Mastering Advanced Python: Decorators to Metaclasses
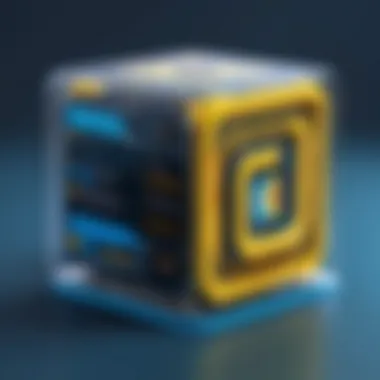
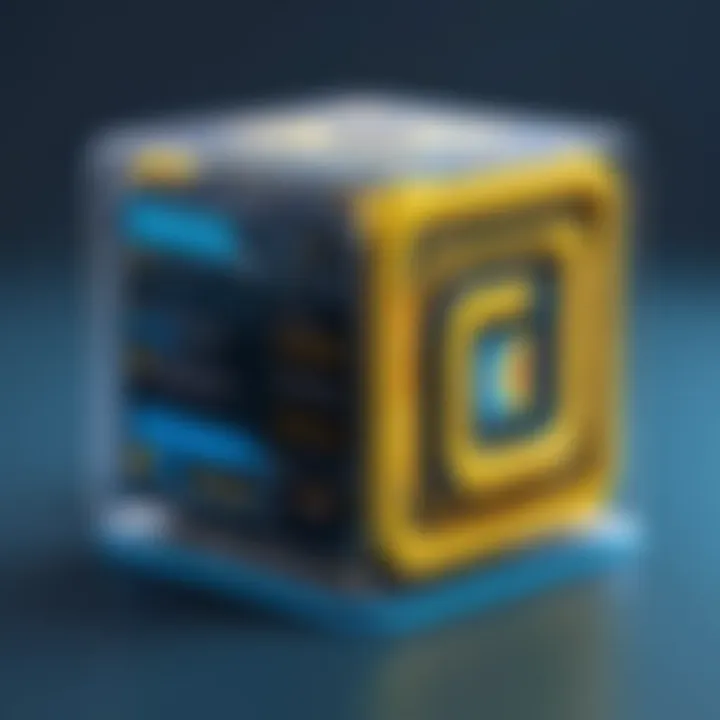
Intro
In the realm of programming, Python stands out as a flexible and powerful language. Widely regarded for its clean syntax and versatility, it accommodates both beginners and seasoned programmers alike. However, diving deeper into its advanced concepts can truly elevate one's coding prowess. This exploration focuses on various intricate topics, providing insight that goes beyond mere surface-level understanding.
History and Background
Python was conceived in the late 1980s by Guido van Rossum. The language was designed with a focus on code readability and simplicity, making it particularly appealing for those just starting their programming journey. Over the years, Python has evolved significantly, fostering a rich community that contributes to its expansion through libraries and frameworks. Its design philosophy emphasizes the importance of streamlined code, which resonates well with both new and experienced developers.
Features and Uses
Python's strength lies in its array of features. Key among them is its support for multiple programming paradigms, including procedural, object-oriented, and functional programming. This flexibility allows developers to tackle a wide variety of applications ranging from web development to data analysis and artificial intelligence. Features such as dynamic typing and automatic memory management further enhance its usability, making Python a go-to language for many.
Popularity and Scope
The popularity of Python has surged in recent years, penetrating various sectors from academia to industry. According to various surveys, its user base continues to expand, solidifying its position as one of the top programming languages in the world. From small startups to tech giants like Google and Facebook, organizations adopt Python for its functionality and ease of integration. The language's extensive libraries and frameworks bolster its appeal, offering robust tools for developers tackling complex problems.
"Python is not just a language, it's an ecosystem that fosters creativity and efficiency."
In summary, understanding Python's background, features, and scope sets the stage for a deeper dive into its more advanced concepts. As we move forward, we will explore practical applications and techniques that truly harness Python's power.
Understanding the Importance of Advanced Python Concepts
In the world of programming, particularly with Python, learning the more intricate aspects of the language is no mere luxury; it's a necessity. Understanding advanced Python concepts allows not just for improved coding efficiency, but it also opens the doors to creating highly optimized and scalable applications. As Python continues to gain traction across various domains such as data science, web development, and artificial intelligence, the need to grasp advanced functionalities becomes increasingly evident.
Diving into these complex areas cultivates a mindset geared toward problem-solving and creativity. When one moves beyond the elementary syntax and basic constructs, the journey into advanced topics like decorators, generators, and context managers develops skills that can significantly enhance both performance and maintainability of code. This elevated proficiency leads to the ability to write cleaner, more efficient code that not only performs tasks but also adheres to high standards of readability and reusability.
The significance of advanced programming cannot be overstated. Here are a few key considerations:
- Increased Efficiency: Advanced constructs can optimize code execution. For example, generators save memory while processing data by yielding items one at a time, rather than loading entire datasets into memory.
- Improved Code Quality: Understanding and utilizing decorators means writing cleaner, more manageable code. They allow for the separation of concerns, making it easier to apply cross-cutting functions like logging or authentication without cluttering the core business logic.
- Community and Ecosystem Benefits: The Python community is rich with libraries and frameworks that leverage advanced features. By mastering these concepts, programmers can contribute more effectively to open-source projects and collaborate with others in the community.
"With great power comes great responsibility." - A reminder that as one gains knowledge in advanced programming, itâs essential to utilize it wisely.
Defining Advanced Programming
Advanced programming can be seen as the ability to write code that effectively solves complex problems while utilizing the full range of features offered by a language. In Python, this includes not just understanding the basic syntax but being familiar with aspects such as data structures, algorithms, and design patterns that inform better programming practices. Advanced programming often requires a paradigm shift, where one not only learns how to implement features but also considers how those features interact with each other and impact the overall efficiency of the application.
For instance, familiarity with functional programming concepts can influence how one thinks about data transformation and manipulation. The use of lambdas and map/filter functions allows for elegant one-liners that can perform operations succinctly. Similarly, understanding object-oriented principles in depth enables programmers to apply such methods effectively, leading to a more seamless development workflow in complex projects.
Why Move Beyond the Basics?
Embracing aspects of advanced programming is essential for several reasons:
- Depth of Understanding: Basics provide the foundation, but advanced topics deepen oneâs understanding of Python. This knowledge can help in troubleshooting and optimizing existing code.
- Versatile Skillset: Mastering advanced concepts enhances adaptability in various projects, whether data-oriented or problem-solving focused. It increases marketability in the job landscape.
- Community Contributions: With a solid understanding of advanced topics, aspiring developers can more readily contribute to libraries, frameworks, or even create their own. This fosters a higher level of engagement with the community.
- Career Advancement: In the tech industry, employers value candidates who can work independently and tackle challenges without constant oversight. As knowledge of advanced concepts can prove beneficial in task management, it often leads to better career advancement opportunities.
Moving beyond the basics is akin to leveling up in a video game. Each advanced concept learned unlocks more potential and a greater understanding of how things work under the hood. Thus, diving into advanced Python concepts doesnât just equip developers with tools but rather empowers them to harness the full capabilities of the language.
Decorators in Python
Decorators are like the icing on a cake in the realm of Python programming. They offer a way to modify or enhance the behavior of functions or methods without altering their actual code. This flexibility is crucial, especially in larger projects, where adhering to the DRY principleâDon't Repeat Yourselfâis essential for maintainability and clarity. By using decorators, developers can easily apply enhancements uniformly across various functions, making the code cleaner and more efficient.
Function Decorators Explained
To start off, let's get our heads around function decorators. They are simply functions that receive another function as an argument and extend or change its behavior. Think of them as a fancy wrapper. When you define a decorator, you typically use the syntax right before the function definition.
Here's a simple example:
In this snippet, enhances the function by adding print statements before and after its execution. This is a common usage pattern, where the decorator enhances or augments the function's behavior without altering its code directly.
Class Decorators and Their Usage
Now let's look at class decorators. These are somewhat similar to function decorators; however, they focus on classes instead. Class decorators can be useful for modifying or extending classes without changing their code base directly.
Imagine you have a class responsible for logging:
In this case, the decorator captures when an instance of is created, allowing you to add logging functionality unobtrusively. It keeps your code organized and simplifies debugging and tracking.
Practical Examples of Decorators
As we navigate through the practical landscape of decorators, a few examples can shed light on their versatile role.
- Access Control: Use decorators to restrict access based on roles. For instance, you can create a decorator to check if a user has the right permissions before executing a function.
- Memoization: This is an optimization technique that caches results of expensive function calls. If a function is called again with the same inputs, it returns the cached result instead of recalculating it. Here's how you might do it:
- Timing Functions: You can measure the execution time of a function. This can be useful in optimizing code performance. Simple as a pie, a timing decorator might look like this:
Decorators serve not just to enhance your coding experience but also to promote code quality. They wrap utilities in a neat package while enhancing reusability and readability. Being well-versed in decorators is a stepping stone to becoming proficient in Python programming.
Generators: Efficient Data Processing
Generators are a powerful feature in Python that facilitate efficient data handling. They allow a programmer to produce a sequence of results lazily, meaning that values are generated one at a time and only when needed. This system can hugely improve performance, especially with large datasets, as it saves on memory usage. Instead of loading an entire dataset into memory, a generator fetches data piece by piece, reducing the strain on system resources.
The elegance of generators lies in their simplicity. They enhance code readability and maintainability, offering a more intuitive way to handle iteration compared to traditional methods.
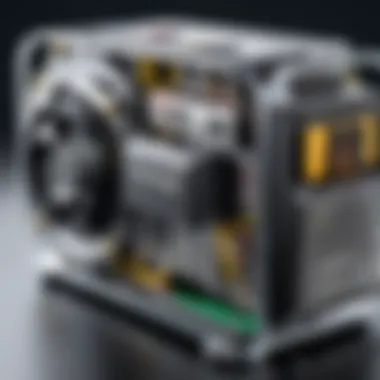
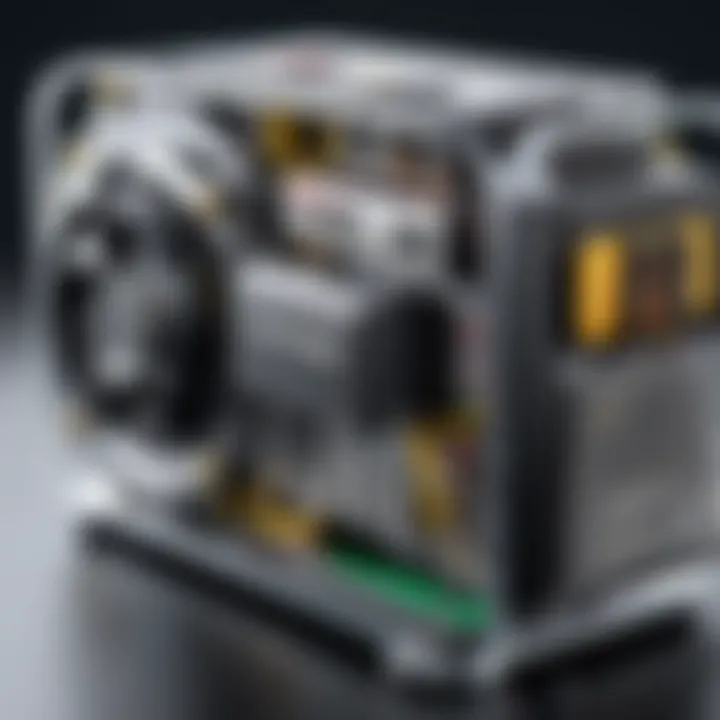
Understanding Yield and Its Mechanism
At the heart of generator functions is the yield statement. When a function is called that includes yield, it doesnât run immediately. Instead, it returns a generator object that can be iterated over. Each invocation of the generator will execute the function and run until it hits the next yield statement, at which point it pauses and sends a value back. This process continues until the function terminates.
A simple example can clarify this:
When you call , it creates a generator object. Each call to retrieves the next number in the sequence until it reaches the end. If you were to wrap this in a loop:
This prints the numbers 1 to 5, one at a time, demonstrating how yield helps control the flow of data generation.
Creating Generator Functions
Creating a generator involves using the yield statement instead of return. Unlike normal functions that return data and terminate, generator functions maintain their state between calls. This characteristic is particularly useful in scenarios where large amounts of data are involved, since you can work with chunks of data while keeping other information readily available instead of re-fetching or re-creating it every time.
Hereâs another simple case:
This code snippet will yield the Fibonacci sequence, generating each number on the fly without creating a full list. Thus, for a large Fibonacci number, you wonât end up exhausting your system.
Use Cases for Generators
Generators are ideal for various scenarios, including:
- Iterating through large files: Instead of loading an entire file into memory, you can process it line-by-line. For example:
- Web scraping: You can effectively scrape and process data without overwhelming memory resources, yielding results as they are fetched.
- Real-time data: When working with sensors or streaming information, generators can handle incoming data continuously without losing performance.
- Data pipelines: For scenarios that involve processing data through different functions, you can create a pipeline of generators for smoother transitions.
In summary, the advantages of using generators are manifold. They don't just optimize performance; they also clarify and simplify your code, making tasks such as dealing with large datasets or streaming data manageable and efficient. To grasp this concept fully, examining the yield statement and practice writing your own generator functions is crucial.
Context Managers: Managing Resources Wisely
In the realm of programming, how you handle resources, like files or network connections, is pivotal. Context managers are a nifty feature in Python that help ensure resources are properly managed. They simplify code and make it cleaner by handling setup and teardown logic automatically. This not only makes your code look more professional but also helps prevent resource leaks, which can be a common pitfall for programmers, especially when working with multiple resources concurrently.
With context managers, you're ensuring that resources are properly allocated and released. This can give you peace of mind, knowing that your programs won't crash because a file wasn't closed properly, or a database connection was left hanging. This section delves into the purpose, creation, and effective usage of context managers in Python.
The Purpose of Context Managers
Context managers serve a specific and crucial purpose in programming. They fundamentally manage the acquisition and release of resources neatly. The primary function is to ensure that setup and cleanup code can be bundled together, making programs more reliable. For instance, consider file handling: rather than manually opening and closing files, context managers abstract this process, ensuring that files are closed properly even if an error occurs.
Here are some key elements to consider regarding context managers:
- Automatic Resource Management: They automate the process of allocating and deallocating resources.
- Improved Code Readability: Using context managers can make it clear at a glance where resources are being managed.
- Error Handling: They simplify error handling, ensuring that resources are cleaned up even in the face of unexpected events.
"The key to smart resource management lies in automation. Context managers shine in this aspect: they ensure resources are handled without you lifting a finger on cleanup."
Creating Context Managers with Classes
Creating context managers can be done either with functions or classes, but using classes offers more flexibility and control over how resources are managed. To create a context manager using a class, you need to implement two magic methods: and .
The method is what runs when the execution flow enters the context of the manager. Conversely, the method is called when the flow exits the context, allowing for the cleanup tasks to be executed.
Here's a simple example showing how to create a context manager:
In this example, when is called, the method executes, acquiring the resource. After the block of code under finishes, automatically runs, ensuring resources are cleaned up.
Using the 'with' Statement
One can't discuss context managers without mentioning the statement. This is the heart and soul of using context managers in Python. The statement streamlines resource management by ensuring that resource release happens reliably, even if exceptions occur within the block.
Hereâs why the statement is so advantageous:
- Clarity: It makes it clear which section of your code manages the resources.
- Safety: It diminishes the risk of forgetting to release resources.
- Error Handling: It ensures that any errors within the context are handled appropriately without leaving resources in an uncertain state.
Consider this simple file handling example:
In this code, if the file does not open correctly, or another error happens, Python will still handle closing the file properly. Using the statement creates a safer, more readable codebase â one that you will appreciate as your projects grow and complexity escalates.
In summary, context managers are an invaluable tool in Python programming, helping to streamline resource management, enhance code clarity, and prevent resource leaks.
Metaclasses: Customizing Class Creation
Metaclasses are like the unsung heroes of Python programming. They're not often talked about in casual conversations but hold a remarkable ability to govern how classes are created. Grasping metaclasses allows programmers to mold their classes in extraordinary ways, opening doors to sophisticated programming practices. The importance of understanding metaclasses lies in their capability to enhance code functionality, enforce constraints, and provide a foundation for implementing design patterns.
When we dive into metaclasses, we hit upon the essentials of code maintainability and extensibility. Using them effectively means that one can easily change behavior without modifying each individual class. Imagine having a shared behavior applied uniformly across numerous classes without redundancy â that's the power of metaclasses.
What Are Metaclasses?
At its core, a metaclass is a class of a class. If you're familiar with how classes define the properties and behaviors of objects, metaclasses do the same thing for classes themselves. In simpler terms, while regular classes instantiate objects, metaclasses instantiate classes. That might sound a bit like playing with words, but it has substantive implications.
Every class in Python is an instance of a metaclass. If you donât actively specify a metaclass, Python defaults to using its built-in . However, crafting a custom metaclass gives you control over class instantiation, and it allows programmers to modify class definitions at creation time.
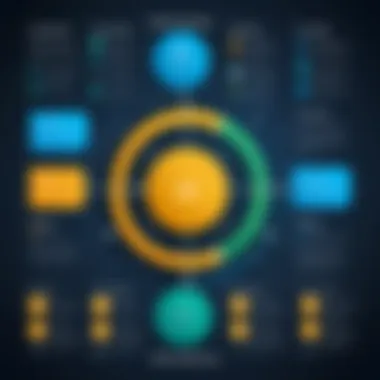
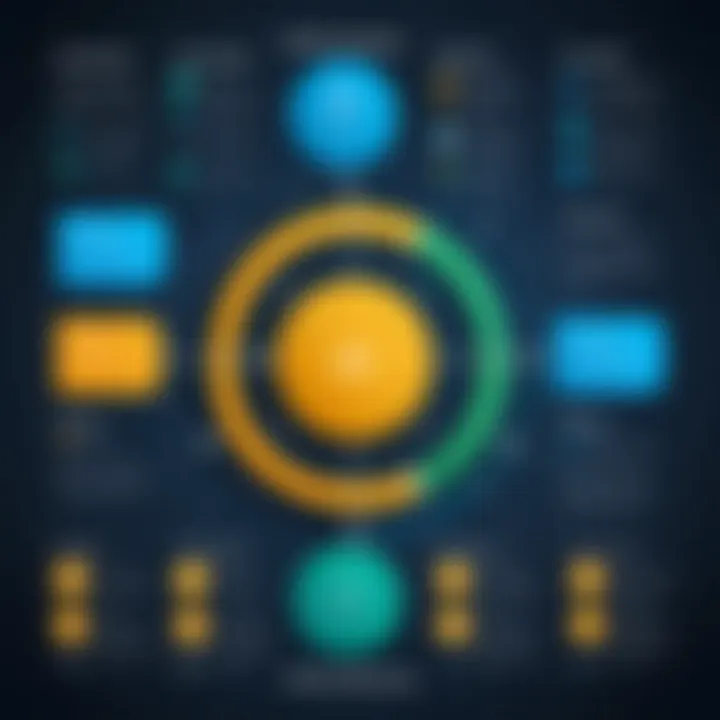
"Metaclasses are a kind of 'class factory' â they allow you to tweak the class creation process."
When you work with metaclasses, you're dealing with an advanced tool, something that might not be necessary for every project, but it provides powerful customization options for specialized needs.
Defining Your Own Metaclasses
Creating your own metaclass is like painting a picture. You set the canvas and study the overall vision of what you want your classes to be. It typically involves inheriting from the built-in . The structure looks like this:
In this example, allows you to intercept class creation. You can modify, add, or even remove attributes. Why would someone want to do this? It could be for enforcing naming conventions, registering classes automatically, or injecting certain functionality across several classes.
For example, if you want to log every class creation, you might put a logging statement inside your method. This insight gained during class creation can come in handy for debugging or enhancing application behavior.
Metaclass Use Cases
Metaclasses might seem niche, but they have practical applications in various scenarios. Here are a few notable use cases:
- Enforcing Constraints
Imagine you have a set of rules that all subclasses need to follow. A metaclass can enforce these rules at the time of class creation, ensuring that any child classes comply. - Class Registration
If you need to keep track of all classes derived from a common base class, a metaclass can register them automatically, eliminating manual tracking. - Customizing Method Behavior
You can utilize metaclasses to modify or override methods when a class is instantiated. This is handy when you want to implement class-level functionality without affecting each method individually.
Functional Programming in Python
Functional programming is a distinctive paradigm that enables programmers to approach coding with a different mindset, especially within the Python ecosystem. This section dives into the importance of functional programming in Python. While Python is often seen as an object-oriented programming language, it possesses powerful functional programming capabilities that can improve the clarity and efficiency of code.
One major benefit of using functional programming is its emphasis on using pure functions. These functions take inputs and produce outputs without causing side effects, making them predictable and easier to test. By reducing state-related complexities, code becomes more maintainable, and bugs are less likely to sneak in, allowing developers to keep their sanity intact.
Moreover, functional programming enables modularity in the code. Functions can stand alone and can be composed together in countless ways. This flexibility not only fosters code reusability but also promotes clean and organized coding practices. Consequently, projects become simpler to manage as they grow larger.
Another reason to embrace functional programming in Python is its compatibility with other programming paradigms, allowing developers to synergize the best of both worlds. Whether building a web application or automating repetitive tasks, integrating functional programming can lead to elegant and succinct solutions.
Key Principles of Functional Programming
At the core of functional programming are key principles that are essential to mastering this approach in Python. These principles shape the way developers think about data and functions:
- First-Class Functions: In Python, functions are first-class citizens. This means they can be passed as arguments, returned from other functions, and assigned to variables. Such flexibility is foundational in functional programming, allowing for higher-order functions to shine.
- Pure Functions: A pure function, as mentioned earlier, consistently produces the same output given the same input and has no side effects. Using pure functions greatly enhances predictability throughout your codebase.
- Function Composition: This is the process of combining simple functions to build more complex ones. Itâs a powerful technique that can simplify your code when executed correctly.
Understanding these principles is crucial for tapping into the power of functional programming.
Using Higher-Order Functions
Higher-order functions are those that either take other functions as parameters or return them as results. This feature of Python is vital for functional programming, as it allows for more abstract and flexible code.
For example, consider the concept of mapping a function over a list. The function is a built-in higher-order function that applies a given function to every item in an iterable:
In this case, a lambda function is defined right inside the , squaring each number in the range from 0 to 9. The ability to pass around functions as arguments and return them enhances the expressiveness of Python programs, leading to concise and readable code.
Immutable Data Structures
In functional programming, the concept of immutability is pivotal. Immutable data structures are those whose state cannot be modified after they are created. This characteristic is often observed in tuples and frozensets in Python.
Utilizing immutable data structures can lead to safer code. Since these structures do not change, they help avoid unexpected side effects that can complicate state management. Additionally, immutability can improve performance in multi-threaded environments, as it enables better concurrency without the need for complex locking mechanisms.
"Immutability reminds us that some data must remain constantâlike the truths we hold in our work."
Concurrency in Python: Threads and Asyncio
When it comes to programming in Python, understanding concurrency is key, especially in today's world where applications need to handle many tasks at the same time. Concurrency allows a program to deal with multiple operations simultaneously, which can greatly enhance performance and user experience. In this section, we will dive into threads and asyncio, exploring their significance and the unique benefits they bring to Python programming.
Understanding Threads and the Global Interpreter Lock
Threads are essentially lightweight processes that allow your program to perform multiple operations concurrently. However, Python has a unique feature, known as the Global Interpreter Lock (GIL), which ensures that only one thread can execute Python bytecode at a time. This means that even though we can create multiple threads in Python, they can't all run at the same time when executing Python code.
The existence of the GIL often raises eyebrows, especially among developers coming from other programming languages. They wonder how effective threading can truly be in Python. Despite the GIL, threads remain useful for I/O-bound tasks, such as waiting for file or network operations, where threads can operate while others are blocked. Here's a simple analogy: it's like having several cashiers (threads) in a grocery store (the program) handling customers, but only one cashier can serve customers at any moment when it comes to cash register tasks.
Utilizing Asyncio for Asynchronous Programming
Asyncio is a modern way of writing concurrent code in Python, enabling an entirely different approach compared to traditional threading. With asyncio, you define asynchronous functions using the syntax, allowing you to pause execution in an efficient manner without blocking the entire program.
Think of asyncio as a highly efficient air traffic controller, directing planes to land smoothly without affecting each other's schedules. When an statement is encountered in an asyncio function, it pauses and yields control back to the event loop, letting other tasks run concurrently. This model is particularly beneficial for high-level I/O operations, such as web scraping or network requests, improving performance without the complications inherent to managing threads.
For quick reference, hereâs how you might define a simple asynchronous function:
Comparison of Concurrency Models
In Python, when it comes to concurrency, you primarily have three models: threads, asyncio, and multiprocessing. Each has its own strengths and is suited to different types of tasks:
- Threads: Good for I/O-bound tasks where waiting for data to process is involved. Useful when you want to manage multiple tasks that are not CPU-intensive.
- Asyncio: Excellent for writing scalable I/O-bound applications. Ideal for situations where tasks spend a lot of time waiting (e.g., network communications) because it uses an event loop mechanism to handle requests.
- Multiprocessing: Best for CPU-bound tasks. It allows you to spread your tasks across multiple CPU cores, making full use of the system resources.
Ultimately, the choice of concurrency model largely depends on the nature of the tasks at hand. With proper understanding, you can exploit the strengths of each model to develop efficient Python applications.
"Concurrency is not about how many things you can do at once, but about how many things can be done effectively in parallel."
With this knowledge, developers can better navigate the complexities of modern Python programming, leveraging the right tools to optimize their code and enhance performance.
Error Handling: Exceptions and Assertions
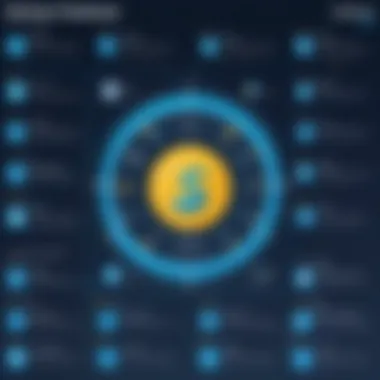
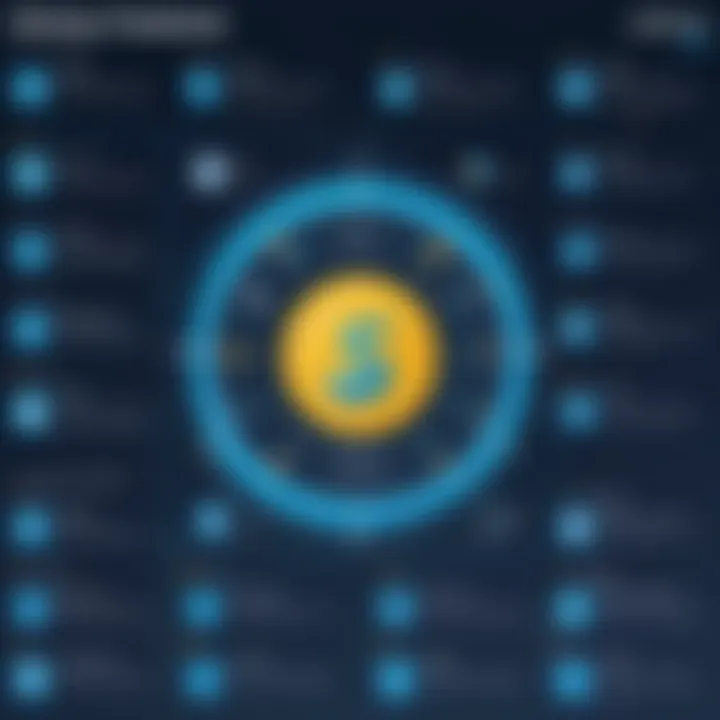
Error handling plays a critical role in creating robust, maintainable, and effective software. In Python, managing errors is not just about fixing bugs but also about ensuring that your code can gracefully handle unexpected situations. When things go sidewaysâwhether due to user input, external data, or even simple typos in the codeâhaving a solid error handling strategy can save the day.
The Exception Hierarchy
In Python, exceptions are organized into a hierarchy, where each type of exception corresponds to different error scenarios. At the top of this hierarchy is the class, followed closely by the class, from which almost all built-in exceptions arise. Here's a quick peek at how this hierarchy breaks down:
- BaseException
- KeyboardInterrupt
- SystemExit
- Exception
- StandardError
- ValueError
- TypeError
- KeyError
Understanding this hierarchy not only helps in selecting the right exception to raise or catch but allows developers to pinpoint issues effectively. For instance, when you want to handle invalid input, catching is a direct approach without clashing with more serious exceptions like , which indicates a user-initiated program exit.
Custom Exception Classes
While Python provides plenty of built-in exceptions to deal with unforeseen errors, sometimes, you find the need to create custom exceptions. This often comes into play in larger applications where specific error handling is required. Creating a custom exception helps clarify the problem context even further. Here's a simple way to define a custom exception:
Using this class, you can raise it like so:
By defining your own exceptions, you clarify the nature of the issues your application encounters, making debugging and maintenance a smoother journey.
Using Assertions for Debugging
Assertions are simple, yet powerful, tools for maintaining code correctness. They act as internal checkpoints, validating whether certain conditions hold true during execution. If an assertion fails, Python raises an . This is how you'd typically use assertions:
If the condition fails, the assertion raises an error, disrupting the program flow and signaling a critical issue right at that moment. It's worth noting that assertions should be used primarily for debugging purposes; they should not be relied upon for handling user input or expected error states.
Remember: Assertions are meant to catch programmer errors and not user errors. Use them wisely to catch bugs in your logic rather than misbehaviors from your users' actions.
In summary, mastering error handling using exceptions and assertions equips you with the tools to build more resilient applications. Youâll handle errors elegantly, communicate failures clearly, and maintain control over your programâs behavior even in the most unpredictable situations.
Working with Python Libraries: An Overview
In the vast landscape of programming, libraries serve as powerful allies that can streamline processes and enhance productivity. In Python, libraries are collections of pre-written code that allow developers to perform common tasks without having to rewrite everything from scratch. Understanding how to effectively utilize these libraries is vital for anyone stepping beyond the initial stages of programming.
Libraries often encapsulate complex functionalities behind simple function calls. This means that by leveraging existing libraries, programmers can save time, reduce the likelihood of bugs, and focus their energy on solving unique problems that mean more to their projects.
Benefits of Using Python Libraries
- Time Efficiency: Libraries allow you to implement solutions quickly. Instead of writing a function that reads data from a file, you can simply call the appropriate function from a library like Pandas.
- Community Support: Popular libraries often have a massive community backing them. This means a wealth of tutorials, documentation, and forum discussions are available, aiding both novice and experienced programmers.
- Standardization: Libraries tend to follow standard practices and conventions. Utilizing these libraries can help enforce best practices and improve code readability.
However, itâs worth noting that while libraries offer significant advantages, they also have specific considerations. Dependencies may result in compatibility issues, and over-reliance on libraries can lead to a lack of understanding of the underlying code.
"In the age of information, not having the right tools can keep you in the slow lane."
Popular Libraries for Data Analysis
When it comes to data analysis, Python is often praised for its powerful libraries that facilitate data manipulation, analysis, and visualization. Below are some of the most commonly used libraries in the field:
- Pandas: Renowned for its data manipulation capabilities, Pandas provides data structures like Series and DataFrames, making it easy to handle tabular data. It simplifies tasks such as data filtering, aggregation, and time series analysis.
- NumPy: This library is the backbone of numerical computing in Python. It introduces array objects, which can perform mathematical operations efficiently. Understanding NumPy is often a stepping stone to leveraging other libraries such as SciPy and Pandas.
- Matplotlib: For data visualization, Matplotlib is a go-to. It helps create static, dynamic, and interactive visualizations in Python. Getting familiar with Matplotlib is key for anyone who wants to present their findings visually.
Libraries for Building Web Applications
Web development has also thrived in the Python environment. A few critical libraries and frameworks simplify the web development process:
- Flask: This lightweight web framework is ideal for beginners. Flask gives you the flexibility to structure your apps as you see fit and is perfect for smaller projects.
- Django: Known for its robustness, Django follows the "batteries included" philosophy, which means it provides a wide range of features out of the box. Itâs designed to help you build secure and maintainable web applications quickly.
- FastAPI: This modern framework is gaining traction for its speed and efficiency, especially when creating APIs. It uses Python type hints, making code more readable and easier to debug.
In summary, working with Python libraries is a necessary skill for advancing programming capabilities. From data analysis to building dynamic web applications, the libraries available empower developers to create efficient, maintainable, and robust programs.
Best Practices in Advanced Python Programming
Navigating the world of advanced Python programming requires more than just mastering intricate concepts and techniques. It calls for a commitment to best practices that not only elevate the quality of your code but also streamline your development process. Adhering to these best practices enhances collaborative efforts, resulting in cleaner, more maintainable, and efficient code.
Code Readability and Style Guides
Code is not only written for machines to execute; it's also read by humans. Clear, readable code makes it easier to maintain and understand, especially when working in a team. Python's community embraces a set of conventions and principles encapsulated in Style Guides, primarily outlined in PEP 8. PEP 8 serves as a comprehensive guide covering various elements, such as naming conventions, indentation, and line length.
Tips for Writing Readable Code:
- Consistent Naming: Use meaningful variable and function names. For instance, prefer over merely . This gives insight into what the function does at first glance.
- Comment Strategically: While your code should be self-explanatory, provide comments where necessaryâspecifically for complex algorithms or functions. However, avoid over-commenting; too many comments can clutter the code.
- Maintain Proper Indentation: Python relies on indentation to define code blocks. Stick to an indentation of four spaces to keep things tidy.
By following these conventions, not only do you foster a cleaner coding environment, but you also encourage others to contribute without hesitation.
Testing and Debugging Strategies
Testing and debugging are integral to producing high-quality code. They ensure reliability and facilitate the identification of bugs before they morph into larger issues in production. Itâs crucial to integrate effective testing strategies into your workflow.
Common Testing Techniques:
- Unit Testing: This focuses on individual components, ensuring each part behaves as expected. Pythonâs module is a great resource for this.
- Integration Testing: Once unit tests are successful, check how different modules work together.
- Continuous Integration: Utilize tools like Travis CI or CircleCI to automate the integration process. This helps catch errors early when new code is integrated into existing projects.
Key Debugging Tips:
- Use Print Statements: While traditional, these can effectively pinpoint where the logic might break.
- Leverage Debugger Tools: Pythonâs built-in module allows you to step through code interactively and inspect variables dynamically.
- Review Error Messages Carefully: Python's error messages are often clear and can guide you toward understanding what went wrong.
Implementing robust testing and debugging methods not only improves your code's reliability but also boosts your confidence as a developer.
In summary, embracing these best practices in advanced Python programming lays a strong foundation for quality software development. It equips you to adapt and grow in an ever-evolving landscape, allowing your projects to flourish in both collaborative and independent settings.