Mastering C Programming for Arduino Projects
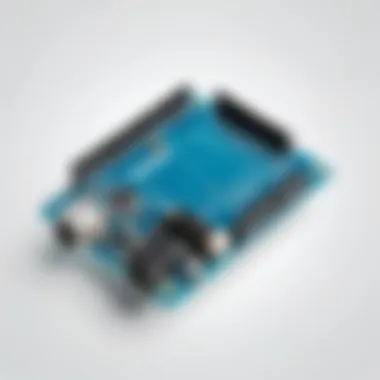
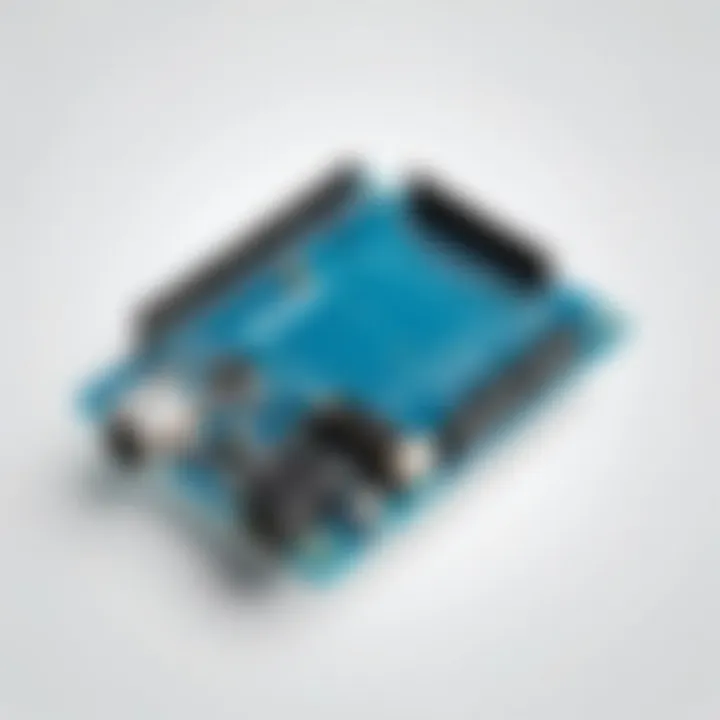
Intro
C programming serves as the backbone of many embedded systems, and with the advent of the Arduino platform, it has become an accessible entry point for countless students and hobbyists. Arduino revolutionizes how we perceive programming by allowing individuals to turn ideas into tangible projects. The unique ecosystem enhances the learning experience, facilitating an immersive journey into robotics and electronics.
This guide seeks to demystify the various elements of C programming tailored specifically for Arduino. Whether you're a novice just dipping your toes into the coding pool or an aspiring developer looking to hone your skills, this resource is designed for you. We’ll delve into fundamental concepts, practical applications, and examples that will solidify your understanding and enable you to create real-world projects.
Why for Arduino?
C has a robust history in programming, having sprouted from the late 1970s, designed initially by Dennis Ritchie at Bell Labs. This language's features—such as its efficiency and control over hardware—make it a suitable choice for Arduino programming. Unlike more abstract languages, C provides precise control over the microcontroller’s hardware resources.
As we journey through the realms of C programming for Arduino, our discussions will cover a variety of topics, including:
- Basic syntax and concepts fundamental to coding.
- Detailed exploration of hands-on examples, which will bolster your coding proficiency.
- Advanced topics like functions and exception handling, designed to give you depth in understanding.
Let's pave the way towards becoming a proficient programmer in the vibrant world of Arduino.
Prolusion to Arduino and Programming
Understanding the fundamentals of Arduino and C programming is essential for anyone stepping into the world of embedded systems and electronics. Arduino stands at the intersection of software and hardware, allowing enthusiasts and professionals alike to create interactive and functional projects with relative ease. By delving into the basics of C programming within the Arduino environment, individuals can effectively harness the power of this versatile platform to bring their ideas to life.
Overview of Arduino
Arduino is not just a simple circuit board; it represents an entire ecosystem that marries hardware and software seamlessly. This open-source platform serves as a gateway for developers at all skill levels to craft everything from simple LED blinks to complex robotics. At its core, each Arduino board is equipped with a microcontroller that processes inputs from various sensors and executes instructions laid out in the code.
The beauty of Arduino lies in its versatility. A common misconception is that one needs a strong background in electronics to get going, but that’s far from the truth. The platform provides abundant resources, including pre-defined libraries and a user-friendly Integrated Development Environment (IDE), making it more accessible for beginners. Moreover, the large community around Arduino contributes to forums and tutorials, providing solutions to common hurdles faced by novices. A key feature of Arduino is its compatibility with a wide array of shields and modules, enabling users to expand their projects as desired.
The Role of in Arduino Programming
C programming forms the backbone of Arduino sketch coding. It is the language that allows you to communicate with the board and control its functioning. With a syntax that’s more straightforward than many modern languages, C gives you an efficient means to write performance-centric code for your projects.
Key Benefits of Programming in Arduino
- Performance: C is a compiled language, meaning it turns your code into machine language before execution. This results in faster execution, which is critical in time-sensitive applications.
- Control: C offers granular control over system resources, like memory management. This is especially important in embedded systems where resources are typically limited.
- Learning Foundation: Mastering C programming can be a stepping stone to learning other languages. From web development to game programming, many languages share similar principles.
The integration of C in Arduino isn’t just about coding; it encapsulates the essence of problem-solving and designing solutions in a hands-on way. As you explore this guide, you will find that each concept builds on the previous ones, making it easier to grasp the intricate relationship between coding and hardware operation. In other words, it’s not just pushing text onto a screen; it’s about seeing how that text translates into real-world actions.
"The combination of Arduino’s accessible hardware and C’s powerful programming capabilities enables you to transform imaginative concepts into tangible outcomes."
In summation, the importance of understanding both Arduino and C programming cannot be overstated. This knowledge equips individuals with the tools to innovate and create projects that were once confined to the realm of imagination.
Getting Started with Arduino
Embarking on a journey with Arduino is akin to opening a treasure chest filled with possibilities in the world of electronics and programming. Understanding the essentials of setting up your Arduino environment is crucial. This step lays the foundation for success in your future projects. Whether you're a seasoned coder or just stepping into programming, the importance of the initial setup cannot be overstated. A well-prepared workspace boosts productivity and creativity, allowing you to focus on innovation rather than troubleshooting issues caused by improper configurations.
Setting Up the Arduino IDE
The Arduino Integrated Development Environment (IDE) is the main tool you'll use to write and upload code onto your Arduino board. It's user-friendly, but there are a few hurdles to clear before you start cooking up your first sketch (that’s what Arduino calls a program, by the way.).
- Download the IDE: Head over to the official Arduino website. You'll find versions for Windows, Mac, and Linux. Simply download the one that matches your operating system.
- Install the IDE: Run the installer. Follow the prompts. Just a heads up, be sure to install any drivers suggested during installation, especially if you're on Windows.
- Connecting the Board: Use a USB cable to connect your Arduino board to your computer. When you plug it in, your computer should recognize the device.
- Configuration: Open the IDE, navigate to and select your Arduino model. This step is often overlooked, but it's pivotal for ensuring that the IDE communicates properly with your hardware.
After completing these steps, your IDE is ready, and you're all set to dive into the world of code.
Installing Necessary Libraries
Libraries in Arduino are like handy toolkits that save you from reinventing the wheel. They contain pre-written code that simplifies complex functions, allowing you to delve into sophisticated projects more efficiently. When starting out, you might not realize their significance but missing out on libraries will undoubtedly limit your project's potential.
To install libraries, follow this simple procedure:
- Finding Libraries: In the Arduino IDE, navigate to . This will bring up a Library Manager window.
- Searching and Installing: You can search for libraries by name or functionality. For beginners, look for libraries like Servo, WiFi, and LiquidCrystal to get started with various components. Once you find a library of interest, hit the Install button.
- Using Libraries: Once installed, libraries can be included in your sketch by adding at the top of your code.
This small step can open up new avenues in your projects!
Creating Your First Project
Now that your IDE is installed and your libraries are ready, it’s time to put theory into practice. The first project you create should be simple, yet it should also encapsulate the core concepts of Arduino programming. A classic beginner project is blinking an LED. It's straightforward and serves as a solid introduction.
Here's how to get started:
- Connect an LED to Your Board: Use a breadboard and jumper wires. Connect one leg of the LED to digital pin 13 on the Arduino and the other leg to ground.
- Writing the Code: Open a new sketch in the IDE and enter the following code:
- Upload the Code: Click on the upload button (the right arrow icon) in the IDE. If all goes well, your LED should start blinking!
This small project not only gives insight into the fundamentals of programming with Arduino but also serves as a rewarding first step into the realms of electronics. Each flicker of the LED is a testament to the skills you're beginning to acquire.
"Every journey begins with a single step, or in this case, a single blink."
Through these initial stages, you'll form the crucial links between theory and application, paving the way for more complex projects down the line. With these foundations in place, you're now ready to advance your skills and explore the myriad opportunities that Arduino has to offer.
Fundamental Concepts of Programming
The foundational bricks of C programming are essential for anyone diving into the world of Arduino development. Understanding these concepts not only aids comprehension of the programming language but also enhances the overall efficiency of coding and troubleshooting in Arduino projects. Imagine standing at the base of a tall skyscraper; without a solid foundation, that majestic structure might tumble down. Likewise, mastering the fundamental concepts of C is vital to building robust applications that can interact effectively with hardware.
Understanding Data Types
In programming, data types function like categories, defining the kind of data that can be stored and manipulated. C programming offers a variety of data types such as integer, float, char, and more. Knowing these types is crucial for allocating memory and ensuring that operations on data are performed correctly. For instance, using an integer data type when you actually need a float can lead to unexpected results—like slicing and dicing a decimal into a whole number.
The primary data types you'll encounter are:
- int – for integers, whole numbers.
- float – for floating point numbers with decimals.
- char – for single characters.
- void – indicates no value.
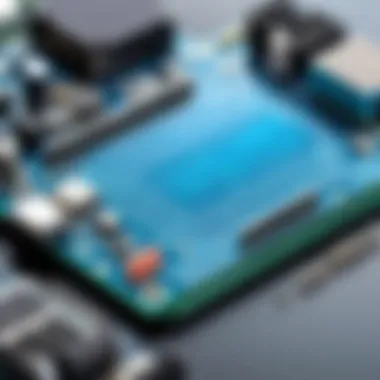
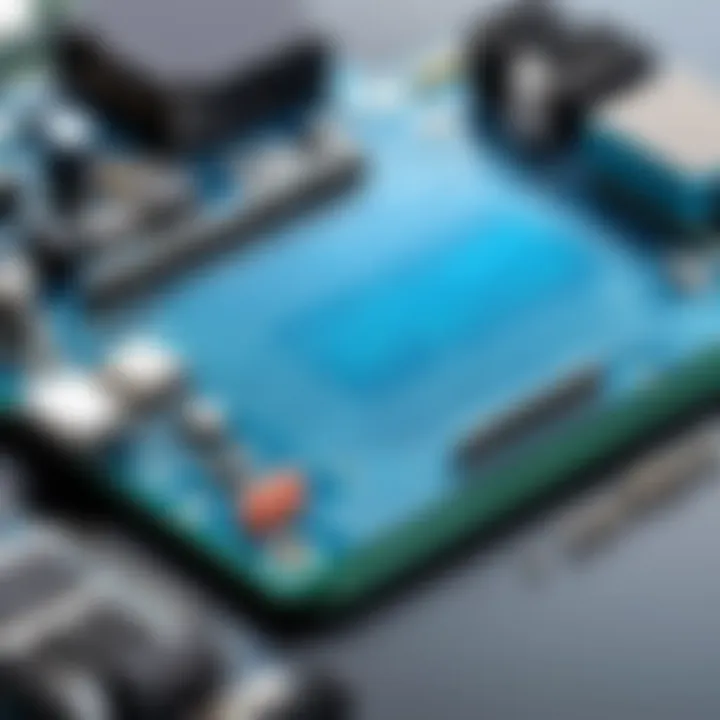
These data types are important not just for declaring variables but also for controlling the behavior of functions in your code. Misunderstandings here can derail even the simplest of programs.
Control Structures
Control structures dictate the flow of execution of code, enabling the program to make decisions, repeat tasks, or change paths based on certain conditions.
Conditional Statements
Conditional statements allow your program to respond to different inputs or situations. They are pivotal because they enable checks and balances within the code. For example, if you're reading data from a sensor, the code might check if the value is over a certain threshold. If so, it can trigger an alert or change the operational mode of an actuator.
A typical conditional statement in C looks like this:
The versatility of these statements makes them a popular choice in various projects, as they allow you to make logical decisions in your programs. However, using too many nested conditions can lead to complex, tangled logic, which can be a downside when maintaining or debugging code.
Loops
Loops are powerful structures that allow repetitive execution of blocks of code without the need to manually repeat them. This capability is particularly useful in scenarios where a task must be performed multiple times, such as reading multiple sensor values within a fixed period.
In C, the common types of loops include:
- for loop – for executing a block of code a specific number of times.
- while loop – continues executing as long as a given condition is true.
Example of a simple while loop:
Their ability to simplify code and improve efficiency makes loops indispensable in programming. However, using loops incorrectly can lead to endless repetition, commonly known as infinite loops, which can stall your system.
Functions and Scope
Functions encapsulate blocks of code into reusable segments that can be called upon whenever needed. This organization is not just more efficient; it makes your code cleaner and easier to follow. The concept of scope comes into play here, defining the visibility and lifetime of variables.
For instance, a variable declared in a function is not accessible outside that function, which is a fundamental aspect of programming in C. Understanding how scope works helps avoid conflicts and makes your code more modular.
Functions in a program can also return values, which allows for greater flexibility and modularity. Properly structuring functions and respecting variable scope are crucial for building well-organized code.
Each of these fundamental concepts contributes significantly to effective C programming within the Arduino environment. Mastery of these elements enables you to build increasingly complex applications, interact with hardware more effectively, and ultimately, brings your creative ideas to life. Remember, a strong understanding of these basics is like having a map while navigating a new city; it provides guidance and clarity, helping you avoid pitfalls along the way.
"Programming isn't about what you know; it's about what you can figure out." - Chris Pine
Working with Arduino Hardware
When it comes to developing with Arduino, working with hardware is a pivotal part of the journey. It’s not just about writing code; it’s about how that code interacts with the physical world. This section dives into the crucial elements of Arduino hardware, exploring its nuances, benefits, and a few considerations that developers should watch out for.
Arduino boards serve as the brain behind many projects. Comprehending the various types and their capabilities is key for any budding programmer or hobbyist. With a multitude of models available, ranging from the compact Arduino Nano to the feature-packed Arduino Mega, understanding each type's strengths can significantly influence project decisions.
Another major aspect is the input and output capabilities. The way an Arduino receives information from sensors and sends commands to actuators determines the scope of what can be achieved. Being aware of these functionalities not only enhances one’s programming skills but also opens the door to innovative project ideas.
So, while it might seem like a rabbit hole to go down, grasping Arduino's hardware elements will empower individuals to implement their ideas effectively.
Understanding Arduino Boards
Arduino boards are the cornerstone of projects. Each board functions similarly, but variations cater to different project demands. For instance, the Arduino Uno is often the starting point for most beginners, as it's adaptable to various projects. Meanwhile, the Arduino Due provides more processing power and supports advanced applications.
An important takeaway is the form factor. Some boards are made for compactness, others for versatility, and some for raw power. When deciding on which board to use, consider the project's size and complexity.
Here’s a quick rundown of a couple of popular boards:
- Arduino Uno: Ideal for learning and straightforward projects.
- Arduino Mega: Suited for complex tasks that require more I/O ports.
- Arduino Nano: A compact choice for smaller, more delicate applications.
Input and Output in Arduino
Input and output functionality plays a critical role in making an Arduino actually work in the real world. Understanding this topic is essential for anyone looking to build projects that effectively respond to their environment.
Digital /O
Digital I/O is a core concept in Arduino programming. It involves using pins to read or send binary signals—either HIGH (on) or LOW (off). This simplicity is one of the reasons it sits at the heart of many Arduino projects.
The strength of digital I/O lies in its versatility. For example, controlling LEDs is a quintessential task teaching beginners about binary signals. It’s straightforward enough for newcomers while still providing a solid foundation for more complex work down the line. Keeping the wiring and setup simple encourages experimentation and learning through success, or even failure.
Nevertheless, while digital I/O is invaluable, there are limitations. Because it only reads two states, it may not suit applications needing nuanced data input or output, such as measuring temperature with precision.
Analog /O
On the other hand, Analog I/O introduces complexity that opens new doors. Unlike digital pins, analog pins can read a range of values, allowing for more subtle input such as varying sensor readings or controlling motors with precision. The ability to read these continuous signals makes it a powerful tool for many applications.
One primary characteristic of analog I/O is its use of pulse-width modulation (PWM), allowing one to simulate analog outputs using digital pins for tasks like dimming LEDs or controlling motor speed. Such techniques elevate a project’s functionality and make them a popular choice among developers.
However, working with analog I/O comes with its own set of challenges. The analog readings can fluctuate and may require filtering or conditioning to ensure accuracy, which adds an extra layer of complexity. Thus, while it offers expansive capabilities, careful planning and understanding of its intricacies are necessary for effective implementation.
In summary, revisiting both the digital and analog I/O capabilities enables programmers to maximize their understanding and launch successful projects.
"Understanding the hardware is as vital as the code itself."
Whether it’s about interfacing sensors or controlling actuators, knowing these facets will enhance your Arduino experience and lead to more innovative creations.
Implementing Sensors and Actuators
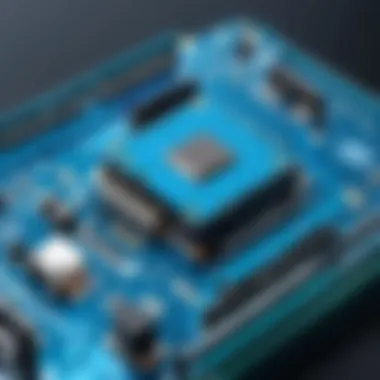
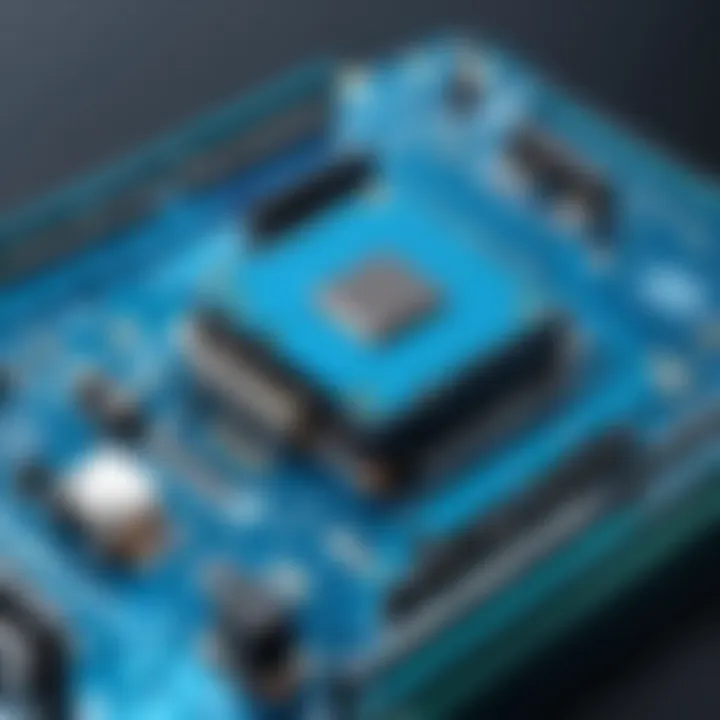
Implementing sensors and actuators in Arduino projects forms a cornerstone in creating interactive and responsive electronic systems. This combination enables a microcontroller to communicate with the physical world, interpreting environmental changes and responding accordingly. Understanding how to effectively use these tools is vital, as they not only enhance the functionality of a project but also allow developers to experiment with a variety of application scenarios. This section delves deep into two subcategories: working with sensors and controlling actuators.
Working with Sensors
Sensors act as the eyes and ears of a project, collecting data from the environment that can be interpreted by the Arduino. They provide the necessary input to trigger responses from actuators. In this subsection, we'll examine two popular types of sensors: temperature sensors and distance sensors.
Temperature Sensors
Temperature sensors play a critical role in many applications, from weather stations to smart thermostats. Their primary function is to measure changes in temperature and convert that information into a signal that the Arduino can read. A standout among these is the LM35, known for its accuracy and ease of use.
One of the most remarkable features of the LM35 is its analog output, which makes interfacing with the Arduino straightforward. It provides a voltage output that is directly proportional to the temperature in Celsius. This means less hassle in converting units for developers. This sensor is particularly beneficial for projects that require precise temperature readings without external calibration, making it a popular choice among hobbyists and engineers alike.
However, it’s worth mentioning a potential drawback. While the LM35 is accurate over a wide range, it can be susceptible to noise in certain environments, which may lead to fluctuating readings. Careful circuit design can help mitigate this issue, and it’s a small price to pay for its advantages.
Distance Sensors
Distance sensors enable Arduino projects to gauge proximity, which becomes vital for navigation systems and obstacle detection in robotics. One prominent example is the HC-SR04 ultrasonic sensor. This sensor emits ultrasonic waves and measures the time it takes for the sound to bounce back. By knowing the speed of sound, it calculates the distance with impressive accuracy.
The HC-SR04 excels in versatility and ease of use, making it popular within the Arduino community. It can measure distances from about 2 cm to 4 meters, capable of adapting to a range of applications—be it a simple robot or a more complex automation project.
Despite its merits, the HC-SR04 has limitations in terms of precision in certain environments, particularly where reflective surfaces may distort measurements, or in areas with ambient noise interference. Still, the advantages generally outweigh these drawbacks, especially for those learning about robotics and automation.
Controlling Actuators
Actuators are the engines of action, translating electronic signals back into physical motion or control. By using them in conjunction with sensors, it's possible to create dynamic, responsive environments. This section will focus on two common types of actuators: servos and motors.
Servos
Servos are incredibly useful for achieving precise movements in projects. They are typically employed in applications such as robotic arms and camera gimbals, where specific angles and positions need to be controlled. The standout feature of servos is the feedback mechanism within them, enabling them to know the position of the arm accurately.
Their reliability in delivering accurate positioning makes servos a favored choice for many Arduino projects that require repeatability and precision. They are usually easier to code with as well, thanks to libraries that developers can readily rely on for performing complex movements.
That said, one of the main downsides is that servos can draw a hefty amount of current, especially when fully loaded, which might require additional power sources in more demanding applications. Managing power effectively becomes key, particularly where multiple servos are involved.
Motors
On the other hand, DC motors are the go-to for applications requiring rotational movement. They are often found in projects like mobile robots or fans. Unlike servos, DC motors provide continuous rotation, allowing for more extensive motion—perfect for driving wheels or rotating components.
The simplicity of using DC motors is a vital characteristic, as they can run on simple voltage pulses, making integration straightforward. However, motors lack the precise control that servos offer; instead, they require additional components like encoders for position feedback if accuracy is a priority.
Another consideration with motors relates to controlling speed and direction, which necessitates the use of motor drivers. Depending on the use case, this can introduce added complexity but is necessary for achieving desired behavior.
In summary, both sensors and actuators are foundational to Arduino programming, allowing developers to create systems that observe and influence their environment—making the implementation of them highly valuable in any project.
Advanced Programming Techniques
Understanding advanced C programming techniques is essential for maximizing the capabilities of Arduino projects. As the complexity of your applications grows, so do the demands on your programming skills. Mastering these techniques allows you to write code that is not only efficient but also manageable and scalable. Advanced implementations like memory management, pointers, and data structures can profoundly enhance the functionality and performance of your Arduino programs.
Pointer Fundamentals
Pointers in C programming are variables that store memory addresses instead of data directly. They provide a way to directly access and manipulate memory, giving you powerful control over your data structures. This capability is especially crucial when working on embedded systems like Arduino, where memory constraints are often a significant factor. Understanding pointers enables efficient resource management, as you can allocate, access, and deallocate memory dynamically.
However, they come with their challenges. Mismanagement of pointers can lead to errors such as segmentation faults, which can be more challenging to debug. Thus, while they are powerful, using pointers requires careful consideration of memory use and the potential impact on program stability.
Dynamic Memory Management
Dynamic memory management involves allocating and freeing memory while the program runs. Unlike stack memory, which is fixed at compile time, dynamic memory gives flexibility in handling varying amounts of data. This becomes invaluable in Arduino programming when you want to allocate space for structures or arrays that change size during execution.
One common method for dynamic allocation is using functions like and . These methods allow you to request a specified amount of memory and later release it when it is no longer needed. This frees resources and helps prevent memory leakage, a common pitfall for novice programmers. However, it’s essential to ensure you free every piece of dynamically allocated memory to avoid these issues, which can lead your program astray.
Working with Data Structures
Arrays
Arrays are a cornerstone of C programming, particularly in Arduino work. They allow storage of multiple values in a single variable, making it easy to manage lists and collections of data. This characteristic makes arrays a favorite choice in projects where a series of related values needs to be processed, such as sensor readings.
A key advantage of arrays is their straightforward implementation and ease of access. You can iterate through an array with simple loops, making it a go-to solution for many programming problems. However, one downside is their fixed size; once declared, the size cannot change, which can be limiting in scenarios where the number of elements may vary significantly.
Structs
Structs enhance the capability of the C language by allowing you to create complex data types that group variables under one name. They are particularly beneficial in Arduino programming, where they can encapsulate related data such as sensor readings and their respective timestamps.
One defining feature of structs is their ability to combine different data types into a single entity. This makes it easy to transport and manage data as one unit instead of managing several associated variables individually. However, unlike arrays, accessing data within structs requires additional syntax, which could pose a slight learning curve for beginners. Despite this, the clarity and organization they provide make structs a staple in Arduino development, offering a pathway to rich data representation and manipulation.
Debugging and Troubleshooting
Debugging and troubleshooting are essential components of programming, particularly when working with embedded systems like Arduino. Understanding how to efficiently identify and correct errors not only enhances your coding skills but also helps in developing reliable and efficient applications. When something goes awry in your project, knowing how to trace the issue can save you from frustration and ultimately lead to better coding practices.
In the realm of C programming, issues can arise from syntax errors, logical missteps, or even hardware interactions. Each of these categories presents unique challenges that require different strategies and techniques to resolve. Familiarizing yourself with common pitfalls allows for quicker resolution and a smoother overall development experience.
Common Errors in Programming
When delving into C programming for Arduino, it's crucial to be aware of the frequent errors that programmers encounter. Here’s a closer look at some of the most common ones:
- Syntax Errors: These are the simplest type of errors where the code does not conform to the language rules. Forgetting a semicolon at the end of a statement or misplacing parentheses can lead to compilation failures.
- Logical Errors: These errors occur when the code runs without crashing, but the output is not as expected. A typical example is using the wrong condition in an if statement or miscalculating values in your algorithm.
- Runtime Errors: These errors arise when the code runs into situations that it cannot handle, such as dividing by zero or attempting to access an array out of its bounds. It's important to anticipate and handle these scenarios in your code.
- Hardware Interaction Errors: When working with sensors and actuators, hardware-related issues can cause failures that are hard to trace. Incorrect wiring, faulty components, or configuration mistakes can lead to unexpected behaviors.
By recognizing these errors, you can better prepare yourself to tackle them when they arise, avoiding the classic pitfalls that many new programmers face.
Debugging Techniques
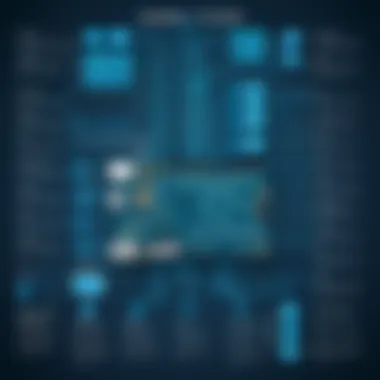
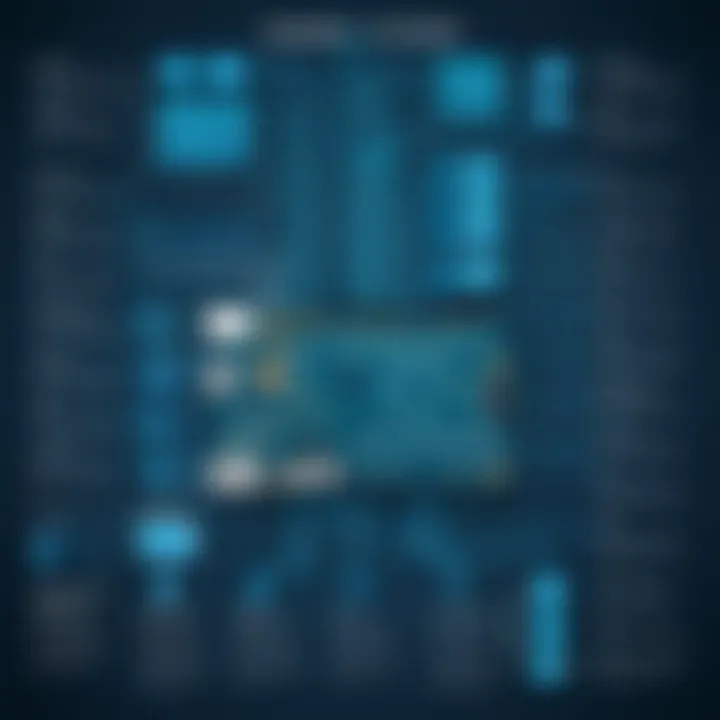
Once you’ve identified errors, it’s time to tackle debugging. Here are several techniques that can help you debug C programs effectively:
- Print Statements: A simple yet effective method is inserting print statements to track variable values and the flow of execution. For example, you might want to log the values of variables during an iteration to see where things go astray.
- Arduino IDE Debugger: Using the built-in debugging tools available in the Arduino IDE can assist you in pinpointing errors more efficiently. With this tool, you can set breakpoints and step through the code to monitor how it executes.
- Logic Analyzers and Oscilloscopes: When working with hardware, these instruments can help visualize signals and track down unexpected behavior resulting from electrical issues.
- Reviewing the Documentation: Often overlooked, revisiting the libraries and hardware documentation can provide insights into functionality that may not be behaving as intended.
By implementing these techniques, you can not only solve current issues but also build a strong foundation for future projects, ensuring you’re well-prepared for the uncertainties that can arise during programming.
Integration with Other Technologies
In today's world, the interplay of different technologies plays a pivotal role in maximizing the potential of any project. This section delves into the integration of Arduino with various technologies, highlighting the significance and advantages while offering insights into practical applications. Incorporating external technologies enhances the capabilities of Arduino, opening pathways for innovative solutions and more complex functionalities.
Interfacing with Bluetooth and WiFi
The advent of wireless communication has revolutionized the approach to programming and project development. Through Bluetooth and WiFi, Arduino can connect to various devices, facilitating remote control and data exchange.
Connecting via Bluetooth is often the first step for those venturing into wireless technology. A common use case involves using Arduino to control devices like home appliances through smartphones or tablets. For example, employing a simple Bluetooth module, such as the HC-05, allows your Arduino to communicate with an app on your phone. Here's a basic setup you might consider:
- Hardware Setup: Connect the HC-05 module to the Arduino.
- Code Development: Use the built-in Bluetooth libraries in C to send and receive data.
- Mobile Interface: Design a basic mobile app using platforms like MIT App Inventor that sends commands to the Arduino.
This setup empowers anyone to craft a simple home automation control system without diving too deep into hardware complexities. It's not just about controlling motors or LEDs;
next, let’s peek at WiFi options. Integrating WiFi, perhaps via an ESP8266 module, transitions projects to more sophisticated arenas like the Internet of Things (IoT). Here, we observe applications that include remote monitoring and sensor data logging.
Some practical steps might look like:
- Connect the ESP8266 to your Arduino, ensuring proper power supply.
- Accessing APIs: Using libraries to send and receive HTTP requests can provide real-time data updates or control commands over the internet.
- Dashboard Creation: Leverage platforms like ThingSpeak to visualize the data remotely, enhancing the project’s interactivity.
The implications of this technology are vast. Now your Arduino board could communicate with multiple devices over great distances, offering timely data updates that keep you in the loop, whether monitoring weather conditions or managing a smart garden.
Using Cloud Services with Arduino
Cloud services can elevate Arduino projects from standalone systems to interconnected networks. By harnessing cloud capabilities, developers can store data and enable remote access from anywhere.
Engaging a platform such as Firebase can initially seem daunting, but the benefits greatly outweigh the learning curve. This setup allows for real-time database storage, meaning data from an Arduino can be logged instantly and accessed through various devices without constant physical connection to the hardware. Consider the following:
- Real-time Communication: For instance, building a weather station that collects data on temperature and humidity. As the data gets updated in your Firebase cloud, it can be accessed by anyone with the correct permissions almost immediately.
- Command Execution: You can trigger actions remotely. An example is an Arduino controlling relays based on tasks triggered from web inputs, managing home appliances or garden watering schedules all from the cloud.
Bringing it all together, integrating cloud services with Arduino systems unlocks features such as automated alerts, remote configuration, and data sustainability. It significantly cuts down on physical requirements while expanding the range of what can be achieved.
In summary, marrying Arduino with Bluetooth, WiFi, and cloud services showcases the sheer potential in modern programming. As technology evolves, so too does the need for developers to stay adaptable and forward-thinking. Embracing these integrations will undoubtedly equip you for the fast-paced progress of tomorrow’s tech landscape.
Real-World Applications and Projects
In the rapidly evolving landscape of technology, the power of C programming for Arduino shines through its practical applications. This section aims to underscore the significant impact that real-world projects can have on the understanding of C programming principles, fostering creativity and innovation among budding developers. The hands-on experience gained through building projects is invaluable, as it not only reinforces theoretical knowledge but also allows for a deeper comprehension of hardware-software integration and system dynamics.
The projects detailed in this guide serve dual purposes: they provide both educational value and tangible problem-solving capabilities. As the world increasingly shifts toward automation and smart devices, knowing how to harness Arduino's capabilities can open doors to various fields, including engineering, home automation, and robotics.
Home Automation Projects
Home automation is one of the most exciting areas where Arduino and C programming converge. The ability to control lights, appliances, and other electronic devices through programmable solutions is not just convenient but offers a glimpse into the future of smart homes. With the right mix of hardware and C programming finesse, anyone can create a personalized, automated environment.
A simple home automation project might involve setting up a system to automatically turn on outdoor lights at dusk. To achieve this, you can use a light-dependent resistor (LDR) with Arduino. When the LDR detects low light levels, it sends a signal to the Arduino to activate a relay connected to the lights.
"Through practical projects, learning becomes an engaging journey rather than just rote memorization."
Key benefits of home automation projects include:
- Improved convenience and energy efficiency in daily life.
- Enhanced understanding of sensor integration and actuators.
- Opportunities to explore IoT (Internet of Things) principles.
To start a home automation project, consider using Arduino kits that include basic components like relays, sensors, and LEDs. Begin with simpler tasks, gradually increasing complexity as your confidence grows. Not only does this build your coding skills, but it also deepens your understanding of the electronics involved.
Robotics Applications
Robotics is another realm where the fusion of C programming and Arduino applications flourishes. Whether it's a simple robotic arm or a fully autonomous mobile robot, the principles of C programming are essential for processing inputs and controlling outputs.
For instance, building a line-following robot can be a great introductory project. Using light sensors to detect the line on the ground, the robot's behavior can be programmed so that it follows the path continuously. This project requires a grasp of both hardware wiring and control logic, challenging the programmer to think critically about how to keep the robot on track.
Robotics projects not only spur creativity but also develop critical skills such as:
- Problem-solving and logical thinking.
- Real-time processing and control algorithms.
- Understanding of mechanics, electronics, and programming all at once.
Diving into robotics via Arduino allows newcomers to work on complex systems gradually, building essential skills needed in engineering and tech fields. It’s a fine blend of fun and learning—like playing with high-tech toys that make a difference.
In summary, real-world applications such as home automation and robotics represent the pinnacle of learning in C programming for Arduino. They bridge the gap between academic concepts and practical existence, preparing learners for careers in technology while fueling their creative impulses.
Epilogue and Future Perspectives
Understanding the intersection of C programming and Arduino is vital for both aspiring programmers and seasoned developers. This blend not only bolsters technical skills but also enhances creative problem-solving. Throughout the article, we've delved deep into the nitty-gritty of C language fundamentals, the nuances of hardware interaction, and the potential for integrating various technologies with Arduino. This comprehensive guide serves as a solid foundation, equipping readers with insights that extend beyond theory into practical application.
One of the significant benefits of mastering these skills is the ability to develop innovative solutions for real-world problems. From home automation to robotics, the understanding of how to write efficient C code for Arduino empowers you to bring your ideas to life. Moreover, as technology evolves, so does the demand for proficient programmers who can adapt to the changing landscape. This adaptability is crucial in a field that is constantly changing.
Summary of Key Learnings
In summarizing the key points from our exploration, it's important to note the following:
- Core Concepts: We’ve covered essential C programming concepts, including data types, control structures, and functions. These are the building blocks for any programming endeavor.
- Hardware Interactions: Understanding how to effectively communicate with sensors and actuators broadens your scope of projects significantly. Knowledge of both digital and analog inputs allows for rich interfacing and control.
- Practical Applications: Real-world projects provided examples that not only harnessed theoretical concepts but also held profound relevance, showcasing how practical these skills can be.
- Debugging & Troubleshooting: Learning to identify and resolve issues is as important as writing code. This article offers insights into how you can troubleshoot common C programming errors within the Arduino framework.
"Learning to program is a self-instruction, as life is a continual learning experience."
Future Trends in Arduino Programming
The future of Arduino programming appears promising, influenced by evolving trends in technology and application demands. Here are several anticipated trends:
- Increased Integration with IoT: As the Internet of Things expands, the role of Arduino in connecting everyday objects to the web will become more significant. Enhanced connectivity will open doors for smarter applications.
- Adoption of Machine Learning: Implementation of machine learning on Arduino platforms will allow for more intelligent projects capable of processing complex data and making autonomous decisions.
- Growing Community and Resources: The Arduino community is vibrant and ever-growing. This increased collaboration will facilitate the sharing of knowledge, resources, and projects, further spurring innovation.
- Sustainability Initiatives: As environmental concerns grow, Arduino projects focusing on sustainability—like energy-efficient systems or smart agricultural solutions—will likely proliferate.
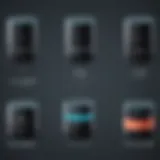
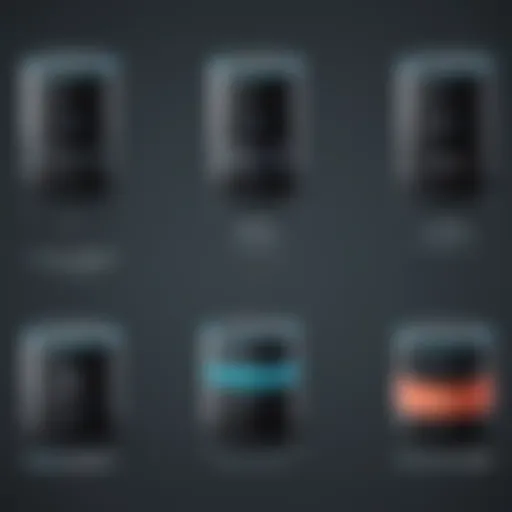