Mastering Graphical User Interfaces Development in C++
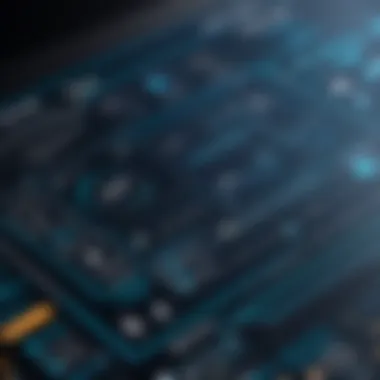
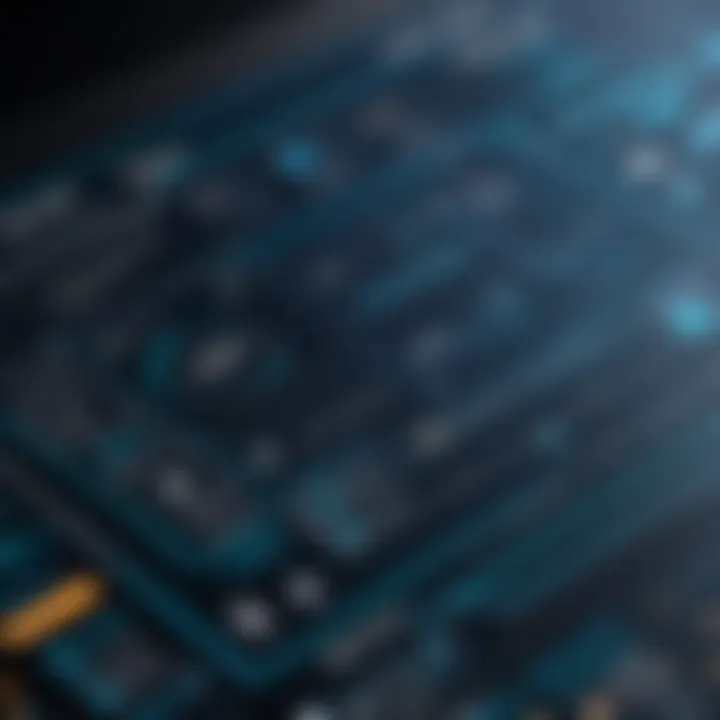
Intro
Building graphical user interfaces (GUIs) with C++ requires an understanding of both the programming language and the specific libraries suited for GUI creation.
C++ serves as a versatile tool for both system-level programming and high-level applications. It's used across diverse domains, from game development to enterprise applications. To grasp the full extent of GUI development, it is essential to understand the fundamentals of C++. This intro section will guide the way.
Preamble to Programming Language
History and Background
C++ was created by Bjarne Stroustrup in the late 1970s at Bell Labs. Initially an enhancement to the C programming language, C++ introduced features like classes and objects. This expansion allowed for complex program structures, marking a pivotal turn in computer science.
Features and Uses
Some noteworthy features of C++ include:
- Object-Oriented Programming: Promotes code reusability and organization.
- Rich Library Support: Various libraries, including C++ Standard Library and specific GUI libraries likeQt, facilitate development.
- Performance: C++ provides powerful performance due to low-level memory manipulation capabilities.
C++ finds popularity in sectors requiring high performance. Such fields include:
- Real-time systems
- Application development
- Game development
Popularity and Scope
C++ remains one of the top languages for systems and applications programming. Its ability to deliver speed and efficient memory usage keeps it relevant in an ever-evolving software field. Its applications span multiple domains, and learning C++ paves the way to grasp snippets of high-performance computing and intricate GUI development tasks.
Basic Syntax and Concepts
Effective GUI programming starts with chhoosing the right syntax. By understanding basic concepts, beginners can dive deeper into application design.
Variables and Data Types
Variables in C++ represent storage locations with distinct name and type. Main data types include:
- int: Represents integers.
- double: For floating-point numbers.
- char: Represents single characters.
- std::string: For strings.
Using the correct data type is crucial as it influences memory use and application performance.
Operators and Expressions
C++ supports various operators such as:
- Arithmetic Operators: For calculating values.
- Relational Operators: To compare values.
- Logical Operators: For combining conditions.
Creating expressions using these operators is fundamental in handling data.
Control Structures
Control structures, like loops and conditionals, direct the flow of execution in programs. Key examples include:
- if-statement: To execute control based on conditions.
- for-loop: For iterating through collections or ranges.
Advanced Topics
Once basic syntax is mastered, advanced topics become vital.
Functions and Methods
Functions help to encapsulate behavior in a C++ program. A function defines input and output, providing clear function reusability.
Object-Oriented Programming
Understanding principles like encapsulation, inheritance, and polymorphism within object-oriented programming greatly enhances GUI design. GUIs often consist of many interconnected objects, making OOP essential.
Exception Handling
In application development, managing errors is key. C++ uses try-catch blocks to handle exceptions gracefully, ensuring that the user experience remains uninterrupted.
Hands-On Examples
Practical examples solidify learning.
Simple Programs
Start with CLI applications which can smoothly transition into GUI later. For example, calculating the sum of two numbers using input/output:
Intermediate Projects
Building a simple text editor or calculator can offer practical experience. Such projects involve GUI components and introduce real programming challenges.
Code Snippets
Sharing short segments of code – like initializing a window or responding to button clicks - helps understand GUI framework structure. Leveraging the Qt library is beneficial. For example:
Resources and Further Learning
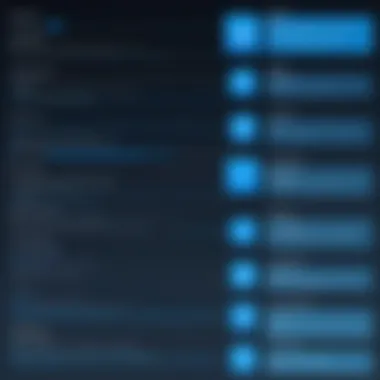
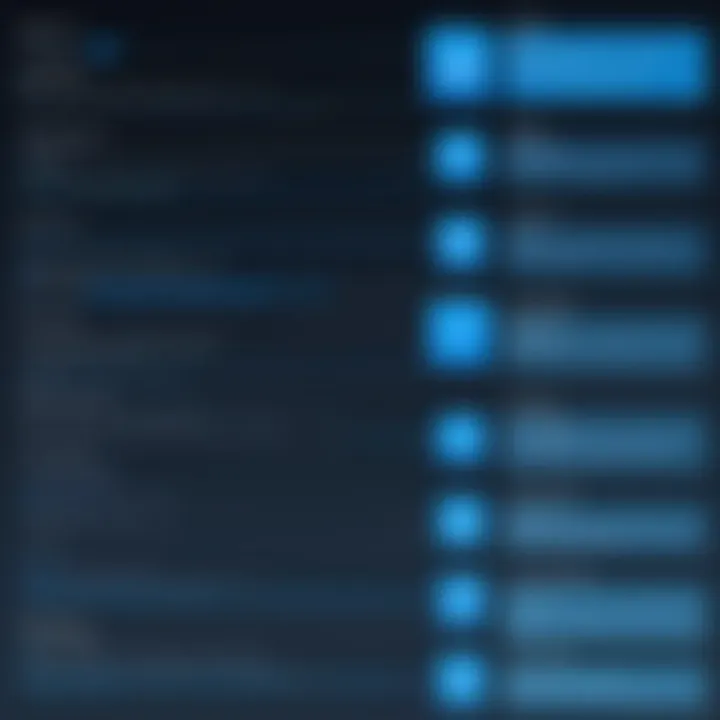
Continuous is key to mastering C++. Some helpful resources include:
- Recommended Books: *
Foreword to GUI Programming in ++
Graphical User Interfaces, or GUIs, are fundamental to modern software application usability. In an era where user experience dictates success or failure, establishing a solid grounding in GUI programming is essential for any developer, especially in C++. This discipline allows programmers to create interfaces that users interact with visually.
Understanding Graphical User Interfaces
Graphical User Interfaces serve as the bridge between users and computer systems. Unlike command-line interfaces, GUIs employ visual components like windows, menus, and buttons to communicate functionality. These elements collectively create an engaging experience for the users. The perception of how well software performs can heavily rely on the quality of its GUI.
The development of GUIs involves considering several critical components:
- Usability: A compelling GUI must be intuitive and easy to navigate.
- Aesthetics: Visual appeal matters, as a stylish application can prompt longer usage.
- Functionality: A GUI needs to effectively perform its intended tasks seamlessly.
?id## Importance of GUI in Software Development
GUIs play a crucial role in software evolution. The significance of graphical interfaces in modern applications can be broken down into several benefits:
- Enhanced User Accessibility: An intuitive GUI allows even non-technical users to interact with software efficiently.
- Learning Curve Mitigation: Users can familiarize themselves with software quicker through visual elements compared to text-based interfaces.
- Rapid Interaction: Users can perform actions quickly without memorizing commands or syntax.
- User Engagement: A well-designed GUI encourages longer interaction times and improved user satisfaction.
Quote: Modern software development almost exclusively prioritizes user experience and interface aesthetics alongside functionality.
Overview of ++ and Its Capabilities
C++ stands as a powerful and widely used programming language, particularly notable for its performance and versatility. Its core capabilities lay the foundation for creating robust applications, including graphical user interfaces (GUIs). This section highlights what makes C++ suitable for GUI development by discussing its principal features and why developers often choose it over other programming languages.
Core Features of ++
C++ incorporates several core features that contribute to its functionality and efficiency in building GUIs. These include:
- Object-Oriented Programming (OOP): C++ promotes an OOP approach, allowing developers to create classes and objects which encapsulate data and functions. This principle enhances code organization and reusability, crucial for scalable GUI applications.
- Memory Management: C++ offers fine control over memory allocation and deallocation. Through pointers and references, developers manage resources effectively, fostering better performance, essential for resource-intensive GUIs.
- Compiled Language: C++ is a compiled language, meaning the code converts directly into machine code. This results in the high execution speed of programs, making C++ ideal for applications requiring efficient performance.
- Standard Template Library (STL): The STL provides ready-to-use algorithms and data structures, simplifying development. Items like vectors and lists can be handy when managing GUI elements dynamically.
In summary, these core features empower C++ developers to create high-quality interfaces that are both responsive and scalable, establishing a strong case for using this language.
Why Use ++ for GUI Development?
Choosing C++ for GUI development is often based on several significant considerations and perks:
- Cross-Platform Compatibility: Applications built in C++ can run on various operating systems including Windows, macOS, and Linux. This versatility taps into a larger audience without requiring extensive modification of code.
- Performance Optimization: Developers enjoy the inherent speed and efficiency native to C++. It makes C++ inherently suitable for resource-heavy applications, which is often the case with graphical interfaces.
- Rich Library Support: The availability of numerous libraries like Qt and wxWidgets facilitates GUI development. These libraries provide tools and functions that help speed up development processes.
- Advanced Functionality: C++ allows implementing advanced features like custom widgets or multimedia handling, which could elevate the user experience in GUI applications. The capability to harness complex features effectively sets C++ apart from lower-level languages.
- Community and Resources: Ongoing contribution through platforms like reddit.com and documentation found on sites like en.wikipedia.org fosters a supportive ecosystem. This facilitates problem-solving and brings a wealth of shared knowledge.
These factors underscore the suitability of C++ in a landscape laden with competition among programming languages.
C++ is not just a language; it's an ecosystem that promotes robust application development, including GUIs, making it a favored choice among developers.
Popular Libraries for GUI Development in ++
Choosing the right library is crucial for successful GUI development in C++. A library provides essential functionalities that help accelerate the development process while enabling the software to run efficiently. The significance of selecting the right library lies not only in its feature set but also in factors like ease of use, community support, and compatibility with different platforms.
When considering libraries for GUI development, it is important to note some key elements:
- Development Speed: Libraries offer pre-built components, which can reduce development time.
- Platform Support: Not all libraries are cross-platform. The right choice depends on your project’s requirements.
- Customizability: Some libraries allow extensive tweaks to the user interface, providing more freedom in design.
- Community: A strong community can offer support and updates, making development smoother.
Qt Framework
The Qt Framework is one of the most popular C++ libraries for GUI development. It is renowned for its powerful features and capabilities. Qt provides a rich set of tools, including widgets, layout managers, and features like signals and slots that simplify event-driven programming.
The advantages of Qt include:
- Cross-Platform Support: Qt enables developers to create applications that run on multiple platforms with little to no alteration in the codebase.
- Comprehensive Documentation: The extensive documentation aids both beginners and experienced programmers in understanding how to utilize its components efficiently.
- Strong Community and Commercial Support: Users benefit from forums and resources, ensuring help is available when needed.
However, there are considerations; its licensing can be costly for commercial projects. For educational and personal projects, it often feels more inviting.
wxWidgets Library
wxWidgets is another versatile library for GUI programming in C++. Known for its native look and feel, wxWidgets provides developers with tools to create applications that integrate seamlessly with various platforms. This library empowers cross-platform app development by retaining the native aesthetics of the operating system.
The library has several strengths, such as:
- Native Performance: wxWidgets uses the native API of each platform. Thus, users experience less overhead.
- Abundant Widgets: The extensive collection of pre-built widgets simplifies the task of design and layout creation.
- Flexible Licensing: wxWidgets is open-source and offers more favorable licensing for commercial apps compared to other libraries like Qt.
Yet, some may find wxWidgets less modern in its programming style, which can be a hurdle for new developers.
GTK+ for ++
GTK+ stands out for applications that aim for a Linux-centric experience. Though originally developed for the GNOME desktop environment, GTK+ can be used across various operating systems. Its primary strength lies in its adaptability for graphical applications.
Key aspects of GTK+ include:
- Rich Widget Set: Developers can use numerous widgets to assemble their interfaces effortlessly.
- Excellent for Themed Applications: GTK+ is particularly advantageous for projects that emphasize graphical styles, such as those targeting the GNOME platform.
- Active Community: Continuous contributions from its community lead to regular enhancements.
Developers should consider GTK+ if they mainly target Linux but may encounter challenges on Windows. Whichever library is chosen, understanding its unique strengths and limitations can determine the success or failure of the GUI project.
Setting Up the Development Environment
Setting up the development environment is a foundational step in creating graphical user interfaces (GUIs) in C++. The environment you select can have a significant impact on your productivity and effectiveness as a developer. It is crucial first to highlight the main components that comprise this environment - a compiler, an IDE (Integrated Development Environment), and various GUI libraries or frameworks. Each part plays a distinctive role, and understanding these elements allows for smoother development.
Choosing the Right Tools
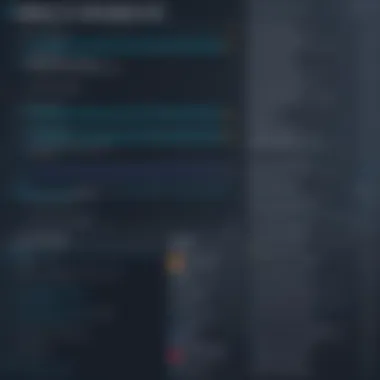
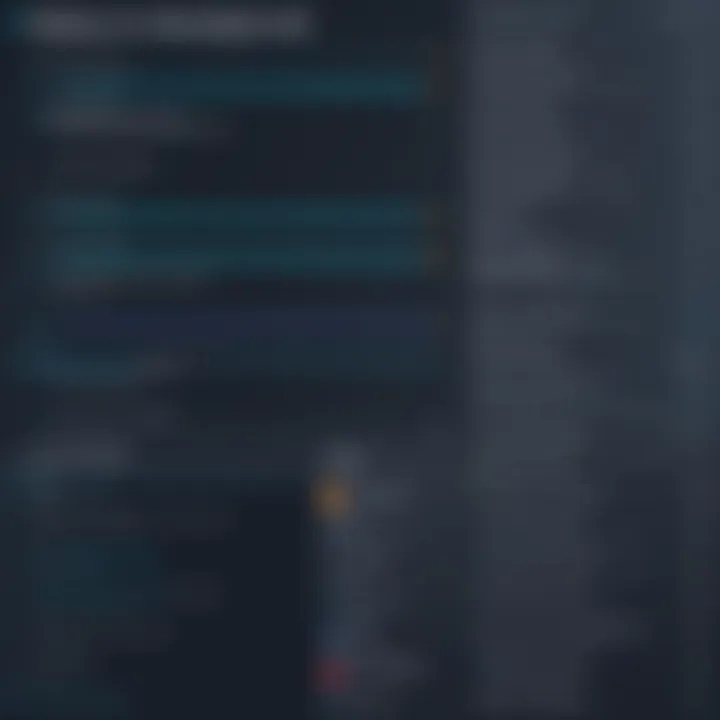
Selecting the right tools is essential. The right tools can simplify the development process and offer numerous functionalities tailored for GUI development. You usually start with a compiler tailored for C++, such as GCC or Clang. They are reliable and provide good support for C++ standards. An IDE such as Visual Studio, Qt Creator, or Code::Blocks can enhance your code management and debugging capabilities significantly.
- Visual Studio is popular for its advanced debugging tools and community support.
- Qt Creator is well integrated with the Qt framework; therefore, it is an excellent choice for Qt-based development.
- Code::Blocks appeals for its lightweight structure and customizabilty, features preferred by some developers.
Making this decision affects your coding efficiency. An intuitive IDE allows you to focus on writing rather than configuring environments.
Installing GUI Libraries
After selecting tools, installing GUI libraries is the next step. These libraries provide pre-coded elements you can use to build your user interfaces, saving time and effort. Two of the most used GUI libraries in C++ are Qt and wxWidgets.
The installation process can vary depending on the choices made earlier. Usually, you begin by downloading the libraries from the official websites. Many IDEs have integrated functionality to manage library installations.
Simple steps for installation include:
- Download the installation package from the respective library's site.
- Follow the instructions provided for your operating system. For instance, on Windows, you may use MSVC for easy integration with Qt.
- Post installation, configure your IDE to recognize the library paths, ensuring your IDE can find these resources.
This setup phase determines how efficiently you can utilize these libraries within your applications. A well-configured development environment can make it easier to manage projects and implement new functionalities. Remember that keeping libraries updated reduces risks of bugs and avails new features, facilitating a better development experience.
To build a successful GUI application, laying a solid foundation is key—choosing the right tools and libraries ensures every project starts on the right path.
Basic Concepts of GUI Development
Understanding the basic concepts in GUI development is essential for anyone aspiring to create effective graphical interfaces using C++. These concepts form the foundation for building user-friendly applications. Grasping these elements allows developers to enhance user interaction within their software. There are several key areas that encompass GUI development, such as event-driven programming and the utilization of widgets and layouts. Each area contributes to how end-users experience an application, directly impacting usability and satisfaction.
Event-Driven Programming
Event-driven programming deviates from traditional sequential programming models, allowing applications to respond to user actions dynamically. In GUI applications, user actions such as clicks, keyboard inputs, and mouse movements are considered events. The core principle revolves around the event loop, which listens for these events before dispatching them to the appropriate handlers.
This method promotes more interactive user interfaces. It gives the application the ability to remain responsive even while processing tasks in the background. Here are significant points regarding event-driven programming in C++:
- Responsiveness: This model keeps the application lively. Users can interact while the program undertakes various functions.
- Modularity: Event handlers can be independently designed. This leads to greater maintainability and reduced complexity in the codebase.
- Asynchronous operations: Handling multiple events at once can effectively manage concurrent processes—an essential quality in modern applications.
An example in C++ might look like this:
This code emphasizes how functions are triggered in response to events, showcasing the core of an event-driven model. By mastering event-driven programming, developers gain a robust toolset to create interfaces that accommodate varied user actions effectively.
Widgets and Layouts
Widgets, the building blocks of GUIs, are essential components that convey functionality within an interface. Common widgets in C++ GUI libraries include buttons, text boxes, labels, and sliders. Each widget serves a definite purpose and significantly enhances user interaction. Here are details concerning their roles:
- Buttons: Initiate actions upon being clicked.
- Text boxes: Enable users to input data conveniently.
- Labels: Provide information or guidance relevant to other widgets.
- Sliders: Allow users to choose from a range of values visually.
Layouts, on the other hand, dictate the arrangement of these widgets in a user interface. A well-planned layout is crucial for good usability. Layouts help in organizing the workspace more intuitively. They need to be both aesthetically pleasing and functional.
Often, developers must decide between different layout patterns, such as grid layouts, box layouts, or flow layouts. The choice impacts how visually accessible and user-centric the application becomes. For creating optimal user experiences, attention must be given to how widgets are grouped and how users flow from one part of the interface to another.
To sum up, both event-driven programming and the proper arrangement of widgets and layouts lead to better-crafted applications. These fundamental concepts should be prioritized when developing graphical user interfaces, as mastery of these elements can drive an innovative and user-centric design approach.
Creating a Simple GUI Application
Creating a simple GUI application serves as a foundational step for anyone interested in engaging designs in software. This initial crafting emphasizes essential principles and allows developers to understand how various components function together to create a cohesive user experience. Simplicity in design and functionality leads to better engagement from users and streamlines interactions.
Moreover, building a rudimentary interface offers significant benefits:
- Enhanced Understanding: Focusing on basic components aids in grasping core concepts vital in more complex applications.
- Platform Familiarity: Developers become acquainted with tools and frameworks that may encapsulate tasks in bigger projeccts.
- Foundation for Growth: Once the simple designs are mastered, expansion into feature-rich GUI is nearly a natural progression.
In summary, launching into simple applications aligns both learning objectives with practical applications. It encourages systematic exploration and innovation across the evolving field of GUI development in C++.
Designing the Interface
An intuitive interface emerges from careful planning and execution. The design phase requires foresights about indicators, buttons, and fields users will interact with. The principles here involve symmetry, visual hierarchy, and productive space utilization to make the application user-friendly.
Key considerations for designing an interface include:
- Cohesion in Visualization: All elements should make sense together visually, followed branding principles where applicable.
- User-Centric Approach: Consider user expectations and behaviors. Engagement flows easier when users feel their workflow was observed when designing steps.
- Accessibility Elements: Cater to those with disabilities, ensuring navigation is simple. Think about color choices, font sizes, and additional needs the users may have.
With each aspect designed positively influences user navigation, handshaking learning curves to engagement points, stimulating overall interaction and functionality.
Implementing Functionality
Once the interface design aligns effectively, turn to functional aspects. This section tidies all proposed features ultimately striking a balance between logic and imteractivity. Implementing functionality focuses on making sure that controls perform as intended and enhance the outcome for users actively engaging with the application.
Essential activities in functionality implementation include:
- Event Handling: Capture user inputs and translate them into helpful actions, such as responding to clicking buttons or typing in text boxes.
- Maintaining State: Effectively manage the interface state particularly where user choices directly influence outcomes or visible alterations in the interface.
- Scoped Interaction Logic: Include breadth towards how features influences overall performance within visual framework designated.
Functional integrity aligns crucial path where everything works, thus achieved user-satisfactory design which leads to an ultimately valuable application entrenched within learning points.
Effective implementation of the GUI and managing functionality enhance user experience, leading to satisfaction and engagement.
Advanced GUI Features
The development of graphical user interfaces (GUIs) involves more than just creating aesthetically pleasing layouts. Advanced GUI features play a crucial role in enhancing both functionality and user experience. These features are not just optional enhancements; they can significantly impact how the application operates and how users interact with it. As you delve deeper into the world of C++ GUI programming, understanding these advanced options should be a priority.
Custom Widgets
Custom widgets are a vital aspect of advanced GUI programming. While libraries like Qt and wxWidgets provide a variety of pre-built widgets—such as buttons, text fields, and sliders—there are often unique needs that standard widgets cannot address. This is where custom widgets come into play. Creating your own widgets gives developers the ability to design elements that can match a specific style or functionality required by the application.
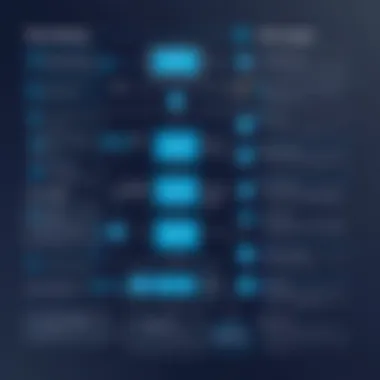
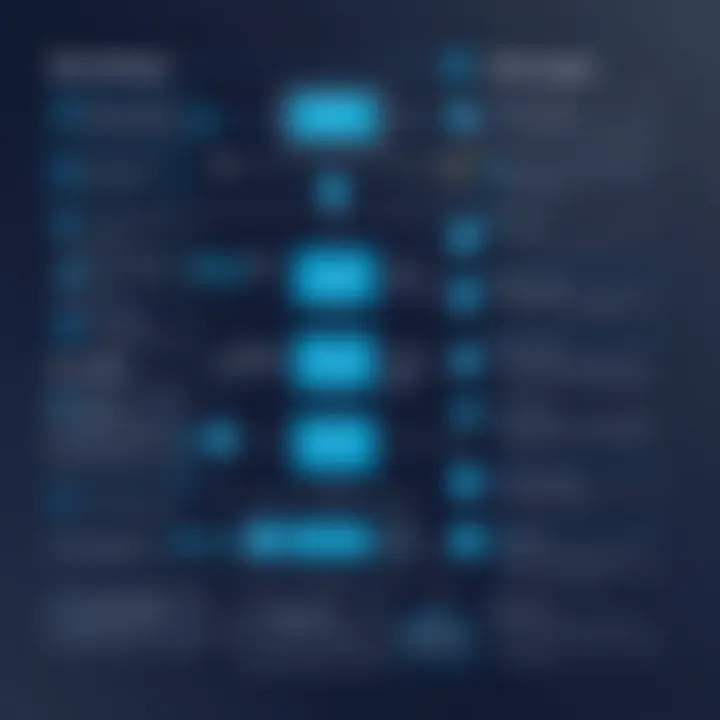
The benefits of using custom widgets include:
- Tailored Functionality: Custom widgets enable precise control over the behavior and appearance of graphical objects in your application.
- Enhanced User Interaction: By crafting non-standard widgets, you can improve the user experience and offer interaction that is unnatural with traditional widgets.
- Reusability: Once a custom widget is created, it can easily be utilized in other parts of the application or in future projects, maintaining consistency throughout.
However, creating efficient and effective custom widgets can be challenging. It involves knowledge of the rendering process and event handling. With C++, you have the power to delve deep into both. Careful planning and testing ensure that these custom elements don’t negatively affect performance.
Multimedia and Graphics Integration
In today’s competitive landscape, enticing users often requires the integration of multimedia and graphics. This feature transforms a simple application into an engaging product that can capture the audience’s attention. The role of multimedia extends beyond mere enhancement; it is a tool for increasing comprehension and retaining user interest.
C++ excels at implementing multimedia features through libraries and frameworks such as SDL or SFML, which facilitate sound and image management.
Key considerations for multimedia integration include:
- Performance Optimization: While multimedia can add richness, it can also incur performance costs. Ensure that graphics and sounds are optimized for smooth operation without delays.
- Support for Various Formats: Plan to support a wide range of file formats for both audio and images to offer flexibility. Libraries often abstract most complexities, allowing you to focus on functionality.
- User Settings: Give users the ability to adjust settings related to multimedia. This includes volume control, video quality, and aspect ratio adjustments to cater to varied preferences.
Incorporating multimedia elements can dramatically elevate the user interface. Regardless of whether it is implementing simple sound notifications or embedding complex animations, thoughtful integration is paramount. Developers must balance functionality, esthetics, and user preferences to create meaningful GUI applications while understanding tools and resources at their disposal.
"The quality of user experience can be vastly improved through thoughtful integration of multimedia features." - User Experience Designer
Testing and Debugging GUI Applications
In the realm of GUI development, testing and debugging are critical phases that ensure the functionality and usability of the application. With the complex interactions inherent in graphical user interfaces, unforeseen behaviors may arise when users engage with various components. Therefore, paying close attention to testing is not merely a consideration, it is an essential practice in the development lifecycle. Failing to account for this can lead to increased support costs and diminished user satisfaction.
Best Practices for Testing
When developing a GUI application in C++, adhere to these best practices to enhance the effectiveness of your testing process:
- Use Automated Testing Tools: Incorporate frameworks such as Qt Test or Google Test within your workflow. These tools allow for efficient execution of tests, which can also be run alongside continuous integration processes.
- Conduct User Acceptance Testing: Engage real users to validate whether the GUI meets their requirements. This real-world feedback can reveal aspects of usability that automated tests might miss.
- Test on Multiple Platforms and Devices: If your application is cross-platform, ensure testing occurs on every targeted operating system. Differences in performance can slope user expectations considerably, affecting acceptance rates.
- Coverage Analysis: Use test coverage tools to identify untested code paths. This can help prioritize your testing processes on critical areas that have not yet been evaluated.
- Iterative Test Cycles: Regularly revisit tests throughout different stages of development. Frequent iterations can accommodate new features, thus ensuring stability as the code evolves.
“Quality is never an accident; it is always the result of intelligent effort.” - John Ruskin
Common Issues and Solutions
GUI applications often encounter particular issues that can be meticulously resolved through established solutions. Some common issues include:
- UI Freezing: This may occur if long operations are performed on the main UI thread. To tackle this, offload heavy tasks onto worker threads.
- Mismatched Event Handlers: As elements are added or modified, event handlers may not associate correctly. Be diligent in maintaining compatibility after updates.
- Memory Leaks: Monitoring tools can help identify locations in the code where objects are not being properly disposed of, potentially leading to application crashes over time. Apply smart pointers in C++ coding to manage resource allocation effectively.
- Responsiveness: Use timers judiciously to prevent lagging interfaces and to maintain a smooth user experience. Testing performance under various conditions will also shed light on any bottlenecks.
The adept application of these practices and solutions cultivates robust and resilient GUI applications that perform optimally for end-users, enriching their interaction with the application itself.
User Experience and Usability Considerations
Building graphical user interfaces is more than just coding elements and functions; it is about creating an engaging and functional experience for users. User Experience (UX) and usability are critical components in GUI development that can determine how effectively software meets the needs of its users. When done right, good UX enhances user satisfaction and can become a competitive advantage.
The concept of usability refers to how easy and efficient a program is for the user. It has several dimensions that include:
- Learnability: How easy it is for users to perform basic tasks on the interface after minimal instruction?
- Efficiency: How quickly can users perform those tasks?
- Memorability: When returning after a period of not using the interface, how easily can users re-establish proficiency?
- Error Management: How many errors do users make, how severe are they, and how easily can these errors be recovered?
- Satisfaction: How pleasant is it to use?
These elements are essential as they directly influence user engagement and overall satisfaction with the product. If a GUI is confusing or poorly designed, users may abandon it in favor of alternatives, no matter the quality of its underlying functionality. Therefore, thoughtful design that considers these usability elements from the outset can greatly improve the software’s performance and users' willingness to engage with it.
Principles of Effective GUI Design
Effective GUI design rests upon several key principles that foster usability:
- Consistency: Keeping visual and functional aspects uniform across the application aids users. It can involve using similar shapes, colors, and terminology for the same actions or elements.
- Feedback: Users should receive input or notifications regarding their actions. For instance, a loading icon informs users that something is occurring in the background. This way, users do not remain uncertain about whether the application is still active.
- Simplicity: Interfaces should avoid unnecessary complexity. Users tend to favor clear pathways to tasks over convoluted menus and options. Clear design layouts improve learnability and satisfaction.
- Visual Hierarchy: Elements should be organized to indicate order and importance, guiding users toward key features without overwhelming them with information.
- Accessible Design: This will be linked closely to accessibility, focusing users reaching an optimal experience, ensuring that designed interfaces address the needs of users irrespective of their ability level.
By applying these principles, developers create intuitive GUIs that feel comfortable for users while fulfilling their requirements.
Accessibility in GUI Development
A fundamental responsibility of any developer is to ensure accessibility. This concept centers on creating software that can be accessed by people with a wide range of disabilities, whether they pertain to visual, auditory, motor or cognitive challenges. Types of considerations include:
- Color Contrast: Ensuring there are sufficient contrasts between foreground and background elements helps users with visual impairments.
- Keyboard Navigation: Providing an interface that can be fully navigated using keyboard shortcuts ensures inclusivity for those who cannot use a mouse.
- Screen Reader Compatibility: Proper labeling of UI elements is essential for users utilizing screen readers, which verbally convey textural information.
- Flexible Layouts: Allowing users to resize or adapt interfaces means that individuals can manipulate how they view information to suit their needs better.
Essentially, combining user experience and accessibility in GUI design Union can distinctly improve not only how pleasant software feels to interact with, but also who can effectively utilize it.
Note: Regularly updating interfaces based on user feedback can bring engaging creativity while inclusive well. Utilitizing these insights ensures that popularity straightens with user dependency instead of making دس滓.
Taking full consideration of user experience and usability during GUI development will enable seamless interaction, flipping inquiry. Developers investing time in these points will ultimately see higher satisfaction, better security in usability, and perhaps extended use of the software product.
Future Trends in GUI Development with ++
Future trends in GUIs are crucial for any developer who wishes to remain competitive. With rapid advancements in technology, GUI development in C++ must adapt to meet user expectations and functionalities. One cannot dismiss the significance of this field since user interaction dictates software success. Linear legacy designs will likely not suffice in an аver changing digital landscape.
Cross-Platform Development
Cross-platform development is a pivotal trend shaping the future of GUI creation. C++ offers robust solutions for building applications that function on multiple operating systems. Developers can utilize frameworks like Qt or wxWidgets to achieve this flexibility.
Advantages include:
- Wider Reach: Create applications accessible to a broader audience.
- Cost-Effectiveness: Developing once and deploying across platforms reduces resource expenditure.
- Consistent User Experience: If done correctly, users experience similar interactions irrespective of their operating system.
Furthermore, cross-platform development makes maintenance simpler. As platforms and tools evolve, developers need to update only one codebase to enhance user interface across all systems. This efficiency appeals not only to large tech firms, but also to independent developers and startups.
Integration with Emerging Technologies
Emerging technologies play a vital role in the evolution of GUI development. Aspects like virtual reality (VR), augmented reality (AR), and Artificial Intelligence (AI) are shaping how users interact with applications.
Consider some of the benefits of integrating these technologies:
- Enhanced User Engagement: Incorporating VR or AR can transform standard GUIs into more immersive experiences.
- Automation Via AI: Smart functionalities can analyze user behavior and dynamically adjust the interface to suit preferences.
- New Interaction Methods: Voice command or gesture control streamline user tasks and improve accessibility.
C++ provides the means to leverage these technologies. Modern libraries and tools that support VR and AI can be harnessed using C++. This ensures that developers remain at the forefront of innovation as they build influential applications.
Innovation in GUI development depends on embracing new technologies. Those who adapt will undoubtedly have an edge in the competitive landscape.