Comprehensive Guide to Android Programming for All Levels
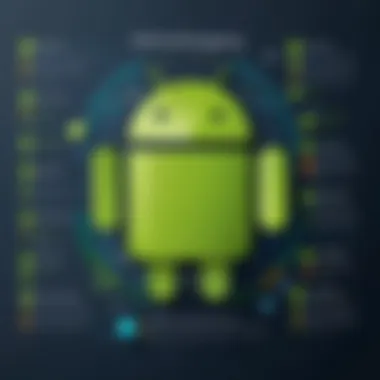
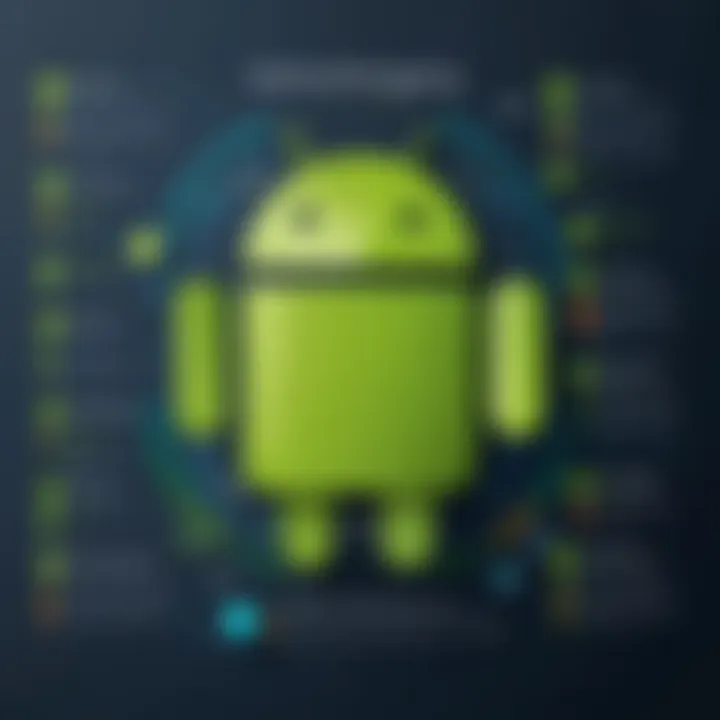
Prologue to Programming Language
One cannot truly grasp the essence of Android programming without first looking into the very language that underpins its functionality. Java has historically been the backbone of Android development, giving developers the tools needed to create robust applications. But as Android has evolved, so too has its ecosystems; languages like Kotlin have emerged, elevating the programming landscape.
History and Background
Java was introduced in the mid-1990s, and its versatility allowed it to become a cornerstone of many application frameworks. Google adopted it for Android because of its portability and object-oriented principles. Kotlin, on the other hand, came into play more recently, becoming the preferred language for many developers due to its modern features and safety mechanisms. This transition signifies a shift in how developers approach app development, focusing more on efficiency and less on verbosity.
Features and Uses
Both Java and Kotlin provide unique features suitable for Android app development. Here are some of their standout traits:
- Java: Strongly typed, object-oriented, platform-independent.
- Kotlin: Concise syntax, null safety, great interoperability with Java.
These features make it easier to maintain and scale applications, addressing both performance and programmer productivity.
Popularity and Scope
Java was the king of Android for years. In the last decade, though, Kotlin has carved out its space, now known as a "first-class" language for Android development, as endorsed by Google. This shift has been noticeable, as Kotlin's use has surged in codebases across various projects, signifying developers' preference for more expressive and less error-prone coding. According to recent surveys, Kotlin has surpassed Java in popularity among Android developers, demonstrating its widespread adoption.
In summary, understanding the programming languages integral to Android development is crucial. The journey begins with Java, exploring its roots and significance, and continues with Kotlin, embracing its modern features. Both together pave the pathway for aspiring developers to engage with Android programming in a more intuitive and efficient manner.
"Programming isn’t about what you know; it’s about what you can figure out."
As we continue this guide, we will explore the basic syntax and concepts of programming, crucial for building your foundational knowledge in Android development.
Preamble to Android Programming
Android programming holds a crucial spot in the realm of application development, especially as mobile devices continue to dominate the tech landscape. This segment serves as a gateway for those stepping into the world of coding and app creation. In this article, we will navigate through the nuts and bolts of Android programming, from grasping basic concepts to executing sophisticated applications.
Engaging with Android development not only enables you to create applications for millions of users but also sharpens your problem-solving skills. The focus on practical experience means that the skills you develop while programming can be thresholds into other areas of software development and technology.
Understanding the Android Ecosystem
Android is not just an operating system; it’s a sprawling ecosystem that incorporates a multitude of devices, platforms, and services. The vastness of the ecosystem can be both exhilarating and daunting for newcomers. At its core, Android is an open-source platform based on the Linux kernel, allowing developers to create applications that run on a variety of devices, from smartphones to wearable tech.
Several components make up the Android ecosystem:
- Android OS: The foundation that ensures an interface for developers to interact with device hardware.
- Android SDK: The Software Development Kit provides tools necessary to build Android apps.
- Google Play Store: The primary marketplace where apps are published and downloaded, giving developers a broad audience.
With the growth of the Internet of Things, understanding this ecosystem also means staying updated with how Android integrates with other technologies. Being familiar with it helps developers leverage existing technologies and create innovative applications.
Significance of Android Development
The impact of Android development cannot be overstated. With over two billion monthly active devices, Android has established itself as a primary platform across the globe, offering developers robust opportunities to reach users.
Here’s why diving into Android development is important:
- High Demand for Android Apps: As businesses increasingly embrace mobile solutions, there’s a growing need for Android developers. Organizations continually seek skilled professionals who can navigate the complexities of app lifecycle management.
- Diverse Opportunities: Whether you're nurturing your entrepreneurial spirit by launching your own app or joining established companies, the choices are diverse.
- Community Support: The Android developer community is extensive and welcoming. Platforms like Reddit and various forums offer support and resources that can aid both beginners and seasoned developers.
- Evolving Technologies: The continuous development in areas like Artificial Intelligence and augmented reality creates new opportunities within the Android arena.
As you embark on this journey, remember that every line of code you write is an opportunity to expand not just your skill set but also your understanding of the digital world we inhabit.
"Every great app started as an idea. Android programming is your first step towards bringing that idea to life."
Whether you aspire to just create handy tools or to change the landscape of mobile applications. Understanding the broader significance will fuel your passion and drive your development journey forward.
Setting Up the Development Environment
Establishing a solid foundation in your development environment is crucial for any Android programmer. This section focuses on the essential setup tasks that can not only streamline your development process but also enhance the overall effectiveness of your programming efforts. A well-prepared environment allows developers to test applications quickly, manage resources efficiently, and troubleshoot issues with ease.
Here are some key benefits of setting up a proper development environment:
- Efficiency: An organized setup can significantly reduce the time spent on repetitive tasks.
- Compatibility: Ensures that all the components work seamlessly together, reducing headaches later on.
- Learning Curve: Makes it easier for beginners to follow along and understand the processes involved in Android programming.
Choosing the Right IDE
The choice of Integrated Development Environment (IDE) can make or break an Android developer's experience. IDEs are powerful tools that offer features like code completion, debugging, and project management. In the landscape of Android development, two dominant players are Android Studio and several noteworthy alternatives.
Android Studio Overview
Android Studio is the official IDE for Android development and carries with it several characteristics that make it a prime choice for developers. One key feature is its built-in support for both Java and Kotlin, the two primary programming languages used in Android programming. This feature makes transitioning between languages smooth, as developers can easily switch based on project needs or personal preferences.
Another significant aspect of Android Studio is its comprehensive layout editor, which allows developers to design user interfaces visually. Dragging and dropping components simplifies a sometimes daunting task, especially for beginners who are still getting the hang of XML layouts.
However, Android Studio isn't without its drawbacks. As a resource-heavy application, it demands significant system performance. Developers with modest hardware might experience lag, particularly when working on larger projects, which could lead to frustration.
Other Notable IDEs
While Android Studio is widely regarded, it's beneficial to keep other IDEs on your radar. For instance, IntelliJ IDEA provides a solid alternative, especially for experienced users who prefer a familiar environment built upon the same foundation as Android Studio. The ability to integrate various plugins allows developers to customize their setup significantly.
A unique feature of IntelliJ IDEA is its intelligent code assistance, which can be quite helpful for catching errors and suggesting improvements in real time. However, the extensive features may not be necessary for beginners, making the learning curve steeper compared to Android Studio.
Eclipse is another IDE worth mentioning, previously popular for Android development before Android Studio took over. It still has its followers, mainly due to its compatibility with multiple languages and platforms. Yet, the shifting preference towards Android Studio means that help and resources for Eclipse may not be as readily available, which can hinder new developers.
Finally, let’s not forget that the choice of IDE can boil down to personal preference. While Android Studio offers comprehensive features intended primarily for Android development, alternatives like IntelliJ IDEA or Eclipse can bring tailored functionalities to the table.
Installing Android SDK
The Android Software Development Kit (SDK) is a collection of tools that helps developers build, debug, and run Android applications. Installing the Android SDK correctly is essential to ensure that your development environment is ready for action.
The installation process is typically tied to your IDE; for instance, Android Studio facilitates it during the setup stage. However, if you’re using a different IDE, you may need to download the SDK separately. It’s essential to pay attention to the SDK tools and packages you select during installation.
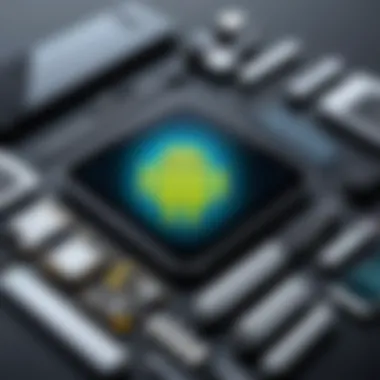
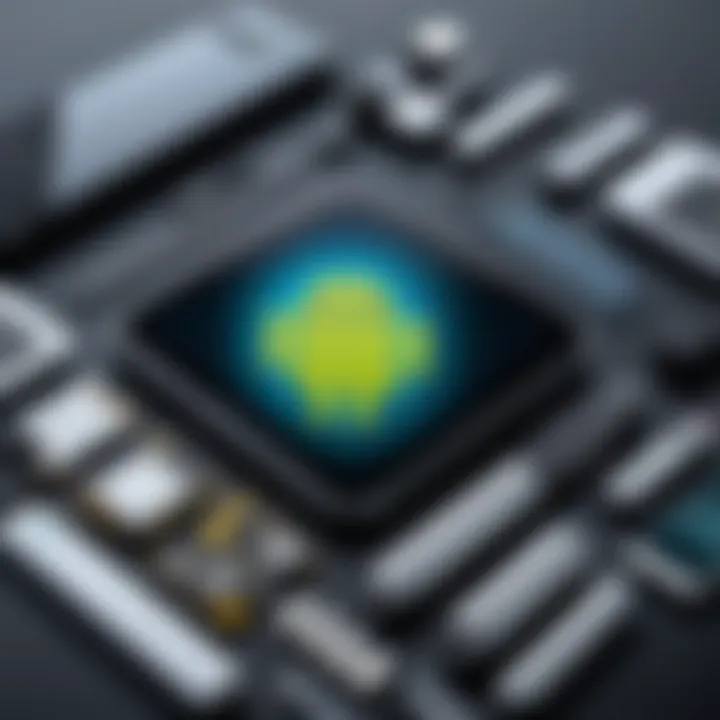
Once installed, the SDK provides resources like emulators, libraries, and debugging tools, which are indispensable for Android development. Keeping the SDK updated is also paramount to leveraging the latest features and security patches.
"A robust SDK ensures a smoother development journey, making it easier to implement new features and support various device functionalities."
Setting up your development environment carefully is like laying a solid foundation for a house; it simply makes all the difference when you're ready to build your applications.
Core Concepts of Android Programming
Understanding the core concepts of Android programming is essential for anyone embarking on a journey into mobile app development. These concepts lay the groundwork for how apps are developed and function within the Android operating system. By grasping these principles, developers can more effectively harness the platform’s capabilities, ensuring robust and efficient applications.
At the heart of these concepts is the understanding of architecture, languages, and the environment in which applications run. Let’s dig deeper into a couple of key components: the choice of programming language and the Android architecture itself.
Java vs. Kotlin for Android
Key Differences
When it comes to programming languages for Android, Java has long been the traditional choice, representing stability and a massive developer community. In contrast, Kotlin has gained traction as a modern alternative. One could argue that the key difference lies in their syntax and functional capabilities. Kotlin’s succinctness allows developers to write less boilerplate code, which is often a sticking point in Java.
This succinctness doesn't just reduce code clutter; it can lead to fewer bugs by decreasing the amount of code needing inspection. Simply put, a cleaner codebase often leads to a smoother development process.
Additionally, Kotlin’s null safety feature significantly reduces the chances of encountering the dreaded NullPointerException, a common pitfall for Java developers. This aspect alone can save hours of debugging, making Kotlin a sought-after choice in many new projects.
Incorporating Kotlin in business apps can also enhance maintainability, which ultimately supports long-term project sustainability.
Choosing Your Language
When deciding between Java and Kotlin, personal preference plays a hefty role, but so does project type. For those delving into Android coding for a hobby or a small project, Kotlin might feel more inviting due to its straightforward syntax. Conversely, if you're stepping into an established enterprise environment where Java is entrenched, sticking with Java may make more sense.
From a practical standpoint, both languages converge towards the same goals, but their paths diverge in execution. A unique feature of Kotlin, similar to Java’s object-oriented principle, is its integration capabilities with existing Java code. This compatibility can be a game changer for teams transitioning from Java to Kotlin without needing to rewrite their entire codebase. So, taking time to weigh your options is crucial.
Understanding Android Architecture
To further enrich our understanding of the core concepts, let ’s explore Android architecture. Android’s architecture is structured in layers, beginning with the Linux Kernel at the bottom, upon which all other components depend. This layer is vital since it manages the device hardware, memory, and processes, ensuring smooth operation.
The next layer is the Android Runtime, which includes core libraries and Dalvik, an execution environment. Here is where you gain access to essential functionalities such as file handling, networking, and resource management. A strong grasp of this layer empowers developers to write more optimized applications.
Above this is the Application Framework, encapsulating the key services and features that developers interact with when building apps—think of content providers, activity managers, and the view system.
Lastly, there are the applications, your actual end products visible to users. This stratified approach not only simplifies app development but also provides a detailed blueprint for future scalability and maintenance.
Understanding these core concepts is not simply an academic exercise; it's a practical necessity for effective app development. It’s what allows you to streamline your work, improve efficiency, and ultimately lead to a better product in the hands of users.
Building Your First Android Application
Creating your first Android application is not just a box to tick off your learning journey—it's the gateway to bringing your ideas to life in the mobile space. When you embark on this path, you’re engaging in an act that combines creativity with technical prowess. The process is invigorating and serves to underscore the principles laid out earlier in this guide. By crafting an application, you gain hands-on experience that enhances comprehension of concepts such as user interface design, application lifecycle, and debugging.
Building an application also helps in establishing a solid foundation in Android development. This foundation is crucial as it allows you to tackle more complex projects down the road. The seemingly simple act of creating an app is filled with important lessons that offer substantial value in your overall learning experience.
Creating a Simple App
Defining Your App's Purpose
At any stage as a developer, especially when venturing into Android programming, defining your app's purpose is like laying down a map before a long journey. It sets the direction and clarifies what you aim to achieve. When you outline the purpose, you hone in on your target audience and understand their needs. For instance, you might want to develop a tool for tracking healthy eating habits, something that resonates with health-conscious users.
One key characteristic of defining your app's purpose is its ability to narrow your focus. By having a clear purpose, you avoid scope creep—where your app's intended functionalities expand uncontrollably, leading to confusion and burnout. This clarity becomes an invaluable asset as it streamlines the development process, making it easier to decide on features and design choices.
Nevertheless, defining your app’s purpose does come with challenges. It can sometimes be tempting to pivot based on what you think is trendy rather than sticking to your initial idea. That said, staying true to your original purpose will often resonate more with users even if it means choosing a less glamorous path.
Designing the User Interface
Designing the user interface is where the magic of interaction happens. It’s an essential aspect of app development that influences how users feel about your app. A clean, intuitive UI either invites users in or pushes them away. Ultimately, it contributes significantly to user satisfaction and retention.
A noteworthy aspect of designing the user interface is usability. When you concentrate on making your app user-friendly, it enhances engagement levels. This could range from how buttons react upon being pressed to how menus are organized. Focusing on these details means you're also considering less technical users, thus broadening your app’s appeal.
One unique feature of UI design in Android is the use of Material Design principles; it’s adaptable and provides a uniform experience across devices. However, its nuances can sometimes pose a learning curve, particularly regarding responsiveness across different screen sizes. Balancing aesthetic elements with functional requirements is often a task that developers face; getting this balance wrong can result in an application that looks great on paper but fails when it undergoes real-world testing.
Understanding Android Manifest
The Android Manifest is your application's calling card. It serves as a guidepost to both the Android system and other developers. In short, it outlines key attributes about your application: its components, permissions, and the devices on which it can run.
Understanding this file is crucial because it impacts the app’s lifecycle and how it interacts with the Android operating system. For example, it declares activities, services, and content providers, setting the stage for how your app behaves within its ecosystem.
By mastering the Android Manifest, you are better equipped to manage permissions effectively—vital for user trust and legal compliance. In this digital age, users want assurances regarding data security. This particular aspect is not just a technical detail but a necessity in building a reputable app.
To summarize, building your first Android application is a foundational step, rich with lessons that extend well beyond mere coding. Each decision, from defining the app's purpose to the intricacies of the Android manifest, impacts the journey ahead. Embrace this phase, as it’s where your developmental skills truly begin to blossom.
User Interface Development
User Interface Development plays a pivotal role in Android programming. A well-crafted interface elevates user engagement, enhances usability, and directly impacts app success. This section delves into the elements essential for crafting intuitive and appealing interfaces that resonate with users.
Layout and Views
Understanding Layouts
XML layouts serve as the backbone for designing user interfaces in Android applications. They organize UI components in a structured manner, allowing developers to separate design from logic. One key characteristic of XML layouts is their versatility; they can accommodate diverse screen sizes and densities, making them an ideal choice for creating responsive interfaces.
A unique feature of XML layouts is the ability to define UI elements through a markup language. This separation of definitions from code leads to cleaner code bases, important for maintaining and scaling applications effectively. The simplicity of XML makes it approachable for beginners. However, there’s a learning curve in mastering the complexity of hierarchical layouts that XML can produce.
"The essence of effective UI is not just in how it looks, but in how it feels."
The graphical representation provided by XML helps visualize layouts before implementation, allowing quicker iterations based on feedback. One thing to remember is that complex layouts can affect performance; hence, it’s vital to minimize nesting to enhance load times.
Creating Custom Views
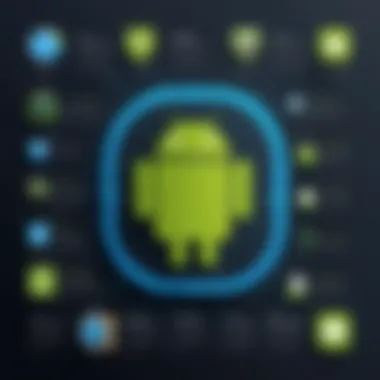
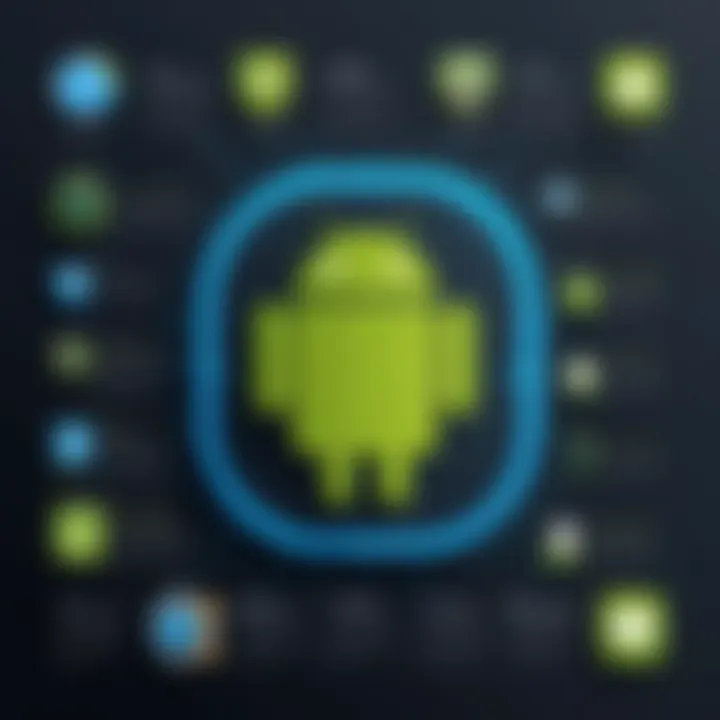
Creating custom views allows developers to break free from the limitations of standard components. It contributes to personalization and ensures applications stand out in a crowded marketplace. A standout characteristic of custom views is their flexibility; they can be tailored to specific needs and brand identities, enhancing the overall aesthetic and functionality of the app.
The process of creating a custom view involves extending a View class and overriding necessary methods to suit your requirements. This gives developers substantial control over rendering and user interactions, making it a popular choice for those who want precise UI control. Nevertheless, creating custom views requires a deep understanding of Android's rendering pipeline, which can be intricate. This complexity might intimidate novice developers, but mastering it can significantly benefit the overall presentation of the application.
Handling User Interaction
User interaction is the actual touchpoint between users and the app; it’s how users engage with the UI. Understanding how to capture and respond to user inputs is crucial for enhancing the user experience.
The richness of user interaction goes beyond basic touch events; it includes gestures, animations, and transitions that make applications feel responsive and alive. Effective handling of user interactions retains users and minimizes frustration during the app's usage.
For instance, if you create a form, ensuring fields are easily accessible, with clear instructions and responses, can lead to higher completion rates. Moreover, implementing smooth animations and feedback mechanisms involves valuable considerations in design that can make or break the first impression users form about the application. Ultimately, user interaction design is about empathy; knowing users’ needs and frustrations is vital for developing successful apps.
To conclude, UI Development manifests as an intricate dance between aesthetics and functionality. Crafting layouts in XML simplifies the visual structure, while custom views enhance creativity and personalization. At the same time, mastering user interaction is essential for facilitating seamless navigation and fostering user loyalty. These aspects connect to form the heart of an engaging Android application.
Data Management in Android
When you're diving into Android programming, understanding data management is crucial. Imagine your app being a busy restaurant. Guests (users) come in, place orders (input data), and expect their meals (processed data) to arrive promptly. Just like a good restaurant needs an efficient system to track orders, your app needs effective data management strategies to handle user information and app data efficiently.
Data management refers to how your application handles, stores, and retrieves information. It encompasses different methodologies and technologies that allow your app to function smoothly and deliver a great user experience. In this section, we will cover two important aspects of data management on Android: using SQLite databases and leveraging shared preferences.
Using SQLite Databases
SQLite is a light-weight database that runs directly on the device. Think of it as a small, reliable storage room located right in the kitchen of your restaurant. It is particularly beneficial for applications that require structured data storage. The key advantages of SQLite include:
- Local Storage: Data is stored on the device, allowing quick access even without internet.
- Structured Querying: You can use SQL queries to perform complex data operations effortlessly.
- Performance: SQLite is fast because it avoids network latency associated with online servers.
Using SQLite requires setting up a few components: a database helper class, data model classes, and CRUD (Create, Read, Update, Delete) operations. Here's a simplistic example that illustrates this:
This class lays down a basic structure for managing your database. The method is called when the database is first created, setting up the necessary tables for data management. When you grow your restaurant (add new features to your app), you might need to modify the database structure, handled in .
Shared Preferences
On the other hand, consider shared preferences as a small notepad where you jot down quick orders. It’s simple and effective for storing small amounts of data. This is ideal for user settings, configurations, or simple flags.
Shared preferences work well for storing key-value pairs. For instance, if you want to remember a user’s preferred theme or language preference, shared preferences are a perfect fit. The major benefits include:
- Simplicity: Easy to implement and quick to access.
- Lightweight: Not meant for heavy or complex data, but perfect for small bits.
Here's how you can use shared preferences to store and retrieve data effectively:
Important Note: While shared preferences provide an easy way to persist data, remember it’s best suited for lightweight data and user preferences. It’s not meant for complex data structures or large datasets.
Testing and Debugging Android Applications
Testing and debugging are two critical components of the Android development lifecycle. They ensure that applications are not only functional but also user-friendly and reliable. When you brew a cup of coffee, you don’t just throw in the beans and water; you test and adjust until you nail the perfect mix. Similarly, in Android programming, ensuring your app operates smoothly can save a boatload of headaches down the line. Without proper testing and debugging, applications can end up filled with bugs, crashes, and a poor user experience—all things that can lead to bad reviews and diminished user outreach.
Benefits of Testing:
- Identifies Bugs Early: Addressing issues before the app reaches users helps in minimizing negative feedback.
- Enhances User Experience: A smooth, bug-free app increases user satisfaction and retention.
- Facilitates Maintenance: Code that has been tested thoroughly is often easier to maintain.
Considerations:
- Time and Resources: While testing is crucial, finding the right balance between development speed and thorough testing can be a challenge for developers.
- Choosing the Right Tools: There are many testing frameworks available, and selecting the appropriate ones for your project is crucial.
At the heart of effective testing and debugging lies the toolset provided by Android Studio, the most widely used Integrated Development Environment (IDE) for Android development.
Debugging Tools in Android Studio
Debugging tools in Android Studio are like a Swiss army knife for developers. They offer numerous functionalities that help pinpoint issues in codefast. The debugging process allows developers to run their apps in a controlled environment, receive real-time feedback, and fix issues on the fly—think of it as troubleshooting your vehicle before taking a long road trip.
Key features of these debugging tools include:
- Breakpoints: Marking lines of code where the program will pause allows you to examine variables and program flow.
- The Debugger Window: Displays current state, values of variables, and call stack—all crucial for determining where things might have gone haywire.
- Logcat: This is your app's diary. It captures logs generated by the application, making it easier to follow its behavior over time.
Unit Testing Fundamentals
Unit testing focuses on individual components of the codebase, allowing developers to validate each part's functionality. It’s like checking each ingredient in a recipe to ensure they’ll yield a delicious dish when combined. For Android, unit tests help verify the logic of each code unit, making it easier to catch potential issues before they escalate.
Using JUnit for Testing
JUnit stands out as a popular testing framework among Android developers. It brings a degree of simplicity and structure, allowing programmers to write repeatable tests. One key characteristic of JUnit is its assertion-based testing, which checks whether a certain condition is true, thus confirming the functionality of the code.
- Why Choose JUnit: It’s lightweight, easy to integrate, and widely recognized in the Java community, making finding resources and support a walk in the park for beginners.
- Unique Feature: JUnit supports annotations, which streamline the organization and execution of test cases, saving developers from messy code.
- Advantages and Disadvantages:
- Advantages: With a comprehensive suite of features, it’s perfect for unit testing, compatibility with other frameworks, and a vast community.
- Disadvantages: Its primary focus on unit tests means more complex integration testing requires other tools or frameworks.
Integration Tests
Integration tests come into play when you want to ensure that multiple components work together as intended. Unlike unit testing, which isolates parts of the code, integration testing checks the interactions between these parts, making it crucial for catching issues in real-world scenarios. It’s like testing out a new recipe after cooking each ingredient separately; you gotta see how they blend together.
- Why They Matter: Integration tests verify that the modules work cohesively, helping to reduce unexpected issues when the app is running.
- Key Characteristic: This type of testing evaluates how different modules of code interact, assessing their communications and data exchanges.
- Unique Feature: They can be automated, which is a time-saver, allowing consistent and easy testing frequencies.
- Advantages and Disadvantages:
- Advantages: Offers a better understanding of how components coalesce, ensuring functional harmony.
- Disadvantages: More complex to set up than unit tests and can require more significant resources, thereby extending development time.
Publishing Your Android App
Publishing your Android application is not just the final step in a long journey; it embodies the culmination of countless hours of coding, testing, and refining your work. It is a significant milestone that transforms your app from a local concept into a global entity accessible to millions. Understanding the nuances of this process can help developers ensure their hard work pays off, maximizing visibility and user adoption.
Preparing for Release
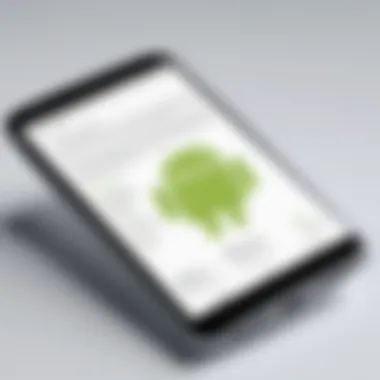
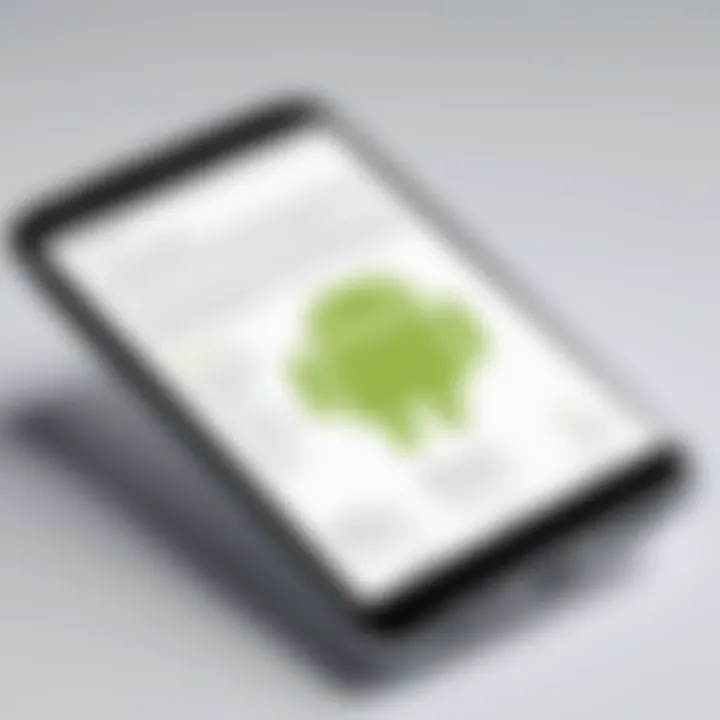
Getting ready for the big debut of your app involves critical steps that ensure your application not only functions well but is also ready for public scrutiny.
Creating a Signed APK
One of the first tasks you'll encounter is creating a Signed APK. A Signed APK is essentially the packaged version of your application that has been digitally signed by you, the developer. This signature reassures users that they are downloading software from a verified source.
This step is crucial because, without it, you cannot upload your app to the Google Play Store.
- Key Characteristic: The creation of a Signed APK grants the application an identity. It’s like an ID card for your app—without it, you’re not getting into the club.
- Why It’s Beneficial: It makes your app trustworthy, convincing users that they can install it without concern.
- Unique Feature: If you’re working on updates or multiple versions of your app, having a Signed APK allows for easier version management. However, managing keys can be challenging if mishandled, potentially leading to access issues later on.
Versioning Your Application
The second aspect to consider is Versioning Your Application. Proper versioning is not just about numbers; it’s a comprehensive strategy that reflects the evolution of your app.
- Key Characteristic: Each version number typically consists of three components: major, minor, and patch versions. This systematic approach lets users understand the significance of updates at a glance.
- Why It’s Beneficial: Clear versioning can significantly enhance user experience, as users can easily determine if they are using the latest features or fixes.
- Unique Feature: With careful version control, you can revert to a previous version if newer updates induce issues. This strategy can save face and avoid user dissatisfaction, but if done incorrectly, it may leave your app stuck in an earlier state, missing out on enhancements.
Submitting to Google Play Store
Once you've prepared your app for release, the next significant step is submitting it to the Google Play Store, allowing users to access your creation formally.
Compliance with Policies
Ensuring compliance with the stated policies is essential. Google enforces community, safety, and data policies that every developer must adhere to. This isn’t just red tape—it’s a safeguard for users who trust the platform.
- Key Characteristic: Each app undergoes a review process where adherence to Google’s policies is assessed.
- Why It’s Beneficial: Following these guidelines increases the approval rate and expedites the release process.
- Unique Feature: Being compliant builds credibility. If users find out you’ve violated policies, not only can your app face removal, but your developer account might be flagged too, limiting future projects.
Marketing Best Practices
Once your application has been approved, implementing robust marketing strategies is key to standing out in a crowded marketplace.
- Key Characteristic: Effective marketing includes leveraging social media, app store optimization (ASO), and perhaps utilizing PR campaigns to create buzz.
- Why It’s Beneficial: Well-planned marketing can result in higher download rates, giving your app momentum right out of the gate.
- Unique Feature: Engaging early users with beta testing or exclusive access can create brand ambassadors before the official launch. However, poorly targeted marketing can lead to wasted resources and low engagement.
In summary, the journey from coding to publishing can be a winding road. Paying attention to the finer details during this phase is critical for not only launching your app successfully but also for establishing it as a reliable and valuable entry in the app ecosystem.
Navigating Advanced Topics in Android Development
The world of Android programming is an ever-evolving landscape, and grasping advanced topics can set a developer apart from the pack. As technology surges ahead, understanding intricate aspects like APIs and third-party libraries becomes crucial. These areas not only enhance application functionality but also streamline the development process. By navigating these advanced subjects, developers can create more robust applications that meet the demands of users everywhere.
Understanding APIs and Web Services
Application Programming Interfaces (APIs) are foundational in modern software development. They serve as contracts that enable different software components to communicate efficiently. In Android development, APIs allow applications to pull data from various sources, opening a floodgate of possibilities for integrating features and services.
Benefits of Using APIs:
- Enhanced Functionality: By leveraging external data, you enrich your app’s features, tapping into platforms like Twitter or Google Maps.
- Efficiency: You don’t have to reinvent the wheel; APIs allow you to use existing technologies to build your app with less effort.
- Speed of Development: Accessing pre-built functionalities can save time, getting your app to market faster.
When working with APIs, it’s important to understand RESTful principles and JSON data format, both of which are commonly used in web services. Whether it’s an HTTP request or parsing responses, comprehending how APIs function is essential.
One common interaction might look something like this in an application:
By employing APIs effectively, developers position themselves to tap into collective data, expanding their applications’ capabilities manifold.
Integrating Third-Party Libraries
In the programming realm, not every solution needs to be custom-built. Third-party libraries act like Lego blocks—developers can snap them together to create complex functionalities without having to start from scratch. This concept is fundamental in speeding up development cycles and enhancing application features.
Why Use Third-Party Libraries?
- Saves Time: Instead of coding everything in-house, using libraries can drastically reduce development time.
- Maintenance: The broader developer community often oversees these libraries, meaning they can receive continuous updates and bug fixes.
- Innovation: Numerous libraries focus on specialized functionalities, enabling developers to introduce cutting-edge features that capture user attention.
Some popular libraries to consider include Retrofit for network calls, Glide for image loading, and Gson for parsing JSON. Integrating these can often be as simple as adding a dependency in your :
However, it’s essential to exercise caution. Relying too heavily on third-party libraries can lead to bloated applications or introduce vulnerabilities if not vetted properly. Developers should evaluate the health of the project behind a library, check for recent commits, and read user feedback before diving in.
Integrating advanced topics like APIs and third-party libraries not only elevates your application’s capabilities but also sharpens your competitive edge in the dynamic world of Android development.
In summary, navigating advanced topics such as APIs and third-party libraries can be a game changer for aspiring Android developers. As these concepts are carefully woven into your development process, you'll find that they not only enhance the user experience but also ease the development journey, arming you with the tools needed for success.
Future of Android Programming
The future of Android programming is both exciting and complex, shaping the mobile landscape in ways we can only begin to fathom. As markets and technologies evolve, developers must stay ahead of the curve. This section will delve into emerging trends and key technologies that will influence how Android applications are developed in the coming years. Understanding these shifts is pivotal, not just for building future-proof applications, but also for ensuring relevance in an ever-changing tech world.
Trends in Mobile Development
Mobile development is constantly evolving. Each year, new trends emerge that can fundamentally change how developers create apps. Let’s explore a few of these trends:
- Cross-Platform Development: There’s a rising preference for frameworks like Flutter and React Native, which allow for the creation of applications that work seamlessly across different platforms. This efficiency can save both time and resources, making it easier for developers to reach a wider audience.
- 5G Technology Integration: With the roll-out of 5G, applications can perform tasks that were impossible or impractical before. High-speed internet can dramatically improve app responsiveness and enrich user experiences, enabling features like augmented reality (AR).
- Focus on User Experience (UX): Developers are increasingly prioritizing UX design, ensuring interfaces are not only visually appealing but also functional. Apps that provide a smooth, intuitive user experience are likely to retain users better than those that don’t.
Emerging Technologies
As we look ahead, it's clear that emerging technologies will shape the future of Android programming. Two standouts in this realm are Artificial Intelligence and the Internet of Things.
Artificial Intelligence
Artificial Intelligence (AI) offers remarkable possibilities in mobile development, particularly within Android programming. One significant aspect is how AI can personalize user experiences through intelligent algorithms. Key Characteristics: AI learns from user behaviors, enabling developers to create highly customized applications. This feature is especially beneficial because it enhances user engagement, leading to better retention rates.
Unique Features: AI integrations can vary from simple recommendation engines to advanced functionalities like voice recognition and natural language processing. However, it’s crucial to balance AI's advantages with its downsides—like ethical concerns over data privacy, which can be a major hurdle for developers to navigate.
Internet of Things
The Internet of Things (IoT) is another major player altering the landscape of Android programming. This technology refers to a network of interconnected devices that collect and share data over the Internet. Key Characteristic: IoT’s capacity for real-time data exchange makes it a powerful tool for developers aiming to create smart applications that can interact with other devices. This is highly beneficial as it opens up avenues for innovative applications in home automation, wearable technology, and various industrial applications.
Unique Features: IoT can facilitate seamless integration with other systems, giving users an efficient and unified experience. However, programmers must also consider potential drawbacks such as increased security risks and the complexity of managing myriad connected devices.
"Staying current with trends and leveraging new technologies is the cornerstone of successful Android programming in the 21st century."
This dedication to future-focused programming will not only enrich users’ experiences but will also equip developers with the necessary skills to thrive in this dynamic field.