C Program Software for PC: A Comprehensive Guide
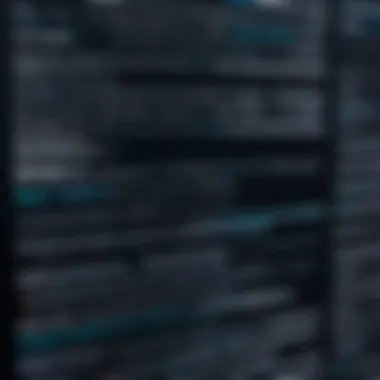
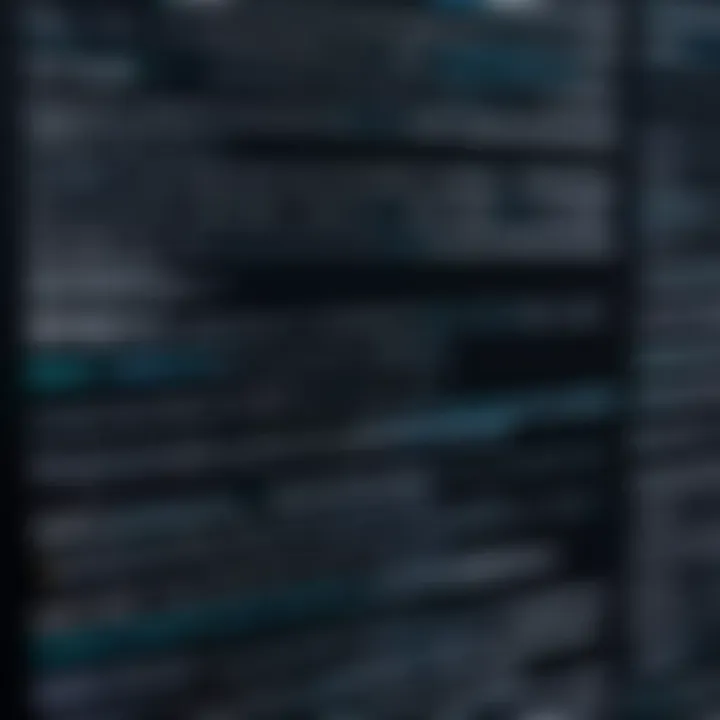
Preface to Programming Language
Programming languages are the backbone of software development. They allow developers to communicate with computers and instruct them to perform tasks. Among these programming languages, C stands out due to its simplicity and power. It was developed in the early 1970s by Dennis Ritchie at Bell Labs. Its design is centered around giving control to the programmer, making it widely adopted for system and application programming.
History and Background
C programming language was created to write system software for the Unix operating system. This connection helped establish C as a popular language in the computing world. Its syntax and structure influenced many modern languages like C++, Java, and Python. The language evolved through the years, with the ANSI C standard being established in the late 1980s. This standardization solidified its role in academia and industry.
Features and Uses
One of the key features of C is its efficiency. The language allows low-level memory manipulation, providing programmers with the ability to optimize performance. C is widely used for system programming, embedded systems, and developing applications where performance is crucial. Its portability means code written in C can run on various platforms with little modification.
Popularity and Scope
C remains highly relevant in various fields of programming. It is taught in many computer science courses, serving as an introductory language due to its clear structure and patterns. Its longevity in the industry is a testament to its robustness. Many operating systems, including Windows, Unix, and Linux, have components written in C. Its use continues to expand in areas like game development and high-performance applications.
C is not just a language; it's a foundational tool for understanding programming concepts and systems-level architecture.
Basic Syntax and Concepts
Understanding C requires familiarity with its syntax and fundamental concepts. These elements serve as the building blocks for effective programming.
Variables and Data Types
In C, a variable is a storage location with a name. Variables must be declared before use, which sets their data type. Common data types in C include , , and . Each data type serves a purpose based on the kind of data it holds. For example, is used for integers, while is for floating-point numbers.
Operators and Expressions
Operators in C perform operations on data. They can be categorized into arithmetic, relational, and logical operators. Expressions are combinations of variables and operators that evaluate to a value. For instance, is an expression that adds two variables.
Control Structures
Control structures dictate the flow of control in a program. The three primary types are sequential, selection, and iteration. Conditionals like and control the selection process, while loops such as and manage repetition in code.
Advanced Topics
As programmers gain proficiency, exploring advanced topics becomes essential for deeper understanding and capability.
Functions and Methods
Functions are blocks of code designed to perform a specific task. They improve code organization and reusability. A function in C has a name, return type, and can accept parameters. Understanding how to create and call functions is vital for effective programming.
Object-Oriented Programming
While C is not inherently an object-oriented language, principles from object-oriented programming can be applied through structuring programs. This allows developers to simulate behavior associated with classes and objects effectively.
Exception Handling
Handling errors gracefully is a crucial aspect of programming. In C, this typically involves using return values to signal errors, as well as understanding how to manage memory correctly. Proper error handling enhances the stability and reliability of applications.
Hands-On Examples
A practical approach to learning C involves writing simple programs and gradually moving to more complex projects.
Simple Programs
Creating a "Hello, World!" program is a common first step. This program introduces printing output:
Intermediate Projects
As skills develop, creating basic calculator programs or mini-games can help consolidate learning by applying more concepts.
Code Snippets
A code snippet to find the maximum of two numbers using if statements:
Resources and Further Learning
Diving deeper into C programming requires various resources that support learning.
Recommended Books and Tutorials
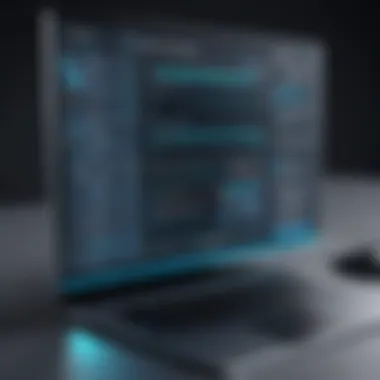
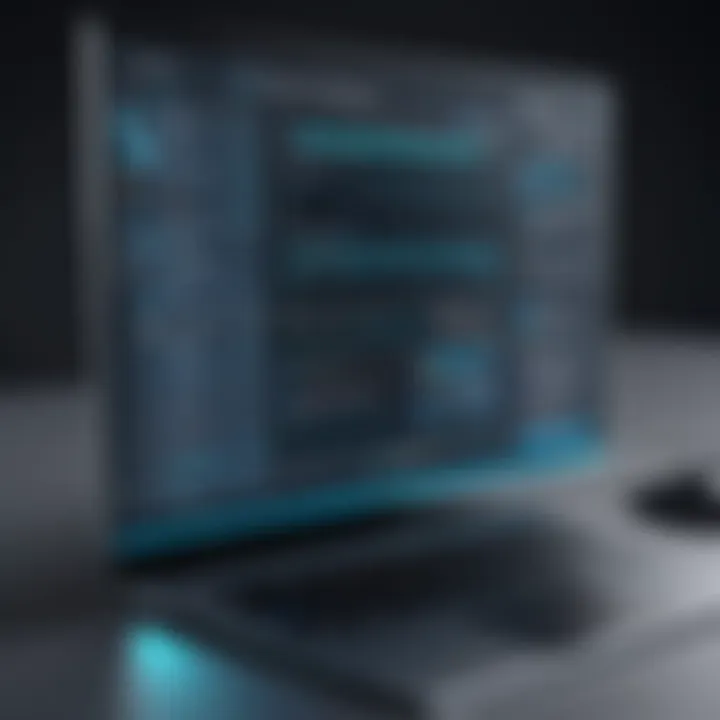
Books like "The C Programming Language" by Brian Kernighan and Dennis Ritchie provide comprehensive knowledge. Many online tutorials offer step-by-step guidance.
Online Courses and Platforms
Platforms like Coursera and Udemy offer C programming courses ranging from beginner to advanced levels. They allow for structured learning and practical exercises.
Community Forums and Groups
Joining forums like Reddit's r/programming or Stack Overflow connects learners with a community of fellow programmers. Sharing knowledge and getting feedback is vital for growth.
This comprehensive guide aims to serve as a starting point for those keen on mastering C programming. By exploring the topics outlined, readers can progressively enhance their skills and understanding.
Preface to Programming Software
Understanding the significance of C programming software is essential for both aspiring and seasoned developers. C language holds a pivotal role in the landscape of programming, acting as a foundation for many other languages. This section discuses various aspects that highlight the importance of C programming software, guiding readers towards deeper insights about the tools available.
Historical Context of Programming
C programming language was created in the early 1970s by Dennis Ritchie at Bell Labs. It was designed to enhance the functioning of the Unix operating system. At that time, programming resources were limited. C provided a way to write system-level programs efficiently. Over the years, C gained popularity due to its versatility and performance, becoming a backbone for many modern programming languages, such as C++, Java, and even Python. Cโs ethos of allowing fine control over system resources continues to appeal to systems programmers today.
The evolution of C programming also intersected with major technological shifts. In the 1980s, the ANSI C standard was adopted, enhancing its portability across different platforms. Understanding this history allows programmers to appreciate C's legacy and its design choices, which still influence software development today.
Importance of Language in Software Development
C programming language remains crucial in software development for several reasons. One major benefit is its performance. C allows developers to write high-performance applications close to the hardware level. This is particularly vital in system programming and embedded systems where resource constraints are common. Furthermore, C serves as a teaching language due to its simplicity and structured approach, making it ideal for learning fundamental concepts of computer science.
Another aspect of Cโs importance is its role in developing compilers for other languages. Understanding C gives programmers an edge in software engineering, enabling them to grasp more complex programming concepts easily. Additionally, major operating systems, including Linux and Windows, have components written in C, highlighting its relevance in real-world applications.
C language also encourages writing modular code through its support for functions. This aspect promotes code reusability and better organization, which are key for maintaining large software projects.
"C remains the language that stands the test of time in a world of constant technological change. Its applicability across domains ensures its continued relevance."
Ultimately, mastering C programming software equips developers with a robust toolkit for building efficient and portable applications. The growing demand for performance-oriented software underlines the necessity of understanding C in the development landscape.
Understanding Programming Software
C programming software encompasses a range of tools designed to aid in the development of software applications using the C programming language. This section aims to clarify the essential elements of C programming software, discussing its broad functions, benefits, and other considerations that make it significant for both learners and experienced programmers alike.
Definition of Programming Software
C programming software refers to any application designed to facilitate the writing, testing, and debugging of code written in the C language. These tools help developers manage the entire programming process more effectively. They can include text editors, compilers, and debuggers, among other utilities. The software can be as simple as a basic text editor, which allows for writing code, or as complex as an integrated development environment (IDE), which offers multiple functions in a single interface. Some popular C programming software includes Turbo C, GCC, and Clang; each offering unique features that address various programmer needs.
How Software Differs from Other Languages
C programming software has distinctive characteristics when compared to software for other programming languages. One notable difference is the way C handles memory management. C gives programmers a low-level access to memory, allowing for better performance but requiring more caution to avoid errors like memory leaks. Additionally, C requires explicit compilation, a process that transforms source code written by the programmer into machine code, something that is not always necessary in interpreted languages such as Python.
- C emphasizes both performance and control. Programmers often engage directly with system memory, maximizing speed.
- Languages like Java or Python abstract memory management from programmers, which simplifies development but may come at the cost of efficiency.
In summary, understanding C programming software is crucial for those looking to grasp the fundamentals of software development in the C language. This knowledge not only streamlines the development process but also equips programmers with the tools to create efficient, high-performance applications.
Key Features of Program Software for PC
The key features of C Program Software significantly contribute to its usability and accessibility for both novice and seasoned programmers. These software solutions serve a critical role in not only writing and executing code but also in debugging and providing a user-friendly environment. In this section, we will delve into specific elements such as compilation and execution, debugging tools, and Integrated Development Environments (IDEs).
Compilation and Execution
Compilation is a fundamental process in C programming. It involves converting human-readable source code into machine language that a computer's CPU can execute. Most C programming software includes a built-in compiler that performs this task efficiently. When a programmer writes code in a C editor, they must ensure it adheres to syntax rules. The compiler checks for errors during the compilation phase, providing immediate feedback. This instant feedback loop is crucial for learning, as it helps the user correct mistakes on the spot.
Once the code is free of errors, execution follows. Execution is the moment the compiled code is run, allowing programmers to see how their solutions perform. This feature allows developers to iterate quickly, testing different approaches and refining their programs.
In a typical workflow, a programmer:
- Writes the code in a text editor or IDE.
- Compiles the code to check for errors.
- Executes the code to see the outcome.
This workflow fosters a continuous learning process, which is essential in growing oneโs skills.
Debugging Tools and Their Importance
Debugging is an integral part of software development. It is the process of identifying and resolving bugs or errors within the code. C programming software often includes dedicated debugging tools that assist programmers in navigating and solving issues efficiently. These tools can set breakpoints, inspect variables, and analyze code line-by-line, making the task of finding bugs simpler.
The importance of debugging tools cannot be overstated. They save time and reduce frustration, especially for those who are still mastering the language. A well-designed debugging tool can lead to:
- Efficiency in fixing mistakes: Immediate insight into code behavior while running can quickly clarify issues.
- Improved understanding of code flow: Learning how the program executes can enhance a programmer's grasp of logical structures.
- Greater confidence: As errors are identified and corrected, programmers become more secure in their coding abilities.
"Debugging is twice as hard as writing the code in the first place. Therefore, if you're as clever as you can be when you write it, how will you ever debug it?"
โ Brian Kernighan
Integrated Development Environments (IDEs)
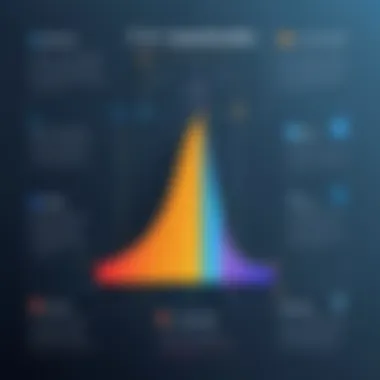
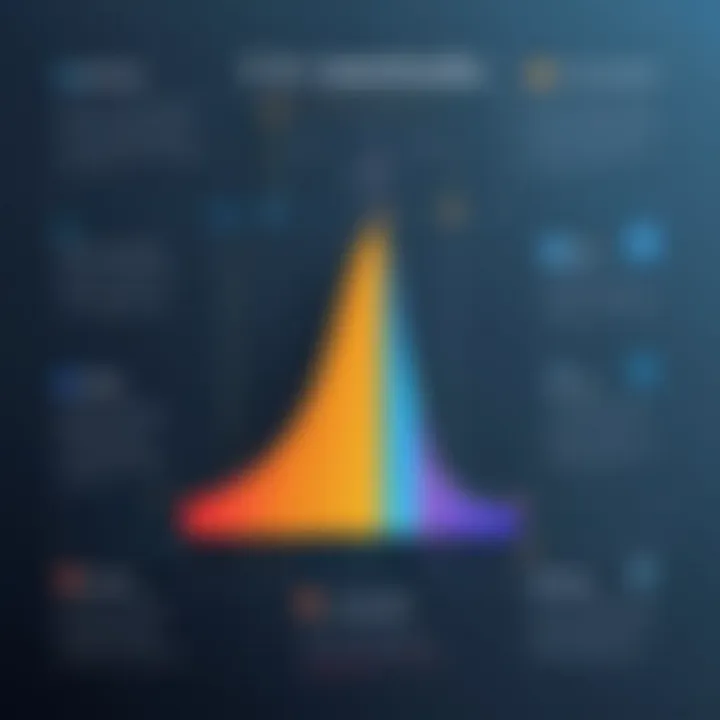
Integrated Development Environments, or IDEs, are software applications that provide comprehensive facilities to programmers for software development. The inclusion of an IDE can dramatically enhance the programming experience. C programming IDEs offer a suite of tools and features designed to optimize coding, including syntax highlighting, code completion, and project management.
Some popular IDEs for C programming include Code::Blocks, Dev-C++, Eclipse CDT, and Visual Studio. Each of these platforms provides unique features:
- Code::Blocks: It is open-source, lightweight, and highly customizable.
- Dev-C++: Known for its simplicity and beginner-friendly interface.
- Eclipse CDT: Offers extensive plug-in support and is favored in enterprise environments.
- Visual Studio: A robust platform with powerful debugging and profiling tools.
By using an IDE, programmers can focus more on writing code rather than managing the development process. The comprehensive tools in an IDE streamline the coding experience, allowing more time for creative problem-solving and less time troubleshooting environment setup issues.
Popular Programming Software for PC
In the realm of C programming, selecting the right software not only enhances coding efficiency but can also significantly impact the learning curve for new programmers. Different software options cater to varying needs, from beginner-friendly to more advanced users. Understanding the landscape of popular C programming software is crucial for anyone pursuing development in C or wanting to deepen their programming skills. This section provides valuable insights into notable C compilers and integrated development environments (IDEs) that can optimize the coding experience.
Overview of Notable Compilers
C compilers are critical tools for developers. They translate the C code into executable files, enabling the actual running of programs. Notable compilers include GCC (GNU Compiler Collection), Clang, and Microsoft C/C++ Compiler. Each of these compilers has its strengths.
- GCC is renowned for its portability and strong adherence to the C standard. It is often used in Unix-based systems.
- Clang is recognized for its fast compilation times and useful error messages, making debugging simpler for many users.
- Microsoft C/C++ Compiler integrates well with Windows systems, providing deep features for developers within the Visual Studio environment.
Choosing the right compiler depends on factors like platform compatibility, project requirements, and personal preferences.
Evaluating Different IDEs for Programming
IDEs greatly enhance productivity by combining tools relevant to C programming in one interface. Selecting an IDE can be overwhelming with the options available. Below are evaluations of four well-known IDEs:
Code::Blocks
Code::Blocks is a versatile IDE that is well-suited for both beginners and experienced programmers. Its strength lies in its open-source nature, which allows for extensive customization. One key characteristic is its ability to engage multiple compilers, giving users flexibility. This feature means that users can switch compilers if needed without changing the IDE itself.
Advantages of Code::Blocks include:
- Lightweight and fast
- Highly customizable layout
- Support for various operating systems
One possible disadvantage is that sometimes it may require additional plugins for advanced features, which may not be as smooth in installation as other IDEs.
Dev-C++
Dev-C++ is another popular choice among new programmers. Its simplicity is appealing, presenting a user-friendly interface. The key characteristic is its built-in MinGW compiler, which streamlines the setup process, making it easier for beginners to get started.
Key features include:
- Lightweight and straightforward installation
- Integrated compiler for ease of use
- Basic debugging features
However, its maintainance has become sporadic, leading to concerns about updates and long-term support.
Eclipse CDT
Eclipse CDT stands out with its robust set of features that cater to professional developers. It extends the capabilities of the Eclipse software framework, providing excellent support for C/C++ development. A significant feature is the powerful debugging tool integrated into the platform, which allows developers to identify issues efficiently.
Pros of using Eclipse CDT are:
- Strong support for large projects
- Rich plugin ecosystem for added functionality
- Comprehensive documentation
On the downside, the initial setup can be somewhat complex for new users, creating a steeper learning curve.
Visual Studio
Visual Studio is a comprehensive development environment favored among C programmers, especially for Windows development. It offers an extensive suite of tools for efficient coding and debugging. A unique feature is its IntelliSense capability, which provides intelligent code completion and suggestions, improving productivity.
Advantages include:
- Rich feature set with advanced debugging capabilities
- Deep integration with Git for version control
- Strong community support and resources
However, Visual Studio's size and resource demands can make it less suited for smaller projects or systems with limited resources.
Choosing the right IDE or compiler can significantly impact the coding experience and efficiency. By evaluating these options, programmers can select software that aligns with their skill level and project needs.
Installing Programming Software on PC
Installing C programming software on a PC is a pivotal step for any aspiring programmer. It opens the door to a world of coding possibilities. Understanding the right steps to take ensures a smooth process, leading to a productive programming environment.
Proper installation of software is not just about running a setup file. It requires careful consideration of several factors such as system requirements and compatibility. These elements can drastically affect your programming efficiency. Moreover, the installation process itself can sometimes present challenges, making it crucial to be well-prepared.
System Requirements and Compatibility Issues
Before diving into installation, evaluating system requirements is essential. Each C programming software, like GCC or Visual Studio, has specific hardware and software requirements.
- Operating System Compatibility: Ensure the software is compatible with your version of Windows, Linux, or macOS.
- Processor and RAM Requirements: Most modern IDEs recommend a minimum of 4 GB RAM and a dual-core processor. More complex software might need more.
- Disk Space: Check for sufficient disk space. Some software packages can exceed 1 GB after installation.
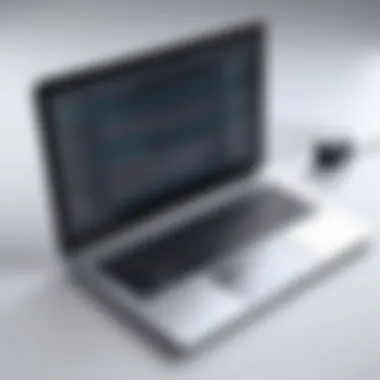
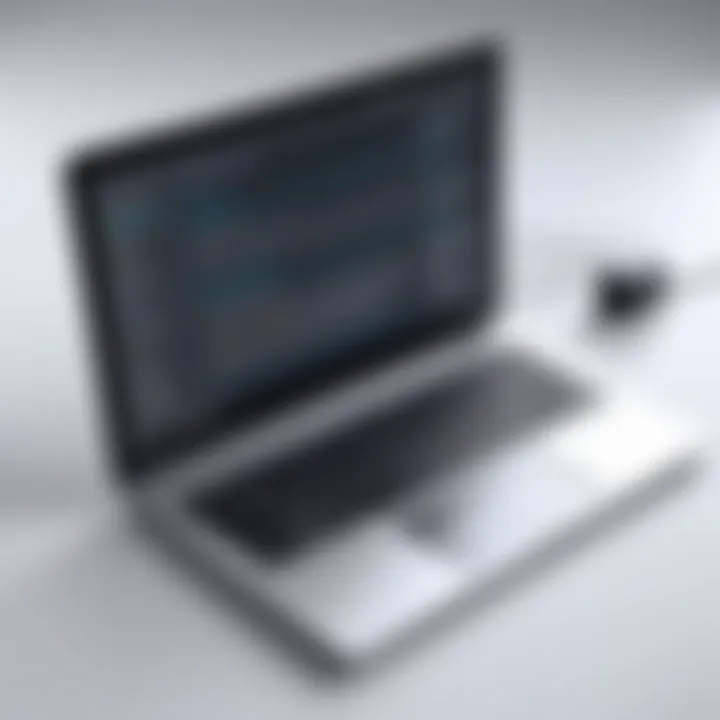
Assessing these requirements beforehand can save you from unexpected issues later.
Step-by-Step Installation Process
The installation process may vary slightly depending on the software you choose. However, here is a general guideline that applies to most C programming tools.
- Download the Installer: Navigate to the official website of the software you selected. For example, if you choose Code::Blocks, download it from the official website to ensure you get the latest version.
- Run the Installer: Locate the downloaded file and double-click it to begin installation.
- Follow On-Screen Instructions: During installation, follow the prompts. You will typically have options to select components and create shortcuts.
- Configure Environment Variables: After installation, configure environment variables, particularly if using a compiler like GCC. This allows your system to recognize commands in the command line.
- Launch the Software: Once installation completes, open the software to verify it works correctly. Creating a simple โHello, World!โ program is often a good test.
By adhering to these steps, you are setting a solid foundation for your C programming journey. A well-installed environment minimizes future frustrations, allowing you to focus on learning and creating.
Best Practices for Programming on PC
Best practices in C programming are indispensable for achieving a solid foundation and enhancing code quality. In this section, we will explore the principles that guide programmers toward better performance and maintainability. Whether you are a novice or someone honing their skills, understanding these practices is crucial.
Writing Clean and Maintainable Code
Writing code that is both clean and maintainable is a skill that comes with practice and awareness. Clean code enhances readability and eases collaboration among developers. Using meaningful variable names and avoiding overly complicated structures is key. Consider:
- Use consistent naming conventions: This makes it easier for others to follow your logic. Consider using camelCase or underscores to distinguish words.
- Break down large functions: Smaller, focused functions are simpler to test and modify.
- Keep indentation consistent: Proper indentation not only helps in readability but also prevents errors.
By adhering to these principles, you foster an environment where your future self and others can quickly understand and work with your code, reducing the chance of future bugs.
Using Comments and Documentation
Comments are vital to explaining the logic behind your code. They are not for replacing self-explanatory code but rather for clarifying the intent. Here are some points to consider:
- Explain why, not what: Your code should describe what it does effectively. Use comments to elucidate why a particular approach was taken.
- Document functions: Include headers for functions that explain parameters, return values, and behavior.
- Regularly update your comments: As code changes, comments must reflect those changes. Stale comments can create confusion.
Documentation can also extend to maintaining a README file or a changelog that details version updates and significant changes to your project. This practice creates a traceable history that can be invaluable to new collaborators.
Testing and Debugging Strategies
In C programming, testing and debugging are vital for ensuring your code functions correctly and efficiently. Adopting a systematic approach can streamline the process.
- Use assertions: Assertions can help catch issues during the development phase. They automatically check conditions of your code at runtime.
- Write unit tests: Consider employing a unit testing framework like CUnit to validate individual units of source code. This ensures that your functions behave as expected.
- Debug systematically: When bugs arise, isolate sections of code to identify the source of the issue. Tools like GDB can assist with this.
"Good code is its own best documentation."
By adhering to these practices, you position yourself for success and contribute positively to the programming community. For more insights on programming principles, consider exploring additional resources such as Wikipedia) or Britannica.
Common Challenges in Programming Software Usage
C programming can be rewarding but also presents several challenges that can be obstacles for learners. Understanding these challenges is crucial for both students and those who are learning programming languages. This section highlights common difficulties and offers solutions, ensuring a smoother programming experience.
Typical Errors and How to Address Them
Errors are an inevitable part of coding in C. They can stem from various sources, including syntax mistakes, logical errors, and type mismatches. Recognizing these errors is the first step to addressing them effectively.
- Syntax Errors: These occur when the rules of the C language are not followed. Missing semicolons or unmatched braces are common culprits. Compilers often highlight these errors, making them easier to fix.
- Logical Errors: These are more subtle. The program compiles and runs but produces incorrect results. To tackle these, you can use systematic testing methods or print statements to trace the execution of code.
- Type Mismatches: C is a strongly typed language, meaning every variable must be declared explicitly. Confusing data types can lead to errors. You can fix this by being diligent about variable declarations and using the right data types for specific tasks.
"Errors in C programming are not just learning experiences; they are stepping stones to mastery."
Troubleshooting Common Installation Issues
Installing C programming software can also present challenges. Various installation issues can hinder progress for beginners. Understanding these difficulties can streamline the installation process.
- Compatibility Issues: Ensure that the software you are installing is compatible with your operating system. Many compilers and IDEs require specific versions of Windows, macOS, or Linux.
- Insufficient Permissions: Sometimes, installation may require administrative rights. If you face this issue, try to run the installation file as an administrator.
- Missing Dependencies: Some C programming software requires additional libraries or components. Check the documentation to ensure all necessary dependencies are installed.
- Inadequate Storage: Ensure that your computer has enough space for the software. Low disk space can interrupt installations and lead to incomplete setups.
Addressing these challenges proactively can lead to a much more efficient learning experience, helping students focus on programming rather than installation issues.
Future of Programming Software
The future of C programming software holds considerable significance in the landscape of technology. As programming languages evolve, C stands as a cornerstone, laying the groundwork for many subsequent languages and paradigms. Understanding how C software will adapt and thrive in the ever-changing coding environment is essential not just for existing programmers, but also for those entering the field. Key areas such as emerging trends, the evolution of tools, and enhancements in usability factor into the discussions surrounding Cโs future.
Emerging Trends in Development Tools
In the realm of C programming, new trends are consistently reshaping development tools. One prominent direction is the rise of cloud-based IDEs. Such platforms allow developers to write and execute code in their browsers, facilitating collaboration regardless of geographical barriers. This increases accessibility and reduces setup complexities.
Another trend is the integration of AI-driven coding assistants. Tools like GitHub Copilot leverage machine learning to suggest code snippets, detect errors, and optimize algorithms in real-time. These advancements aim to streamline the coding process and improve efficiency, especially for beginners.
Furthermore, there is a growing emphasis on cross-platform compatibility. Developers are increasingly required to create applications that not only run on PCs but also function seamlessly on mobile devices and embedded systems. This need drives innovations in C software, guiding developers to create versatile solutions that can adapt to various environments.
"The evolution of programming tools directly influences how effectively developers can implement their ideas," stated a key figure in the field. This encapsulates the necessity for adaptive strategies in teaching C programming.
Continued Relevance in Modern Programming
Despite newer languages gaining attention, C maintains a pivotal role in modern programming. This relevance is attributed to C's efficiency and performance capability. Many operating systems, including UNIX and Linux, are built using C, underscoring its foundational impact on computing.
Moreover, C is lauded for its low-level memory access features, which are indispensable for system-level programming. Understanding these capabilities aids developers in optimizing performance-sensitive applications.
It is also worth noting that many educational institutions continue to teach C as an introductory programming language. This tradition keeps C relevant in an ecosystem that values the principles of programming. While languages like Python and JavaScript may dominate web development and high-level application programming, Cโs robustness ensures it remains a fundamental skill for aspiring programmers.
In summary, as programming evolves, C programming software will adapt to meet new challenges. From advancements in development tools to its enduring relevance in the curriculum, the future of C programming reflects a dynamic interplay between tradition and innovation. By recognizing and embracing these changes, programmers can enhance their capabilities and contribute to the tech landscape.
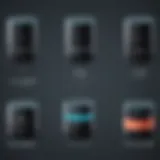
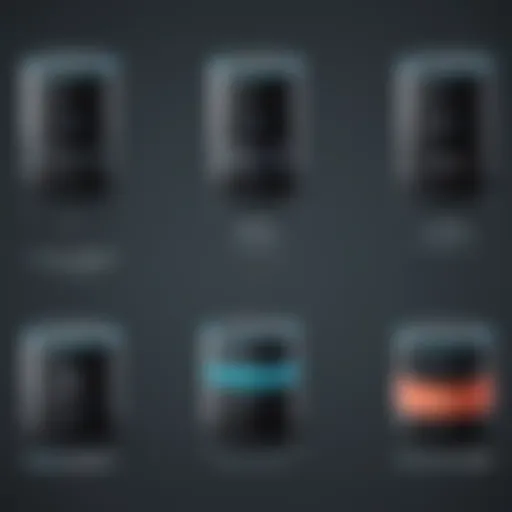