Application Code Examples: A Comprehensive Guide
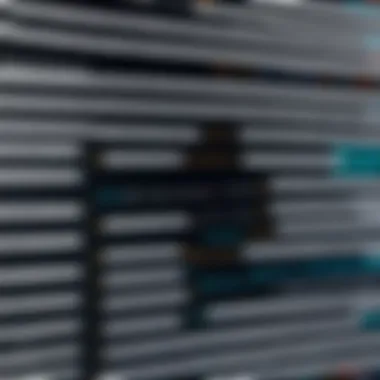
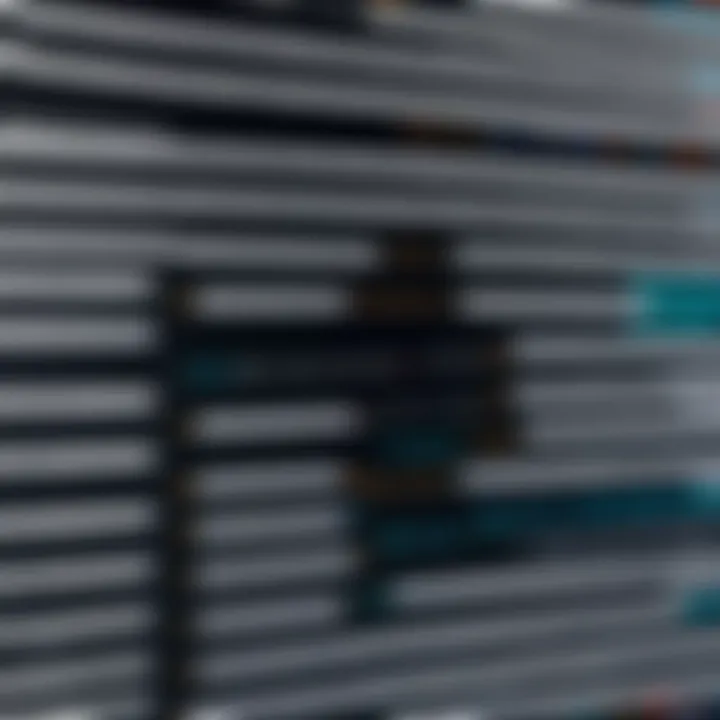
Intro
Understanding programming languages is essential for anyone embarking on the journey of coding. Such languages serve as the foundation for creating software applications, system tools, and even web platforms. Each language has its own historical context, unique features, and specific niches in which it excels. This section will provide an overview of these key aspects to ground your understanding of application code examples.
History and Background
Programming languages have evolved significantly since their inception. Early languages like FORTRAN and COBOL were developed in the 1950s, primarily targeting scientific and business needs. As technology advanced, new languages emerged, each designed to address specific shortcomings or enhance productivity. For example, C was created in the early 1970s and has laid the groundwork for many modern programming languages, such as C++ and Java.
Features and Uses
Different programming languages offer a variety of features that cater to specific tasks.
- Java is known for its portability and robust security features, making it ideal for large-scale enterprise applications.
- C provides low-level access to memory, which is vital in systems programming.
- C++ incorporates object-oriented concepts which allow for modular and reusable code.
These features help developers select the appropriate language for their particular projects.
Popularity and Scope
The popularity of programming languages can significantly influence a developer's choice. Languages such as Python have gained traction due to their ease of learning and versatility in applications like web development, data science, and automation. In contrast, languages like C# and Java continue to dominate enterprise environments. Popularity often correlates with community support, available libraries, and frameworks that enhance functionality. This creates a cycle where well-supported languages see increased adoption, reinforcing their status in the tech ecosystem.
"A strong community can often add tremendous value to a programming language, influencing its growth and future developments."
This understanding of programming languages is valuable as we transition into more specific syntax and concepts, which are pivotal for writing effective application code.
Next, we will explore the basic syntax and fundamental concepts that form the bedrock of coding proficiency.
Understanding Application Code Examples
Application code examples serve a fundamental role in the landscape of programming education. They provide practical illustrations of how code operates in real-world scenarios, thus bridging the gap between theory and application. Understanding these examples not only helps learners grasp core programming concepts but also aids in tackling more complex problems they may encounter in their coding journeys.
Defining Application Code Examples
Application code examples are snippets of code that demonstrate how to accomplish specific tasks or solve particular problems using programming languages. These examples can vary widely in complexity, from simple functions to intricate algorithms. They typically include key aspects such as:
- Syntax: Illustrating the specific structure of programming languages.
- Functionality: Showing how particular features or libraries can be utilized.
- Annotations: Often accompanied by comments that clarify purpose and function.
By reviewing various application code examples, learners can steadily develop their coding skills. It offers them insight into best practices and common coding patterns, which are crucial for effective programming.
Importance in Learning Programming
The significance of application code examples in learning programming cannot be accentuated enough. They serve several crucial purposes:
- Practical Understanding: They transform abstract programming concepts into tangible implementations.
- Problem-Solving Tools: Application code examples equip learners with the skills to address specific issues they may face while coding.
- Enhanced Confidence: By working through examples, learners cultivate the confidence needed to tackle more difficult challenges.
- Motivation for Learning: Real-world applications of code help in maintaining enthusiasm for learning, as students see their progress in action.
"Code examples not only teach how to write code but also propagate the thought process behind coding solutions."
In summary, the process of exploring application code examples establishes a foundation for programming knowledge, fostering both confidence and competence among learners. Through critical analysis and practice, students can develop their own approaches to coding, which will serve them throughout their programming careers.
Structure of Application Code Examples
The structure of application code examples plays a critical role in understanding how programming works. A well-organized code structure can enhance clarity and make the learning process more effective. Understanding the key components of this structure can greatly benefit learners by providing them with a roadmap as they navigate through the complexities of programming languages. This section will explore the basic components and common syntax patterns, revealing their significance in mastering coding.
Basic Components
The basic components of application code examples include variables, data types, control structures, and functions. Each of these elements contributes to the overall functionality of the code. A variable serves as a storage location for data, whereas data types define what kind of data a variable can hold. Control structures, such as loops and conditionals, direct the flow of the program based on logical conditions. Functions, on the other hand, encapsulate specific tasks, promoting reusable code. Understanding each of these components is essential. Learners should focus on how they interconnect to build functional, efficient applications.
- Variables are declared to hold values, which may change during program execution.
- Data Types can include integers, strings, and booleans, each serving different purposes.
- Control Structures can be if-else statements or loops, guiding the program's execution paths.
- Functions allow for modular programming, making the code easier to read and maintain.
A clear grasp of these components enables students to break down complex problems into manageable parts. This approach to understanding reinforces the importance of systematically structuring code examples so that they promote better coding practices.
Common Syntax Patterns
Common syntax patterns are essential as they form the basis of how code is written across different programming languages. These patterns not only enhance readability but also ensure that code is standardized, making collaboration easier. Patterns such as loops, conditionals, and expressions help in establishing a predictable syntax that programmers can rely on.
Here are a few common syntax patterns:
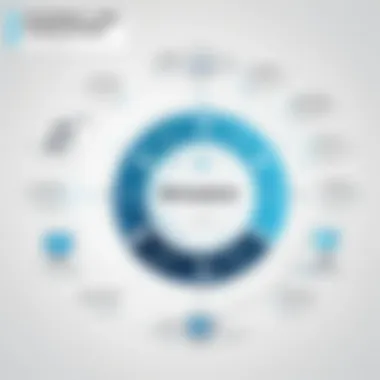
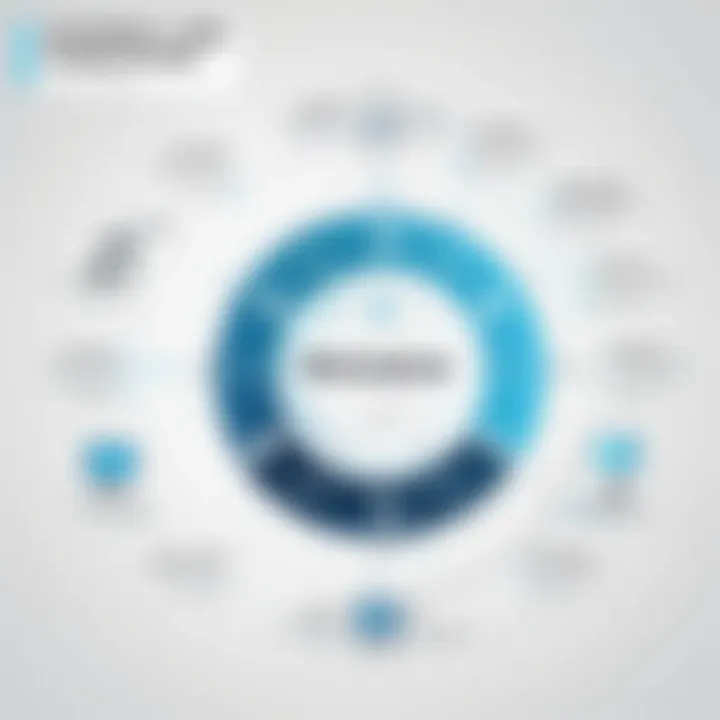
- Loops (for, while): Used to execute a block of code multiple times, useful for iterating over arrays or collections.
- Conditionals (if, switch): Allow the program to make decisions based on conditions, executing different code paths based on specific criteria.
- Functions/METHODS: Encapsulate specific functionalities with a defined input and output, promoting clarity and reusability.
Understanding these syntax patterns aids learners in identifying issues and debugging effectively. By recognizing common patterns, they can quickly adapt their skills to different programming languages, enhancing their ability to code proficiently across a range of environments.
Ultimately, a solid understanding of structure in application code examples not only clarifies the mechanics of programming but also fosters a more intuitive approach to coding. Mastering these components and patterns provides learners with the foundation they need to write effective, efficient, and maintainable code.
Application Code Examples in Different Languages
Understanding application code examples in various programming languages is crucial for learners and practitioners alike. Different languages have unique syntax and paradigms, which can significantly influence how one approaches coding challenges. By exploring code examples across languages, individuals gain insights into problem-solving techniques that may be applicable in their own work.
This section aims to illuminate the practical benefits of familiarizing oneself with application code examples in Java, C, and C++. It demonstrates how specific constructs and idioms in each language can aid in grasping fundamental programming concepts. This is particularly important for those looking to expand their proficiency or transition between different languages.
Java Code Examples
Basic Java Example
A basic Java example often includes simple elements like variables, control structures, and methods. This contributes to understanding the core syntax and semantics of the Java language. The simplicity and readability of basic Java examples make them a popular choice among new learners.
One standout characteristic of a basic Java example is its use of clear and concise code structures. It helps beginners grasp the flow of execution and the logic behind their code. However, while basic examples are great for newcomers, they may lack complexity, which can limit insight into more nuanced programming challenges.
Advanced Java Techniques
Advanced Java techniques encompass a range of topics, such as multithreading, Java Collections Framework, and design patterns. These techniques are vital for achieving efficiency and scalability in software applications. Learning these advanced concepts helps learners comprehend how to write more potent and maintainable code.
The key feature of advanced Java techniques is their ability to optimize performance. While powerful, they can become complex and overwhelming. Beginners may find these examples less approachable, making it important to gradually build up to them through structured learning.
Language Code Examples
Fundamental Programs
Fundamental C programs typically focus on basic constructs like loops, conditional statements, and functions. These programs offer insight into procedural programming and memory management, which is critical for understanding how software operates at a lower level. The straightforward nature of these examples makes them beneficial for learners seeking to build foundational skills.
The unique feature of fundamental C programs is their efficiency. C allows close-to-hardware programming, a valuable capability in systems programming. However, such efficiency requires a deeper understanding of memory management, which could pose challenges for beginners.
Programming with Pointers
C programming with pointers is an essential topic, revealing how variable memory addresses can be manipulated to optimize performance. It plays a crucial role in dynamic memory allocation and data structure implementation. Understanding pointers allows learners to write more efficient code and manage resources effectively.
This aspect of C has a key characteristic: its direct memory manipulation, which can lead to significant performance enhancements. Nonetheless, pointers can also introduce risks, such as memory leaks or segmentation faults, if not used carefully. Thus, while pointers are a powerful tool, they require careful handling and understanding.
++ Application Code Examples
Object-Oriented ++ Examples
Object-oriented C++ examples introduce concepts like classes, inheritance, and polymorphism. These examples are paramount in understanding how to structure larger programs and promote code reusability. They showcase the advantages of using an object-oriented approach, which is characteristic of modern software development practices.
The main benefit of exploring object-oriented C++ examples is their facilitation of software design principles. However, the complexity of object-oriented programming means that learners must invest adequate time to grasp its nuances, making scaffolding in learning important to avoid confusion.
Templates in ++
Templates in C++ allow developers to create functions and classes that operate with any data type. This feature promotes generic programming, enabling more flexible and reusable code. Learning about templates is crucial for mastering advanced C++ features that enhance the language's capabilities.
A defining characteristic of templates is their capability to create highly efficient code without sacrificing type safety. However, template programming can become complex, especially for those new to C++. The use of templates involves understanding concepts such as template specialization, which can be advanced for beginners but rewarding once mastered.
Best Practices for Writing Application Code Examples
Writing application code examples effectively is quite essential for both learners and seasoned programmers. These practices ensure that code is not only functional but also understandable. Emphasizing best practices fosters an environment that enables clearer learning and improved application of programming knowledge. The following segments will explore three critical areas: clarity and readability, commenting and documentation, and error handling strategies. Each of these elements play a unique role in crafting valuable code examples that can educate.
Clarity and Readability
When it comes to code, clarity is king. Code should be as simple as possible, enabling learners to grasp concepts quickly. Clear code reduces the cognitive load on the reader. Here are some key points to improve clarity:
- Use Descriptive Names: Variable and function names must indicate their purpose. For instance, instead of using a name like , utilize . This helps others understand the intent behind the code easily.
- Limit Line Length: Long lines of code can confuse users. Aim for a line length of about 80-100 characters. If a statement is too long, consider breaking it into multiple lines.
- Consistent Formatting: Maintain uniform spacing, indentation, and structuring. This not only helps keep your code looking tidy but makes it easier for others to follow.
Clarity and readability are foundational to effective application code examples. Careful attention to these principles elevates the quality of the code presented.
Commenting and Documentation
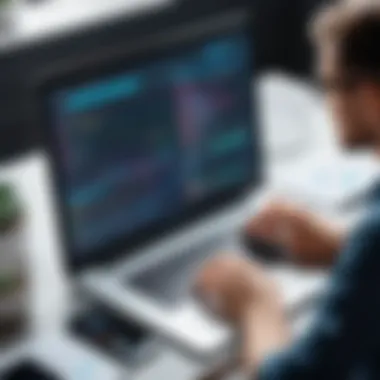
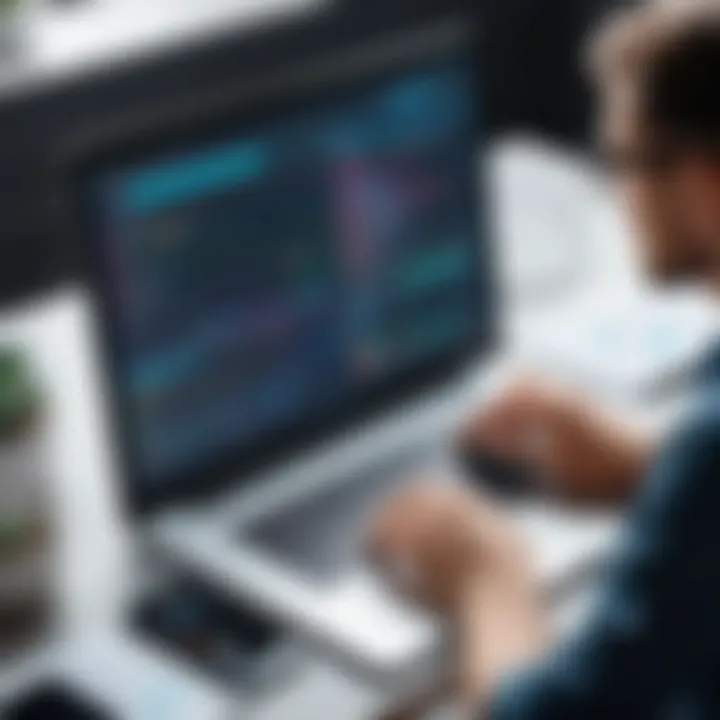
Comments are often undervalued, yet they are indispensable for understanding the intent behind the code. Well-placed comments can significantly enhance the learning experience. Here are a few aspects to consider:
- Briefly Explain Complex Logic: If a section of code accomplishes a complicated task, provide a simple comment beforehand explaining its goal. This helps clarify the thought process without deciphering every line.
- Document Functions: Use docstrings to describe what a function does, its parameters, and its return type. This is particularly useful in languages like Python where documentation can be auto-generated.
- Keep Comments Updated: Outdated comments can lead to confusion. Ensure comments reflect the current logic of the code, thereby maintaining their utility over time.
Commenting and documentation lend themselves as a guide for learners, allowing followers of the code to understand not just what the code does, but why it does it.
Error Handling Strategies
Proficient error handling is a reflection of robust coding. When examples incorporate error handling, they demonstrate a mature approach to programming. Here are effective strategies to employ:
- Use Try-Catch Blocks: Encapsulation of risky operations with try-catch structures can prevent programs from crashing unexpectedly. Clearly indicate the types of errors being caught, so the reader understands the potential pitfalls.
- Validate Inputs: Ensure user inputs are validated before processing. This reduces runtime errors and guards against improper data entries that could compromise functionality.
- User-Friendly Messages: When an error occurs, provide explanatory messages. Clear feedback helps the user understand what went wrong and how to correct it.
Effective error handling not only protects your code but also prepares learners to write their own robust applications.
Common Pitfalls in Application Code Examples
Understanding the common pitfalls in application code examples is crucial for anyone learning programming. These pitfalls can hinder a learnerโs progress and development. Being aware of them allows programmers to avoid making the same mistakes. In this section, we will discuss three significant pitfalls: ignoring edge cases, overcomplicating code, and neglecting code optimization.
Ignoring Edge Cases
Ignoring edge cases is a common mistake that many beginners make. Edge cases are scenarios that occur at the extreme ends of input ranges. For example, consider a function that processes user input. If the function only considers regular inputs, it may fail when fed unexpected or unusual data. This can lead to runtime errors or incorrect outputs.
To prevent this, always think about different types of inputs, including edge cases. These might include inputs like very large numbers, negative values, or even empty strings. Testing against such inputs ensures the code handles all potential situations. A well-rounded application anticipates and manages these edge cases to enhance reliability and user experience.
Overcomplicating Code
Overcomplicating code is another pitfall to be cautious about. Many learners tend to write complex code instead of simpler solutions. This usually happens due to a desire to impress others or a misunderstanding of the problem. Complex code can be difficult to read and maintain. It can also introduce additional bugs.
Emphasizing simplicity is key. Focus on writing clear and straightforward code that accomplishes the task at hand. Good practices include breaking the code into manageable functions, avoiding unnecessary logic, and adhering to established coding standards. This not only makes the code easier to understand but also improves maintainability in the long run.
Neglecting Code Optimization
Neglecting code optimization can lead to performance issues. While developing an application, many find it easy to focus on functionality and forget how efficiently the code runs. Ignoring optimization can result in slow applications, which can frustrate users.
Optimizing code does not require extensive changes. Sometimes, simply reviewing algorithms and eliminating unnecessary computations can lead to improvements. Tools like profilers can help identify bottlenecks and inefficiencies in the code. Make optimization a regular part of your coding process to ensure applications run smoothly and efficiently.
"Optimizing code is not an end goal but a continuous practice that enhances software performance."
Being mindful of these common pitfalls can significantly influence oneโs coding journey. As learners navigate their path in programming, knowledge of these pitfalls encourages better coding practices and ultimately results in higher-quality applications.
Real-world Applications of Code Examples
Understanding the real-world applications of application code examples is crucial for programming students and enthusiasts. These examples serve as tangible representations of how theoretical concepts are put into practice. When learners can see how code functions in real projects, they become more motivated and better equipped to apply their knowledge in various scenarios. Over time, incorporating practical applications into the learning process aids in reinforcing programming skills and concepts.
Code examples allow learners to experience firsthand the development processes within diverse fields. They provide insight into coding practices, tools, and methodologies that professionals regularly use. This exposure fosters a more profound understanding of the software development lifecycle and emphasizes the need for best practices and efficient coding habits.
Case Studies from Industry
Case studies offer practical illustrations of how code examples are applied in real scenarios across various industries. For example, consider how a tech company might use Java to develop a web application. By studying a case like this, students can learn about specific challenges, solutions, and best practices. They see how a code example transitions from concept to deployment, which provides deeper insight into the coding process.
Moreover, the examination of industry case studies emphasizes the importance of collaboration in software development. Teams often work together to solve problems, refine code, and enhance overall functionality. This perspective gives students a glimpse into the collaborative nature of the industry, equipping them with essential teamwork skills.
In addition, learners can analyze case studies that highlight successful applications, such as Spotify's use of Java and Python for backend services. By dissecting these examples, they can understand how the code exemplifies important programming principles, such as modular design or efficient use of resources.
Frameworks Utilizing Application Code
Numerous frameworks utilize application code examples, showcasing their practical implications and solidifying their importance in programming education. Frameworks like Angular, Django, and Spring provide robust environments for developing applications. These tools often come with built-in functionalities that simplify common tasks, yet they rely heavily on proper application code.
Consider Django, for instance. It is a Python web framework that makes it easier to build web applications efficiently. Learning to use Django through application code examples helps students grasp concepts like MVC architecture and RESTful services. Likewise, exploring Angular allows learners to understand client-side programming through real-world applications.
Utilizing frameworks in conjunction with application code examples fosters a better understanding of how real-life applications are constructed, how data flows, and how libraries can enhance functionality. This exposure enables upcoming programmers to build more complex and efficient applications in their future careers.
"Real-world applications of code examples bridge the gap between theoretical learning and practical implementation. They prepare learners for the challenges they will face in their professional journeys."
This emphasis on practical application can make the world of coding feel more accessible and relevant. By engaging directly with code examples derived from frameworks and case studies, learners can enhance their hands-on experience and confidence in their abilities.
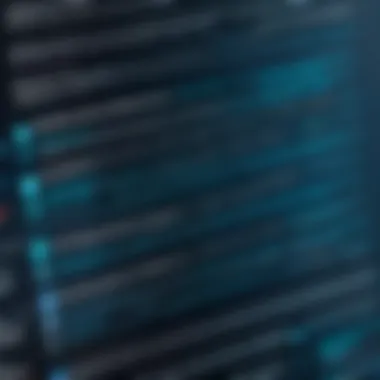
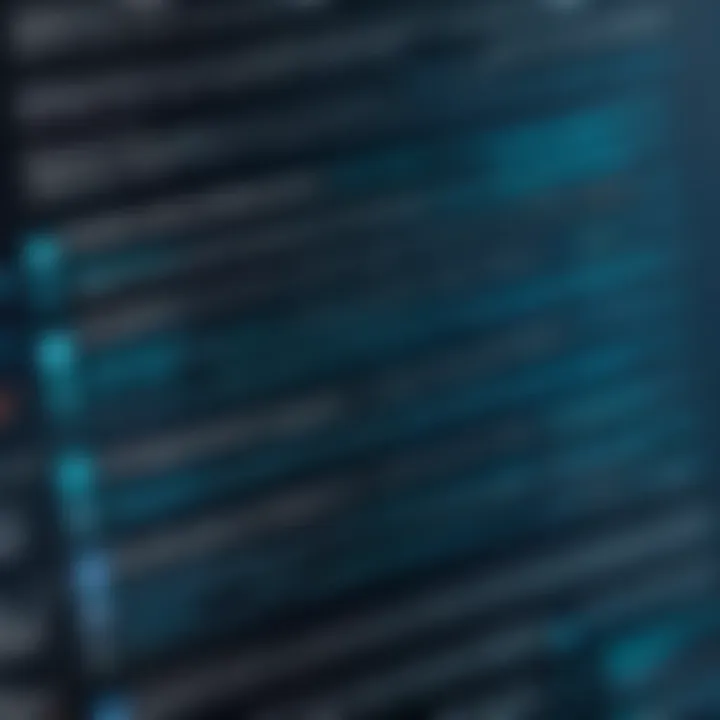
Navigating Online Resources for Application Code Examples
The abundance of online resources for application code examples is both a blessing and a challenge. With numerous platforms offering free access to code snippets, tutorials, and forums, understanding how to effectively navigate these resources is crucial for learners at any stage of their programming journey. Utilizing online resources can significantly enhance your coding skills, allow for real-world applications of theoretical knowledge, and expose you to diverse coding styles and methodologies.
Identifying Reliable Sources
When searching for application code examples, the validity of the sources cannot be overstated. Not all online resources maintain stringent quality control. Reliable sources, such as official documentation, well-regarded educational sites, or established coding platforms, should be prioritized. For instance, websites like en.wikipedia.org provide foundational knowledge, while platforms like reddit.com can be extremely helpful in finding community-curated content.
Consider these elements when identifying reliable sources:
- Accuracy: Ensure the code examples are tested and verified by professionals or experienced programmers.
- Reputation: Look for sources that are well-regarded within the programming community.
- Recency: Technology evolves rapidly. Ensure the examples reflect current standards and practices.
By being discerning about the sources, you will avoid falling into the trap of misinformation and learn more efficiently.
Leveraging Programming Communities
Programming communities can serve as a valuable resource for finding application code examples. Engaging with these communities can provide access to a wealth of knowledge that comes from shared experiences and collaboration. Many coding forums allow users to ask questions and receive guidance from seasoned developers.
Here are some benefits of leveraging programming communities:
- Variety of Perspectives: Exposure to different approaches to problem-solving can enhance your flexibility as a programmer.
- Real-user Feedback: Discussions often yield feedback that can improve your understanding of best practices.
- Code Reviews: Many communities offer code review services, allowing you to receive constructive criticism on your code examples.
Joining programming communities on platforms like Facebook can connect you with fellow learners and professionals alike. As you participate in discussions, consider contributing your own insights and code examples. This not only aids your learning but also strengthens the community at large.
"The best way to learn is to teach."
By embracing the resources available online and the support offered by programming communities, you can effectively navigate through the vast world of application code examples. This strategic approach allows you to build a robust foundation in coding, fostering both confidence and competence.
Future Trends in Application Code Examples
The world of programming is dynamic and constantly evolving. Understanding the future trends in application code examples is crucial for those engaged in coding and software development. These trends not only reflect changes in technology but also influence the way coding is taught and learned. Staying aware of these trends helps programmers adapt to emerging standards and practices, reinforcing their relevance in an ever-competitive environment.
Evolving Coding Standards
As technology advances, coding standards are not static; they evolve to meet new challenges. This evolution aims to improve readability, maintainability, and efficiency of code.
In recent years, many programming languages have seen substantial updates. For instance, Java has introduced features such as lambda expressions and optional parameters. These updates enhance code expressiveness and support functional programming paradigms. Similarly, C++ has embraced concepts from newer programming languages, incorporating features like smart pointers and auto keyword to contribute to safer memory management.
The significance of adhering to these evolving standards lies in cultivating a consistent coding style. A codebase that follows modern practices is often easier to read and understand, which is vital when teams collaborate across various levels of expertise. To capitalize on these evolving standards, programmers should:
- Immerse themselves in documentation and resources.
- Participate in relevant workshops or online courses.
- Engage in coding communities to share knowledge and discuss developments.
This mindset cultivates a culture of continuous learning essential for any programmer wanting to remain relevant in the field.
Automation in Code Generation
Automation in code generation is reshaping the landscape of programming. Tools that automate repetitive coding tasks significantly increase productivity and reduce errors. This trend is becoming more pronounced with the rise of integrated development environments (IDEs) and code generation frameworks.
Automating mundane tasks allows developers to focus on more complex problems. For example, tools like Yeoman assist in creating project scaffolds, while functionalities in Visual Studio Code offer autocomplete suggestions and error detection. Such tools simplify the coding process, allowing programmers to produce higher quality work in a shorter timeframe.
Nevertheless, while automation provides efficiencies, it is vital to understand its limitations. Relying too heavily on automated tools may lead to a decrease in foundational coding skills. Therefore, balancing automated solutions with hands-on coding practice is prudent. Developers should:
- Regularly review and refine their understanding of core programming principles.
- Utilize automation for appropriate tasks while keeping manual coding sharp.
- Monitor industry trends to stay informed on useful automation tools.
In summary, embracing automation thoughtfully can enhance programming capabilities without superseding essential skills.
"The future of application code examples is not just in knowing how to write code, but in understanding how to leverage tools that can amplify our efforts."
The trends outlined in this section showcase significant shifts in the coding landscape, reinforcing the need to adapt to the changing programming environment. By keeping pace with evolving coding standards and leveraging automation, programmers can enhance their skill set and improve their coding practices.
Closure
The conclusion serves as a pivotal section in any article, particularly in a comprehensive guide focused on application code examples. It provides a moment for reflection on the insights discussed throughout the previous sections. A well-rounded conclusion encapsulates the essence of what has been explored, reinforcing the knowledge imparted and ensuring that readers leave with clear takeaways.
In this article, we have traversed various essential components, from understanding the significance and structure of application code examples to best practices and pitfalls to avoid. Each element we discussed builds a foundation for effective learning and implementation. By summarizing these key points, we help learners consolidate their understanding, making it easier to reference and apply the knowledge gained.
The benefits of a definitive conclusion extend beyond mere summary. It encourages readers to think critically about the information presented. When they engage with the concluding sentences, they can reflect on how these concepts connect to their personal coding journey. Furthermore, this section presents an opportunity to inspire readers to continue their exploration of coding, propelling them into further study and practice.
One consideration when crafting conclusions is to encourage ongoing learning. The realm of programming is constantly evolving. This reality emphasizes the importance of staying updated with the latest practices, languages, and technologies. When readers are mindful of their learning journey, they are more likely to seek additional resources and interactions within programming communities.
"For every master coder, there exists a series of foundational codes and examples that pave the way for expertise."
By keeping these elements in focus, the conclusion serves not only to summarize but also to motivate. It is a clarion call for readers to engage further in programming, creating a continuous loop of learning and application that makes becoming proficient in software development not just possible, but an expansive journey.