Building Graphical User Interfaces in Java: A Comprehensive Guide
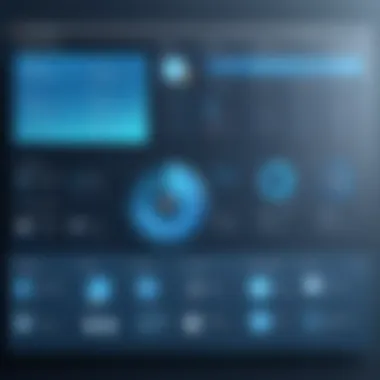
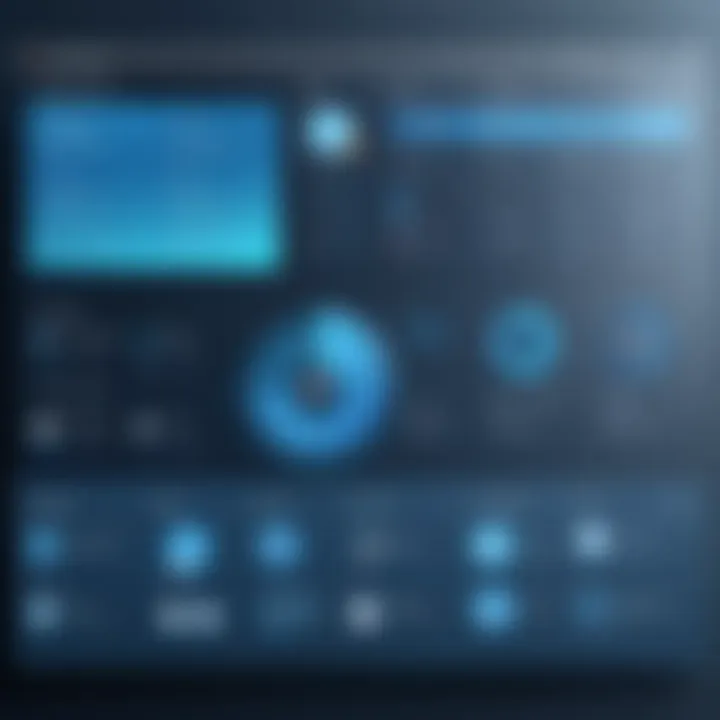
Prelude to Programming Language
Java has evolved into a powerful tool for developing applications, especially in the realm of Graphical User Interfaces (GUIs). Understanding Java's history provides context for how it became a prominent choice among developers.
History and Background
Java was created by Sun Microsystems in the mid-1990s. It aimed to be an object-oriented language that could run on different platforms without requiring major changes. Over the years, Java gained widespread acceptance due to its security features and versatility in application development.
Features and Uses
Java offers several features that make it suitable for GUI development:
- Platform Independence: Code can run on any device equipped with the Java Virtual Machine.
- Robustness: Java’s strong memory management and error handling make applications stable.
- Rich API: Extensive libraries facilitate quick development processes.
Due to these advantages, Java is used in various domains, including enterprise applications, mobile applications, and, of course, GUI development.
Popularity and Scope
Java remains one of the most popular programming languages in the world. Its community continues to thrive, offering resources and support. Frameworks like Swing, JavaFX, and AWT specifically cater to GUI creation, each with unique benefits. The scope of Java in GUI design is vast, covering everything from simple user interfaces to complex applications found in financial services, healthcare, and entertainment.
Basic Syntax and Concepts
Before diving into GUI development, it's crucial to understand Java's basic syntax and concepts that act as the foundation for building applications.
Variables and Data Types
Java variables must be declared before use. The basic data types include:
- int: For integers.
- double: For floating-point numbers.
- char: For single characters.
- String: For sequences of characters.
Operators and Expressions
Operators in Java perform various tasks. They are categorized into:
- Arithmetic Operators: +, -, *, /, %
- Relational Operators: ==, !=, >,
- Logical Operators: &&, ||, !
Expressions combine variables and operators to compute values, a fundamental part of programming logic.
Control Structures
Control structures direct the flow of execution:
- if statements: To execute code conditionally.
- for loops: For repetitive tasks.
- switch statements: For multi-way branching.
Advanced Topics
Once familiar with the basics, exploring advanced Java concepts is essential for effective GUI design.
Functions and Methods
Functions in Java are blocks of code that perform specific tasks. Methods are similar but are associated with a class. Understanding these will help organize code effectively.
Object-Oriented Programming
Java is an object-oriented language, emphasizing the concept of classes and objects. This approach allows developers to model real-world scenarios, which is particularly useful in GUI development.
Exception Handling
Exception handling is vital for creating robust applications. Using try-catch blocks helps manage errors gracefully, ensuring user interfaces remain responsive, even when issues arise.
Hands-On Examples
To cement learning, practical examples are invaluable. Below are illustrative projects to help students apply their knowledge.
Simple Programs
Start with a basic window using JFrame:
Intermediate Projects
Creating a calculator application serves as a great intermediate project. It integrates multiple Java concepts – utilizing buttons and displaying results in a text field.
Code Snippets
Code snippets are a great way to experiment with different elements of Java GUI development. Simple components can include buttons, text fields, and panels, to create interactive interfaces.
Resources and Further Learning
For further exploration, several resources are recommended:
- Books: "Effective Java" by Joshua Bloch is an excellent choice for deeper understanding.
- Online Courses: Platforms like Coursera or Udemy provide structured courses on Java GUI programming.
- Community Forums: Engaging in discussions on Reddit or Stack Overflow can lead to new insights and problem-solving techniques.
Investing time in Java GUI learning today will pay substantial dividends in applications you can create tomorrow.
Foreword to GUI Development in Java
Understanding how to build graphical user interfaces (GUIs) in Java is critical for many developers today. Java provides several frameworks that enable the creation of effective user interfaces. These allow users to interact with software applications more intuitively. This section lays the groundwork for understanding these principles and their significance in the realm of software development.
Understanding GUI Concepts
A graphical user interface facilitates interaction between users and computers through visual elements. Instead of relying solely on text-based commands, GUIs allow users to operate programs using graphical elements such as buttons, windows, and icons. This interaction is more intuitive for users, making applications more accessible.
Key concepts in GUI development include:
- Components: These are visual elements, like buttons or text fields, that users interact with.
- Containers: These hold components and organize layouts.
- Event Handling: This refers to managing user interactions like clicks or key presses.
Understanding these core concepts is essential as they form the basis of any GUI framework, particularly in Java. Mastery of these elements can result in more engaging and efficient applications.
Importance of GUI in Software Applications
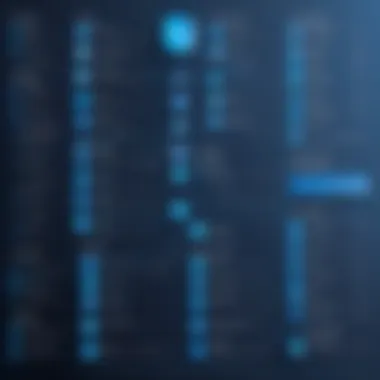
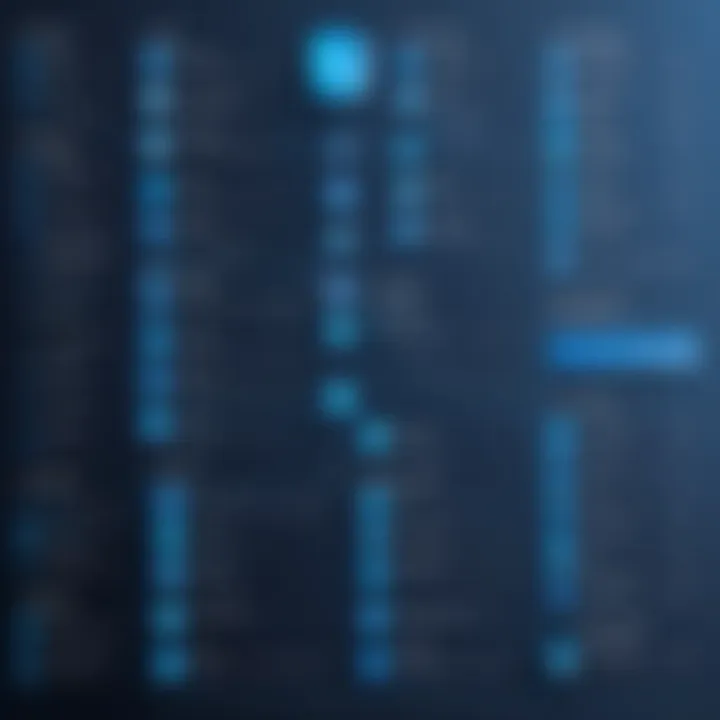
The necessity of GUIs in software applications cannot be overstated. They enhance user experience significantly, which can in turn improve user satisfaction and productivity. Applications equipped with GUIs are generally easier to learn and use, which is vital in today’s fast-paced technological landscape.
Some important reasons for the significance of GUIs include:
- Enhanced Usability: GUIs offer user-friendly access to complex functionalities.
- Increased Productivity: Users can accomplish tasks more quickly with visual navigation.
- Wider Audience Reach: Intuitive interfaces allow less tech-savvy users to engage with software.
Thus, GUIs are not just a nice-to-have feature; they are a fundamental aspect of creating software that users will embrace.
In summary, this section has provided a foundational understanding of GUI concepts and their importance in application development. This knowledge is critical as we move forward in exploring specific frameworks and tools available in Java.
Java Swing: An Overview
Java Swing is a pivotal toolkit in the realm of Java GUI development. Its significance lies in its ability to create sophisticated and portable graphical user interfaces. Swing is a part of the Java Foundation Classes (JFC) and offers a rich set of components and features that enhance the application development process.
Swing provides a flexible framework for designing user interfaces. It is built on the top of AWT (Abstract Window Toolkit) and overcomes many of its shortcomings, making it more versatile. This section aims to elucidate what Java Swing is and the benefits it brings to developers.
What is Java Swing?
Java Swing is a GUI toolkit that is part of the Java Standard Library. It allows developers to build graphical interfaces for Java applications. Swing is composed of components such as buttons, text fields, and tables that can be used to create interactive applications. Unlike its predecessor, AWT, Swing does not rely on the native system’s GUI but instead provides a more consistent look and feel across different platforms. This means that Swing applications can operate in a similar manner on Windows, Mac, and Linux operating systems.
Swing is lightweight, meaning that it does not require heavy system resources to run, which is an additional benefit for developers. It also supports a pluggable look and feel, allowing developers to create interfaces that can be easily customized to meet specific design requirements. This flexibility makes Swing a popular choice for developers looking to craft a unique user experience in their applications.
Advantages of Using Swing
The primary advantage of using Swing is its rich set of features. Some benefits include:
- Cross-Platform Compatibility: Swing applications can run on any platform with a Java Virtual Machine, ensuring a broad reach for your applications.
- Advanced Features: Swing includes a wide range of advanced components such as tables, trees, and lists, enabling developers to create complex and functional interfaces with ease.
- Customizable Appearance: The pluggable look and feel feature allows developers to change the appearance of their applications easily, enhancing user experience without significant additional overhead.
- Lightweight Components: The Swing components are mostly lightweight, which means they use minimal resources and can run smoothly even on less powerful machines.
- Rich Documentation and Community Support: Swing has extensive documentation and a dedicated community, which can be a valuable resource during the development process.
"Java Swing offers the flexibility and feature set that caters to nuanced requirements of GUI applications, making it a valuable tool for developers."
In summary, Java Swing is integral to GUI development in Java. Its rich feature set, customization options, and cross-platform capabilities makes it an indispensable component for developers aiming to build interactive applications.
JavaFX: Modern GUI Development
JavaFX represents a significant progression in GUI development within the Java ecosystem. As technology evolves, applications require interfaces that are not only functional but also visually appealing and responsive. JavaFX meets these requirements by offering advanced features that enhance performance, enrich user experiences, and simplify development. Understanding JavaFX is essential for developers looking to create modern applications that can run across various platforms seamlessly.
Foreword to JavaFX
JavaFX is a rich client platform for Java, which enables developers to create striking, visually impactful desktop applications and rich internet applications. Introduced as a successor to Swing, JavaFX leverages hardware-accelerated graphics to deliver more sophisticated user interfaces. This shift allows for smoother animations, enhanced multimedia support, and a more modern look and feel compared to its predecessor. Furthermore, JavaFX supports FXML, an XML-based language, which enables developers to separate user interface design from application logic.
The relevance of JavaFX is profound, especially when dealing with the increasing demands for interactive and user-friendly applications. It is also designed to run on various devices, including desktops, mobiles, and embedded systems, making it highly versatile for a variety of development scenarios.
Key Features of JavaFX
JavaFX offers several key features that distinguish it from other GUI frameworks. Some of these features include:
- Scene Graph: This structure hierarchically organizes graphical elements, making it easier to manage complex UIs.
- CSS Styling: Similar to web development, JavaFX allows the use of CSS for styling applications, promoting a clean separation between presentation and logic.
- FXML: This allows designers to work in an XML-based format. Thus, they can create the interface without writing Java code, which streamlines collaboration between developers and designers.
Additional features such as audio and video support provide developers with the tools to create immersive experiences without needing third-party libraries.
"JavaFX stands out not only for its rich features but also for its commitment to real-time interaction and responsive design, making it an ideal choice for modern applications."
JavaFX’s ability to easily integrate with Java libraries is significant. This compatibility facilitates the use of robust, existing Java frameworks and resources, which can save considerable time and effort in development processes. The active community surrounding JavaFX further enhances its utility, providing a wealth of resources, tutorials, and documentation.
Setting Up Your Development Environment
Setting up your development environment is a crucial aspect when working on Java Graphical User Interface (GUI) applications. It provides the foundation where all coding, testing, and debugging takes place. A properly configured environment not only enhances efficiency but also minimizes potential errors during development. In addition, it ensures that all necessary components are available for building and running your applications smoothly.
Required Software and Tools
Before diving into GUI development using Java, several tools and software should be installed to streamline the process. Common requirements include:
- Java Development Kit (JDK): Essential for compiling and running Java applications. Always ensure you have the latest version to access new features and bugs fixes.
- Integrated Development Environment (IDE): Employed to write and manage code. Popular choices include IntelliJ IDEA, Eclipse, and NetBeans. Each offers unique features that can aid in designing GUIs more effectively.
- JavaFX SDK: If opting for JavaFX, this package must be included. It provides ative libraries required for modern GUI development.
- Version Control System: Tools like Git can considerably help manage project revisions. This is particularly useful when collaborating with others or keeping track of changes made.
Additionally, consider installing build tools like Maven or Gradle. These tools can assist in managing project dependencies and automating build processes.
Configuration Steps
Once the necessary software and tools are acquired, the next step involves configuration, allowing you to create a functional development environment. Here are several steps to consider:
- Install the JDK: Download the JDK from the official Oracle website. Make sure to configure the environment variables correctly to ensure the Java commands can be accessed from the command line.
- Choose and Install an IDE: Select an IDE that suits your workflow best. Follow the installation instructions specific to the IDE chosen. After installation, configure the IDE settings to link it with the JDK.
- Setting Up JavaFX: If using JavaFX, download the JavaFX SDK from a reliable source. Unzip the SDK and note the path for future configuration in your IDE.
- Integrate Additional Tools: For those working with version control or build tools, install and configure them accordingly. Verify the integration by running a simple command in the terminal to ensure proper functioning.
- Create a Sample Project: Test the setup by creating a new project in your IDE. Make sure the environment is correctly set by running a basic Java application.
Following these steps will ensure your development environment is robust and ready for Java GUI programming. A well-prepared setup not only saves time but also enhances the coding experience as you progress in your development journey.
Creating Your First GUI Application
Creating your first GUI application in Java is a significant milestone for any developer beginning their journey into graphical interfaces. Understanding how to construct a GUI not only builds foundational skills in event-driven programming but introduces the developer to the principles of user interaction within applications. The process offers insights into the underlying architecture of GUI frameworks, as well as essential visual design elements that contribute to the user experience.
In this section, we will cover two crucial aspects of creating a GUI application: the basic structure of such applications and the implementation of a straightforward example that consolidates these principles. By comprehending these elements, developers can cultivate the knowledge necessary to design applications that meet user expectations and provide a clear path for functionality.
Basic Structure of a GUI Application
The basic structure of a GUI application typically includes several key components: the main frame, panels, components, and event listeners. Each component serves a vital role in ensuring the application functions effectively while providing an intuitive user experience.
- Main Frame: This is the primary window where all components reside. It acts as a container for other elements and typically defines the overall layout and behavior of the application.
- Panels: Panels are sub-containers within the main frame. They organize other components into logical groups, providing a structured visual appearance. Using panels effectively can minimize complexity and improve code readability.
- Components: These are the interactive elements of the GUI, such as buttons, labels, text fields, and menus. Each serves a specific purpose, allowing users to perform actions and view information.
- Event Listeners: Event listeners respond to user inputs, such as mouse clicks or keyboard presses. They are integral to the event-driven programming model, allowing the application to react appropriately to user actions.
Combining these elements forms the backbone of any GUI application. A well-structured application promotes maintainability and scalability, making it easier for developers to add features or adjust design as needed.
Implementing a Simple GUI Example
Let's implement a simple GUI example using Java Swing. This example will create a basic window with a button that responds when clicked. The following code demonstrates how to construct this application:
This simple application creates a window containing a single button labeled "Click Me!". When the button is clicked, a message dialog pops up, confirming the action. The structure follows the guidelines discussed earlier: a main frame that houses the button and an event listener that handles the button click.
This example provides a foundational understanding of how to build basic GUI applications. As you become more familiar with these concepts, building more complex user interfaces will become increasingly intuitive.
Components of a GUI
Understanding components of a Graphical User Interface (GUI) is fundamental for any Java developer aiming to build effective and user-friendly applications. Components are the building blocks that allow users to interact with software. Each component serves a specific purpose, contributing to a cohesive and functional interface. They can be anything from simple buttons to complex tables.
While developing a GUI, one must consider component selection carefully. This choice impacts usability and user experience. A well-chosen set of components can dramatically improve the functionality and intuitiveness of an application. Conversely, poor choices may confuse users, leading to frustration.
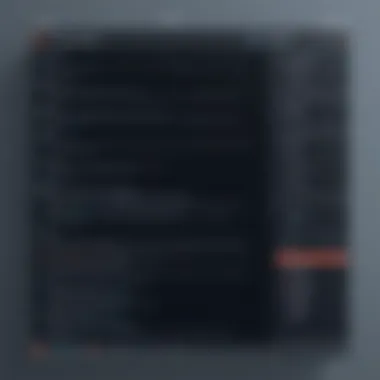
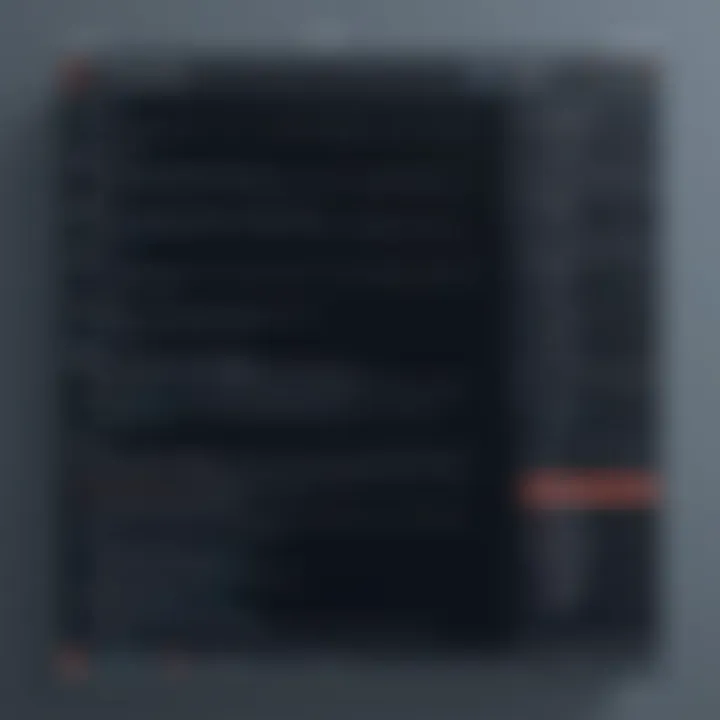
Common GUI Components
Numerous components are widely used in Java GUI development. Here are some of the most common ones:
- Buttons: Used to trigger actions. They are pivotal for user interaction.
- Labels: Display text or images but do not allow user interaction. They help provide context or instructions to the user.
- Text Fields: Allow users to enter text input. Important for forms and data collection where user input is needed.
- Check Boxes: Enable users to select or deselect options easily. Useful for preferences and multiple selections.
- Radio Buttons: Allow users to select one option from a set. They are critical when providing mutually exclusive choices.
- Combo Boxes: A combination of a text box and a drop-down list, allowing users to select an option or enter their own. Great for space-saving.
- Panels: Used to organize and group components. They help in managing layout complexity.
- Tables: Display tabular data in a structured format. Essential for applications dealing with large data sets.
Each of these components serves a unique role, and mastering them is imperative for effective GUI development.
Creating Custom Components
While the standard components fulfill many needs, sometimes they lack that necessary touch for unique user interfaces. Creating custom components becomes essential in such scenarios. Java provides flexibility and capability to develop components tailored to specific requirements.
To create a custom component:
- Extend an Existing Component: Start from existing classes. This method allows retaining default properties while adding new features.
- Override Methods: Add new functionalities by overriding default methods. This customizes the behavior of the component.
- Paint Methods: If the standard rendering does not fit your need, implementing custom paint methods helps in redesigning the visual output.
When creating these components, always keep user experience in mind. Ensure that your custom elements maintain consistency with the overall interface design. The result should blend seamlessly with standard components, ensuring that users find the interface intuitive and navigable.
"A user interface is like a joke. If you have to explain it, it's not that good."
Event Handling in Java GUIs
Event handling is a critical component of Java GUI development. It enables applications to respond to user actions such as clicks, keystrokes, and interactions with components. Understanding this aspect is crucial for creating dynamic and interactive user interfaces. When users engage with a GUI, their actions generate events that must be captured and processed appropriately. This section will dissect the principles of event-driven programming and the implementation of event listeners, both essential for responsive applications.
Understanding Event-Driven Programming
Event-driven programming is a paradigm used predominantly in GUI applications. In this model, the program flow is determined by events—system-generated or user-initiated actions. This paradigm allows developers to create applications that react to user input in real time, greatly enhancing usability.
The core of event-driven programming is the event loop. This loop continuously checks for events and triggers corresponding responses. For instance, when a user clicks a button, an event is generated, and the event handler is invoked to perform a specific action.
Key aspects of event-driven programming include:
- Decoupling: Event handling separates the user interface from application logic, allowing clearer and more manageable code.
- Asynchronous Processing: Events can be processed in parallel to the main application flow, making applications more responsive.
- Reactivity: Users receive immediate feedback based on interactions, improving the overall user experience.
Implementing Event Listeners
In Java, event listeners are interfaces that define methods to handle specific events. By implementing these interfaces, developers attach behavior to GUI components, making them dynamic and interactive. There are several types of event listeners in Java that cater to various events:
- ActionListener: Used for handling button clicks or menu selections.
- MouseListener: Monitors mouse events such as clicks and movements.
- KeyListener: Responds to keyboard input.
To implement an event listener, the following process is typically used:
- Define the Listener: Create a class that implements the respective listener interface.
- Override the Listener Methods: Implement the methods defined in the interface to specify what happens during the event.
- Register the Listener: Attach the listener to a GUI component using a method such as for ActionListener.
Here’s a simplified example of implementing an :
In this code, we create a GUI with a button. When the button is clicked, it triggers the method, printing a message to the console.
In summary, mastering event handling is essential for Java GUI development. It allows for creating engaging and responsive applications that meet user expectations. By developing a firm grasp of event-driven programming and the implementation of event listeners, developers can enhance their applications significantly.
User Experience Best Practices
User experience (UX) best practices are vital in the development of graphical user interfaces in Java. They ensure that applications are not only functional but also easy to navigate and interact with for users. A strong focus on UX can greatly improve user satisfaction and retention. Moreover, a positive user experience can enhance the perception of an application, leading to more favorable reviews and recommendations.
Creating Intuitive Interfaces
An intuitive interface is crucial for engaging users effectively. It allows users to achieve their goals with minimum effort. Key components include:
- Consistency: Interface elements should behave similarly across the application. Users should feel at home, recognizing patterns and expected behaviors.
- Feedback: Providing immediate feedback when users interact with an interface reassures them that actions are registered. This can be achieved through visual cues or audible sounds.
- Clarity: Buttons and labels should be clear and self-explanatory. Avoid jargon and opt for straightforward language that everyone can understand.
By implementing these components, developers create an atmosphere where users feel confident and informed when using the application.
Accessibility Considerations
Accessibility is an essential aspect that cannot be overlooked. It ensures that users with disabilities can effectively use the application. Guidelines like the Web Content Accessibility Guidelines (WCAG) can serve as a reference. Consider these practices:
- Color Contrast: Ensure there is sufficient contrast between text and background colors. This helps users with visual impairments read text easily.
- Keyboard Navigation: All interactive elements should be operable via keyboard shortcuts. This is beneficial for users who may not use a mouse.
- Alternative Text: Images and non-text content should have descriptive alternative text. This is vital for screen reader users, allowing them to understand visual elements in the interface.
In summary, putting focus on accessibility not only widens your user base but also shows a commitment to inclusivity. It is crucial to remember that a well-rounded user experience encompasses all users, regardless of their abilities.
Advanced GUI Topics
Advanced GUI topics are crucial for both the performance and usability of applications developed in Java. Mastering these areas can greatly enhance how an application operates, ensuring responsiveness and user satisfaction. This section will cover two major elements: threading in GUI applications and using layout managers effectively.
Threading in GUI Applications
Threading is a fundamental aspect of GUI development, particularly because GUIs are inherently event-driven. When developing applications, it's critical to keep the interface responsive while performing time-consuming tasks, such as data processing or network calls. If these tasks are performed on the main thread, the application may freeze or become unresponsive, leading to a poor user experience.
Java provides the class, which is a useful tool for managing background tasks in Swing applications. By employing , developers can execute long-running tasks in a background thread without freezing the GUI. This is how the flow typically works:
- Initialization: The class is instantiated, where you define the task that will run in the background.
- Execution: Calling will start the worker in a new thread.
- Updating GUI: After the background task is complete, you can safely update the GUI components using methods like for intermediate updates or for final updates.
Implementing threading correctly is essential. It ensures that the user interface remains interactive while performing necessary tasks. This is not just a recommendation; it's a core requirement in ensuring modern applications provide a robust user experience.
Using Layout Managers Effectively
Layout managers play a critical role in Java GUI development. They control how components are arranged within a container, allowing for flexible and adaptive interfaces. Java provides various layout managers, such as , , , and , each serving distinct purposes.
- FlowLayout: This is the simplest layout manager, arranging components in a left-to-right flow, much like lines of text. It's useful for simple forms and applications with fewer components.
- BorderLayout: This manager divides the container into five regions: North, South, East, West, and Center. This is effective for maximizing the use of screen space.
- GridLayout: Ideal for creating a grid of components, allows developers to organize components in rows and columns easily. It is suitable for structured data displays.
- BoxLayout: This manager arranges components either vertically or horizontally, providing an intuitive method for creating complex and responsive layouts.
Using layout managers effectively can mean the difference between a messy, unorganized application and a clean, professional one. They help ensure that GUIs adapt to various screen sizes and orientations, enhancing user experience across multiple devices.
Testing and Debugging Java GUIs
Testing and debugging are critical components in the lifecycle of any software development, particularly in Java GUI applications. As graphical user interfaces are directly interacted with by users, ensuring their correctness and usability is paramount. With the intricacies involved in GUI elements, thorough testing helps identify potential issues that could impair user experience.
Common Pitfalls and Issues
Developers often encounter several common pitfalls while testing and debugging Java GUIs. Understanding these can significantly reduce headaches during development. Here are some notable issues:
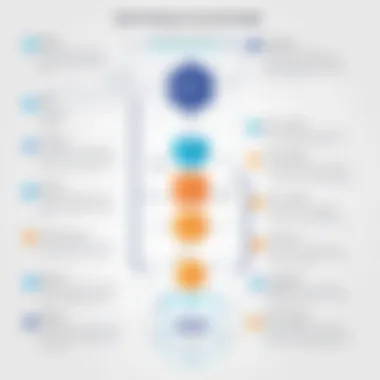
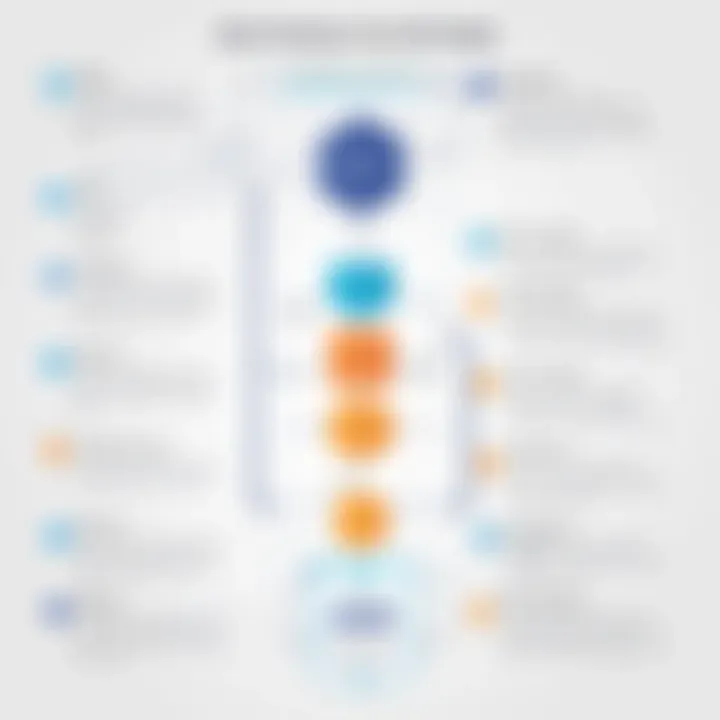
- Inconsistent Behavior: GUI components can behave differently depending on the underlying operating system. Testing across various platforms is essential to catch discrepancies.
- Event Handling Bugs: Event-driven programming in GUIs can lead to missed or improperly handled events, which may cause unexpected behaviors. Misalignment between event listeners and GUI actions is particularly common.
- Layout Issues: Improper use of layout managers can lead to components being displayed incorrectly, especially when the application is resized. Developers should rigorously test the application in different window sizes to uncover these issues.
- Resource Management: Leaking resources such as database connections or file handles can cause the application to slow down or crash. It is important to validate that all resources are appropriately released after use.
Addressing these issues requires a systematic approach. Developers should prioritize writing test cases that replicate user interactions and environmental conditions in which their application will run.
Testing Frameworks for Java GUIs
To aid in the testing of Java GUI applications, several frameworks exist. Utilizing these frameworks enhances the testability of applications and provides a systematic way to identify defects. Here are some notable testing frameworks:
- JUnit: A widely used framework for unit testing in Java. JUnit can be extended for GUI testing by integrating it with other tools designed for this purpose.
- TestFX: Designed specifically for testing JavaFX applications, TestFX provides a fluent API for functional testing. It simplifies the process of simulating user interactions and verifying expected outcomes.
- Abbot: This framework allows for automating tests of Swing-based applications. Abbot captures GUI interactions and can replay them, which helps in regression testing.
"Effective testing in GUIs not only catches flaws but also enhances user satisfaction by creating a reliable application."
Implementing a combination of these frameworks enables developers to cover a wide range of testing scenarios. It is essential to create a testing strategy that is both comprehensive and efficient, ensuring that every critical aspect of the user interface is accounted for.
By focusing on testing practices and strategies, developers can significantly improve the reliability and usability of their Java GUI applications. In a domain where first impressions matter, investing time in testing pays off in the long run.
Deployment of Java GUI Applications
The deployment of Java GUI applications is a critical phase in the software development lifecycle. It encompasses various steps, aimed at ensuring that applications are ready for use by the end-user. Proper deployment not only enhances user experience but also solidifies the application's performance across different systems. Therefore, understanding how to effectively deploy applications is essential for any developer aiming to ensure their software runs smoothly in real-world scenarios.
When it comes to deploying Java GUI applications, developers should consider several key elements. These include the packaging of the application, platform compatibility, and ease of installation. Addressing these factors is vital, as they directly impact user satisfaction. Benefits of effective deployment range from simplified application updates to improved security and reduced potential for user errors. A well-deployed application can lead to an increased adoption rate among users, which is often a major goal for software developers.
Packaging Applications for Distribution
Packaging applications for distribution is a fundamental part of deployment. It involves gathering all necessary resources, including libraries, configuration files, and any dependencies required by the application, into a single distributable format. This ensures that when users download and install the application, all components are included, minimizing issues that can arise from missing files.
Here are some considerations when packaging Java GUI applications:
- Dependency Management: Utilize tools like Apache Maven or Gradle to manage external libraries. This helps avoid compatibility issues.
- File Structure: Organize the application files logically. A clear structure aids users in locating specific components if issues arise.
- Documentation: Include user guides or readme files to provide users with instructions on installation and troubleshooting. This can significantly improve user experience.
Proper packaging ultimately leads to a smoother installation process and reduces the likelihood of errors post-deployment.
Creating Executable JAR Files
Creating executable JAR (Java Archive) files is another critical aspect of deploying Java GUI applications. JAR files bundle all the necessary class files and resources into a single file, making it easier for users to run the application. This format ensures that end users do not have to manually manage multiple files and directories, as everything is contained within one executable unit.
To create an executable JAR file in Java, follow these steps:
- Compile Your Java Files: Ensure that all your Java source files are compiled into .class files.
- Manifest File Creation: Create a text file named . This file should specify the main class of your application, which will be executed when the JAR is run. It typically looks like this:
- Package Files into JAR: Use the command available in the Java Development Kit (JDK) to create the JAR file. The command in the terminal would look like this:
- Test Your JAR File: Test the executable JAR to confirm that it runs correctly with the command:
Using JAR files simplifies distribution and ensures that users have a straightforward method to execute your Java applications effectively.
Resources for Learning Java GUI Development
Learning Java GUI development is crucial for both students and aspiring developers aiming to create engaging and functional applications. A good grasp of GUI design can greatly enhance user experience. Resources in this realm provide structured knowledge, practical examples, and community support which are invaluable for mastering the nuances of Java GUIs.
The importance of specific resources cannot be overstated. Books and courses can serve as excellent foundational tools. They often present concepts in a systematic manner, offering not just theories, but real-world applications. On the other hand, online communities play a significant role in ongoing learning. Interactions in forums allow developers to share experiences, troubleshoot issues, and collaborate on projects. This aspect is particularly beneficial in a fast-evolving field like GUI development.
Recommended Books and Courses
Several resourceful books and courses are available for individuals at varying levels of expertise. Here are some noteworthy recommendations:
- Core Java Volume II - Advanced Features by Cay S. Horstmann: This book deeply covers features in Java, including Swing and JavaFX.
- Java: A Beginner's Guide by Herbert Schildt: A great starting point for beginners, offering an introduction to Java programming and its GUI frameworks.
- JavaFX: A Beginner’s Guide by J.F. DiMarzio: This book focuses on modern GUI development with JavaFX, providing practical examples.
- Online Course: Java Programming and Software Engineering Fundamentals by Duke University on Coursera: A comprehensive course covering the basics of Java programming and applications.
These resources provide a solid understanding of Java GUI development fundamentals while equipping learners with the skills necessary for practical implementation.
Online Communities and Forums
Online communities and forums also play an essential role in learning. They offer places for developers to connect, discuss ideas, and seek assistance. Participating in these spaces fosters collaboration and encourages real-time learning. Some recommended communities include:
- Reddit's r/java: A community dedicated to informal discussions about Java, including GUI topics.
- CodeProject: A website hosting articles, tutorials, and forums regarding Java programming.
- Stack Overflow: A Q&A platform where both novice and experienced programmers can seek help and discuss problems.
- JavaRanch: A friendly community that focuses on all aspects of Java programming.
These platforms not only provide support but also expose learners to diverse perspectives, enhancing their understanding of various Java GUI concepts. By leveraging these resources, students and programmers can improve their competence and confidence in building effective user interfaces.
Future Trends in Java GUI Development
Java GUI development is at a crossroads as it encounters new challenges and opportunities. Understanding future trends is pivotal, not just for the enhancement of current practices, but also for anticipating the direction in which the technology will evolve. For developers, staying informed about these trends means they can leverage new tools and techniques to build more efficient, visually appealing, and user-friendly applications.
Emerging Technologies
The landscape of GUI development is increasingly being influenced by emerging technologies. Frameworks and libraries are evolving, driven by demands for enhanced performance and user engagement.
- Web-based Interfaces: As web applications gain prominence, many Java developers are shifting focus towards building web-based GUIs using Java tools like Vaadin and GWT. This trend allows developers to create rich, interactive user interfaces that can be accessed across various platforms seamlessly.
- Desktop Solutions with Modern Design: With tools like JavaFX gaining traction, developers can now create applications that possess modern aesthetics to rival native applications. JavaFX introduces features such as 3D graphics and CSS styling, essential for creating competitive graphical interfaces.
- Responsive Design Principles: The principle of responsive design is now becoming essential in GUIs. Developers must ensure that applications are adaptive to different screen sizes and resolutions, enhancing usability across devices.
- Artificial Intelligence Integration: Integrating AI into GUIs to create smarter user interfaces is another emerging trend. AI can anticipate user needs, offering personalized experiences and making applications more intuitive.
Adopting these technologies can lead to several benefits, including enhanced user experience and improved functionality. However, it is essential to consider that it may also require additional skills and familiarity with new tools and programming paradigms.
The Role of Java in Modern Development
As modern development shifts towards multi-platform solutions, Java remains a significant player. Its enduring popularity can be attributed to several factors:
- Cross-Platform Compatibility: Java's ability to run on various operating systems continues to make it a go-to language for GUI development, especially in enterprise settings. Its principle of "write once, run anywhere" enables developers to design applications that can function seamlessly across different platforms.
- Strong Ecosystem: Java boasts a robust ecosystem that includes libraries and frameworks specifically for GUI development. The continued enhancement of libraries like JavaFX and Swing remains crucial for maintaining relevancy in a fast-evolving landscape.
- Community Support: The extensive community surrounding Java facilitates knowledge-sharing and problem-solving. Platforms such as Reddit and various forums provide developers access to insights and assistance that can be invaluable.
- Commitment to Innovation: Java consistently adapts to modern needs, ensuring that it integrates with current trends and technologies. The consistent updates from Oracle and the open-source community help Java developers keep pace with the latest trends and tools available.
In summary, future trends in Java GUI development reflect a broader shift towards incorporating new technologies and user expectations. Keeping pace with these trends will enable developers to create superior applications that meet modern usability standards and embrace innovation.
Closure and Next Steps
The conclusion serves as an important segment of this comprehensive guide on Java GUI development. It not only wraps up the essential elements discussed across the article but also provides a pathway for continuous learning and improvement. Understanding what has been covered helps to reinforce knowledge. In the ever-evolving landscape of technology, staying updated is vital, especially when growing and refining your skills in GUI development.
Recap of Key Points
Throughout the article, several significant aspects of building Graphical User Interfaces in Java have been highlighted:
- Understanding GUI Development: We explored the fundamental concepts of GUIs, their role in software applications, and why they matter.
- Java Swing and JavaFX: Both frameworks were discussed in depth, including their advantages and features.
- Setting Up and Creating Applications: Practical steps to set up the development environment and build your first GUI were outlined.
- Components and Event Handling: Insight into various components and implementing event listeners was provided, which are critical to creating interactive interfaces.
- User Experience Best Practices: Emphasis was placed on creating intuitive designs and considering accessibility.
- Testing, Deployment, and Future Trends: The importance of testing frameworks and emerging technologies in Java GUI development were also addressed.
Each of these points builds a foundation for both beginners and intermediate developers, allowing you to grasp the theories and approaches necessary for effective GUI design in Java.
Encouraging Further Exploration
As you conclude your reading, it is beneficial to consider further steps. Delving deeper into specific frameworks such as JavaFX can enhance your GUI-building capabilities. Experimentation is key. Practice developing applications, utilizing various components and layouts that were discussed in this guide.
Engaging with online communities, like Reddit or Facebook, can provide support and insights from other developers who share your interests. Resources such as programming courses or books can substantially aid in your learning. Places like Wikipedia and Britannica offer detailed explanations of concepts that may further your understanding of GUI development in Java.
Continuously expand your understanding of user experience design and accessibility considerations. Be curious about emerging tools and technologies that will shape the future of GUI development. Your journey into the world of Java GUI design doesn’t have to end here; it is a field designated for ongoing discovery and enhancement.