Building a Dynamic Website with Java: A Complete Guide
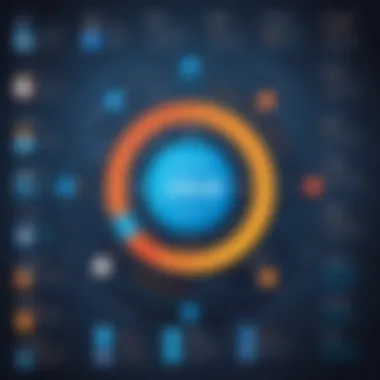
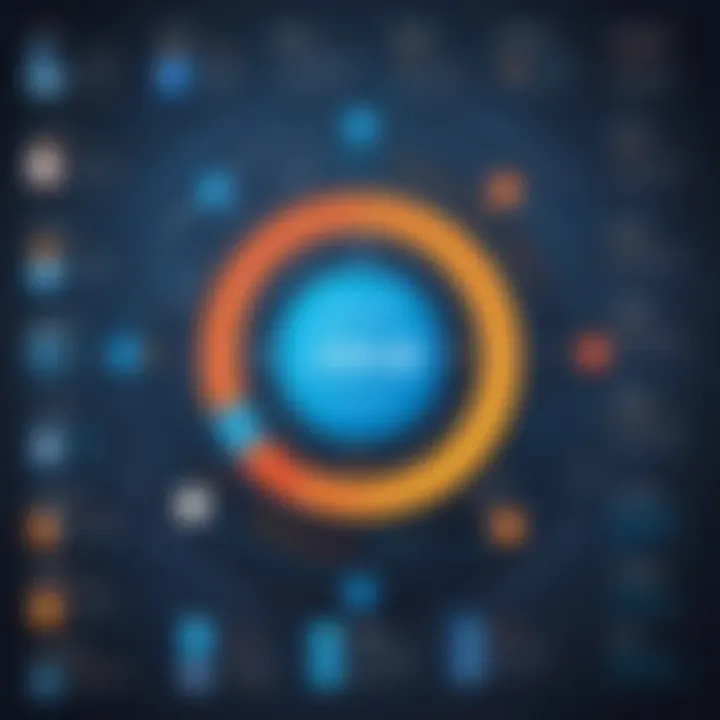
Intro
Creating a website is a fundamental skill in today's digital world. Among the array of programming languages, Java stands out for its robust performance, scalability, and versatility. This guide aims to explore the complete process of developing a website using Java, a language that has cemented itself in the landscape of web development.
Preamble to Programming Language
History and Background
Java was created by James Gosling and his team at Sun Microsystems in 1995. It was designed to be a platform-independent language, allowing developers to write code once and run it anywhere, thanks to the Java Virtual Machine (JVM). Over the years, it has evolved significantly, adapting to new technologies and trends in software development.
Features and Uses
Java is known for its object-oriented programming capabilities, strong memory management, and built-in security features. It is commonly used in web applications, Android applications, and enterprise-level solutions. The language's syntax is similar to C++, making it accessible to many programmers. The usage of Java extends into various domains, such as banking systems, online retail, and scientific applications.
Popularity and Scope
Given its extensive capabilities, Java continues to be highly popular among developers. According to the TIOBE index, Java has consistently ranked as one of the top programming languages globally. Its community has matured, leading to the development of numerous frameworks and tools that simplify web development tasks.
Basic Syntax and Concepts
Variables and Data Types
Variables in Java are essential as they hold data that a program can manipulate. Java supports several data types such as integers, floats, doubles, and strings. For example:
Operators and Expressions
Java provides various operators like arithmetic, relational, and logical operators. These tools help developers write expressions that can compute values or compare them. For example, the expression evaluates to .
Control Structures
Java supports multiple control flow structures, like if-else statements and loops. These are crucial for guiding the execution of code based on specific conditions. For instance, a simple loop can run a specific block of code multiple times:
Advanced Topics
Functions and Methods
In Java, functions are defined as methods associated with classes. They help break down complex tasks into manageable pieces. For example:
Object-Oriented Programming
Java is an object-oriented language, which means it relies heavily on the concept of objects and classes. This approach promotes reusability and organization within code. Key principles include:
- Encapsulation: Hiding the internal state of an object.
- Inheritance: Creating new classes based on existing ones.
- Polymorphism: Allowing objects to be processed in multiple forms.
Exception Handling
Java has a powerful exception handling framework that allows developers to manage runtime errors effectively. By using blocks, one can handle potential problems gracefully. For example:
Hands-On Examples
Simple Programs
Starting with simple Java applications is crucial. A basic "Hello World" program demonstrates compiling and running Java code:
Intermediate Projects
Building a small web application using Java Servlets and JSP is a great way to practice. For example, creating a simple online form that captures user input showcases the integration of Java to handle web requests.
Code Snippets
Code snippets are valuable for quick implementations. The following Java snippet illustrates how to connect to a database:
Resources and Further Learning
Recommended Books and Tutorials
- Effective Java by Joshua Bloch
- Java: A Beginner's Guide by Herbert Schildt
Online Courses and Platforms
- Coursera
- Udacity
- Codecademy
Community Forums and Groups
- Reddit's /r/java
- Stack Overflow
- Facebook Programming Groups
Remember, practice is key when learning Java. Hands-on experience solidifies concepts and prepares you for real-world applications.
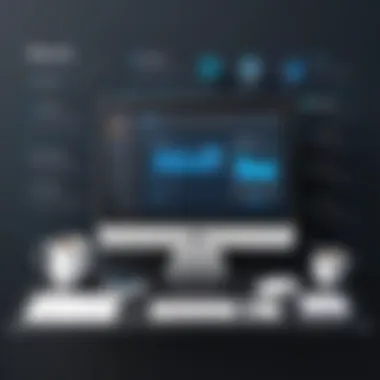
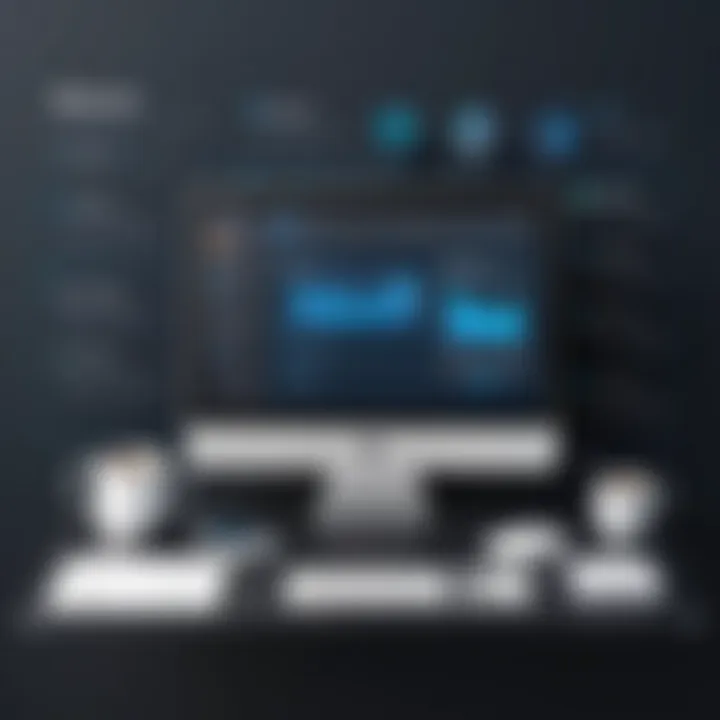
As you proceed with this guide, you will develop a solid understanding of how to use Java for web development. With every line of code, you bring your ideas to life.
Prelude to Website Development with Java
In the current digital landscape, building a website is a fundamental skill. Java plays a crucial role in web development, offering robust capabilities that suit dynamic and complex applications. This article will guide readers through various aspects of web development using Java, highlighting its significance in creating functional websites.
The integration of Java into web development provides several benefits. It is a highly portable language, which means developers can write code that runs on different platforms without modification. This is particularly valuable as it ensures consistency across multiple environments. Furthermore, Java is known for its strong security features. Websites built with Java can efficiently manage user data, making it a preferred choice for applications requiring secure transactions.
Considerations in using Java involve understanding its architecture. The Java platform operates on a multi-tier architecture, which creates a separation of concerns. This allows developers to manage the presentation layer, application logic, and database management distinctly, which streamlines the development process. Additionally, the extensive ecosystem surrounding Java, including frameworks and libraries, facilitates rapid application development. This article will explore these aspects in detail, equipping aspiring developers with essential knowledge and skills to leverage Java in building efficient web applications.
"Java continues to be a popular choice for web development due to its scalability and maintainability."
Understanding Java in Web Development
Java is a versatile programming language that has been used for various applications since its release. In web development, it serves as a server-side language that enhances user experience. It allows dynamic page generation, enabling developers to create interactive, data-driven websites.
Java's ecosystem includes various technologies, such as Servlets, JavaServer Pages (JSP), and JavaServer Faces (JSF). These tools support the development of scalable web applications. By utilizing these frameworks, developers can build web applications that are modular and easier to maintain. Consequently, the understanding of Java in this context is indispensable for modern developers.
History and Evolution of Java
Java was created in the mid-1990s by Sun Microsystems. Initially, it aimed to run on any platform without modification. The release of Java 2 introduced significant updates that enhanced its use for web development, including the introduction of Servlets and JSP.
Over the years, Java has evolved through numerous updates, introducing features like Java Enterprise Edition (Java EE), which supports building large-scale applications. The evolution has also seen a shift toward frameworks that simplify development tasks. Frameworks such as Spring have become essential for building enterprise-level applications, expanding Java's application beyond simple web pages to full-fledged web services.
The ongoing evolution of Java is marked by continuous improvements to performance and security, making it a robust candidate for modern web development. Understanding the history of Java gives developers insight into its capabilities and underlying principles, which enhances their ability to apply the language effectively in real-world situations.
Core Java Concepts for Web Development
Understanding core Java concepts is vital for creating efficient web applications. These concepts serve as the foundation for many advanced discussions on Java's application in web development. When embarking on building a website, a solid grasp of Java syntax, object-oriented principles, and error handling mechanisms will greatly enhance your development workflow.
Java is a versatile programming language, and having a strong comprehension of its fundamental aspects will allow developers to write cleaner, more maintainable code. Consequently, mastering these concepts leads to better application performance and user satisfaction. Let's delve into the essential components necessary for web development using Java.
Java Syntax and Structure
Java syntax is the set of rules that defines the combinations of symbols that are considered to be correctly structured Java programs. Grasping the syntax is crucial, as it serves as the building block for writing any Java application.
A few important aspects of Java syntax include:
- Case Sensitivity: Java is case sensitive. This means that variable names like and would be treated as distinct entities.
- Semicolons: Every statement in Java must end with a semicolon. Missing this can lead to compilation errors.
- Braces: Blocks of code are defined by opening and closing braces, denoting scopes of classes, methods, and control structures.
Hereβs a basic structure of a Java program:
This simple program demonstrates how to define a class and the main method, which serves as the entry point of any Java application. Understanding how to write and structure such code is the first step in effective web development.
Object-Oriented Programming in Java
Object-oriented programming (OOP) is a programming paradigm based on the concept of "objects", which can contain data, in the form of fields, and code, in the form of procedures. Java is inherently an object-oriented language, which means that it allows developers to create modular, reusable code.
Key principles of OOP include:
- Encapsulation: This is the bundling of data and methods that operate on that data within one unit, typically a class.
- Inheritance: This allows a new class to inherit characteristics from an existing class, promoting code reusability.
- Polymorphism: This principle enables objects to be treated as instances of their parent class, allowing for more flexible code.
By leveraging these OOP principles, developers can construct web applications that are easier to understand and modify. For instance, using encapsulation, developers can restrict access to certain components of their classes, maintaining control over data manipulation.
Exception Handling in Web Applications
No software is free from errors. Hence, understanding exception handling is crucial to enhance the robustness of web applications. In Java, exceptions are events that disrupt the normal flow of execution, and how they are handled can significantly affect the application's performance.
Java uses a structured format for handling exceptions with keywords such as , , and . Hereβs a sample code snippet illustrating exception handling:
In this example, an attempt to divide by zero generates an , which is caught by the block. This prevents the program from crashing unexpectedly. Utilizing exception handling ensures smoother experiences for users and reduces the occurrence of app failures.
Mastering core Java concepts is essential for building resilient and high-performing web applications.
Essential Tools and Frameworks
When embarking on the journey of web development with Java, selecting the right tools and frameworks is crucial. These essential elements can significantly influence the quality and efficiency of the development process. They facilitate smoother coding, offer powerful features, and ensure that projects are manageable and scalable. By understanding the available tools, developers can streamline their workflows and increase productivity. It is important to make informed choices that resonate with the specific needs of a project.
Setting Up Your Development Environment
A well-configured development environment lays the foundation for successful web applications. The first step is to install the Java Development Kit (JDK), which provides the necessary libraries and tools for Java programming. Additionally, utilizing integrated development environments, or IDEs, can enhance productivity. IDEs offer features like code completion, debugging tools, and project management capabilities which simplify the development process.
Integrated Development Environments (IDEs)
IDEs play a critical role in simplifying the workflow for developers. Tools such as Eclipse and IntelliJ IDEA provide robust environments that cater to Java developers' needs. These IDEs support user features such as real-time syntax checking and built-in debuggers, making it easier to identify and fix issues. The ability to manage projects and dependencies efficiently is one of the main advantages of using an IDE. They help in organizing files and resources, which can be especially beneficial for large applications.
Popular Java Frameworks for Web Development
Frameworks significantly enhance Java's ability to serve web applications. They provide commonly used tools, libraries, and functions, allowing developers to focus on specific features without reinventing the wheel. Here are some of the most popular Java frameworks:
Spring Framework
The Spring Framework stands out for its comprehensive programming and configuring model. It is highly versatile, suitable for building both complex enterprise applications and simple web services. Its key characteristic is dependency injection, which promotes loose coupling and facilitates testing. This aspect makes Spring a beneficial choice as it allows developers to manage bean lifecycles effectively. Additionally, its rich ecosystem includes Spring Boot, which simplifies the process of setting up new applications. However, its steep learning curve might be challenging for beginners.
JavaServer Faces (JSF)
JavaServer Faces (JSF) is another important framework that simplifies the development of user interfaces for Java web applications. Its primary feature is its component-based architecture, which allows developers to create reusable UI components. This is particularly beneficial as it speeds up the development process and enhances maintainability. JSF is supported by major application servers, providing integration with various tools. One downside is that it may not perform as efficiently as other frameworks in certain scenarios, especially when added complexity from multiple components arises.
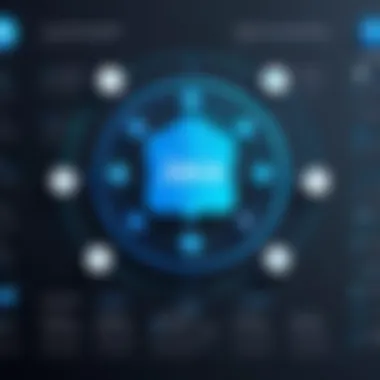
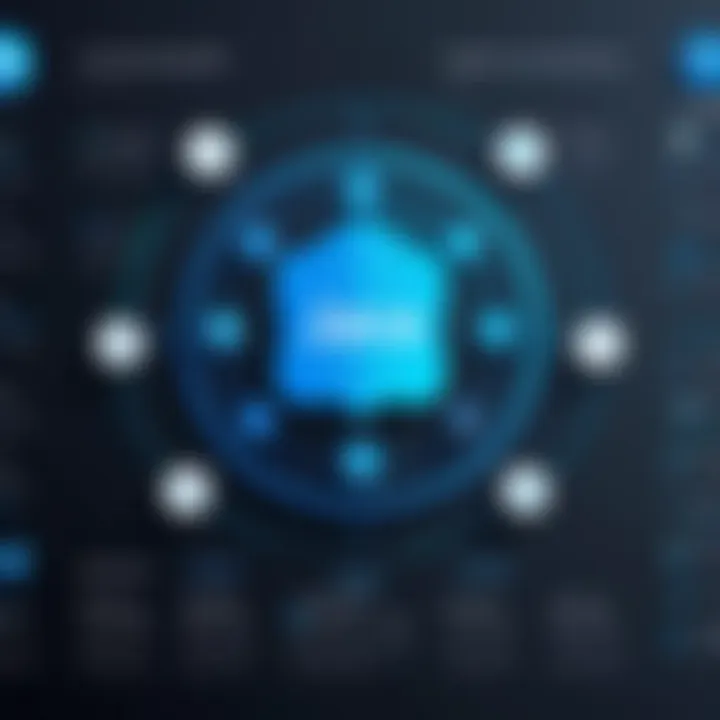
JavaServer Pages (JSP)
JavaServer Pages (JSP) serve as a technology to create dynamic web content. They allow developers to embed Java code into HTML pages, enabling the generation of dynamic content. One key characteristic is its ability to separate presentation from business logic, as JSP can utilize servlets for processing. This separation aids in enhancing code maintainability. However, JSP is often criticized for mixed responsibilities, which can lead to unmanageable code in larger applications.
"Choosing the right tools and frameworks can dramatically impact the efficiency and quality of web applications."
Developing the Backend
In the world of web development, the backend acts as the engine of a website. It is the part of the website that users do not see but is crucial for its functionality. Developing the backend involves creating the server, database, and application logic that process user requests and deliver the appropriate response. For a Java-based website, mastering backend development is essential. It ensures that the application can handle data securely, perform server-side logic efficiently, and remain scalable as traffic grows.
The benefits of a well-developed backend include improved performance, security, and user experience. Performance increases when code is optimized for speed, while security ensures that user data is protected from vulnerabilities. Moreover, a robust backend can support complex functionalities, allowing for features like user authentication, data storage, and real-time updates. Considering these elements while developing the backend will greatly impact the overall success of the web application.
Creating a Simple Java Servlet
A Java Servlet is a powerful tool for processing web requests and generating dynamic content for users. Servlets run on a server and interact with web clients. To create a simple Java Servlet, several key steps need to be followed:
- Set Up the Environment: First, ensure that the necessary environment is configured. You need an application server such as Apache Tomcat, and the Java Development Kit (JDK) installed.
- Create the Servlet Class: The servlet class must extend . It overrides methods like and to handle requests.
- Register the Servlet: You will need to register the servlet in the deployment descriptor or use annotations to inform the server about the servlet.
Here is a basic code example for creating a servlet:
This simple servlet responds to GET requests with a greeting. This shows the basic structure of a servlet and its role in generating dynamic responses.
Database Connectivity with JDBC
Databases are crucial for storing and managing data in web applications. Java Database Connectivity (JDBC) provides a standard API for connecting and executing queries with databases from Java applications. Using JDBC, developers can interact with databases such as MySQL, PostgreSQL, and Oracle. Its importance lies in enabling applications to handle data persistently, which is essential for any dynamic application.
When using JDBC, the following steps are crucial:
- Load the Database Driver: This allows the application to communicate with the specified database.
- Establish a Connection: Use a connection string to set up a connection to the database.
- Create a Statement Object: This object will be used to execute SQL queries.
- Execute Queries: Run insert, update, or select commands and process the results accordingly.
For example:
This code snippet establishes a connection to a MySQL database. Connecting your Java application with a database is fundamental to make data-driven applications.
Implementing RESTful Services
Representational State Transfer (REST) is an architectural style used in designing networked applications. It implies a set of constraints such as statelessness, cacheability, and a uniform interface. In Java, implementing RESTful services involves using frameworks such as Spring MVC, which simplifies service creation by providing built-in methods for handling HTTP requests.
To implement RESTful services, follow these steps:
- Define the API Endpoints: Specify the URIs that clients will use to access the services.
- Handle HTTP Methods: Create methods in your Java classes to map to specific HTTP methods like GET, POST, PUT, and DELETE.
- Return Data in JSON or XML: Format the response data in widely used formats like JSON or XML to facilitate client communication.
An example using Spring:
In this code, a simple RESTful service is created that responds to GET requests made to the endpoint. Implementing RESTful services is essential for creating APIs that allow different software systems to communicate with each other.
Front-End Integration
Front-end integration is a critical aspect of web development that bridges the gap between the server-side and the user interface. When building a website using Java, it is essential to grasp how front-end technologies interact with Java applications. The front end is where users engage with the website. Therefore, ensuring a smooth integration with back-end functionalities is key to providing a seamless user experience.
The front-end consists of three fundamental technologies: HTML, CSS, and JavaScript. Each of these elements plays a significant role in shaping the user experience. HTML (HyperText Markup Language) provides the structure of the web pages. CSS (Cascading Style Sheets) offers styling and presentation. JavaScript, on the other hand, facilitates interactivity and dynamic content generation.
One of the benefits of front-end integration is that it allows for a responsive design. This means the website can adjust to various screen sizes, enhancing usability across devices. Furthermore, through effective front-end integration, developers can ensure data fetched from the server is efficiently displayed to users, which is crucial for applications that demand real-time information.
When considering front-end integration, developers must also focus on performance and load times. Users tend to abandon a website that takes too long to load, so optimizing front-end resources is important. Utilizing tools such as minification for CSS and JavaScript can reduce file sizes and improve loading speeds.
Lastly, front-end integration is not just about aesthetics. A well-integrated front end also involves accessibility, ensuring that all users, regardless of their abilities, can effectively navigate the website.
Understanding HTML, CSS, and JavaScript Basics
Understanding the basics of HTML, CSS, and JavaScript is paramount for anyone looking to delve into web development.
- HTML is the backbone of a webpage. It organizes content and provides structure. Upon writing HTML, developers create elements like headers, paragraphs, and links which form the skeleton of the web application.
- CSS comes into play to enhance the visual appeal. It allows developers to apply styling rules to HTML elements, creating a more engaging user experience. The difference between plain HTML and styled elements is profound. Without CSS, websites would only display unformatted text and images.
- JavaScript adds the final touch by enabling dynamic interactions. It permits the manipulation of the DOM (Document Object Model), allowing elements to change in response to user actions without needing to refresh the page.
A solid understanding of these technologies provides a strong foundation for integrating Java-based applications with front-end functionalities effectively. For in-depth reading on these topics, resources like Wikipedia can be useful.
Using Java for Dynamic Content Generation
Java can be effectively employed to generate dynamic content on websites. Utilizing Java's server-side capabilities, developers can manipulate and serve content that changes based on user requests or actions. The process of generating dynamic content usually involves the following steps:
- User Input: A user interacts with the front end, such as submitting a form.
- Processing: The Java servlet or Java-based framework processes the input, potentially querying a database for relevant information.
- Response Creation: Based on the processing, Java generates an HTML response tailored to the user's request.
For example, if a user requests to view a list of products from a database, Java can retrieve the necessary data and dynamically create an HTML page displaying those products. This adaptability provides a richer user experience compared to static web pages.
Incorporating dynamic content generation makes websites interactive and user-focused, adapting to individual needs beyond just a one-size-fits-all approach.
Incorporating JavaScript Frameworks
Incorporating JavaScript frameworks into Java-based web applications amplifies functionality and enhances user interaction. Frameworks such as React, Angular, and Vue.js streamline the development process by offering pre-built components and efficient ways to manage state.
These frameworks allow developers to create complex user interfaces that can be easily maintained. Furthermore, by using JavaScript frameworks:
- Development time can be reduced significantly due to reusable components.
- Performance optimization is more straightforward as many frameworks support virtual DOM systems, increasing rendering speed.
- Applications can implement sophisticated features like asynchronous data fetching, enriching user experience without page reloads.
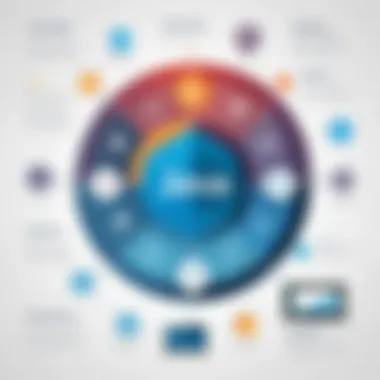
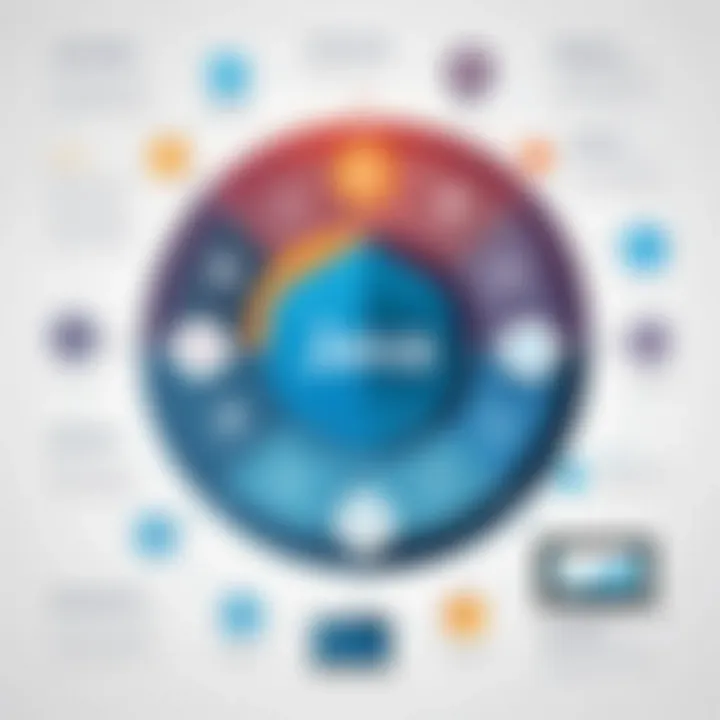
For instance, integrating a framework like React with a Java backend allows developers to build single-page applications where user interactions do not require full page refreshes. Instead, only the necessary information updates, making the application swift and responsive. This synergy between Java and JavaScript frameworks exemplifies effective web development practices.
Testing and Debugging
Testing and debugging are critical aspects of software development, especially in Java web development. They ensure that the application is functioning correctly and efficiently before it goes live. Many developers often underestimate the importance of these processes, but neglecting them can lead to unexpected errors and system failures. Testing allows developers to identify and rectify bugs, improving the overall quality and performance of the application.
Testing can take many forms, including unit testing, integration testing, and system testing. Each type serves a different purpose but ultimately contributes to code reliability. Debugging, on the other hand, involves diagnosing problems in the code and solving them. Effective debugging practices can save significant time and effort by addressing issues early in the development cycle.
Ultimately, a robust testing and debugging strategy not only ensures a smoother deployment but also enhances user satisfaction by delivering a stable and functional web application.
"Quality software is built through repeated tests and debugging, not luck."
Unit Testing with JUnit
Unit testing is a key practice in software development that involves testing individual components of the codebase to ensure they behave as expected. In Java, JUnit is the most widely used framework for unit testing. It provides a simple way to write and execute tests. By creating test cases for each unit of code, developers can validate that changes or new features do not introduce bugs into existing functionality.
Using JUnit, one can follow these basic steps to conduct unit testing:
- Set up the JUnit environment: Ensure that JUnit is included in the project build path. Generally, it is included through libraries in IDEs like Eclipse or IntelliJ.
- Create a Test Class: Each class that requires testing should have a corresponding test class annotated with . This tells JUnit which methods are tests.
- Write Test Methods: Inside the test class, write methods that test specific functionality of the class being tested. Assertions are used to check expected outcomes against actual results.
- Run Tests: Execute the tests in the IDE or command line. JUnit provides feedback on which tests have passed and which have failed.
Here is a simple code example using JUnit:
This code sets up a test for a hypothetical method in the . It verifies that adding 2 and 3 returns 5.
Debugging Techniques in Java
Debugging is the process of identifying and fixing bugs or issues in your code. Java provides various debugging techniques that can aid developers in finding errors effectively. Here are several commonly used techniques:
- Using Debugger Tools: Integrated Development Environments (IDEs) like Eclipse and IntelliJ come equipped with built-in debuggers. These tools allow you to set breakpoints and step through the code line by line, monitoring variable values and execution flow.
- Print Statements: Adding statements can help track the flow of execution and understand the state of variables at various points. This is a simple yet effective way of debugging.
- Logging: Using logging frameworks like SLF4J or Log4j can help you record information about your application's operation. Logs can be invaluable when trying to understand system behavior in production environments.
- Code Reviews: Engaging colleagues in code reviews can reveal potential issues that you might have overlooked. Collaboration helps improve the quality of the codebase.
In summary, both unit testing with JUnit and effective debugging techniques are integral to developing high-quality Java web applications. Ensuring that your code is well-tested and debugged not only enhances its reliability but also contributes to a robust user experience.
Deployment Strategies
Deploying a web application correctly is crucial for its long-term success. Deployment strategies take into account the ways a website is published and maintained. The right strategy ensures that the application functions efficiently under heavy loads, is secure, and can be updated seamlessly without downtime. Ignoring these aspects can lead to performance issues and user dissatisfaction. Hence, understanding deployment strategies becomes a fundamental part of the website development process.
A few key considerations when looking into deployment strategies include both the hosting environment and the specific methods used to deploy application code. These elements will dictate how well your Java application performs in real-world scenarios.
Choosing the Right Hosting Environment
When selecting a hosting environment for a Java web application, it is essential to assess the specific needs of your project. Several types of hosting options exist:
- Shared Hosting: This is a cost-effective option. However, it may not provide the performance or reliability needed for complex Java applications.
- Virtual Private Server (VPS): This offers dedicated resources and greater control, making it suitable for mid-sized applications.
- Dedicated Hosting: A powerful option for large applications that require high availability and performance.
- Cloud Hosting: Offers scalability and flexibility, allowing applications to handle sudden traffic spikes effectively.
In addition to the type of hosting, look out for the following factors:
- Support for Java Frameworks: Make sure that the hosting provider supports the specific frameworks needed for your application.
- Performance Metrics: Pay attention to server speed, uptime, and load balancing capabilities.
- Security Features: An essential aspect of any hosting environment is the security measures in place to protect your application.
"Choosing your hosting environment wisely can affect not only the performance of your application but also its overall user experience."
Deploying with Apache Tomcat
Apache Tomcat is a widely used open-source server for running Java applications. It is often chosen for its robust feature set and ease of use. Deploying a Java application on Tomcat involves several steps:
- Download and Install Tomcat: Begin by downloading the latest version of Apache Tomcat from the official website. Follow the installation instructions specific to your operating system.
- Configure Tomcat: Before deploying your application, it is important to configure server settings, such as port number and memory allocation. This ensures optimal performance.
- Package the Application: Use the Java Archive (JAR) or Web Application Archive (WAR) format to bundle your application. Ensure all dependencies are included.
- Deploy the Application: Place the packaged application into the directory of Tomcat. You can also use the Tomcat manager application for easier deployments.
- Start Tomcat Server: Launch the server using the startup script. Access the web application via a browser to check if it is functioning correctly.
During and after deployment, continuously monitor the performance and address any issues.
In summary, deploying a Java web application demands careful planning and execution. By choosing the right hosting environment and understanding how to use tools like Apache Tomcat effectively, developers can ensure their applications perform well and remain accessible to users.
Post-Deployment Management
Post-deployment management is a crucial phase in the website creation process. Once your Java application goes live, the work is far from finished. It is where maintenance, monitoring, and security come into play. Proper management ensures that the website remains functional, performs well, and is secure from threats. Notably, neglecting this stage can lead to performance issues or significant security vulnerabilities. Understanding how to navigate post-deployment effectively can set a solid foundation for long-term success of your web application.
Monitoring Application Performance
Monitoring application performance is vital for maintaining a healthy user experience. It allows developers to identify bottlenecks and optimize system resources. Tools such as New Relic, AppDynamics, and Google Analytics help track various performance metrics. Key metrics to consider include:
- Response Time: Measure the time taken to process requests. Slow response times can deter users.
- Error Rates: Identify how often requests result in errors. A high error rate can indicate underlying problems.
- Traffic Load: Understand user traffic patterns. This helps ensure that your infrastructure can handle peak loads.
Regular performance reviews enable timely adjustments, enhancing site reliability and user satisfaction. Furthermore, it is prudent to establish alerts to notify you of performance dips, prompting swift actions to mitigate issues.
Implementing Security Measures
Implementing security measures is non-negotiable in today's digital environment. Securing your Java web application should start during development and continue post-deployment. Here are several strategies:
- Input Validation: Always validate user inputs to prevent attacks like SQL injection.
- Encrypted Connections: Utilize HTTPS to secure data in transit, safeguarding sensitive information.
- Regular Updates: Keep your Java version and frameworks up to date. Security patches often address vulnerabilities found in older versions.
- Security Audits: Conduct periodic security reviews and penetration testing. This will help you identify and rectify weaknesses.
"A proactive approach to security often prevents issues before they arise."
In addition to these tactics, educating your team about security best practices is essential. Awareness can significantly reduce the likelihood of human error causing a security breach. Post-deployment management is about continuously evaluating and fortifying the security posture of your web application.
Finale
In this article, we have explored various facets of web development using Java. The journey through Java's role in creating websites has illuminated several important considerations. Understanding these elements is crucial for anyone wishing to become proficient in web development.
One significant aspect discussed is the importance of back-end development. Java's robustness allows for the creation of scalable and secure web applications. The emphasis on core Java concepts like object-oriented programming and exception handling equips developers with the skills necessary for building functional applications.
Additionally, we touched upon the significance of front-end integration. While Java excels in server-side development, it is essential to ensure a seamless connection between the front end and back end of a web application. A solid grasp of HTML, CSS, and JavaScript is beneficial, as these technologies complement Java's capabilities.
Moreover, the article highlights various tools and frameworks. Understanding popular frameworks like Spring and JavaServer Faces can greatly enhance productivity and streamline development efforts. Developers need to be aware of deployment strategies as well. Choosing the right hosting environment influences not only performance but also the overall user experience.
Post-deployment management is equally as critical. Monitoring performance and implementing security measures help maintain the integrity of the web application over time.
The future of Java web development looks promising. Emerging trends, like cloud computing and microservices architecture, continue to shape how applications are developed and deployed. By staying updated on these trends, aspiring developers can position themselves favorably in an evolving landscape.