Beginner's Guide to ASP.NET Framework Essentials
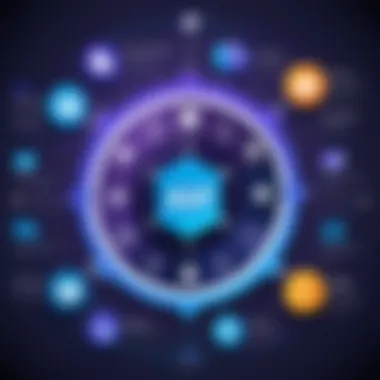
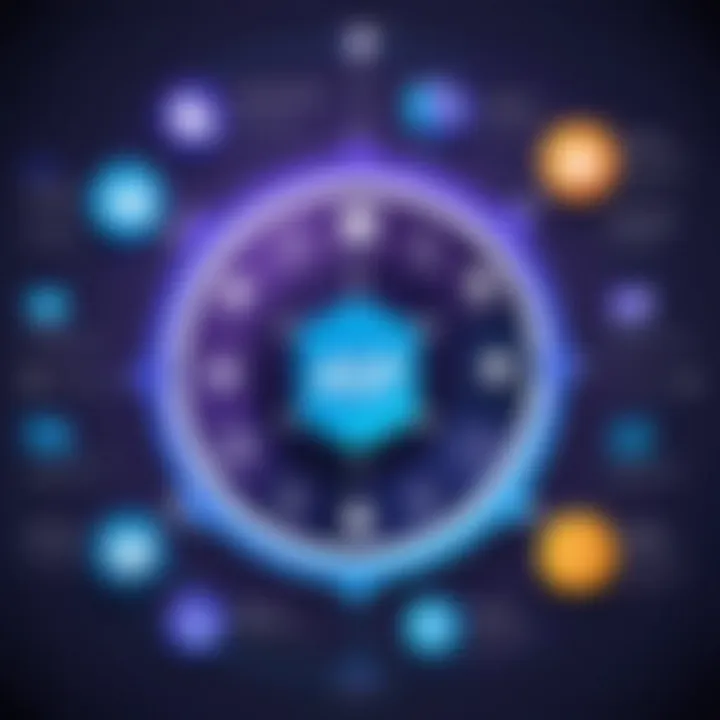
Prologue to Programming Language
ASP.NET is a widely used framework developed by Microsoft, designed primarily for building robust web applications and services. To appreciate the significance of ASP.NET in todayās programming ecosystem, one must first understand its historical context and evolution. From its early days as an ongoing answer to web development challenges, it has matured into a versatile environment leveraging various technologies to suit developersā needs.
History and Background
Launched in the early 2000s, ASP.NET emerged as a response to the growing demand for dynamic web content. At that time, developers sought a programmatic solution that would streamline the process of creating web applications. Initially, it was tied closely to the .NET framework, which offered functionality far beyond traditional web development environments. Over the years, ASP.NET has undergone several transformations, notably the introduction of ASP.NET Core, which allowed for cross-platform deployment and made it appealing for a wider range of projects.
Features and Uses
The core features of ASP.NET highlight its strength in web development. Here are some notable attributes:
- Performance: ASP.NET applications are fast due to their compiled nature and efficient memory management.
- Security: Built-in security features help mitigate common threats, such as cross-site scripting and SQL injection.
- Scalability: It easily accommodates the growth of applications as user demand increases.
- MVC Framework: The Model-View-Controller architecture promotes organized code structure, making it easier to manage.
This framework is predominantly used for creating various types of applications including e-commerce sites, content management systems, and enterprise-level systems. Its adaptability makes it suitable for both small-scale projects and large-scale enterprise solutions.
Popularity and Scope
In terms of popularity, ASP.NET holds a significant position among web developers. While various programming languages and frameworks vie for attention, ASP.NET remains a mainstay due to a few compelling reasons:
- Strong Community Support: The number of developers working with ASP.NET contributes to vast reservoirs of resources and shared knowledge.
- Integration with Microsoft Products: Seamless integration with tools such as Visual Studio enhances its appeal.
- Cross-Platform Capabilities: With ASP.NET Core, developers can build and run applications on Windows, macOS, and Linux alike.
Such features have placed ASP.NET comfortably within the realms of enterprise development and modern web applications, cementing its relevance in the programming world today.
"ASP.NET bridges the gap between static HTML pages and dynamic content, enabling a richer user experience without compromising on performance."
In understanding this frameworkās intricacies, newcomers can begin to navigate the vast landscape of web development, laying the groundwork for more advanced techniques and practices to follow.
Preface to ASP.NET
Understanding ASP.NET is crucial for anyone stepping into the world of web development. This framework offers a solid groundwork for creating dynamic, scalable, and high-performing web applications. With the surge of digital platforms, the demand for proficient developers is at an all-time high. Knowing ASP.NET can significantly boost your skillset, making you an attractive candidate in a competitive job market.
ASP.NET is not just about building websites; it encapsulates an extensive ecosystem that promotes clean coding practices, robust security measures, and seamless integration with various databases. Throughout this article, we will delve deeper into the vital components of ASP.NET, ensuring you grasp both foundational and advanced concepts.
What is ASP.NET?
At its core, ASP.NET is an open-source framework designed specifically for building web applications. Developed by Microsoft, it allows developers to create dynamic websites, web applications, and web services. One of the hallmark features of ASP.NET is its ability to employ multiple programming languages, including C# and VB.NET, thereby providing developers with flexibility in their coding practices.
Here are some key points about ASP.NET:
- Framework Versatility: ASP.NET supports a range of application types, from traditional web pages to modern web APIs and microservices.
- Performance Optimization: It includes a host of built-in optimization features which enhance the overall user experience.
- Security Features: Provides strong security protocols like authentication and authorization, allowing your applications to safely handle sensitive information.
In essence, ASP.NET bridges the gap between ease of use and powerful functionality, making it a go-to choice for aspiring and seasoned developers alike.
The Evolution of ASP.NET
Since its inception, ASP.NET has undergone significant changes, evolving into a robust framework that caters to the needs of modern web development.
- Initial Release: ASP.NET was first introduced in January 2002. The framework quickly gained traction as it simplified the web development process, offering server-side scripting capabilities through a plethora of built-in controls.
- ASP.NET MVC: In 2009, Microsoft launched ASP.NET MVC, which brought a new architectural pattern to the table. MVC stands for Model-View-Controller, and it equipped developers with the ability to create manageably complex applications by separating the application logic, user interface, and input handling.
- ASP.NET Core: The latest iteration, ASP.NET Core, hit the scene in 2016. It marked a significant shift by allowing cross-platform development, thus extending beyond the Windows environment. With ASP.NET Core, developers can build applications for Windows, macOS, and Linux.
The transition from ASP to ASP.NET Core illustrates technological adaptation over time, ensuring that developers have access to modern tools that can meet the demands of today's online experience.
As we continue through this tutorial, you will learn how ASP.NET architecture works, the tools it encompasses, and how these elements come together to create successful web applications.
Understanding the Architecture
Understanding the architecture of ASP.NET is foundational to effectively leveraging its capabilities. This knowledge serves as a bridge between the conceptual and practical aspects of web development. Recognizing how ASP.NET is structured allows developers to harness its components efficiently, optimize performance, and troubleshoot issues that may arise during application development.
The architecture is designed not only for ease of use but also for the scalability and maintainability of applications. With a modular setup, developers can pick and choose components that best fit their projectās needs, reducing unnecessary overhead. This adaptability is invaluable especially in a rapidly evolving tech landscape, where flexibility translates to speed and success in deployment.
Core Components of ASP.NET
ASP.NET's architecture is comprised of core components that work in harmony to deliver robust applications. Here are the key elements:
- Common Language Runtime (CLR): This component of the .NET framework runs the code and provides services such as memory management, security, and exception handling. Itās like the engine under the hood that makes sure everything runs smoothly.
- Framework Class Library (FCL): This expansive collection of reusable classes and functions is like the toolbox for developers. It provides components for everything from web services to data access.
- ASP.NET Web Forms: This component enables developers to build dynamic websites and applications with a simplified event-driven model.
- ASP.NET MVC: A framework that encourages a separation of concerns, facilitating better organization and management of code through the model-view-controller design pattern.
- ASP.NET Web API: This technology is crucial for building RESTful applications, allowing for seamless interaction between client-side applications and server-side data.
These components work together to create a versatile environment that caters to various web application needs. Understanding these elements helps developers make more informed decisions when choosing how to approach a project.
Request-Response Cycle in ASP.NET
The request-response cycle in ASP.NET is the backbone of web application communication. Understanding this process is critical for developers, as it directly affects how users interact with the application. Every time a user makes a request, here's how it generally unfolds:
- Client Request: This begins when a user sends an HTTP request (usually by clicking a link or submitting a form).
- Request Routing: ASP.NET takes the request and routes it to the appropriate handler. This could be a Web Form or an MVC controller based on how the application is structured.
- Processing: Once directed, the appropriate handler processes the request. This processing might involve querying a database, executing business logic, etc.
- Response Generation: After processing, ASP.NET generates a response. This is typically rendered as HTML, but can also be formatted as JSON or XML for APIs.
- Client Response: Finally, the response is sent back to the clientās browser, completing the cycle. The browser then displays the results to the user.
This cycle emphasizes the need for effective code management and structure to ensure that applications remain responsive and performant. Implementing best practices during the request-response cycle can significantly impact overall user experience and application efficiency.
Understanding the request-response cycle is critical to building responsive and performant ASP.NET applications. This foundational knowledge empowers developers to optimize user interactions effectively.
Setting Up the Development Environment
Setting up your development environment is a crucial step in embarking on your journey with ASP.NET. A well-configured environment can streamline your workflow, minimize frustrating hiccups, and enhance your overall productivity. Think of it like laying a strong foundation before constructing a building; without it, the entire structure may crumble under pressure.
In this section, weāll discuss the specific components that are essential for developing ASP.NET applications. Weāll explore the benefits of using an Integrated Development Environment (IDE), the specific features you should consider, and how to optimize your setup for success. By the end, you should feel fully prepared to dive into ASP.NET development.
Installing Visual Studio
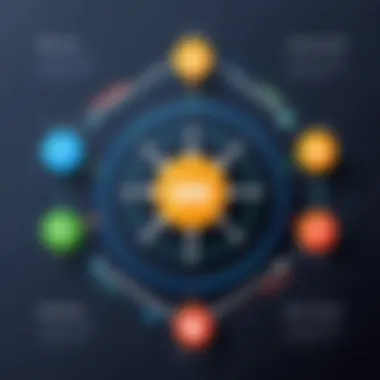
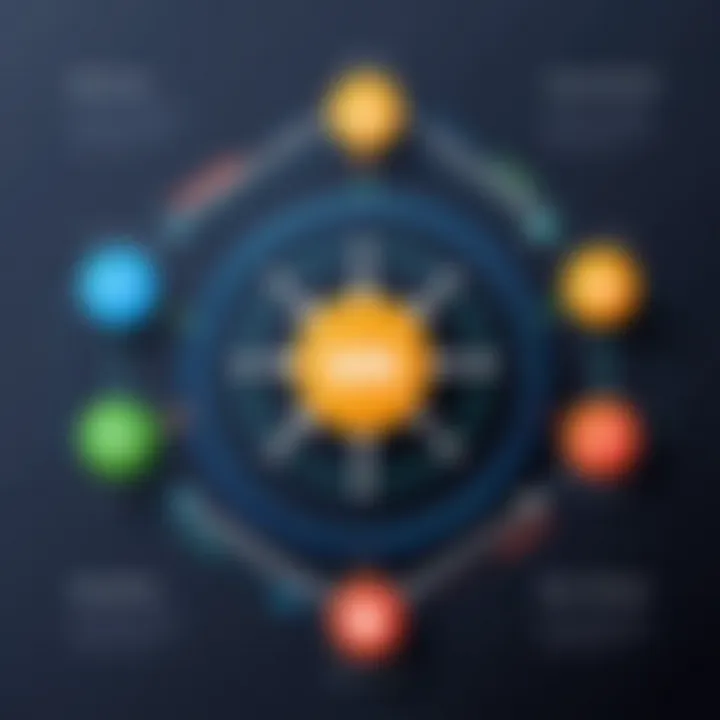
Visual Studio is arguably the most recommended IDE for ASP.NET development. It provides a robust set of tools and features that cater specifically to .NET developers. Letās break down why itās beneficial to use Visual Studio and how to install it.
- User-Friendly Interface: The layout of Visual Studio is intuitive, even for those who are just stepping into the world of programming.
- Integrated Version Control: Having Git integration within the IDE facilitates easier collaboration with other developers by keeping your code versioned.
- Debugging Tools: Visual Studio presents an array of debugging functionalities that can help you track down and fix errors efficiently.
- Extensions and Plugins: The IDE offers a marketplace filled with plugins to enhance your development experience, allowing you to customize it to fit your needs.
Steps to Install Visual Studio:
- Visit the official Visual Studio website to download the installer.
- Choose the version that suits your needs. For beginners, the Community edition is free and packed with useful features.
- Run the downloaded installer and select the workloads that are relevant to your ASP.NET development, such as "ASP.NET and web development."
- Follow the on-screen instructions to complete the installation.
- Once installed, launch Visual Studio and set up your development preferences.
By the end of this process, you will have a robust environment that is capable of handling all your ASP.NET projects smoothly.
Creating Your First ASP.NET Project
After you've installed Visual Studio, it's time to create your first ASP.NET project. This step is often where the magic begins. You don't just want to dive into coding; you want to understand the structure and components that make up an ASP.NET application.
Hereās how you can start your journey:
- Open Visual Studio and select "Create a new project."
- In the project templates, filter by selecting if your focus is on the latest technology.
- Choose the framework version and hit Create.
- Fill in the project details, like the project name and location on your computer. Hereās a tip: keep it descriptive yet brief.
- After that, select the type of authentication you prefer, or you can leave it at No Authentication for now.
- Click on Create, and in a few moments, Visual Studio will set up a skeleton project for you.
This skeleton will include an initial folder structure, built-in properties, and even some starter code. Youāll notice a number of files and folders created automatically, giving you a solid starting point.
Hereās a brief rundown of what youāll see:
- The wwwroot folder holds static files like CSS and JavaScript.
- The Controllers folder will contain your controllers where the logic resides.
- The Views folder is where your views are located if youāre following MVC architecture.
"The best way to learn is by doing. Start building small projects, and youāll soon find yourself capable of tackling larger ones."
Diving into Web Forms
When it comes to web development using ASP.NET, understanding Web Forms is essential. This framework allows developers to design dynamic and interactive web applications with ease. Web Forms abstracts away much of the complexity involved in handling HTTP requests, making it easier for programmers to build pages that generate content dynamically. In a world where user experience is paramount, the ability to craft responsive interfaces efficiently cannot be underestimated.
Web Forms are particularly appealing for beginners, given their event-driven nature, leveraging a familiar approach that many developers associate with desktop applications. This can be a smoother transition for those who have experience in other programming languages or environments. However, grasping Web Forms also comes with its own set of considerations like maintaining state across post-backs and understanding view state management. Here's why it matters:
- Rapid Application Development: Web Forms offer a rich set of server controls that can be quickly assembled to create a user interface without diving into all the markup necessary in other systems.
- State Management: Knowing how Web Forms handle state is critical because web applications are stateless by default. Learning how to properly manage state can separate a great web application from a good one.
- Integration with Backing Logic: ASP.NET Web Forms effectively connect with databases and other business logic, making data-driven applications easier to create.
- Rich Component Library: The framework comes with a wealth of built-in controls that can cater to a vast array of functionalities, from buttons to data grids.
Overall, diving into Web Forms introduces a way to build robust applications while easing the learning curve that beginners often face in web development.
Understanding Web Forms Basics
To get started with Web Forms, itās crucial to grasp the fundamental concepts that underpin the framework. At its core, a Web Form is a modular unit that encapsulates data and behavior. This setup provides a base for creating applications where you can process user input and generate output seamlessly. Here are a few key components to familiarize yourself with:
- Server Controls: These are essential building blocks that offer functionality and UI elements. They react to user events, making it easy to handle input without writing excessive amounts of code.
- View State: This feature allows Web Forms to maintain state information across requests. Understanding how view state works will empower you to build interfaces that remember user interactions.
- Page Lifecycle: Each Web Form goes through several stages during its lifecycle, from initialization to rendering. Knowing these stages helps in debugging and optimizing performance.
Taking the time to navigate through these elements will give you the groundwork needed to effectively utilize the power of ASP.NET Web Forms.
Creating a Simple Web Form
Creating your first Web Form is a straightforward process. Follow these steps to set up a basic example:
- Open Visual Studio: Start your IDE of choice. Open a new project and select an ASP.NET Web Forms template.
- Add a Web Form: Right-click on your project in the Solution Explorer, then choose to add a new item. Select the Web Form template and give it a name.
- Design the Layout: Use the drag-and-drop features to lay out your controls. Begin by adding a TextBox for user input and a Button to submit the data.
- Code the Backend Logic: Double-click on the Button control, and youāll be taken to the code-behind file where you can write the logic to handle the event. Hereās a simple example:
- Run Your Application: Hit F5 to compile and run your application. You should see the Web Form interface, and upon entering your name and clicking the button, a greeting appears.
This practical experience gets your feet wet in the ASP.NET development process and helps demystify the workings of Web Forms.
Exploring Framework
The Model-View-Controller (MVC) framework serves as an essential architecture in ASP.NET, emphasizing separation of concerns which creates a clear division between the user interface, application logic, and data. This structure is pivotal as it not only promotes organized coding practices but also enhances the maintainability and scalability of web applications. It fosters a development environment where multiple developers can work simultaneously without stepping on each other's toes. In the following sections, we dive deeper into the core components of the MVC architecture and provide a hands-on approach to building a web application utilizing this framework.
Preamble to Architecture
At its core, the MVC pattern divides an application into three interconnected components:
- Model: This part encapsulates the data and business logic. It manages the data of the application and informs the View (UI) about updates or changes to the data.
- View: The View is essentially the user interface; it displays the data from the Model and sends user commands to the Controller. Itās where the interaction with end-users occurs.
- Controller: The Controller acts as an intermediary between the Model and the View. It processes requests, handles user input, and updates the Model or View accordingly.
This architecture is beneficial in fostering a clean separation of concerns, ultimately making it easier for developers to manage complex applications. By isolating the different components of the application, modifications can be made in one layer without impacting the others. For instance, if a developer decides to redesign the user interface, the underlying business logic in the Model can remain unchanged. It's this flexibility that makes MVC a go-to pattern in modern web development.
"MVC shines in its ability to maintain a clean codebase. This is crucial for agile dev processes."
Building an Application
Creating an MVC application in ASP.NET means diving into code that is often more structured than other web frameworks. To bring theory into practice, let's outline the steps typically involved in building a simple MVC application:
- Set Up the Project: Begin by creating a new project in your IDE, such as Visual Studio. When prompted, select the MVC template to kickstart your project setup.
- Define the Model: Create a new class file that represents the data structure you will manage. This is where more complex business logic can sometimes reside.
- Create the Controller: Add a controller that will handle incoming requests and return appropriate responses. For instance, you might create a to manage product-related requests.
- Develop the Views: Define how the data will be presented using Razor syntax. Razor views simplify creating dynamic content and will be used to display product information.
- Routing: Ensure that URL routing is properly set up so that requests to different endpoints are handled correctly by the appropriate controller actions.
By following these steps, developers can create a cohesive MVC application that is not only functional but adheres to the principles of the model-view-controller architecture. For those who are keen learners or new to programming, taking time to grasp the MVC pattern is a worthwhile investment toward mastering ASP.NET.
Working with Data
When developing applications with ASP.NET, one cannot underestimate the crucial role that data management plays. When users interact with your web application, itās likely that theyāll be pushing, retrieving, or manipulating data in some manner. The way you handle and structure this data can be the difference between an application that sings and one that stutters. This section will delve into two fundamental aspects of working with data: connecting to a database and utilizing the Entity Framework.
Connecting to a Database
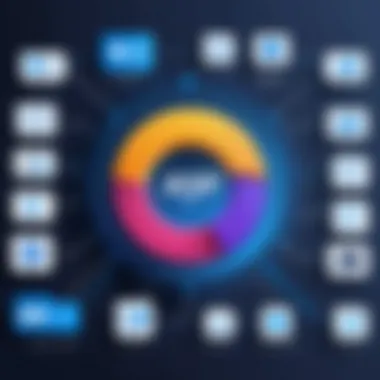
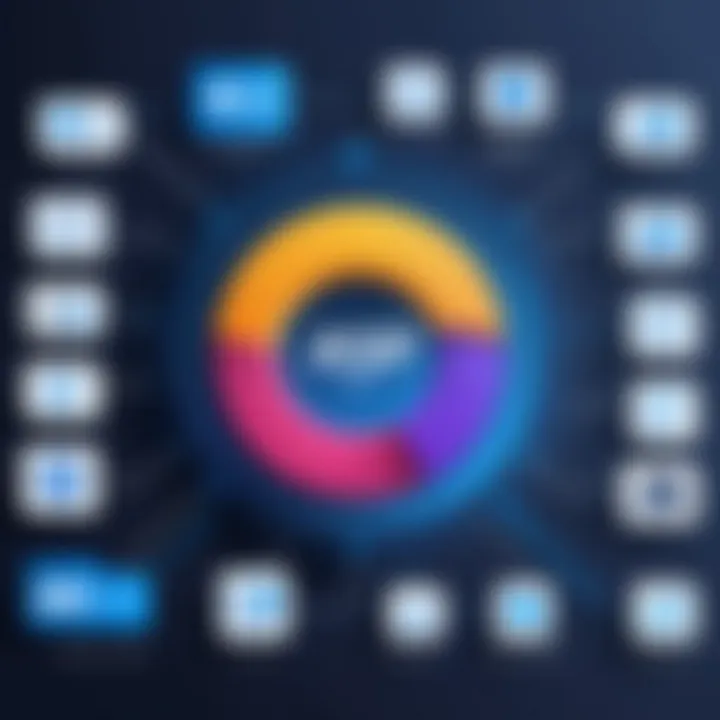
To make any application truly functional, it needs to communicate with a database. A database serves as the backbone of data storage, allowing for ease of access and management. In ASP.NET, connecting to a database is seen as setting the stage for a performance ā without that connection, nothing truly happens.
The most common relational databases used with ASP.NET include SQL Server, MySQL, and PostgreSQL. Here are some key points to consider:
- Connection String: This is a critical piece. It includes the server name, database name, user credentials, and other parameters necessary to establish a connection. For example:
- Data Provider: In ASP.NET, data providers like ADO.NET allow you to execute commands and handle data retrieval. They offer a bridge between the application and the database.
To connect to a database in your ASP.NET project, you typically use the class from the namespace. Hereās a simple illustration:
By establishing this connection, you set everything in motion. But it's essential to manage this connection properly to avoid memory leaks and ensure efficiency.
Using Entity Framework
Once youāve established a connection to a database, the next logical step is to simplify and streamline your data operations. This is where Entity Framework comes into play. Entity Framework (EF) is an Object-Relational Mapper (ORM) that allows developers to work with data as domain-specific objects, rather than dealing directly with database tables.
Benefits of Entity Framework:
- Productivity: With EF, you write much less code than with traditional approaches. For instance, fetching data can be as simple as querying a collection of objects rather than writing dozens of SQL statements.
- Maintainability: The clear mapping of objects to tables makes your code easier to understand and maintain.
- Type Safety: Since youāre working with strongly-typed objects, you catch many errors at compile-time rather than at runtime, saving time during development.
Getting Started with Entity Framework:
Setting up Entity Framework in ASP.NET involves a few essential steps. First, you must install the EF package via NuGet. You can do this in the Package Manager Console:
Next, you define your data model using classes. For instance:
Using EF, you can then write queries in a more intuitive manner. Want to find all products? Simply run:
Tip: It is a good practice to familiarize yourself wih EF migrations. This allows for smooth transitions when your data model evolves over time.
ASP.NET Web API
ASP.NET Web API is a powerful framework for building services that can be consumed by a wide array of clients, including browsers and mobile devices. With the rise of applications that demand seamless data interaction, understanding ASP.NET Web API becomes crucial for developers today. This section examines its significance in the web development world, emphasizing the benefits it brings to the table as well as the considerations developers should keep in mind when working with it.
Why is ASP.NET Web API considered important? A few key reasons include:
- Simplicity: It provides a straightforward way to build RESTful services that can be easily consumed by other applications. This simplifies the development process.
- Flexibility: It supports a variety of media types such as JSON, XML, and others which allows the API to communicate with any client that can consume these formats.
- Scalability: It can handle a high number of requests thanks to its stateless nature and is very efficient in handling data operations across platforms.
- Integration: ASP.NET Web API is also designed to integrate well with other ASP.NET features such as MVC, making it easier for developers familiar with these frameworks to adopt it.
However, it is essential to consider aspects like security and versioning while utilizing Web API, to ensure a robust and maintainable application. Understanding these components ensures both developers and users have a smooth experience while interfacing with web services.
Preamble to RESTful Services
REST (Representational State Transfer) is an architectural style that became a blueprint for designing networked applications. When you dive into RESTful services within ASP.NET Web API, you find they facilitate standard web protocols. Understanding the key principles of REST is essential for maximizing the effectiveness of your services.
Here are some fundamental characteristics of RESTful services:
- Stateless Communication: Each request from a client must include all the information needed to understand and process the request independent of the previous requests.
- Resource-Based: It focuses on resources, which are identified using URIs. This allows better organization and retrieval of information.
- Using HTTP Methods: Actions like creating, reading, updating, and deleting are mapped to standard HTTP methods: POST, GET, PUT, and DELETE.
- Representations: Resources can have multiple representations, allowing clients to request data in a format that best suits their needs.
By adhering to these principles, your RESTful services can become powerful tools for data exchange, ensuring both performance and reliability.
Creating a Web API Project
To set up your first ASP.NET Web API project, you first need Visual Studio installed on your machine. Hereās a step-by-step guide to help get you started:
- Open Visual Studio: Make sure you have the latest version to access all the features.
- Create a New Project: Click on , choosing the template.
- Select the Web API Template: Once prompted, choose the Web API template. This includes all necessary references to get you up and running.
- Configure the Project: Set up the project name and location as per your preference. You can also specify the framework version here.
- Build Your Model: Start building your data models which represent your business logic. This can include classes that define how data is structured and how it interacts with your Web API.
- Add Controllers: Create controllers to handle incoming requests and produce appropriate responses. This is where you'll define the routes for accessing your resources.
- Test Your API: Utilizing tools such as Postman or even your browser, perform GET, POST, PUT, and DELETE operations to ensure everything is functioning as expected.
Hereās a quick code snippet to define a simple API endpoint:
By following these steps, you'll have laid the groundwork for a functioning Web API that can be expanded and refined as needed.
In summary, ASP.NET Web API stands as a cornerstone of modern web application architecture. Learning its nuances equips developers with the skills to create applications that are both interactive and efficient.
Security in ASP.NET
Security in ASP.NET is a cornerstone of developing robust web applications. In our increasingly digital world, protecting user data and maintaining the integrity of applications are paramount. Without a solid security framework, even the most beautifully crafted websites can become vulnerable to a plethora of threats, ranging from data breaches to unauthorized access.
ASP.NET provides a structured approach to security, which is critical as applications often operate over public networks where potential threats lurk. Issues like Cross-Site Scripting (XSS), SQL Injection, and Cross-Site Request Forgery (CSRF) can make anyone shudder, but with the right knowledge and practices, developers can fortify their applications against these risks.
Understanding Authentication and Authorization
Authentication and authorization are two sides of the same coin but serve different purposes. Authentication is the process of verifying the identity of a user, while authorization determines what resources a user can access after they have been authenticated.
In ASP.NET, developers often utilize the built-in membership system, which offers support for user registration, login, and management of users. This system simplifies the process immensely but understanding how it works under the hood is crucial. For example, hereās how these processes break down:
- Authentication: This typically involves users providing credentials like usernames and passwords. Once these are entered, ASP.NET verifies them against stored records, indicating whether users are who they claim to be.
- Authorization: After successful authentication, roles and permissions dictate access levels to different resources. For example, general users might be limited to regular content, while admins may have access to sensitive areas of the application.
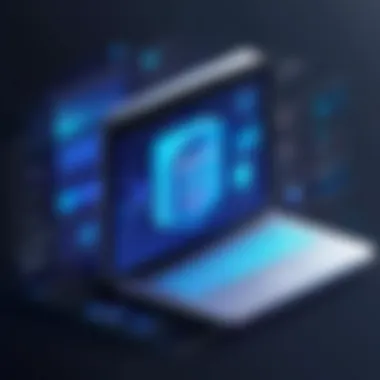
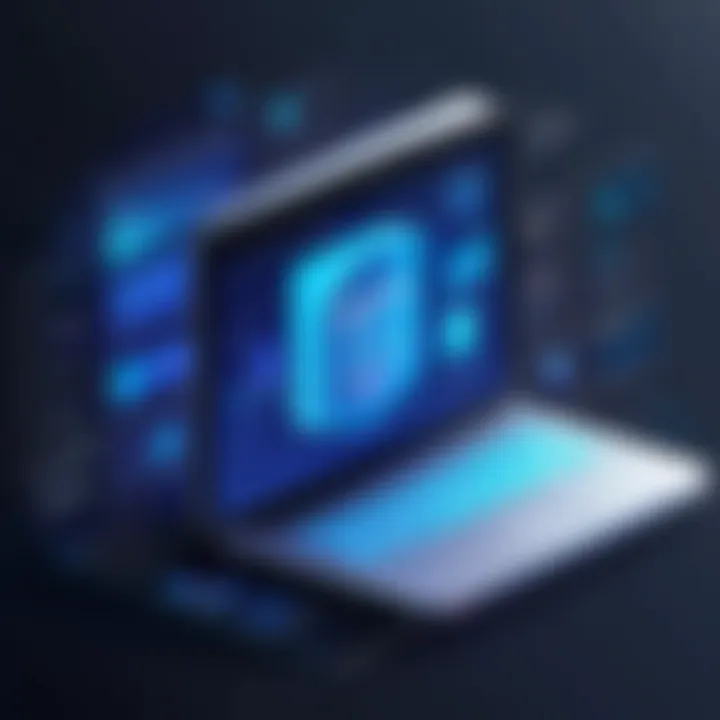
Using methods like Claims-based authentication, developers can also convey additional information about users that can aid in finely tuning permissions across your application, making it smarter and more flexible.
Here's an example of how you might define roles for granular access control:
Implementing Security Best Practices
Implementing security best practices is like putting on a seatbelt before you drive; it might feel unnecessary at times, but itās vital for safety. Here are several strategies to consider when securing your ASP.NET applications:
- Use HTTPS: Ensure that your site uses SSL to encrypt data between the client and the server. Not only does this protect sensitive information, it also enhances trust with your users.
- Input Validation: To avoid attacks such as SQL Injection and XSS, validate and sanitize all user inputs. Trust no one. Always assume user input could be malicious.
- Regularly Update Framework and Libraries: Keep ASP.NET and its dependencies up-to-date to shield against known vulnerabilities.
- Multi-Factor Authentication (MFA): Introduce an additional layer of protection by requiring users to verify their identity through something they have (like a phone) in addition to their password.
Always keep your ear to the ground for new security threats in the development community. Staying informed is half the battle.
- Error Handling: Avoid revealing sensitive application error details. Use generic error pages that provide users with no hint about your setup.
Each of these practices contributes to a holistic security posture that can significantly reduce risk and elevate the integrity of your ASP.NET applications.
Deployment Strategies
Deploying an ASP.NET application isnāt just a tick-box exercise. Itās a decisive step that can dramatically impact the performance, accessibility, and overall user experience of your web application. Understanding deployment strategies is crucial for anyone looking to take their development efforts live. Choosing an appropriate deployment strategy ensures that your application is reliable, scalable, and maintainable over time.
One of the key elements to consider during deployment is where your application will reside after it's published. This location ā can be a Local Server, a Cloud Provider, or a dedicated Hosting Service. Choosing the right environment affects speed, reliability, and cost. Moreover, itās important to implement a robust strategy for continuous deployment, enabling frequent updates while minimizing disruptions.
Publishing Your ASP.NET Application
Publishing an ASP.NET application is a meticulous process that requires attention to detail. But, donāt sweat it; once you get the hang of it, it becomes straightforward. The primary goal when you publish is to transfer your local build to a server where it can be accessed by users. Hereās how this can typically go:
- Choose a Publishing Method: ASP.NET provides several ways to publish your application:
- Configuration: Make sure your application settings, such as connection strings for database access, are configured correctly for the production environment. Hereās a critical point: your local settings often differ from what you need in production.
- Test: Just because it worked locally doesnāt mean it will on the server! Make sure to conduct thorough testing to identify any issues that could disrupt user access.
- Web Deploy: This option allows you to deploy directly to IIS servers, maintaining functionality while updating your app.
- ZIP Package: Packaging your application into a ZIP file can make it simple to upload later to your server.
- FTP: While somewhat old-school, FTP remains a popular choice for smaller applications.
Tip: Always maintain a backup of the original working application before deployment. That way, if anything goes haywire, you can quickly restore it.
- Monitor Performance: After deploying, donāt just sit back; use tools to monitor performance and catch any potential hiccups. Performance analytics can provide insight into how your application is functioning in the wild.
Choosing a Hosting Provider
Choosing a hosting provider might feel akin to choosing a car in a crowded showroom ā lots of features and options, but what do you really need? With so many options available, every developer must weigh factors that impact their applicationās performance and cost.
When selecting a hosting provider, consider the following elements:
- Scalability: Ensure your provider can accommodate your growing needs. As your application gains traction, itās crucial to scale without suffering downtimes.
- Support Services: Find a host with reliable customer support. If you encounter issues, having live support can save you headaches.
- Security Features: Look for providers that prioritize security, particularly if your application handles sensitive data. Features like SSL certificates, firewalls, and automatic backups can be lifesavers.
- Cost: Compare services and what you get for your bucks. Sometimes, the lowest price can come with significant restrictions, while some mid-tier options may offer non-essential perks.
- Type of Hosting: Decide between Shared Hosting, VPS, Dedicated Servers, or Cloud Hosting based on your needs. Each type has its advantages and drawbacks; for instance, Shared Hosting is cost-effective but can impact performance during high traffic.
In short, the right hosting provider should align with both your technical needs and your budget. Take the time to do research and read user testimonials or feedback. The right choice will not only enhance your applicationās performance but also bolster user trust and satisfaction.
Troubleshooting Common Issues
Troubleshooting common issues is essential for anyone venturing into ASP.NET development. Understanding how to identify and resolve problems fast is crucial in maintaining the efficacy of your applications. As you dive into coding, it's easy to encounter errors, and knowing how to diagnose them not only saves time but also fosters a deeper understanding of the framework and how all its components interact. Problems can arise from various areas such as code bugs, configuration errors, or environmental mismatches, and each of these requires a careful approach to solve.
Debugging Techniques
Debugging techniques are the bread and butter of successful problem-solving. Rather than throwing your hands in the air at the first sign of trouble, employing systematic debugging strategies will help you trace the root of issues.
- Use Breakpoints: In Visual Studio, placing breakpoints allows you to pause execution at a specific line of code. This way, you can inspect variable values and application state at that point without rushing through the execution.
- Step Through Code: Use the step in and step out features. This lets you go through your code line-by-line, providing insight into the flow of execution and allowing you to spot where things might be going sideways.
- Check the Output Window: The output window can be a treasure trove of information. It logs significant events, error messages, and other useful information that can point you in the right direction.
- Utilize Logging: Adding logging statements at various points in your application helps track the flow of execution and capture unexpected behavior. This could be logs for data entering a method or capturing exceptions thrown when trying to access a database.
Above all, maintaining a calm head when debugging is vital. Often, stepping back and reviewing your code with fresh eyes can yield surprising results. Remember, every programmer runs into issues, and it's part of the learning journey.
Common Error Messages Explained
Encountering error messages is an inevitable part of developing with ASP.NET. However, deciphering them can feel like solving a puzzle. Here are some of the more common error messages you might come across, along with what they typically signify:
- NullReferenceException: This error pops up when you try to access a member on a rigged object reference that is null. To solve this, ensure the object is instantiated before use.
- HTTP 404 - Not Found: This indicates that the requested resource cannot be found on the server. It's possible the user has d a URL or a file is missing from the specified path.
- InvalidOperationException: This error hints that a method call is invalid given the objectās current state. Reviewing the conditions under which you're calling methods will often help here.
"Errors are the keys to success. Debugging teaches you to think critically."
As you work through these messages, each one can teach you valuable lessons about ASP.NET and its environment. While it may feel tedious at times, gaining experience in interpreting and resolving these errors will undoubtedly strengthen your skills as a developer. By embracing the troubleshooting process, you'll find it becomes less of a chore and more of an enlightening aspect of your ASP.NET journey.
Ending and Further Resources
As we wrap up this comprehensive guide on ASP.NET for beginners, it's crucial to reflect on what you've learned throughout this journey. Mastering ASP.NET is not just about understanding its various components or the multitude of tools at your disposal; itās about grasping how to effectively apply these concepts in the real world. By now, you should have developed a solid foundation that empowers you to tackle web application development with confidence.
In this article, we explored core topics such as the architecture of ASP.NET, the mechanics of the MVC framework, data handling with Entity Framework, and securing applications against common threats. Each of these elements plays a significant role in shaping a well-rounded understanding of ASP.NET. Moreover, having a grasp on problem-solving techniques, like debugging, helps in navigating through the inevitable challenges that come with tech development.
Recap of Key Learning Points
To reiterate, here are the key points we covered:
- What is ASP.NET?
ASP.NET is a powerful framework that allows developers to build dynamic web applications and services using languages like C# and VB.NET, providing a robust environment for creating scalable applications. - Architecture Fundamentals:
Understanding the structure of ASP.NET, including its request-response lifecycle and key components like HTTP modules and handlers, sets the stage for building effective apps. - MVC Framework Overview:
The Model-View-Controller pattern promotes separation of concerns, allowing for better organization of code, making it easier to manage, test, and scale applications. - Entity Framework and Data Management:
Using Entity Framework simplifies database interactions through Object-Relational Mapping, enabling developers to work with data in a more intuitive manner. - API Development:
The ASP.NET Web API framework facilitates the creation of RESTful services, giving developers the tools to build APIs that are easy to interact with and integrate into various applications.
Recommended Books and Online Courses
For those looking to deepen their understanding of ASP.NET, I suggest exploring the following resources:
- Books:
- Online Courses:
- Discussion Forums: For ongoing support and community interaction, platforms like Reddit and Stack Overflow provide valuable insights and answers to your ASP.NET queries.
- Pro ASP.NET Core MVC 2 by Adam Freeman: A well-structured guide that focuses on modern development practices within ASP.NET.
- ASP.NET Core in Action by Andrew Lock: Offers hands-on projects and clear explanations that make it suitable for both beginners and intermediates.
- Microsoft Learn: Engage with interactive courses tailored to beginners, which thoroughly cover ASP.NET and its applications. Check it out here.
- Coursera: Look up their ASP.NET courses which often include real-world projects that cement your learning.
Lastly, take your time to apply what you've learned, explore projects independently, and engage with the community. Continued practice and inquiry will bolster your skills and position you for success in your web development endeavors.