Unlocking Advanced JavaScript Techniques for Elevated Programming Skills
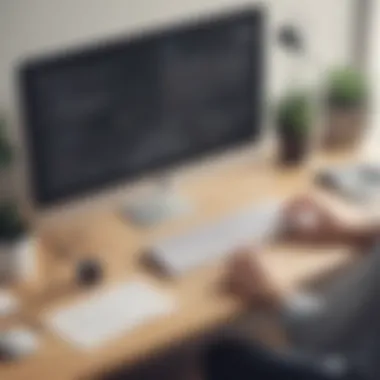
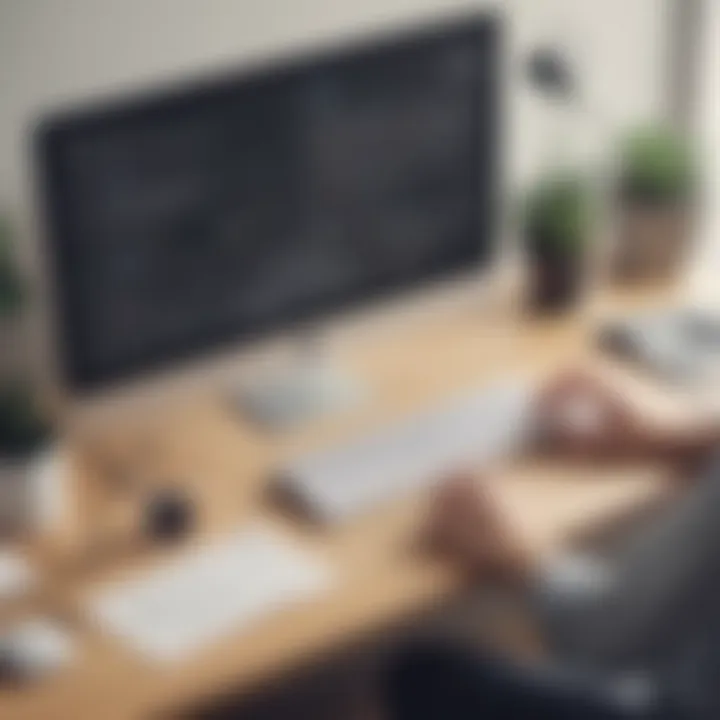
Introduction to JavaScript
When delving into the world of programming languages, one cannot overlook the significance of JavaScript. Originally developed in the mid-1990s, JavaScript has evolved to become one of the most popular and versatile languages in the realm of web development. Its dynamic nature and wide-ranging applications make it a fundamental tool for modern developers to master.
History and Background
JavaScript was created by Brendan Eich in 1995 while he was working at Netscape Communications. Initially named Mocha, it was later renamed to LiveScript before settling on JavaScript. Despite some early skepticism, JavaScript gained traction rapidly due to its ability to add interactivity to web pages.
Features and Uses
Javascript is renowned for its versatility, serving as the backbone for dynamic content, interactive elements, and client-side operations on websites. Its scripting capabilities enable developers to create responsive and engaging web applications, making it an essential part of modern web development.
Popularity and Scope
In the contemporary digital landscape, the demand for skilled JavaScript developers is ever-increasing. From powering front-end frameworks like React and Angular to server-side environments with Node.js, JavaScript's versatility continues to drive its popularity. Mastering advanced JavaScript concepts opens up a myriad of career opportunities for aspiring developers.
Basic Concepts of JavaScript
To establish a strong foundation in JavaScript, one must grasp its basic syntax and core concepts. These fundamental aspects lay the groundwork for understanding the more intricate elements of the language.
Variables and Data Types
Variables in JavaScript serve as containers for storing data values. They can hold various types of data, such as numbers, strings, arrays, and objects. Understanding data types and their manipulation is essential for effective programming in JavaScript.
Operators and Expressions
Operators enable developers to perform operations on variables and values, facilitating tasks like arithmetic calculations, logical comparisons, and assignment operations. Employing operators effectively allows for efficient data manipulation and program execution.
Control Structures
Control structures, including conditional statements and loops, dictate the flow of a JavaScript program. By utilizing control structures, developers can implement decision-making processes and iterative actions, enhancing the functionality and flexibility of their code.
Advanced JavaScript Topics
Transitioning to advanced JavaScript topics delves into more complex concepts that elevate programming proficiency and empower developers to create sophisticated applications.
Functions and Methods
Functions in JavaScript encapsulate reusable blocks of code that perform specific tasks. Understanding functions and methods enables developers to modularize code, promote code reusability, and enhance the efficiency of their applications.
Object-Oriented Programming
Object-oriented programming (OOP) is a paradigm that structures code around objects, allowing for the creation of reusable and scalable codebases. In JavaScript, OOP concepts like classes, inheritance, and encapsulation facilitate the development of robust and extensible applications.
Exception Handling
Exception handling in JavaScript enables developers to manage and respond to unexpected errors or exceptional situations during program execution. Implementing robust error-handling mechanisms enhances the reliability and resilience of JavaScript applications.
Practical Application with Examples
Mastering advanced JavaScript concepts is best achieved through hands-on practice with practical examples and projects. Applying theoretical knowledge to real-world scenarios promotes skill development and hones problem-solving abilities.
Simple Programs
Beginners can engage with simple JavaScript programs to practice basic syntax, data manipulation, and algorithmic logic. These programs lay the groundwork for more complex projects and offer a hands-on approach to learning JavaScript.
Intermediate Projects
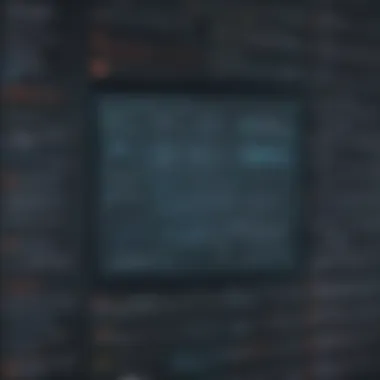
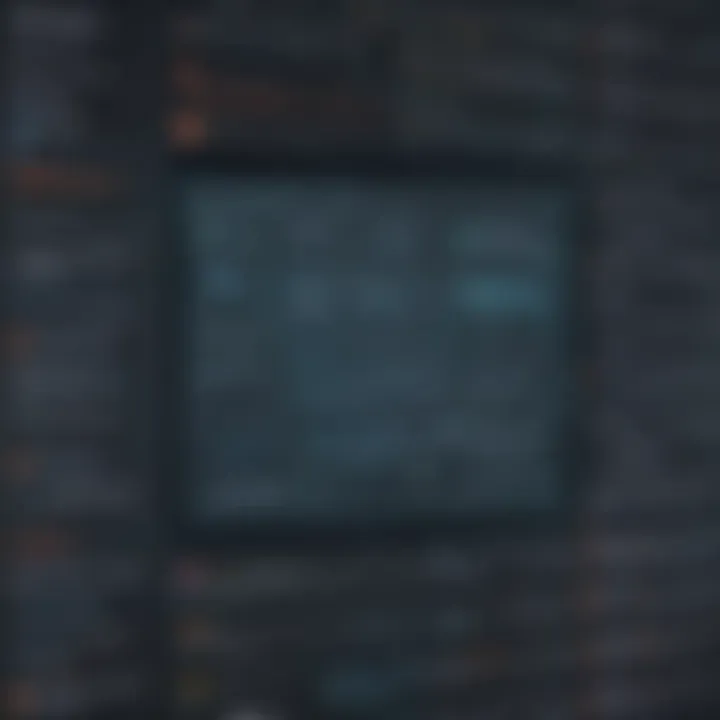
Intermediate projects challenge developers to apply advanced JavaScript concepts in the development of interactive web applications, games, or utility tools. These projects foster creativity, problem-solving skills, and a deeper understanding of JavaScript's applications.
Code Snippets
Exploring code snippets and snippets of pre-written code offers insight into programming best practices, time-saving techniques, and innovative solutions. Analyzing and modifying code snippets enhances coding proficiency and encourages knowledge sharing within the developer community.
Resources for Further Learning
Continuous learning and exploration are crucial in the ever-evolving landscape of JavaScript development. Leveraging resources and tools for further education equips developers with the knowledge and skills needed to stay competitive.
Recommended Books and Tutorials
Engaging with comprehensive books and tutorials on JavaScript expands understanding and proficiency in the language. These resources cover a wide array of topics, from fundamental concepts to advanced techniques, catering to learners of all levels.
Online Courses and Platforms
Online courses and learning platforms offer interactive and structured environments for honing JavaScript skills. Hands-on exercises, video tutorials, and community support enrich the learning experience, enabling developers to enhance their expertise effectively.
Community Forums and Groups
Engaging with JavaScript communities and forums fosters collaboration, networking, and knowledge sharing among developers. Participating in discussions, seeking advice, and sharing insights contribute to a vibrant and supportive learning ecosystem, propelling professional growth and skill development in JavaScript.
Introduction to Advanced JavaScript
In this section, we delve into the significance of advancing one's proficiency in JavaScript, particularly focusing on mastering asynchronous operations, ES6 features, and functional programming. Understanding these concepts is crucial for developers looking to enhance their coding skills and efficiency. Advanced JavaScript techniques allow for more elegant and efficient solutions to complex problems, ultimately leading to more robust and scalable applications.
Understanding Asynchronous JavaScript
Callbacks
Callbacks play a pivotal role in asynchronous programming, enabling functions to execute out of a specific order. They are essential for handling tasks that require waiting for an operation to complete before moving on to the next step. Despite their widespread use, callbacks can lead to callback hell and make code harder to read and maintain. However, with proper structuring, callbacks can still be effective for managing asynchronous tasks.
Promises
Promises offer a cleaner alternative to callbacks by providing better control over asynchronous operations. They simplify error handling and sequenced execution of functions. Promises are highly versatile and easier to chain together, enhancing code readability and maintainability. Embracing promises can improve code organization and make asynchronous operations more manageable and intuitive.
Asynchronous Functions
Asynchronous functions, including asyncawait in ES6, streamline asynchronous coding by allowing developers to write synchronous-style code that executes asynchronously. This syntactic sugar simplifies error handling and makes asynchronous code resemble synchronous code, enhancing its readability and maintainability. Asynchronous functions improve code clarity and reduce the chances of bugs caused by asynchronous operations, making them an invaluable asset in modern JavaScript development.
Mastering ES6 Features
Arrow Functions
Arrow functions offer a concise syntax for writing functions, avoiding the verbosity of traditional function declarations. They provide lexical scoping, eliminating the need for the 'this' keyword when accessing parent scopes. While arrow functions enhance code readability and are favored for their simplicity, they may not be suitable for all situations due to their differences in handling the 'this' context compared to regular functions.
Destructuring
Destructuring simplifies the extraction of data from arrays and objects, allowing developers to unpack values into distinct variables. This ES6 feature enhances code clarity, making assignments more concise and readable. Destructuring also facilitates working with complex data structures by enabling easy access to individual elements. However, excessive nesting or destructuring of deeply nested objects can reduce code maintainability and increase complexity.
Modules
ES6 modules provide a robust system for organizing code into reusable and independent components. They enhance code modularity, allowing better structure and encapsulation of functionality. Modules facilitate code sharing and collaboration among developers, promoting code maintainability and scalability. By reducing code redundancy and dependency, modules improve the overall quality and efficiency of JavaScript applications.
Functional Programming in JavaScript
Higher-Order Functions
Higher-order functions accept functions as arguments or return them, enabling developers to create more flexible and dynamic code. They promote code reusability and abstraction, leading to cleaner and more concise solutions. By treating functions as first-class citizens, higher-order functions empower developers to employ functional programming paradigms effectively, enhancing the robustness and extensibility of their code.
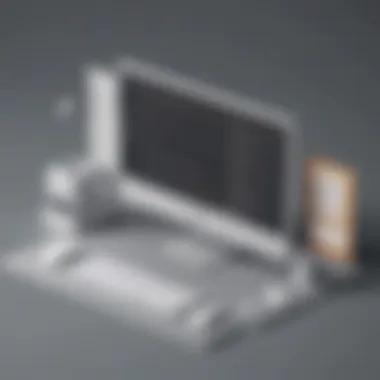
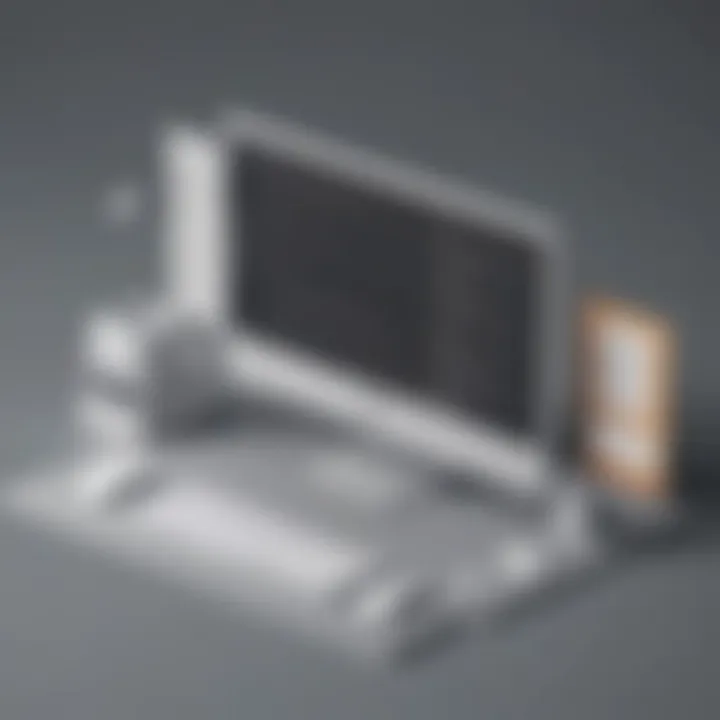
Pure Functions
Pure functions have no side effects and always return the same output for a given input, promoting predictability and testability of code. By isolating logic and dependencies, pure functions increase code reliability and maintainability. Their deterministic nature simplifies debugging and reasoning about code behavior, making them essential for functional programming and immutable state management in JavaScript development.
Immutability
Embracing immutability in JavaScript involves creating data structures that cannot be changed once defined. Immutable data ensures data consistency and avoids unintended mutations, contributing to predictable and safer code. Immutability simplifies state management and concurrency control, significantly reducing the risk of bugs caused by shared state modification. While immutability offers many benefits for developing scalable and error-resilient applications, transitioning to an immutable mindset may require adjustments to familiar coding practices and paradigms.
Advanced JavaScript Patterns
In this section, the focus is on elucidating the significance of Advanced JavaScript Patterns within the broader framework of the article. Advanced JavaScript Patterns play a pivotal role in enhancing code organization, scalability, and maintainability. By employing well-established design patterns, programmers can streamline their development process, improve code reusability, and facilitate collaboration among team members. Understanding and implementing these patterns are crucial for aspiring developers looking to fortify their JavaScript skills. The discussion will delve into specific elements such as the Singleton Pattern, Factory Pattern, and Observer Pattern, shedding light on their benefits and considerations.
Design Patterns
Singleton Pattern
The Singleton Pattern, a fundamental creational pattern, is pivotal in ensuring that a class has only one instance and provides a global point of access to it. This pattern's key characteristic lies in restricting instantiation of a class to a single object, making it a popular choice for scenarios where a sole, shared instance is required across the application. The unique feature of the Singleton Pattern promotes global access to a singleton instance, which can be advantageous for managing resources efficiently. However, drawbacks may include potential inflexibility concerning unit testing and the tight coupling it introduces.
Factory Pattern
The Factory Pattern, another creational pattern, centralizes object creation by delegating the responsibility to a factory class or method. Its key characteristic involves defining an interface for creating objects while allowing subclasses to alter the type of objects that will be created. This flexibility makes the Factory Pattern a beneficial choice for scenarios where object creation logic is subject to change. The unique feature of dynamic object creation sets the Factory Pattern apart, with advantages including encapsulating object creation and promoting code scalability. Nonetheless, disadvantages may arise from increased complexity in the codebase and potential difficulty in debugging.
Observer Pattern
The Observer Pattern, a behavioral design pattern, facilitates a one-to-many dependency between objects, ensuring that when one object changes state, all its dependents are notified and updated automatically. Its key characteristic lies in establishing a loosely coupled communication mechanism between subjects and observers, making it a favorable choice for event-handling scenarios. The unique feature of event propagation and subscription-based management enhances code modularity and extensibility. Advantages of the Observer Pattern include decoupling of concerns and promoting reusability. However, drawbacks may include potential performance overhead and managing multiple subscriptions effectively.
Optimizing Performance
In the pursuit of optimized performance in JavaScript, developers often leverage techniques such as Memoization, Optimizing Loops, and Lazy Loading. These strategies are instrumental in enhancing code efficiency, reducing computational overhead, and improving user experience through faster response times.
Memoization
Memoization is a powerful optimization technique that involves caching the results of expensive function calls and retrieving them when the same inputs reoccur. By storing computed values and returning the cached result for subsequent calls with the same inputs, Memoization significantly boosts performance in scenarios involving repeated computations. The key characteristic of Memoization lies in memory-efficient memoizing of function outputs, making it a popular choice for speeding up computations. The unique feature of reducing redundant calculations and enhancing algorithm efficiency provides advantages in optimizing performance. However, drawbacks may include potential memory consumption concerns and managing cache invalidation.
Optimizing Loops
Optimizing Loops focuses on refining loop structures to minimize unnecessary iterations and improve code execution speed. By optimizing loops through techniques like loop unrolling, loop hoisting, and loop fusion, developers can enhance computational efficiency and reduce operation overhead. The key characteristic of Optimizing Loops involves streamlining loop operations to expedite data processing, presenting a beneficial choice for performance-critical applications. The unique feature of loop optimization techniques enables developers to fine-tune code performance for optimal results. Advantages of Optimizing Loops include significant speed enhancements and resource optimization. Nevertheless, drawbacks may encompass increased code complexity and potential issues in code readability.
Lazy Loading
Lazy Loading is a mechanism that defers the loading of non-essential resources until they are required, thereby conserving bandwidth and accelerating initial page load times. By loading content asynchronously or on-demand, Lazy Loading enhances user experience by prioritizing critical content and deferring secondary resources. The key characteristic of Lazy Loading revolves around deferred resource loading to minimize upfront data transfer, making it a favorable choice for optimizing website performance. The unique feature of dynamic resource loading as needed enables efficient resource utilization and refined page loading times. Advantages of Lazy Loading include improved site speed and bandwidth efficiency. However, drawbacks may include potential content accessibility concerns and intricacies in managing dynamic loading sequences.
Advanced DOM Manipulation
In this section of the article, we will delve into the significance of Advanced DOM Manipulation within the context of mastering JavaScript. DOM Manipulation plays a crucial role in web development, allowing programmers to dynamically interact with the content and structure of web pages. It involves manipulating the HTML and CSS elements of a webpage in real-time, enabling dynamic updates and user interactions. Advanced DOM Manipulation is essential for creating responsive and interactive web applications, enhancing the overall user experience. By understanding and implementing advanced DOM manipulation techniques, developers can create dynamic, data-driven websites that respond dynamically to user input.
Event Handling
Event Delegation
Event Delegation is a key concept in JavaScript event handling, focusing on optimizing event listeners to enhance performance and scalability. Instead of assigning individual event listeners to multiple elements, event delegation involves assigning a single event listener to a common ancestor element. This approach allows for more efficient event management, particularly in situations where there are numerous dynamically generated elements. Event Delegation simplifies event handling code, reduces memory consumption, and improves the responsiveness of web applications. While Event Delegation offers advantages in terms of efficiency and scalability, it requires careful consideration of event propagation to ensure the correct handling of events.
Custom Events
Custom Events provide a way to define and dispatch custom events in JavaScript, enabling developers to create their own event types for specific application requirements. By defining custom events, developers can establish custom communication channels between different parts of the application, facilitating modular design and encapsulation. Custom Events enhance code organization and maintainability by providing a structured approach to event-driven programming. However, overusing custom events can lead to code complexity and reduced code readability, so their usage should be judicious.
Event Bubbling
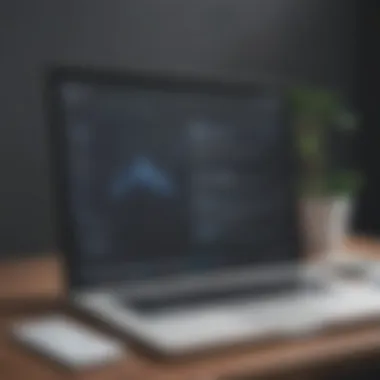
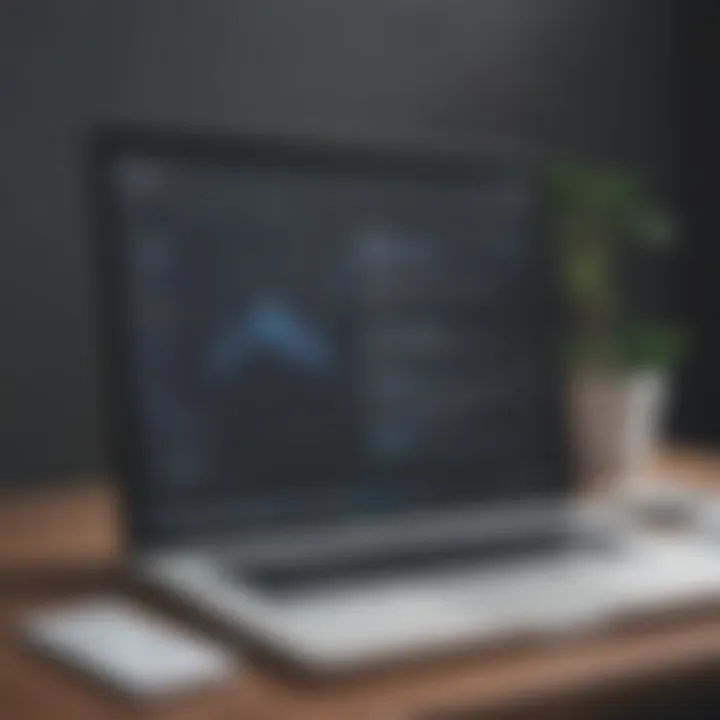
Event Bubbling is a fundamental concept in JavaScript event propagation, where events fired on an inner element will cascade up through its ancestors in the DOM tree. This bubbling effect allows multiple elements to handle the same type of event, propagating it upwards until a common ancestor processes the event. Event Bubbling simplifies event handling by reducing the need to assign individual event listeners to each element, promoting code reusability and efficiency. While Event Bubbling streamlines event handling, developers need to understand the event flow to prevent unintended event propagation or handling conflicts.
Modern JavaScript Tools and Libraries
Modern JavaScript tools and libraries play a pivotal role in the realm of web development, enabling programmers to streamline their workflows and enhance the quality of code they produce. In this section of the article, we will delve into the significance of modern JavaScript tools and libraries, focusing on their specific elements, benefits, and considerations within the context of advanced JavaScript programming.
Webpack and Babel
Webpack and Babel are indispensable tools in the modern JavaScript ecosystem, providing developers with robust features to optimize their code. ### Module Bundling Module Bundling is a crucial aspect of modern JavaScript development, as it allows developers to package multiple modules into a single file efficiently. This process aids in reducing load times and enhancing performance, making it a popular choice among developers aiming for efficiency in this article. Module Bundling's key characteristic lies in its ability to manage dependencies effectively, optimizing the application's size and improving loading speeds. However, one drawback could be the complexity that arises when bundling large-scale projects.
Transpiling ES6+ Code
Transpiling ES6+ code is essential for ensuring cross-browser compatibility and leveraging the latest language features. The key characteristic of transpiling ES6+ code is its ability to convert modern JavaScript syntax into a compatible format for older browsers or environments. This aspect is highly beneficial for developers seeking to utilize modern language features without compromising compatibility. One unique feature of transpiling is its role in enabling developers to write code without worrying about browser support, enhancing the development process. However, a disadvantage may arise from the added step of transpilation, potentially impacting build times.
Optimizations
Optimizations in modern JavaScript tools are geared towards enhancing performance and efficiency in code execution. The key characteristic of optimizations lies in their ability to identify and implement improvements in code performance, reducing redundancy and enhancing speed. This aspect is particularly advantageous for developers looking to optimize their applications for speed and scalability. The unique feature of optimizations is their potential to significantly boost application performance, making them a valuable addition to the development process. However, the disadvantage of optimizations could be the increased complexity in code implementation and maintenance.
Node Package Manager (npm)
Node Package Manager (npm) plays a vital role in managing dependencies and supporting the development process in JavaScript projects.### Dependency Management Dependency management is a critical aspect of npm, allowing developers to easily install, update, and remove project dependencies. The key characteristic of dependency management is its ability to streamline the dependency resolution process, ensuring that the required packages are seamlessly integrated into the project. This feature is highly beneficial for developers seeking efficient package management within their projects. A unique feature of dependency management is its support for version control and dependency tracking, facilitating project stability and consistency. However, a disadvantage may arise from potential conflicts between different package versions, requiring careful management.
Script Execution
Script Execution in npm enables developers to define custom scripts for various tasks, automating repetitive processes within the project. The key characteristic of script execution is its flexibility in allowing developers to create custom commands for tasks like testing, building, and deployment. This feature is beneficial for enhancing productivity and streamlining the development workflow. A unique feature of script execution is its integration with lifecycle events, enabling scripts to run at specific project stages. However, the disadvantage could stem from the need for proper script documentation and management to avoid clutter and maintain readability.
Package Publishing
Package Publishing in npm empowers developers to share their packages with the broader community, contributing to the open-source ecosystem. The key characteristic of package publishing is its simple process of publishing and versioning packages, making it accessible to developers of all levels. This feature is beneficial for those aiming to contribute to the developer community and establish a reputation in the industry. A unique feature of package publishing is its integration with the npm registry, making packages easily discoverable and accessible to a global audience. However, a disadvantage may arise in managing package versions and ensuring compatibility across different projects.
Testing and Debugging in JavaScript
Testing and debugging in JavaScript play a pivotal role in ensuring code quality and functionality. In the realm of programming, meticulous testing and effective debugging practices are crucial for identifying and rectifying errors in code efficiently. By incorporating a robust testing and debugging strategy, developers can streamline the development process, enhance code reliability, and expedite the resolution of issues.
Unit Testing with Jest
Unit testing with Jest is a fundamental aspect of testing in JavaScript. Writing test suites using Jest allows developers to systematically evaluate individual units or components of their code for correctness. Jest simplifies the unit testing process by providing a user-friendly framework with built-in functionalities for assertions and mocking. Its ease of use and comprehensive documentation make it a popular choice among developers for writing reliable and efficient unit tests.
Writing Test Suites
Writing test suites is a key part of the unit testing process. It involves creating a set of test cases to verify the behavior of functions, methods, or modules within the code. Test suites outline the expected outcomes for different scenarios, allowing developers to validate the functionality of their code systematically. By meticulously crafting test suites, developers can ensure code robustness and identify potential bugs early in the development cycle, contributing to overall code quality.
Mocking Functions
Mocking functions in Jest enables developers to simulate the behavior of dependencies or external functions within a controlled environment. This practice is beneficial for isolating specific components of the codebase during testing without invoking actual external resources. By mocking functions, developers can focus on testing individual units independently, leading to more efficient and targeted testing processes.
Asynchronous Testing
Asynchronous testing in Jest empowers developers to test functions that involve asynchronous behavior, such as API calls or timers. Jest provides mechanisms to handle asynchronous code, such as asyncawait syntax and the done callback, simplifying the testing of asynchronous functions. By leveraging Jest's asynchronous testing capabilities, developers can ensure the reliability and responsiveness of their async code, enhancing overall code quality and performance.
Debugging Techniques
Debugging techniques are essential for identifying and resolving errors in JavaScript code efficiently. By employing effective debugging practices, developers can pinpoint and rectify issues in their codebase, leading to enhanced code quality and expedited development cycles. Understanding various debugging techniques and tools is paramount for maintaining code integrity and optimizing performance.
Console Debugging
Console debugging is a foundational technique for diagnosing code issues in JavaScript. Developers utilize console.log statements to output specific values or messages at key points in the code execution flow, allowing them to track variables, function outputs, or errors. Console debugging provides real-time visibility into the code's behavior, aiding developers in understanding and addressing potential issues swiftly during development.
Using Breakpoints
Utilizing breakpoints in debugging facilitates the suspension of code execution at specific lines, enabling developers to inspect variables and verify program state at critical junctures. Breakpoints offer developers the ability to pause code execution dynamically, analyze variable values, and identify the root causes of bugs or unexpected behavior. By strategically placing breakpoints, developers can gain deeper insights into code logic and effectively troubleshoot complex issues.
Chrome DevTools
Chrome DevTools is a powerful set of web developer tools integrated into the Chrome browser, offering a plethora of features for debugging JavaScript, analyzing performance, and enhancing web development workflows. Developers can leverage Chrome DevTools to inspect elements, monitor network activity, profile code performance, debug JavaScript in real-time, and access a range of utilities for optimizing web applications. The comprehensive capabilities of Chrome DevTools make it an indispensable resource for JavaScript developers seeking to streamline debugging processes and optimize code efficiency.