Mastering Java: Essential Code Examples for All Levels
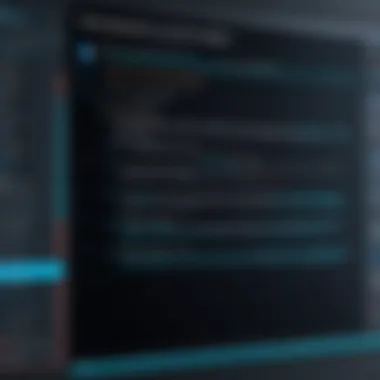
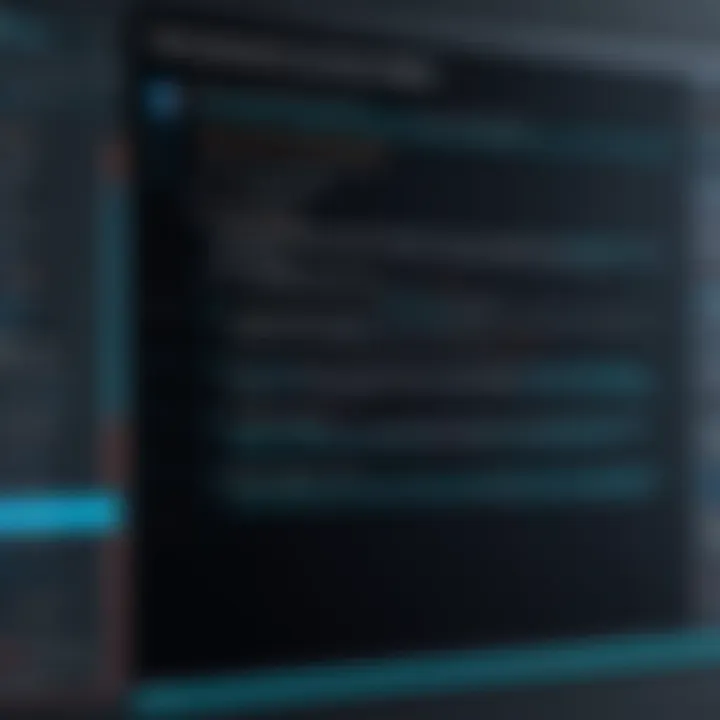
Prelims to Programming Language
Java stands as one of the most influential programming languages in the world. Its inception can be traced back to the early 1990s when James Gosling and his team at Sun Microsystems embarked on a project aimed at creating a platform-independent language that could foster the development of interactive television. This project soon evolved into what we now recognize as Java.
History and Background
Java was officially released in 1995 and quickly gained traction. Its ability to run on any device equipped with Java Virtual Machine (JVM) made it unique. This "write once, run anywhere" principle became a hallmark of the language. Given its versatility, Java found applications in web development, enterprise applications, mobile apps through Android, and embedded systems.
Features and Uses
The characteristics of Java contribute significantly to its popularity. It is an object-oriented, class-based programming language that emphasizes portability, security, and performance. Java's multithreading capabilities allow users to execute multiple threads simultaneously, enhancing efficiency.
Common uses of Java include:
- Web Development: Server-side applications via technologies like JavaServer Pages (JSP) and Servlets.
- Mobile Development: Android applications primarily use Java.
- Enterprise Solutions: Frameworks like Spring make Java a preferred choice for backend services.
Popularity and Scope
Java maintains a strong position in the programming community. Despite the emergence of numerous languages, it continues to rank highly among developers. Its role in building enterprise-level solutions further solidifies its relevance. The language’s robustness, combined with a vast ecosystem of libraries and frameworks, continues to attract new learners and experienced programmers alike.
"Learning Java prepares students not only for programming, but also for problem-solving and logical thinking."
Basic Syntax and Concepts
Understanding the basic syntax is essential for writing effective Java programs. Here are the key components:
Variables and Data Types
In Java, a variable is a container for storing data values. Each variable must be declared with a specific data type such as int, double, or String. These data types define the kind of data a variable can hold.
Example of variable declaration:
Operators and Expressions
Java uses various operators to perform operations on variables and values. These include:
- Arithmetic Operators: +, -, *, /, %
- Comparison Operators: ==, !=, >,
- Logical Operators: &&, ||, !
Operators can be combined to form expressions, which evaluate to a single value. For instance,
Control Structures
Control structures dictate the flow of a program. Essential control structures in Java include:
- If-else statements: For conditional execution.
- Switch statements: For executing one block of code from multiple options.
- Loops: Such as "for", "while", and "do-while" to execute a block of code multiple times.
Advanced Topics
Once the basics are mastered, moving on to advanced topics is essential for enhancing Java skills.
Functions and Methods
Functions, also known as methods in Java, enable a programmer to write reusable code. Defining a method consists of specifying a return type, a name, and parameters.
Example:
Object-Oriented Programming
Java is built on principles of object-oriented programming (OOP). Concepts like inheritance, encapsulation, and polymorphism are crucial in designing robust applications. In OOP, classes serve as blueprints for creating objects, enabling better organization of code.
Exception Handling
Exception handling is vital for creating resilient applications. Java employs try-catch blocks to handle exceptions gracefully, ensuring that the program can recover from unexpected errors without crashing.
Example:
Hands-On Examples
Implementing knowledge through hands-on practice is essential.
Simple Programs
Starting with simple programs can help solidify understanding. Programs like "Hello World" or basic calculators serve as effective introductions to coding.
Intermediate Projects
As skills develop, intermediate projects that encompass multiple programming concepts can be tackled. This might include creating command-line applications or simple games.
Code Snippets
Using code snippets is a useful way to see how functions and structures work. For instance:
Resources and Further Learning
Continuous learning is pivotal in programming.
Recommended Books and Tutorials
Books like "Effective Java" offer in-depth insights into best practices and advanced techniques. Online tutorials provide step-by-step guides on a variety of topics.
Online Courses and Platforms
Platforms like Coursera and Udemy host numerous Java courses catering to all skill levels. Enroll in courses that best fit personal learning objectives.
Community Forums and Groups
Joining communities on platforms like Reddit and Facebook can foster learning through discussions and support. Engaging with fellow learners can provide different perspectives on problem-solving.
By exploring these sections, readers can gain a solid foundation in Java. Following the principles and examples provided enhances programming skills effectively. A thorough understanding of Java allows learners to take on more complex challenges and explore the vast landscape of software development.
Preamble to Java
Java stands as a cornerstone in the field of programming languages. Its prominence stems from its versatility and the wide range of applications it supports. The introduction of Java is pivotal for any aspiring programmer because it lays a solid foundation for understanding not just the language itself, but also broader programming concepts. By engaging with Java, learners are positioned to grasp essential topics ranging from basic constructs, data types, and control structures to advanced object-oriented principles.
The significance of this topic goes beyond language syntax. Java is known for its platform independence, making it suitable for various environments including web, mobile, and enterprise applications. Furthermore, its ease of learning and robust community support enhance its appeal to beginners and intermediates alike. Java's consistent design and syntax as derived from C and C++ give a familiarity that can smooth the transition for those already exposed to programming.
Java Overview
Java was initially developed by Sun Microsystems and released in 1995. It is an object-oriented programming language that is particularly known for its ability to allow developers to create applications that are platform-independent. This unique feature is broadly termed as "write once, run anywhere," meaning that Java code can be executed on any device that has a compatible Java Virtual Machine (JVM). This trait makes Java an attractive option for developing cross-platform applications.
Additionally, Java enhances security through its robust runtime environment and comes equipped with a variety of libraries and frameworks that streamline development processes. Its structured approach fosters cleanliness in code, which not only aids in debugging but also in long-term maintenance and enhancement of software projects. The enterprise usage of Java, especially with tools like Spring and Hibernate, further cements its place as a dominant force in the software development landscape.
Features of Java
Java possesses several key features that distinguish it from other languages:
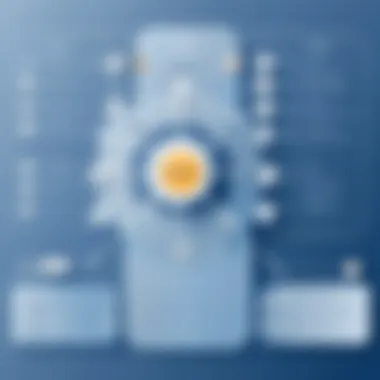
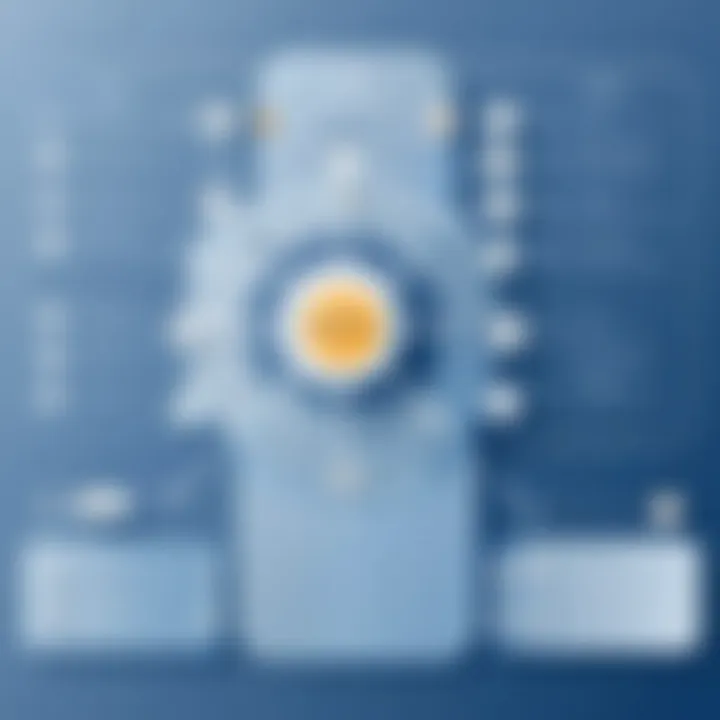
- Object-Oriented: Everything in Java is an object, which means it is built around data rather than functions. This promotes code reusability and maintenance.
- Platform Independence: As mentioned, Java's ability to run on any platform due to the JVM makes it extremely flexible.
- Robust and Secure: Java’s strong type-checking mechanism, garbage collection, and exception handling create a robust code environment. Its security features allow for developing secure applications.
- Multithreading Capability: Java can handle multiple tasks simultaneously. This is particularly useful for high-performance applications and those requiring real-time processing.
- Rich API: The extensive application programming interfaces (APIs) provide developers with tools to tackle almost any challenge, from database connections to networking and beyond.
By understanding these features, programmers can appreciate the competencies of Java and can utilize them effectively in application development. As we delve further into the article, these principles will serve as the bedrock for more specific examples and advanced topics in Java programming.
Setting Up the Java Environment
Setting up the Java environment is crucial for any developer aiming to write and run Java applications effectively. This process involves the installation of the necessary software components and configuring them correctly. Proper setup not only aids in efficient development but also minimizes potential issues down the line. Having a correctly configured environment can streamline coding, testing, and debugging.
Installing Java Development Kit
The Java Development Kit (JDK) is essential for any Java programmer. It contains the tools required to develop Java applications, including the Java Runtime Environment (JRE), an interpreter, and a compiler. The installation process of the JDK can be straightforward.
- Download the JDK: Visit the official Oracle website or another trusted source to download the JDK suitable for your operating system. Ensure you select the correct version.
- Run the Installer: Open the downloaded file to start the installation wizard. Follow the prompts. It is advisable to take default settings if you are unsure.
- Set the Environment Variables: After installation, configure environment variables. This step is critical for your system to recognize Java commands.
- For Windows, navigate to System Properties > Environment Variables. Under System Variables, add a new entry for pointing to your JDK installation directory.
- On macOS and Linux, add to your shell configuration file such as or .
This process ensures that the Java compiler and other tools are accessible from the command line, enhancing your ability to work with Java efficiently.
Configuring the IDE
An Integrated Development Environment (IDE) significantly boosts productivity while coding in Java. Popular choices include IntelliJ IDEA, Eclipse, and NetBeans. Each IDE has its own strengths, and the choice may depend on personal preference or project requirements.
- Choose your IDE: Select an IDE that you feel comfortable using. For beginners, Eclipse and IntelliJ IDEA are often recommended.
- Download and Install: Download the chosen IDE from its official site and run the installer. This will guide you through the setup process.
- Setting up your workspace: Launch the IDE and configure your workspace settings. Set up project folders and default settings according to your preferences.
- Linking the JDK: It is important to point your IDE to the JDK installation. In most IDEs, you can find this option under project settings. Make sure to select the JDK version you installed earlier.
An adequately configured IDE can enhance code suggestions, error detection, and debugging capabilities, greatly benefitting your development experience.
"The right tools can transform how you code and achieve your goals."
Java Basic Syntax
Java Basic Syntax is essential to understanding the programming language. A solid grasp of syntax forms the foundation for all programming concepts that follow. Java syntax governs how to write statements, define variables, and structure programs. It dictates everything from naming conventions to how operations are executed. Learning these fundamentals is crucial to avoiding errors and enabling effective troubleshooting.
There are several specific elements within Java syntax that every programmer should become familiar with:
- Comments: These are essential for understanding code. Comments in Java consist of single-line () and multi-line () formats. They help explain what the code does.
- Identifiers: Java requires naming conventions for variables, methods, and classes. Identifiers should be meaningful and follow rules such as no starting with a digit and no special characters, except underscores.
- Keywords: These are reserved words in Java, like , , and . Using keywords correctly is vital because they control the flow and operations of your code.
- Operators: Java has a variety of operators, such as arithmetic, relational, and logical. Operators allow programmers to perform operations on variables and values.
Mastering Java basic syntax leads to better programming skills and more readable code. It creates a blueprint for how to write and organize programs, enhancing collaboration among developers and the ease of revisiting code later on.
Structure of a Java Program
Understanding the structure of a Java program is paramount. Every Java program follows a consistent layout, which simplifies coding and debugging. A basic Java program structure typically includes:
- Package Declaration: This begins with the keyword .
- Import Statements: Used to include classes from other packages.
- Class Definition: This contains the program code and is defined using the keyword.
- Main Method: This is the entry point of any Java application.
Each of these components serves a distinct purpose and forms the framework necessary to create functional applications.
Data Types and Variables
Data types play a critical role in Java programming. They define the kind of data you can store and manipulate. Java is a statically typed language, meaning the type of a variable must be declared before it can be used. Common data types include:
- Primitives: Includes , , , , etc. They represent simple values.
- Non-Primitives: These include , , and . Non-primitive types can hold complex data structures.
Variables, on the other hand, are containers that hold data values. Defining a variable involves specifying the data type followed by the identifier. For example:
When selecting data types, consider memory requirements and the nature of the data being handled. Using appropriate data types enhances the efficiency of the Java application and improves performance.
Control Flow in Java
Control flow refers to the order in which individual statements, instructions, or function calls are executed or evaluated in a programming language. In Java, control flow is essential as it determines how different pieces of a program interact and respond to conditions or repetitions. This section explains two main categories of control flow: conditional statements and loops. Understanding these constructs is vital for making decisions in your code and handling repetitive tasks efficiently.
Conditional Statements
Conditional statements allow the program to execute certain sections of code based on specific conditions being true or false. In Java, the most common conditional statements include if-else statements and switch case statements. Both these structures guide the flow of execution, granting control over how data is processed.
if-else Statements
The if-else statement is one of the most fundamental programming structures. It enables decision-making by evaluating boolean expressions. If the condition is true, one block of code runs; otherwise, another runs. This two-path choice is crucial for many applications.
One key characteristic of the if-else statement is its versatility. Developers can nest multiple conditions inside, allowing complex logic to unfold. This feature makes it popular in scenarios where multiple branches of logic are needed. However, the drawback is that deeply nested conditions can make the code harder to read and maintain.
The following is a simple code example:
Switch Case
The switch case statement presents an alternative to if-else for handling multiple discrete values. This structure is notably effective when dealing with multiple cases, enhancing clarity. Each case can act on various possible values of a variable.
The key characteristic of the switch case is that it often simplifies the flow of commands. When comparing several constants against a variable, switch can be more readable than multiple if-else statements. However, one limitation is its inability to evaluate complex expressions, which makes it less flexible in some contexts.
A simple switch case example is as follows:
Loops in Java
Loops are another vital aspect of control flow, allowing repetitive execution of code blocks. Java provides three main types of loops: for loop, while loop, and do-while loop. Each loop has its unique features and benefits, suited for different tasks.
for Loop
The for loop is widely used for iterations, particularly when the number of iterations is known in advance. A classic scenario is iterating through array elements.
A key characteristic of the for loop is its compactness, combining initialization, condition-checking, and iteration in one line. This characteristic makes it a popular choice for both beginners and seasoned developers. However, it might seem less intuitive for new programmers who may struggle to understand its structure.
Here is a basic example:
while Loop
The while loop is more flexible in terms of condition evaluation. The code block inside will keep executing as long as the condition remains true. It is well suited for scenarios where the number of iterations is not predetermined.
A key characteristic is that it examines the condition every time before executing the loop body. This allows for dynamic behavior but can cause problems if not managed carefully, leading to infinite loops.
Example of a while loop:
do-while Loop
The do-while loop is similar to the while loop but guarantees that the code block executes at least once. This is useful when the initial execution is needed before any condition check.
The key characteristic of do-while is its structure, which checks the condition after execution. It can occasionally lead to confusion about the flow if not documented well.
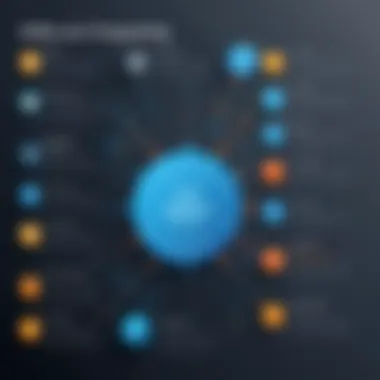
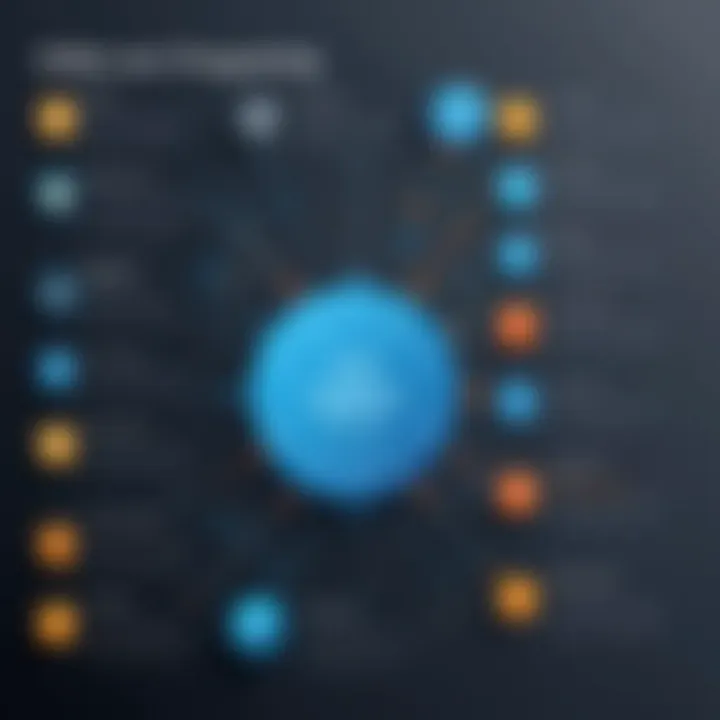
An example of a do-while loop:
Methods in Java
Methods are a fundamental building block in Java programming. They encapsulate functionality, allowing for code reuse and organization. By defining methods, programmers can break complex problems into manageable pieces, making the code easier to understand and maintain. Methods help in reducing redundancy, since common tasks can be written once and reused multiple times throughout the codebase. This section will delve into a critical aspect of Java programming that is essential for both beginners and experienced developers: how to define methods and the concept of method overloading.
Defining Methods
In Java, a method is a block of code that performs a specific task. Every method has a name, a return type, and parameters (if necessary). The basic syntax for defining a method is as follows:
Here are key components:
- Return Type: It specifies the type of value the method should return. If it does not return a value, the return type is .
- Method Name: This should be a meaningful identifier that describes the task the method performs. Following Java naming conventions, method names should typically start with a lowercase letter.
- Parameters: These are input values passed to the method. You can define multiple parameters, separated by commas.
For example, here's a simple method that adds two integers:
This method takes two integer parameters and returns their sum. Defining methods like this promotes modularity and enhances code readability.
Method Overloading
Method overloading is a powerful feature in Java that allows multiple methods to have the same name but with different parameters. This is particularly useful when the same operation needs to handle different types or numbers of inputs. It improves code readability and allows developers to define more intuitive method names.
To overload a method, you simply create multiple versions of the method with the same name but different parameter lists. Here’s a simple example:
In this example, the method is defined three times with varying parameters—two integers, two doubles, and three integers. The Java compiler determines which method to invoke based on the arguments passed during the call. This ability to redefine methods enhances flexibility and facilitates better programming practices.
Method overloading exemplifies the concept of polymorphism and is a vital tool for Java developers, enabling cleaner and more manageable code.
In summary, methods and method overloading in Java are not just about writing code. They are about writing efficient, readable, and maintainable code. Understanding these concepts is crucial for anyone looking to improve their programming skills in Java.
Object-Oriented Programming Concepts
Object-oriented programming (OOP) is a foundational aspect of Java that enables programmers to create modular and reusable code. Understanding OOP concepts is crucial for anyone learning Java, as it allows for more efficient code management and promotes a clearer structure in program development. The key elements of OOP include classes, objects, inheritance, polymorphism, and encapsulation. Each of these components plays a specific role in enhancing software development practices, leading to improved code maintenance and scalability.
Classes and Objects
In Java, a class serves as a blueprint for creating objects. A class defines the properties (attributes) and behaviors (methods) that the objects created from it will have. An object, on the other hand, is an instance of a class. For example, consider a class named . This class may have attributes like , , and , and methods like , , and . To create a specific car, one would instantiate the class:
This declaration creates as an object of the class, allowing the programmer to access its properties and methods easily. Understanding classes and objects is vital because it establishes the groundwork for OOP practices that promote organized coding.
Inheritance
Inheritance is a mechanism that allows one class to inherit properties and methods from another class. This feature enables code reusability and establishes a hierarchical relationship between classes. For instance, if there is a class called , a subclass called can inherit from it. The class will then have access to all methods and attributes defined in the class, along with the ability to add new features or override existing ones. This can be illustrated as follows:
Inheritance reduces code duplication and enhances maintainability while establishing clear classifications within the software's architecture.
Polymorphism
Polymorphism allows methods to do different things based on the object invoking them. It provides a way for a single interface to represent different underlying forms (data types). In Java, polymorphism can be achieved through method overriding and method overloading. Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. Conversely, method overloading allows multiple methods to have the same name but different parameter lists within the same class. This concept increases program flexibility and can simplify code readability.
Encapsulation
Encapsulation is the practice of restricting access to the internal state of an object and exposing only necessary functionality through public methods. This is often achieved by using private and public access modifiers. For example:
Here, the attribute is private and cannot be accessed directly from outside the class. The methods and provide controlled access to the , promoting data integrity. Encapsulation helps in maintaining the internal state of an object more securely, preventing unintended interference and misuse.
OOP principles like inheritance, polymorphism, and encapsulation are essential for developing software that is both scalable and maintainable.
Understanding these concepts thoroughly prepares learners for advanced topics in Java and enhances their programming prowess. Having a firm grasp of OOP principles is not just about knowing how to code; it is also about carrying out effective software design.
Java Exception Handling
Java Exception Handling is a crucial topic in Java programming. It addresses how a Java application deals with unexpected conditions that may arise during execution. Understanding exceptions is essential as it directly affects the way software operates. Proper exception handling enhances program robustness and reliability. It ensures that a program can gracefully handle errors without crashing, providing a better user experience.
When an exception occurs, the normal flow of execution disrupts. If not managed correctly, this can lead to unpredictable behavior or application failure. Java’s built-in mechanisms for handling exceptions, such as try-catch blocks, allow developers to anticipate problems and implement solutions. This practice not only minimizes downtime but also makes debugging easier. Therefore, mastering Java exception handling is a valuable skill for any programmer.
Understanding Exceptions
Exceptions in Java are events that disrupt the normal execution of a program. They may arise from various situations, including:
- Invalid user input
- Network connectivity issues
- File not found errors
- Arithmetic errors, such as division by zero
There are two primary categories of exceptions in Java:
- Checked Exceptions: These are exceptions that the compiler checks at compile-time. Examples include FileNotFoundException and IOException. Developers are forced to handle these exceptions with either try-catch blocks or by declaring them in the method signature.
- Unchecked Exceptions: These are not checked at compile-time. They include runtime exceptions like NullPointerException and ArrayIndexOutOfBoundsException. While they can be caught, they do not need to be, as they generally indicate programming errors.
Understanding these types of exceptions is essential for code reliability. Being prepared to manage both checked and unchecked exceptions can save significant time during debugging and enhance software stability.
Try-Catch Blocks
A fundamental aspect of Java exception handling is the use of try-catch blocks. They provide a mechanism for catching exceptions and executing specific code in response to those exceptions. Here’s a breakdown of how try-catch blocks work:
- Try Block: Code that may throw an exception is placed within a try block. If an exception occurs, control transfers to the catch block.
- Catch Block: This block handles the exception thrown by the try block. It specifies the type of exception it can handle.
- Finally Block (optional): This is an optional block that follows catch blocks and executes regardless of whether an exception occurred or not. It is often used for cleanup activities like closing file streams.
Here is an example of a try-catch block:
In this code snippet, the division by zero will trigger the ArithmeticException, which will be caught and handled without terminating the program. The finally block will execute regardless of whether an exception was caught. This level of control is what makes try-catch blocks an integral part of Java programming.
Java Collections Framework
The Java Collections Framework is a vital component in Java programming, serving as a unified architecture for managing groups of objects. As Java developers, it is crucial to understand collections because they provide essential data structures for storing and manipulating data efficiently. By leveraging the Collections Framework, programmers can enhance application performance, simplify code complexity, and improve maintainability. Moreover, the framework offers robust methods for handling collections of data, which are essential in various programming scenarios.
Preface to Collections
Collections in Java are objects that hold references to other objects. They can hold multiple elements in a single variable. This makes collections flexible and powerful for organizing data. The core collections framework provides interfaces such as List, Set, and Map among others, ensuring that developers can select the appropriate collection type based on specific needs. By choosing the right collection, unnecessary overhead can be minimized, leading to better performance in applications.
Common Collection Types
List
A List in Java is an ordered collection that allows for duplicates. The most commonly used List implementations are ArrayList and LinkedList. Lists support positional access and can be modified dynamically. This makes them ideal for scenarios where a sequence of elements is needed. One key characteristic of Lists is their ability to maintain insertion order, which can be crucial in applications where the sequence of operations matters. ArrayLists offer fast random access, while LinkedLists provide better performance for insertions and deletions.
Advantages of using Lists include easy element insertion and retrieval, but they also come with some disadvantages. For example, ArrayLists can have slower performance if elements are constantly inserted or removed from the middle of the list.
Set
A Set is a collection that cannot contain duplicate elements. This property makes Sets particularly useful when uniqueness is a requirement. The most notable Set implementations are HashSet and TreeSet. HashSet is known for its constant time performance for basic operations, making it a popular choice in many scenarios where the order of elements is not important. On the other hand, TreeSet maintains a sorted order but typically has slower performance due to its tree structure.
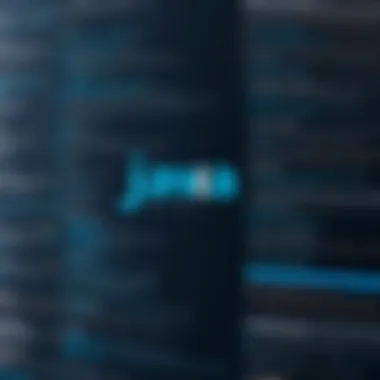
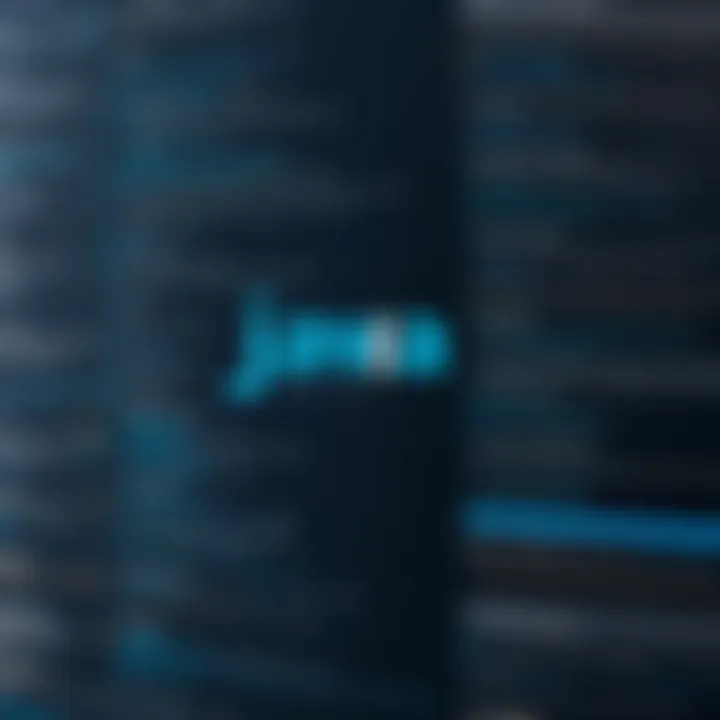
The uniqueness of elements in a Set can simplify logic in applications, reducing the necessity for manual checks for duplicates. However, the overhead associated with the implementation can impact performance in certain use cases.
Map
Maps are collections that store key-value pairs. Each key is unique, and each key maps to exactly one value. This structure is advantageous for lookup operations. The primary Map implementations are HashMap and TreeMap. HashMap provides fast access and is not ordered, while TreeMap maintains a sorted order based on the key.
Using Maps allows for efficient retrieval of values based on keys, making it suitable for scenarios such as caching and associative data. While they provide significant advantages for data retrieval, they may require additional memory overhead, which is a consideration in performance-sensitive applications.
In summary, understanding the different types of collections in the Java Collections Framework is essential for effective programming. Each type offers unique advantages and suitable use cases that can enhance overall application performance.
By grasping these core concepts, developers can make informed decisions that lead to optimized, maintainable code.
File Handling in Java
File handling is a crucial aspect of programming, enabling communication with the file system on a computer. In this section, we will discuss its significance, particularly in the context of Java programming. Being able to read from and write to files is essential for many applications. It allows developers to store data persistently, hence making it viable across sessions. As applications often require data storage, understanding file handling provides users with a practical skill set.
Several benefits come with mastering file handling in Java. For instance,
- It permits the development of applications that can handle user-generated data.
- It enhances a program's capability to manage large volumes of information, which is not feasible to retain in memory.
- It allows interaction with external systems, significantly when data exchange is involved.
However, developers must consider certain elements when managing files. Error handling becomes necessary when dealing with file operations because files might be missing or inaccessible. Additionally, understanding different file types and formats is essential to manipulate data appropriately.
Reading Files
Reading files in Java involves accessing data stored in files and importing it into the application for processing. The class is commonly used for text files, while the class is required for binary files. Here’s a basic approach for reading a text file:
In this example, a file named "example.txt" is opened, and its contents are read line by line. The use of improves performance by providing a buffer to read larger files efficiently. Proper error handling is also demonstrated, as potential exceptions must be caught to avoid program crashes.
Writing to Files
Writing data to files is equally important for storing output generated by applications. The class comes into play when you want to write character files, while is for binary files. Here’s a simple example of writing to a text file:
In this code snippet, a new file named "output.txt" is created, and text is written to it. Closing the is crucial to ensure that all data is flushed from the buffer to the file. Without closing, some data might not be written, resulting in data loss.
File handling in Java ensures that user data can be stored and retrieved effectively, forming the backbone of many software applications. Understanding these basic operations can greatly enhance a developer's ability to handle data and build more robust Java applications.
Basic Java Code Examples
In any programming journey, having practical code examples is essential. It helps new learners apply theory to actual practice. Basic Java code examples provide a window into the core principles of Java programming. Understanding these examples is a stepping stone for grasping more complex concepts later. You will see how small snippets can demonstrate significant programming concepts clearly.
Hello World Example
The "Hello World" program is often the first program written by anyone learning a new programming language. It is simple but powerful, teaching the essential syntax and structure of Java. The code for this example looks like this:
In this code:
- public class HelloWorld defines a class named .
- public static void main(String[] args) is the main method, where the program starts.
- System.out.println("Hello, World!") outputs the text to the console.
This example illustrates the foundational elements of a Java program. It combines the definition of a class, a main method, and output operations in a concise way. Even for experienced programmers, this example serves as a reminder of simplicity in coding.
Basic Calculator Program
Creating a basic calculator is a practical way to apply Java's arithmetic operations. The calculator can perform addition, subtraction, multiplication, and division. Here is a simple example:
This code:
- Imports the class for user input.
- Asks the user for two numbers and an operation.
- Uses a statement to perform different operations based on user choice.
- Outputs the result.
Such examples are not just exercises. They represent the practical application of Java’s control structures, user input handling, and arithmetic operations.
Simple Banking System
Another compelling example is a simple banking system. This system can illustrate storing user information and performing transactions. Below is basic functionality without intricate detail:
In this example:
- A stores account holders and their balances.
- It allows a user to check balance and deposit money.
- Basic input and conditional checks make it user-friendly.
This example allows learners to comprehend concepts of data structures, conditionals, and user interactions in Java programming.
Overall, basic Java code examples serve as invaluable tools for learners. They encapsulate the essence of how programming works, serving both educational purposes and enhancing problem-solving skills.
Debugging Java Code
Debugging is an essential part of the software development process, particularly in Java programming. It involves identifying and resolving errors or bugs in code. Effective debugging can significantly improve code quality and reliability. Readers should understand that even the most seasoned programmers encounter bugs. Learning to debug is critical for every programmer, whether they are beginners or more experienced.
Debugging helps not only in fixing errors but also in understanding the code flow. It allows programmers to see how their code executes and to identify areas for optimization. Additionally, debugging reinforces best coding practices, encouraging developers to write cleaner and more maintainable code. When done right, debugging can enhance a developer's problem-solving skills, leading to a deeper understanding of programming concepts. This section will go into common techniques for debugging and the use of debugging tools.
Common Debugging Techniques
There are several techniques that programmers can use to debug their Java code effectively. Each method has its strengths and weaknesses, and the choice often depends on the programmer's familiarity and the specific issues being faced.
- Print Statements: One of the simplest forms of debugging is using print statements. Inserting throughout the code helps track variable values and program flow. However, excessive print statements can clutter code and complicate readability.
- Code Review: Sharing code with a peer or mentor can uncover hidden errors. Fresh eyes often catch mistakes the original coder may overlook. Code reviews also encourage collaboration and learning.
- Rubber Duck Debugging: Explaining the code to an inanimate object, like a rubber duck, can help verbalize thought processes. This technique may trigger realizations about mistakes that were not previously noticed.
- Unit Tests: Writing unit tests allows for testing small pieces of functionality independently. If a test fails, it identifies the section of code that may have an issue. This method promotes writing testable and modular code.
- Logging: Instead of print statements, using a logging framework can help monitor application behavior in production environments. It gives detailed insights without cluttering the standard output.
Using Debuggers
Java Integrated Development Environments (IDEs) like Eclipse and IntelliJ IDEA come equipped with powerful debugging tools. These tools provide a way to set breakpoints and inspect variable values while the program is running.
- Setting Breakpoints: A breakpoint pauses the execution of the program at a specific line, allowing programmers to inspect the state of the application before the code continues. This is particularly useful for identifying the moment when an error occurs.
- Step Through Code: Debuggers enable developers to step through their code line by line. This helps in tracking the program's execution path and pinpointing exactly where issues arise.
- Variable Inspection: During a debugging session, developers can inspect the current state of variables, which helps understand their values at various points in execution. This assists in identifying unexpected changes that may cause bugs.
- Exception Breakpoints: Breakpoints can also be set to trigger on exceptions. This allows developers to investigate what causes an exception and how it affects program flow.
Debugging is not just about fixing bugs; it is about understanding code behavior and improving programming skills.
Arming oneself with these techniques and tools allows for effective debugging in Java. The goal should be to foster a mindset of debugging as a continuous learning process, ultimately leading to better programming proficiency.
Finale
The conclusion serves as a critical element in understanding the broader purpose of this article on Java code examples. It encapsulates the key takeaways and synthesizes the core concepts presented throughout the sections. Understanding Java is not just about grasping syntax or writing lines of code; it is about developing a mindset that creatives problem-solving skills using programming.
In this article, we discussed various fundamental topics of the Java programming language, ranging from basic syntax to object-oriented principles. Each section contributed to building a comprehensive understanding of programming constructs. More importantly, newcomers to Java can see how these principles interconnect, thus facilitating a more holistic learning path.
The use of engaging code examples highlights real-world applications of Java, making it easier for learners to relate to abstract concepts. With practical coding scenarios, the challenges of programming feel more achievable. Consequently, learners gain confidence, which is essential in a subject often seen as daunting.
Regular practice and engagement with such examples encourages retention of knowledge, paving the way for advanced studies in Java. Whether one aims for application development, systems programming or Android development, the foundational concepts discussed here will undoubtedly serve as building blocks.
Summary of Java Essentials
The essentials of Java programming revolve around understanding core principles such as syntax, control flow, and object-oriented programming. Key elements include the following:
- Core Syntax: Learning the basic structure of a Java program is fundamental for creating functional applications.
- Data Types and Variables: Grasping how to choose and manipulate different data types is crucial for efficient coding.
- Control Structures: Mastery of conditional statements and loops is essential for controlling the flow of a program.
- Object-Oriented Concepts: Understanding classes, inheritance, and polymorphism lays the foundation for designing robust systems.
- Error Handling: Exception handling is pivotal in making applications reliable and error-free.
- Data Structures: Familiarity with Java Collections helps in organizing and interacting with groups of data efficiently.
These elements form the crux of programming in Java, serving as a toolkit for aspiring developers.
Next Steps in Java Learning
For learners ready to progress further, several routes can be taken:
- Advanced Java Topics: Explore frameworks like Spring or Hibernate to construct large-scale applications.
- Project-Based Learning: Engage in personal projects that challenge your current understanding and push your ability. Consider creating a web application or a game.
- Join Online Communities: Platforms such as Reddit or Stack Overflow can be valuable for community support and knowledge sharing.
- Consistent Practice: Websites like LeetCode or HackerRank offer coding challenges that can sharpen your skills.
- Formal Education: Consider enrolling in courses which delve deeper into Java or related technologies.
Continuous learning is vital in the ever-evolving tech landscape. Embracing these next steps will enhance your skills and prepare you for a successful career in programming.