In-Depth Exploration of Basic C# Code for All Levels
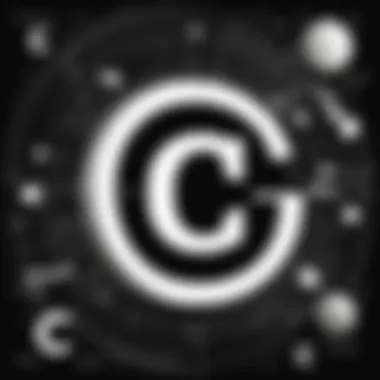
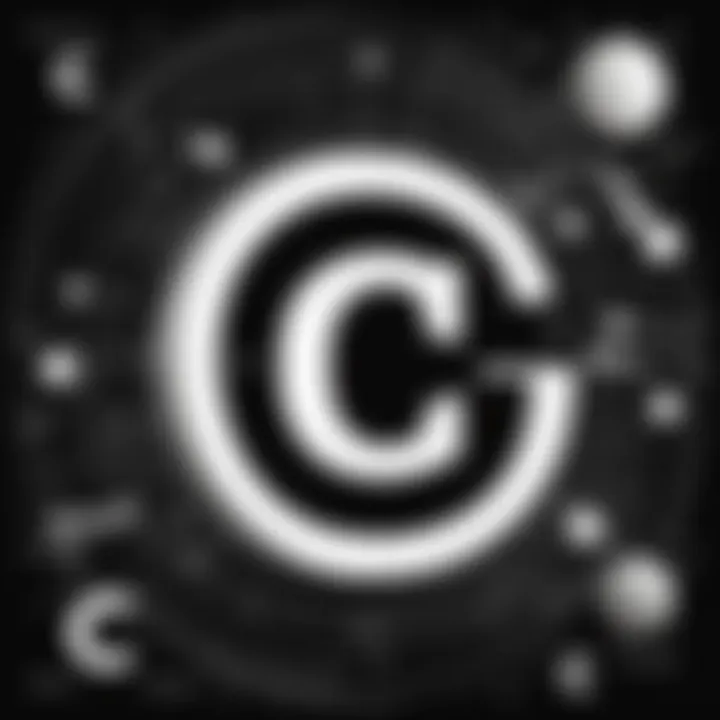
Intro
Preface to Programming Language
Programming languages form the backbone of software development. Among them, C# stands out for its versatility and ease of use. Initially developed by Microsoft, C arrived on the programming landscape in the early 2000s, fulfilling the need for a modern language suitable for building applications on the .NET framework.
History and Background
The inception of C# can be traced back to 2000, when Anders Hejlsberg, a key figure at Microsoft, aimed to simplify the complexities associated with other programming languages. Designed with a syntax similar to C and C++, its primary goal was to fill gaps left by these matured languages. Since then, C has evolved remarkably, often incorporating features that enhance programmer capabilities, ensuring robust application development.
Features and Uses
C# is valued for several characteristics that boost productivity:
- Type safety: This ensures fewer runtime errors, allowing developers to catch issues at compile-time.
- Management of memory: Automatic garbage collection helps to reduce memory leaks.
- Object-oriented nature: C# promotes code reuse and modularity, which creates maintainable codebases across various projects.
Common applications include desktop software, web apps with ASP.NET, game development using Unity, and mobile applications. This breadth makes C# an attractive choice for a diverse range of programming tasks.
Popularity and Scope
The appeal of C# is reflected in its usage by many organizations around the world. Available on multiple platforms now, thanks to initiatives like .NET Core, programming in C holds room for growth across diverse environments, from enterprise solutions to small-scale applications.
C# is often a choice for novice programmers who seek clarity while also holding potential for expert-level applications.
Basic Syntax and Concepts
Understanding the essential syntax and concepts is foundational for effective C# programming. Familiarity with these elements can facilitate smoother transitions into more complex material down the line.
Variables and Data Types
Variables are foundational elements in any programming language. In C#, variables are used to store data. They possess types, which define the kind of information they can hold. Some common data types include:
- (for integers)
- (for floating-point numbers)
- (for text)
Each variable name must adhere to certain naming rules, such as starting with a letter and avoiding spaces don't. Good variable naming practices can greatly enhance code readability.
Operators and Expressions
C# includes various operators for arithmetic and assignment. These operators are essential for manipulating data. Examples are:
- Arithmetic operators: , , , , which perform standard math calculations.
- Comparison operators: , , ``, for evaluating conditions.
At the fundamental level, an expression combines variables and operations to yield results. Mastery of these can aid in constructing functional programming logic.
Control Structures
Control structures dictate the flow of the program. They enable decision-making processes and loop iterations.
- Conditional statements include , , and to execute certain parts of code under specific conditions.
- Loops, like , , and , allow for repetitive execution until a condition is met.
These constructs are essential, encouraging logical reasoning and methodic processes in programming.
Advanced Topics
As constructors of modern applications navigate their challenges, other integral elements also emerge which are critical to writing effective code.
Functions and Methods
Functions, or methods, encapsulate tasks or operations. In C#, a simple function is laid out as:
Calling this function with an argument executes the block of code, which is conducive to organization and code reuse.
Object-Oriented Programming
C# heavily leans on object-oriented programming paradigms. The key concepts include:
- Encapsulation: Keeping related data and methods within a defined scope.
- Inheritance: Allowing new classes to inherit attributes and behaviors from existing ones.
- Polymorphism: Thousands hustling can later become equipped to utilize the same method with different objects.
Mastering these principles wil help when developing extensive applications while ensuring structural thoroughness.
Exception Handling
Exception handling is a significant topic aimed at managing errors and keeping solutions stable during runtime. The , , and keywords facilitate control when exceptions arise, allowing developers to handle unexpected failures efficiently without crashing the software.
Hands-On Examples
Novices will benefit greatly from practical examples. Starting with simple programs can build confidence before tackling more substantial projects. Various programs can demonstrate C# basics:
Simple Programs
A basic
Intro to
C# is a prominent programming language that stands at the center of modern development practices. As a versatile language created by Microsoft, it has carved its place within many technology stacks. Understanding C helps programmers harness the power of .NET for various applications, from web development to game programming. Its object-oriented approach and strong support for robust frameworks make C a favored choice among developers.
What is #?
C# is a powerful, multi-paradigm programming language that was designed to be simple and intuitive. It enables developers to create a range of software applications comfortably, thanks to its clean syntax. It is strongly typed, supports both imperative and declarative programming, and adheres to object-oriented methods. By offering safety and flexibility, C allows for efficient coding practices without sacrificing performance. Key elements also include automatic garbage collection which helps in managing memory safely. Here are some characteristics:
- Platform Independence: With the creation of .NET Core, C# applications can run on multiple platforms.
- Rich Standard Library: C# includes extensive built-in libraries bringing numerous utilities at developers' fingertips.
- Community Support: The active community around C# fosters sharing of knowledge and troubleshooting resources.
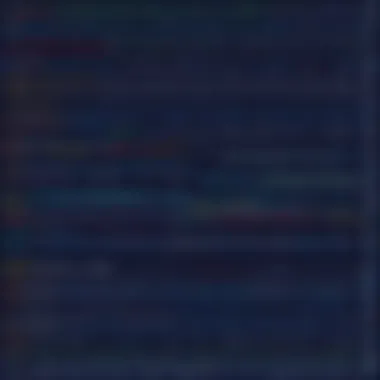
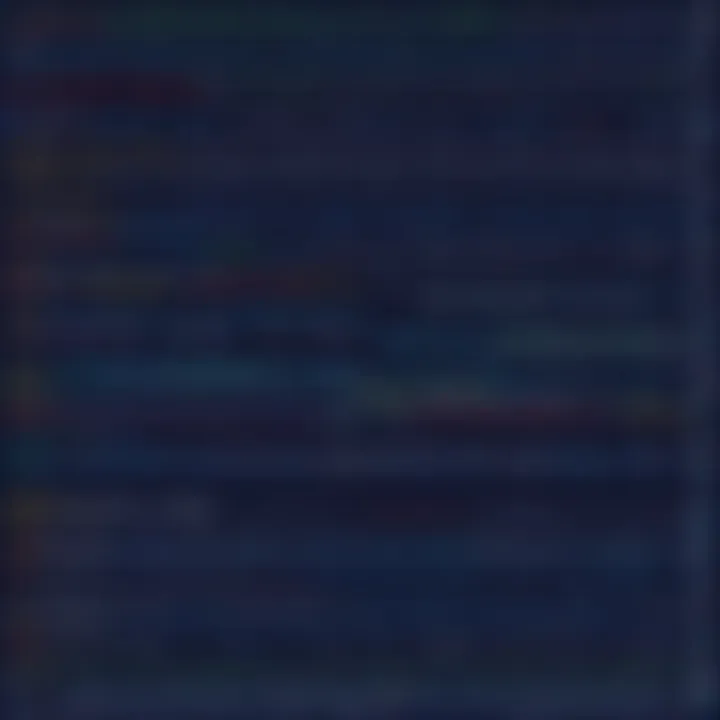
History and Evolution of
C# was developed in the early 2000s as part of the .NET framework initiative. The first version was released in 2002, driven by Anders Hejlsberg. It was designed to combine high productivity with high-performance capabilities. Over the years, C has evolved significantly, introducing new features in each version, such as LINQ for data manipulation, async programming capabilities, and more modern syntax enhancements.
Core changes evolved to meet growing development demands, and in phases, notable versions were rolled out:
- C# 1.0: Introduced in 2002; established the basics of the language.
- C# 2.0: Released with generics, enhancing the flexibility of C#.
- C# 3.0: Introduced features such as anonymous types and extension methods in 2007, improving data handling.
- C# 7.0 and Beyond: Maintained a focus on performance and usability, refining features like tuples and pattern matching.
These generations reflect C#'s adaptability and commitment to facilitating modern software development, constantly aligning with changes in technology standards and developer expectations. The evolutionary timeline demonstrates C#’s importance, as its evolution mirrors the overall progress and rising demands in the programming world.
C# has shown a remarkable capability to adapt and grow within an increasingly complex programming landscape.
Setting Up the Environment
Setting up the environment is a crucial first step when embarking on the journey of learning C# programming. A well-configured environment provides the tools and resources needed to write, test, and run C code effectively. It not only enhances programming efficiency but also minimizes frustration. By establishing a solid foundation, learners can focus more on coding and less on troubleshooting setup issues.
Installing Visual Studio
Visual Studio is the most popular Integrated Development Environment (IDE) for C# developers. It offers an extensive set of features that cater to both newcomers and seasoned developers. The installation process is straightforward, yet a few important considerations exist.
- Download: Start by visiting the official Microsoft website to download Visual Studio. Choose the Community version if you're a student, hobbyist, or learning as it is free.
- Installation Options: During installation, you will encounter different workloads. Selecting the .NET desktop development workload is essential for C#.
- Customization: Visual Studio allows customization of the interface and settings. Take time to configure these to fit your personal preferences, as efficient layouts can improve your coding experience.
Installing Visual Studio successfully prepares you for practical coding work.
Choosing the Right Version
Selecting the correct version of Visual Studio can dramatically impact your learning experience. Here are key factors to consider when choosing the right version:
- Community vs. Enterprise: The Community version is adequate for learning purposes. It offers core features needed for C# development without extra complexity.
- Updates and Support: Always consider the latest available version. New releases of Visual Studio include important security and feature updates that optimize the workflow and improve performance.
- Platform Compatibility: Understand whether you need Windows, macOS, or both, since only Windows fully supports the complete C# features in Visual Studio.
Choosing the right version will ensure a smooth and productive development environment that supports your growth as a C# programmer. In summary, setting up the environment requires attention to detail. A thoughtful installation and version choice can lay the foundation for successful learning and development.
Basic Syntax of
Basic syntax is the backbone of any programming language, and C# is no exception. A solid understanding of the syntax enables programmers to write clear, effective, and efficient code. This section parses essential elements pivotal in mastering C#. It embraces core concepts relevant to variables, operators, expressions, and control structures, complex features that provide order and logic to the programming tasks. It is not merely a set of rules; it embodies clarity and organization, which is crucial for developing robust applications. Furthermore, gaining a dual understanding of how these elements interact elevates one’s programming prowess.
Variables and Data Types
Variables are fundamental units of storage designed to hold data values. C# has strong typing, which dictates that every variable has a specific data type. Correctly declaring and using dimensions such as integers, strings, and arrays, allows efficient data manipulation. Understanding the standard data types is powerful as it dictates what kind of data can be stored and how operations can be performed on that data. This knowledge guards against errors during compilation and runtime.
Operators and Expressions
Operators in C# perform operations on variables and values. There are several types including arithmetic, relational, and logical operators. Each of these serves their shared purpose and enhances the expression via which operations are written. For example, arithmetic operators like , , or guide basic numerical processing. Logical operators allow creating complex truths, incorporating decision-making logic directly in the code. Knowing how to tactfully use these operators allows a programmer to create concise and powerful expressions that convey logic and decisions succinctly.
Control Structures
Control structures streamline the flow of execution depending on evaluations. We categorize these into two main elements: Conditional Statements and Loops.
Conditional Statements
Conditional statements are crucial because they control the application's logic pathway. They allow decisions based on defined criteria. Upon an analytical insight into the expression's boolean status, it navigates through blocks of code in a desired way. A fundamental structure is the statement; it checks a condition and allows variation in execution. This adaptability makes it a popular choice for code implementation, ultimately contributing to refined decision-making in applications. Conditional statements' primary strength lies in their ability to smartly select routes according to real-time inputs.
Loops
Loops provide functionality for repeated execution of blocks of code. A significant loop in C# is the loop, which can repeatedly execute a segment a defined number of times. This characteristic shrinks down programmer workload by automating repetitive actions, thus optimizing code efficiency. However, careful handling is necessary to avoid infinite loops that can freeze applications. Loops enable programmers to construct more robust applications through manageable and repetitive code with less human input.
Functions and Methods
Functions and methods play a crucial role in C#. They allow programmers to break down code into reusable sections. When you define functions, their visibility and reusability become important features that enhance overall code organization. This article covers how functions can streamline coding and foster better maintenance practices.
Defining Functions
Defining functions in C# is straightforward, yet it needs an understanding of several components. A function can be likened to a mini-program that accomplishes a specific task. The main parts of a function include:
- Return type: This indicates what type of data the function will return.
Object-Oriented Programming in
Object-oriented programming (OOP) is a core feature of C#. It provides a framework that facilitates the organization of code into manageable and modular components. This paradigm allows programmers to think in terms of real-world entities and how they relate to each other. The importance of OOP lies in its ability to improve code reusability, scalability, and maintainability.
In C#, OOP is tightly knitted with its features. The approach addresses significant considerations such as defining classes, creating objects, and managing relationships through polymorphism and inheritance. To understand why learning OOP is essential, let's hone in on three fundamental concepts - classes and objects, inheritance and polymorphism, encapsulation and abstraction.
Classes and Objects
At the heart of C# OOP are classes, which serve as blueprints for creating objects. A class encapsulates data for the object and methods to manipulate that data.
Here’s an example of a simple class definition:
In the example above, becomes a blueprint where you can define various attributes like , and encapsulate intrernal state with accessors. Each car can be created as an instance of the class, thus representing objects.
- Why are classes key?
- Likewise, objects signify instances of classes that interact in a defined manner, emulating how entities function in the real world. This alignment to real-life applications promotes both understandability and efficiency.
- They provide a structure to encapsulate related data and functionality.
- They enhance organization in code, facilitating easier debugging.
Inheritance and Polymorphism
Inheritance is a mechanism that allows one class to inherit properties and methods from another. This relationship promotes code reuse, as common functionality can be placed in a base class and shared among derived classes.
A simple inheritance example could look like this:
In this scenario, inherits from , allowing it to utilize the method while also containing its own specific behaviors. Here, polymorphism enables a superclass reference to point to a subclass object, allowing methods to behave differently based upon the type of object being referenced.
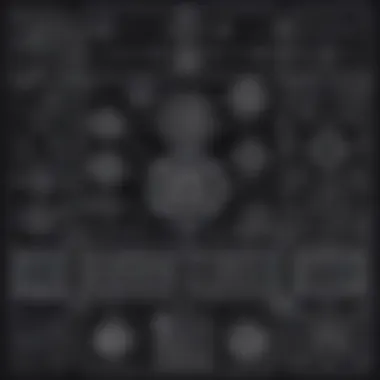
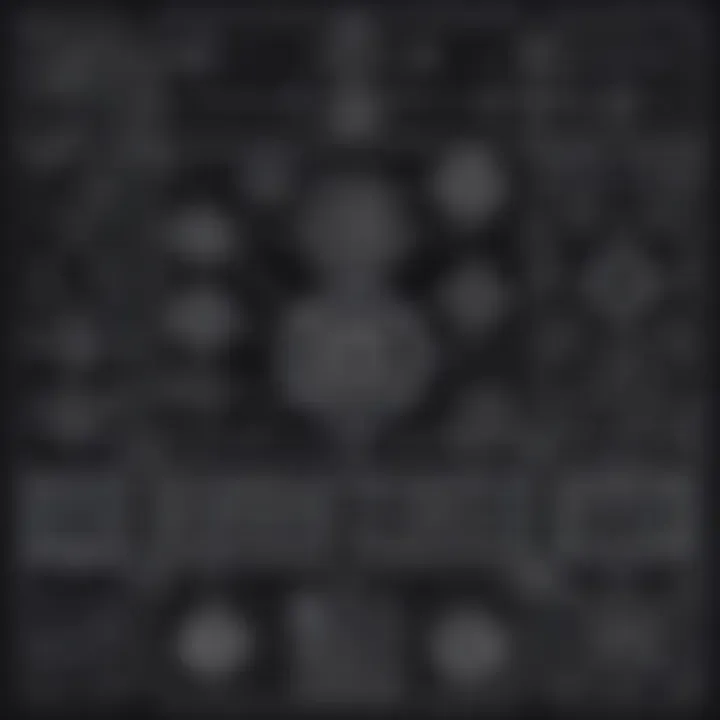
- The benefits of inheritance include:
- Reduced redundancy in code.
- Easier management of software as the complexity grows.
- Greater flexibility in extending functionality without altering existing code.
Encapsulation and Abstraction
Encapsulation refers to the bundling of data and methods that operate on that data within a single unit, which is a class. It also restricts access to some of the object's components, protecting an object's internal state. This shields it from unintended interference and misuse.
Consider the following encapsulation example:
With encapsulation, the internal cannot be accessed directly; it can only be modified through the method. This defines a clear interface for the user of the class.
On the other hand, abstraction is the concept of exposing only the essential features of a class while hiding the internal implementation. This reduces complexity and increases efficiency, allowing users to interact with classes at a high level, without needing to understand intricate details.
OOP is thus quer entscheidene uh monitoring legacy and helps engagedlarge systems into mote modular ones, making them scalable and easier to manage.
Considering these fundamental principles provides a solid foundation for extensive and broad understanding of C# programming as a whole. Delving into even deeper concentrations facilitates advance projects, where one can seamlessly integrate various OOP methodologies in practice.
Exception Handling
Exception handling is a crucial part of robust programming in C#. It allows developers to manage errors effectively without program crashes. Understanding exceptions and how to handle them can greatly improve the user experience and stability of an application.
An exception can occur at many points within a program. It may arise from unexpected input, system failures, or even bugs in the code itself. Handling these exceptions in a structured way helps maintain program flow and integrity. Without proper handling, a small error can lead to significant failures, affecting end-users. Thus, the ability to anticipate and manage these errors is vital for any developer.
Try-Catch Blocks
Try-catch blocks are the core mechanism for handling exceptions in C#. A try block allows you to test a piece of code for errors. If an error occurs, the code in the catch block executes. This separation of error-prone code and error handling is critical.
Structure of Try-Catch
The basic structure of a try-catch block looks like this:
In this structure, replace with the specific type of exception you want to handle. The catch block can access the exception object , providing information about what went wrong. It is common to have multiple catch blocks to handle different exception types separately.
For example, consider the following code:
In this snippet, is specifically caught, providing a tailored response for that error type. Having catch blocks allows for granular handling of various exceptions, making code cleaner and more efficient.
Throwing Exceptions
Throwing exceptions is another key aspect of exception handling. In C#, you can create your exceptions when you encounter a situation that justifies it. This informs other developers or users about an error condition that cannot be handled internally.
How to Throw Exceptions
You can throw exceptions using the statement. It indicates that a program has encountered an error. Here’s an example:
This line of code checks for a negative number and throws an exception if the condition is met. This provides clear feedback, and the code will end up in the relevant catch block, allowing for appropriate error management.
Following best practices in exception handling contributes to clean, understandable code. Thorough documentation of thrown exceptions can also guide future developers on how to manage potential errors efficiently.
Proper exception handling is essential; it exemplifies a developer’s intent to enhance the user experience while safeguarding against unexpected events.
In summary, the combination of try-catch blocks with effective exception throwing creates a powerful safety net in any C# application. Grasping and implementing exception handling principles leads to strong, enduring software design.
Working with Collections
Working with collections in C# is a fundamental aspect that requires attention. Collections allow the storage and manipulation of groups of objects in a single structure. This capability enhances efficiency and organization within code, making it more readable and manageable. The importance of collections lies in their adaptability and ability to cater to various programming needs. They allow developers to handle dynamic data and even perform complex operations more gracefully. Collections, are widely used in everyday programming, are a cornerstone of effective C development.
Arrays
An array in C# is a collection that contains a fixed number of elements of the same type. Arrays are useful when you know the size of data in advance and when the dataset usually does not change. They provide a way to group related items, making code simpler and cleaner.
To declare an array, you use the following syntax:
The above code creates an array named that can store five integer values. Assigning values to an array is crucial for accessing them later. You can do this in different ways:
Arrays support operations such as traversing, searching, and sorting. However, arrays have fixed size, meaning elements cannot be added or removed after creation. This can add limitations if the application requires flexibility for data size.
Lists and Dictionaries
Lists and dictionaries are more flexible collection types in C#. A provides a dynamic array-like structure. It allows adding or removing elements easily, making it suitable for most scenarios where size varies.
Creating a list follows this pattern:
In contrast, dictionaries enable storage of key-value pairs. This structure efficiently supports quick lookups, enhancing retrieval performance. For example, you can create a dictionary as follows:
You can access a value by its key:
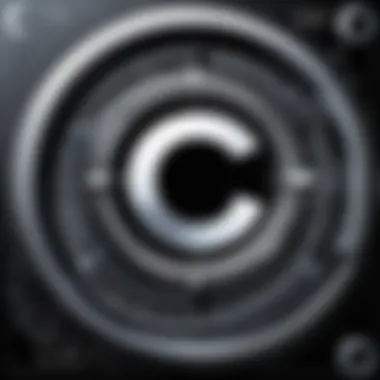
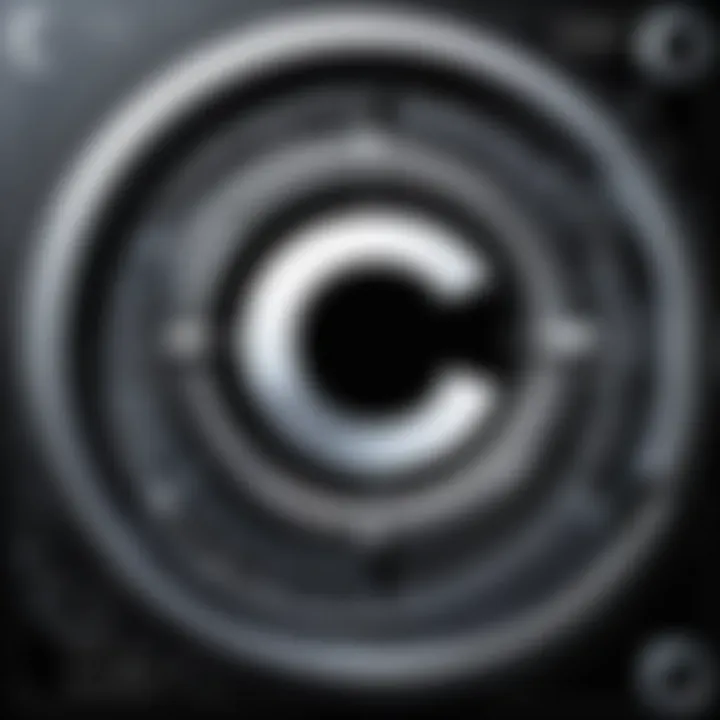
Using lists and dictionaries provides considerable advantages. They handle data dynamically, minimize overhead when retrieving, and aid in ensuring total control over their contents. In complex applications, these collections prove indispensable, significantly impacting the overall efficiency of C# code.
Working with collections brings advantages in terms of efficiency, organization, and flexibility in programming. Clear use of collections can lead to better structured and more maintainable code.
File Handling in
File to handle is a critical component in software development. It allows programs to read and write data efficiently, which is essential for most applications in various sectors. Learning how to manage files is not delight. C# excels on this front, providing simple yet powerful mechanisms for both file reading or writing. This section focuses on the core aspects of file handling in C#, importance, and basic methods to perform these operations.
Reading Files
Reading files in C# primarily involves opening a file for reading data from it. This is essential when an application needs to gather input, retrieve configurations, or analyze stored data. Understanding the appropriate techniques is crucial for beginners being a small mistake can lead to losing large amount of data.
To read a file, you can use classes from the namespace. One of the most common methods is . Its use is straightforward. First, you create an instance of this class by specifying the file path. Second, you can use methods like or to access data in the file.
Example of Reading a File:
Benefits of Reading Files in #:
- Flexibility: You can read text-based file types, making it possible to select a format that best suits your requirements.
- Error handling: C# supports exception management. You can anticipate potential issues while file handling situations, ensuring your application works correctly in various scenarios.
- Direct data access: You can efficiently manage large sets of data that may not fit in memory.
Writing Files
Writing files in C# involves the saving of data into a file system. This feature allows programs to store outcomes, export logs, or manage user settings. Knowing file writing options is one sign of becoming a proficient programmer. It also comes handy for handling user-generated data produced during application runtime.
The is a commonly used class for writing data into files. Similar to reading, it found in the namespace. It lets you create a file and write to it easily. Using is a frequent operation as well since it writes strings followed by a newline.
Example of Writing to a File:
Benefits of Writing Files in #:
- Persistent Data Storage: Storing important information allows development for work continuously over time.
- Users Interactions: Applications can collect user data, leading to enhanced user experiences.
- Data Management: You can efficiently back up, process, and distribute results according to business needs.
An effective handling of files is key to achieving robust and user-friendly applications in C#. As one explores these methods further, the aspects of how to read and write files dive deeper into efficient practices every adept programmer should adhere to.
Developing a Simple Application
Developing a simple application is a crucial section in learning C#. It combines theoretical knowledge and practical coding skills. This section allows learners to apply the concepts previously covered. When a student creates a simple application, they gain insight into how different components of the language fit together.
Importance of Simple Applications
A simple application serves various purposes in a learning environment:
- Practical Application: It reinforces theoretical concepts.
- Error Recognition: Learners can identify and fix mistakes.
- Building Confidence: Completing a project increases confidence in programming.
- Foundation for Complexity: Simple apps are stepping stones to more complex projects.
This topic focuses on practical implementations. Understanding how C# works in a real-world context is valuable. It prepares students for further challenges in programming and enhances problem-solving skills.
Creating a Console Application
Creating a console application is often the first step for many programmers in C#. This method involves using the command line interface (CLI) rather than graphical elements. The process highlights essential components of application development.
- Defining the Project: Use Visual Studio to set up a new project. Choose
Debugging Techniques
Debugging is an essential aspect of the development process in C#. It refers to the systematic approach of identifying and rectifying errors or bugs in the code. Knowing how to debug effectively can save an immense amount of time and frustration, particularly when working on complex projects. The importance of mastering debugging techniques cannot be overstated; it enhances coding efficiency and overall software quality.
Learning debugging skills provides numerous benefits:
- Improvement in Problem Solving Skills: Debugging hones analytical abilities and proves useful across various stages of development.
- Increased Code Quality: By systematically finding and correcting mistakes, developers produce cleaner, more functional code.
- Better Understanding of Code Execution: Debugging gives insights into how code operates, ultimately leading to a deeper comprehension of the programming language itself.
Moreover, debugging encourages programmers to adopt a mindset focused on precision and action. This section discusses two primary techniques: setting breakpoints and using the debugger tool to trace code execution.
Setting Breakpoints
Setting breakpoints is a fundamental part of finding issues in code. A breakpoint is a marker that you place on a specific line in the code. When the application runs, it halts execution at this line, allowing you to inspect the current state of the program. You can examine variables, the flow of execution, and any errors that arise at this point.
To set a breakpoint in Visual Studio, follow these steps:
- Open your code file in Visual Studio.
- Click in the margin next to the line of code where you want to pause execution.
- The breakpoint appears as a red dot.
Utilizing breakpoints has several practical advantages:
- Breif observations of variable content at crucial stages of execution.
- The ability to execute code step-by-step through commands like
Ending
The conclusion is a pivotal section of any comprehensive guide to C#. It encapsulates and reinforces the main themes presented throughout the article. Here, the reader should gain clarity on the various topics discussed, acknowledging how they fit into the larger framework of understanding C# programming.
Summary of Key Concepts
Within this section, it is crucial to reflect on the major concepts explored. These include the basic syntax encompassing variables, data types, and operators. Beyond that, the article covers crucial control structures, functions, methods, and object-oriented programming principles like classes, inheritance, and encapsulation. Each segment serves to strengthen the reader's foundation in C#.
Also noteworthy is the discussed exception handling methods, allowing developers to create more robust and error-tolerant applications. The insight into collections, such as lists and dictionaries, provides readers with methods to effectively store and manage data.
When a reader approaches this article's content, they should remember its intended progression—from understanding data types, creating objects, to file handling and debugging. Each section weaved together supports a greater comprehension of how C# functions as a programming language. Overarching all these details is the emphasis on practicing coding through practical application.
Through programming, we are not just learning a language but also critical thinking.
Next Steps in Learning
Moving forward from this conclusion segment, there are Encouraged actions for readers to progress further in their learning journey. An initial suggestion involves rigorous practice through coding exercises. These can be found on diverse platforms like Hackerrank and LeetCode which focus specifically on C# problems.
Additionally, engaging with the C# community on forums like reddit.com can provide support when facing challenges. Interaction with experienced developers can illuminate best coding practices, guiding better approaches.
Readers should consider deeper dives into advanced topics like asynchronous programming, design patterns, or cloud-based application development. Online courses can also be tremendously valuable. Websites, such as Codecademy or Coursera, offer structured C# programs to enhance understanding.
By understanding the outlined material and committing to further engagement with programming resources and communities, anyone can improve their C# skills effectively.