code readability, refactoring enhances program efficiency and facilitates easier maintenance. The unique feature of code refactoring lies in its ability to enhance code quality without changing its functionality, ensuring improved performance and code manageability.
Efficient Memory Management
Efficient memory management techniques optimize program memory allocation and deallocation, preventing memory leaks and enhancing program stability. By utilizing dynamic memory allocation tools and implementing memory-efficient data structures, programmers can minimize memory overhead and boost program performance. The unique feature of efficient memory management lies in its ability to optimize memory usage, ensuring optimal program performance and resource utilization without memory wastage.