AI Programming with Python: A Comprehensive Guide
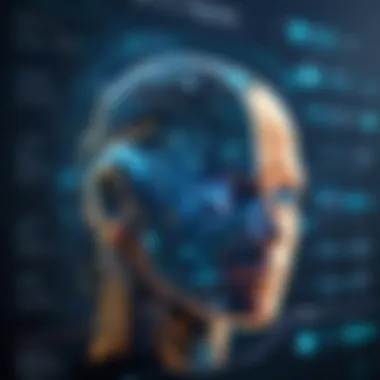
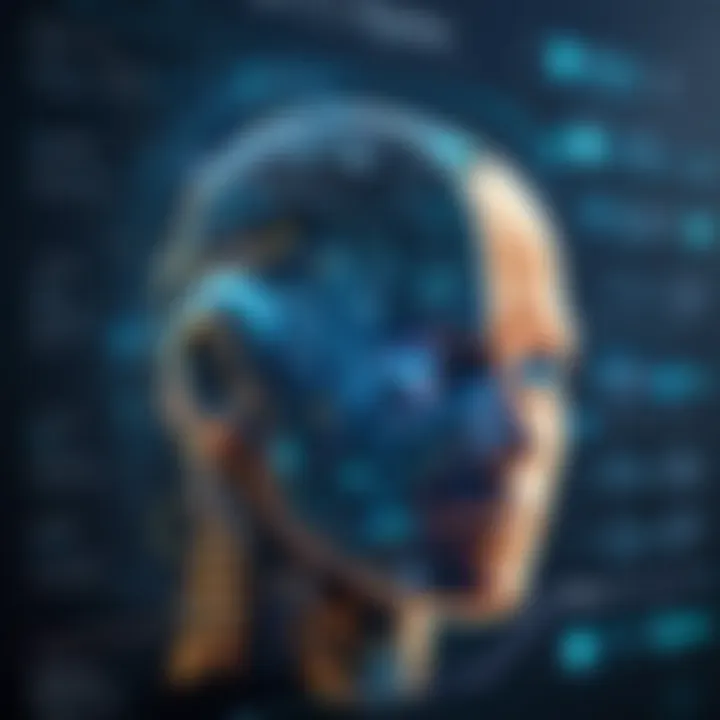
Foreword to Programming Language
Artificial Intelligence (AI) has become an integral part of modern computing. Python is a programming language often associated with AI due to its simplicity and versatility. It simplifies complex tasks and is highly readable. This section explores Python’s history, key features, and its relevance in AI development.
History and Background
Python was created by Guido van Rossum and released in 1991. It aimed to be a clean and easy language. Over time, it gained popularity in various fields, including web development, data analytics, and notably AI. Major libraries like TensorFlow, Keras, and PyTorch have emerged, further establishing Python as a go-to for AI projects.
Features and Uses
Python's major features include:
- Readability: Python's syntax is clear, making it easy for beginners to learn.
- Extensive Libraries: Libraries such as NumPy, Pandas, and scikit-learn facilitate data manipulation and machine learning tasks.
- Community Support: A vibrant community provides a wealth of resources and support.
These features help developers create effective AI solutions.
Popularity and Scope
Python is one of the most popular programming languages today. Its use in AI spans several sectors, from healthcare to finance. According to the TIOBE index, Python's popularity continues to increase, making it a vital skill for aspiring data scientists and AI developers.
Basic Syntax and Concepts
Understanding Python's syntax is crucial for effective programming. This section covers key concepts such as variables, data types, and control structures.
Variables and Data Types
In Python, variables can hold various types of data, including:
- Integers: Whole numbers.
- Floats: Decimal numbers.
- Strings: Text data, enclosed in quotes.
- Booleans: True or False values.
Operators and Expressions
Python supports several operators for mathematical and logical operations. Here are some common ones:
- Arithmetic Operators: +, -, *, /.
- Logical Operators: and, or, not.
Control Structures
Control structures direct the flow of the program. Common control structures include:
- If Statements: Allows for conditional execution.
- Loops: and loops enable repetitive tasks.
Advanced Topics
Delving into advanced topics enhances programming skills. This includes understanding functions, object-oriented programming, and exception handling.
Functions and Methods
Functions are reusable blocks of code. They are defined using the keyword. For example:
Methods are functions associated with an object.
Object-Oriented Programming
Object-oriented programming (OOP) allows for organizing code into objects that can contain data and methods. Essential concepts include:
- Classes: Blueprint for creating objects.
- Inheritance: Mechanism to create new classes based on existing ones.
Exception Handling
Error management is crucial in programming. Python uses and blocks to handle exceptions gracefully. For example:
Hands-On Examples
Practical experience is vital in learning. Below are some programming examples.
Simple Programs
A basic program in Python might look like this:
This outputs a simple greeting.
Intermediate Projects
Building projects enhances understanding. A simple calculator can involve user inputs and basic operations.
Code Snippets
Code snippets are helpful for repeated tasks. For instance, a function for squaring numbers:
Resources and Further Learning
To deepen your Python knowledge, consider these resources:
- Recommended Books and Tutorials: Automate the Boring Stuff with Python, Python Crash Course.
- Online Courses and Platforms: Coursera, Udemy offer structured courses.
- Community Forums and Groups: Reddit and Stack Overflow are great for discussions and support.
Continuous learning is vital in AI programming to keep up with advances in technology.
Prelims to AI Programming with Python
Artificial Intelligence (AI) has emerged as a transformative force across multiple industries. This section serves to illustrate the important foundational aspects of AI programming using Python. By understanding these elements, one might appreciate the depth of the topic and its relevance in today’s digital landscape.
Incorporating AI into applications is no longer a luxury; rather, it is rapidly becoming a necessity. The ability to write programs that can analyze data, learn from it, and make predictions is an essential skill. Python, with its simplicity and flexibility, acts as a preferred language for many AI practitioners and researchers.
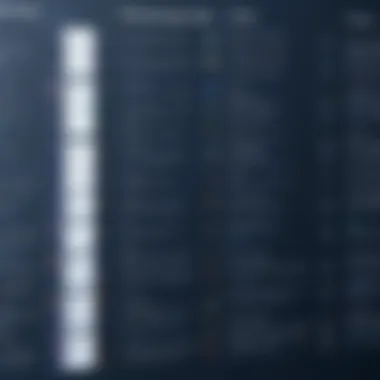
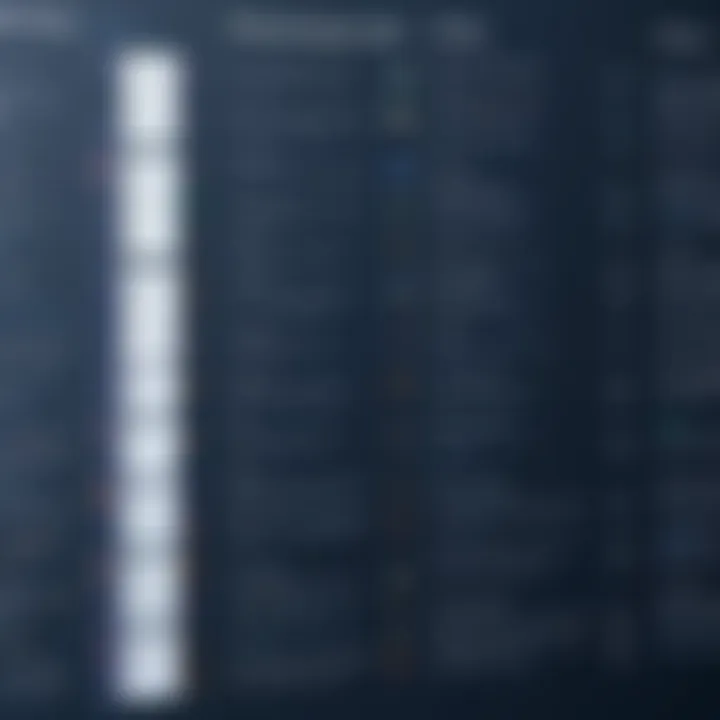
Among the key benefits of pursuing AI programming with Python are the vast libraries and frameworks available. Tools like TensorFlow, Keras, and Scikit-learn allow developers to harness powerful AI capabilities without needing extensive background knowledge in mathematics or complex algorithms. As a result, Python stands out as an accessible gateway for both novices and experienced programmers wanting to delve into AI.
When engaging with AI programming, one must also consider the evolving nature of the field. Understanding the fundamental concepts is essential, as is having the knowledge to adapt when new trends emerge. This guide aims to build a strong intellectual foundation and offer practical insights into AI, ensuring readers are equipped to navigate this exciting domain.
Understanding Artificial Intelligence
Artificial Intelligence refers to the capability of a machine to imitate intelligent human behavior. AI systems can perform complex tasks, such as problem-solving, understanding natural language, and recognizing patterns in data. In essence, AI is a broad field that encompasses various methods and techniques to simulate human-like intelligence.
A commonly explored branch of AI is machine learning, which focuses on building systems that learn from data. This is particularly relevant in a world where data drives decision-making processes across businesses and technology. Familiarity with AI principles can significantly enhance one’s ability to innovate and implement solutions in various domains.
The Role of Python in AI Development
Python plays a crucial role in AI development. Its clear syntax and readability make it an ideal choice for both beginners and professionals. Many programming languages can be used for AI, but Python stands out due to its extensive ecosystem of libraries and frameworks.
The rise of data-centric fields has further solidified Python's status. Libraries like NumPy and Pandas streamline data manipulation, while Matplotlib and Seaborn facilitate effective data visualization. Additionally, frameworks such as TensorFlow and PyTorch have made it easier to create sophisticated algorithms used in AI and machine learning tasks.
Python not only supports various approaches to programming but also encourages collaborative development through open-source initiatives. This aspect further enhances the Python community, sharing knowledge and tools that foster innovation.
In summary, the burgeoning field of AI programming with Python presents unparalleled opportunities for those looking to deepen their expertise and contribute meaningfully to technological advancements.
Setting Up Your Python Environment
Setting up your Python environment is a crucial step in the journey of AI programming. This environment serves as the platform where all coding occurs. The correct configuration ensures smooth development and execution of AI models. It can make a significant difference in learning and productivity. A well-organized Python environment allows for easy access to libraries, tools, and resources necessary for AI programming.
Developing AI applications often involves various libraries that support tasks such as data manipulation, visualization, and machine learning. Thus, attention to detail during the setup phase directly impacts the efficacy of your coding experience. Below are some considerations to keep in mind while setting up your environment.
- Operating System: Ensure that your system supports the latest Python version. Python runs on Windows, macOS, and Linux, but differences exist in library support.
- Python Version: Use a stable version of Python, typically the most recent long-term support version. Versions can introduce new features or deprecate old ones, so choose wisely.
- Package Manager: Utilize a package manager like pip or conda to install necessary libraries easily.
- Environment Management: Consider using virtual environments to separate project dependencies. This keeps libraries organized and avoids conflicts.
Managing your Python environment lays the groundwork for successful AI programming.
Installing Python and Necessary Libraries
Installing Python is the first step toward engaging with AI programming. The installation process varies depending on the operating system. You can download Python from the official website. Once installed, it is essential to confirm the installation by checking the version in the command line. An example command is:
Installing libraries is the next logical step. Libraries expand functionality beyond the core features of Python. Some key libraries for AI development include:
- NumPy: Essential for numerical computations and array manipulation.
- Pandas: Facilitates data manipulation and analysis through data frames.
- Matplotlib: A library for creating static, interactive, and animated visualizations in Python.
- TensorFlow: An open-source framework for building machine learning models.
- Scikit-learn: A library that provides simple tools for data mining and data analysis.
To install these libraries, you can use pip by running commands like:
Choosing the Right IDE for AI Development
The choice of Integrated Development Environment (IDE) plays a significant role in coding efficiency. An IDE provides a user-friendly interface while integrating tools that enhance the programming process.
Several factors influence the choice of IDE:
- Features: Look for features like syntax highlighting, debugging tools, and code completion.
- Ease of Use: A complex IDE might reduce efficiency for beginners. Simplicity can be more advantageous.
- Support for Libraries: Ensure the IDE supports libraries you plan to use for AI development.
Some popular IDEs for Python include:
- PyCharm: Offers great support for web frameworks and data science tools. Its features are tailored for Python.
- Visual Studio Code: A lightweight and versatile editor that supports various languages. Extensions enhance its capabilities, especially for AI libraries.
- Jupyter Notebooks: Particularly valuable for data science, allowing for interactive coding and visualization.
Selecting the right IDE impacts not just coding efficiency, but also the learning curve for new developers. Consider trying out a few options to find which fits your workflow the best.
Fundamental Libraries for AI Programming
In the realm of AI programming with Python, foundational libraries hold significant importance. These libraries not only enhance efficiency but also simplify complex tasks. A well-chosen library can streamline workloads, whether in data manipulation, numerical computations, or visualization. The right tools can accelerate the development process, allowing developers to focus on solving problems rather than reinventing the wheel.
Adopting these fundamental libraries early in your programming journey can lead to improved project outcomes. They provide readers and developers with structured approaches to tackle substantial tasks in AI, paving the way for innovative solutions.
NumPy: The Foundation of Numerical Computing
NumPy stands as a cornerstone in numerical computing. This powerful library facilitates efficient operations on large arrays and matrices. It provides diverse mathematical functions for performing operations on these data structures. Developers rely on NumPy for tasks such as linear algebra, Fourier transforms, and random number generation.
Key benefits of NumPy include:
- Speed: Its performance often surmounts that of traditional Python lists.
- Functionality: It offers a vast array of operations, reducing the need for external tools.
- Integration: Other libraries, such as Pandas and Matplotlib, depend on NumPy for data structures.
Using NumPy is straightforward. For instance, creating a simple array can be done with:
This example illustrates the simplicity and power of NumPy in handling numerical data.
Pandas: Data Manipulation and Analysis
Next, Pandas emerges as a vital library for data manipulation and analysis. This library introduces data structures and functions designed to work with structured data seamlessly. The primary data structures, Series and DataFrame, allow users to conduct complex data manipulations straightforwardly.
Pandas is beneficial in various scenarios. Key features include:
- Data Cleaning: It offers tools for handling missing data and transforming datasets into usable forms.
- Data Aggregation: It allows users to summarize and group data efficiently.
- File I/O: Pandas can read from and write to multiple file formats, such as CSV, Excel, and SQL databases.
An example of loading data from a CSV file is as simple as:
This showcases how easily you can start manipulating data with Pandas.
Matplotlib and Seaborn: Data Visualization Tools
For visualizing data, Matplotlib and Seaborn serve as indispensable tools in the AI programmer’s toolkit. Matplotlib provides the basis for creating static, animated, and interactive visualizations in Python. It offers extensive capabilities and customization options, making it a preferred choice for many data scientists.
On the other hand, Seaborn enhances Matplotlib’s functionality. It is specifically designed for statistical plotting, allowing for more beautiful and informative graphics.
Consider the benefits of these libraries:
- Versatility: Both libraries allow for a wide range of plotting options.
- Customization: Developers can create personalized visualizations easily.
- Integration: They work seamlessly with NumPy and Pandas, facilitating a smooth workflow.
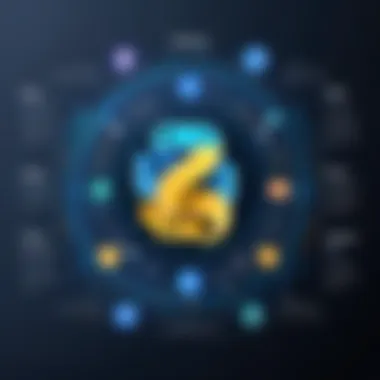
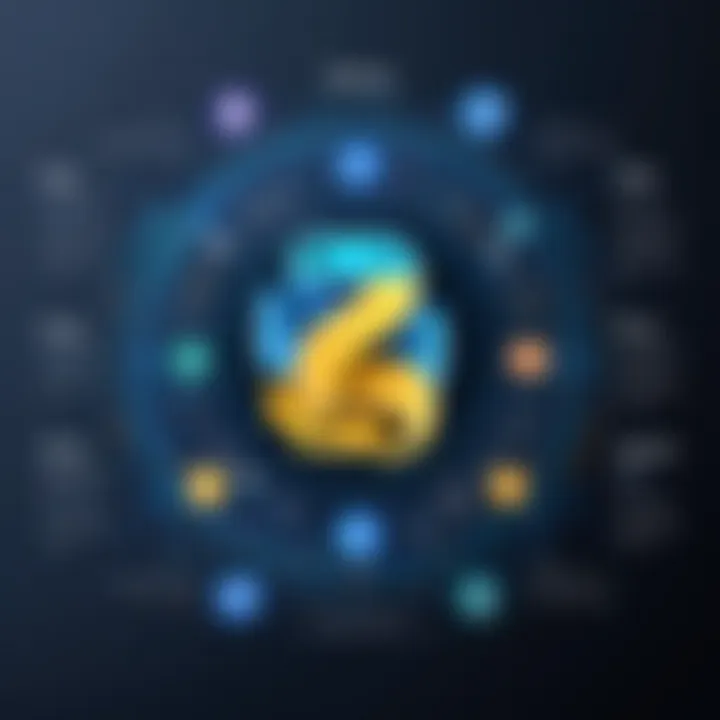
Here’s a simple example of creating a line plot with Matplotlib:
This underscores how straightforward it is to generate useful visualizations in Python.
Machine Learning Fundamentals
Machine learning is a vital area in the field of artificial intelligence. It empowers computer systems to learn from data, identify patterns, and make decisions with minimal human intervention. Understanding machine learning fundamentals is essential for anyone looking to develop automated systems and applications. This section lays the groundwork for those who want to harness the potential of Python to implement machine learning techniques effectively.
Supervised Learning: Concepts and Algorithms
Supervised learning refers to the type of machine learning where the model is trained on a labeled dataset. A labeled dataset consists of input-output pairs, the input being the features and the output being the target label. This approach allows the model to learn the mapping from inputs to outputs, making it crucial for tasks such as classification and regression.
Common algorithms include:
- Linear Regression
- Logistic Regression
- Decision Trees
- Support Vector Machines
- Neural Networks
Each algorithm has its strengths and weaknesses. For example, decision trees are easy to interpret, while neural networks tend to perform better on complex tasks but require a larger amount of data and computational resources.
Unsupervised Learning: An Overview
Unsupervised learning, unlike supervised learning, is used with datasets that do not have labeled outputs. The algorithm attempts to identify hidden patterns or intrinsic structures within the data. This technique is useful for tasks such as clustering and dimensionality reduction.
Common techniques within unsupervised learning include:
- K-Means Clustering
- Hierarchical Clustering
- Principal Component Analysis (PCA)
Unsupervised learning is particularly beneficial when the goal is to explore data, perform data compression, or prepare datasets for further analysis without prior knowledge of outcomes.
Reinforcement Learning: Principles and Applications
Reinforcement learning is distinct from both supervised and unsupervised learning. In this paradigm, an agent learns to make decisions through trial and error in an environment. The agent receives rewards or penalties based on its actions, thus informing which actions yield the best results. This feedback loop helps the agent improve its performance over time.
Key concepts in reinforcement learning include:
- Agents and Environments
- Actions and States
- Rewards
- Policies
- Value Functions
Applications of reinforcement learning are abundant. They include game development, robotics, and even complex decision-making scenarios in finance and marketing.
Understanding these machine learning fundamentals sets the stage for more advanced applications and exploration in the realm of artificial intelligence. The knowledge gained in this section will empower students and aspiring developers to approach machine learning projects with confidence.
Deep Learning with Python
Deep learning is a significant area within artificial intelligence, focusing on algorithms inspired by the structure and function of the brain. This advanced computing technique leverages neural networks to perform tasks such as image recognition, natural language processing, and even game playing. Python plays a pivotal role in deep learning with its powerful libraries, such as TensorFlow and PyTorch, which streamline the development of complex models. These libraries facilitate the training, optimization, and deployment of deep learning architectures with ease, making Python a preferred language for both researchers and practitioners in this domain.
The benefits of leveraging deep learning methods include the ability to work with vast amounts of unstructured data and the potential to achieve higher accuracy in predictions. However, this approach comes with considerations such as the need for substantial computational resources and expertise in managing and tuning models effectively. Hence, an understanding of deep learning with Python is essential for anyone looking to excel in AI programming.
Prelims to Neural Networks
Neural networks are at the heart of deep learning. They are structured in layers, consisting of interconnected nodes or neurons. The architecture typically includes an input layer, one or more hidden layers, and an output layer. Each connection between nodes has an associated weight that adjusts as learning occurs. This process allows the network to recognize patterns and make intelligent decisions based on input data.
Key components of a neural network include:
- Activation Functions: These determine whether a neuron should be activated or not, impacting how the network learns from data. Common examples involve ReLU (Rectified Linear Unit) and Sigmoid functions.
- Loss Functions: These assess how well the neural network's predictions align with actual results, guiding the optimization of weights.
- Optimization Algorithms: These include techniques like Stochastic Gradient Descent (SGD) that update weights to minimize the loss function during training.
Learners should understand these elements to build effective neural networks.
Convolutional Neural Networks for Image Processing
Convolutional Neural Networks (CNNs) are specifically designed for processing structured grid data, such as images. Unlike traditional neural networks, CNNs utilize convolutional layers that apply filters to input data, thereby detecting patterns like edges and textures. This capability significantly enhances the performance of image recognition tasks.
The key features of CNNs are:
- Convolutional Layers: These layers apply multiple filters to capture different features from the input. As the layers deepen, CNNs can recognize more complex patterns.
- Pooling Layers: Pooling reduces the dimensionality of the feature maps, retaining essential information while minimizing computation.
- Fully Connected Layers: The final layers convert the extracted features into output classifications or regression outputs.
Given the rise of applications in domains like healthcare and autonomous vehicles, proficiency in using CNNs with Python is a valuable skill for aspiring developers.
Recurrent Neural Networks and Sequence Data
Recurrent Neural Networks (RNNs) are designed to work with sequential data where previous inputs influence current outputs. This characteristic makes RNNs ideal for tasks like language modeling, time series prediction, and video analysis. RNNs have feedback connections, enabling them to maintain memory of past inputs.
Key concepts include:
- Long Short-Term Memory (LSTM): This architecture addresses the limitation of standard RNNs, namely, their difficulty in learning long-range dependencies. LSTMs allow for the retention of important information over long sequences, making them suitable for tasks like machine translation.
- Gated Recurrent Units (GRU): A simpler alternative to LSTMs, GRUs require fewer parameters and can be more efficient for certain tasks.
Understanding RNNs and their variants is essential for handling complex data types and improving performance in applications requiring sequence learning.
Natural Language Processing with Python
Natural Language Processing (NLP) has emerged as a pivotal field within artificial intelligence, particularly when leveraging Python as the programming language. NLP focuses on the interaction between computers and human language, enabling machines to interpret, understand, and respond to textual data. This capability is integral for creating applications that require understanding of human communication, thus making Python an essential tool given its rich ecosystem of libraries and frameworks that ease the development process.
The importance of NLP in this context cannot be understated. As society increasingly relies on digital communication, the necessity for computers to understand language is paramount. Python offers comprehensive libraries such as NLTK, SpaCy, and TextBlob, which streamline tasks related to language processing. These tools help programmers extract useful information from text, perform sentiment analysis, and even develop chatbots that mimic human conversation.
Moreover, the advantages of employing Python for NLP include:
- Ease of Use: Python’s syntax is straightforward and intuitive, which allows for rapid prototyping and iteration.
- Community Support: A vast community exists to support developers working on NLP, providing forums, tutorials, and libraries.
- Integration Capabilities: Python can easily integrate with other languages and platforms, making it versatile for different projects.
As we delve into specific components of NLP, it is crucial to understand foundational aspects that can significantly influence the effectiveness of AI applications in language processing.
Text Processing and Feature Extraction
In NLP, text processing is a foundational step that involves converting raw text into a format that is understandable by machines. This includes tasks like tokenization, stemming, and lemmatization. Each of these processes helps in reducing the complexity of text data, thus enabling more straightforward analyses.
Feature extraction then takes this a step further, identifying and selecting essential features from the processed text. This could involve statistical methods or machine learning techniques to gauge which aspects of a text are most significant for a given project.
Common approaches include:
- Bag of Words: This method represents text data in the form of a matrix, counting the number of occurrences of each word in the document.
- TF-IDF (Term Frequency-Inverse Document Frequency): This technique assigns weights to each word based on its frequency in a document relative to its frequency across other documents.
These methods are critical in preparing the data for tasks such as classification or regression.
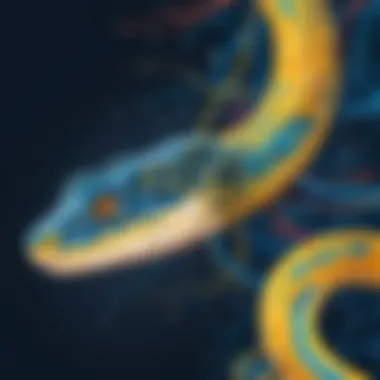
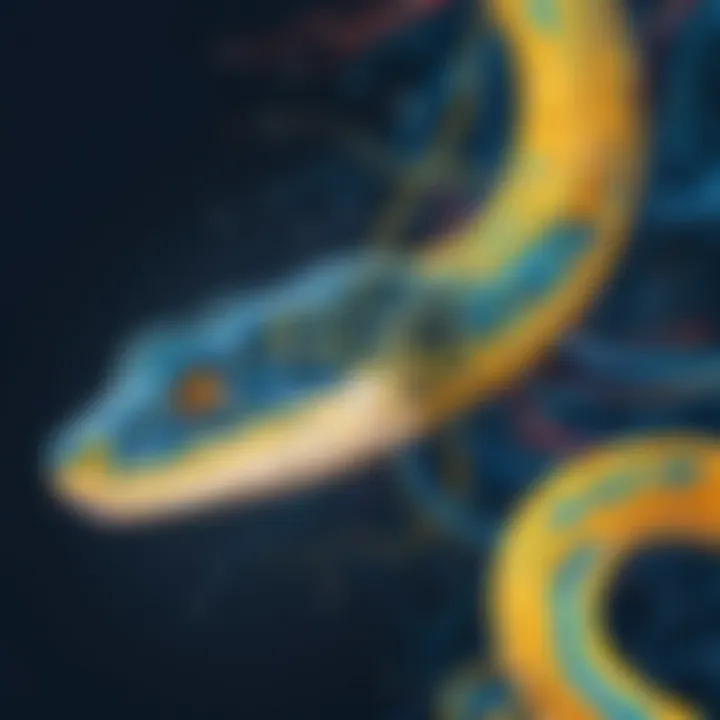
Sentiment Analysis Techniques
Sentiment analysis is another significant application of NLP that uses machine learning to evaluate opinions expressed in text. This technique can determine whether the sentiment behind a piece of writing is positive, negative, or neutral. It has applications in various domains, from market research to customer feedback analysis.
To implement sentiment analysis using Python, several techniques may be employed:
- Lexicon-Based Approaches: These rely on predefined lists of words associated with positive or negative sentiments.
- Machine Learning Models: Supervised models can be trained using labeled data, enhancing accuracy by learning from examples.
- Deep Learning Models: Advanced models, such as LSTMs or BERT, provide state-of-the-art performance by taking context into account.
The choice of approach depends on the nature of the data and the specific requirements of the project.
Chatbot Development Using NLP
The design and implementation of chatbots represent a trending use case for NLP. Chatbots are programs that can simulate conversations with users, providing a user-friendly interface for accessing information and services.
Using Python, developers can build chatbots that can understand and process natural language input via libraries like Rasa or ChatterBot. Key considerations include:
- Intent Recognition: Understanding what a user intends to achieve with their input is crucial for a chatbot's effectiveness.
- Entity Recognition: Identifying specific data points in user requests enhances the bot's ability to execute tasks accurately.
- Response Generation: The ability to generate human-like responses is vital. This can range from pulling predefined answers to dynamically creating responses based on context.
As the field of NLP expands, so too do the capabilities of chatbots, with applications across industries including healthcare, finance, and customer service.
"NLP transforms the way we interact with technology, seamlessly blending human language with machine understanding."
AI Programming Best Practices
AI programming best practices are essential for developers in the field of artificial intelligence. Following these practices improves the quality of the code and contributes to the overall effectiveness of AI systems. These practices enhance maintainability, facilitate collaboration, and minimize errors. They also ensure that AI models produce reliable and reproducible results. In this section, we will discuss important aspects like code readability and documentation, version control with Git, and testing and validation of AI systems.
Code Readability and Documentation
Code readability is crucial in programming for many reasons. Well-structured code allows for easier understanding by others, both in collaborative projects and future self-development. For instance, using consistent naming conventions improves clarity. A straightforward code layout can help to quickly catch issues or bugs during reviews.
Documentation plays a significant role alongside readability. It offers insights into the code's purpose, functionality, and structure. Good documentation should include:
- Comments that explain complex logic or important decisions made in the code.
- README files that provide an overview and guide on how to set up and use the project.
Adhering to these principles ensures that anyone who works with the code, including future developers or machine learning engineers, can easily grasp the project scope and functionality.
Version Control with Git
Version control is another key practice in AI programming. Git is commonly used for this purpose. It allows developers to track changes, collaborate effectively, and manage code versions systematically. When dealing with complex AI models that may undergo numerous iterations, using Git helps maintain organization.
A few benefits to consider when using Git include:
- Branching allows you to work on multiple features or experiments concurrently without disrupting the main codebase.
- Commit messages help document what changes were made and why, creating a clear history of development.
For effective collaboration, it is advisable to adopt a Git workflow, such as Feature Branch Workflow or Git Flow. This way, you and your team can work together seamlessly, making it easier to integrate various contributions into the final project.
Testing and Validation of AI Systems
Finally, testing and validation are essential steps in the AI development lifecycle. Reliable AI systems must undergo rigorous testing to ensure they perform as expected under various conditions.
Testing may include:
- Unit tests to check if individual components work correctly.
- Integration tests to ensure that the interaction between components is successful.
- Performance tests to validate the efficiency and speed of the AI model.
Validation also requires thorough evaluation against established benchmarks. This helps confirm that the model's predictions are accurate and relevant, promoting trust in its use in real applications.
"Testing is the only way to ensure that the AI models behave correctly in deployment."
In summary, adhering to AI programming best practices not only improves individual code quality but also fosters a positive environment for collaboration and innovation. By focusing on code readability and documentation, implementing robust version control with Git, and establishing comprehensive testing and validation strategies, developers can build more reliable AI systems.
Case Studies: Successful AI Applications in Python
Examining real-world implementations of AI in Python sheds light on its practicality and effectiveness across various industries. Through case studies, it is possible to observe how different sectors have harnessed Python's capabilities to solve complex problems, improve efficiencies, and drive innovations. These examples reveal the versatility of Python in developing AI applications, demonstrating its relevance in both academic and professional contexts. As technology evolves, understanding successful applications also helps emerging programmers to grasp the challenges and methodologies relevant to AI programming.
Healthcare: Diagnostic Predictive Models
In healthcare, Python is increasingly used for developing predictive models that can enhance diagnostic accuracy. One significant application is in the realm of patient monitoring and disease detection. For example, employing machine learning algorithms, hospitals can analyze patient data to predict potential health issues. Models trained on historical patient records can forecast outcomes, allowing physicians to take preventive measures.
Python libraries such as Scikit-learn are essential in crafting these predictive models. They offer tools for regression analysis, classification, and clustering, leading to improved patient outcomes. Moreover, some clinical studies have shown that these predictive models can reduce hospital readmission rates by recognizing at-risk patients early.
Finance: Algorithmic Trading Systems
Algorithmic trading represents another area where Python excels. Financial institutions leverage Python's robustness to automate trading strategies. The language allows for easy integration of APIs to pull real-time data and execute trades autonomously based on pre-defined conditions.
For instance, quantitative traders often employ libraries like Pandas and NumPy for analyzing stock data, while backtesting results against historical performance using frameworks such as Zipline. This approach has enabled firms to optimize their trading strategies, reducing human error and increasing efficiency. The ability to analyze vast amounts of data in a short period gives firms a competitive edge in the fast-paced financial markets.
Retail: Personalized Recommendation Engines
In the retail sector, personalized recommendation engines built with Python have transformed how companies interact with customers. E-commerce giants like Amazon utilize sophisticated algorithms to analyze user behavior and preferences, offering tailored product suggestions based on prior purchases and browsing history.
Using libraries like TensorFlow, retailers can create deep learning models that process vast datasets to identify patterns in consumer behavior. This contributes to increased sales and enhanced customer satisfaction, as users receive relevant product recommendations. Furthermore, understanding consumer trends through these AI-driven insights allows retailers to adjust their marketing strategies more effectively.
Case studies in healthcare, finance, and retail illustrate Python's versatility in AI programming. Each industry benefits uniquely from tailored AI applications that address specific needs.
Through these case studies, it becomes evident that Python's role in AI is significant. The language facilitates the development process and provides the tools necessary for constructing sophisticated programs that can solve real-world problems across diverse fields.
Future Trends in AI and Python Programming
The realm of artificial intelligence is evolving at a remarkable pace, and Python plays an instrumental role in this transformation. Understanding the future trends in AI and Python programming is crucial for both developers and organizations aiming to stay competitive. As new technologies emerge, practitioners must adapt to these changes. This section delves into significant trends that are shaping the future landscape of AI and its integration with Python.
The Growth of AI Ethics
With the power of AI comes the responsibility of ethical considerations. The growth of AI ethics is becoming a focal point in discussions surrounding the development and deployment of AI technologies. Ethical concerns include privacy, bias, accountability, and transparency. As AI systems increasingly influence decision-making processes in various sectors, the demand for ethical frameworks that govern their use becomes essential.
The integration of ethics in AI programming involves educating developers on the social implications of their work. This includes understanding how algorithms can unintentionally perpetuate bias if not carefully managed. Python, with its extensive libraries and frameworks, provides tools to analyze data for fairness and to create models that prioritize ethical considerations.
Future trends will likely see organizations prioritizing ethical AI. This can mean integrating comprehensive audits into their development processes to ensure that their systems not only perform well but also uphold societal values. Practitioners will need to be equipped with skills that help them identify ethical dilemmas during programming and application of AI.
"As AI becomes more autonomous, the ethical considerations behind its development will also need to evolve."
The Integration of AI in Internet of Things
The integration of AI into the Internet of Things (IoT) marks another significant trend. IoT devices generate vast amounts of data, which AI can analyze to derive insights and make predictions. This relationship enhances both the efficiency of data use and the capabilities of devices.
Python is particularly well-suited for IoT applications due to its simplicity and the robust libraries available for machine learning and data analysis. By leveraging Python, developers can create AI algorithms that improve device functionality and user interaction. For example, smart home devices can use AI to learn patterns in user behavior and adjust settings accordingly.
Moreover, the synergy between AI and IoT leads to advancements in automation across various fields, including healthcare, agriculture, and supply chain management. Developers need to stay updated on the latest libraries and tools that facilitate the implementation of AI within IoT ecosystems. This trend is set to redefine numerous industries by creating smarter environments that can react to real-time data.
In summary, understanding these future trends in AI and Python programming is essential. The growth of AI ethics will shape how developers approach their projects. Concurrently, the integration of AI in IoT will offer exciting possibilities for innovation. Staying ahead in these areas will ensure that practitioners remain relevant and effective in their work.